Users
Overview
The Users is a Component, showcasing an accessible list of all available users. It provides an integral search functionality, allowing you to locate any specific user swiftly and easily. For each user listed, the widget displays the user's name by default, in conjunction with their avatar when available. Furthermore, it includes a status indicator, visually informing you whether a user is currently online or offline.
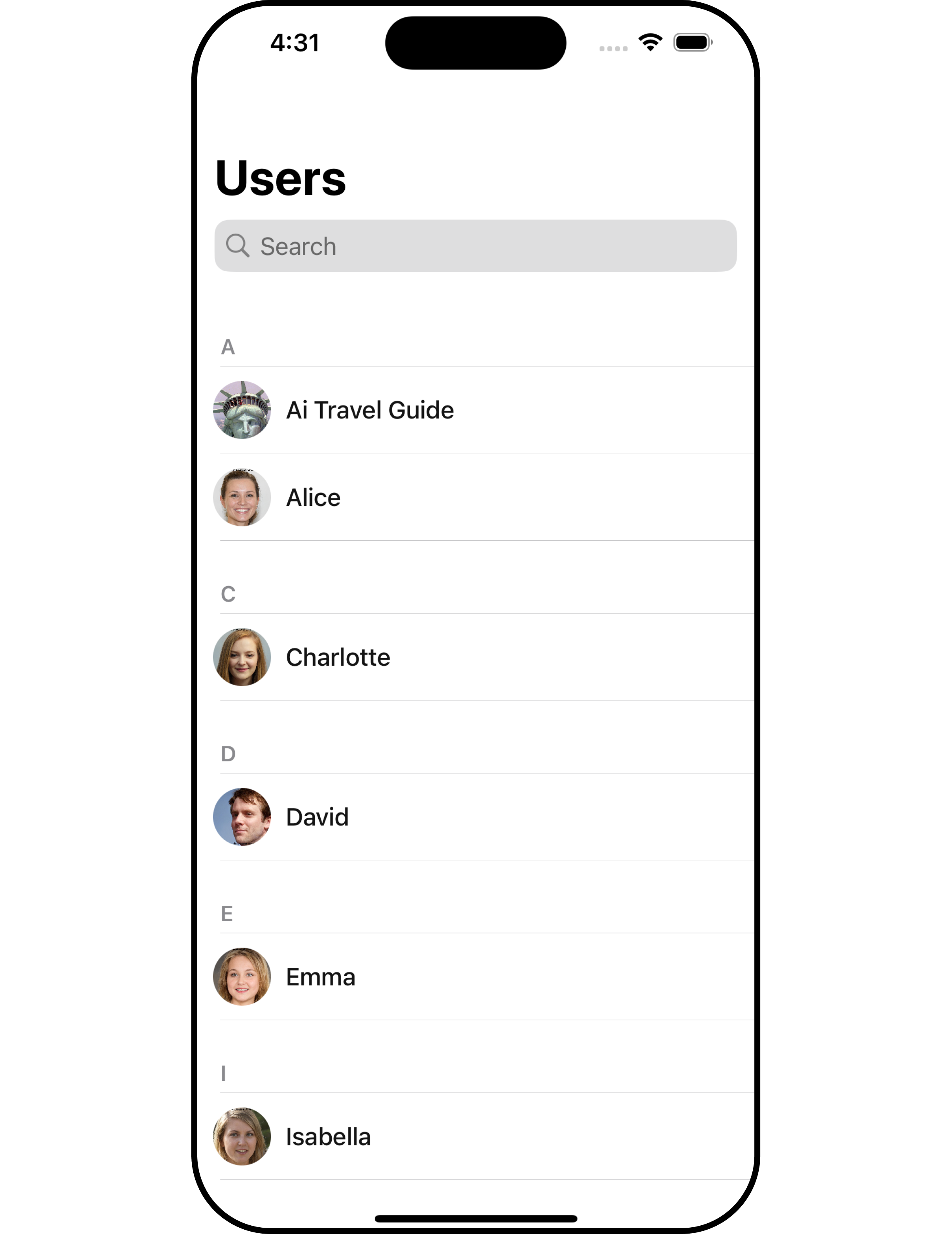
The Users component is composed of the following BaseComponents:
Components | Description |
---|---|
ListBase | a reusable container component having title, search box, customisable background and a List View |
ListItem | a component that renders data obtained from a User object on a Tile having a title, subtitle, leading and trailing view |
Usage
Integration
As CometChatUsers
is a custom view controller, it can be launched directly by user actions such as button clicks or other interactions. It's also possible to integrate it into a tab view controller. CometChatUsers
offers several parameters and methods for UI customization.
- Swift
let cometChatUsers = CometChatUsers()
let naviVC = UINavigationController(rootViewController: cometChatUsers)
self.present(naviVC, animated: true)
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. OnSelection
When the onSelection
event is triggered, it furnishes the list of selected users. This event can be invoked by any button or action within the interface. You have the flexibility to implement custom actions or behaviors based on the selected users.
This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- Swift
let cometChatUsers = CometChatUsers()
.onSelection { [User] in
//Perform Your Action
}
2. SetOnItemClick
The setOnItemClick
method is used to override the onClick behavior in CometChatUsers.
