Call Logs
Overview
CometChatCallLogs
is a Component that shows the list of Call Log available . By default, names are shown for all listed users, along with their avatar if available.
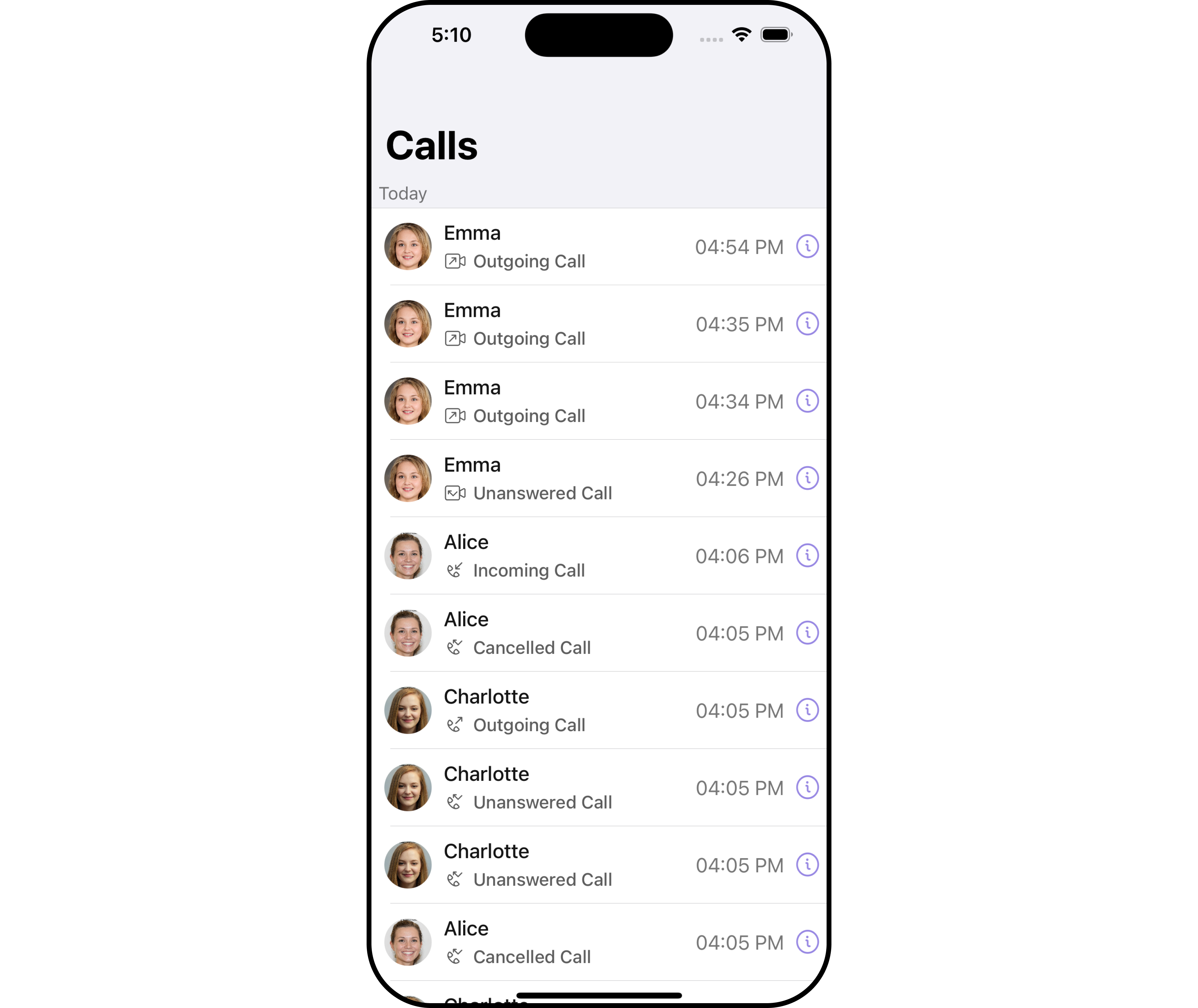
The Call Logs
component is composed of the following BaseComponents:
Components | Description |
---|---|
CometChatListBase | CometChatListBase is a container component featuring a title, customizable background options, and a dedicated list view for seamless integration within your application's interface. |
CometChatListItem | This component displays data retrieved from a CallLog object on a card, presenting a title and subtitle. |
Usage
Integration
CometChatCallLogs
being a custom view controller, offers versatility in its integration. It can be seamlessly launched via button clicks or any user-triggered action, enhancing the overall user experience and facilitating smoother interactions within the application.
