Message Information
The MessageInformation is a Component designed to display message-related information, such as delivery and read receipts. It serves as an integral part of the CometChat UI UI Kit, extending the List Item class, which provides the underlying infrastructure for CometChat UI components. With its rich set of methods and properties, developers can easily customize and tailor the appearance and behavior of the message information view to suit the specific requirements of their application.
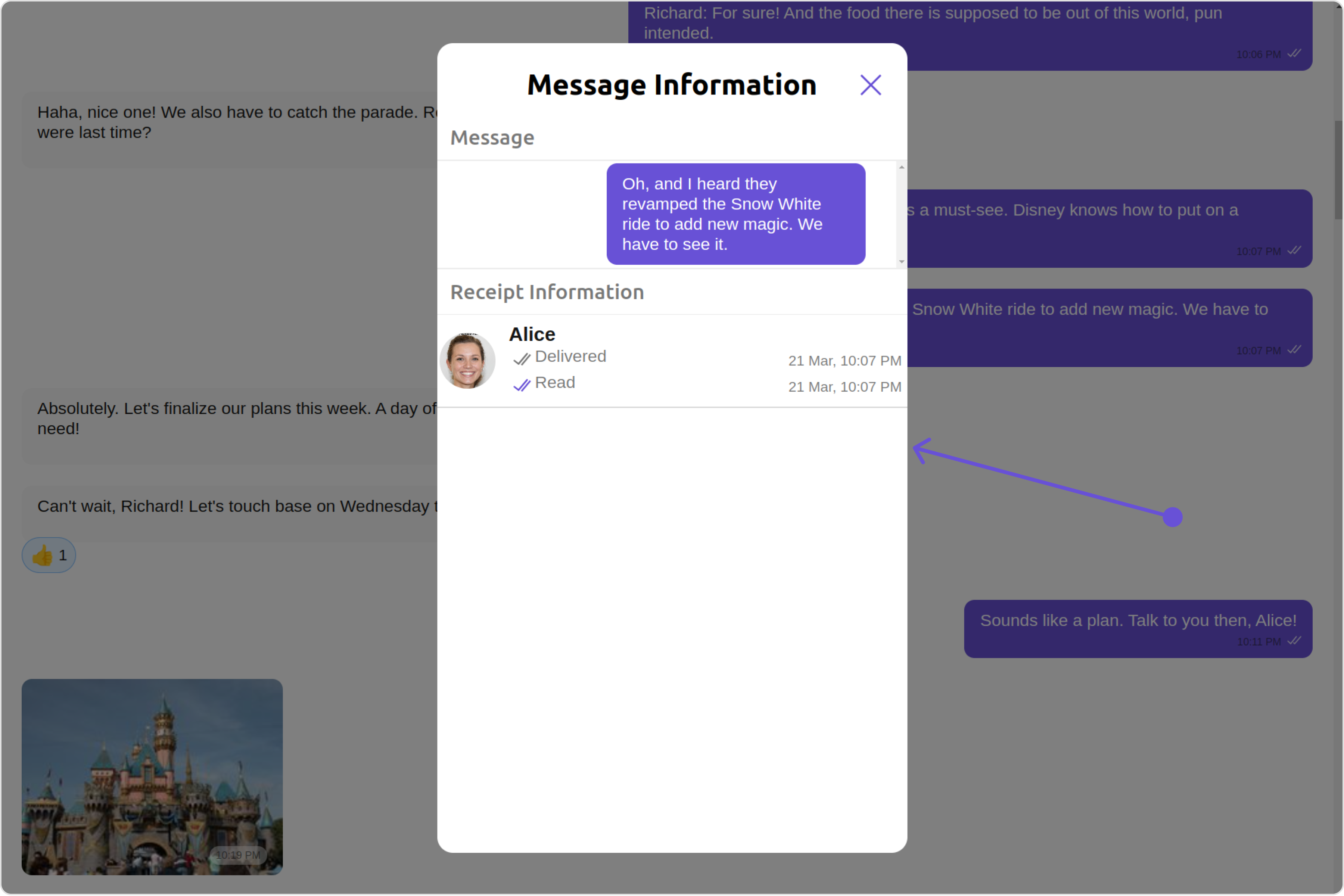
MessageInformation is comprised of the following Base Components:
Base Components | Description |
---|---|
List Item | This renders common components used across Conversations , Groups & Users . |
Usage
Integration
The following code snippet illustrates how you can directly incorporate the Message Information component into your Application.
