Message Composer
Overview
MessageComposer is a Component that enables users to write and send a variety of messages, including text, image, video, and custom messages.
Features such as Attachments, and Message Editing are also supported by it.
Usage
Integration
The following code snippet illustrates how you can directly incorporate the MessageComposer component into your app.
- MessageComposerDemo.tsx
- App.tsx
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatMessageComposer } from "@cometchat/chat-uikit-react";
export function MessageComposerDemo() {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
return chatUser ? (
<div>
<CometChatMessageComposer user={chatUser} />
</div>
) : null;
}
import { MessageComposerDemo } from "./MessageComposerDemo";
export default function App() {
return (
<div className="App">
<div>
<MessageComposerDemo />
</div>
</div>
);
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. OnSendButtonClick
The OnSendButtonClick
event gets activated when the send message button is clicked. It has a predefined function of sending messages entered in the composer EditText
. However, you can overide this action with the following code snippet.
- MessageComposerDemo.tsx
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatMessageComposer } from "@cometchat/chat-uikit-react";
export function MessageComposerDemo() {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
function handleSendButtonClick(message: CometChat.BaseMessage): void {
console.log("your custom on send buttonclick action");
}
return chatUser ? (
<div>
<CometChatMessageComposer onSendButtonClick={handleSendButtonClick} />
</div>
) : null;
}
2. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the MessageList component.
- MessageComposerDemo.tsx
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatMessageComposer } from "@cometchat/chat-uikit-react";
export function MessageComposerDemo() {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
function handleError(error: CometChat.CometChatException) {
throw new Error("your custom error action");
}
return chatUser ? (
<div>
<CometChatMessageComposer user={chatUser} onError={handleError} />
</div>
) : null;
}
3. onTextChange
This event is triggered as the user starts typing in the Message Composer.
- MessageComposerDemo.tsx
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatMessageComposer } from "@cometchat/chat-uikit-react";
export function MessageComposerDemo() {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
function handleTextChange(text: string) {
console.log("onTextChange", text);
}
return chatUser ? (
<div>
<CometChatMessageComposer
user={chatUser}
onTextChange={handleTextChange}
/>
</div>
) : null;
}
Filters
MessageComposer component does not have any available filters.
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The list of events emitted by the Messages component is as follows.
Event | Description |
---|---|
ccMessageEdited | Triggers whenever a loggedIn user edits any message from the list of messages .it will have three states such as: inProgress, success and error. |
ccMessageSent | Triggers whenever a loggedIn user sends any message, it will have three states such as: inProgress, success and error. |
Adding CometChatMessageEvents
Listener's
- JavaScript
import { CometChatMessageEvents } from "@cometchat/chat-uikit-react";
const ccMessageEdited = CometChatMessageEvents.ccMessageEdited.subscribe(() => {
// Your Code
});
const ccMessageSent = CometChatMessageEvents.ccMessageSent.subscribe(() => {
// Your Code
});
Removing CometChatMessageEvents
Listener's
- JavaScript
ccMessageEdited?.unsubscribe();
ccMessageSent?.unsubscribe();
Customization
To fit your app's design requirements, you can customize the appearance of the MessageComposer component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
To modify the styling, you can customise the css of MessageComposer Component.
