Getting Started
Start your first conversation
CometChat UI Kit for iOS is a package of pre-assembled UI elements crafted to streamline the creation of an in-app chat equipped with essential messaging functionalities. Our UI Kit presents options for light and dark themes, diverse fonts, colors, and extra customization capabilities.
CometChat UI Kit supports both one-to-one and group conversations. Follow the guide below to initiate conversations from scratch using CometChat iOS UI Kit.
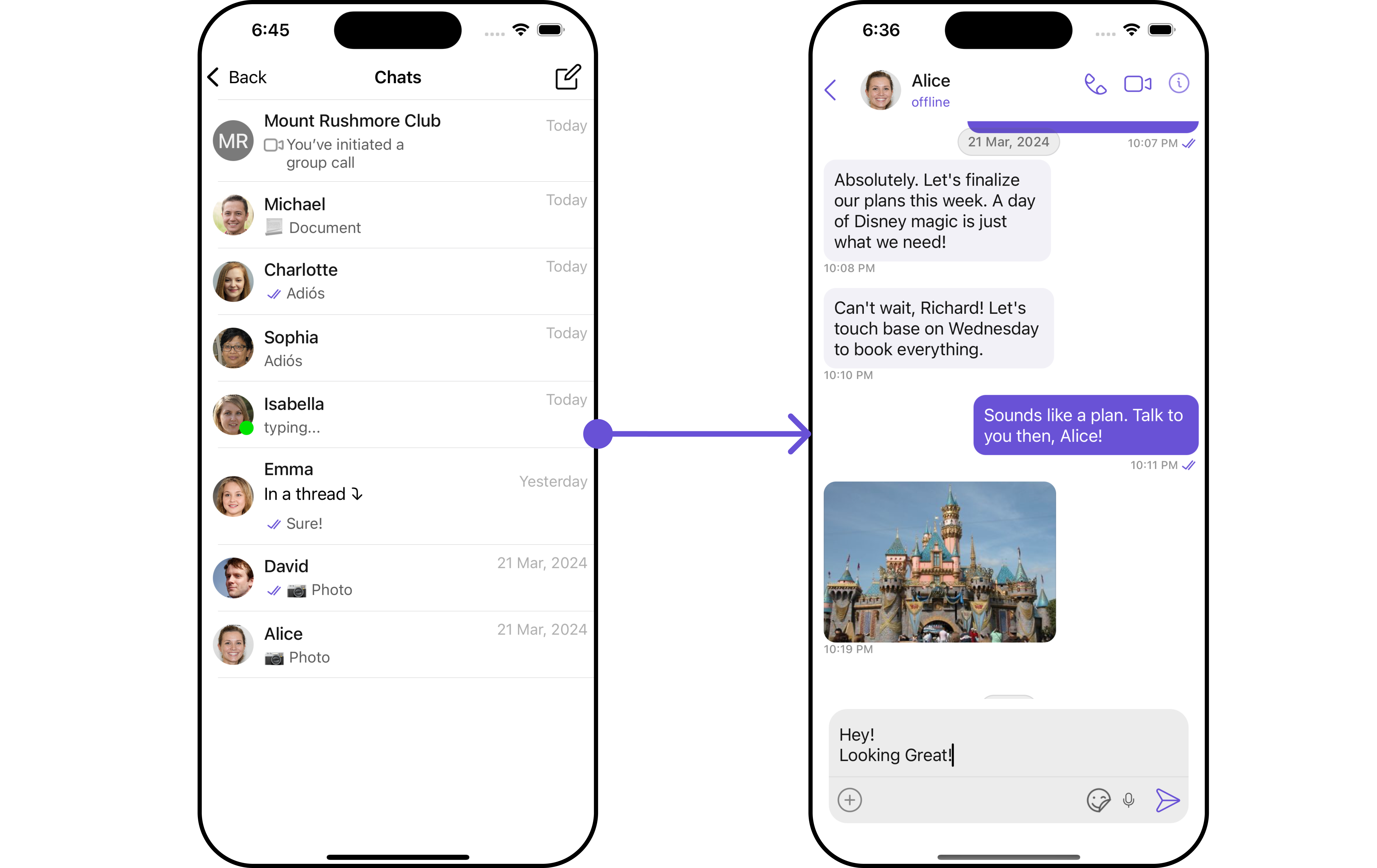
Prerequisites
Before installing the UI Kit, you need to create a CometChat application on the CometChat Dashboard, which includes all the necessary data for a chat service, such as users, groups, calls, and messages. You will require the App ID
, AuthKey
, and Region
of your CometChat application when initializing the SDK.
i. Register on CometChat
- You need to register on the CometChat Dashboard first. Click here to sign up.
ii. Get Your Application Keys
- Create a new app
- Head over to the QuickStart or API & Auth Keys section and note the App ID, Auth Key, and Region.