- MessageComposerDemo.tsx
- App.tsx
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { CometChatMessageInformation, ListItemStyle } from '@cometchat/chat-uikit-react'
import React from 'react'
const MessageInformationDemo = () => {
const [message, setmessage] = React.useState<CometChat.BaseMessage | undefined>();
React.useEffect(() => {
CometChat.getMessageDetails(166).then((message) => {
setmessage(message);
})
}, []);
const bubbleView = () => {
const listItemStyle = new ListItemStyle({
width: "500px",
height: "100%",
border: "2px solid #6615e8",
borderRadius:"12px",
titleColor:"#ffffff",
background:"rgb(104, 81, 214)",
titleFont:"12px sans-serif"
});
return(
<div>
<cometchat-list-item title ={(message as CometChat.TextMessage).getText()} listItemStyle={JSON.stringify(listItemStyle)} />
</div>
)
};
return (
<>
{
message &&
<CometChatMessageInformation
message={message}
bubbleView={bubbleView}
/>
}
</>
)
}
export default MessageInformationDemo;
import { MessageInformationDemo } from "./MessageInformationDemo";
export default function App() {
return (
<div className="App">
<div>
<MessageInformationDemo />
</div>
</div>
);
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onClose
The onClose
event is typically triggered when the close button is clicked and it carries a default action. However, with the following code snippet, you can effortlessly override this default operation.
- MessageComposerDemo.tsx
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { CometChatMessageInformation, ListItemStyle } from '@cometchat/chat-uikit-react'
import React from 'react'
const MessageInformationDemo = () => {
const [message, setmessage] = React.useState<CometChat.BaseMessage | undefined>();
React.useEffect(() => {
CometChat.getMessageDetails(166).then((message) => {
setmessage(message);
})
}, []);
const bubbleView = () => {
const listItemStyle = new ListItemStyle({
width: "500px",
height: "100%",
border: "2px solid #6615e8",
borderRadius:"12px",
titleColor:"#ffffff",
background:"rgb(104, 81, 214)",
titleFont:"12px sans-serif"
});
return(
<div>
<cometchat-list-item title ={(message as CometChat.TextMessage).getText()} listItemStyle={JSON.stringify(listItemStyle)} />
</div>
)
};
const getOnClose = () => {
//your custom onClose action
};
return (
<>
{
message &&
<CometChatMessageInformation
message={message}
bubbleView={bubbleView}
onClose={getOnClose}
/>
}
</>
)
}
export default MessageInformationDemo;
2. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the MessageInformation component.
- MessageComposerDemo.tsx
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { CometChatMessageInformation, ListItemStyle } from '@cometchat/chat-uikit-react'
import React from 'react'
const MessageInformationDemo = () => {
const [message, setmessage] = React.useState<CometChat.BaseMessage | undefined>();
React.useEffect(() => {
CometChat.getMessageDetails(166).then((message) => {
setmessage(message);
})
}, []);
const bubbleView = () => {
const listItemStyle = new ListItemStyle({
width: "500px",
height: "100%",
border: "2px solid #6615e8",
borderRadius:"12px",
titleColor:"#ffffff",
background:"rgb(104, 81, 214)",
titleFont:"12px sans-serif"
});
return(
<div>
<cometchat-list-item title ={(message as CometChat.TextMessage).getText()} listItemStyle={JSON.stringify(listItemStyle)} />
</div>
)
};
const getOnError = (error: CometChat.CometChatException) => {
console.log("error:",error);
};
return (
<>
{
message &&
<CometChatMessageInformation
message={message}
bubbleView={bubbleView}
onError={getOnError}
/>
}
</>
)
}
export default MessageInformationDemo;
Filters
MessageInformation component does not have any available filters.
Events
MessageInformation component does not have any available events.
Customization
To fit your app's design requirements, you can customize the appearance of the MessageInformation component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. MessageInformationStyle
To modify the styling, you can apply the MessageInformationStyle to the MessageInformation Component using the messageInformationStyle
property.
