Message Composer
Overview
MessageComposer is a Component that enables users to write and send a variety of messages, including text, image, video, and custom messages.
Features such as Live Reaction, Attachments, and Message Editing are also supported by it.
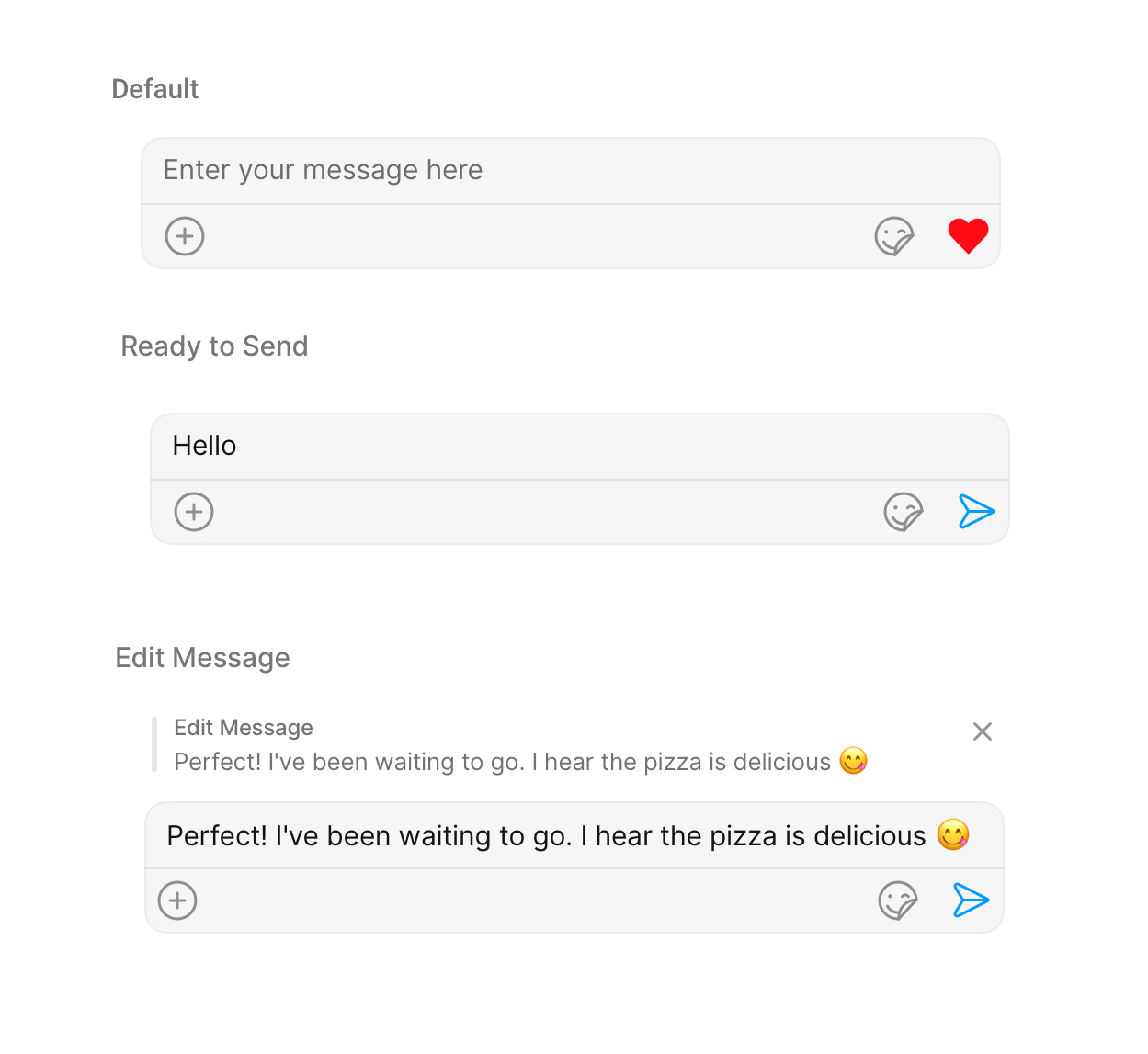
MessageComposer is comprised of the following Base Components:
Base Components | Description |
---|---|
MessageInput | This provides a basic layout for the contents of this component, such as the TextField and buttons |
ActionSheet | The ActionSheet component presents a list of options in either a list or grid mode, depending on the user's preference |
Usage
Integration
The following code snippet illustrates how you can directly incorporate the MessageComposer component into your file.
// syntax for set(user: User)
let messageComposer = CometChatMessageComposer()
messageComposer.set(user: user)
messageComposer.set(parentMessageId: 20)
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. OnSendButtonClick
The OnSendButtonClick
event gets activated when the send message button is clicked. The following code snippet Overrides the action of the send button in CometChatMessageComposer.
- Swift
// syntax for set(sendIcon: UIImage)
messageComposer.setOnSendButtonClick { message in
// return custom action here
}
Filters
MessageComposer component does not have any available filters.
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The MessageComposer Component does not emit any events of its own.
Customization
To fit your app's design requirements, you can customize the appearance of the MessageComposer component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. MessageComposer Style
To customize the styling, you can apply the MessageComposerStyle
to the MessageComposer component.
