Groups with Messages
Overview
The GroupsWithMessages is a Composite Component encompassing components such as Groups and Messages. Both of these component contributes to the functionality and structure of the overall GroupsWithMessages component.
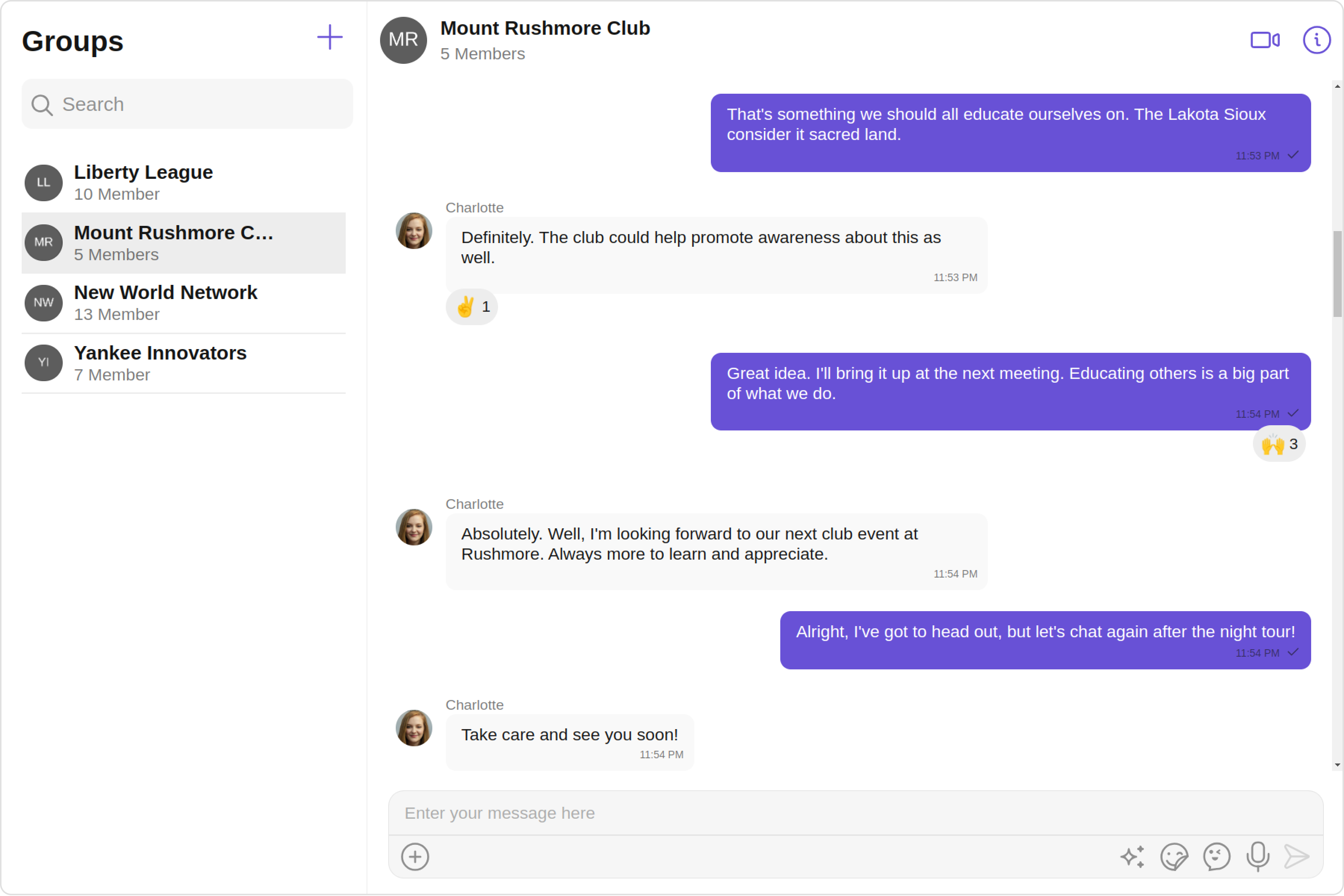
Components | Description |
---|---|
Groups | The Groups component is designed to display a list of Groups . This essentially represents the recent conversation history. |
Messages | The Messages component is designed to manage the messaging interaction for Group's conversations. |
Usage
Integration
- GroupsWithMessagesDemo.tsx
- App.tsx
import { CometChatGroupsWithMessages } from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
return <CometChatGroupsWithMessages />;
};
export default GroupsWithMessagesDemo;
import GroupsWithMessagesDemo from "./GroupsWithMessagesDemo";
export default function App() {
return (
<div className="App">
<GroupsWithMessagesDemo />
</div>
);
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the GroupsWithMessages component.
- TypeScript
- JavaScript
import { CometChatGroupsWithMessages } from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const handleOnError = (error: CometChat.CometChatException) => {
console.log("Your custom on error actions");
};
return <CometChatGroupsWithMessages onError={handleOnError} />;
};
export default GroupsWithMessagesDemo;
import { CometChatGroupsWithMessages } from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const handleOnError = (error) => {
console.log("Your custom on error actions");
};
return <CometChatGroupsWithMessages onError={handleOnError} />;
};
export default GroupsWithMessagesDemo;
The child components - Groups and Messages - both have their own set of action events.
The Action of the components can be overridden through the use of the Configurations object of its components. Here is an example code snippet.
