Call Log With Details
Overview
The CometChatCallLogsWithDetails
is a Composite Component encompassing components such as Call Logs and Call Log Details. Both of these component contributes to the functionality and structure of the overall CallLogsWithDetails
component.
- iOS
- Android
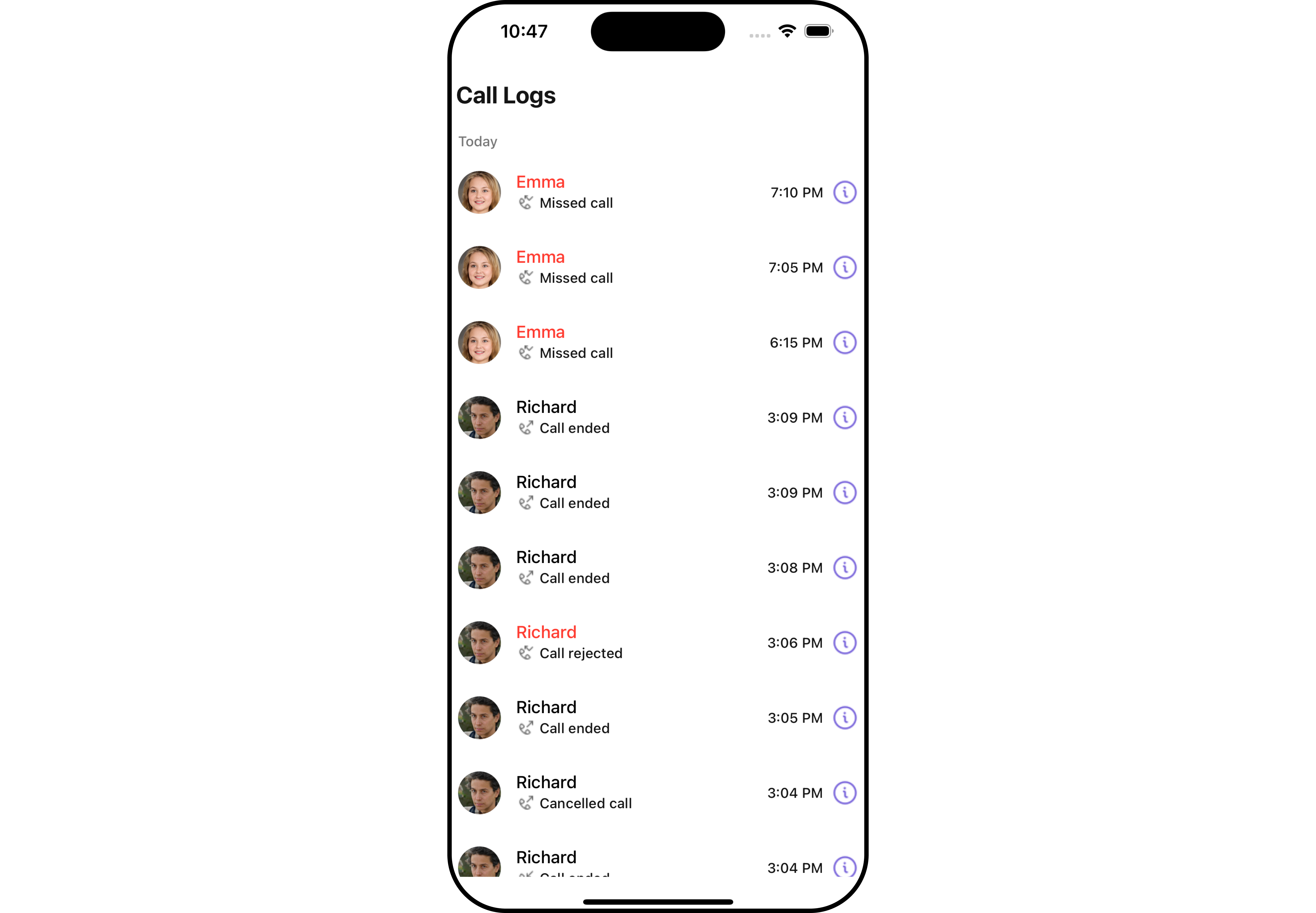
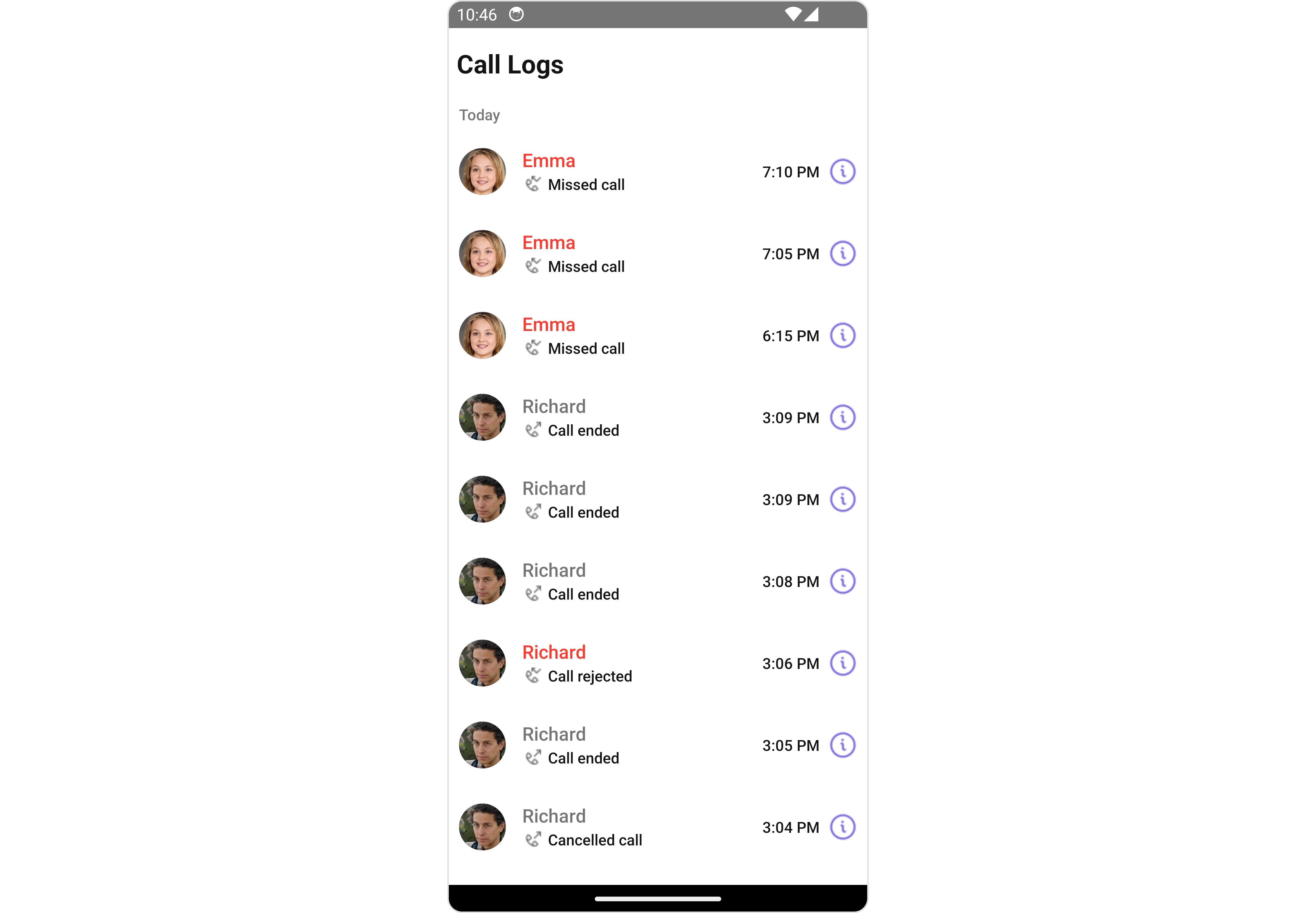
Components | Description |
---|---|
Call Logs | The Call Logs component is designed to show the list of Call Log available . By default, names are shown for all listed users, along with their avatar if available. |
Call Log Details | The Call Log Details component is designed to displays all the information related to a call. This component displays information like user/group information, participants of the call, recordings of the call (if available) & history of all the previous calls. |
Usage
Integration
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatCallLogsWithDetails } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
return <>{loggedInUser && <CometChatCallLogsWithDetails />}</>;
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
While the CallLogsWithDetails component does not have its actions, its components - Call Logs, and Call Log Details - each have their own set of actions.