- Swift
let messageComposerStyle = MessageComposerStyle()
let messageComposerConfiguration = MessageComposerConfiguration()
.set(messageComposerStyle: messageComposerStyle)
The following properties are exposed by MessageComposerStyle:
Property | Description | Method |
---|---|---|
Set BorderWidth | Sets the border width for message composer | .set(borderWidth: CGFloat) |
Set BorderColor | Sets the border color for message composer | .set(borderColor: UIColor) |
Set CornerRadius | Sets the corner radius for message composer | .set(cornerRadius: CometChatCornerStyle) |
Set Background | Sets the background color for message composer | .set(background: UIColor) |
Set InputBackgroundColor | Sets the input background color of message composer | .set(inputBackground: UIColor) |
Set TextFont | Sets the input text font of message composer | .set(textFont: UIFont) |
Set InputBoxPlaceholderFont | Sets the placeholder text font for message composer input field | .set(inputBoxPlaceholderFont: UIFont) |
Set PlaceHolderTextColor | Sets the placeholder text color for message composer input field | .set(placeHolderTextColor: UIColor) |
Set AttachIconTint | Sets the attachment icon tint color | .set(attachmentIconTint: UIColor) |
Set SendIconTint | Sets send button icon tint color | .set(sendIconTint: UIColor) |
Set SeparatorTint | Sets the separator color for message composer | .set(separatorTint: UIColor) |
Set InputBorderWidth | Sets the border width for message composer input view | .set(inputBorderWidth: CGFloat) |
Set InputBorderColor | Sets the border color for message composer input view | .set(inputBorderColor: UIColor) |
Set ActionSheetTitleColor | Sets the title color for action sheet of message composer | .set(actionSheetTitleColor: UIColor) |
Set ActionSheetTitleFont | Sets the title font for action sheet of message composer | .set(actionSheetTitleFont: UIFont) |
Set ActionSheetLayoutModelIconTint | Sets action sheet layout mode icon tint color for message composer | .set(actionSheetLayoutModelIconTint: UIColor) |
Set ActionSheetCancelButtonIconTint | Sets action sheet cancel button icon tint color for message composer | .set(actionSheetCancelButtonIconTint: UIColor) |
Set ActionSheetCancelButtonIconFont | Sets the action sheet cancel button icon font color for message composer | .set(actionSheetCancelButtonIconFont: UIFont) |
Set ActionSheetSeparatorTint | Sets the separator color for action sheet items | .set(actionSheetSeparatorTint: UIColor) |
Set ActionSheetBackground | Sets the background color of action sheet | .set(actionSheetBackground: UIColor) |
Set VoiceRecordingIconTint | Sets the voice recorder icon tint color | .set(voiceRecordingIconTint: UIColor) |
Set AiIconTint | Sets the ai icon tint color | .set(aiIconTint: UIColor) |
Set InfoTextColor | Sets the text color for info message displayed | .set(infoTextColor: UIColor) |
Set InfoIconTintColor | Sets the tint color for info icon | .set(infoIconTintColor: UIColor) |
Set InfoBackgroundColor | Sets the background color for info message view | .set(infoBackgroundColor: UIColor) |
Set InfoSeparatorColor | Sets the separator color for info view | .set(infoSeparatorColor: UIColor) |
2. MediaRecorder Style
To customize the styles of the MediaRecorder component within the MessageComposer Component, use the .set(mediaRecorderStyle:)
method. For more details, please refer to MediaRecorder styles.
- Swift
let mediaRecorderStyle = MediaRecorderStyle()
.set(borderColor: .orange)
.set(borderWidth: 10)
let messageComposerConfiguration = MessageComposerConfiguration()
.set(mediaRecorderStyle: mediaRecorderStyle)
The following properties are exposed by MessageComposerStyle:
Property | Description | Code |
---|---|---|
Set PlayIconTint | Sets play voice button tint color | .set(playIconTint: UIColor) |
Set StopIconTint | Sets stop voice button tint color | .set(stopIconTint: UIColor) |
Set PauseIconTint | Sets pause voice button tint color | .set(pauseIconTint: UIColor) |
Set SubmitIconTint | Sets submit voice button tint color | .set(submitIconTint: UIColor) |
Set TimerTextColor | Sets text color for voice record timer | .set(timerTextColor: UIColor) |
Set TimerTextFont | Sets text font for voice record timer | .set(timerTextFont: UIFont) |
Set DeleteIconTint | Sets delete voice button tint color | .set(deleteIconTint: UIColor) |
3. AI Options Style
To customize the styles of the MediaRecorder component within the MessageComposer Component, use the .set(aiOptionsStyle:)
method. For more details, please refer to MediaRecorder styles.
