Ongoing Call
Overview
The Ongoing Call
is a Component that provides users with a dedicated interface for managing real-time voice or video conversations. It includes features like a video display area for video calls, call controls for mic and camera management, participant information, call status indicators, and options for call recording and screen-sharing.
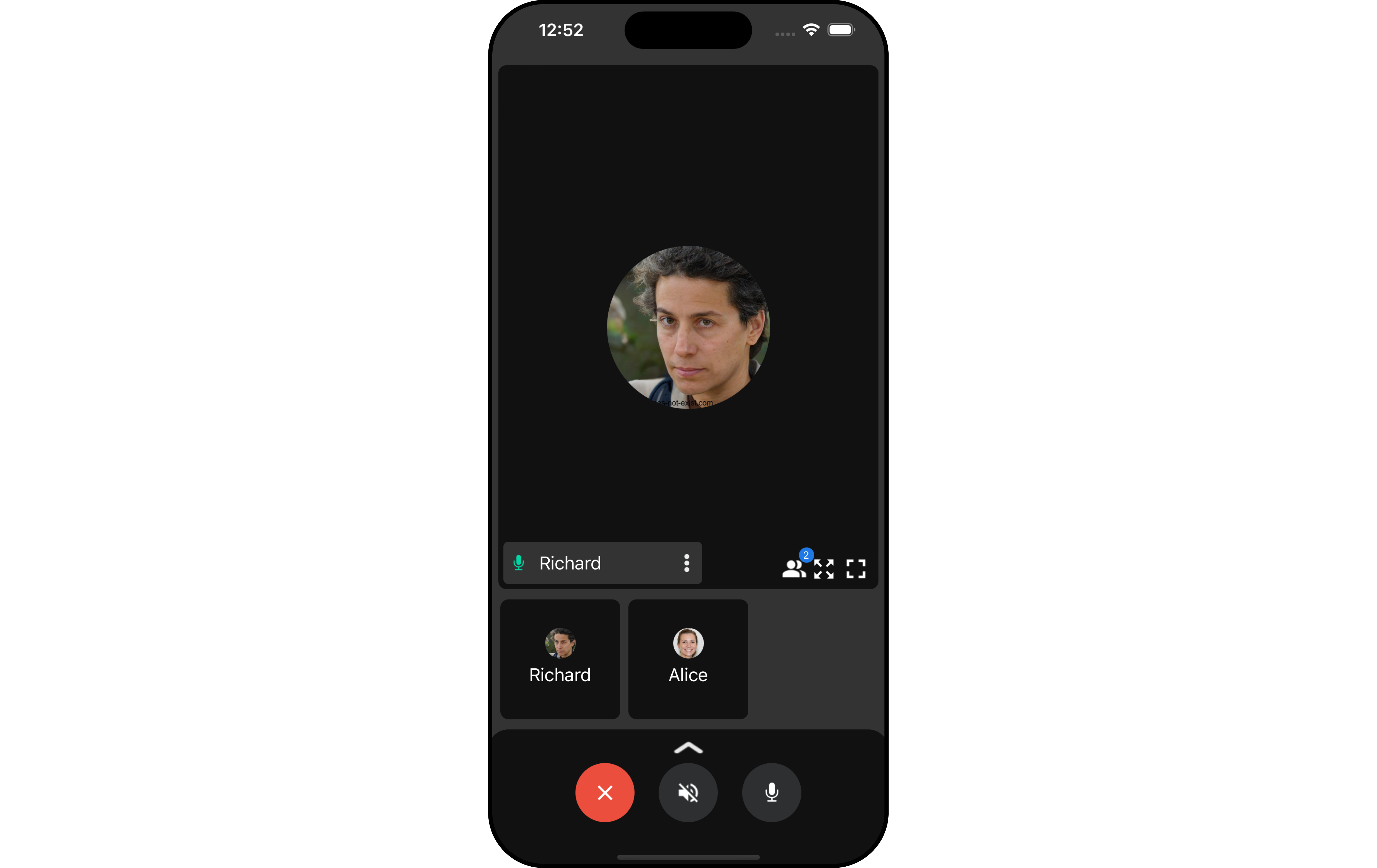
Usage
Integration
CometChatOngoingCall
being a custom view controller, offers versatility in its integration. It can be seamlessly launched via button clicks or any user-triggered action, enhancing the overall user experience and facilitating smoother interactions within the application.
- Swift
// The sessionID should be received from CometChat SDK when the call was initiated or received
let sessionID = "your_session_id"
let cometChatOngoingCall = CometChatOngoingCall()
cometChatOngoingCall.set(sessionID: sessionID)
cometChatOngoingCall.modalPresentationStyle = .fullScreen
self.present(cometChatOngoingCall, animated: true)
If you are already using a navigation controller, you can use the pushViewController
function instead of presenting the view controller.
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. SetOnCallEnded
The setOnCallEnded
action is typically triggered when the call is ended, carrying out default actions. However, with the following code snippet, you can effortlessly customize or override this default behavior to meet your specific needs.