- Swift
// To navigate to the CometChatCallLogs
let callLogs = CometChatCallLogs()
self.navigationController?.pushViewController(callLogs, animated: true)
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. OnItemClick
This method proves valuable when users seek to override onItemClick functionality within CometChatCallLogs, empowering them with greater control and customization options.
The setOnItemClick
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- Swift
let callLogsConfiguration = CallLogsConfiguration()
.set (onItemClick:{ callLog in
//Perform Your Action
})
2. OnError
You can customize this behavior by using the provided code snippet to override the On Error
and improve error handling.
- Swift
let callLogsConfiguration = CallLogsConfiguration()
.set (onError:{ error in
//Perform Your Action
})
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of ChatSDK.
1. CallRequestBuilder
The callRequestBuilder enables you to filter and customize the call list based on available parameters in callRequestBuilder. This feature allows you to create more specific and targeted queries during the call. The following are the parameters available in callRequestBuilder
Method | Description | Code |
---|---|---|
fetchPrevious | Fetches previous call logs | fetchPrevious(authToken: String, onSuccess: (([CallLog]) -> Void), onError: (_ error: CometChatCallException?) -> Void) |
fetchNext | Fetches next call logs | fetchNext(onSuccess: (([CallLog]) -> Void), onError: (_ error: CometChatCallException?) -> Void) |
limit | Sets the limit for the call logs request | .set(limit: Int) |
callType | Sets the call type for the call logs request | .set(callType: CallType) |
callStatus | Sets the call status for the call logs request | .set(callStatus: CallStatus) |
hasRecording | Sets the recording status for the call logs request | .set(hasRecording: Bool) |
callDirection | Sets the call direction for the call logs request | .set(callDirection: CallDirection) |
uid | Sets the user ID for the call logs request | .set(uid: String) |
guid | Sets the group ID for the call logs request | .set(guid: String) |
authToken | Sets the auth token for the call logs request | .set(authToken: String?) |
build | Builds the call logs request | .build() |
Example
In the example below, we are applying a filter based on limit , calltype and call status.
- Swift
let callRequestBuilder = CallLogsRequest.CallLogsBuilder()
.set(limit: 2)
.set(callType: .audio)
.set(callStatus: .initiated)
// To navigate to the CometChatCallLogs
let callLogs = CometChatCallLogs()
.set(callRequestBuilder: callRequestBuilder)
self.navigationController?.pushViewController(callLogs, animated: true)
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The Call Logs
component does not have any exposed events.
Customization
To fit your app's design requirements, you can customize the appearance of the conversation component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. CallLog Style
You can customize the appearance of the CallLog
Component by applying the CallLogStyle
to it using the following code snippet.