- Swift
let aiOptionsStyle = AIOptionsStyle()
.set(aiIconTint: .orange)
.set(buttonBorder: 2)
let messageComposerConfiguration = MessageComposerConfiguration()
.set(aiOptionsStyle: aiOptionsStyle)
The following properties are exposed by MessageComposerStyle:
Property | Description | Code |
---|---|---|
Set AIIconTint | Sets ai button tint color | .set(aiIconTint: UIColor) |
Set ButtonBorder | Sets border for ai options button | .set(buttonBorder: CGFloat) |
Set ButtonBackground | Sets background color for ai options button | .set(buttonBackground: UIColor) |
Set ButtonTextFont | Sets ai options button text font | .set(buttonTextFont: UIColor) |
Set ButtonTextColor | Sets ai options button text color | .set(buttonTextColor: UIColor) |
Set ButtonBorderColor | Sets border color for ai options button | .set(buttonBorderColor: UIColor) |
Set ButtonBorderRadius | Sets border radius for ai options button | .set(buttonBorderRadius: CGFloat) |
Set CancelBackground | Sets cancel button background color | .set(cancelBackground: UIColor) |
Set CancelButtonFont | Sets cancel button text font | .set(cancelButtonFont: UIFont) |
Set CancelButtonColor | Sets cancel button text color | .set(cancelButtonColor: UIColor) |
Set CancelButtonBorder | Sets border for cancel button | .set(cancelButtonBorder: CGFloat) |
Set CancelButtonBorderColor | Sets cancel button border color | .set(cancelButtonBorderColor: UIColor) |
Set CancelButtonBorderRadius | Sets border radius for cancel button | .set(cancelButtonBorderRadius: CGFloat) |
Set Background | Sets background color for ai options action sheet | .set(background: UIColor) |
Set BorderWidth | Sets border width for ai options action sheet | .set(borderWidth: CGFloat) |