Example
- MessageComposerDemo.tsx
- MessageComposerDemo.css
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatMessageComposer } from "@cometchat/chat-uikit-react";
<CometChatMessageComposer user={chatUser} />;
.cometchat-message-composer .cometchat-message-composer__input {
font-family: "SF Pro";
}
.cometchat-message-composer .cometchat-button .cometchat-button__icon {
background: #f19fa1;
}
.cometchat-message-composer .cometchat-message-composer__send-button {
background: #e5484d;
}
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- MessageComposerDemo.tsx
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatMessageComposer } from "@cometchat/chat-uikit-react";
<CometChatMessageComposer user={chatUser} disableTypingEvents={true} />;
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
Initial Composer Text | The initial text pre-filled in the message input when the component mounts. | initialComposerText="Hello" |
Disable Typing Events | Disables the typing indicator for the current message composer. | disableTypingEvents={true} |
Disable Mentions | Disables the mentions functionality in the message composer. | disableMentions={true} |
Hide Image Attachment Option | Hides the image attachment option in the message composer. | hideImageAttachmentOption={true} |
Hide Video Attachment Option | Hides the video attachment option in the message composer. | hideVideoAttachmentOption={true} |
Hide Audio Attachment Option | Hides the audio attachment option in the message composer. | hideAudioAttachmentOption={true} |
Hide File Attachment Option | Hides the file attachment option in the message composer. | hideFileAttachmentOption={true} |
Hide Polls Option | Hides the polls option in the message composer. | hidePollsOption={true} |
Hide Collaborative Document | Hides the collaborative document option in the message composer. | hideCollaborativeDocumentOption={true} |
Hide Collaborative Whiteboard | Hides the collaborative whiteboard option in the message composer. | hideCollaborativeWhiteboardOption={true} |
Hide Attachment Button | Hides the attachment button in the message composer. | hideAttachmentButton={true} |
Hide Voice Recording Button | Hides the voice recording button in the message composer. | hideVoiceRecordingButton={true} |
Hide Emoji Keyboard Button | Hides the emoji keyboard button in the message composer. | hideEmojiKeyboardButton={true} |
Hide Stickers Button | Hides the stickers button in the message composer. | hideStickersButton={true} |
Hide Send Button | Hides the send button in the message composer. | hideSendButton={true} |
User | Specifies the recipient of the message (user object). | user={chatUser} |
Group | Specifies the group to send messages to. Used if the user prop is not provided. | group={chatGroup} |
Parent Message ID | Specifies the ID of the parent message for threading or replying to a specific message. | parentMessageId={12345} |
Enter Key Behavior | Determines the behavior of the Enter key (e.g., send message or add a new line). | enterKeyBehavior={EnterKeyBehavior.SendMessage} |
Disable Sound for Message | Disables sound for incoming messages. | disableSoundForMessage={true} |
Custom Sound for Message | Specifies a custom audio sound for incoming messages. | customSoundForMessage="sound.mp3" |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
AttachmentOptions
By using attachmentOptions
, you can set a list of custom MessageComposerActions
for the MessageComposer Component. This will override the existing list of MessageComposerActions
.
Shown below is the default chat interface.
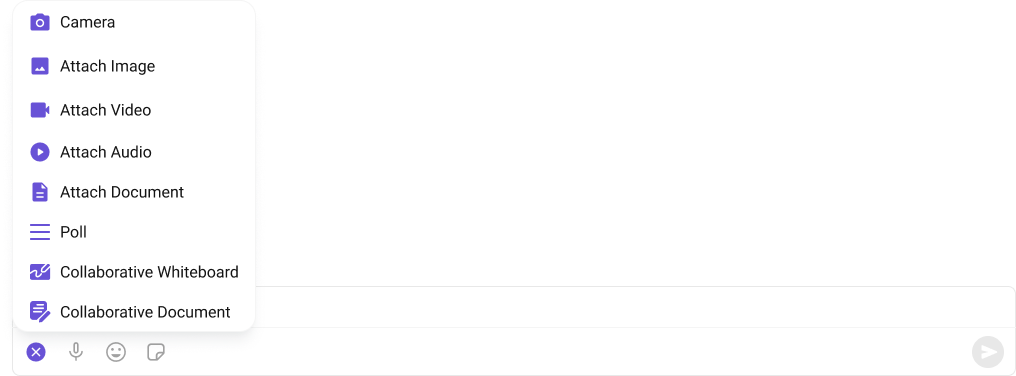
The customized chat interface is displayed below.
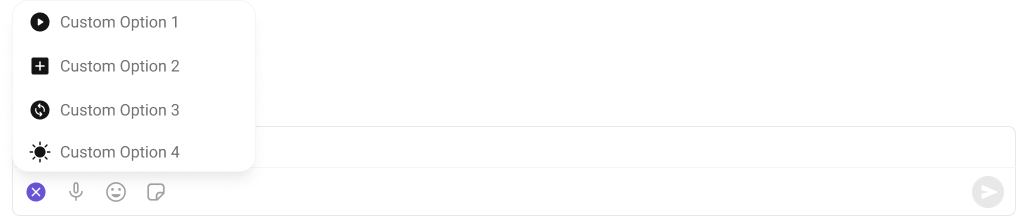
Use the following code to achieve the customization shown above.