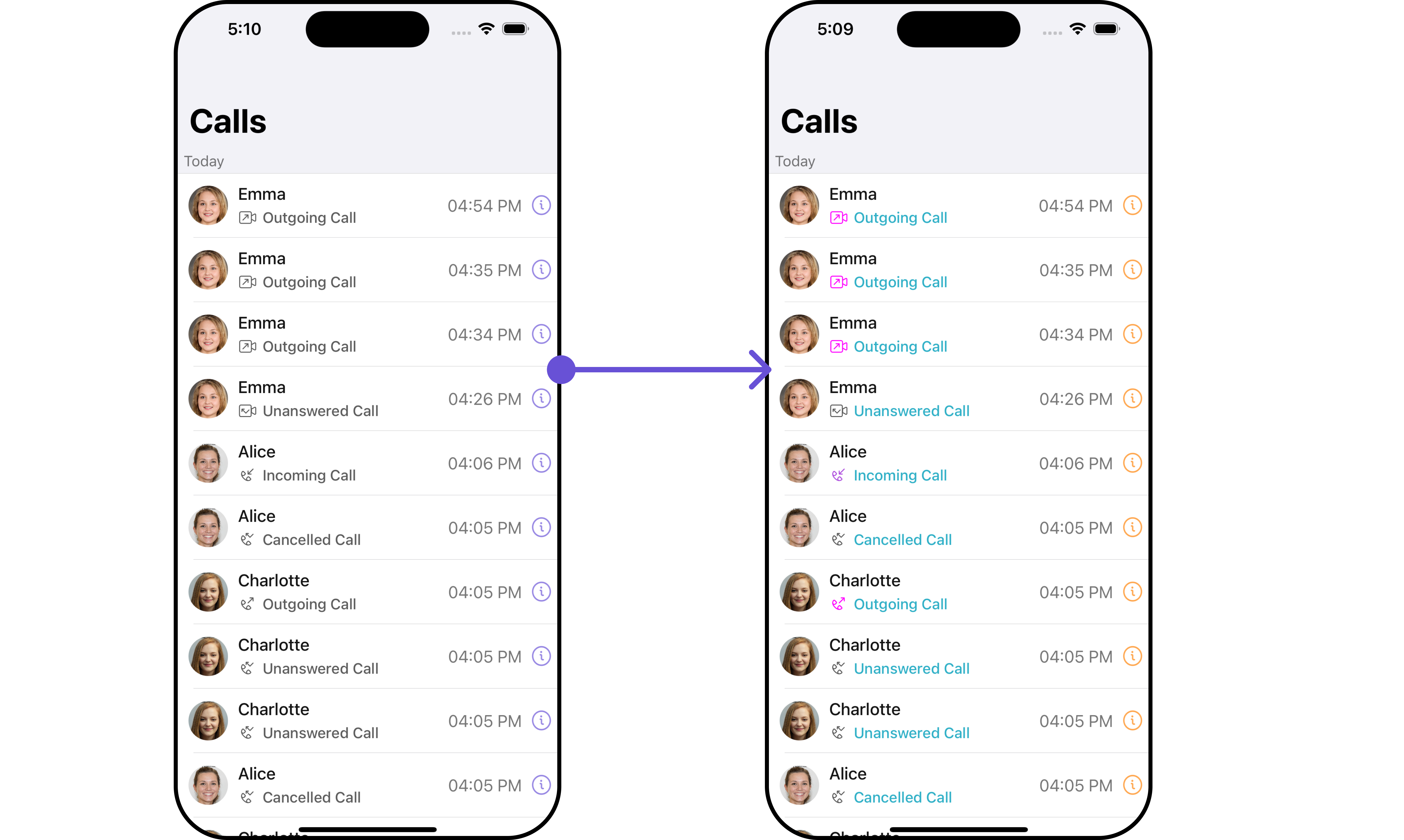
- Swift
let callLogStyle = CallLogStyle()
.set(background: .blue)
.set(callStatusIconTint: .red)
.set(callTimeTextColor: .systemTeal)
.set(infoIconTint: .orange)
.set(borderColor: .green)
.set(incomingCallIconTint: .systemPurple)
.set(outgoingCallIconTint: .magenta)
let callLogsConfiguration = CallLogsConfiguration()
.set(style: callLogStyle)
// To navigate to the CometChatCallLogs
let callLogs = CometChatCallLogs()
.set(configuration: callLogsConfiguration)
List of properties exposed by CallLogStyle
Property | Description | Code |
---|---|---|
callStatusTextFont | Sets the call status font | .set(callStatusTextFont: UIFont) |
missedCallTitleTint | Sets the missed call color | .set(missedCallTitleTint: UIColor) |
callTimeTextFont | Sets the call time font | .set(callTimeTextFont: UIFont) |
dateSeparatorTextFont | Sets the date separator font | .set(dateSeparatorTextFont: UIFont) |
emptyStateTextFon | Sets the empty state font | .set(emptyStateTextFont: UIFont) |
errorStateTextFont | Sets the error state font | .set(errorStateTextFont: UIFont) |
callStatusTextColor | Sets the call status color | .set(callStatusTextColor: UIColor) |
