Banned Members
Overview
CometChatBannedMembers
is a Component that displays all the users who have been restricted or prohibited from participating in specific groups or conversations. When the user is banned, they are no longer able to access or engage with the content and discussions within the banned group. Group administrators or owners have the authority to ban members from specific groups they manage. They can review user activity, monitor behavior, and take appropriate actions, including banning users when necessary.
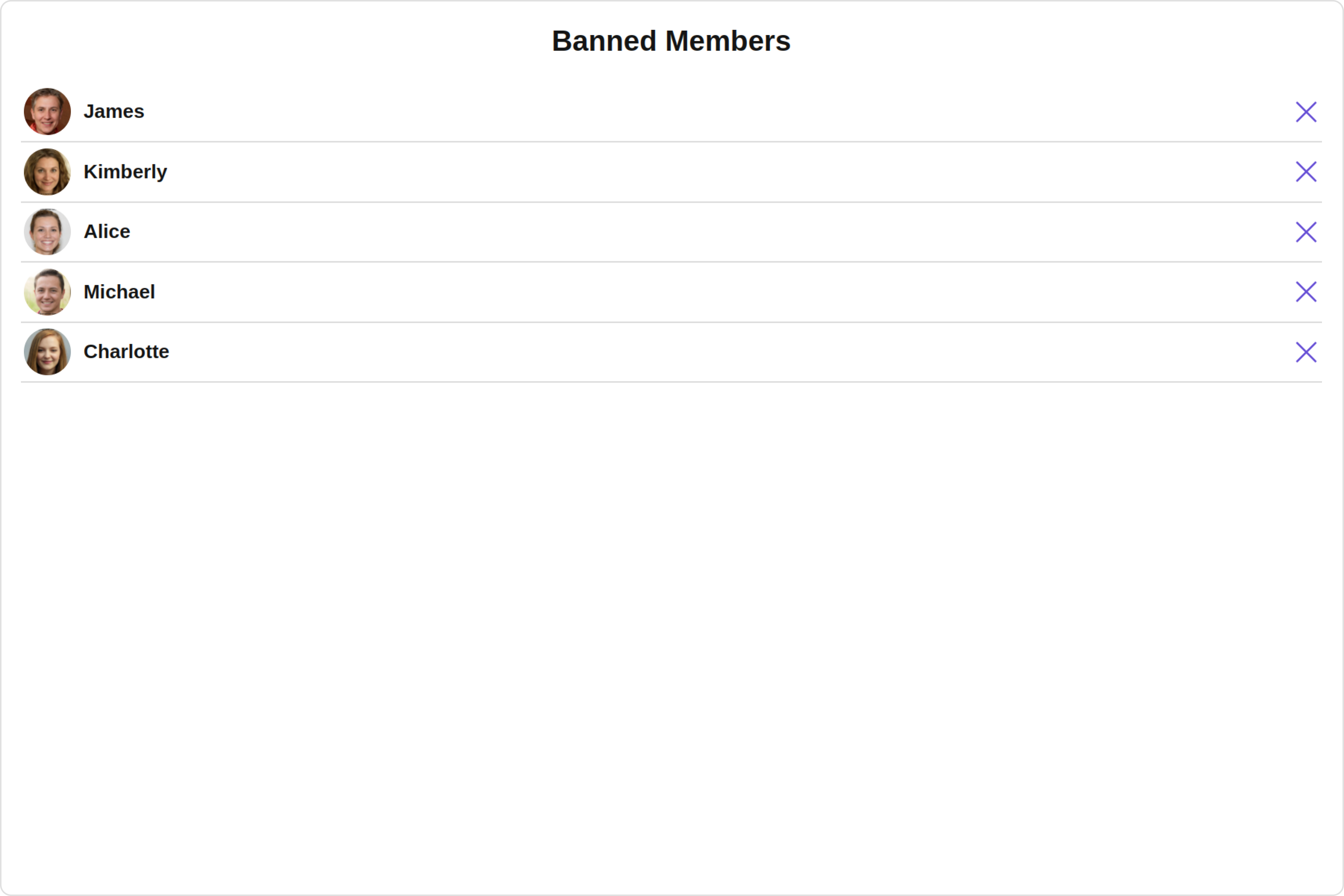
Usage
Integration
The following code snippet illustrates how you can directly incorporate the Banned Members component into your Application.
- BannedMembersDemo.tsx
- App.tsx
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { CometChatBannedMembers } from '@cometchat/chat-uikit-react'
import React from 'react'
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<CometChat.Group | undefined>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
})
}, []);
return (
<>
{
chatGroup &&
<CometChatBannedMembers
group={chatGroup}
/>
}
</>
)
}
export default BannedMembersDemo;
import { BannedMembersDemo } from "./BannedMembersDemo";
export default function App() {
return (
<div className="App">
<BannedMembersDemo />
</div>
);
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onSelect
The onSelect
action is activated when you select the done icon while in selection mode. This returns a list of all the banned members that you have selected.
This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnSelect(
bannedMember: CometChat.GroupMember,
selected: boolean
): void {
console.log(bannedMember);
//your custom onSelect actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} onSelect={handleOnSelect} />
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnSelect(bannedMember, selected) {
console.log(bannedMember);
//your custom onSelect actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} onSelect={handleOnSelect} />
)}
</>
);
};
export default BannedMembersDemo;
2. onItemClick
The OnItemClick
event is activated when you click on the Banned Members List item. This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnItemClick(bannedMember: CometChat.GroupMember): void {
console.log(bannedMember);
//Your Custom on item click actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
onItemClick={handleOnItemClick}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnItemClick(bannedMember) {
console.log(bannedMember);
//Your Custom on item click actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
onItemClick={handleOnItemClick}
/>
)}
</>
);
};
export default BannedMembersDemo;
3. OnBack
OnBack
is triggered when you click on the back button of the Banned Members component. You can override this action using the following code snippet.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnBack(): void {
//Your Custom onBack actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} onBack={handleOnBack} />
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnBack() {
//Your Custom onBack actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} onBack={handleOnBack} />
)}
</>
);
};
export default BannedMembersDemo;
4. onClose
onClose
is triggered when you click on the close button of the Banned Members component. You can override this action using the following code snippet.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnClose(): void {
//Your Custom onClose actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} onClose={handleOnClose} />
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnClose() {
//Your Custom onBack actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} onClose={handleOnClose} />
)}
</>
);
};
export default BannedMembersDemo;
5. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the Banned Members component.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnError(error: CometChat.CometChatException): void {
//Your Custom onError actionssss
}
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} onError={handleOnError} />
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
function handleOnError(error) {
//Your Custom onError actions
}
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} onError={handleOnError} />
)}
</>
);
};
export default BannedMembersDemo;
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of ChatSDK.
