Getting Started
Start your first conversation
CometChat UI Kit for React Native is a collection of prebuilt UI components designed to simplify the development of an in-app chat with all the essential messaging features. Our UI Kit offers light and dark themes, various fonts, colors, and additional customization options.
CometChat UI Kit supports both one-to-one and group conversations. Follow the guide below to initiate conversations from scratch using CometChat React Native UI Kit.
- iOS
- Android
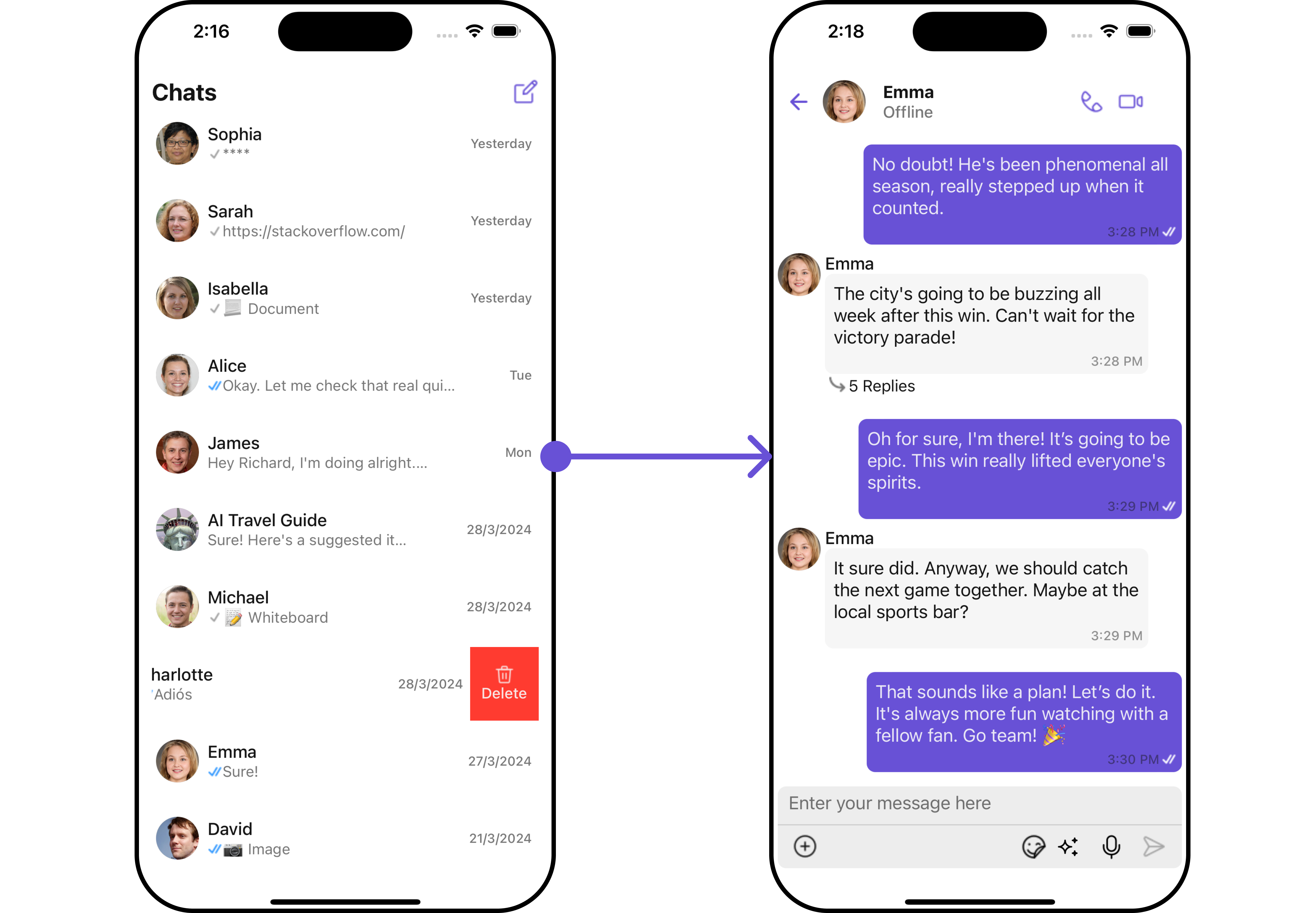
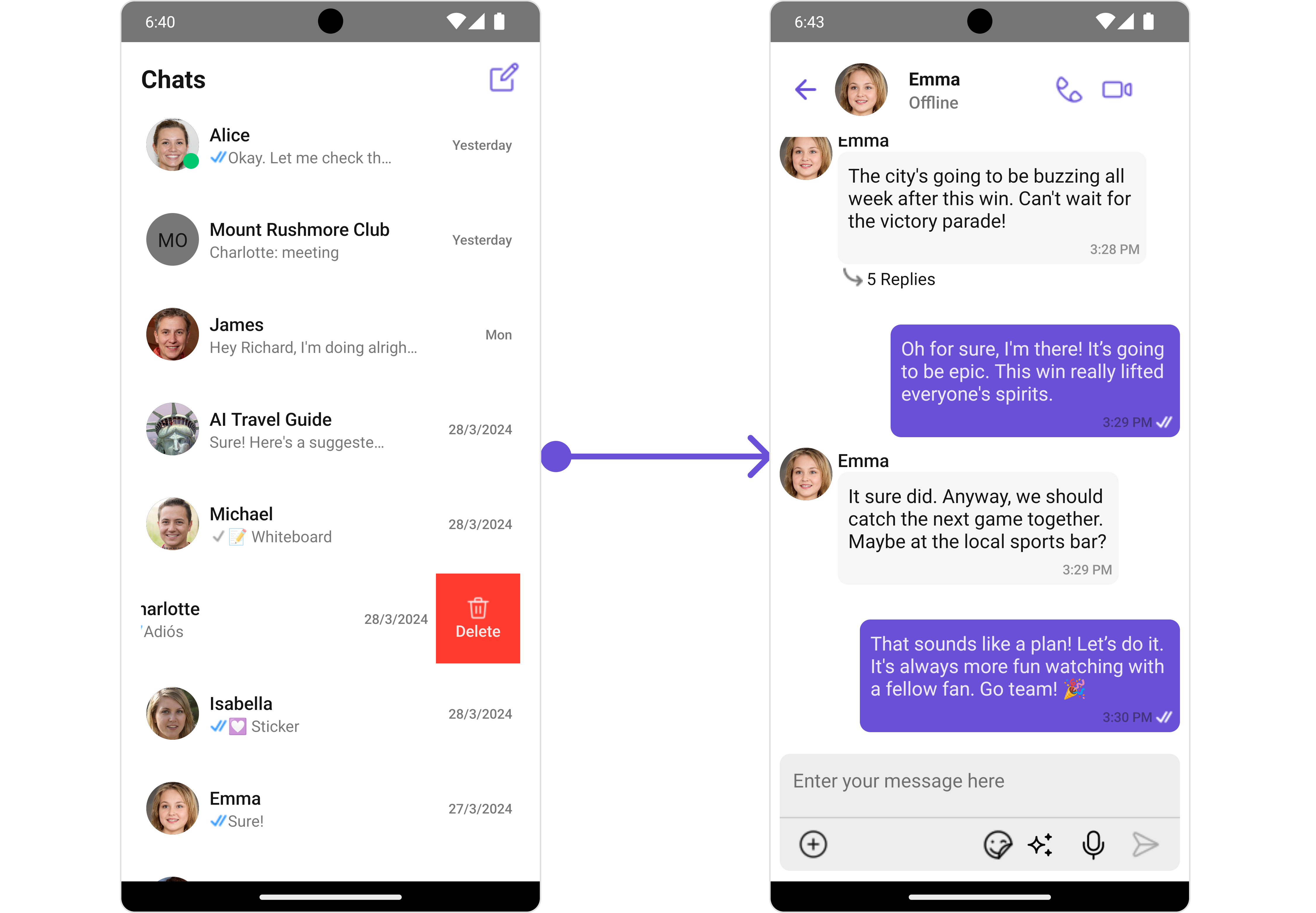
Prerequisites
Before installing UI Kit for React Native, you need to create a CometChat application on the CometChat Dashboard, which comprises everything required in a chat service including users, groups, calls & messages. You will need the App ID
, AuthKey
, Region
of your CometChat application when initialising the SDK.
i. Register on CometChat
- To install UI Kit for React Native, you need to first register on CometChat Dashboard. Click here to sign up.
ii. Get Your Application Keys
- Create a new app
- Head over to the QuickStart or API & Auth Keys section and note the App ID, Auth Key, and Region.