- TypeScript
- JavaScript
import {
CometChatGroupsWithMessages,
MessageHeaderConfiguration,
MessagesConfiguration,
GroupsConfiguration,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
function handleOnItemClick(group: CometChat.Group): void {
console.log(group, "your custom on item click action");
}
function handleOnBack() {
console.log("your custom on back action");
}
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
onItemClick: handleOnItemClick,
})
}
messagesConfiguration={
new MessagesConfiguration({
messageHeaderConfiguration: new MessageHeaderConfiguration({
onBack: handleOnBack,
}),
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import {
CometChatGroupsWithMessages,
MessageHeaderConfiguration,
MessagesConfiguration,
GroupsConfiguration,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
function handleOnItemClick(group) {
console.log(group, "your custom on item click action");
}
function handleOnBack() {
console.log("your custom on back action");
}
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
onItemClick: handleOnItemClick,
})
}
messagesConfiguration={
new MessagesConfiguration({
messageHeaderConfiguration: new MessageHeaderConfiguration({
onBack: handleOnBack,
}),
})
}
/>
);
};
export default GroupsWithMessagesDemo;
The GroupsWithMessages component overrides several actions from its components to reach its default behavior. The list of actions overridden by GroupsWithMessages includes:
- OnItemClick : By overriding the
OnItemClick
of the Groups Component, GroupsWithMessages achieves navigation from Groups to Messages component.
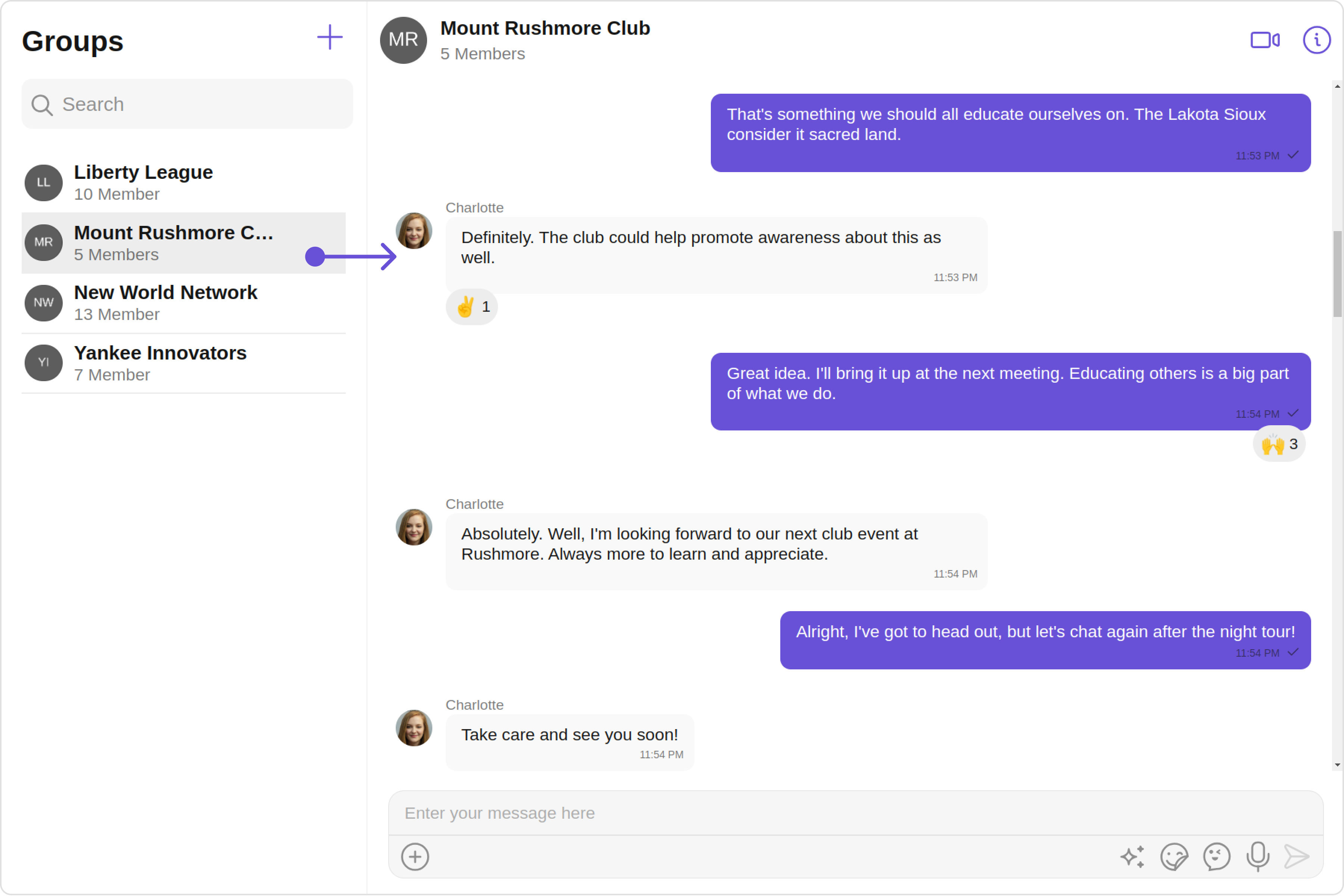
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
While the GroupsWithMessages component does not have filters, its components do, For more detail on individual filters of its component refer to Groups Filters and Messages Filters.
By utilizing the Configurations object of its components, you can apply filters.
In the following example, we are applying a filter to the Group List based on only joined groups and setting the limit to 3 using the groupsRequestBuilder
.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
groupsRequestBuilder: new CometChat.GroupsRequestBuilder()
.setLimit(3)
.joinedOnly(true),
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
groupsRequestBuilder: new CometChat.GroupsRequestBuilder()
.setLimit(3)
.joinedOnly(true),
})
}
/>
);
};
export default GroupsWithMessagesDemo;
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The GroupsWithMessages does not produce any events but its component does.
Customization
To fit your app's design requirements, you have the ability to customize the appearance of the GroupsWithMessages component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. GroupsWithMessages Style
You can set the groupsWithMessagesStyle
to the GroupsWithMessages Component to customize the styling.