1. BannedMembersRequestBuilder
The BannedMembersRequestBuilder enables you to filter and customize the Banned Members list based on available parameters in BannedMembersRequestBuilder. This feature allows you to create more specific and targeted queries when fetching banned members. The following are the parameters available in BannedMembersRequestBuilder
Methods | Type | Description |
---|---|---|
setLimit | number | sets the number of banned members that can be fetched in a single request, suitable for pagination |
setSearchKeyword | String | used for fetching banned members matching the passed string |
setScopes | Array<String> | used for fetching banned members based on multiple scopes |
Example
In the example below, we are applying a filter to the banned members by setting the limit to 2 and setting the scope to show only the moderator.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
bannedMembersRequestBuilder={new CometChat.BannedMembersRequestBuilder(
"guid"
)
.setLimit(2)
.setScopes(["moderator"])}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
bannedMembersRequestBuilder={new CometChat.BannedMembersRequestBuilder(
"guid"
)
.setLimit(2)
.setScopes(["moderator"])}
/>
)}
</>
);
};
export default BannedMembersDemo;
2. SearchRequestBuilder
The SearchRequestBuilder uses BannedMembersRequestBuilder enables you to filter and customize the search list based on available parameters in BannedMembersRequestBuilder. This feature allows you to keep uniformity between the displayed Banned Members list and searched Banned Members.
Example
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
searchRequestBuilder={new CometChat.BannedMembersRequestBuilder(
"guid"
)
.setLimit(2)
.setSearchKeyword("**")}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
searchRequestBuilder={new CometChat.BannedMembersRequestBuilder(
"guid"
)
.setLimit(2)
.setSearchKeyword("**")}
/>
)}
</>
);
};
export default BannedMembersDemo;
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The Banned Members
component does not produce any events.
Customization
To fit your app's design requirements, you can customize the appearance of the Groups component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. BannedMembers Style
You can set the BannedMembersStyle
to the Banned Members Component to customize the styling.
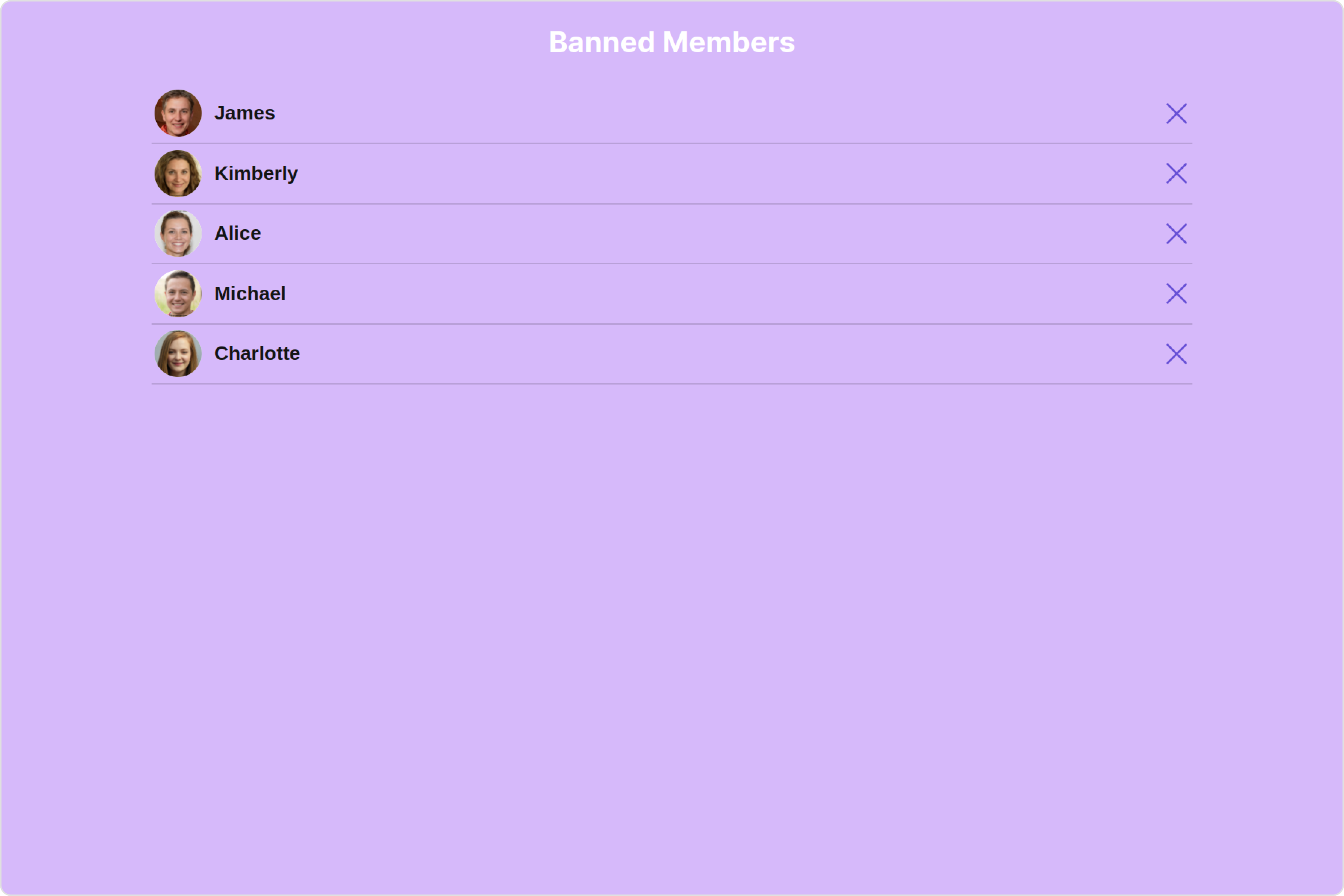
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
BannedMembersStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const bannedMemberStyle = new BannedMembersStyle({
background: "#d6b9fa",
titleTextColor: "#ffffff",
separatorColor: "#6d1fcf",
onlineStatusColor: "#b1f029",
});
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
bannedMemberStyle={bannedMemberStyle}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
BannedMembersStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const bannedMemberStyle = new BannedMembersStyle({