Each CometChat application can be integrated with a single client app. Within the same application, users can communicate with each other across all platforms, whether they are on mobile devices or on the web.
iii. IDE Setup
The minimum requirements for UI Kit for React Native are:
Node 14 or later
- JDK 11
Android Studio
Xcode
iOS 12.0 and later
- Go to finder -> Applications -> Xcode -> Get Info
- Click on
Open using Rosetta
Getting Started
You can start building a modern messaging experience in your app by installing the new UI Kit. This developer kit is an add-on feature to CometChat React Native SDK.
Step 1
Create a project
To get started, open terminal
and create a new project using below command.
- Bash
npx react-native init ChatingApp
Step 2
Add Dependency
You can install UI Kit for React Native through using below command.
- Bash
npm i @cometchat/chat-uikit-react-native
Other Dependencies
- Bash
npm i @cometchat/chat-sdk-react-native
npm i @react-native-async-storage/async-storage@1.17.10
npm i @react-native-clipboard/clipboard@1.13.2
Add Permissions for android
Open AndroidManifest.xml
file from android/app/src/main
location and add below permissions
- XML
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
<uses-permission android:name="android.permission.VIBRATE" />
<uses-permission android:name="android.permission.INTERNET" />
Please make sure Android SDK path is set in the ANDROID_HOME
environment variable or in local.properties
via the field sdk.dir
.
Install @cometchat/calls-sdk-react-native Package (Optional)
If you want calling functionality inside your application then you need to install calling SDK additionally inside your project.
react native ui kits supports calling SDK V3 or higher
-
You can install
@cometchat/calls-sdk-react-native
Calling SDK for React Native using below command.- Bash
npm i @cometchat/calls-sdk-react-native
-
Install dependancies required for call SDK to work
- JSON
// Dependencies required for calls sdk.
{
"@cometchat/chat-sdk-react-native": "^4.0.5",
"@react-native-async-storage/async-storage": "^1.17.5",
"@react-native-community/netinfo": "7.1.7", // for react-native 0.63 & above.
"@react-native-community/netinfo": "6.1.0", // for react-native below 0.63
"react-native-background-timer": "2.4.1",
"react-native-callstats": "3.73.7",
"react-native-webrtc": "1.106.1"
} -
Add permissions
Android:
- XML
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.MODIFY_AUDIO_SETTINGS" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" />IOS:
- XML
<key>NSCameraUsageDescription</key>
<string>This is for Camera permission</string>
<key>NSMicrophoneUsageDescription</key>
<string>This is for Mic permission</string>
Step 3
Initialise CometChatUIKit
To integrate and run CometChat UI Kit in your app, you need to initialize it beforehand.
The Init method initializes the settings required for CometChat. Please ensure to call this method before invoking any other methods from CometChat UI Kit or CometChat SDK.
The Auth Key is an optional property of the UIKitSettings
Class. It is intended for use primarily during proof-of-concept (POC) development or in the early stages of application development. You can use the Auth Token method to log in securely.
- Typescript
import React, {useEffect} from 'react'
import { Platform, PermissionsAndroid } from 'react-native'
import { CometChatUIKit, UIKitSettings } from '@cometchat/chat-uikit-react-native'
import { CometChat } from '@cometchat/chat-sdk-react-native'
// add below code in App.js or App.tsx
const getPermissions = () => {
if (Platform.OS == "android") {
PermissionsAndroid.requestMultiple([
PermissionsAndroid.PERMISSIONS.WRITE_EXTERNAL_STORAGE,
PermissionsAndroid.PERMISSIONS.READ_EXTERNAL_STORAGE,
PermissionsAndroid.PERMISSIONS.CAMERA,
PermissionsAndroid.PERMISSIONS.RECORD_AUDIO,
]);
}
}
useEffect(() => {
getPermissions();
let uikitSettings : UIKitSettings= {
appId: <!--Your appId goes here -->,
authKey: <!-- your app authKey goes here -->,
region: <!-- App region goes here -->,
subscriptionType: CometChat.AppSettings.SUBSCRIPTION_TYPE_ALL_USERS,
};
CometChatUIKit.init(uikitSettings)
.then(() => {
console.log("CometChatUiKit successfully initialized")
})
.catch((error) => {
console.log("Initialization failed with exception:", error)
})
},[]);
Login User
For login, you require a UID. You can create your own users on the CometChat Dashboard or via API. We have pre-generated test users: cometchat-uid-1, cometchat-uid-2, cometchat-uid-3, cometchat-uid-4, cometchat-uid-5.
The Login method returns the User object containing all the information of the logged-in user.