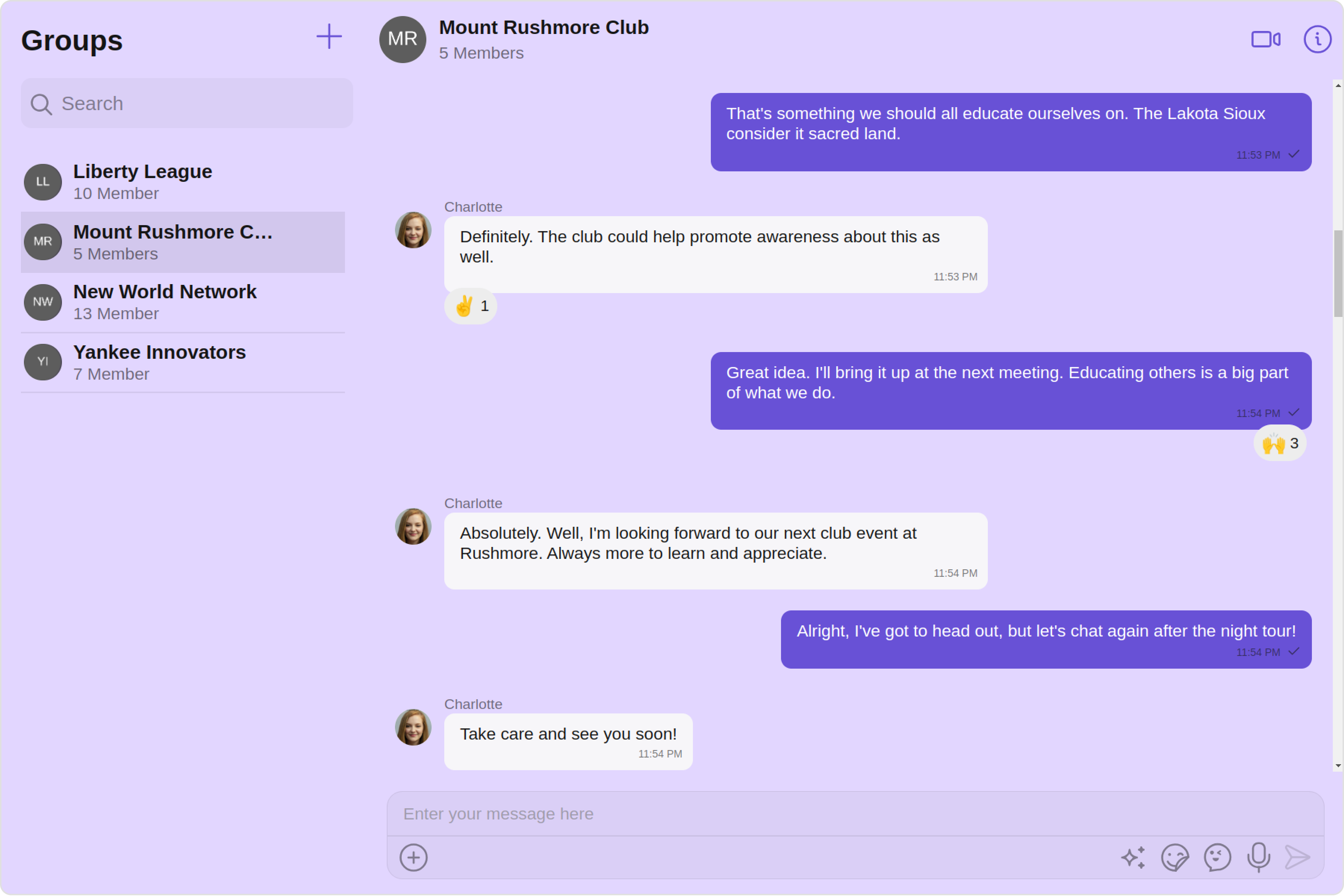
- TypeScript
- JavaScript
import {
CometChatGroupsWithMessages,
WithMessagesStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const groupsWithMessagesStyle = new WithMessagesStyle({
background: "#e2d6ff",
messageTextColor: "#000000",
borderRadius: "12px",
});
return (
<CometChatGroupsWithMessages
groupsWithMessagesStyle={groupsWithMessagesStyle}
/>
);
};
export default GroupsWithMessagesDemo;
import {
CometChatGroupsWithMessages,
WithMessagesStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const groupsWithMessagesStyle = new WithMessagesStyle({
background: "#e2d6ff",
messageTextColor: "#000000",
borderRadius: "12px",
});
return (
<CometChatGroupsWithMessages
groupsWithMessagesStyle={groupsWithMessagesStyle}
/>
);
};
export default GroupsWithMessagesDemo;
You can also customize its component styles. For more details on individual component styles, you can refer Groups Styles and Messages Styles.
Styles can be applied to SubComponents using their respective configurations.
Example
- TypeScript
- JavaScript
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
MessagesConfiguration,
GroupsStyle,
MessagesStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const groupsStyle = new GroupsStyle({
width: "100%",
height: "100%",
border: "1px solid #ee7752",
background: "linear-gradient(#ee7752, #e73c7e, #23a6d5, #23d5ab)",
});
const messagesStyle = new MessagesStyle({
width: "100%",
height: "100%",
border: "3px solid #cb1fff",
background: "linear-gradient(#885df5, #d396ff, #e497f0, #dd20fa)",
messageTextColor: "yellow",
});
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
groupsStyle: groupsStyle,
})
}
messagesConfiguration={
new MessagesConfiguration({
messagesStyle: messagesStyle,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
MessagesConfiguration,
GroupsStyle,
MessagesStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const groupsStyle = new GroupsStyle({
width: "100%",
height: "100%",
border: "1px solid #ee7752",
background: "linear-gradient(#ee7752, #e73c7e, #23a6d5, #23d5ab)",
});
const messagesStyle = new MessagesStyle({
width: "100%",
height: "100%",
border: "3px solid #cb1fff",
background: "linear-gradient(#885df5, #d396ff, #e497f0, #dd20fa)",
messageTextColor: "yellow",
});
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
groupsStyle: groupsStyle,
})
}
messagesConfiguration={
new MessagesConfiguration({
messagesStyle: messagesStyle,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
you can utilize the group
method with a Group object as input to the GroupsWithMessages component. This will automatically direct you to the Messages component for the specified Group
.