background: "#d6b9fa",
titleTextColor: "#ffffff",
separatorColor: "#6d1fcf",
onlineStatusColor: "#b1f029",
});
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
bannedMemberStyle={bannedMemberStyle}
/>
)}
</>
);
};
export default BannedMembersDemo;
List of properties exposed by BannedMembersStyle
Property | Description | Code |
---|---|---|
border | Used to set border | border?: string, |
borderRadius | Used to set border radius | borderRadius?: string; |
background | Used to set background colour | background?: string; |
height | Used to set height | height?: string; |
width | Used to set width | width?: string; |
titleTextFont | Used to set title text font | titleTextFont?: string, |
titleTextColor | Used to set title text color | titleTextColor?: string; |
searchPlaceholderTextFont | Used to set search placeholder font | searchPlaceholderTextFont?: string; |
searchPlaceholderTextColor | Used to set search placeholder color | searchPlaceholderTextColor?: string; |
searchTextFont | Used to set search text font | searchTextFont?: string; |
searchTextColor | Used to set search text color | searchTextColor?: string; |
emptyStateTextFont | Used to set empty state text font | emptyStateTextFont?: string; |
emptyStateTextColor | Used to set empty state text color | emptyStateTextColor?: string; |
errorStateTextFont | Used to set error state text font | errorStateTextFont?: string; |
errorStateTextColor | Used to set error state text color | errorStateTextColor?: string; |
loadingIconTint | Used to set loading icon tint | loadingIconTint?: string; |
searchIconTint | Used to set search icon tint | searchIconTint?: string; |
searchBorder | Used to set search border | searchBorder?: string; |
searchBorderRadius | Used to set search border radius | searchBorderRadius?: string; |
searchBackground | Used to set search background color | searchBackground?: string; |
onlineStatusColor | Used to set online status color | onlineStatusColor?: string; |
separatorColor | Used to set separator color | separatorColor?: string; |
boxShadow | Used to set box shadow | boxShadow?: string; |
backButtonIconTint | Used to set back button icon tint | backButtonIconTint?: string; |
closeButtonIconTint | Used to set close button icon tint | closeButtonIconTint?: string; |
unbanIconTint | Used to set unban icon tint | unbanIconTint?: string; |
padding | Used to set padding | padding?: string; |
2. Avatar Style
To apply customized styles to the Avatar
component in the Banned Members Component, you can use the following code snippet. For further insights on Avatar
Styles refer
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
AvatarStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const avatarStyle = new AvatarStyle({
backgroundColor: "#cdc2ff",
border: "2px solid #6745ff",
borderRadius: "10px",
outerViewBorderColor: "#ca45ff",