Set BorderColor | Sets border color for ai options action sheet | .set(borderColor: UIColor) |
Set CornerRadius | Sets corner radius for ai options action sheet | .set(background: CGFloat) |
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- Swift
let messageComposer = CometChatMessageComposer()
.disable(disableTypingEvents: true)
.hide(liveReaction: true)
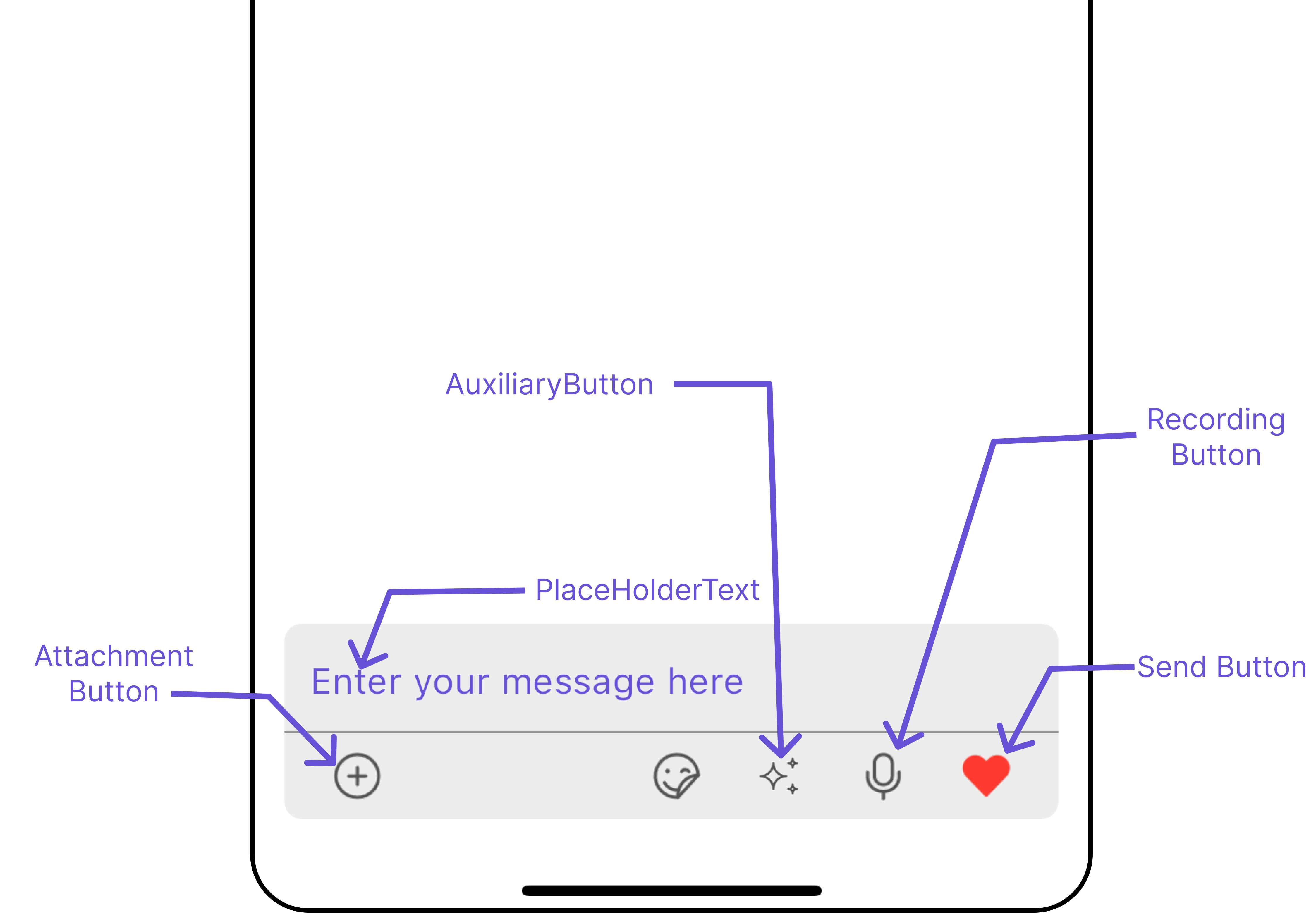
Below is a list of customizations along with corresponding code snippets
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
SetTextFormatters
Assigns the list of text formatters. If the provided list is not null, it sets the list. Otherwise, it assigns the default text formatters retrieved from the data source. To configure the existing Mentions look and feel check out CometChatMentionsFormatter
Example
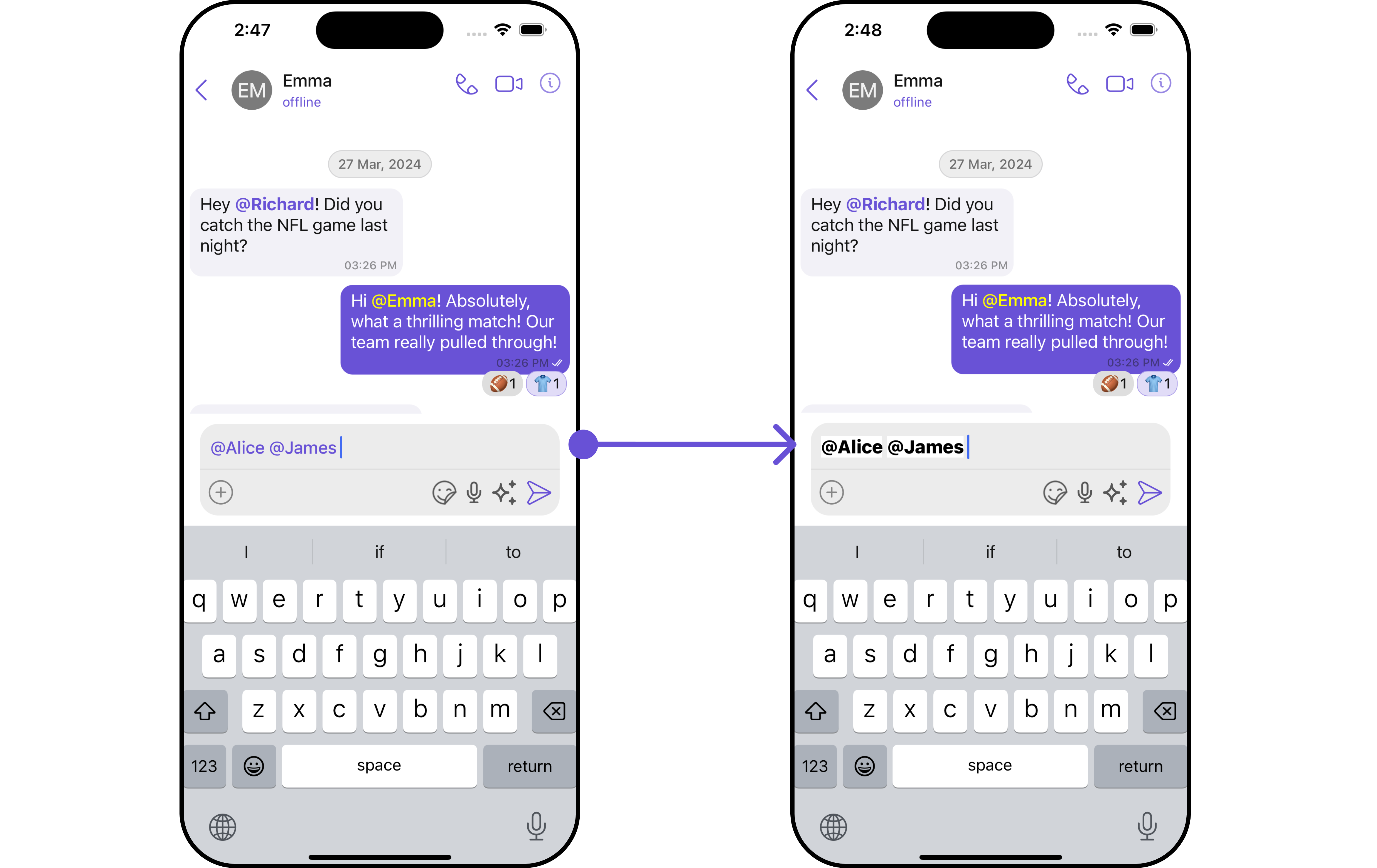
- Swift
let composerTextStyle = MentionTextStyle()
.set(textBackgroundColor: .white)
.set(textColor: UIColor.black)
.set(textFont: UIFont.systemFont(ofSize: 18, weight: .heavy))
.set(loggedInUserTextColor: UIColor.systemTeal)
.set(loggedInUserTextFont: UIFont.systemFont(ofSize: 18, weight: .bold))
let customMentionFormatter = CometChatMentionsFormatter()
.set(composerTextStyle: composerTextStyle)
let messageComposerConfiguration = MessageComposerConfiguration()
.set(textFormatter: [customMentionFormatter])
let cometChatMessages = CometChatMessages()
.set(user: user)
.set(messageComposerConfiguration: messageComposerConfiguration)
AttachmentOptions
By using setAttachmentOptions()
, you can set a list of custom MessageComposerActions
for the MessageComposer Component. This will override the existing list of MessageComposerActions
.