The Action of the components can be overridden through the use of the Configurations object of its components. Here is an example code snippet.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogsConfigurationInterface,
CallLogDetailsConfigurationInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const datePattern: DatePattern = "timeFormat";
const dateSeparatorPattern: DatePattern = "dayDateFormat";
const callLogsConfiguration: CallLogsConfigurationInterface = {
onInfoIconPress: (prop: { call: CometChat.BaseMessage }) => {
//code
},
datePattern: datePattern,
dateSeparatorPattern: dateSeparatorPattern,
};
const callLogDetailsConfiguration: CallLogDetailsConfigurationInterface = {
onBack: () => {
//code
},
};
return (
<>
{loggedInUser && (
<CometChatCallLogsWithDetails
CallLogsConfiguration={callLogsConfiguration}
callLogDetailsConfiguration={callLogDetailsConfiguration}
/>
)}
</>
);
}
The CometChatCallLogsWithDetails
component overrides several actions from its components to reach its default behavior. The list of actions overridden by GroupsWithMessages includes:
- onInfoIconPress : By overriding the
onInfoIconPress
of the Call Logs Component, CallLogsWithDetails achieves navigation from Call Logs to Call Log Details component.
- iOS
- Android
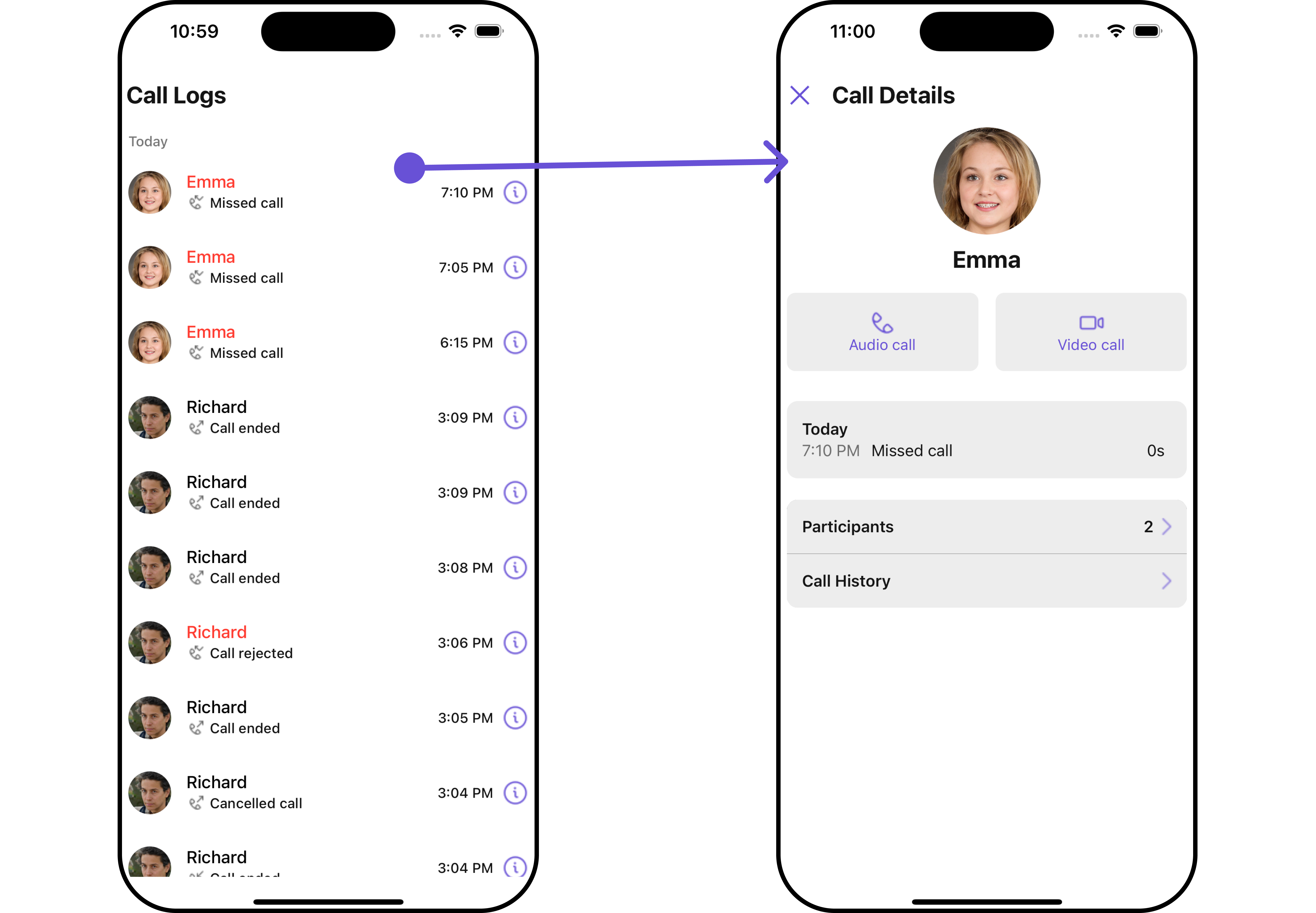
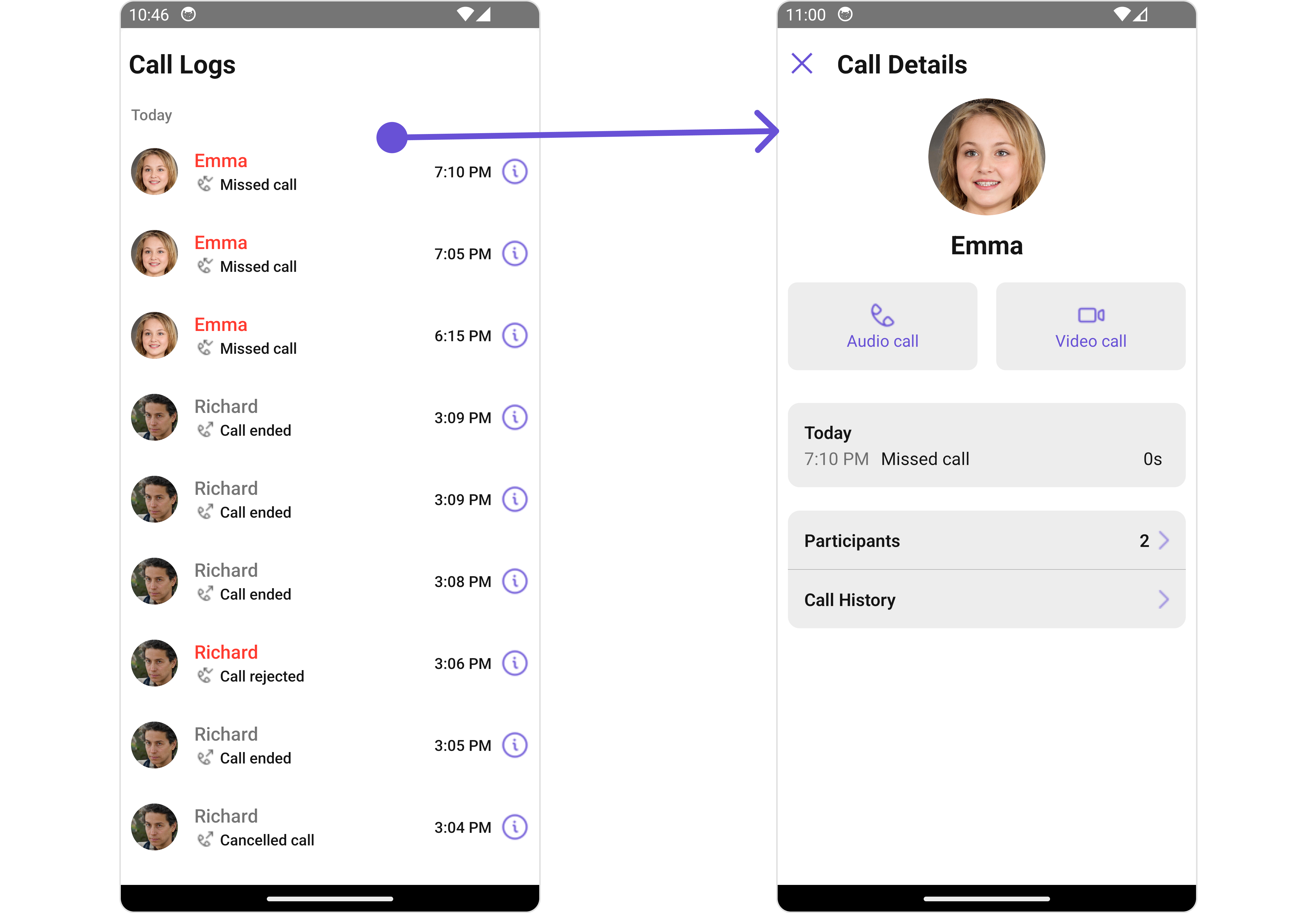
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
While the CallLogsWithDetails component does not have filters, its components do, For more detail on individual filters of its component refer to Call Logs and Call Log Details.