outerViewBorderRadius: "5px",
nameTextColor: "#4554ff",
});
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} avatarStyle={avatarStyle} />
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
AvatarStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const avatarStyle = new AvatarStyle({
backgroundColor: "#cdc2ff",
border: "2px solid #6745ff",
borderRadius: "10px",
outerViewBorderColor: "#ca45ff",
outerViewBorderRadius: "5px",
nameTextColor: "#4554ff",
});
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} avatarStyle={avatarStyle} />
)}
</>
);
};
export default BannedMembersDemo;
3. LisItem Style
To apply customized styles to the ListItemStyle
component in the Banned Members
Component, you can use the following code snippet. For further insights on ListItemStyle
Styles refer
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
ListItemStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const listItemStyle = new ListItemStyle({
width: "100%",
height: "100%",
border: "2px solid #cdc2ff",
});
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
listItemStyle={listItemStyle}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
ListItemStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const listItemStyle = new ListItemStyle({
width: "100%",
height: "100%",
border: "2px solid #cdc2ff",
});
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
listItemStyle={listItemStyle}
/>
)}
</>
);
};
export default BannedMembersDemo;
4. StatusIndicator Style
To apply customized styles to the Status Indicator component in the Banned Members Component, You can use the following code snippet. For further insights on Status Indicator Styles refer
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const statusIndicatorStyle = {
background: "#db35de",
height: "10px",
width: "10px",
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
statusIndicatorStyle={statusIndicatorStyle}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const statusIndicatorStyle = {
background: "#db35de",
height: "10px",
width: "10px",
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
statusIndicatorStyle={statusIndicatorStyle}
/>
)}
</>
);
};
export default BannedMembersDemo;
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
title="Your Custom Title"
titleAlignment={TitleAlignment.left}
unbanIconURL="Your Custom Unban Icon URL"
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
title="Your Custom Title"
titleAlignment={TitleAlignment.left}
unbanIconURL="Your Custom Unban Icon URL"
/>
)}
</>
);
};
export default BannedMembersDemo;
Default:
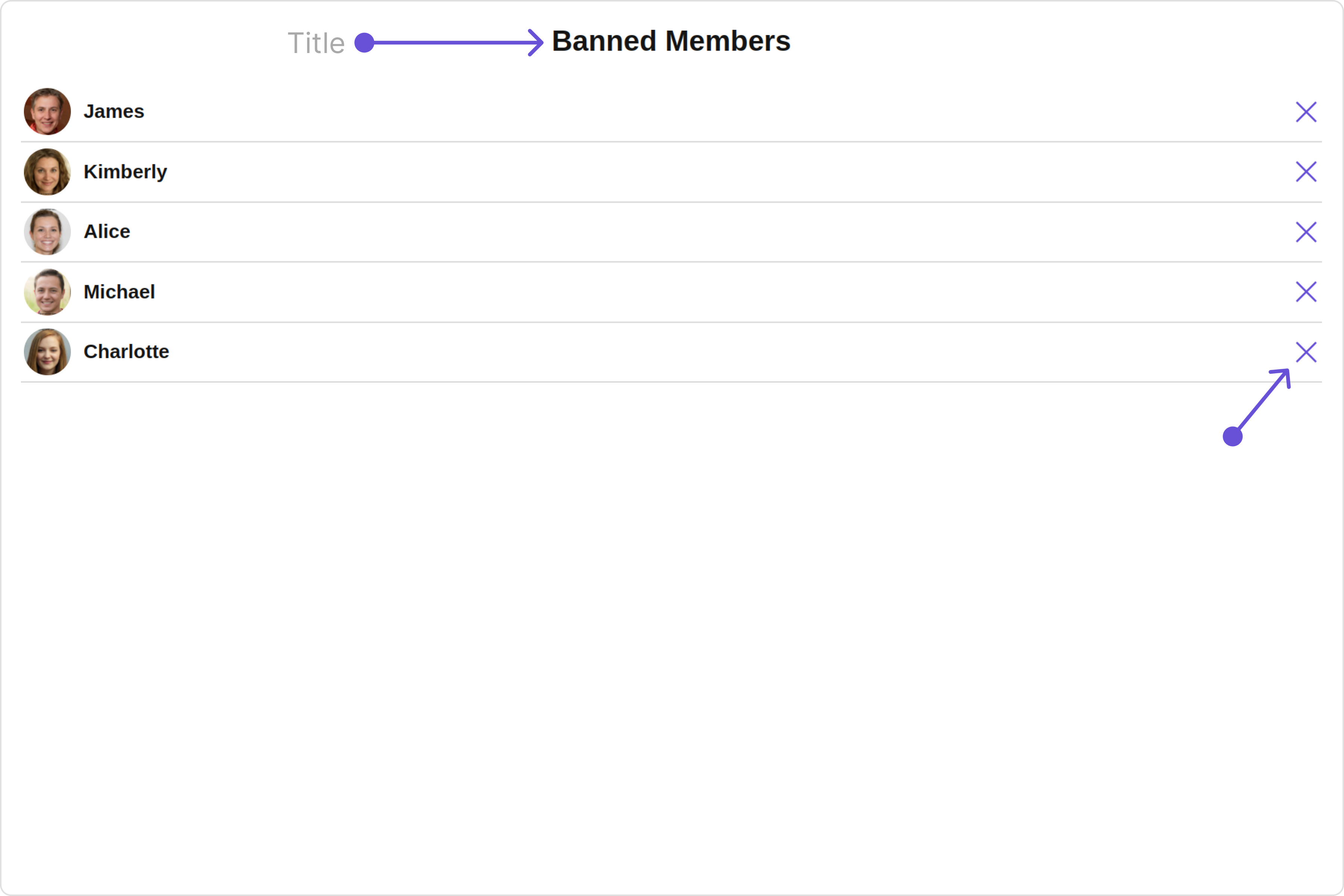
Custom:
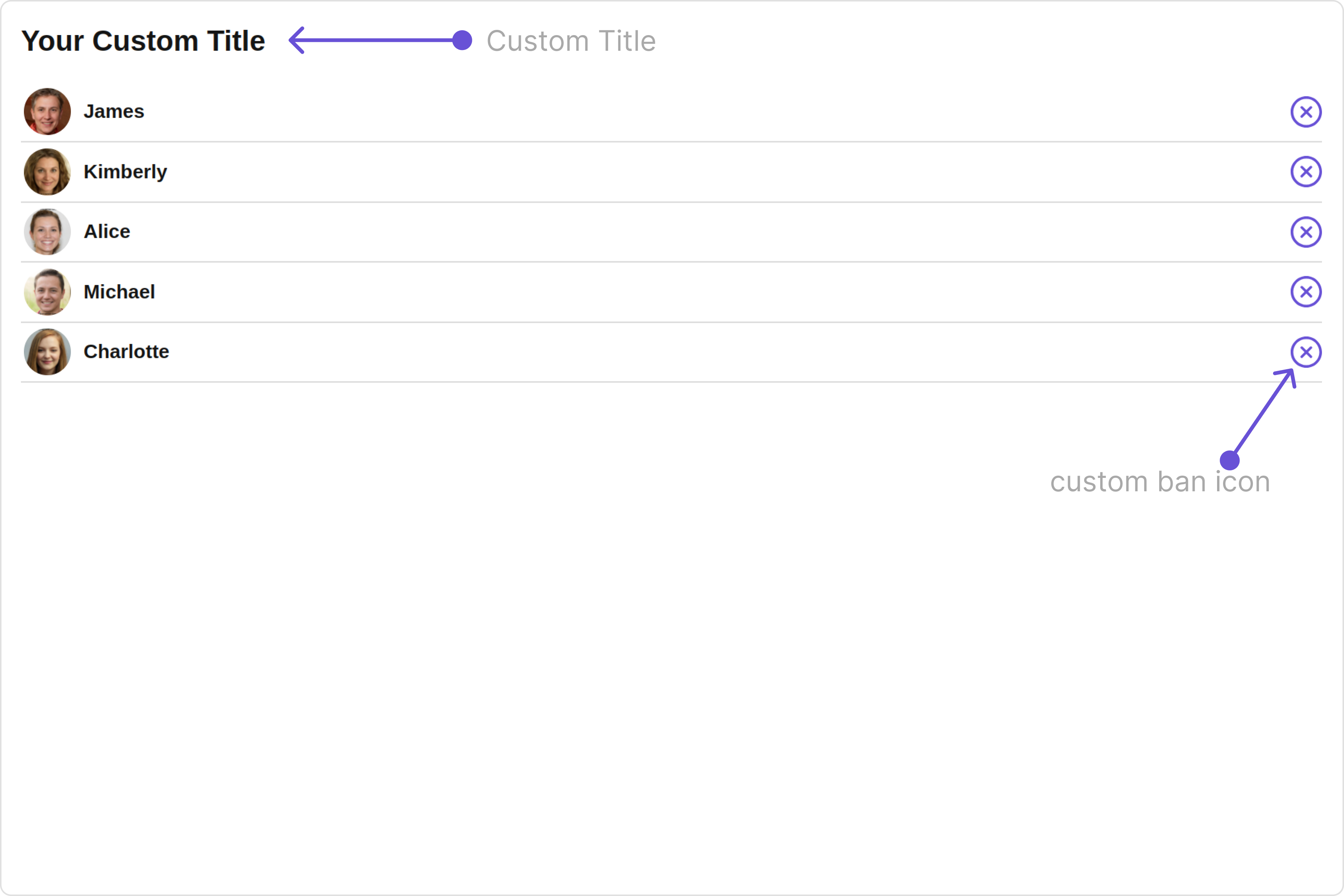
Below is a list of customizations along with corresponding code snippets
Advance
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
ListItemView
With this property, you can assign a custom ListItem to the Banned Members Component.