Each CometChat application can be integrated with a single client app. Within the same application, users can communicate with each other across all platforms, whether they are on mobile devices or on the web.
iii. IDE Setup
- You have Xcode installed on your machine.
- You have an iOS device or simulator with iOS version 13.0 or above.
- Swift 5.0.
- macOS.
Getting Started
Step 1Create a project
To get started, open Xcode and create a new project for UI Kit in the Project window as follows:
- Select iOS App in the Choose a template for your new project window and click Next.
- Enter your project name in the Name field in the Choose options for your new project window.
- Enter your identifier in the Bundle Identifier field in the Choose options for your new project window.
- Select Storyboard in the Interface field in the Choose options for your new project window.
- Select Swift in the Language field in the Choose options for your new project window.
Step 2
Add Dependency (Pods)
This developer kit is an add-on feature to CometChat iOS SDK so installing it will also install the core Chat SDK.
1. CocoaPods
We recommend using CocoaPods, as they are the most advanced way of managing iOS project dependencies. Open a terminal window, move to your project directory, and then create a Podfile by running the following command.
-
CometChatUIKitSwift supports installation through Cocoapods only. Currently, we are supporting Xcode 14 and higher.
-
CometChatUIKitSwift supports iOS 13 and above
$ pod init
Add the following lines to the Podfile.
platform :ios, '13.0'
use_frameworks!
target 'YourApp' do
pod 'CometChatUIKitSwift', '4.3.15'
end
And then install the CometChatUIKitSwift framework through CocoaPods.
$ pod install
If you're facing any issues while installing pods then use the below command.
$ pod install --repo-update
Always get the latest version of CometChatUIKitSwift by command.
$ pod update CometChatUIKitSwift
Always ensure to open the XCFramework file after adding the dependencies.
2. Swift Package Manager.
- Go to your Swift Package Manager's File tab and select Add Package Dependencies.
- Add
CometChatUIKitSwift
into yourPackage Repository
as below:
https://github.com/cometchat-pro/cometchat-chat-uikit-ios-swift.git
- To add the package, select Version Rules, enter Up to Exact Version, 4.3.15, and click Next.
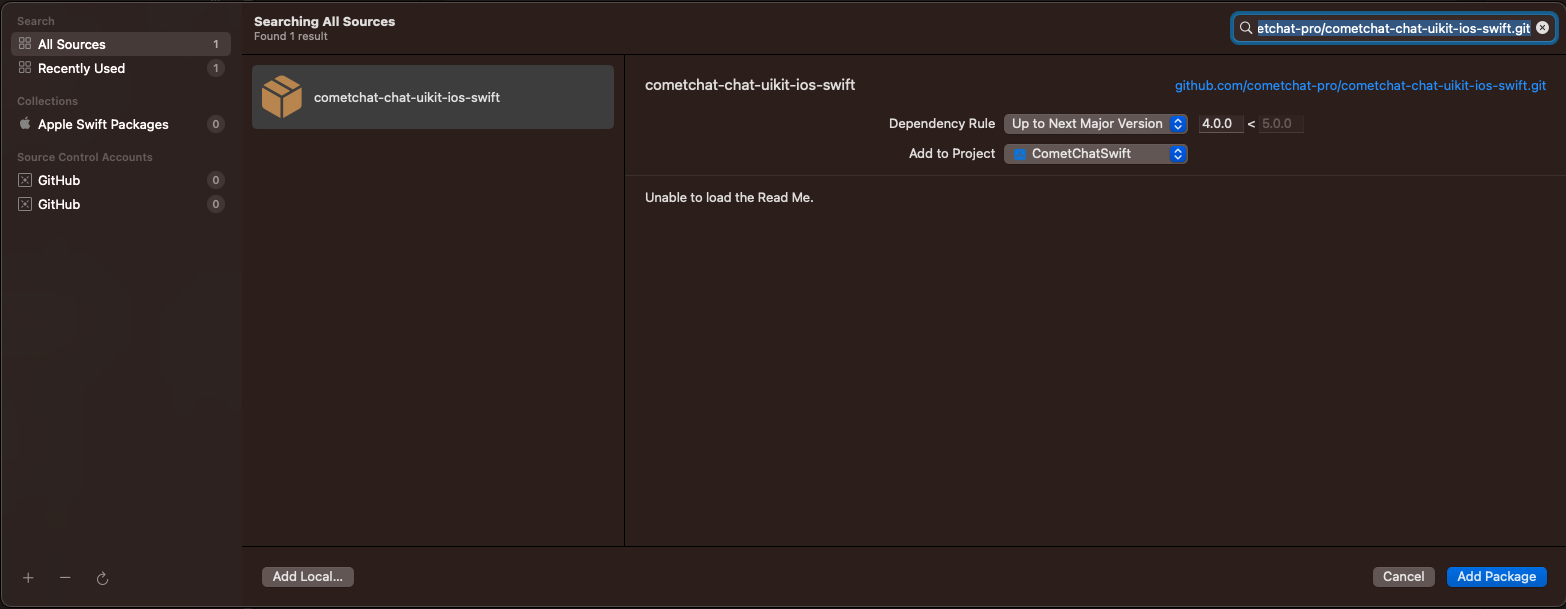
- Once, the package is added it will look like this.