callStatusIconTint | Sets the call status icon tint | .set(callStatusIconTint: UIColor) |
callTimeTextColor | Sets the call time color | .set(callTimeTextColor: UIColor) |
dateSeparatorTextColor | Sets the date separator color | .set(dateSeparatorTextColor: UIColor) |
missedCallIconTint | Sets the missed call icon tint | .set(missedCallIconTint: UIColor) |
outgoingCallIconTint | Sets the outgoing call icon tint | .set(outgoingCallIconTint: UIColor) |
incomingCallIconTint | Sets the incoming call icon tint | .set(incomingCallIconTint: UIColor) |
emptyStateTextColor | Sets the empty state color | .set(emptyStateTextColor: UIColor) |
errorStateTextColor | Sets the error state color | .set(errorStateTextColor: UIColor) |
infoIconTint | Sets the info icon tint | .set(infoIconTint: UIColor) |
2. Avatar Styles
To apply customized styles to the Avatar
component in the CallLogs
Component, you can use the following code snippet. For further insights on Avatar
Styles refer
- Swift
// Creating AvatarStyle object
let avatarStyle = AvatarStyle()
.set(background: .red)
.set(textFont: .systemFont(ofSize: 18))
.set(textColor: .systemTeal)
.set(cornerRadius: CometChatCornerStyle(cornerRadius: 8.0))
.set(borderColor: .systemBlue)
