Conversations
Overview
CometChatConversations
is a component that displays all conversations associated with the currently logged-in user.
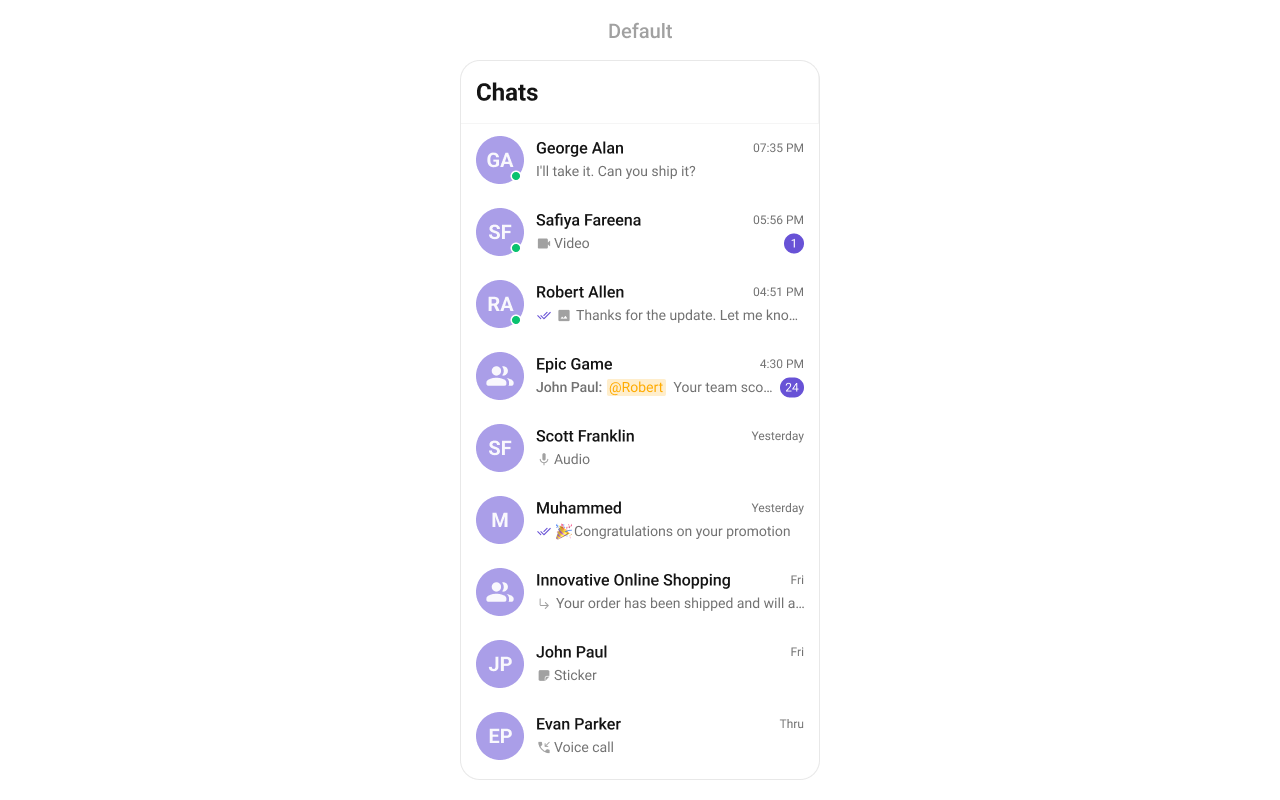
Usage
Integration
To use Conversations in your component, use the following code snippet:
App.tsx
import { CometChatConversations } from "@cometchat/chat-uikit-react-native";
//code
return <CometChatConversations />;
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
onItemPress
Function executed when an item is long-pressed in the conversation list, commonly used to initiate actions like delete, selection, or entering multi-select mode.
- App.tsx
import { CometChatConversations } from "@cometchat/chat-uikit-react-native";
const onPressHandler = (conversationClicked: CometChat.Conversation) => {
//code
};
return <CometChatConversations onItemPress={onPressHandler} />;
onItemLongPress
Function executed when a conversation item is long-pressed, allowing additional actions like delete or select.
- App.tsx
import { CometChatConversations } from "@cometchat/chat-uikit-react-native";
const onLongPressHandler = (conversationClicked: CometChat.Conversation) => {
//code
};
return <CometChatConversations onItemLongPress={onLongPressHandler} />;