By utilizing the Configurations object of its components, you can apply filters.
In the following example, we are applying a filter to the Call Logs by setting the status to show only 'missed' calls and setting the limit to 5 using the callLogRequestBuilder
.
- App.tsx
import {CometChat} from '@cometchat/chat-sdk-react-native';
import { CometChatCallLogsWithDetails, CallLogsConfigurationInterface, CallLogRequestBuilder } from '@cometchat/chat-uikit-react-native';
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({uid: 'uid'})
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const datePattern : DatePattern = 'timeFormat';
const dateSeparatorPattern : DatePattern = 'dayDateFormat';
const callLogsConfiguration : CallLogsConfigurationInterface = {
datePattern: datePattern,
dateSeparatorPattern: dateSeparatorPattern
callRequestBuilder: new CallLogRequestBuilder().setAuthToken('auth-token').setLimit(5).setCallStatus('missed');
}
return (
<>
{ loggedInUser &&
<CometChatCallLogsWithDetails
CallLogsConfiguration={callLogsConfiguration}
/>
}
</>
);
}
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The CallLogsWithDetails does not produce any events but its component does.
Customization
To fit your app's design requirements, you have the ability to customize the appearance of the CallLogsWithDetails component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
You can customize its sub-component styles. For more details on individual component styles, you can refer Call Logs Styles and Call Log Details Styles.
Styles can be applied to SubComponents using their respective configurations.
Example
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogsConfigurationInterface,
CallLogRequestBuilder,
CallLogsStyleInterface,
CallLogDetailsStyleInterface,
CallLogDetailsConfigurationInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const datePattern: DatePattern = "timeFormat";
const dateSeparatorPattern: DatePattern = "dayDateFormat";
const callLogsStyle: CallLogsStyleInterface = {
titleColor: "red",
};
const callLogsConfiguration: CallLogsConfigurationInterface = {
datePattern: datePattern,
dateSeparatorPattern: dateSeparatorPattern,
callLogsStyle: callLogsStyle,
};
const callLogDetailsStyle: CallLogDetailsStyleInterface = {
titleColor: "blue",
};
const callLogDetailsConfiguration: CallLogDetailsConfigurationInterface = {
callLogDetailsStyle: callLogDetailsStyle,
};
return (
<>
{loggedInUser && (
<CometChatCallLogsWithDetails
CallLogsConfiguration={callLogsConfiguration}
callLogDetailsConfiguration={callLogDetailsConfiguration}
/>
)}
</>
);
}
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogsConfigurationInterface,
CallLogRequestBuilder,
} from "@cometchat/chat-uikit-react-native";
import { CallLog } from "@cometchat/calls-sdk-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
const [callLog, setCallLog] = useState<CallLog>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
const CallLogRequest = new CallLogRequestBuilder()