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.setAttachmentOptions { user, group, controller in
}
Example
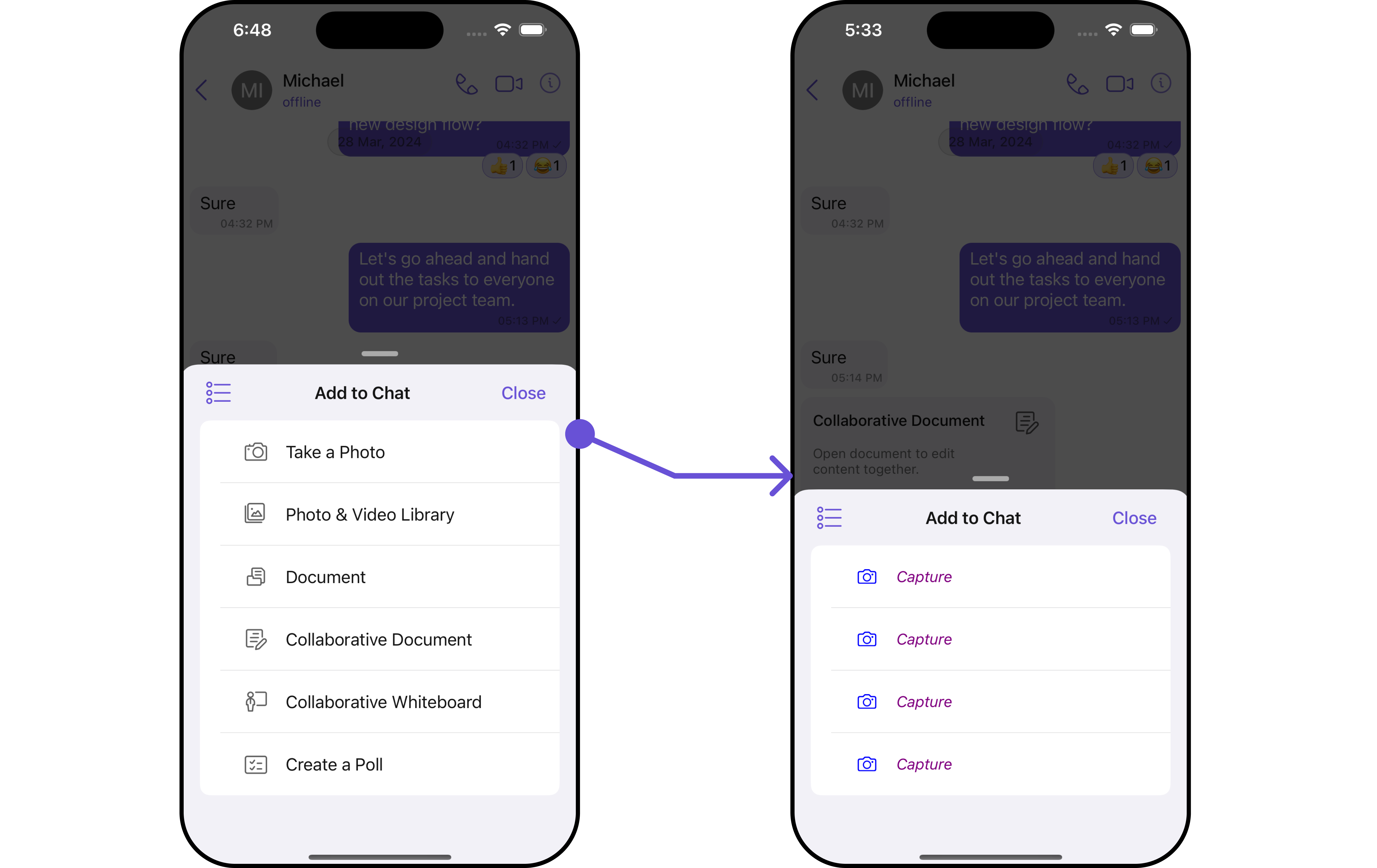
In this example, we are overriding the existing MessageComposerActions List with Capture Photo actions.
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.setAttachmentOptions { user, group, controller in
let customComposerAction1 = CometChatMessageComposerAction(
id: "customAction1",
text: "Capture",
startIcon: UIImage(systemName: "camera"),
endIcon: nil,
startIconTint: .blue,
endIconTint: nil,
textColor: .purple,
textFont: .italicSystemFont(ofSize: 15),
backgroundColour: .white,
borderWidth: 1,
borderColor: .gray,
onActionClick: {
print("Custom Action 1 clicked!")
}
)
return [customComposerAction1 , customComposerAction1, customComposerAction1, customComposerAction1 ]
}
let cometChatMessages = CometChatMessages()
.set(user: user)
.set(messageComposerConfiguration: messageComposerConfiguration)
AuxiliaryButtonView
You can insert a custom view into the MessageComposer component to add additional functionality using the following method.
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.setAuxilaryButtonView { user, group in
}
Please note that the MessageComposer Component utilizes the AuxiliaryButton to provide sticker functionality. Overriding the AuxiliaryButton will subsequently replace the sticker functionality.
Example
In this example, we'll be adding a custom button with click functionality. You'll first need to create a custom UIView file and then inflate it inside the .setAuxiliaryButtonView()
function.
let messageComposerConfiguration = MessageComposerConfiguration()
.setAuxilaryButtonView { user, group in
let customView = UIView()
customView.translatesAutoresizingMaskIntoConstraints = false
let customButton = UIButton()
customButton.setImage(UIImage(systemName: "bell"), for: .normal)
customButton.translatesAutoresizingMaskIntoConstraints = false
customButton.addTarget(self, action: #selector(self.customButtonClicked), for: .touchUpInside)
customView.addSubview(customButton)
NSLayoutConstraint.activate([
customButton.trailingAnchor.constraint(equalTo: customView.trailingAnchor, constant: -8),
customButton.centerYAnchor.constraint(equalTo: customView.centerYAnchor),
customButton.heightAnchor.constraint(equalToConstant: 30),
customButton.widthAnchor.constraint(equalToConstant: 80)
])
return customView
}
let cometChatMessages = CometChatMessages()
.set(user: user)
.set(messageComposerConfiguration: messageComposerConfiguration)
SecondaryButtonView
You can add a custom view into the SecondaryButton component for additional functionality using the below method.
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.setSecondaryButtonView { user, group in
}
Please note that the MessageComposer Component uses the SecondaryButton to open the ComposerActionsList. Overriding the SecondaryButton will replace the ComposerActionsList functionality.