.set(borderWidth: 5)
.set(outerViewWidth: 3)
.set(outerViewSpacing: 3)
let callLogsConfiguration = CallLogsConfiguration()
.set(avatarStyle: avatarStyle)
// To navigate to the CometChatCallLogs
let callLogs = CometChatCallLogs()
.set(configuration: callLogsConfiguration)
3. ListItem Styles
To apply customized styles to the ListItemStyle
component in the CallLogs
Component, you can use the following code snippet. For further insights on ListItemStyle
Styles refer
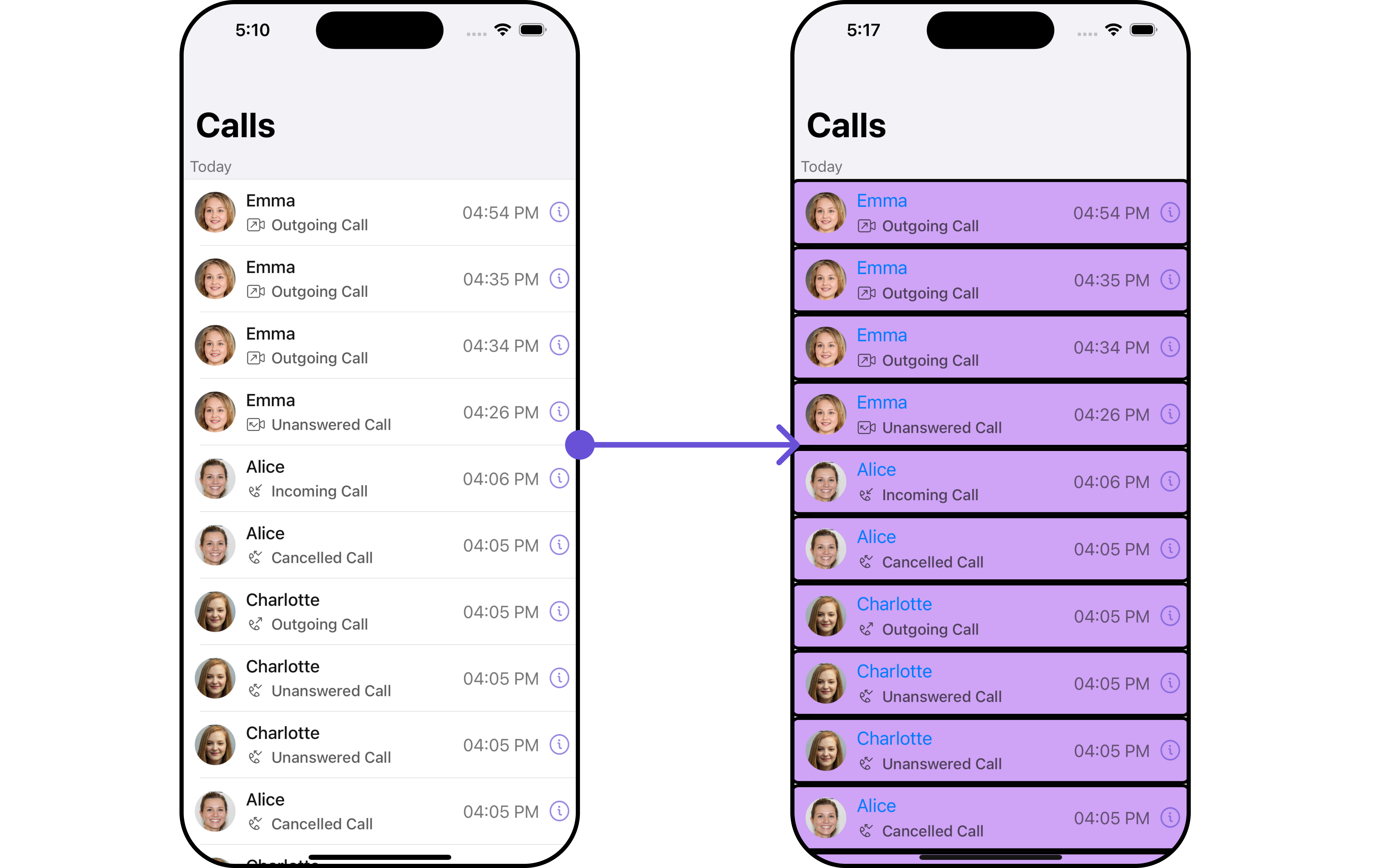
- Swift
let listItemStyle = ListItemStyle()
listItemStyle.set(background: .init(red: 0.81, green: 0.64, blue: 0.96, alpha: 1.00))
.set(titleFont: .systemFont(ofSize: 18))
.set(titleColor: .systemBlue)
.set(cornerRadius: CometChatCornerStyle(cornerRadius: 8.0))
.set(borderColor: .black)
.set(borderWidth: 3)
let callLogsConfiguration = CallLogsConfiguration()
.set(listItemStyle: listItemStyle)
// To navigate to the CometChatCallLogs
let callLogs = CometChatCallLogs()
.set(configuration: callLogsConfiguration)
Ensure to pass and present CometChatCallLogs
. If a navigation controller is already in use, utilize the pushViewController function instead of directly presenting the view controller.