This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- Swift
let cometChatUsers = CometChatUsers()
.setOnItemClick (onItemClick:{ user, indexPath in
//Perform Your Action
})
3. SetOnBack
This method allows users to override the onBack Pressed behavior in CometChatUsers by utilizing the setOnBack
, providing customization options for handling the back action.
By default, this action has a predefined behavior: it simply dismisses the current view controller. However, the flexibility of CometChat UI Kit allows you to override this standard behavior according to your application's specific requirements. You can define a custom action that will be performed instead when the back button is pressed.
- Swift
let cometChatUsers = CometChatUsers()
.setOnBack (onBack:{
//Perform Your Action
})
4. onError
This method setOnError
, allows users to override error handling within CometChatUsers, providing greater control over error responses and actions.
- Swift
let cometChatUsers = CometChatUsers()
.setOnError (onError: { error in
//Perform Your Action
})
5. SetOnItemLongClick
This method setOnItemLongClick
, empowers users to customize long-click actions within CometChatUsers, offering enhanced functionality and interaction possibilities.
- Swift
let cometChatUsers = CometChatUsers()
.setOnItemLongClick { user, indexPath in
//Perform Your Action
}
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
1. UserRequestBuilder
The UserRequestBuilder enables you to filter and customize the user list based on available parameters in UserRequestBuilder. This feature allows you to create more specific and targeted queries when fetching users. The following are the parameters available in UserRequestBuilder
Methods | Type | Description |
---|---|---|
setLimit | int | sets the number users that can be fetched in a single request, suitable for pagination |
setSearchKeyword | String | used for fetching users matching the passed string |
hideBlockedUsers | bool | used for fetching all those users who are not blocked by the logged in user |
friendsOnly | bool | used for fetching only those users in which logged in user is a member |
setRoles | [String] | used for fetching users containing the passed tags |
setTags | [String] | used for fetching users containing the passed tags |
withTags | bool | used to fetch tags data along with the list of users. |
setStatus | CometChat.UserStatus | used for fetching users by their status online or offline |
setUIDs | [String] | used for fetching users containing the passed users |
Example
In the example below, we are applying a filter to the UserList based on Friends.
- Swift
let usersRequestBuilder = UsersRequest.UsersRequestBuilder(limit: 20).friendsOnly(true)
let usersWithMessages = CometChatUsersWithMessages(usersRequestBuilder: usersRequestBuilder)
let naviVC = UINavigationController(rootViewController: usersWithMessages)
self.present(naviVC, animated: true)
2. SearchRequestBuilder
The SearchRequestBuilder uses UserRequestBuilder enables you to filter and customize the search list based on available parameters in UserRequestBuilder. This feature allows you to keep uniformity between the displayed UserList and searched UserList.
Example In the example below, we are applying a filter to the UserList based on Search.
- Swift
let usersRequestBuilder = UsersRequest.UsersRequestBuilder(limit: 20)
.set(searchKeyword: "**")
let usersWithMessages = CometChatUsersWithMessages(usersRequestBuilder: usersRequestBuilder)
let naviVC = UINavigationController(rootViewController: usersWithMessages)
self.present(naviVC, animated: true)
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
To handle events supported by Users you have to add corresponding listeners by using CometChatUserEvents
Events | Description |
---|---|
emitOnUserBlock | This will get triggered when the logged in user blocks another user |
emitOnUserUnblock | This will get triggered when the logged in user unblocks another user |
- Swift
///pass the [User] object of the user which has been blocked by the logged in user
CometChatUserEvents.emitOnUserBlock(user: User)
///pass the [User] object of the user which has been unblocked by the logged in user
CometChatUserEvents.emitOnUserUnblock(user: User)
Usage
// View controller from your project where you want to listen events.
public class ViewController: UIViewController {
public override func viewDidLoad() {
super.viewDidLoad()
// Subscribing for the listener to listen events from user module
CometChatUserEvents.addListener("UNIQUE_ID", self as CometChatUserEventListener)
}
public override func viewWillDisappear(_ animated: Bool) {
// Uncubscribing for the listener to listen events from user module
CometChatUserEvents.removeListener("LISTENER_ID_USED_FOR_ADDING_THIS_LISTENER")
}
}
// Listener events from user module
extension ViewController: CometChatUserEventListener {
func onUserBlock(user: User) {
// Do Stuff
}
func onUserUnblock(user: User) {
// Do Stuff
}
}
Customization
To fit your app's design requirements, you can customize the appearance of the users component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. Users Style
You can set the UsersStyle
to the Users Component to customize the styling.