.setLimit(1)
.setCallStatus("cancelled")
.setAuthToken(user!.getAuthToken())
.build();
CallLogRequest.fetchNext().then((callLogs: CallLog[]) => {
setCallLog(callLogs[0]);
});
})
.catch((error: any) => {
//handle error
});
}, []);
return (
<>
{loggedInUser && callLog && (
<CometChatCallLogsWithDetails call={callLog} />
)}
</>
);
}
- iOS
- Android
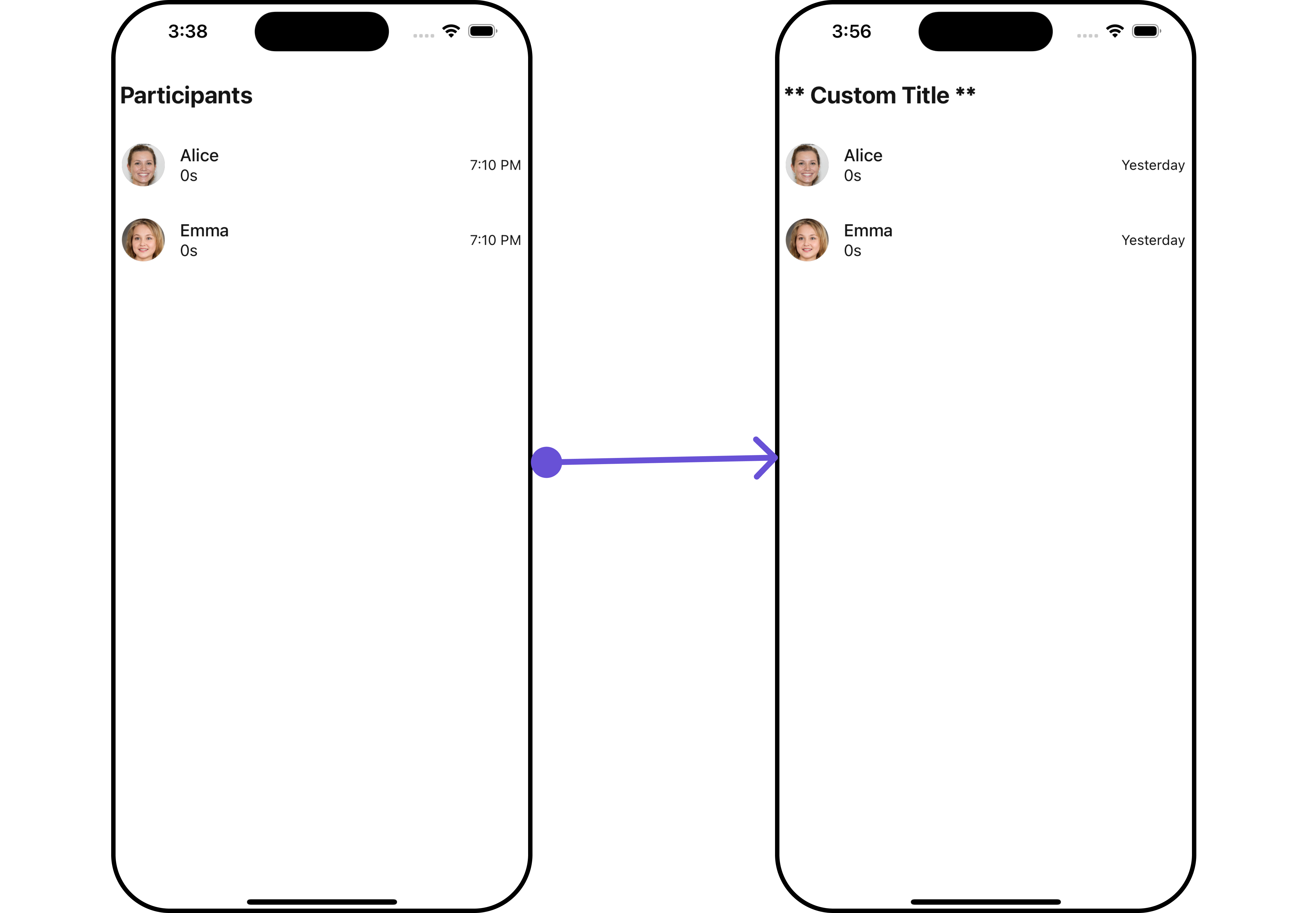
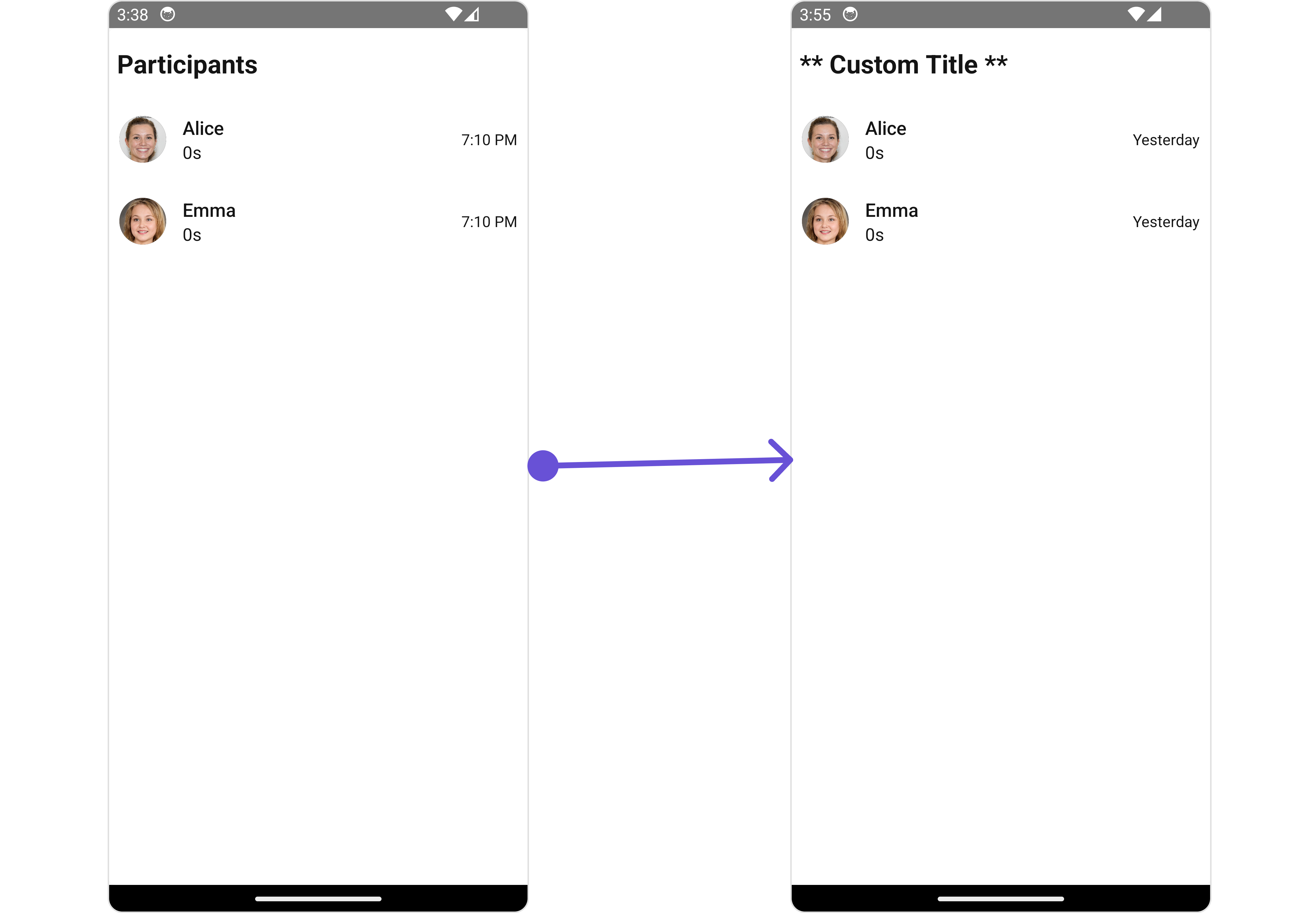
Below is a list of customizations along with corresponding code snippets:
Property | Description | Code |
---|---|---|
call | Used to set the selected Call Log | call?: CallLog |
Components
Nearly all functionality customizations available for a Component are also available for the composite component. Using Configuration, you can modify the properties of its components to suit your needs.
You can find the list of all Functionality customization of individual components in Call Logs and Call Log Details Styles.
Example
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogsConfigurationInterface,
CallLogRequestBuilder,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const datePattern: DatePattern = "timeFormat";
const dateSeparatorPattern: DatePattern = "dayDateFormat";
const callLogsConfiguration: CallLogsConfigurationInterface = {
datePattern: datePattern,
dateSeparatorPattern: dateSeparatorPattern,
showBackButton: true,
};
const callLogDetailsConfiguration: CallLogDetailsConfigurationInterface = {
callLogDetailsStyle: callLogDetailsStyle,
showCloseButton: true,
};
return (
<>
{loggedInUser && (
<CometChatCallLogsWithDetails
CallLogsConfiguration={callLogsConfiguration}
callLogDetailsConfiguration={callLogDetailsConfiguration}
/>
)}
</>
);
}
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your own views, layouts, and UI elements and then incorporate those into the component.
By utilizing the Configuration object of each component, you can apply advanced-level customizations to the GroupsWithMessages.
Example
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogsConfigurationInterface,
CallLogRequestBuilder,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const datePattern: DatePattern = "timeFormat";
const dateSeparatorPattern: DatePattern = "dayDateFormat";
const callLogsConfiguration: CallLogsConfigurationInterface = {
datePattern: datePattern,
dateSeparatorPattern: dateSeparatorPattern,
EmptyStateView: () => {
return <Text>Loading...</Text>;
},
};
return (
<>
{loggedInUser && (
<CometChatCallLogsWithDetails
CallLogsConfiguration={callLogsConfiguration}
/>
)}
</>
);
}
To find all the details on individual Component advance customization you can refer, Call Logs Advance and Call Log Details Advance.
CallLogsWithDetails uses advanced-level customization of both Call Logs & Call Log Details components to achieve its default behavior.
- CallLogsWithDetails utilizes the onInfoIconPress property of the
Call Logs
subcomponent to navigate the Call Log Details from Call Logs to Call Log Details.