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- Swift
// To navigate to the CometChatCallLogs
let callLogs = CometChatCallLogs()
.hide(search: true)
.hide(separator: true)
.set(backButtonTitle: "CometChat")
self.navigationController?.pushViewController(callLogs, animated: true)
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
backButtonFont | Sets the font for the back button | .set(backButtonFont: UIFont?) |
backButtonIcon | Sets the icon for the back button | .set(backButtonIcon: UIImage) |
backButtonTint | Sets the tint for the back button | .set(backButtonTint: UIColor) |
backButtonTitle | Sets the title for the back button | .set(backButtonTitle: String?) |
backButtonTitleColor | Sets the title color for the back button | .set(backButtonTitleColor: UIColor) |
background | Sets the background | .set(background: [CGColor]?) |
borderColor | Sets the border color | .set(borderColor: UIColor) |
borderWidth | Sets the border width | .set(borderWidth: CGFloat) |
corner | Sets the corner style | .set(corner: CometChatCornerStyle) |
emptyStateText | Sets the text for empty state | .set(emptyStateText: String) |
emptyStateTextFont | Sets the font for empty state text | .set(emptyStateTextFont: UIFont) |
errorStateText | Sets the text for error state | .set(errorStateText: String) |
errorStateTextColor | Sets the text color for error state | .set(errorStateTextColor: UIColor) |
errorStateTextFont | Sets the font for error state text | .set(errorStateTextFont: UIFont) |
largeTitleColor | Sets the color for large title | .set(largeTitleColor: UIColor) |
largeTitleFont | Sets the font for large title | .set(largeTitleFont: UIFont) |
searchBackground | Sets the background for the search bar | .set(searchBackground: UIColor) |
searchBarHeight | Sets the height for the search bar | .set(searchBarHeight: CGFloat) |
searchBorderColor | Sets the border color for the search bar | .set(searchBorderColor: UIColor) |
searchCancelButtonFont | Sets the font for the search cancel button | .set(searchCancelButtonFont: UIFont) |
searchCancelButtonTint | Sets the tint for the search cancel button | .set(searchCancelButtonTint: UIColor) |
searchClearIcon | Sets the icon for the search clear button | .set(searchClearIcon: UIImage) |
searchCornerRadius | Sets the corner radius for the search bar | .set(searchCornerRadius: CometChatCornerStyle) |
searchIcon | Sets the icon for the search bar | .set(searchIcon: UIImage?) |
searchPlaceholder | Sets the placeholder for the search bar | .set(searchPlaceholder: String) |
searchTextColor | Sets the color for the search text | .set(searchTextColor: UIColor) |
searchTextFont | Sets the font for the search text | .set(searchTextFont: UIFont) |
title | Sets the title for the title bar | .set(title: String, mode: UINavigationItem.LargeTitleDisplayMode) |
titleColor | Sets the color for the title | .set(titleColor: UIColor) |
titleFont | Sets the font for the title | .set(titleFont: UIFont) |
hide errorText | Hides the error text | .hide(errorText: Bool) |
hide search | Hides the search bar | .hide(search: Bool) |
hide separator | Hides the separator | .hide(separator: Bool) |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
EmptyView
You can set a custom EmptyView using .set(emptyView: UIView)
to match the empty view of your app.
- swift
let callLogs = CometChatCallLogs()
.set(emptyView: UIView) //you can pass your own view
Example
In this example, we will create a Custom_Empty_State_GroupView
a UIView file.
import UIKit
class CustomEmptyStateGroupView: UIView {
// Initialize your subviews
let imageView: UIImageView = {
let imageView = UIImageView(image: UIImage(named: "noDataImage"))
imageView.translatesAutoresizingMaskIntoConstraints = false
return imageView
}()
let messageLabel: UILabel = {
let label = UILabel()
label.text = "No groups available"
label.translatesAutoresizingMaskIntoConstraints = false
label.font = UIFont.boldSystemFont(ofSize: 16)
label.textColor = .black
return label
}()
// Override the initializer
override init(frame: CGRect) {
super.init(frame: frame)
// Add subviews and layout constraints
addSubview(imageView)
addSubview(messageLabel)
NSLayoutConstraint.activate([
imageView.centerXAnchor.constraint(equalTo: centerXAnchor),
imageView.centerYAnchor.constraint(equalTo: centerYAnchor),
imageView.heightAnchor.constraint(equalToConstant: 120),
imageView.widthAnchor.constraint(equalToConstant: 120),
messageLabel.topAnchor.constraint(equalTo: imageView.bottomAnchor, constant: 8),
messageLabel.centerXAnchor.constraint(equalTo: centerXAnchor)
])
}
required init?(coder: NSCoder) {
fatalError("init(coder:) has not been implemented")
}
}
We will be passing a custom empty view to CometChatCallLogs, ensuring a tailored and user-friendly interface.