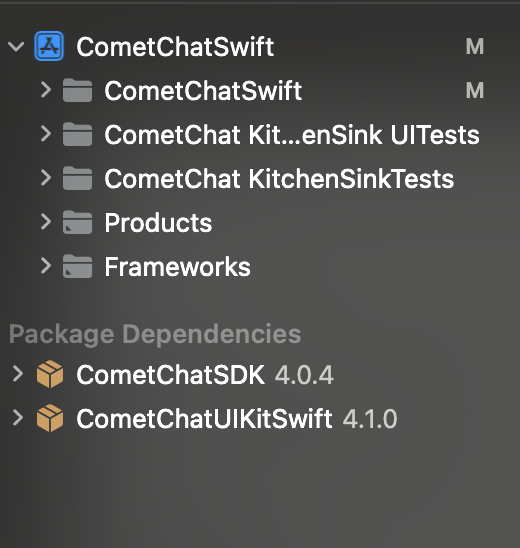
Step 3
Permissions
-
Allow Camera, Microphone and photo Library access in Info.plist
-
UI Kit provides the feature of media messages, so these settings are required.

- Navigate to your build settings.
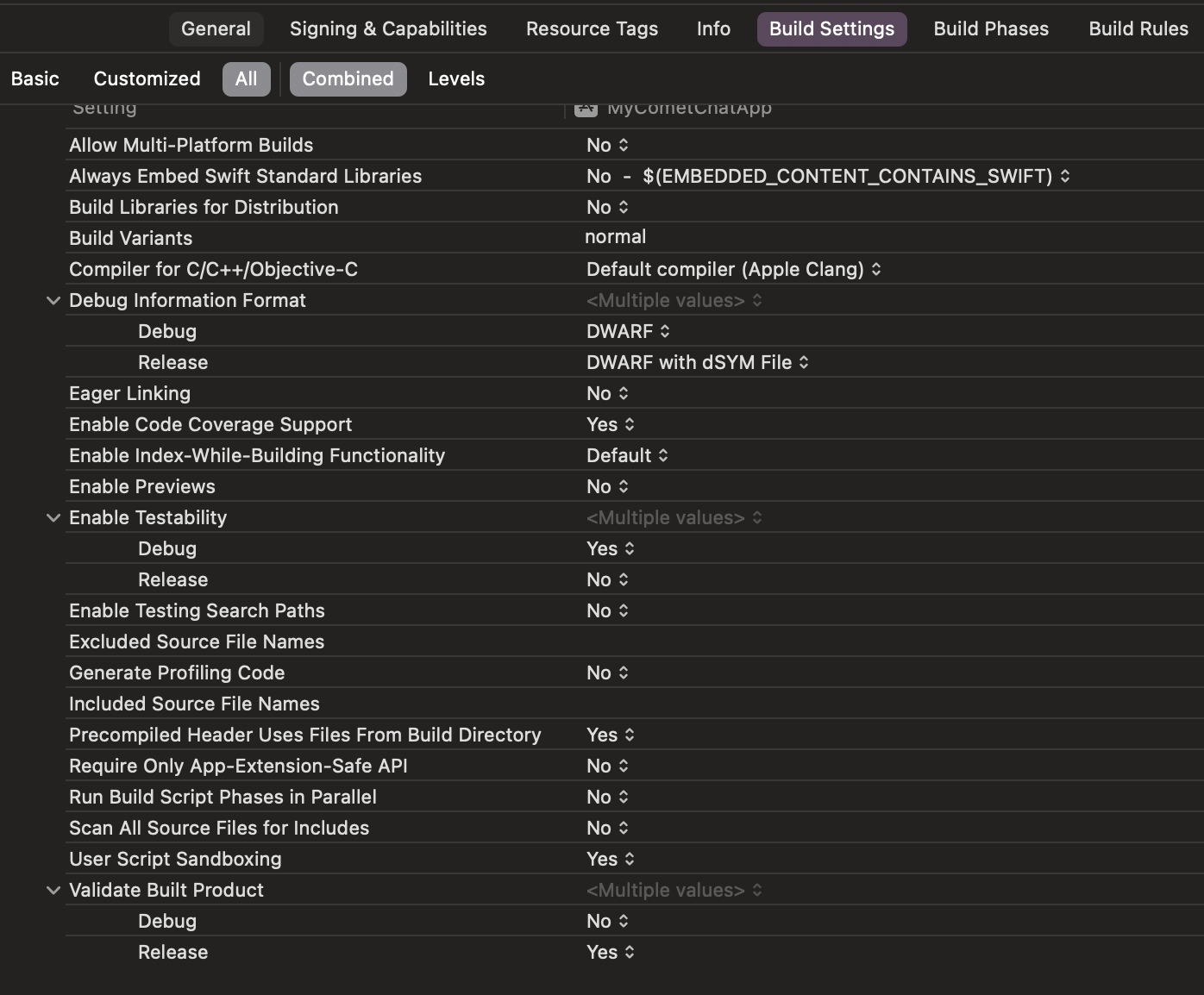
- Disable the User Script Sandboxing option.