- Swift
// Creating UsersStyle object
let usersStyle = UsersStyle()
// Modifying the properties of users
usersStyle.set(background: .black)
.set(cornerRadius: CometChatCornerStyle(cornerRadius: 0.0))
.set(borderColor: .cyan)
.set(borderWidth: 10)
// Setting the users style
let cometChatUser = CometChatUsers()
// Set the usersStyle directly on CometChatUsers instance
cometChatUser.set(usersStyle: usersStyle)
let naviVC = UINavigationController(rootViewController: cometChatUser)
self.present(naviVC, animated: true)
List of properties exposed by MessageListStyle
Property | Description | Code |
---|---|---|
Background | Used to set the background color | .set(background: UIColor) |
BorderWidth | Used to set border width | .set(borderWidth: CGFloat) |
BorderColor | Used to set border color | .set(borderColor: UIColor) |
CornerRadius | Used to set border radius | .set(cornerRadius: CometChatCornerStyle) |
BackIconTint | Used to set the color of the back icon in the app bar | .set(backIconTint: UIColor) |
SearchBackground | Used to set the background color of the search box | .set(searchBackgroundColor: UIColor) |
SearchBorderRadius | Used to set the border radius of the search box | .set(searchBorderRadius: CometChatCornerStyle) |
SearchIconTint | Used to set the color of the search icon in the search box | .set(searchIconTint: UIColor) |
SearchBorderWidth | Used to set the border width of the search box | .set(searchBorderWidth: CGFloat) |
OnlineStatusColor | Used to set the color of the status indicator shown if a group member is online | .set(onlineStatusColor: UIColor) |
BackIconTint | Used to set the back icon tint color for Users | .set(backIconTint: UIColor) |
SearchCancelButtonTint | Used to set the search cancel icon tint for Users | .set(searchCancelButtonTint: UIColor) |
SearchPlaceholderFont | Used to set the search placeholder font for Users | .set(searchPlaceholderFont: UIFont) |
SearchPlaceholderColor | Used to set the search placeholder color for Users | .set(searchPlaceholderColor: UIColor) |
LargeTitleColor | Used to set the large title color for Users | .set(largeTitleColor: UIColor) |
LargeTitleFont | Used to set the large title font for Users | .set(largeTitleFont: UIFont) |
SearchTextColor | Used to set the search text color for Users | .set(searchTextColor: UIColor) |
TitleColor | Used to set the title color for Users | .set(titleColor: UIColor) |
TitleFont | Used to set the title font for Users | .set(titleFont: UIFont) |
2. Avatar Style
To apply customized styles to the Avatar
component in the Users Component, you can use the following code snippet. For further insights on Avatar
Styles refer
- Swift
// Creating AvatarStyle object
let avatarStyle = AvatarStyle()