- Swift
let customEmptyStateGroupView = CustomEmptyStateGroupView()
let callLogs = CometChatCallLogs()
.set(emptyView: customErrorStateGroupView)
self.navigationController?.pushViewController(callLogs, animated: true)
Ensure to pass and present CometChatCallLogs
. If a navigation controller is already in use, utilize the pushViewController function instead of directly presenting the view controller.
ErrorView
You can set a custom ErrorView using .set(errorView: UIView)
to match the error view of your app.
- Swift
let callLogs = CometChatCallLogs()
.set(errorView: UIView) //you can pass your own view
Example
In this example, we will create a UIView file Custom_ErrorState_GroupView
and pass it inside the .set(errorView: UIView)
method.
import UIKit
let CustomErrorStateGroupView: UIView = {
// Create main view
let view = UIView()
view.backgroundColor = .white
// Create an imageView and add it to the main view
let imageView = UIImageView(image: UIImage(systemName: "exclamationmark.triangle"))
imageView.tintColor = .red
imageView.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(imageView)
// Create a label with error message and add it to the main view
let label = UILabel()
label.text = "An error occurred. Please try again."
label.font = UIFont.systemFont(ofSize: 16)
label.textColor = .darkGray
label.numberOfLines = 0
label.textAlignment = .center
label.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(label)
// Create constraints for imageView and label
NSLayoutConstraint.activate([
imageView.centerXAnchor.constraint(equalTo: view.centerXAnchor),
imageView.centerYAnchor.constraint(equalTo: view.centerYAnchor, constant: -50),
label.topAnchor.constraint(equalTo: imageView.bottomAnchor, constant: 20),
label.leadingAnchor.constraint(equalTo: view.leadingAnchor, constant: 20),
label.trailingAnchor.constraint(equalTo: view.trailingAnchor, constant: -20)
])
return view
}()
- Swift
let customErrorStateGroupView = CustomErrorStateGroupView
// To navigate to the CometChatCallLogs
let callLogs = CometChatCallLogs()
.set(errorView: customErrorStateGroupView)
self.navigationController?.pushViewController(callLogs, animated: true)
Ensure to pass and present CometChatCallLogs
. If a navigation controller is already in use, utilize the pushViewController function instead of directly presenting the view controller.
Menus
You can set the Custom Menus to add more options to the CometChatCallLogs component.