- MessageComposerDemo.tsx
- MessageComposerDemo.css
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatMessageComposer,
CometChatMessageComposerAction,
} from "@cometchat/chat-uikit-react";
function getAttachments() {
return [
new CometChatMessageComposerAction({
id: "custom1",
title: "Custom Option 1",
iconURL: "Icon URL",
}),
new CometChatMessageComposerAction({
id: "custom2",
title: "Custom Option 2",
iconURL: "Icon URL",
}),
new CometChatMessageComposerAction({
id: "custom3",
title: "Custom Option 3",
iconURL: "Icon URL",
}),
new CometChatMessageComposerAction({
id: "custom4",
title: "Custom Option 4",
iconURL: "Icon URL",
}),
];
}
<CometChatMessageComposer
attachmentOptions={getAttachments()}
user={userObj}
/>;
.cometchat-message-composer__secondary-button-view-attachment-button
.cometchat-action-sheet {
border: none;
border-radius: inherit;
background: transparent;
box-shadow: none;
width: 100%;
}
.cometchat-message-composer__secondary-button-view-attachment-button
.cometchat-popover__content
> .cometchat {
border-radius: inherit;
}
.cometchat-message-composer__secondary-button-view-attachment-button
.cometchat-popover__content {
height: 240px;
}
.cometchat-message-composer__secondary-button-view-attachment-button
.cometchat-action-sheet__item-icon {
background: #141414;
}
AuxiliaryButtonView
You can insert a custom view into the MessageComposer component to add additional functionality using the following method.
Please note that the MessageComposer Component utilizes the AuxiliaryButton to provide sticker and AI functionality. Overriding the AuxiliaryButton will subsequently replace the sticker functionality.
Shown below is the default chat interface.
The customized chat interface is displayed below.
Use the following code to achieve the customization shown above.
- MessageComposerDemo.tsx
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatMessageComposer,
IconStyle,
CometChatButton,
} from "@cometchat/chat-uikit-react";
const auxiliaryButtonView = (
<CometChatButton
iconURL="Icon URL"
onClick={() => {
// logic here
}}
/>
);
<CometChatMessageComposer
auxiliaryButtonView={auxiliaryButtonView}
user={userObj}
/>;
SendButtonView
You can set a custom view in place of the already existing send button view.
Shown below is the default chat interface.
The customized chat interface is displayed below.
Use the following code to achieve the customization shown above.
- MessageComposerDemo.tsx
- MessageComposerDemo.css
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatMessageComposer,
CometChatButton,
} from "@cometchat/chat-uikit-react";
const sendButtonView = (
<CometChatButton
iconURL="Icon URL"
onClick={() => {
// logic here
}}
/>
);
<CometChatMessageComposer sendButtonView={sendButtonView} user={userObj} />;
.cometchat-message-composer
div:not([class])
.message-composer__send-button
.cometchat-button {
background: #edeafa;
}
.cometchat-message-composer
div:not([class])
.message-composer__send-button
.cometchat-button__icon {
background: #6852d6;
}
HeaderView
You can set custom headerView to the MessageComposer component using the following method.
Shown below is the default chat interface.
The customized chat interface is displayed below.
Use the following code to achieve the customization shown above.
- MessageComposerDemo.tsx
- MessageComposerDemo.css
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatMessageComposer } from "@cometchat/chat-uikit-react";
const getHeaderView = () => {
return (
<div className="message-composer__header-view">
<div className="message-composer__header-view-icon"></div>
<div className="message-composer__header-view-text">
User has paused their notifications
</div>
</div>
);
};
<CometChatMessageComposer user={chatUser} headerView={getHeaderView()} />;
.cometchat-message-composer .message-composer__header-view {
display: flex;
align-items: center;
align-content: center;
gap: 8px var(--cometchat-padding-2, 8px);
align-self: stretch;
flex-wrap: wrap;
width: 100%;
}
.cometchat-message-composer__header {
background: #dcd7f6;
border-radius: var(--cometchat-radius-ax, 1000px);
padding: var(--cometchat-padding-2, 8px) var(--cometchat-padding-5, 20px);
}
.cometchat-message-composer
.message-composer__header-view
.message-composer__header-view-text {
overflow: hidden;
color: var(--cometchat-text-color-primary, #141414);
text-overflow: ellipsis;
font: var(--cometchat-font-body-regular);
}
.cometchat-message-composer
.message-composer__header-view
.message-composer__header-view-icon {
display: flex;
width: 24px;
height: 24px;
justify-content: center;
align-items: center;
background: var(--cometchat-primary-color);
-webkit-mask: url("icon url") center center no-repeat;