1. Group
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatGroupsWithMessages } from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("guid").then((group) => {
setChatGroup(group);
});
}, []);
return <CometChatGroupsWithMessages group={chatGroup} />;
};
export default GroupsWithMessagesDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatGroupsWithMessages } from "@cometchat/chat-uikit-react";
import React, { useEffect, useState } from "react";
const GroupsWithMessagesDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
useEffect(() => {
CometChat.getGroup("guid").then((group) => {
setChatGroup(group);
});
}, []);
return <CometChatGroupsWithMessages group={chatGroup} />;
};
export default GroupsWithMessagesDemo;
Below is a list of customizations along with corresponding code snippets:
Property | Description | Code |
---|---|---|
isMobileView | A boolean indicating if the component should render in mobile view for optimized display on mobile devices. | isMobileView: false |
group | Use the group property with a Group object as input for the GroupsWithMessages component to navigate directly to the Messages component for the specified Group. | group={chatGroup} |
messageText | It represents the textual content which will be replaced with the messages component when user clicks on a particular group chat. | messageText="Your Custom Message Text" |
Components
Nearly all functionality customizations available for a Component are also available for the composite component. Using Configuration, you can modify the properties of its components to suit your needs.
You can find the list of all Functionality customization of individual components in Groups and Messages.
Example
- TypeScript
- JavaScript
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
MessagesConfiguration,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
hideSearch: true,
})
}
messagesConfiguration={
new MessagesConfiguration({
disableTyping: true,
hideMessageHeader: true,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
MessagesConfiguration,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
hideSearch: true,
})
}
messagesConfiguration={
new MessagesConfiguration({