- iOS
- Android
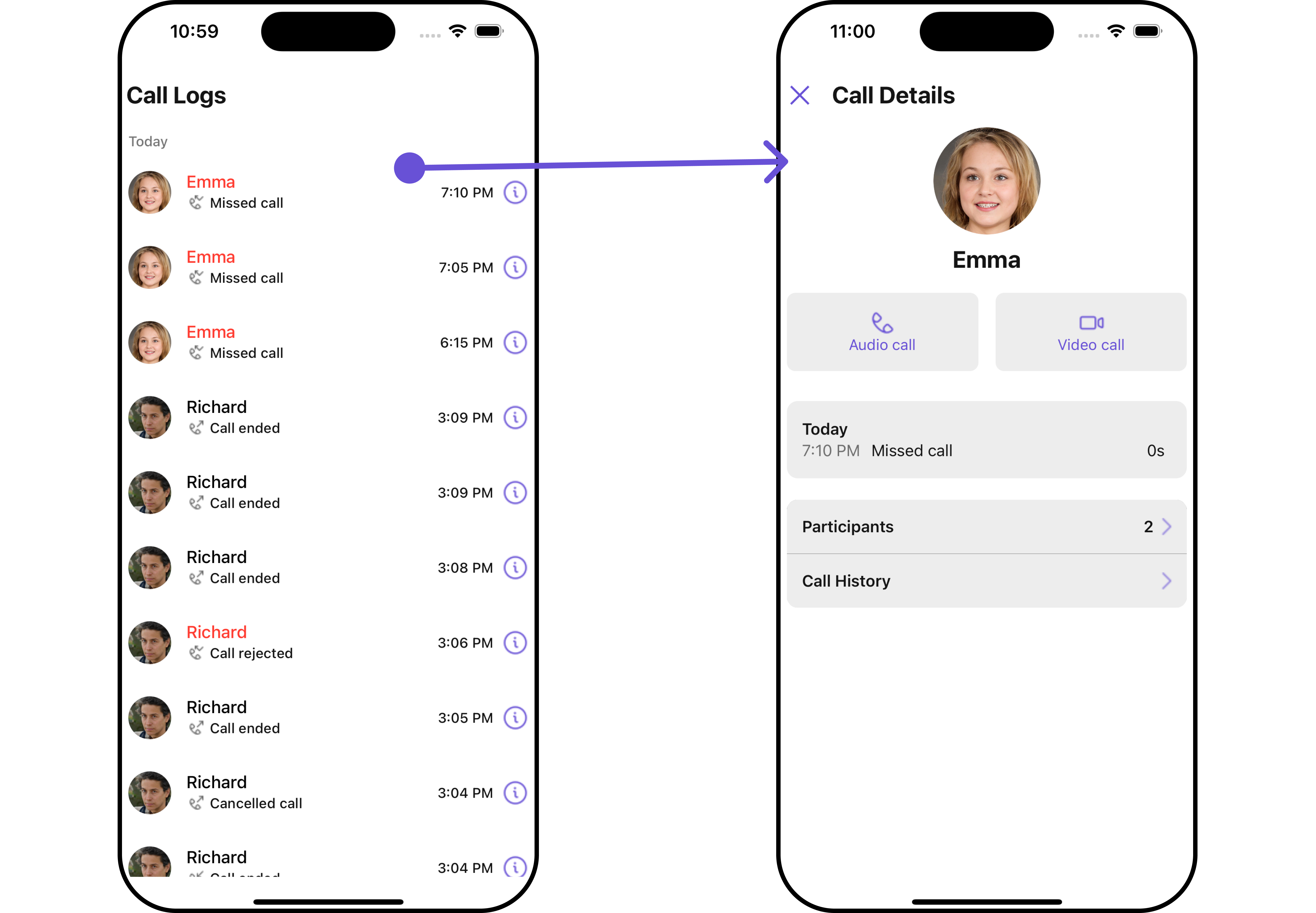
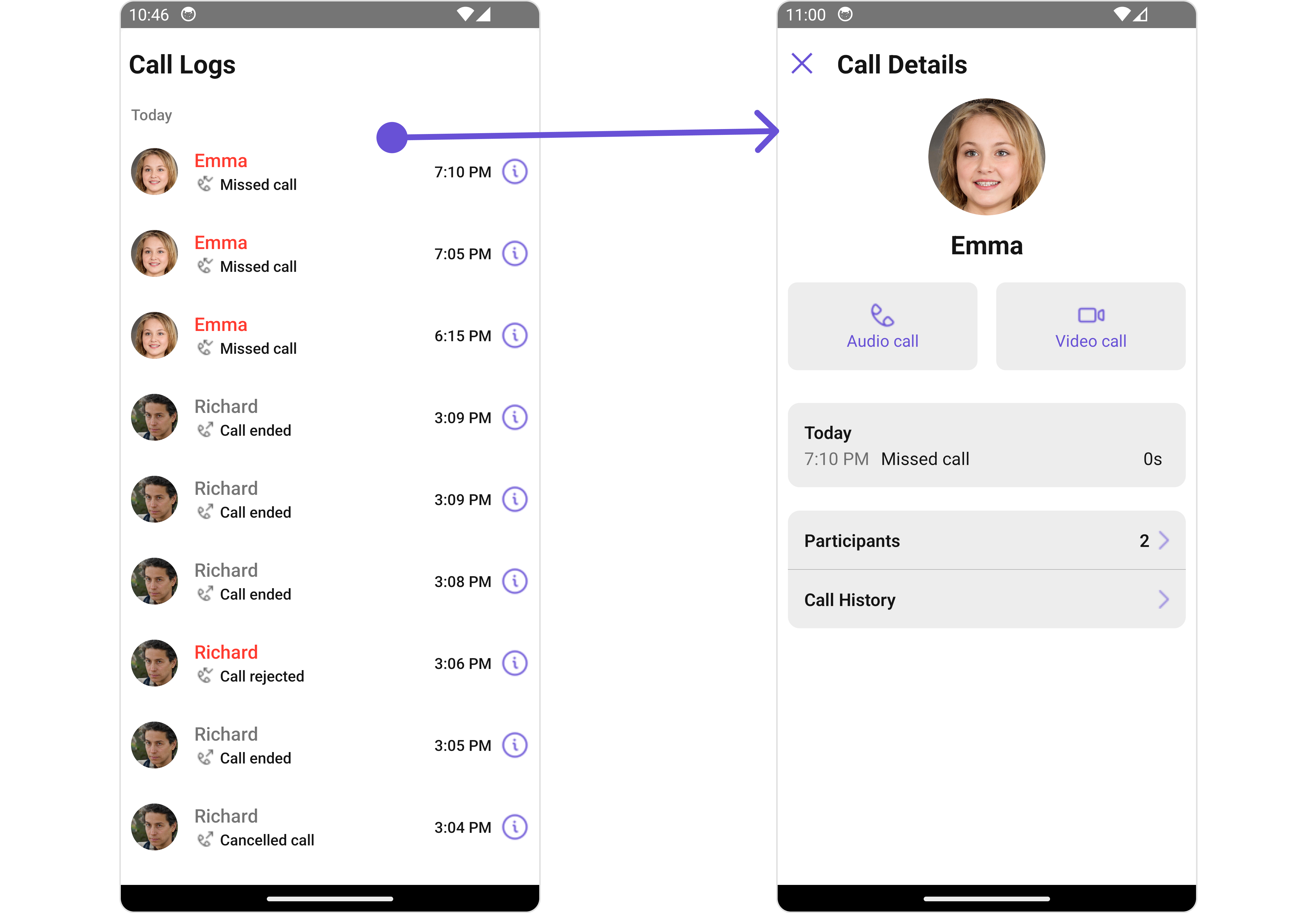
- CallLogsWithDetails utilizes the onBack action of the
Call Log Details
subcomponent to close the Call Log Details Component
- iOS
- Android
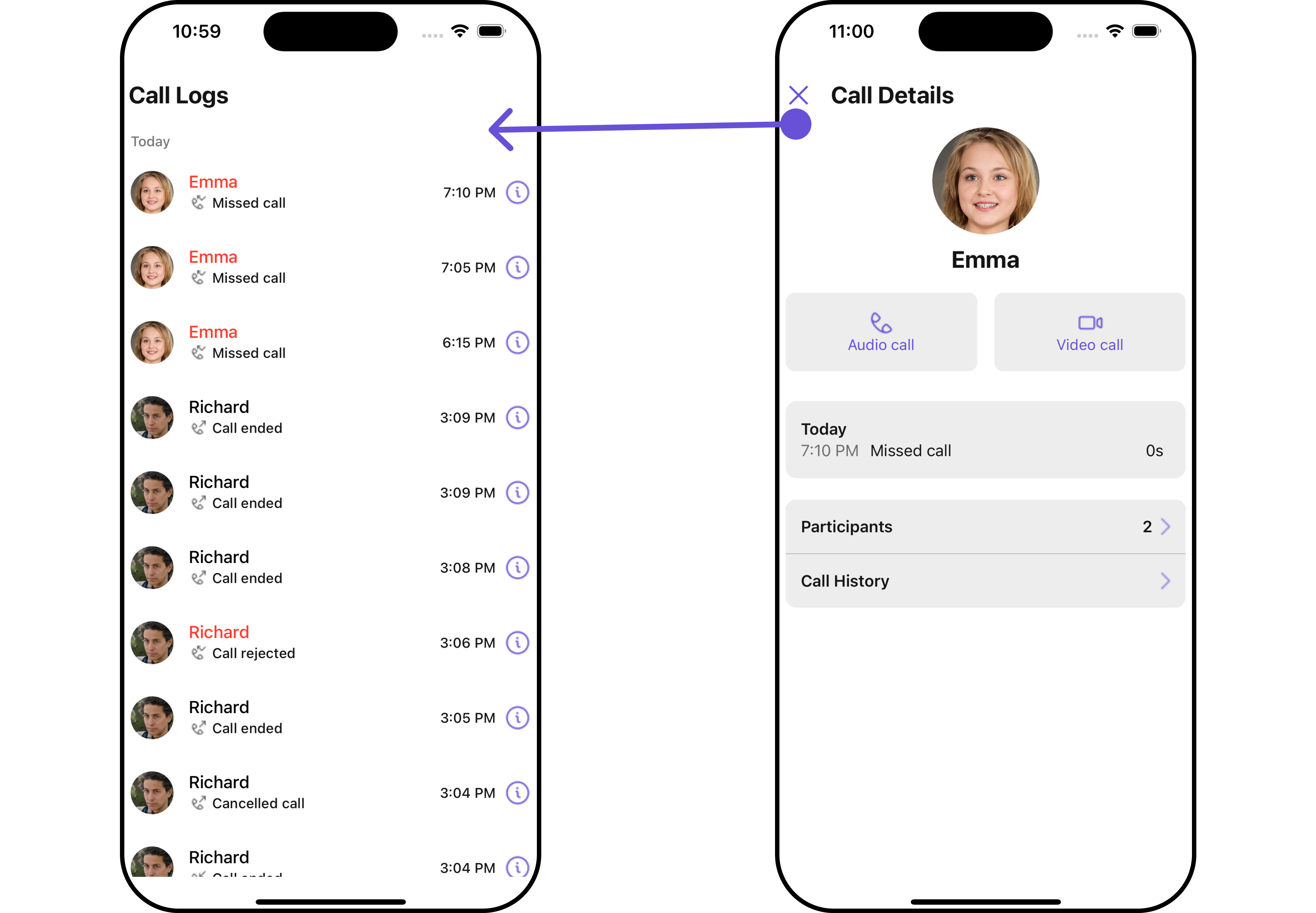
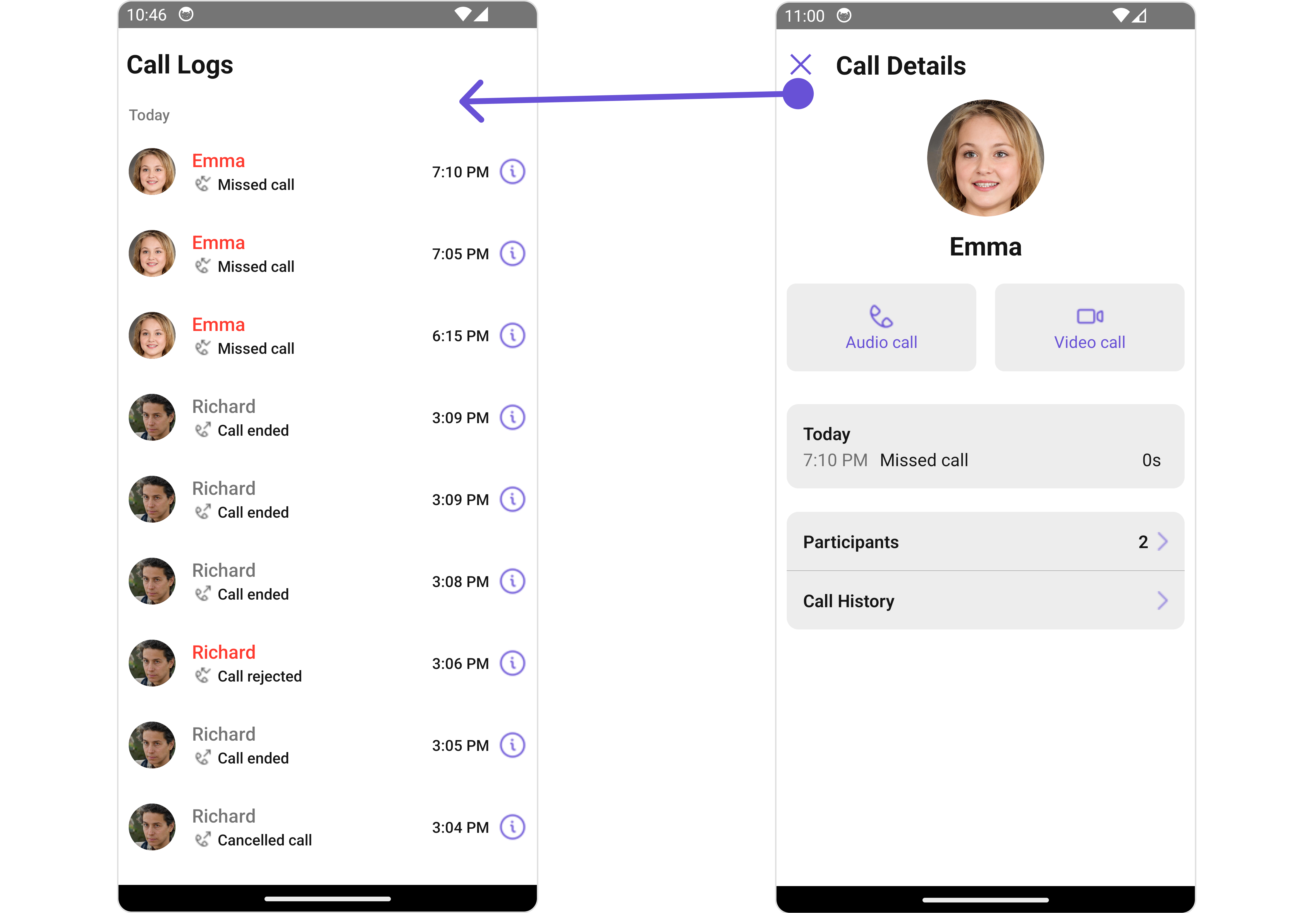
When you override onItemPress
and onBack
, the default behavior of CallLogsWithDetails will also be overridden.
Configurations
Configurations offer the ability to customize the properties of each component within a Composite Component.
CallLogsWithDetails has Call Logs
and Call Log Details
component. Hence, each of these components will have its individual Configuration
.
Configurations
expose properties that are available in its individual components.
Call Logs
You can customize the properties of the Groups component by making use of the callLogsConfiguration
. You can accomplish this by employing the callLogsConfiguration
props as demonstrated below:
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogsConfigurationInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const callLogsConfiguration: CallLogsConfigurationInterface = {
//override properties
};
return (
<>
{loggedInUser && (
<CometChatCallLogsWithDetails
CallLogsConfiguration={callLogsConfiguration}
/>
)}
</>
);
}
All exposed properties of CallLogsConfiguration
can be found under Call Logs. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Call Logs subcomponent and, in addition, you only want to hide the separator of the Call Logs.
You can modify the style using the callLogsStyle
property and show the back button using showBackButton
property.