Step 4
Initialize CometChatUIkit
To seamlessly integrate CometChat UI Kit into your app, initialize it using the init( )
method. This ensures all necessary settings are configured for CometChatUIKit.
It's crucial to call this method on app startup before using any other functions from CometChat UI Kit or CometChat SDK.
Initialize CometChatUIkit within your app delegate's didFinishLaunchingWithOptions method
.
The Auth Key is an optional property of the UIKitSettings
Class. It is intended for use primarily during proof-of-concept (POC) development or in the early stages of application development. You can use the Auth Token method to log in securely.
- Swift
import CometChatUIKitSwift
let uikitSettings = UIKitSettings()
uikitSettings.set(appID: <# Enter Your App ID Here #>)
.set(authKey: <# Enter Your AuthKey Here #>)
.set(region: <# Enter Your Region Code Here #> )
.subscribePresenceForAllUsers()
.build()
CometChatUIKit.init(uiKitSettings: uikitSettings, result: {
result in
switch result {
case.success(let success):
debugPrint("Initialization completed successfully \(success)")
break
case.failure(let error):
debugPrint( "Initialization failed with exception: \(error.localizedDescription)")
break
}
})
Make sure to replace the appId with your CometChat appId and region with your app region in the above code.
Step 5
Login User
For login, you require a UID
. You can create your own users on the CometChat Dashboard or via API. We have pre-generated test users: cometchat-uid-1
, cometchat-uid-2
, cometchat-uid-3
, cometchat-uid-4
, cometchat-uid-5
.
The Login method returns the User object containing all the information of the logged-in user.
This straightforward authentication method is ideal for proof-of-concept (POC) development or during the early stages of application development. For production environments, however, we strongly recommend using an Auth Token instead of an Auth Key to ensure enhanced security.
- Swift
let uid = <# Enter User's UID Here #>
CometChatUIKit.login(uid: uid) { result in
switch result {
case .success(let user):
debugPrint("User logged in successfully \(user.name)")
break
case .onError(let error):
debugPrint("Login failed with exception: \(error.errorDescription)")
break
}
}
Make sure you replace the authKey with your CometChat Auth Key in the above code.
Step 6
Render Conversation With Message
CometChatConversationsWithMessages is a wrapper component that offers functionality to render both the Conversations and Messages components. It also enables opening the Messages by tapping on any conversation rendered in the list of conversations.
Since CometChatConversationsWithMessages is a view controller, it can be launched either by a button click or through any event's trigger. It inherits all the customizable properties and methods of CometChatConversations.
View Controller: To use ConversationsWithMessages in your view controller
, use the following code snippet.
let cometChatConversationsWithMessages = CometChatConversationsWithMessages()
self.navigationController?.pushViewController(cometChatConversationsWithMessages, animated: true)
It will automatically fetch the conversation data upon loading the list. If the conversation list is empty, you can start a new conversation.
Scene Delegate: To use ConversationsWithMessages in your Navigation controller,
use the following code snippet in your willConnectTo
method in SceneDelegate.
- Swift
let cometChatConversationsWithMessages = CometChatConversationsWithMessages()
let naviVC = UINavigationController(rootViewController: cometChatConversationsWithMessages)
window?.rootViewController = naviVC
make sure to import CometChatUIKitSwift