// Creating Modifying the propeties of avatar
avatarStyle.set(background: .black)
.set(textFont: .systemFont(ofSize: 18))
.set(textColor: .green)
.set(cornerRadius: CometChatCornerStyle(cornerRadius: 8.0))
.set(borderColor: .systemOrange)
.set(borderWidth: 5)
.set(outerViewWidth: 3)
.set(outerViewSpacing: 3)
// Setting the avatar style
let cometChatUser = CometChatUsers()
cometChatUser.set(avatarStyle: avatarStyle)
3. StatusIndicator Style
To apply customized styles to the Status Indicator component in the Users Component, You can use the following code snippet. For further insights on Status Indicator Styles refer
- Swift
// Creating StatusIndicatorStyle object
let statusIndicatorStyle = StatusIndicatorStyle()
// Creating Modifying the propeties of avatar
statusIndicatorStyle.set(background: .red)
.set(cornerRadius: CometChatCornerStyle(cornerRadius: 8.0))
.set(borderColor: .red)
.set(borderWidth: 5)
.set(background: .green)
// Setting the statusIndicatorStyle
let cometChatUser = CometChatUsers()
cometChatUser.set(statusIndicatorStyle: statusIndicatorStyle)
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- Swift
let cometChatUser = CometChatUsers()
.set(title: "Hello", mode: .automatic)
.show(backButton: false)
.set(backButtonTint: .purple)
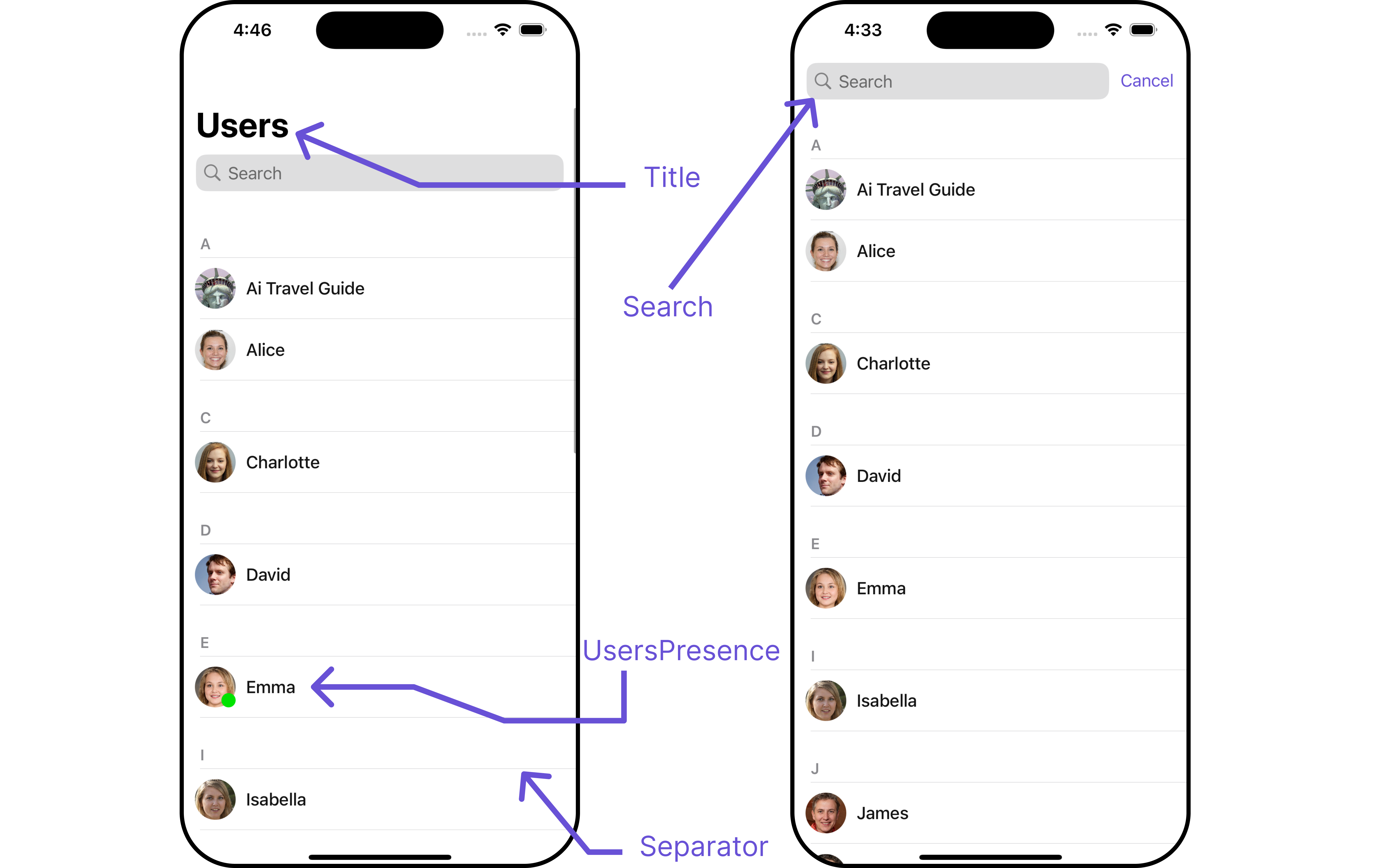
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
Set Title | Used to set title in the app | .set(title: String, mode: UINavigationItem.LargeTitleDisplayMode) |
Set SearchPlaceholderText | Used to set search placeholder text | .set(searchPlaceholder: String) |
Show BackButton | Used to toggle visibility for back button | .show(backButton: Bool) |
Set SearchIcon | Used to set search Icon in the search field | .set(searchIcon: UIImage?) |
Hide Search | Used to toggle visibility for search box | .hide(search: Bool) |
Hide Error Text | Used to hide error text on fetching users | .hide(errorText: Bool) |
Hide Separator | Used to hide the divider separating the user items | .hide(separator: Bool) |
Disable UsersPresence | Used to control visibility of user indicator shown if user is online | .disable(userPresence: Bool) |
EmptyState Text | Used to set a custom text response when fetching the users has returned an empty list | .set(emptyStateText: String) |
ErrorState Text | Used to set a custom text response when some error occurs on fetching the list of users | .set(errorStateText: String) |
Loading StateView Style | Used to set size of loading view icon while fetching the list of users | .set(loadingStateView: UIActivityIndicatorView.Style) |
Advance
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
SetListItemView
With this function, you can assign a custom ListItem to the users Component.