listItemView = { getListItemView };
Example
Default:
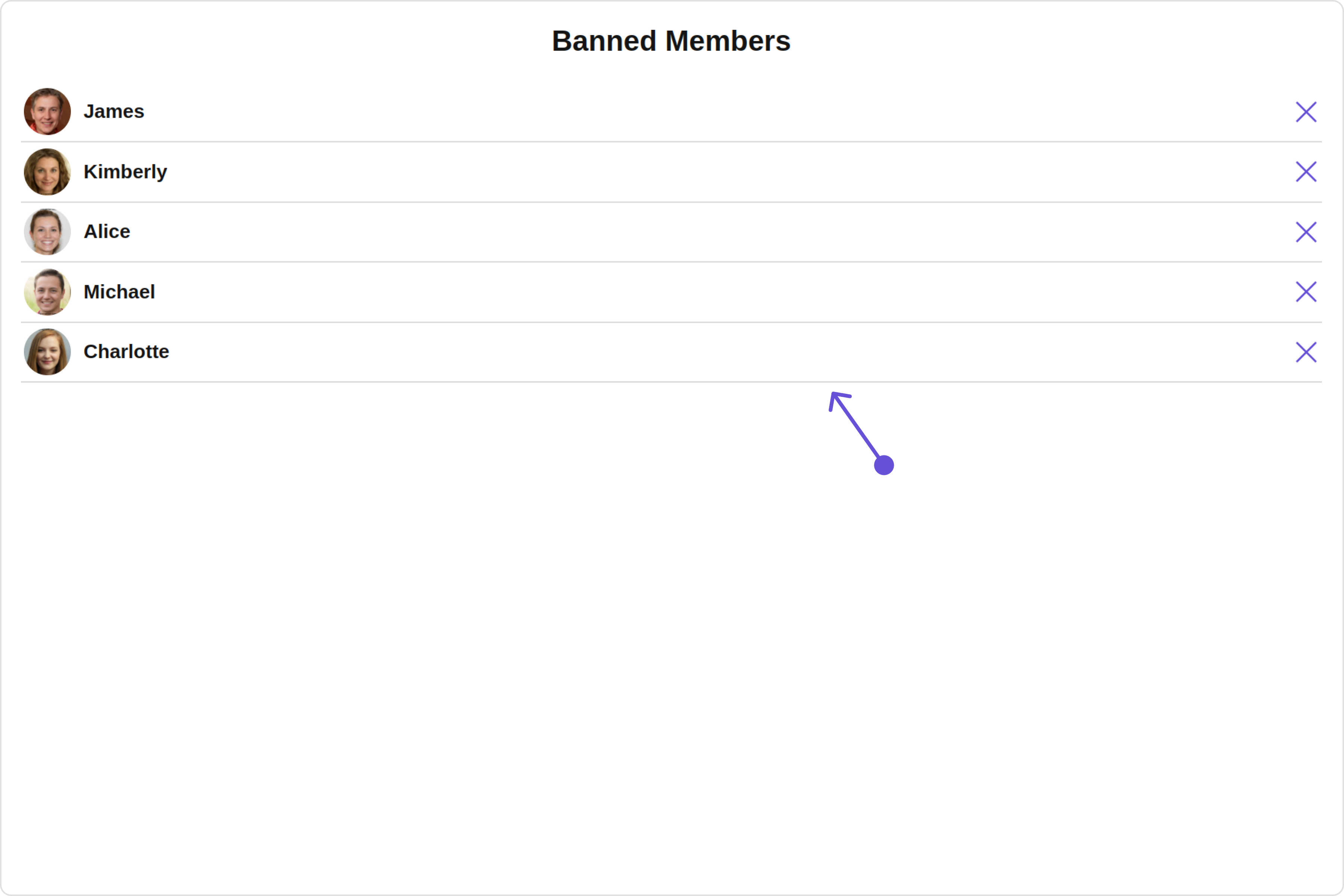
Custom:
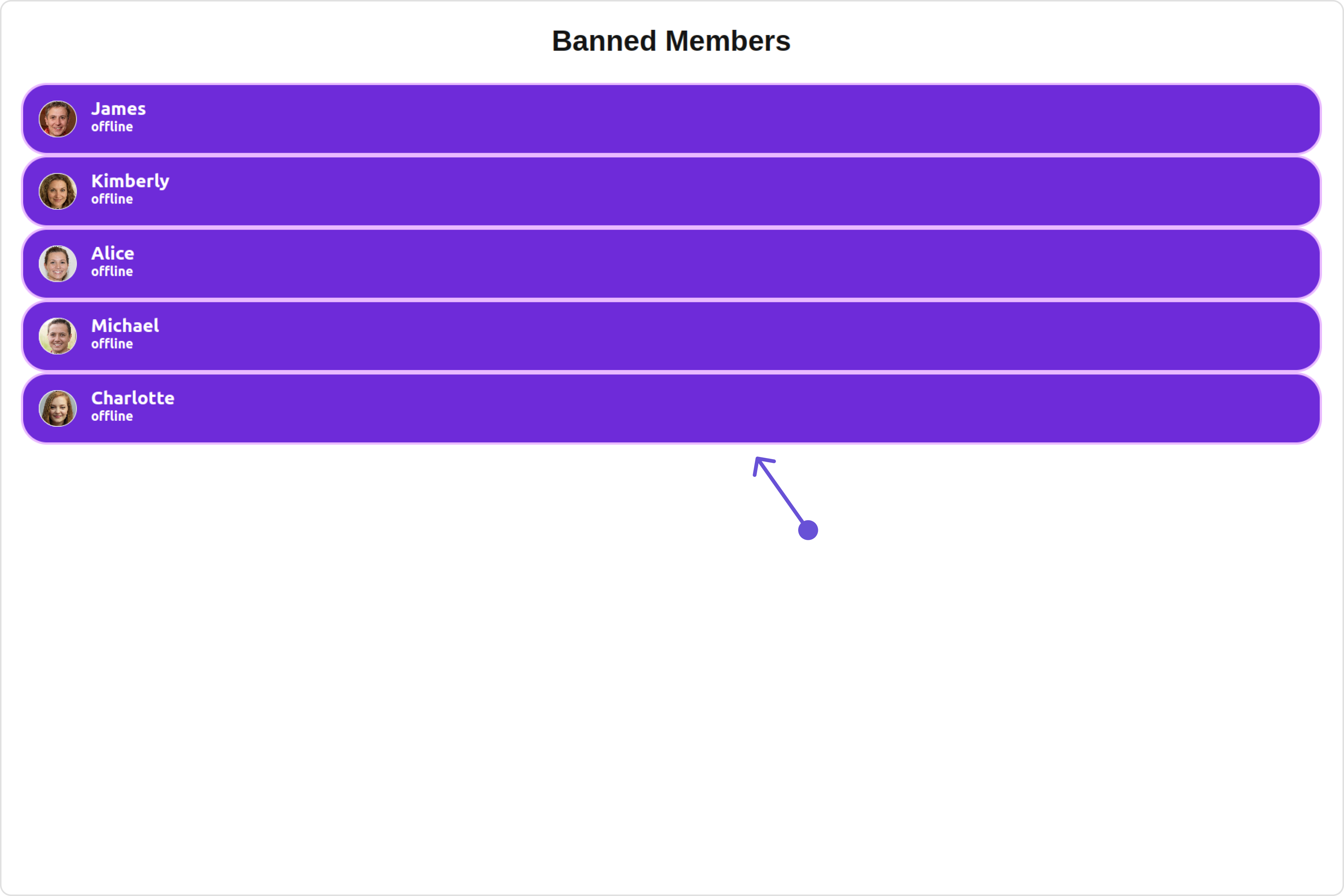
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getListItemView = (bannedMember: CometChat.GroupMember) => {
return (
<div
style={{
display: "flex",
alignItems: "left",
padding: "10px",
border: "2px solid #e9baff",
borderRadius: "20px",
background: "#6e2bd9",
}}
>
<cometchat-avatar
image={bannedMember.getAvatar()}
name={bannedMember.getName()}
/>
<div style={{ display: "flex", paddingLeft: "10px" }}>
<div
style={{ fontWeight: "bold", color: "#ffffff", fontSize: "14px" }}
>
{bannedMember.getName()}
<div
style={{ color: "#ffffff", fontSize: "10px", textAlign: "left" }}
>
{bannedMember.getStatus()}
</div>
</div>
</div>
</div>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