- Swift
let callLogs = CometChatCallLogs()
.set(menus: [UIBarButtonItem])
- You can customize the menus for CometChatCallLogs to meet your requirements
Example
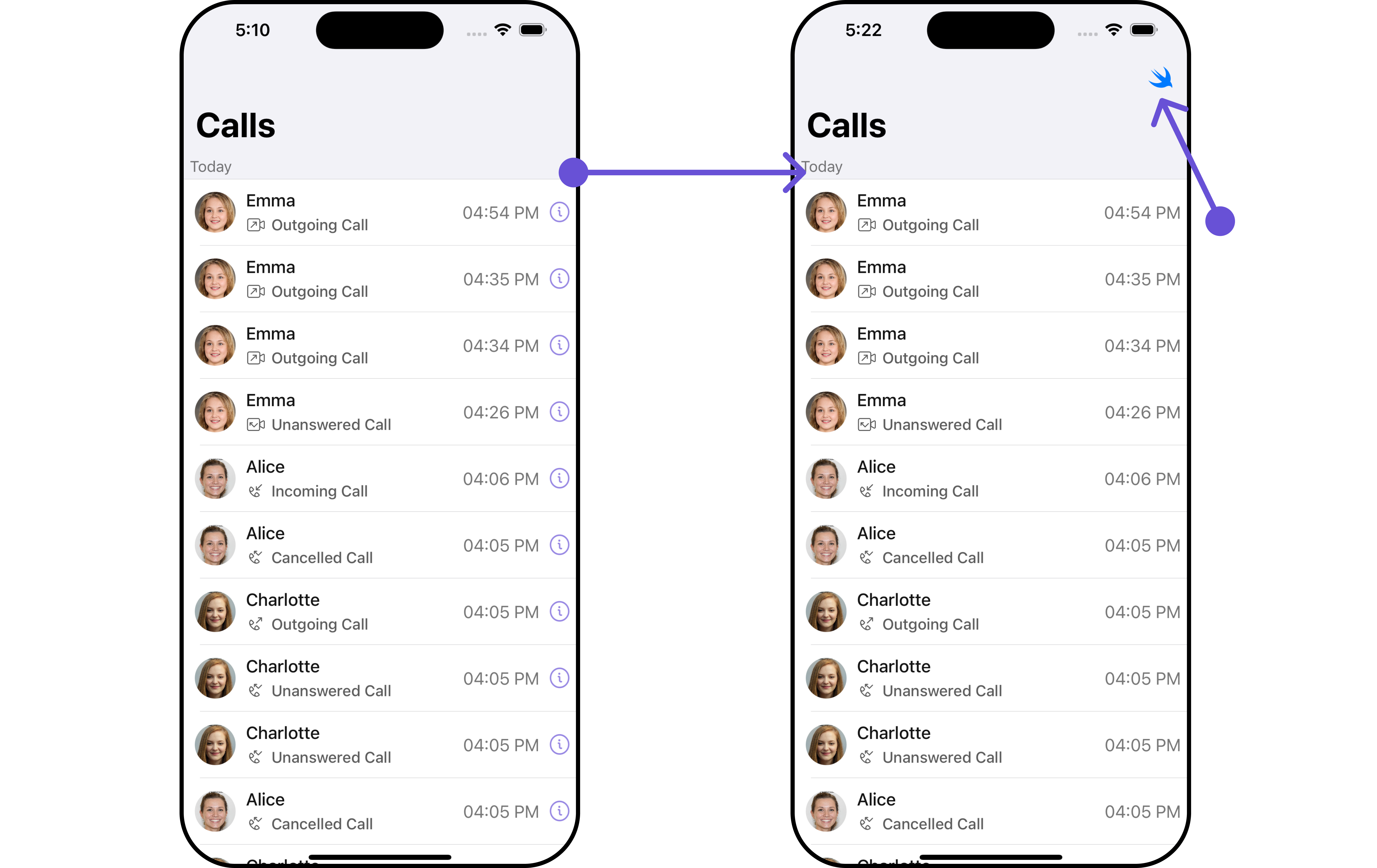
In this example, we'll craft a custom button tailored for CometChatCallLogs
, enhancing its interface with a personalized menu
for a more user-friendly experience.
- Swift
let customMenuButton: UIBarButtonItem = {
let button = UIButton(type: .system)
button.setImage(UIImage(systemName: "swift"), for: .normal)
button.setTitle("", for: .normal)
button.addTarget(self, action: #selector(handleCustomMenu), for: .touchUpInside)
let barButtonItem = UIBarButtonItem(customView: button)
return barButtonItem
}()
let callLogs = CometChatCallLogs()
.set(menus: [customMenuButton])
Ensure to pass and present CometChatCallLogs
. If a navigation controller is already in use, utilize the pushViewController function instead of directly presenting the view controller.