disableTyping: true,
hideMessageHeader: true,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your own views, layouts, and UI elements and then incorporate those into the component.
By utilizing the Configuration object of each component, you can apply advanced-level customizations to the GroupsWithMessages.
Example
- TypeScript
- JavaScript
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const getErrorStateView = () => {
return (
<div style={{ height: "100vh", width: "100vw" }}>
<img
src="image"
alt="error icon"
style={{
height: "100px",
width: "100px",
marginTop: "250px",
justifyContent: "center",
}}
></img>
</div>
);
};
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
errorStateView: getErrorStateView(),
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const getErrorStateView = () => {
return (
<div style={{ height: "100vh", width: "100vw" }}>
<img
src="image"
alt="error icon"
style={{
height: "100px",
width: "100px",
marginTop: "250px",
justifyContent: "center",
}}
></img>
</div>
);
};
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
errorStateView: getErrorStateView(),
})
}
/>
);
};
export default GroupsWithMessagesDemo;
To find all the details on individual Component advance customization you can refer, Groups Advance and Messages Advance.
GroupsWithMessages uses advanced-level customization of both Groups & Messages components to achieve its default behavior.
- GroupsWithMessages utilizes the onItemClick property of the
Groups
subcomponent to navigate the group from Groups to Messages
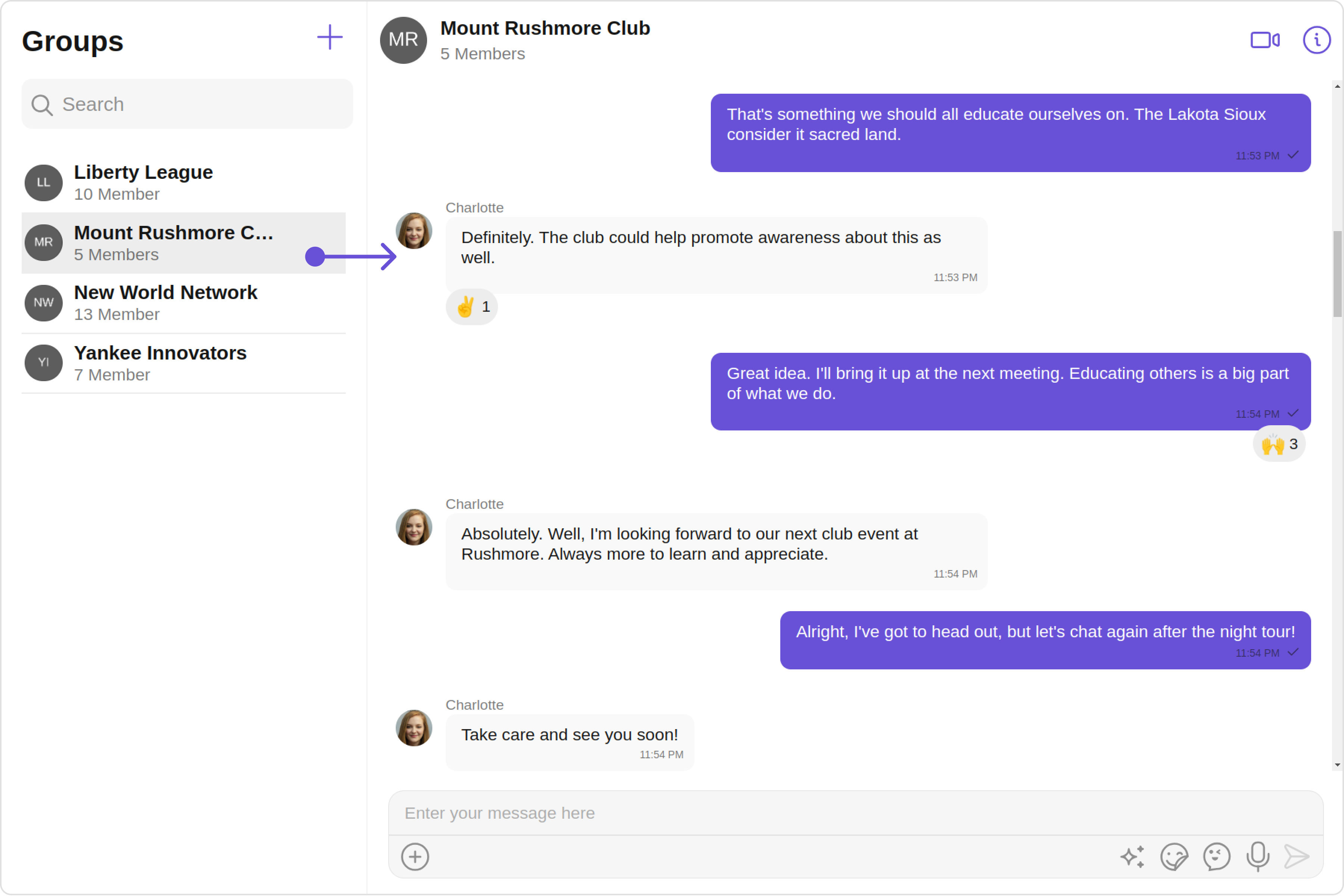
- GroupsWithMessages utilizes the menus of the
Messages
subcomponent to navigate from Messages to Details
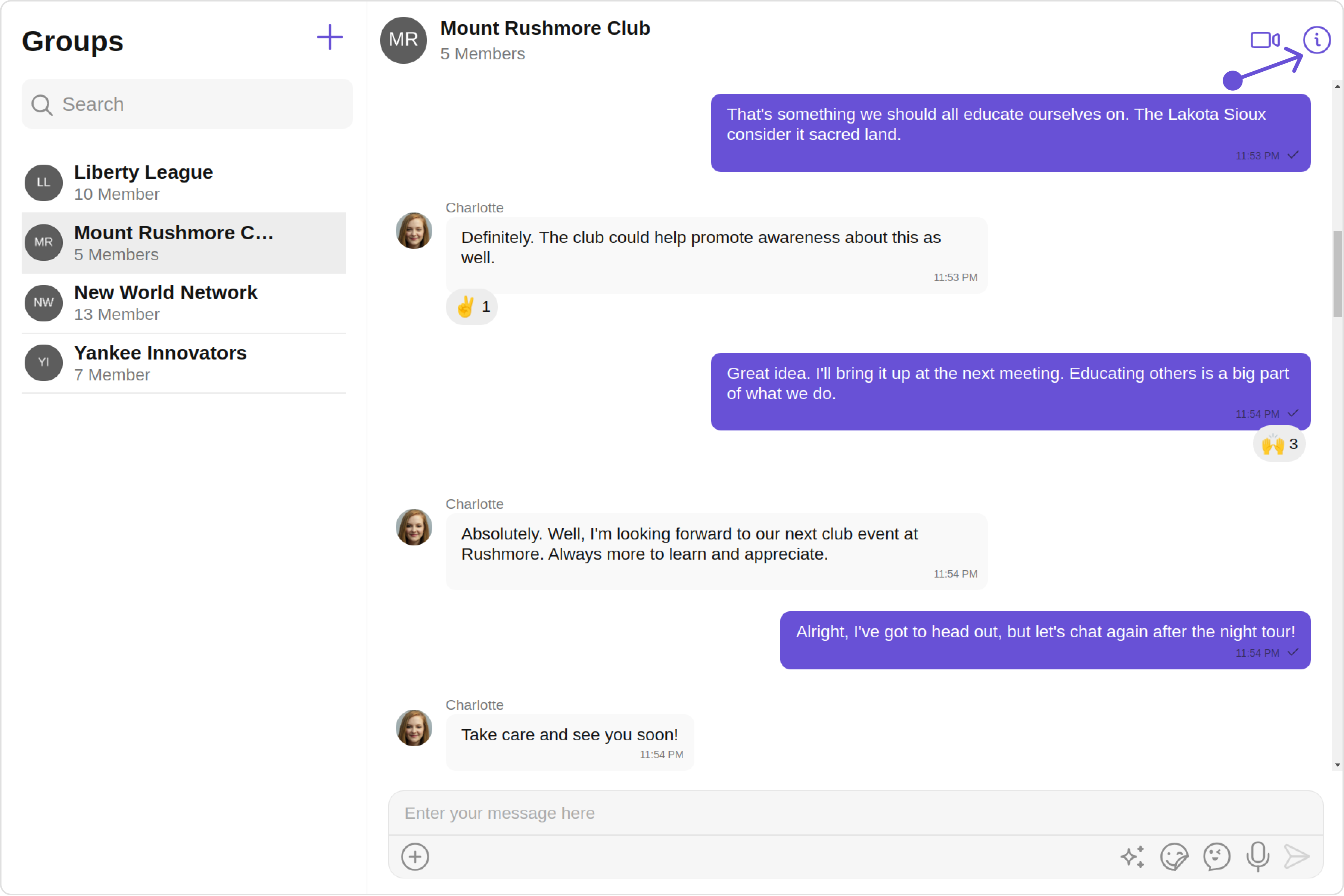
When you override onItemClick
and menus
, the default behavior of GroupsWithMessages will also be overridden.
Configurations
Configurations offer the ability to customize the properties of each component within a Composite Component.
GroupsWithMessages has Groups
and Messages
component. Hence, each of these components will have its individual `Configuration``.
Configurations
expose properties that are available in its individual components.