- Swift
let cometChatUser = CometChatUsers()
.setListItemView { user in
//Perform Your Actions
}
Example
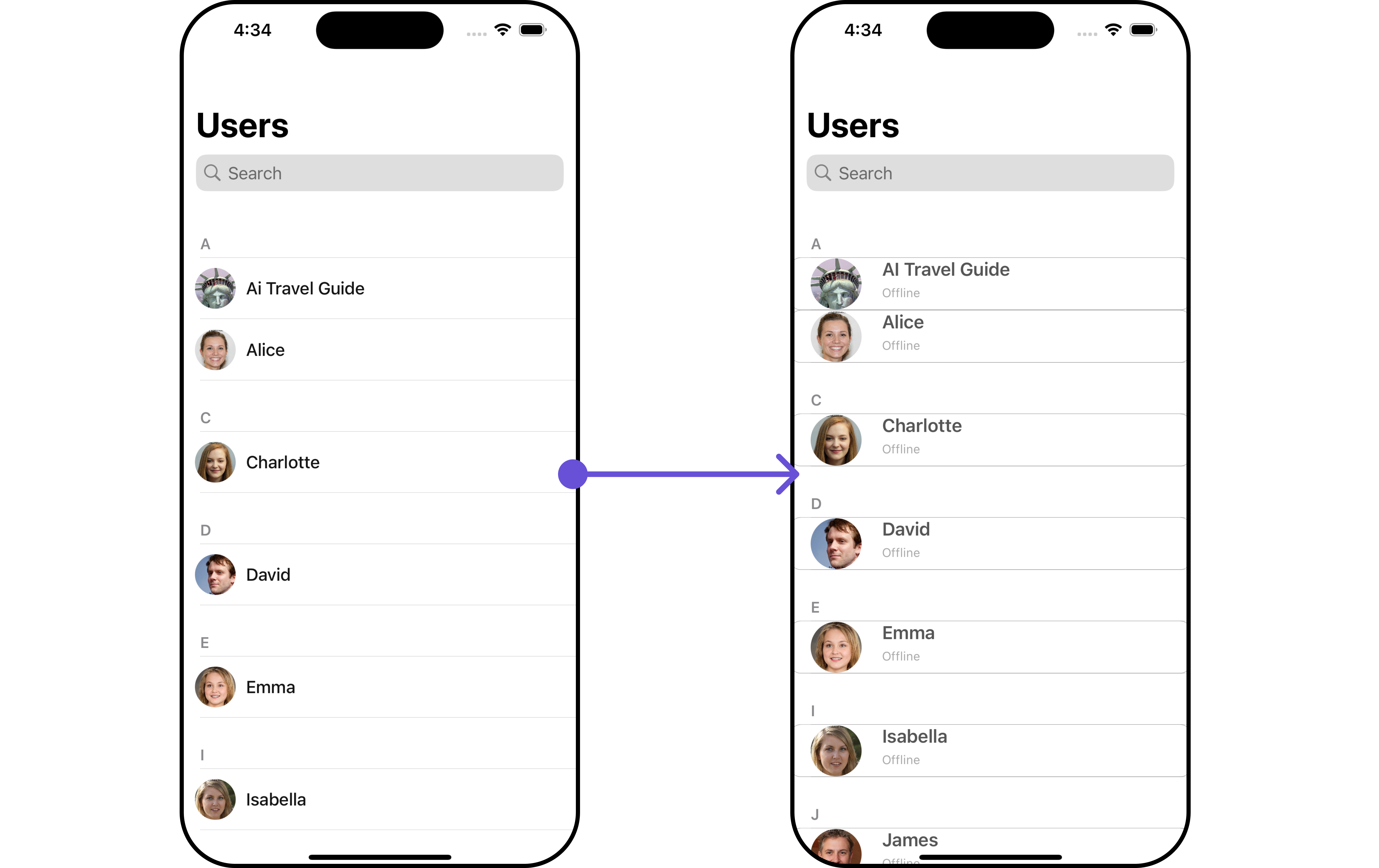
You can indeed create a custom listitem UIView file named Custom_User_ListItem_View
for more complex or unique list items.
Afterwards, seamlessly integrate this Custom_User_ListItem_View
UIView file into the .setListItemView
method within CometChatUsers().
import UIKit
import CometChatSDK
class CustomUserListItemView: UIView {
private let avatarImageView: UIImageView = {
let imageView = UIImageView()
imageView.translatesAutoresizingMaskIntoConstraints = false
imageView.contentMode = .scaleAspectFill
imageView.layer.cornerRadius = 25 // reducing the avatar size
imageView.layer.masksToBounds = true
return imageView
}()
private let nameLabel: UILabel = {
let label = UILabel()
label.font = UIFont.boldSystemFont(ofSize: 18)
label.textColor = UIColor.darkGray
label.translatesAutoresizingMaskIntoConstraints = false
return label
}()
private let statusLabel: UILabel = {
let label = UILabel()
label.font = UIFont.systemFont(ofSize: 12)
label.textColor = UIColor.lightGray
label.translatesAutoresizingMaskIntoConstraints = false
return label
}()
var user: User! {
didSet {
nameLabel.text = user.name
statusLabel.text = statusString(from: user.status)
if let avatarUrl = URL(string: user.avatar ?? "") {
DispatchQueue.global().async {
if let data = try? Data(contentsOf: avatarUrl) {
DispatchQueue.main.async {
self.avatarImageView.image = UIImage(data: data)
}
}
}
}
}
}
func statusString(from status: CometChatSDK.CometChat.UserStatus) -> String {
switch status {
case .online:
return "Online"
case .offline:
return "Offline"
default:
return "Unknown"
}
}
override init(frame: CGRect) {
super.init(frame: frame)
backgroundColor = UIColor.white
layer.cornerRadius = 10
layer.masksToBounds = true
layer.borderWidth = 0.5
layer.borderColor = UIColor.lightGray.cgColor
addSubview(avatarImageView)
addSubview(nameLabel)
addSubview(statusLabel)
NSLayoutConstraint.activate([
avatarImageView.leadingAnchor.constraint(equalTo: leadingAnchor, constant: 20),
avatarImageView.centerYAnchor.constraint(equalTo: centerYAnchor),
avatarImageView.widthAnchor.constraint(equalToConstant: 50),
avatarImageView.heightAnchor.constraint(equalToConstant: 50),
nameLabel.leadingAnchor.constraint(equalTo: avatarImageView.trailingAnchor, constant: 20),
nameLabel.topAnchor.constraint(equalTo: avatarImageView.topAnchor),
statusLabel.leadingAnchor.constraint(equalTo: nameLabel.leadingAnchor),
statusLabel.topAnchor.constraint(equalTo: nameLabel.bottomAnchor, constant: 5)
])
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
- Swift
let cometChatUser = CometChatUsers()
cometChatUser.setListItemView { user in
let customListItemView = CustomUserListItemView()
customListItemView.user = user
return customListItemView
}
let naviVC = UINavigationController(rootViewController: cometChatUser)
self.present(naviVC, animated: true)
SetSubTitleView
You can customize the subtitle view for each users item to meet your requirements
- Swift
let cometChatUser = CometChatUsers()
.setSubtitle { user in
//Perform Your Actions
}
Example
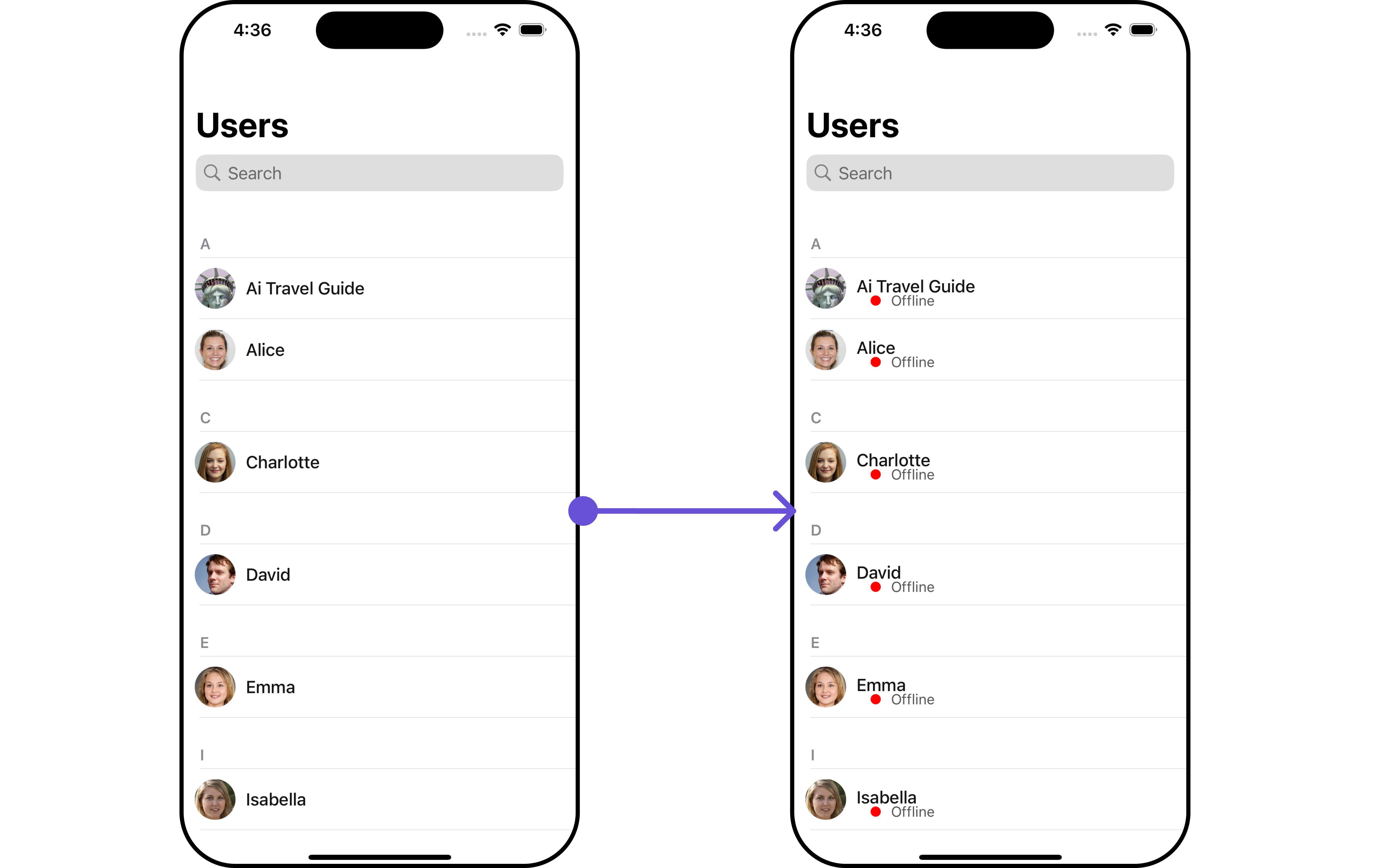
You can indeed create a custom Subtitleview UIView file named custom_user_subtitleview
for more complex or unique list items.
