Direct Calling
Overview
This guide demonstrates how to start One on One call in to an iOS application. Before you begin, we strongly recommend you read the calling setup guide.
Generate Token
To start the call you need callToken and sessionID. SessionID is something you can define to identify your call, it can be a random string or any thing that is defined by the user. For that SessionID you need to generate callToken using Calls SDK.
Generate token method takes two parameter authToken
and sessionId
.
authToken
: User can get the auth token from CometChatSDK that has a static method getUsersAuthToken().
sessionId
: Any random string.
- Swift
// jwtToken requires in the 3rd step. so when user get from the generate token
// store in jwtToken variable.
var callToken: String?
CometChatCalls.generateToken(authToken: authToken as NSString, sessionID: sessionId) { token in
self.callToken = token
} onError: { error in
print("CometChatCalls generateToken error: \(String(describing: error?.errorDescription))")
}
Parameter | Description |
---|---|
sessionId | The unique random session ID. In case you are using default call then session ID is available in the Call object. |
userAuthToken | The use auth token is logged in user auth token which you can get by calling CometChat Chat SDK method CometChat.getUserAuthToken() |
Error Code | Error Message |
---|---|
INVALID_ARGUMENT_EXCEPTION | If you pass invalid param to a function or you don't pass the required params. example: Authtoken and session are required, Invalid callToken passed, please verify. |
Call Settings
Next you have to create your own CallSettingsBuilder by defining the properties you need in that call.
The CallSettings
class is the most important class when it comes to the implementation of the Calling feature. This is the class that allows user to customize the overall calling experience. The properties for the call/conference can be set using the CallSettingsBuilder
class. This will eventually give user an object of the CallSettings
class which user can pass to the startCall()
method to start the call.
The mandatory parameters that are required to be present for any call/conference to work are:
- UIView i.e a view in which user want to show the calling view inside it.
- Video Settings.
CallSettingsBuilder | ||
---|---|---|
Properties | Values | Description |
setDefaultLayout(Bool) | true false | If set to true, enables the default layout for handling the call operations. if set to false it hides the button layout and just displays the CallView default value = true |
setIsAudioOnly(Bool) | true false | If set to true, the call will be strictly an audio call. If ser to false, the call will be an audio-video call. Default value = false |
setIsSingleMode(Bool) | true false | |
setShowSwitchToVideoCall(Bool) | true false | If set to true it displays the SwitchCameraButton in Button Layout. if set to false it hides the SwitchCameraButton in Button Layout |
setEnableVideoTileClick(Bool) | true false | if set to true, video tile is clickable otherwise not. |
setEnableDraggableVideoTile(Bool) | true false | if set to true, video tile can draggable, otherwise not. this is only work in SPOTLIGHT Mode. |
setEndCallButtonDisable(Bool) | true false | If set to true it displays the EndCallButton in Button Layout. if set to false it hides the EndCallButton in Button Layout Default value = true |
setShowRecordingButton(Bool) | true false | If set to true it displays the ShowRecordingButton in Button Layout. if set to false it hides the ShowRecordingButton in Button Layout Default value = true |
setSwitchCameraButtonDisable(Bool) | true false | If set to true it displays the SwitchCameraButton in Button Layout. if set to false it hides the SwitchCameraButton in Button Layout Default value = true |
setMuteAudioButtonDisable(Bool) | true false | If set to true it displays the MuteAudioButton in Button Layout. if set to false it hides the MuteAudioButton in Button Layout |
setPauseVideoButtonDisable(Bool) | true false | If set to true it displays the PauseVideoButton in Button Layout. if set to false it hides the PauseVideoButton in Button Layout Default value = true |