Example
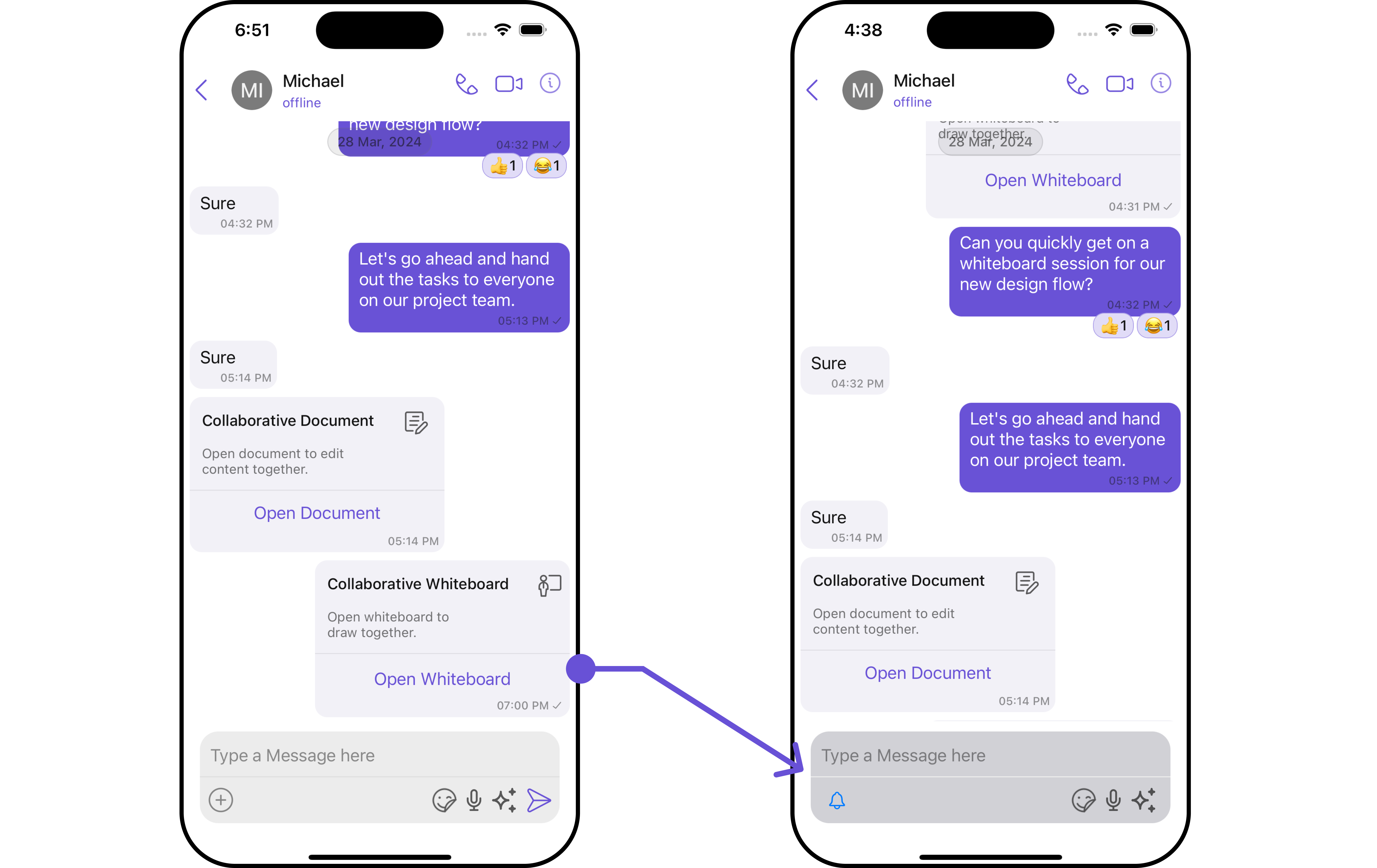
In this example, we'll be adding a custom button with click functionality. You'll first need to create a custom UIView file and then inflate it inside the .setSecondaryButtonView()
function.
let messageComposerConfiguration = MessageComposerConfiguration()
.setSecondaryButtonView { user, group in
let customView = UIView()
customView.translatesAutoresizingMaskIntoConstraints = false
let customButton = UIButton()
customButton.setImage(UIImage(systemName: "bell"), for: .normal)
customButton.translatesAutoresizingMaskIntoConstraints = false
customButton.addTarget(self, action: #selector(self.customButtonClicked), for: .touchUpInside)
customView.addSubview(customButton)
NSLayoutConstraint.activate([
customButton.leadingAnchor.constraint(equalTo: customView.leadingAnchor, constant: -20),
customButton.centerYAnchor.constraint(equalTo: customView.centerYAnchor),
customButton.heightAnchor.constraint(equalToConstant: 30),
customButton.widthAnchor.constraint(equalToConstant: 80)
])
return customView
}
let cometChatMessages = CometChatMessages()
.set(user: user)
.set(messageComposerConfiguration: messageComposerConfiguration)
Set SendButtonView
You can set a custom view in place of the already existing send button view. Using the following method.