Afterwards, seamlessly integrate this custom_user_subtitleview
UIView file into the .setSubtitle
method within CometChatUsers().
import UIKit
import CometChatSDK
class CustomUserSubtitleView: UIView {
private let subtitleLabel: UILabel = {
let label = UILabel()
label.font = UIFont.systemFont(ofSize: 14)
label.textColor = UIColor.darkGray
label.translatesAutoresizingMaskIntoConstraints = false
return label
}()
private let statusIndicator: UIView = {
let view = UIView()
view.layer.cornerRadius = 5
view.translatesAutoresizingMaskIntoConstraints = false
return view
}()
var user: User! {
didSet {
subtitleLabel.text = statusString(from: user.status)
switch user.status {
case .online:
statusIndicator.backgroundColor = UIColor.green
case .offline:
statusIndicator.backgroundColor = UIColor.red
default:
statusIndicator.backgroundColor = UIColor.gray
}
}
}
func statusString(from status: CometChatSDK.CometChat.UserStatus) -> String {
switch status {
case .online:
return "Online"
case .offline:
return "Offline"
default:
return "Unknown"
}
}
override init(frame: CGRect) {
super.init(frame: frame)
addSubview(statusIndicator)
addSubview(subtitleLabel)
NSLayoutConstraint.activate([
statusIndicator.centerYAnchor.constraint(equalTo: centerYAnchor),
statusIndicator.centerXAnchor.constraint(equalTo: centerXAnchor, constant: -80),
statusIndicator.widthAnchor.constraint(equalToConstant: 10),
statusIndicator.heightAnchor.constraint(equalToConstant: 10),
subtitleLabel.leadingAnchor.constraint(equalTo: statusIndicator.trailingAnchor, constant: 10),
subtitleLabel.trailingAnchor.constraint(equalTo: trailingAnchor, constant: -10),
subtitleLabel.centerYAnchor.constraint(equalTo: centerYAnchor)
])
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
- View Controller
let cometChatUser = CometChatUsers()
.setSubtitle { user in
let customUserSubtitleView = CustomUserSubtitleView()
customUserSubtitleView.user = user
return customUserSubtitleView
}
let naviVC = UINavigationController(rootViewController: cometChatUser)
self.present(naviVC, animated: true)
SetMenu
You can set the Custom Menu view to add more options to the Users component.
- Swift
let cometChatUser = CometChatUsers()
.set(menus: [UIBarButtonItem])
Example
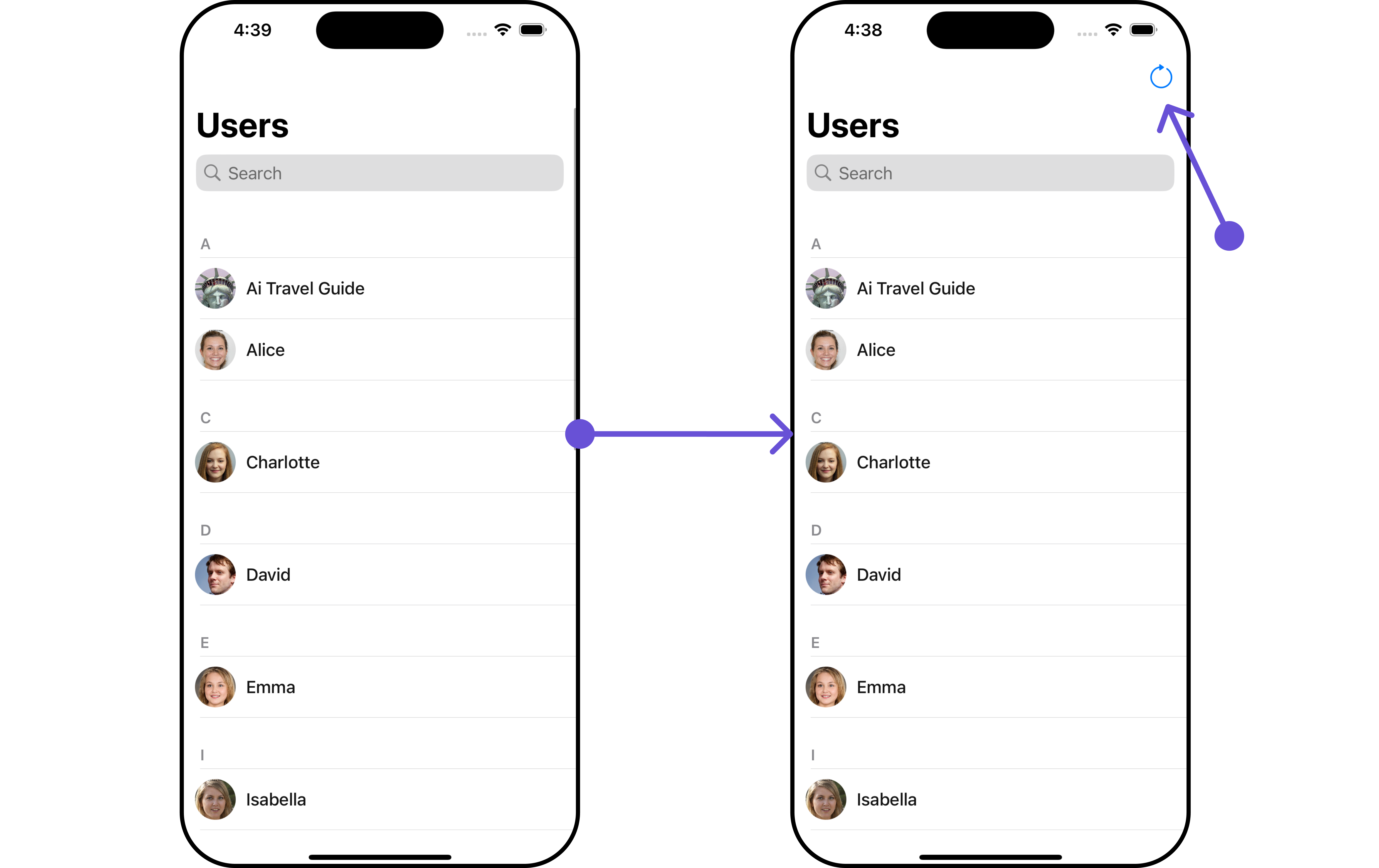
You can create a new array of [UIBarButtonItem] objects. This array will be inflated and then passed to the .setMenu.