- Swift
let cometChatOngoingCall = CometChatOngoingCall()
.setOnCallEnded { call in
//Perform Your Action
}
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
You can adjust the callSettingsBuilder
in the OnGoing Call
Component to customize the OnGoing Call. Numerous options are available to alter the builder to meet your specific needs. For additional details on CallSettingsBuilder
, please visit CallSettingsBuilder.
1. CallSettingsBuilder
The CallSettingsBuilder enables you to filter and customize the call list based on available parameters in CallSettingsBuilder. This feature allows you to create more specific and targeted queries during the call. The following are the parameters available in CallSettingsBuilder
Methods | Description | Code |
---|---|---|
setAudioModeButtonDisable | Disable the audio mode button | .setAudioModeButtonDisable(Bool) |
setAvatarMode | Set the avatar mode | .setAvatarMode("") |
setDefaultAudioMode | Set the default audio mode | .setDefaultAudioMode("") |
setDefaultLayout | Set the default layout | .setDefaultLayout(Bool) |
setDelegate | Set the calls events delegate | .setDelegate(CallsEventsDelegate) |
setEnableDraggableVideoTile | Enable draggable video tile | .setEnableDraggableVideoTile(Bool) |
setEnableVideoTileClick | Enable click actions on video tile | .setEnableVideoTileClick(Bool) |
setEndCallButtonDisable | Disable the end call button | .setEndCallButtonDisable(Bool) |
setIsAudioOnly | Set the call as audio only | .setIsAudioOnly(Bool) |
setIsSingleMode | Set the call as single mode | .setIsSingleMode(Bool) |
setMode | Set the mode | .setMode("NSString") |
setMuteAudioButtonDisable | Disable the mute audio button | .setMuteAudioButtonDisable(Bool) |
setPauseVideoButtonDisable | Disable the pause video button | .setPauseVideoButtonDisable(Bool) |
setShowRecordingButton | Show or hide the recording button | .setShowRecordingButton(Bool) |
setShowSwitchToVideoCall | Show or hide the switch to video call button | .setShowSwitchToVideoCall(Bool) |
setStartAudioMuted | Start with audio muted | .setStartAudioMuted(Bool) |
setStartRecordingOnCallStart | Start recording when the call starts | .setStartRecordingOnCallStart(Bool) |
setStartVideoMuted | Start with video muted | .setStartVideoMuted(Bool) |
setSwitchCameraButtonDisable | Disable the switch camera button | .setSwitchCameraButtonDisable(Bool) |
setVideoContainer | Set the video container | .setVideoContainer(NSMutableDictionary) |
setVideoSettings | Set the video settings | .setVideoSettings(NSMutableDictionary) |
Example
In the example below, we are applying a filter to the calls.
- Swift
let callBuilder = CallSettingsBuilder()
.setAudioModeButtonDisable(true)
.setEndCallButtonDisable(false)
let cometChatOngoingCall = CometChatOngoingCall()
.set(sessionId: "Your Session ID")
.set(callSettingsBuilder: callBuilder)
cometChatOngoingCall.modalPresentationStyle = .fullScreen
self.present(cometChatOngoingCall, animated: true)
Ensure to include an NSMicrophoneUsageDescription
key with a descriptive string value in the app's Info.plist
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
Events emitted by the Ongoing Call component is as follows.
Event | Description |
---|---|
onCallEnded | Triggers when the ongoing or outgoing call ends. |
- Add Listener
// View controller from your project where you want to listen events.
public class ViewController: UIViewController {
public override func viewDidLoad() {
super.viewDidLoad()
// Subscribing for the listener to listen events from user module
CometChatCallEvents.addListener("UNIQUE_ID", self as CometChatCallEventListener)
}
}
// Listener events from user module
extension ViewController: CometChatCallEventListener {
func onCallEnded(call: Call) {
// Do Stuff
}
}
//emit this when logged in user cancels a call
CometChatCallEvents.emitOnCallEnded(call: Call)
- Remove Listener
public override func viewWillDisappear(_ animated: Bool) {
// Uncubscribing for the listener to listen events from user module
CometChatCallEvents.removeListener("LISTENER_ID_USED_FOR_ADDING_THIS_LISTENER")
}
Customization
To fit your app's design requirements, you can customize the appearance of the conversation component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
The OngoingCall component does not have any exposed Styles.
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- Swift
let cometChatOngoingCall = CometChatOngoingCall()
.set(sessionId: "Your Session ID")
.set(callWorkFlow: .defaultCalling)
cometChatOngoingCall.modalPresentationStyle = .fullScreen
self.present(cometChatOngoingCall, animated: true)
If you are already using a navigation controller, you can use the pushViewController function instead of presenting the view controller.
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
CallWorkFlow | Sets the call type to default and direct. | CallWorkFlow |
Session Id | Sets the user object for CometChatOngoingCall. | .set(sessionId: String) |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
The OngoingCall
component does not provide additional functionalities beyond this level of customization.