listItemView={getListItemView}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getListItemView = (bannedMember) => {
return (
<div
style={{
display: "flex",
alignItems: "left",
padding: "10px",
border: "2px solid #e9baff",
borderRadius: "20px",
background: "#6e2bd9",
}}
>
<cometchat-avatar
image={bannedMember.getAvatar()}
name={bannedMember.getName()}
/>
<div style={{ display: "flex", paddingLeft: "10px" }}>
<div
style={{ fontWeight: "bold", color: "#ffffff", fontSize: "14px" }}
>
{bannedMember.getName()}
<div
style={{ color: "#ffffff", fontSize: "10px", textAlign: "left" }}
>
{bannedMember.getStatus()}
</div>
</div>
</div>
</div>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
listItemView={getListItemView}
/>
)}
</>
);
};
export default BannedMembersDemo;
SubtitleView
You can customize the subtitle view for each banned members to meet your requirements
subtitleView = { getSubtitleView };
Default:
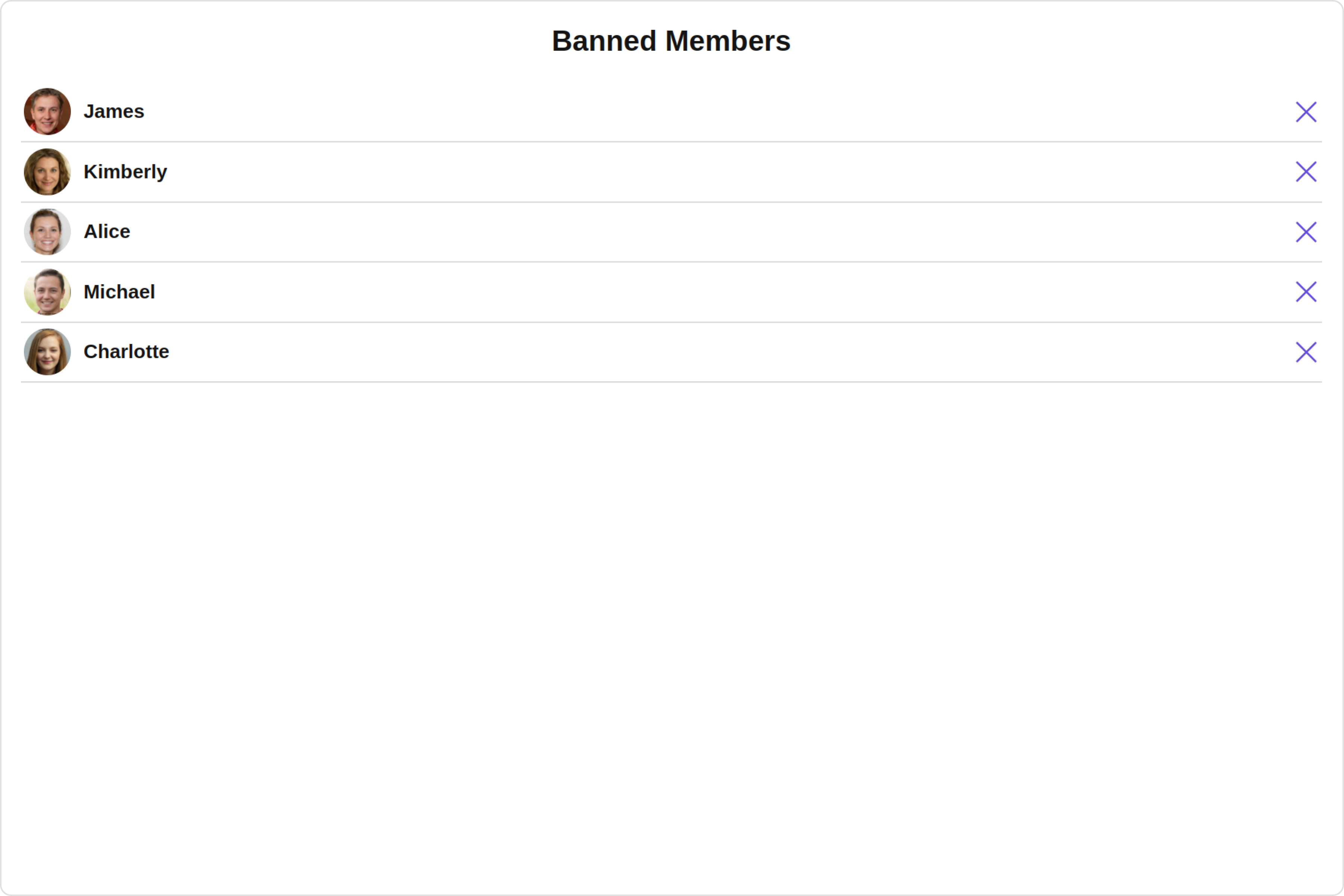
Custom:
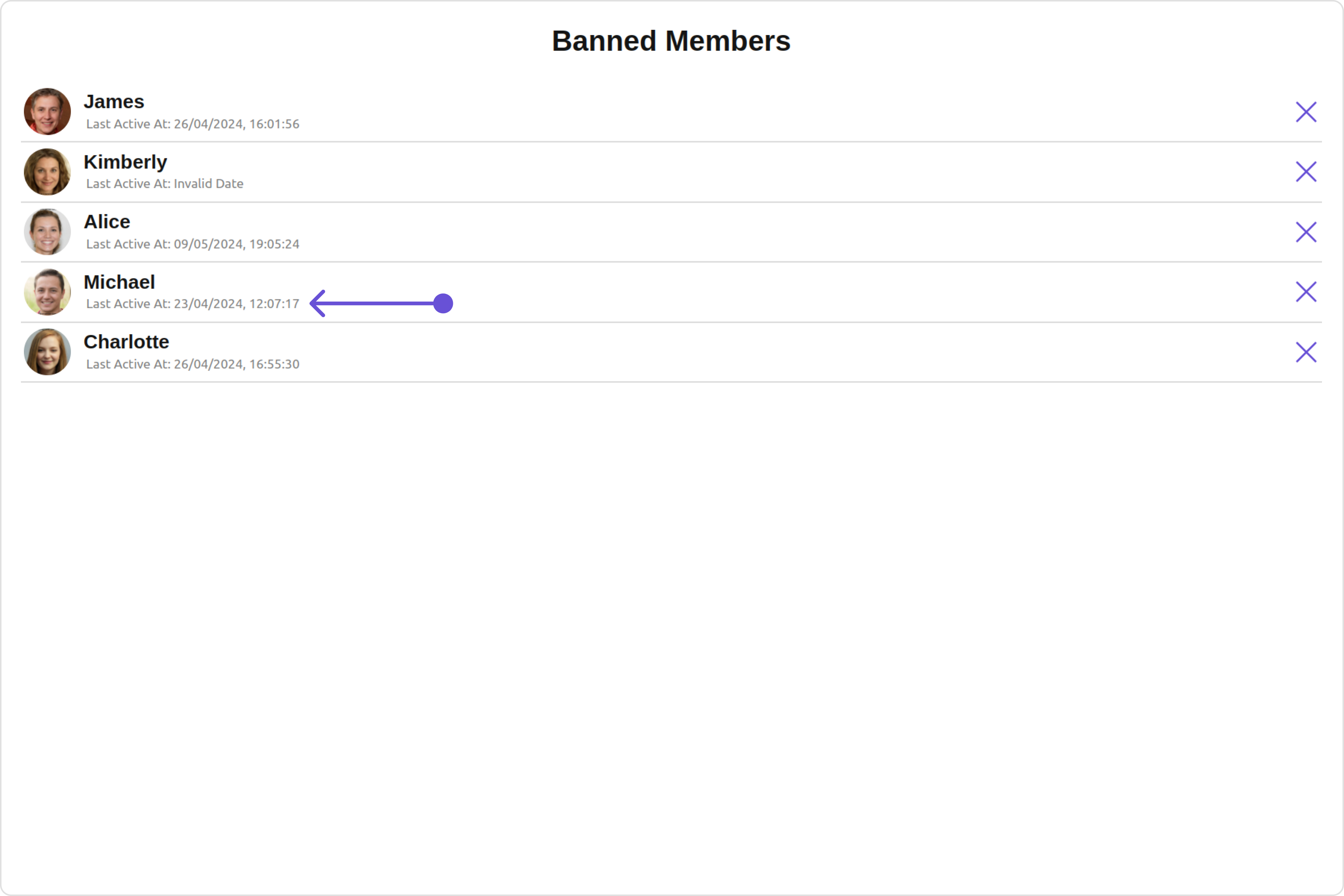
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getSubtitleView = (
bannedMember: CometChat.GroupMember
): JSX.Element => {
function formatTime(timestamp: number) {
const date = new Date(timestamp * 1000);
return date.toLocaleString();
}
if (bannedMember instanceof CometChat.GroupMember) {
return (
<div
style={{
display: "flex",
alignItems: "left",
padding: "2px",
fontSize: "10px",
}}
>
<div style={{ color: "gray" }}>
Last Active At: {formatTime(bannedMember.getLastActiveAt())}
</div>
</div>
);
} else {
return <></>;
}
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