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.setSendButtonView { user, group in
}
Example
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.setSendButtonView { user, group in
let customButton = UIButton()
customButton.setImage(UIImage(systemName: "bell"), for: .normal)
customButton.translatesAutoresizingMaskIntoConstraints = false
customButton.addTarget(self, action: #selector(self.customButtonClicked), for: .touchUpInside)
return customButton
}
let cometChatMessages = CometChatMessages()
.set(user: user)
.set(messageComposerConfiguration: messageComposerConfiguration)
HeaderView
You can set custom headerView to the MessageComposer component using the following method
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.set(headerView: UIView())
Example
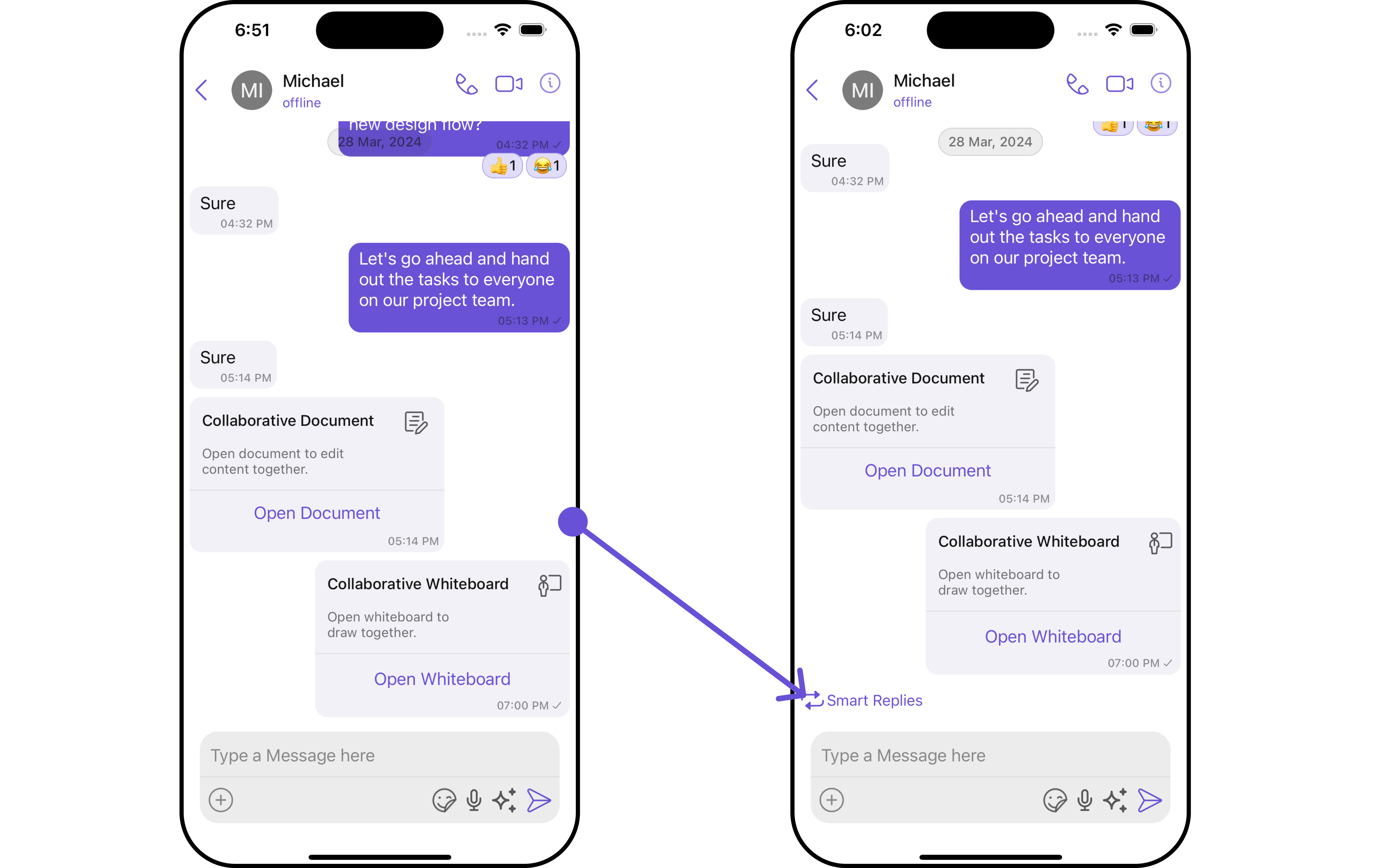
In the following example, we're going to apply a mock smart reply view to the MessageComposer Component using the .set(headerView: UIView?)
method.
import UIKit
class CustomActionView: UIView {
let actionButton: UIButton
override init(frame: CGRect) {
actionButton = UIButton(type: .system)
actionButton.backgroundColor? = .purple
actionButton.tintColor = .init(red: 0.41, green: 0.32, blue: 0.84, alpha: 1.00)
actionButton.setTitle("Smart Replies", for: .normal)
let image = UIImage(systemName: "arrowshape.turn.up.left.2.fill")
actionButton.setImage(image, for: .normal)
actionButton.translatesAutoresizingMaskIntoConstraints = false
super.init(frame: frame)
addSubview(actionButton)
setupButtonConstraints()
actionButton.addTarget(self, action: #selector(buttonTapped), for: .touchUpInside)
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
private func setupButtonConstraints() {
NSLayoutConstraint.activate([
actionButton.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 8),
actionButton.centerYAnchor.constraint(equalTo: self.centerYAnchor)
])
}
@objc private func buttonTapped() {
print("Button was tapped!")
}
}
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.set(headerView: CustomActionView())
let cometChatMessages = CometChatMessages()
.set(user: user)
.set(messageComposerConfiguration: messageComposerConfiguration)
Ensure to pass and present cometChatMessages
. If a navigation controller is already in use, utilize the pushViewController function instead of directly presenting the view controller.