let cometChatUser = CometChatUsers()
let menuButtonItem1 = UIBarButtonItem(image: UIImage(systemName: "goforward"), style: .plain, target: self, action: #selector(self.menuButtonAction))
let menus = [menuButtonItem1]
cometChatUser.set(menus: menus)
You can manage click actions accordingly.
- Swift
@objc func menuButtonAction(sender: UIBarButtonItem) {
// Add your action here
}
SetEmptyStateView
You can set a custom EmptyStateView
using setEmptyStateView
to match the empty view of your app.
- Swift
let cometChatUser = CometChatUsers()
.set(emptyView: UIView)
Examples
You have to create a custom UIView file named Empty_View
, you can set it as the empty state view by passing it as a parameter to the setEmptyView()
method.
import UIKit
class EmptyView: UIView {
override init(frame: CGRect) {
super.init(frame: frame)
setupView()
}
required init?(coder: NSCoder) {
super.init(coder: coder)
setupView()
}
private func setupView() {
backgroundColor = .systemBackground // Or any color matching your application's styles
let imageView = UIImageView()
imageView.image = UIImage(systemName: "message") // Replace with your image
imageView.translatesAutoresizingMaskIntoConstraints = false
addSubview(imageView)
let label = UILabel()
label.text = "No Messages"
label.font = UIFont.systemFont(ofSize: 30)
label.translatesAutoresizingMaskIntoConstraints = false
addSubview(label)
NSLayoutConstraint.activate([
imageView.centerXAnchor.constraint(equalTo: centerXAnchor),
imageView.centerYAnchor.constraint(equalTo: centerYAnchor, constant: -50),
imageView.widthAnchor.constraint(equalToConstant: 100),
imageView.heightAnchor.constraint(equalToConstant: 100),
label.topAnchor.constraint(equalTo: imageView.bottomAnchor, constant: 50),
label.centerXAnchor.constraint(equalTo: imageView.centerXAnchor)
])
}
}
- Swift
let emptyView = EmptyView()
let cometChatUser = CometChatUsers()
.set(emptyView: emptyView)
let naviVC = UINavigationController(rootViewController: cometChatUser)
self.present(naviVC, animated: true)
SetErrorStateView
You can set a custom ErrorStateView
using setErrorStateView
to match the error view of your app.
- Swift
let cometChatUser = CometChatUsers()
.set(errorView: UIView)
Example
We've implemented an error view within the error_view
UIView file, designed to improve user interaction during error states. Simply select the view of your preference and pass it to the .set(errorView: errorView)
method for seamless integration.
import UIKit
import CometChatSDK
import CometChatUIKitSwift
class ErrorView: UIView {
override init(frame: CGRect) {
super.init(frame: frame)
setupView()
}
required init?(coder: NSCoder) {
super.init(coder: coder)
setupView()
}
private func setupView() {
backgroundColor = .systemBackground // Or any color matching your application's styles
let imageView = UIImageView()
imageView.image = UIImage(systemName: "exclamationmark.circle") // Replace with your error image
imageView.translatesAutoresizingMaskIntoConstraints = false
addSubview(imageView)
let label = UILabel()
label.text = "Something Went Wrong"
label.font = UIFont.systemFont(ofSize: 30)
label.translatesAutoresizingMaskIntoConstraints = false
addSubview(label)
NSLayoutConstraint.activate([
imageView.centerXAnchor.constraint(equalTo: centerXAnchor),
imageView.centerYAnchor.constraint(equalTo: centerYAnchor, constant: -50), // Adjust as needed
imageView.widthAnchor.constraint(equalToConstant: 100),
imageView.heightAnchor.constraint(equalToConstant: 100),
label.topAnchor.constraint(equalTo: imageView.bottomAnchor, constant: 50), // Adjust as needed
label.centerXAnchor.constraint(equalTo: imageView.centerXAnchor)
])
}
}
- Swift
let errorView = ErrorView()
let cometChatUser = CometChatUsers()
.set(errorView: errorView)
let naviVC = UINavigationController(rootViewController: cometChatUser)
self.present(naviVC, animated: true)