Groups
You can customize the properties of the Groups component by making use of the groupsConfiguration
. You can accomplish this by employing the groupsConfiguration
props as demonstrated below:
- TypeScript
- JavaScript
groupsConfiguration={new GroupsConfiguration({
//override properties of groups
})}
groupsConfiguration={new GroupsConfiguration({
//override properties of groups
})}
All exposed properties of GroupsConfiguration
can be found under Groups. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Groups subcomponent and, in addition, you only want to display the Group List based on only joined groups and setting the limit to 3.
You can modify the style using the groupsStyle
property and filter the list with the groupsRequestBuilder
property.
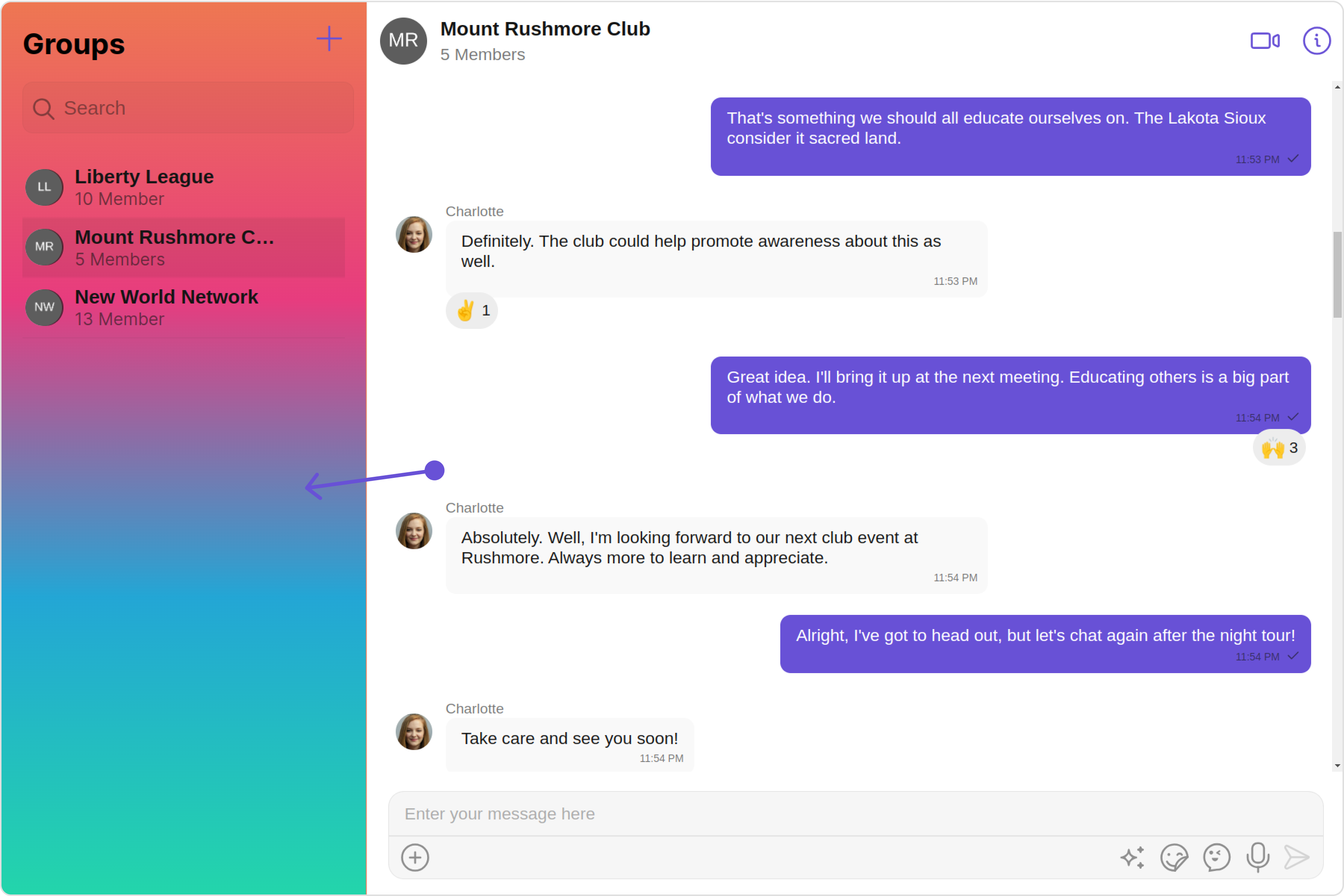
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
GroupsStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const groupsStyle = new GroupsStyle({
width: "100%",
height: "100%",
border: "1px solid #ee7752",
background: "linear-gradient(#ee7752, #e73c7e, #23a6d5, #23d5ab)",
});
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
groupsStyle: groupsStyle,
groupsRequestBuilder: new CometChat.GroupsRequestBuilder()
.setLimit(3)
.joinedOnly(true),
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
GroupsConfiguration,
GroupsStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const groupsStyle = new GroupsStyle({
width: "100%",
height: "100%",
border: "1px solid #ee7752",
background: "linear-gradient(#ee7752, #e73c7e, #23a6d5, #23d5ab)",
});
return (
<CometChatGroupsWithMessages
groupsConfiguration={
new GroupsConfiguration({
groupsStyle: groupsStyle,
groupsRequestBuilder: new CometChat.GroupsRequestBuilder()
.setLimit(3)
.joinedOnly(true),
})
}
/>
);
};
export default GroupsWithMessagesDemo;
Messages
You can customize the properties of the Messages component by making use of the messagesConfiguration. You can accomplish this by employing the messagesConfiguration
props as demonstrated below:
- TypeScript
- JavaScript
messagesConfiguration={new MessagesConfiguration({
//override properties of messages
})}
messagesConfiguration={new MessagesConfiguration({
//override properties of messages
})}
All exposed properties of MessagesConfiguration
can be found under Messages. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Messages subcomponent and, in addition, you only want to hide message header.