setAudioModeButtonDisable(Bool) | true false | If set to true it displays the AudioModeButton in Button Layout. if set to false it hides the AudioModeButton in Button Layout Default value = true |
setStartAudioMuted(Bool) | true false | This ensures the call is started with the audio muted if set to true |
setIdleTimeoutPeriod(Int) Available since v4.1.1 | Int (120 or more) | Sets the idle timeout duration for the call. If the user remains the only participant for the specified time, a prompt will appear 60 seconds before the call is automatically ended. Default value = 180 seconds |
setMode(String) | DEFAULT SINGLESPOTLIGHT | Currently, you can set 3 modes. DEFUALT SINGLE SPOTLIGHT default value = DEFUALT |
setView(UIView) | UIView | it is view in which call screen will load. |
setDefaultAudioMode("BLUETOOTH") | SPEAKER BLUETOOTHHEADPHONES EARPIECE`` | This method can be used if you wish to start the call with a specific audio mode. The available options are SPEAKEREARPIECE BLUETOOTH HEADPHONES |
setDelegate(self) | self | pass the reference of the UIViewController where the CallsEventsDelegate will conform. |
setMode(.spotlight) Available since v4.1.2 | DisplayModes [.default, .single, .spotlight] | Currently, you can set 3 modes. default single spotlight default value = DEFUALT |
setDefaultAudioMode(mode: AudioMode(mode: "SPEAKER")) Available since v4.1.2 | SPEAKER BLUETOOTHHEADPHONES EARPIECE`` | This method can be used if you wish to start the call with a specific audio mode. The available options are SPEAKEREARPIECE BLUETOOTH HEADPHONES |
setAvatarMode(.fullscreen) Available since v4.1.2 | AvatarMode [.circle, .square, .fullscreen] | Currently, you can set 3 avatar modes. circle square fullscreen default value = .circle |
- Swift
// In the callSettings, User have to configure the callingView.
// callSettings is required to start call.
callSettings = CometChatCalls.CallSettingsBuilder
.setDefaultLayout(true)
.setIsAudioOnly(false)
.setIsSingleMode(false)
.setShowSwitchToVideoCall(false)
.setEnableVideoTileClick(true)
.setEnableDraggableVideoTile(true)
.setEndCallButtonDisable(false)
.setShowRecordingButton(true)
.setSwitchCameraButtonDisable(false)
.setMuteAudioButtonDisable(false)
.setPauseVideoButtonDisable(false)
.setAudioModeButtonDisable(false)
.setStartAudioMuted(true)
.setStartVideoMuted(false)
.setMode(.default)
.setAvatarMode(.fullscreen)
.setDefaultAudioMode(mode: AudioMode(mode: "SPEAKER"))
.setDelegate(self)
.build()
Start Call Session
To start a call, user have to pass callToken
, callSettings
and callView
.
callToken
In the success block of generateToken()
, user get the callToken.
callSettings
CallSettings
can be set from the CallSettingsBuilder
callView
callView is a UIView in which you want to display the call UI
- Swift
guard let callToken = self.callToken, let callSettings = self.callSettings, let view = callView else { return }
CometChatCalls.startSession(callToken: callToken, callSetting: callSettings, view: view) { success in
print("CometChatCalls startSession success: \(success)")
} onError: { error in
print("CometChatCalls startSession error: \(String(describing: error?.errorDescription))")
}
Error Code | Error Message |
---|---|
INVALID_PROP_EXCEPTION | If you pass an invalid prop to a functional component or you don't pass the required prop. example: Component requires valid call settings |
API_ERROR | If there is some error thrown by the API server For example unauthorized |
UNKNOWN_API_ERROR | If there is some API error but it didn't come from the server. For example, if the internet is not available and API is called. |
AUTH_ERR_TOKEN_INVALID_SIGNATURE | if Token is invalid |
End Call Session
To release the acquired calling resource and end the call, the end call session must be called.
For Default Call
You only need to call the end call method of the chat SDK for default calling flows. Direct calling flows do not require it.
To end a call in the default call flow, you must call the CometChat.endCall()
method, which belongs to the CometChat Chat SDK, and the CometChatCalls.endSession()
method, which belongs to the CometChat Calls SDK.