This straightforward authentication method is ideal for proof-of-concept (POC) development or during the early stages of application development. For production environments, however, we strongly recommend using an Auth Token instead of an Auth Key to ensure enhanced security.
- Typescript
let uid = <# Enter User's UID Here #>
CometChatUIKit.login({uid: uid})
.then(user => {
console.log("User logged in successfully \(user.getName())")
})
.catch((error) => {
console.log("Login failed with exception:", error)
})
- We have set up 5 users for testing having UIDs: cometchat-uid-1, cometchat-uid-2, cometchat-uid-3, cometchat-uid-4, and cometchat-uid-5.
Step 5
Render Conversation With Message
ConversationsWithMessages is a wrapper component that offers functionality to render both the Conversations and Messages components. It also enables opening the Messages by tapping on any conversation rendered in the list of conversations.
- iOS
- Android
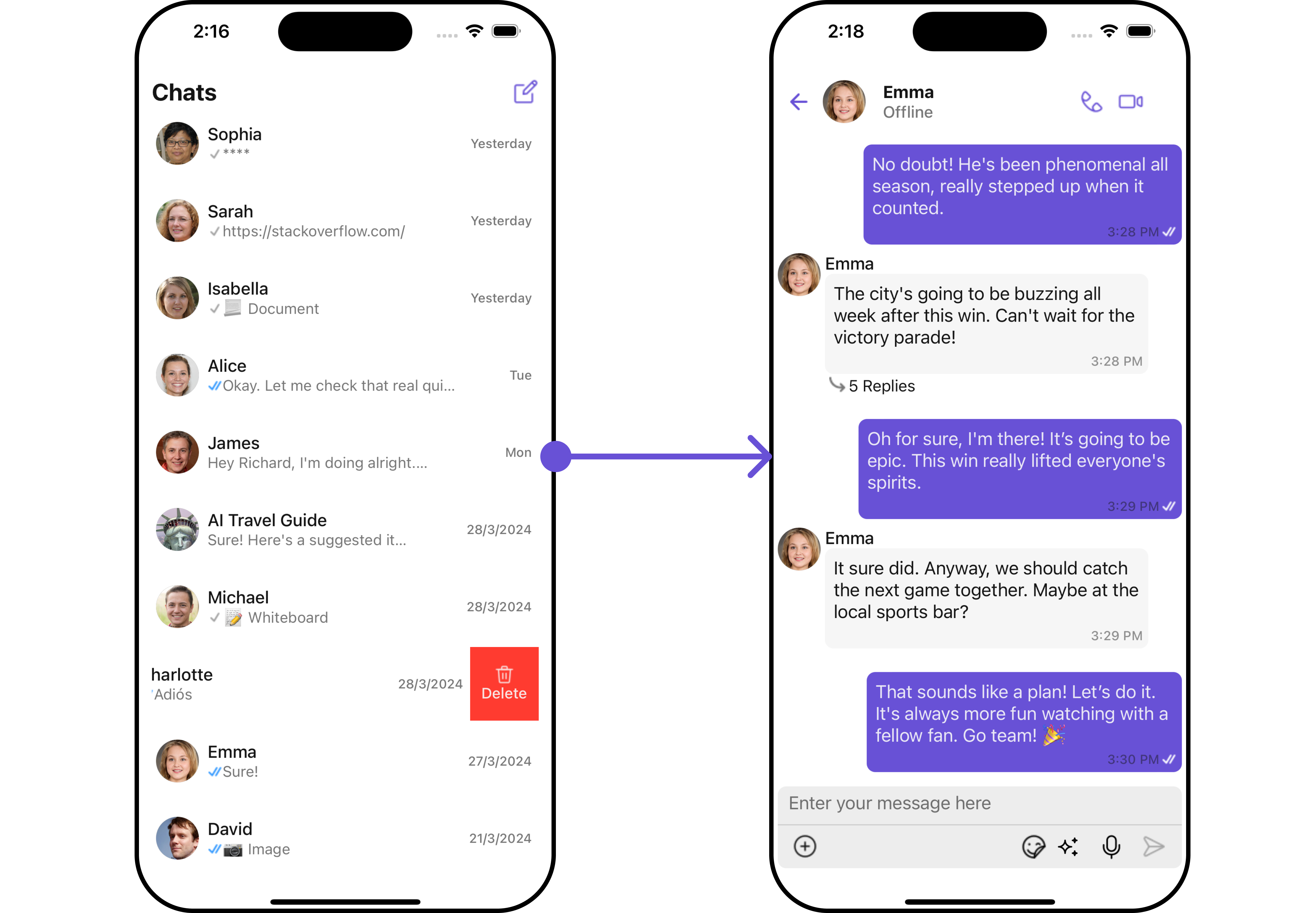
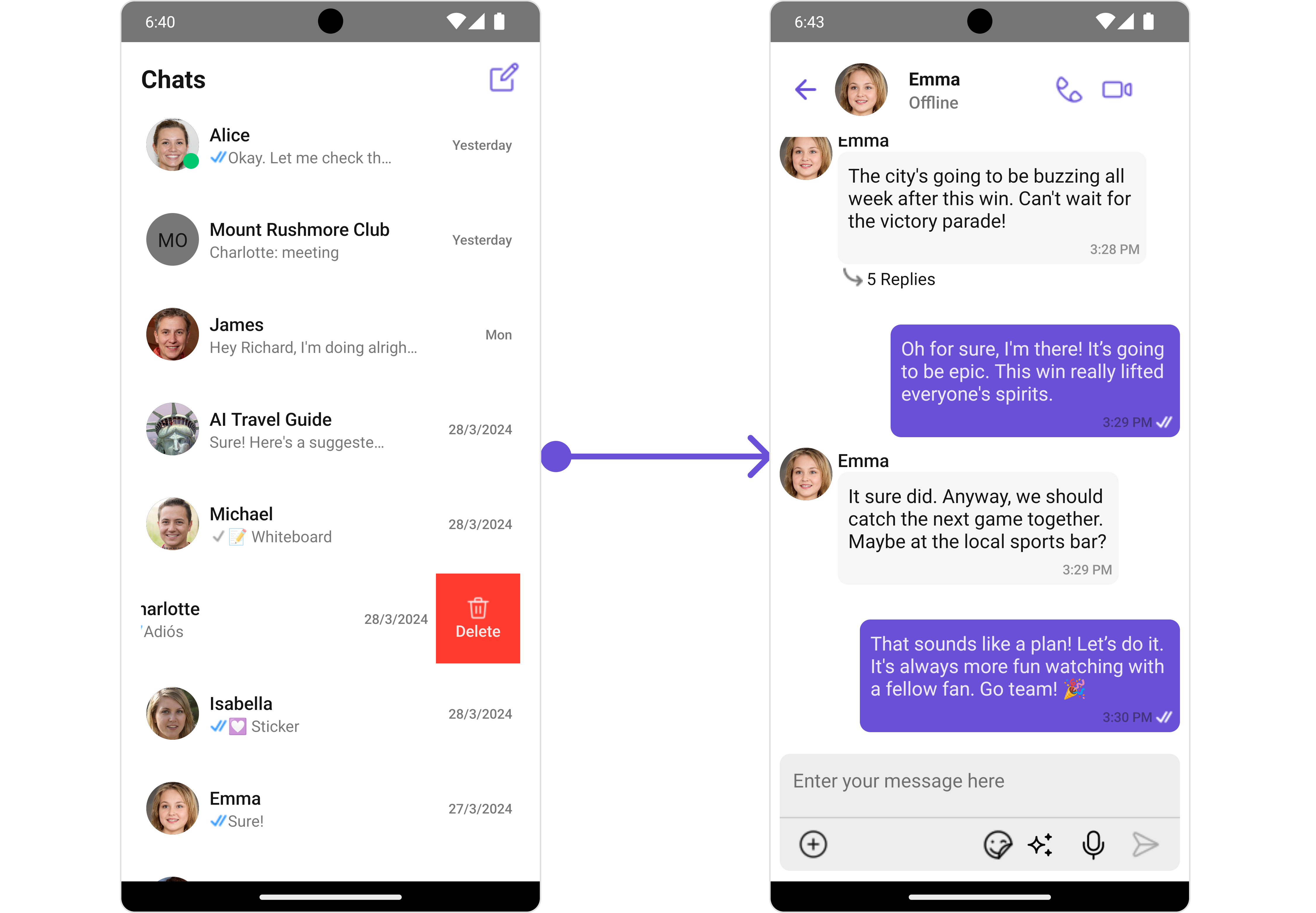
- JSX
<CometChatConversationsWithMessages />
It will automatically fetch the conversation data upon loading the list. If the conversation list is empty, you can start a new conversation.
Make sure you add a Privacy Manifest File to your app before going live