FooterView
You can set a custom footer view to the MessageComposer component using the following method:
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.set(footerView: UIView())
Example
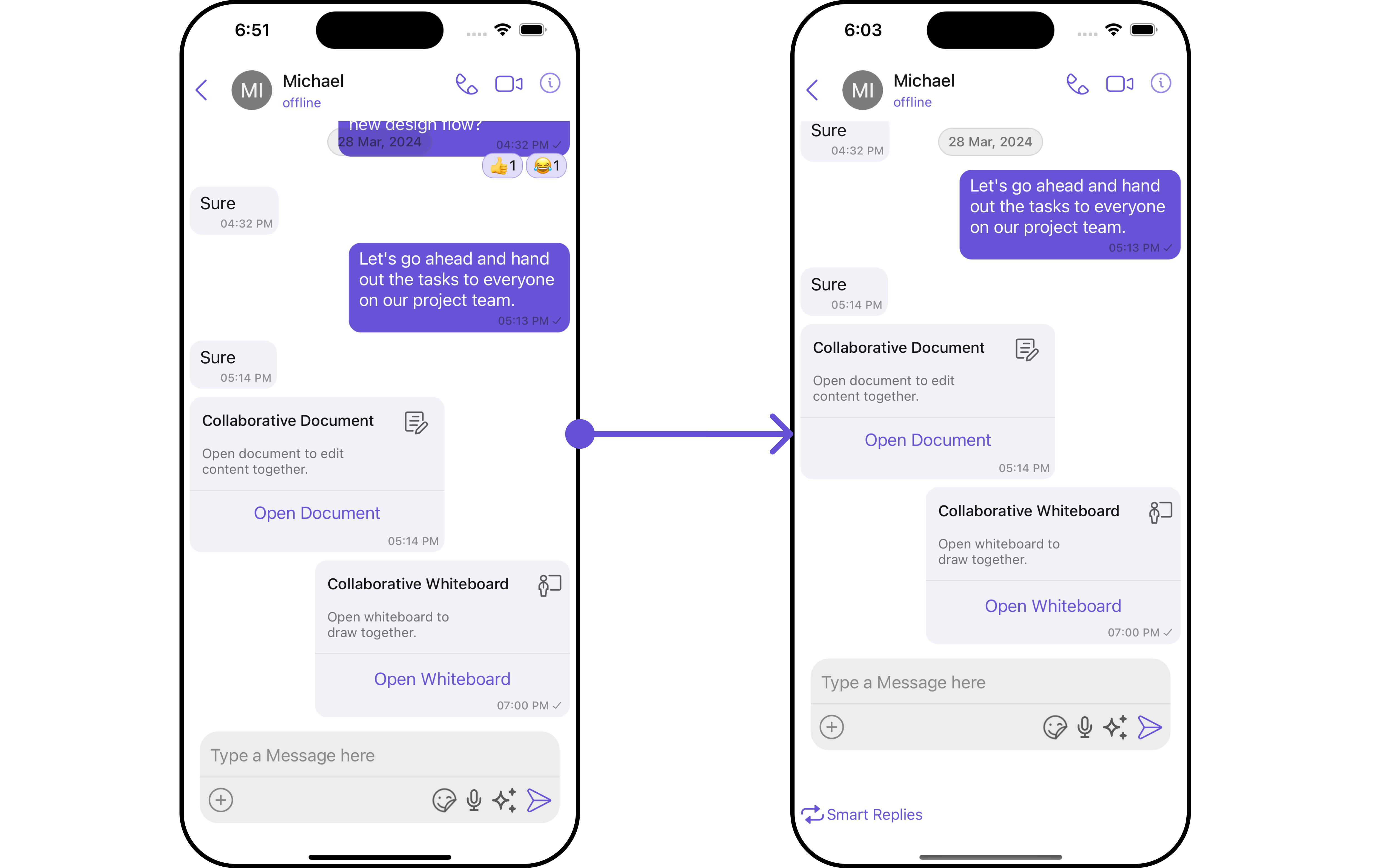
In the following example, we're going to apply a mock smart reply view to the MessageComposer Component using the .set(footerView: UIView?)
method.
import UIKit
class CustomActionView: UIView {
let actionButton: UIButton
override init(frame: CGRect) {
actionButton = UIButton(type: .system)
actionButton.backgroundColor? = .purple
actionButton.tintColor = .init(red: 0.41, green: 0.32, blue: 0.84, alpha: 1.00)
actionButton.setTitle("Smart Replies", for: .normal)
let image = UIImage(systemName: "repeat")
actionButton.setImage(image, for: .normal)
actionButton.translatesAutoresizingMaskIntoConstraints = false
super.init(frame: frame)
addSubview(actionButton)
setupButtonConstraints()
actionButton.addTarget(self, action: #selector(buttonTapped), for: .touchUpInside)
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
private func setupButtonConstraints() {
NSLayoutConstraint.activate([
actionButton.leadingAnchor.constraint(equalTo: self.leadingAnchor, constant: 8),
actionButton.centerYAnchor.constraint(equalTo: self.centerYAnchor)
])
}
@objc private func buttonTapped() {
print("Button was tapped!")
}
}
- Swift
let messageComposerConfiguration = MessageComposerConfiguration()
.set(footerView: CustomActionView())
let cometChatMessages = CometChatMessages()
.set(user: user)
.set(messageComposerConfiguration: messageComposerConfiguration)
Ensure to pass and present cometChatMessages
. If a navigation controller is already in use, utilize the pushViewController function instead of directly presenting the view controller.