You can modify the style using the messagesStyle
property and hide the message header with the hideMessageHeader
property.
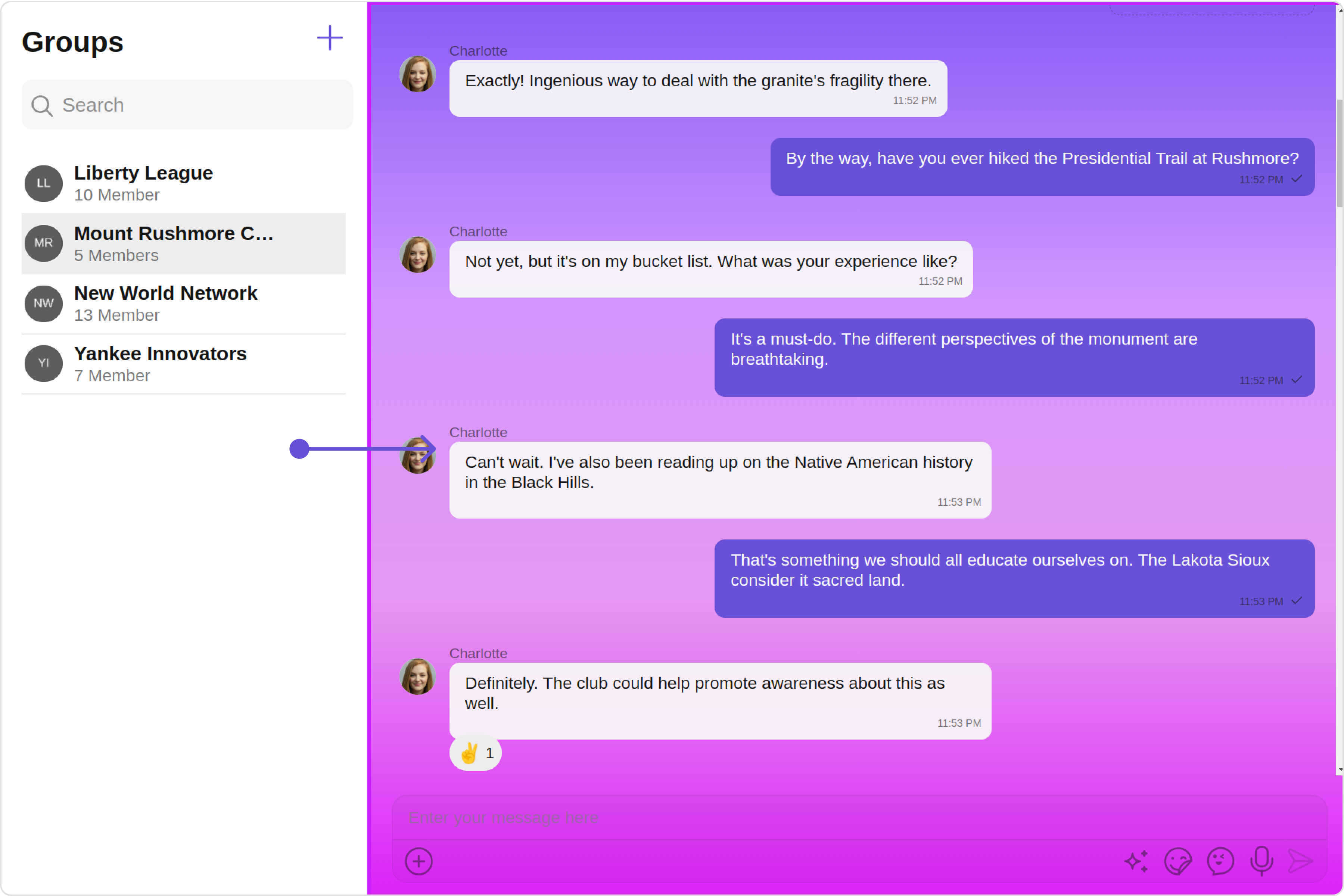
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
MessagesConfiguration,
MessagesStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const messagesStyle = new MessagesStyle({
width: "100%",
height: "100%",
border: "1px solid #ee7752",
background: "linear-gradient(#ee7752, #e73c7e, #23a6d5, #23d5ab)",
messageTextColor: "yellow",
});
return (
<CometChatGroupsWithMessages
messagesConfiguration={
new MessagesConfiguration({
hideMessageHeader: true,
messagesStyle: messagesStyle,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
MessagesConfiguration,
MessagesStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const messagesStyle = new MessagesStyle({
width: "100%",
height: "100%",
border: "1px solid #ee7752",
background: "linear-gradient(#ee7752, #e73c7e, #23a6d5, #23d5ab)",
messageTextColor: "yellow",
});
return (
<CometChatGroupsWithMessages
messagesConfiguration={
new MessagesConfiguration({
hideMessageHeader: true,
messagesStyle: messagesStyle,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
Join Group
You can customize the properties of the Join Group component by making use of the JoinGroupConfiguration. You can accomplish this by employing the joinGroupConfiguration
props as demonstrated below:
- TypeScript
- JavaScript
joinGroupConfiguration={new JoinGroupConfiguration({
//override properties of join group
})}
joinGroupConfiguration={new JoinGroupConfiguration({
//override properties of join group
})}
All exposed properties of JoinGroupConfiguration
can be found under Join Group. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Join Group subcomponent.