- iOS
- Android
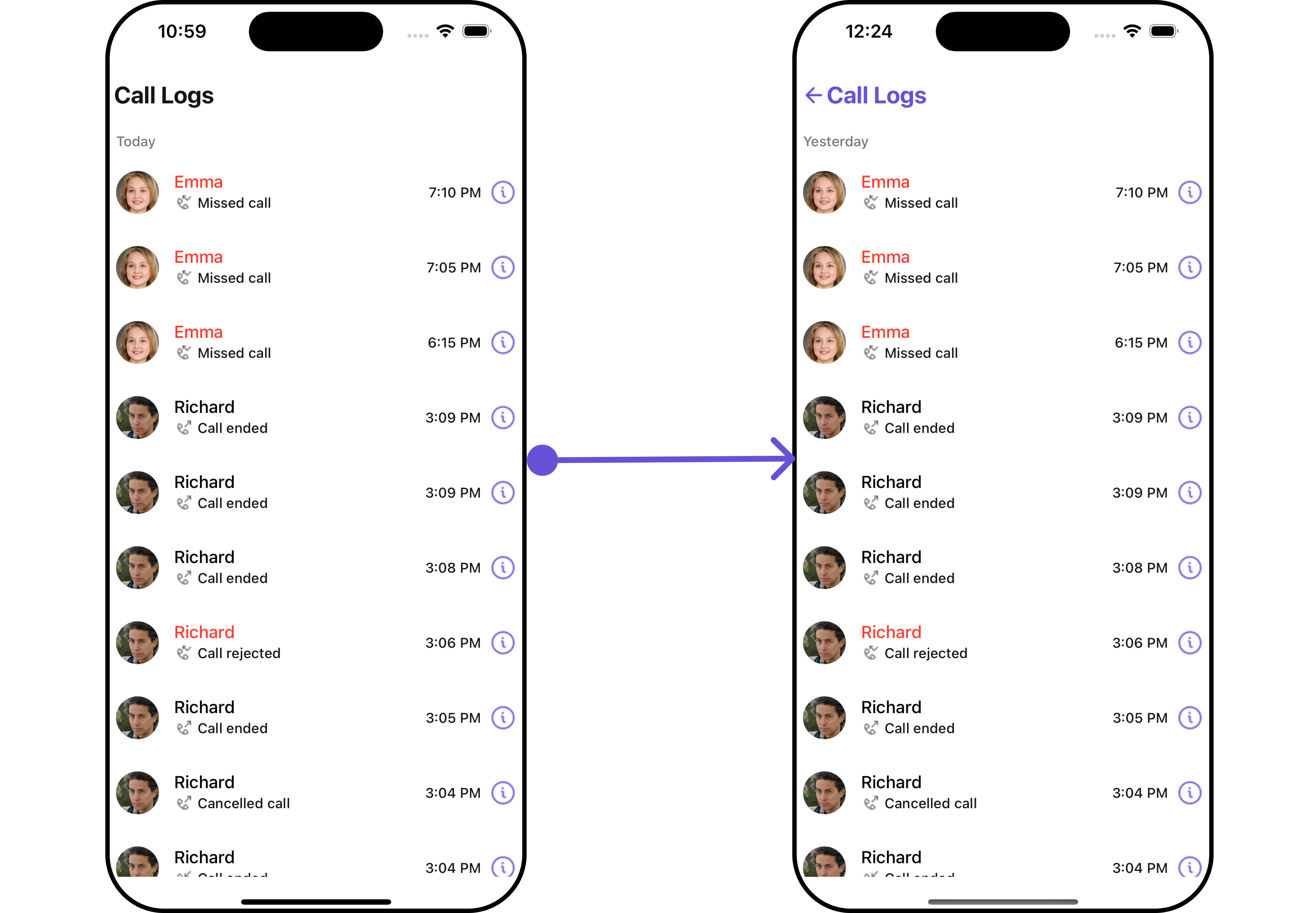
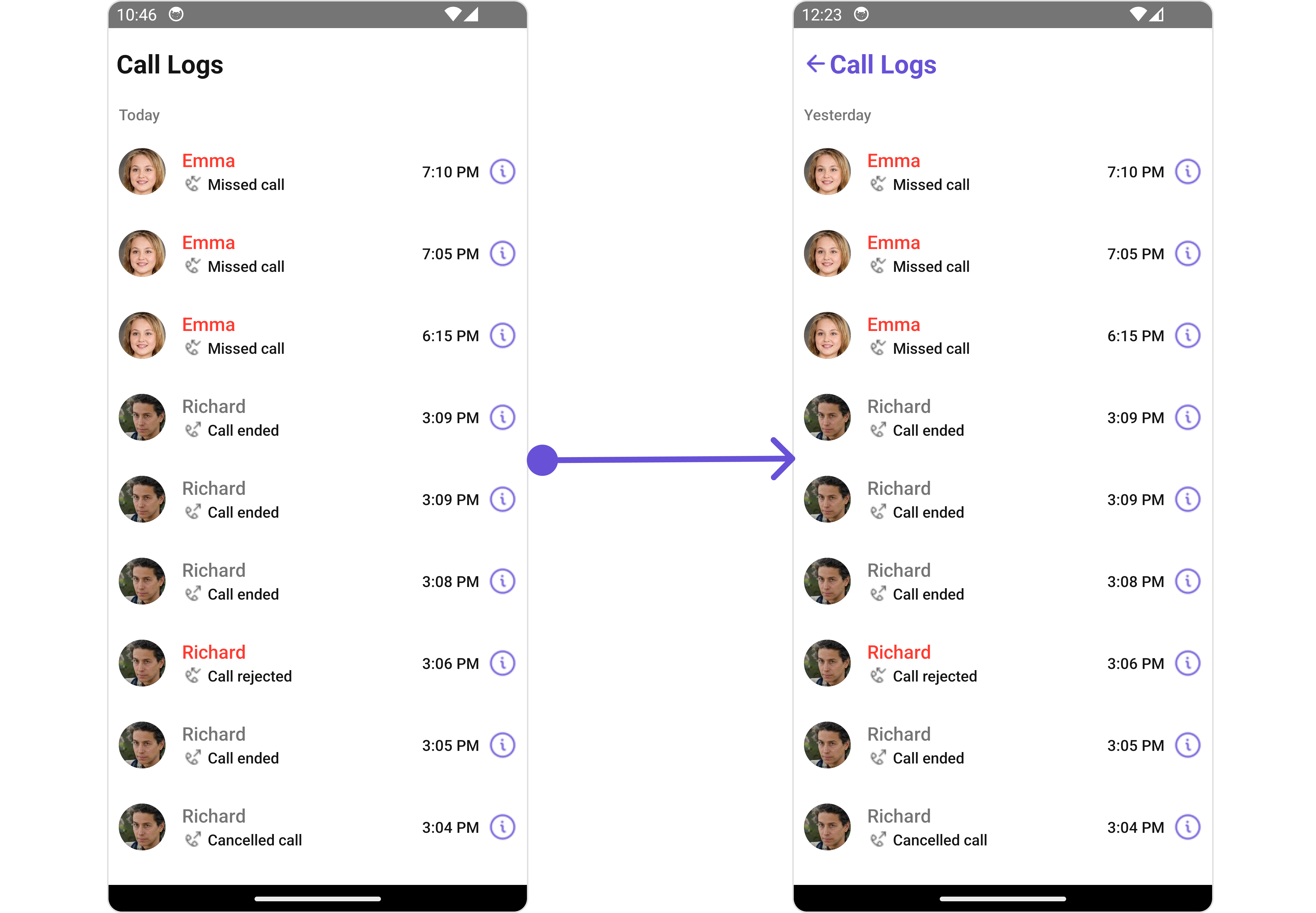
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogsConfigurationInterface,
CallLogRequestBuilder,
CallLogsStyleInterface,
CallLogDetailsConfigurationInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const datePattern: DatePattern = "timeFormat";
const dateSeparatorPattern: DatePattern = "dayDateFormat";
const callLogsStyle: CallLogsStyleInterface = {
titleColor: "#6851D6",
};
const callLogsConfiguration: CallLogsConfigurationInterface = {
datePattern: datePattern,
dateSeparatorPattern: dateSeparatorPattern,
callLogsStyle: callLogsStyle,
showBackButton: true,
};
return (
<>
{loggedInUser && (
<CometChatCallLogsWithDetails
CallLogsConfiguration={callLogsConfiguration}
/>
)}
</>
);
}
Call Log Details
You can customize the properties of the Call Log Details component by making use of the callLogDetailsConfiguration
. You can accomplish this by employing the callLogDetailsConfiguration
props as demonstrated below:
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogDetailsConfigurationInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const callLogDetailsConfiguration: CallLogDetailsConfigurationInterface = {
//override properties
};
return (
<>
{loggedInUser && (
<CometChatCallLogsWithDetails
callLogDetailsConfiguration={callLogDetailsConfiguration}
/>
)}
</>
);
}
All exposed properties of CallLogDetailsConfiguration
can be found under Call Log Details. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Call Log Details subcomponent and, in addition, you only want to change the Back Icon.
You can modify the style using the callLogDetailsStyle
property and change the back icon using `` property.