- MessageComposerDemo.tsx
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { CometChatMessageInformation, ListItemStyle, MessageInformationStyle } from '@cometchat/chat-uikit-react'
import React from 'react'
const MessageInformationDemo = () => {
const [message, setmessage] = React.useState<CometChat.BaseMessage | undefined>();
React.useEffect(() => {
CometChat.getMessageDetails(166).then((message) => {
setmessage(message);
})
}, []);
const bubbleView = () => {
const listItemStyle = new ListItemStyle({
width: "500px",
height: "100%",
border: "2px solid #6615e8",
borderRadius:"12px",
titleColor:"#ffffff",
background:"rgb(104, 81, 214)",
titleFont:"12px sans-serif"
});
return(
<div>
<cometchat-list-item title ={(message as CometChat.TextMessage).getText()} listItemStyle={JSON.stringify(listItemStyle)} />
</div>
)
};
const messageInformationStyle = new MessageInformationStyle({
background:'#ffffff',
border:'2px solid #faf5ff',
borderRadius:'10px',
height:'500px',
width:'400px',
captionTextColor:"#630bba"
})
return (
<>
{
message &&
<CometChatMessageInformation
message={message}
bubbleView={bubbleView}
messageInformationStyle={messageInformationStyle}
/>
}
</>
)
}
export default MessageInformationDemo;
The following properties are exposed by MessageInformationStyle:
Property | Description | Code |
---|---|---|
titleTextFont | Sets the font for the title | titleTextFont?: string; |
titleTextColor | Sets the font for the title | titleTextColor?: string; |
subtitleTextColor | Sets the text color for the subtitle | subtitleTextColor?: string; |
subtitleTextFont | Sets the text font for the subtitle | subtitleTextFont?: string; |
emptyStateTextColor | Sets the color for the empty state text | emptyStateTextColor?: string; |
emptyStateTextFont | Sets the font for the empty state text | emptyStateTextFont?: string; |
errorStateTextFont | Sets the text font for the error state text | errorStateTextFont?: string; |
errorStateTextColor | Sets the text font for the error state text | errorStateTextColor |
captionTextFont | Sets the text font for the caption | captionTextFont?: string; |
captionTextColor | Sets the text color for the caption | captionTextColor?: string; |
sendIconTint | Sets the icon color for the send Icon | sendIconTint?: string; |
loadingIconTint | Sets the icon color for the loading Icon | loadingIconTint?: string; |
readIconTint | Sets the icon color for the read Icon | readIconTint?: string; |
deliveredIconTint | Sets the icon color for the delivered Icon | deliveredIconTint?: string; |
dividerTint | Sets the icon color for the separator | dividerTint?: string; |
border | Used to set border | border?: string, |
borderRadius | Used to set border radius | borderRadius?: string; |
background | Used to set background colour | background?: string; |
height | Used to set height | height?: string; |
width | Used to set width | width?: string; |
2. ListItemStyle
To apply customized styles to the ListItemStyle
component in the MessageInformation Component, you can use the following code snippet. For further insights on ListItemStyle
Styles refer
- MessageComposerDemo.tsx
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { CometChatMessageInformation, ListItemStyle } from '@cometchat/chat-uikit-react'
import React from 'react'
const MessageInformationDemo = () => {
const listItemStyle = new ListItemStyle({
width: "200px",
height: "100%",
border: "2px solid #6615e8",
borderRadius:"12px",
titleColor:"#ffffff",
background:"rgb(104, 81, 214)",
titleFont:"12px sans-serif",
});
return (
<>
{
message &&
<CometChatMessageInformation
listItemStyle={listItemStyle}
/>
}
</>
)MessageInformationStyle
}
export default MessageInformationDemo;
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- MessageComposerDemo.tsx
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { CometChatMessageInformation, ListItemStyle } from '@cometchat/chat-uikit-react'
import React from 'react'
const MessageInformationDemo = () => {
const [message, setmessage] = React.useState<CometChat.BaseMessage | undefined>();
React.useEffect(() => {
CometChat.getMessageDetails(166).then((message) => {
setmessage(message);
})
}, []);
const bubbleView = () => {
const listItemStyle = new ListItemStyle({
width: "500px",
height: "100%",
border: "2px solid #6615e8",
borderRadius:"12px",
titleColor:"#ffffff",
background:"rgb(104, 81, 214)",
titleFont:"12px sans-serif"
});
return(
<div>
<cometchat-list-item title ={(message as CometChat.TextMessage).getText()} listItemStyle={JSON.stringify(listItemStyle)} />