You can modify the style using the joinGroupStyle
property.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
JoinGroupConfiguration,
JoinGroupStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const joinGroupStyle = new JoinGroupStyle({
background: "#e2d6ff",
joinButtonBackground: "#cb1fff",
joinButtonTextColor: "#000000",
});
return (
<CometChatGroupsWithMessages
joinGroupConfiguration={
new JoinGroupConfiguration({
joinGroupStyle: joinGroupStyle,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
JoinGroupConfiguration,
JoinGroupStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const joinGroupStyle = new JoinGroupStyle({
background: "#e2d6ff",
joinButtonBackground: "#cb1fff",
joinButtonTextColor: "#000000",
});
return (
<CometChatGroupsWithMessages
joinGroupConfiguration={
new JoinGroupConfiguration({
joinGroupStyle: joinGroupStyle,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
Create Group
You can customize the properties of the Create Group component by making use of the CreateGroupConfiguration. You can accomplish this by employing the createGroupConfiguration
props as demonstrated below:
- TypeScript
- JavaScript
createGroupConfiguration={new CreateGroupConfiguration({
//override properties of create group
})}
createGroupConfiguration={new CreateGroupConfiguration({
//override properties of create group
})}
All exposed properties of CreateGroupConfiguration
can be found under Create Group. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Create Group subcomponent.
You can modify the style using the createGroupStyle
property.
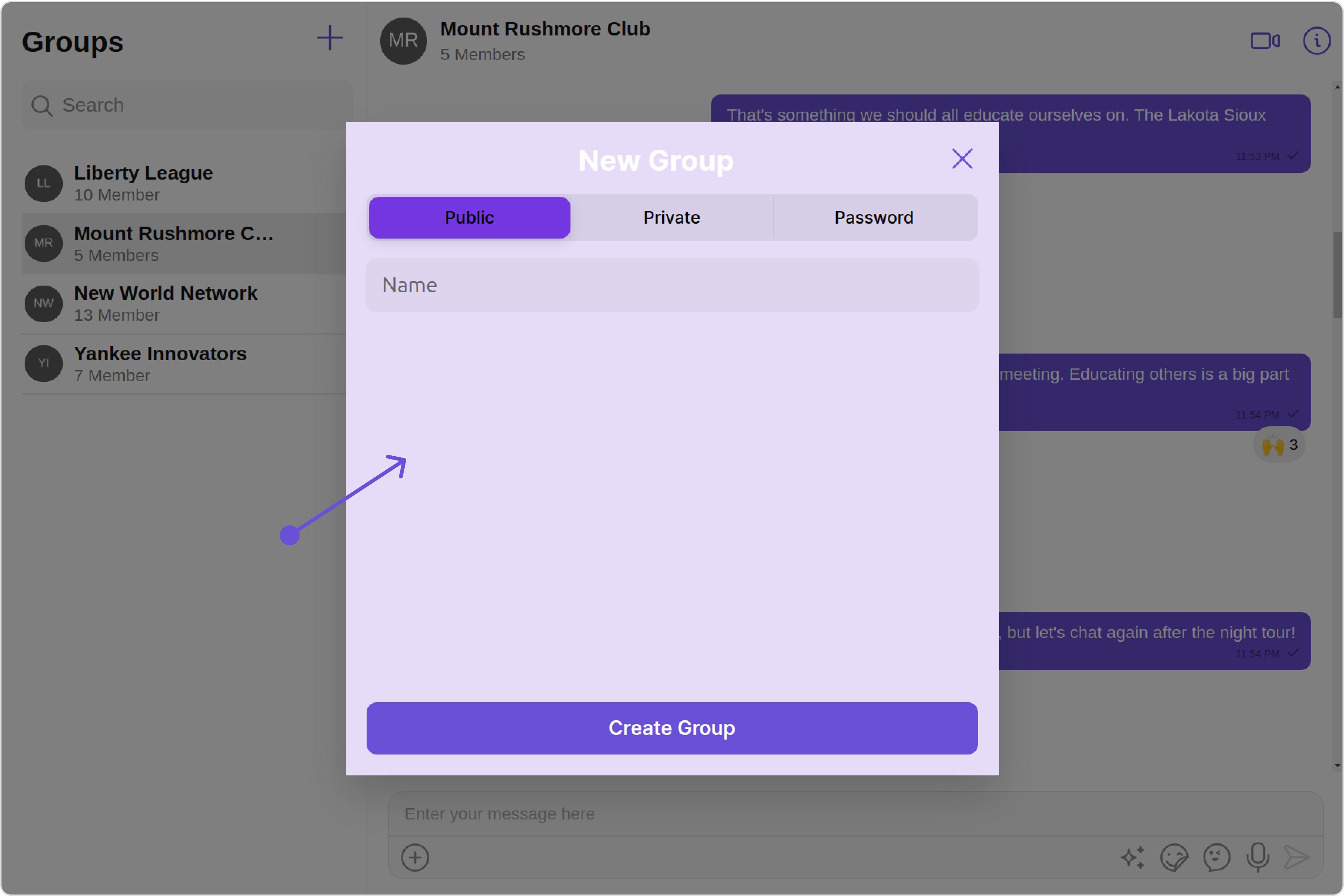
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
CreateGroupConfiguration,
CreateGroupStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const createGroupStyle = new CreateGroupStyle({
background: "#e6dcf7",
activeGroupTypeBackground: "#7436e0",
titleTextColor: "#ffffff",
height: "500px",
width: "500px",
});
return (
<CometChatGroupsWithMessages
createGroupConfiguration={
new CreateGroupConfiguration({
createGroupStyle: createGroupStyle,
})
}
/>
);
};
export default GroupsWithMessagesDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatGroupsWithMessages,
CreateGroupConfiguration,
CreateGroupStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const GroupsWithMessagesDemo = () => {
const createGroupStyle = new CreateGroupStyle({
background: "#e6dcf7",
activeGroupTypeBackground: "#7436e0",
titleTextColor: "#ffffff",
height: "500px",
width: "500px",
});
return (
<CometChatGroupsWithMessages
createGroupConfiguration={
new CreateGroupConfiguration({
createGroupStyle: createGroupStyle,
})
}
/>
);
};
export default GroupsWithMessagesDemo;