-webkit-mask-size: contain;
mask: url("icon url") center center no-repeat;
mask-size: contain;
}
TextFormatters
Assigns the list of text formatters. If the provided list is not null, it sets the list. Otherwise, it assigns the default text formatters retrieved from the data source. To configure the existing Mentions look and feel check out CometChatMentionsFormatter
- ShortCutFormatter.ts
- Dialog.tsx
- MessageComposerDemo.tsx
import { CometChatTextFormatter } from "@cometchat/chat-uikit-react";
import DialogHelper from "./Dialog";
import { CometChat } from "@cometchat/chat-sdk-javascript";
class ShortcutFormatter extends CometChatTextFormatter {
private shortcuts: { [key: string]: string } = {};
private dialogIsOpen: boolean = false;
private dialogHelper = new DialogHelper();
private currentShortcut: string | null = null; // Track the currently open shortcut
constructor() {
super();
this.setTrackingCharacter("!");
CometChat.callExtension("message-shortcuts", "GET", "v1/fetch", undefined)
.then((data: any) => {
if (data && data.shortcuts) {
this.shortcuts = data.shortcuts;
}
})
.catch((error) => console.log("error fetching shortcuts", error));
}
onKeyDown(event: KeyboardEvent) {
const caretPosition =
this.currentCaretPosition instanceof Selection
? this.currentCaretPosition.anchorOffset
: 0;
const textBeforeCaret = this.getTextBeforeCaret(caretPosition);
const match = textBeforeCaret.match(/!([a-zA-Z]+)$/);
if (match) {
const shortcut = match[0];
const replacement = this.shortcuts[shortcut];
if (replacement) {
// Close the currently open dialog, if any
if (this.dialogIsOpen && this.currentShortcut !== shortcut) {
this.closeDialog();
}
this.openDialog(replacement, shortcut);
}
}
}
getCaretPosition() {
if (!this.currentCaretPosition?.rangeCount) return { x: 0, y: 0 };
const range = this.currentCaretPosition?.getRangeAt(0);
const rect = range.getBoundingClientRect();
return {
x: rect.left,
y: rect.top,
};
}
openDialog(buttonText: string, shortcut: string) {
this.dialogHelper.createDialog(
() => this.handleButtonClick(buttonText),
buttonText
);
this.dialogIsOpen = true;
this.currentShortcut = shortcut;
}
closeDialog() {
this.dialogHelper.closeDialog(); // Use DialogHelper to close the dialog
this.dialogIsOpen = false;
this.currentShortcut = null;
}
handleButtonClick = (buttonText: string) => {
if (this.currentCaretPosition && this.currentRange) {
// Inserting the replacement text corresponding to the shortcut
const shortcut = Object.keys(this.shortcuts).find(
(key) => this.shortcuts[key] === buttonText
);
if (shortcut) {
const replacement = this.shortcuts[shortcut];
this.addAtCaretPosition(
replacement,
this.currentCaretPosition,
this.currentRange
);
}
}
if (this.dialogIsOpen) {
this.closeDialog();
}
};
getFormattedText(text: string): string {
return text;
}
private getTextBeforeCaret(caretPosition: number): string {
if (
this.currentRange &&
this.currentRange.startContainer &&
typeof this.currentRange.startContainer.textContent === "string"
) {
const textContent = this.currentRange.startContainer.textContent;
if (textContent.length >= caretPosition) {
return textContent.substring(0, caretPosition);
}
}
return "";
}
}
export default ShortcutFormatter;
import React from "react";
import ReactDOM from "react-dom";
interface DialogProps {
onClick: () => void;
buttonText: string;
}
const Dialog: React.FC<DialogProps> = ({ onClick, buttonText }) => {
return (
<div
style={{
position: "fixed",
left: "300px",
top: "664px",
width: "800px",
height: "45px",
}}
>
<button
style={{
width: "800px",
height: "100%",
cursor: "pointer",
backgroundColor: "#f2e6ff",
border: "2px solid #9b42f5",
borderRadius: "12px",
textAlign: "left",
paddingLeft: "20px",
font: "600 15px sans-serif, Inter",
}}
onClick={onClick}
>
{buttonText}
</button>
</div>
);
};
export default class DialogHelper {
private dialogContainer: HTMLDivElement | null = null;
createDialog(onClick: () => void, buttonText: string) {
this.dialogContainer = document.createElement("div");
document.body.appendChild(this.dialogContainer);
ReactDOM.render(
<Dialog onClick={onClick} buttonText={buttonText} />,
this.dialogContainer
);
}
closeDialog() {
if (this.dialogContainer) {
ReactDOM.unmountComponentAtNode(this.dialogContainer);
this.dialogContainer.remove();
this.dialogContainer = null;
}
}
}
import React from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatMessageComposer } from "@cometchat/chat-uikit-react";
import ShortcutFormatter from "./ShortCutFormatter";
export function MessageComposerDemo() {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
return chatUser ? (
<div>
<CometChatMessageComposer
user={chatUser}
textFormatters={[new ShortcutFormatter()]}
/>
</div>
) : null;
}