The user who pressed the end call button will call the CometChat.endCall()
method, and the another user how was on call will call the two methods which is CometChat.clearActiveCall() and CometChatCalls.endSession()
methods to release the calling resources
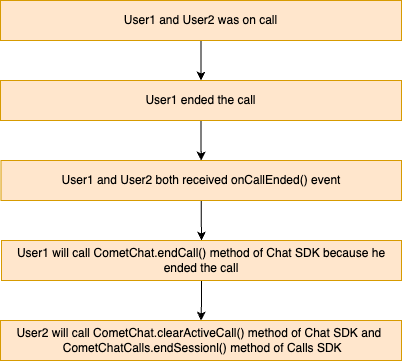
The user who ended the call
- Swift
CometChat.endCall(sessionID: sessionID) { call in
print("End call success")
} onError: { error in
print("End call failed with error: \(error)")
}
The another user who was on call
- Swift
CometChat.clearActiveCall()
CometChatCalls.endSession()
For Direct Call
- Swift
CometChatCalls.endSession(sessionId);
Calling Events Listners
To get calling events, User have to conform the “CallsEventsDelegate” delegate. For these events working, User have to pass “self” to setDelegate() in callSettingsBuilder.
Listener (CallEventsDelegate) | |
---|---|
Listener | Description |
onCallEnded() | This method is called when the call is successfully ended. |
onSessionTimeout() Available since v4.1.1 | This method is called when the call is ended due to session timeout. |
onCallEndButtonPressed() | This method is called when the end call button press. |
onUserJoined(user: NSDictionary) | This method is called when any other user joins the call. The user details can be obtained from the user as parameter. |
onUserLeft(user: NSDictionary) | This method is called when a user leaves the call. The details of the user can be obtained from the provided user as parameter. |
onUserListChanged(userList: NSArray) | This method is triggered when user list changes. |
onAudioModeChanged(audioModeList: NSArray) | This method is triggered when audio mode changes. |
onCallSwitchedToVideo(info: NSDictionary) | This callback is triggered when an audio call is converted into a video call. you will get the information in info as parameter. |
onUserMuted(info: NSDictionary) | This method is triggered when a user is muted in the ongoing call. |
onRecordingToggled(info: NSDictionary) | This method is triggered when a user toggles recording. |
- Swift
// These events will trigger only if user set the setDelegate(self) in callSettings.
extension ViewController: CallsEventsDelegate {
func onCallEnded() {
print("onCallEnded")
}
func onSessionTimeout() {
print("onSessionTimeout")
}
func onCallEndButtonPressed() {
print("onCallEndButtonPressed")
}
func onUserJoined(rtcUser: CometChatCallsSDK.RTCUser) {
print("onUserJoined(rtcUser >>>: ", rtcUser.name, rtcUser.avatar, rtcUser.uid, rtcUser.resource)
}
func onUserLeft(rtcUser: CometChatCallsSDK.RTCUser) {
print("onUserLeft(rtcUser >>>: ", rtcUser.name, rtcUser.avatar, rtcUser.uid, rtcUser.resource)
}
func onUserListChanged(rtcUsers: [CometChatCallsSDK.RTCUser]) {
rtcUsers.forEach { rtcUser in
print("onUserListChanged(rtcUsers >>>: ", rtcUser.name, rtcUser.avatar, rtcUser.uid, rtcUser.resource)
}
}
func onAudioModeChanged(mode: [CometChatCallsSDK.AudioMode]) {
mode.forEach { mode in
print("onAudioModeChanged(mode >>>: ", mode.mode, mode.isSelected)
}
}
func onUserMuted(rtcMutedUser: CometChatCallsSDK.RTCMutedUser) {
print("onUserMuted(rtcMutedUser >>>: ", rtcMutedUser.muted.name, rtcMutedUser.mutedBy.name)
}
func onRecordingToggled(recordingInfo: CometChatCallsSDK.RTCRecordingInfo) {
print("onRecordingToggled(recordingInfo >>>: ", recordingInfo.recordingStarted, recordingInfo.user.name)
}
func onCallSwitchedToVideo(callSwitchedInfo: CometChatCallsSDK.CallSwitchRequestInfo) {
print("onCallSwitchedToVideo(callSwitchedInfo >>>: ", callSwitchedInfo.sessionId, callSwitchedInfo.requestAcceptedBy.name, callSwitchedInfo.requestInitiatedBy.name)
}
}
- Before v4.1.2: Events were returned as
NSDictionary
objects with key-value pairs - From v4.1.2 onwards: Events are returned as structured objects with defined properties and enumerated types
MultiListener For Call Events
Through Multi Listener you can get Inn-call events in any class that accepts CallsEventsDelegate
Add listener for a calls
- Swift
let observerId = "" //unique ID that is going to be needed for removing CallEventListener
CometChatCalls.addCallEventListener(observerId: observerId, delegate: self) //self must conform to CallsEventsDelegate
Remove listener for a calls
- Swift
CometChatCalls.removeCallEventListener(observerId: observerId)
On removing the listener, user will not get callbacks for call events for that particular unique ID associated class
Custom Events
In case user wish to achieve a completely customized UI for the Calling experience, user can do so by embedding default iOS buttons to the screen as per requirements and then use the below methods to achieve different functionalities for the embedded buttons.