</div>
)
};
return (
<>
{
message &&
<CometChatMessageInformation
message={message}
bubbleView={bubbleView}
title='your custom title'
closeIconURL='custom close icon url'
/>
}
</>
)
}
export default MessageInformationDemo;
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
message | Sets the message to be displayed in the message information view. | message={getMessage} |
errorStateView | used to show the error state view in case of an error occurred | errorStateView={getErrorStateView} |
deliveredIcon | It is used to pass user defined image in to the MessageReceipt, to change the icon When Message is delivered. | deliveredIcon='your custom delivered icon' |
readIcon | It is used to pass user defined image in to the MessageReceipt, to change the icon When Message is read. | readIcon='your custom read icon' |
receiptDatePattern | used to set the date pattern for delivered and read date time | receiptDatePattern={getReceiptDatePattern} |
emptyStateText | used to set a custom text response when fetching the users has returned an empty list | emptyStateText='custom empty state text' |
How to integrate CometChatMesssageInformation ?
Since CometChatMesssageInformation
is a component, it can be added directly to your application.
- MessageComposerDemo.tsx
- App.tsx
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { CometChatMessageInformation, ListItemStyle } from '@cometchat/chat-uikit-react'
import React from 'react'
const MessageInformationDemo = () => {
const [message, setmessage] = React.useState<CometChat.BaseMessage | undefined>();
React.useEffect(() => {
CometChat.getMessageDetails(166).then((message) => {
setmessage(message);
})
}, []);
const bubbleView = () => {
const listItemStyle = new ListItemStyle({
width: "500px",
height: "100%",
border: "2px solid #6615e8",
borderRadius:"12px",
titleColor:"#ffffff",
background:"rgb(104, 81, 214)",
titleFont:"12px sans-serif"
});
return(
<div>
<cometchat-list-item title ={(message as CometChat.TextMessage).getText()} listItemStyle={JSON.stringify(listItemStyle)} />
</div>
)
};
return (
<>
{
message &&
<CometChatMessageInformation
message={message}
bubbleView={bubbleView}
/>
}
</>
)
}
export default MessageInformationDemo;
import { MessageInformationDemo } from "./MessageInformationDemo";
export default function App() {
return (
<div className="App">
<div>
<MessageInformationDemo />
</div>
</div>
);
}
Properties
property | type | Description |
---|---|---|
template | CometChatMessageTemplate | Sets a custom message template for the message information view. |
message | BaseMessage | Sets the message to be displayed in the message information view. |
title | String | Sets the title that will be displayed at the top of the message information . |
listItemView | ((messageObject: BaseMessage, messageReceipt?: MessageReceipt | undefined) => JSX.Element) | Sets a custom list item view for individual entries in the message information view. |
subtitleView | ((messageObject: BaseMessage, messageReceipt?: MessageReceipt | undefined) => JSX.Element) | Sets a custom subtitle view for individual entries in the message information view. |
receiptDatePattern | ((timestamp: number) => string) | Sets a custom date pattern for formatting receipt dates in the message information view. |
messageInformationStyle | MessageInformationStyle | Sets the overall style and appearance for the message information view. |
readIcon | string | Sets the custom icon resource for read receipts in the message information view. |
deliveredIcon | string | Sets the custom icon resource for delivered receipts in the message information view. |
listItemStyle | ListItemStyle | Sets the style for list items in the message information view. |
onError | ((error: CometChatException) => void) | Sets the error callback to handle any errors that may occur within the message information view. |
emptyStateText | String | Sets the text to be displayed in the empty state view of the message information view. |
errorStateText | String | Sets the error message to be displayed in the error state view of the message information view. |
emptyStateView | () => JSX.Element | Sets the custom layout resource for the empty state view of the message information view. |
errorStateView | () => JSX.Element | Sets the custom layout resource for the error state view of the message information view. |
loadingStateView | () => JSX.Element | Sets the custom layout resource for the loading state view of the message information view. |