- iOS
- Android
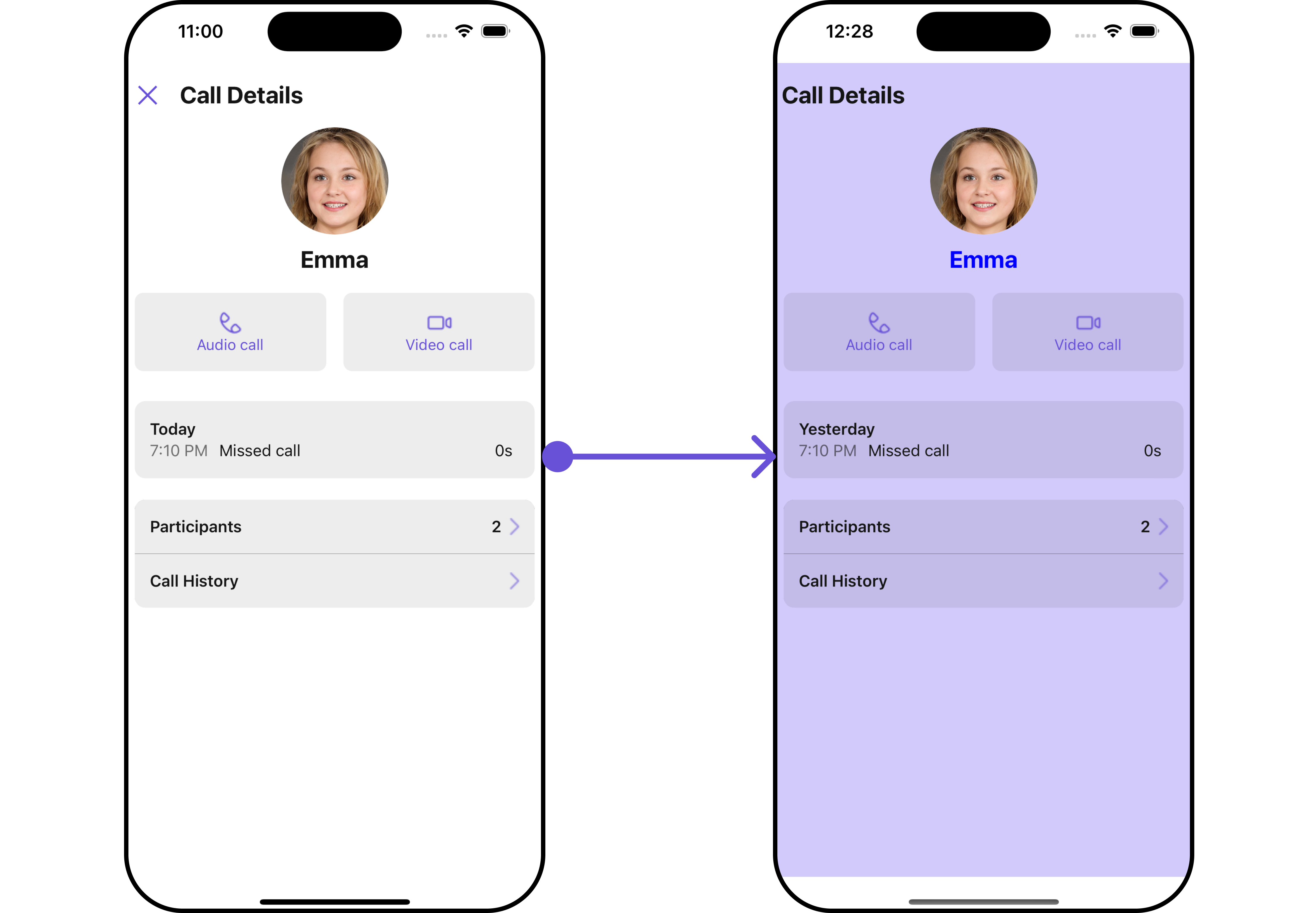
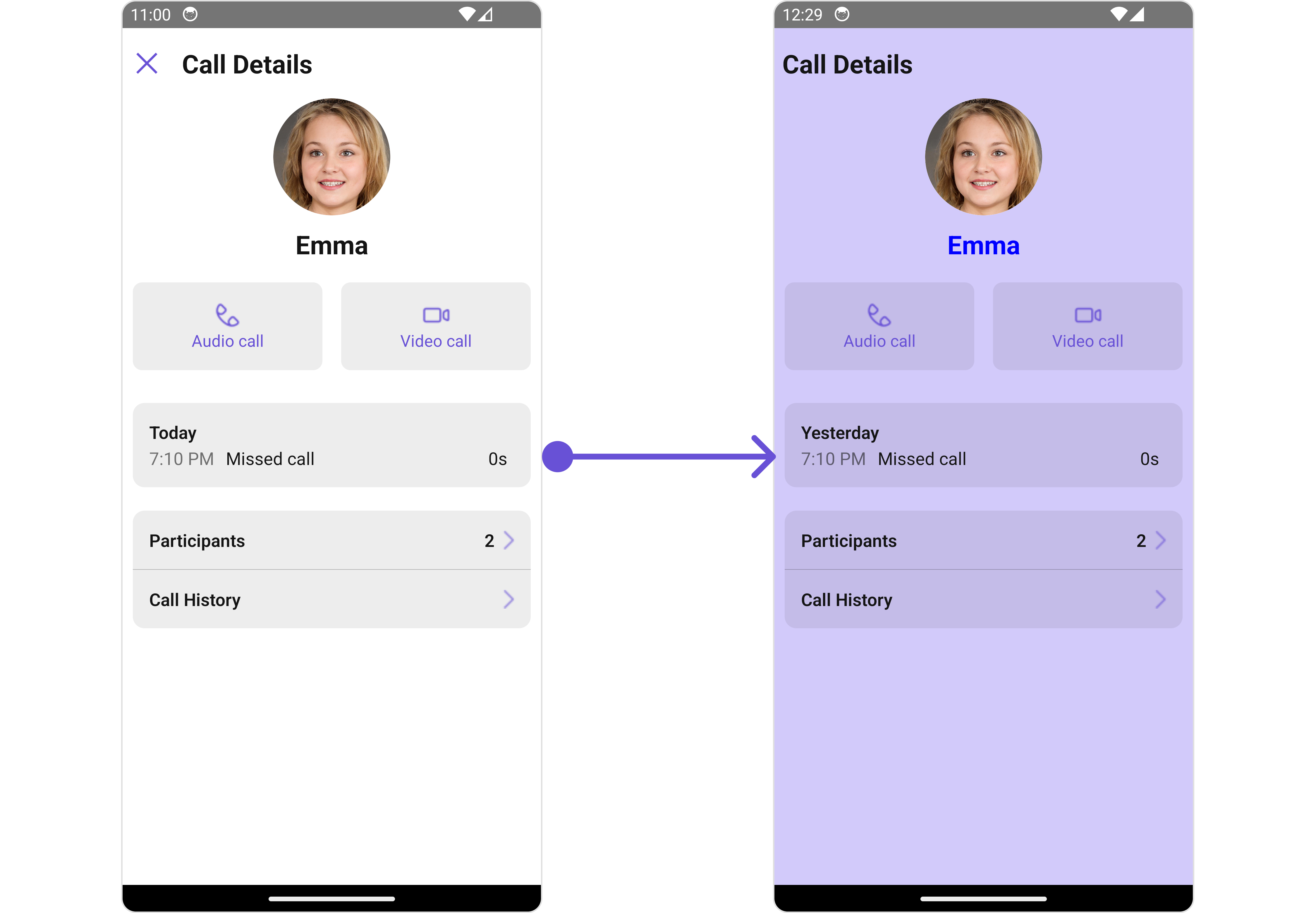
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatCallLogsWithDetails,
CallLogDetailsStyleInterface,
CallLogDetailsConfigurationInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [loggedInUser, setLoggedInUser] = useState<CometChat.User>();
useEffect(() => {
//code
CometChatUIKit.login({ uid: "uid" })
.then(async (user: CometChat.User) => {
setLoggedInUser(user);
})
.catch((error: any) => {
//handle error
});
}, []);
const callLogDetailsStyle: CallLogDetailsStyleInterface = {
titleColor: "blue",
backgroundColor: "#d2cafa",
};
const callLogDetailsConfiguration: CallLogDetailsConfigurationInterface = {
callLogDetailsStyle: callLogDetailsStyle,
};
return (
<>
{loggedInUser && (
<CometChatCallLogsWithDetails
callLogDetailsConfiguration={callLogDetailsConfiguration}
/>
)}
</>
);
}