For the use case where user wish to align own custom buttons and not use the default layout provided by CometChat, user can embed the buttons in the layout and use the below methods to perform the corresponding operations:
Switch Camera
User can end the call by using the CometChatCalls.switchCamera()
- Swift
- Objective C
// To switch Camera from front to rear and vice versa.
// To switch Camera from front to rear and vice versa.
CometChatCalls.switchCamera()
// To switch Camera from front to rear and vice versa.
[CometChatCalls switchCamera];
Audio Muted
User can end the call by using the audioMuted(true)
- Swift
- Objective C
// To mute audio
CometChatCalls.audioMuted(true)
// To mute audio
[CometChatCalls audioMuted:YES];
Audio Unmute
User can end the call by using the audioMuted(false)
- Swift
- Objective C
// To umMute audio
CometChatCalls.audioMuted(false)
// To umMute audio
[CometChatCalls audioMuted:NO];
Pause Video
User can end the call by using the videoPaused(true)
- Swift
- Objective C
// To paused video
CometChatCalls.videoPaused(true)
// To paused video
[CometChatCalls videoPaused:YES];
Unpause Video
User can end the call by using the videoPaused(false)
- Swift
- Objective C
// To unpaused video
CometChatCalls.videoPaused(false)
// To unpaused video
[CometChatCalls videoPaused:NO];
Set Audio Mode
User can end the call by using the setAudioMode(mode: "**_**MODE**_**")
- Swift
- Objective C
// To set audio mode.
CometChatCalls.setAudioMode(mode: "DEFAULT")
// To set audio mode.
[CometChatCalls setAudioModeWithMode:@"DEFAULT"];
- Swift
- Objective C
CometChatCalls.setAudioMode(AudioMode(mode: "SPEAKER"))
// To set audio mode.
[CometChatCalls setAudioModeWithMode:@"DEFAULT"];
Enter PIP Mode
User can end the call by using the enterPIPMode()
- Swift
- Objective C
// To enter PIP mode.
CometChatCalls.enterPIPMode()
// To enter PIP mode.
[CometChatCalls enterPIPMode];
Exit PIP Mode
User can end the call by using the exitPIPMode()
- Swift
- Objective C
// To exit PIP mode.
CometChatCalls.exitPIPMode()
// To exit PIP mode.
[CometChatCalls exitPIPMode];
SwitchToVideoCall
User can end the call by using the switchToVideoCall()
- Swift
- Objective C
// To switch video call.
CometChatCalls.switchToVideoCall()
// To switch video call.
[CometChatCalls switchToVideoCall];
Strat Recording
User can end the call by using the startRecording()
- Swift
- Objective C
// To start recording.
CometChatCalls.startRecording()
// To start recording.
[CometChatCalls startRecording];
Stop Recording
User can end the call by using the stopRecording()
- Swift
- Objective C
// To stop recording.
CometChatCalls.stopRecording()
// To stop recording.
[CometChatCalls stopRecording];