subtitleView={getSubtitleView}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getSubtitleView = (bannedMember) => {
function formatTime(timestamp) {
const date = new Date(timestamp * 1000);
return date.toLocaleString();
}
if (bannedMember instanceof CometChat.GroupMember) {
return (
<div
style={{
display: "flex",
alignItems: "left",
padding: "2px",
fontSize: "10px",
}}
>
<div style={{ color: "gray" }}>
Last Active At: {formatTime(bannedMember.getLastActiveAt())}
</div>
</div>
);
} else {
return <></>;
}
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
subtitleView={getSubtitleView}
/>
)}
</>
);
};
export default BannedMembersDemo;
LoadingStateView
You can set a custom loader view using loadingStateView
to match the loading view of your app.
loadingStateView={getLoadingStateView()}
Default:
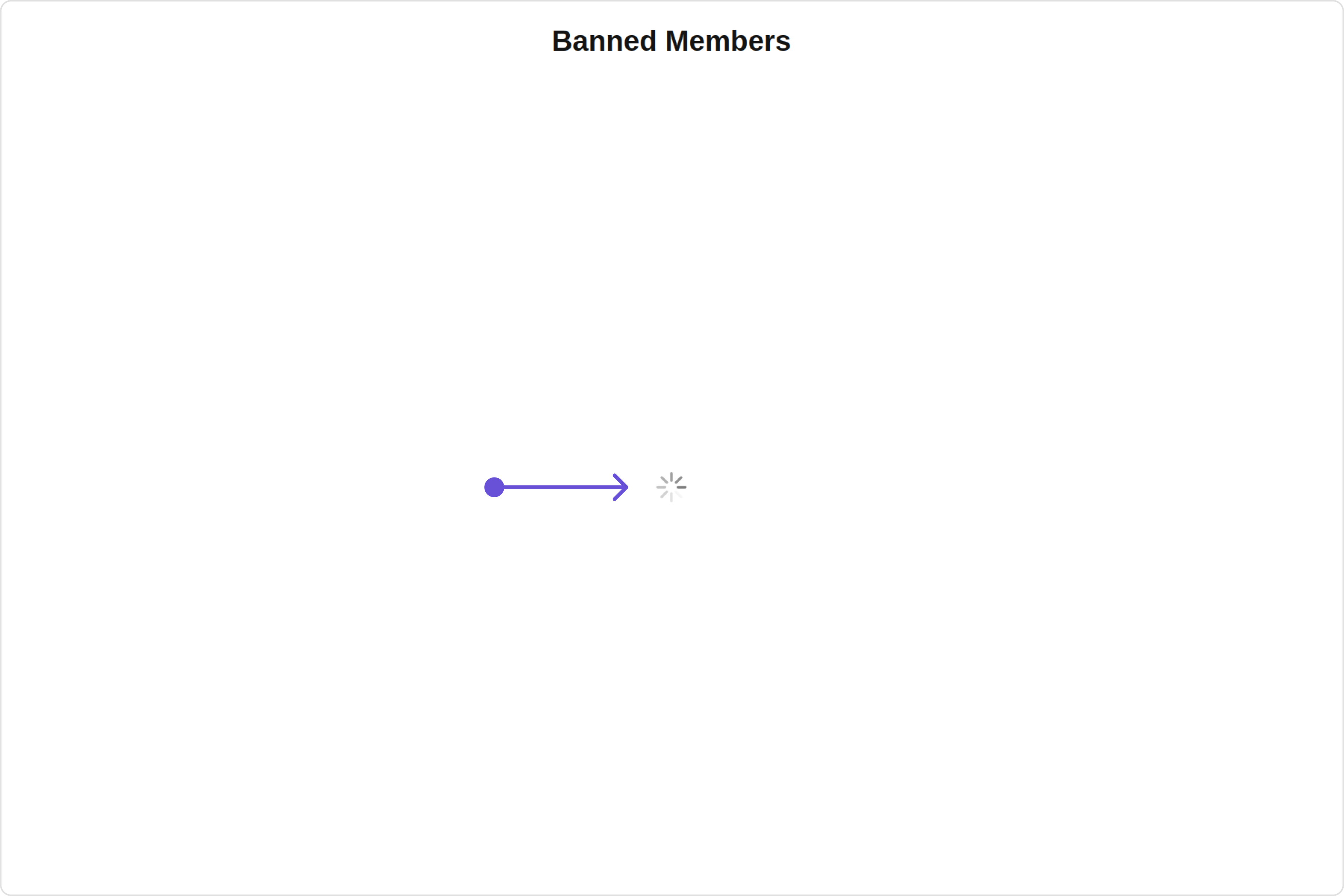
Custom:
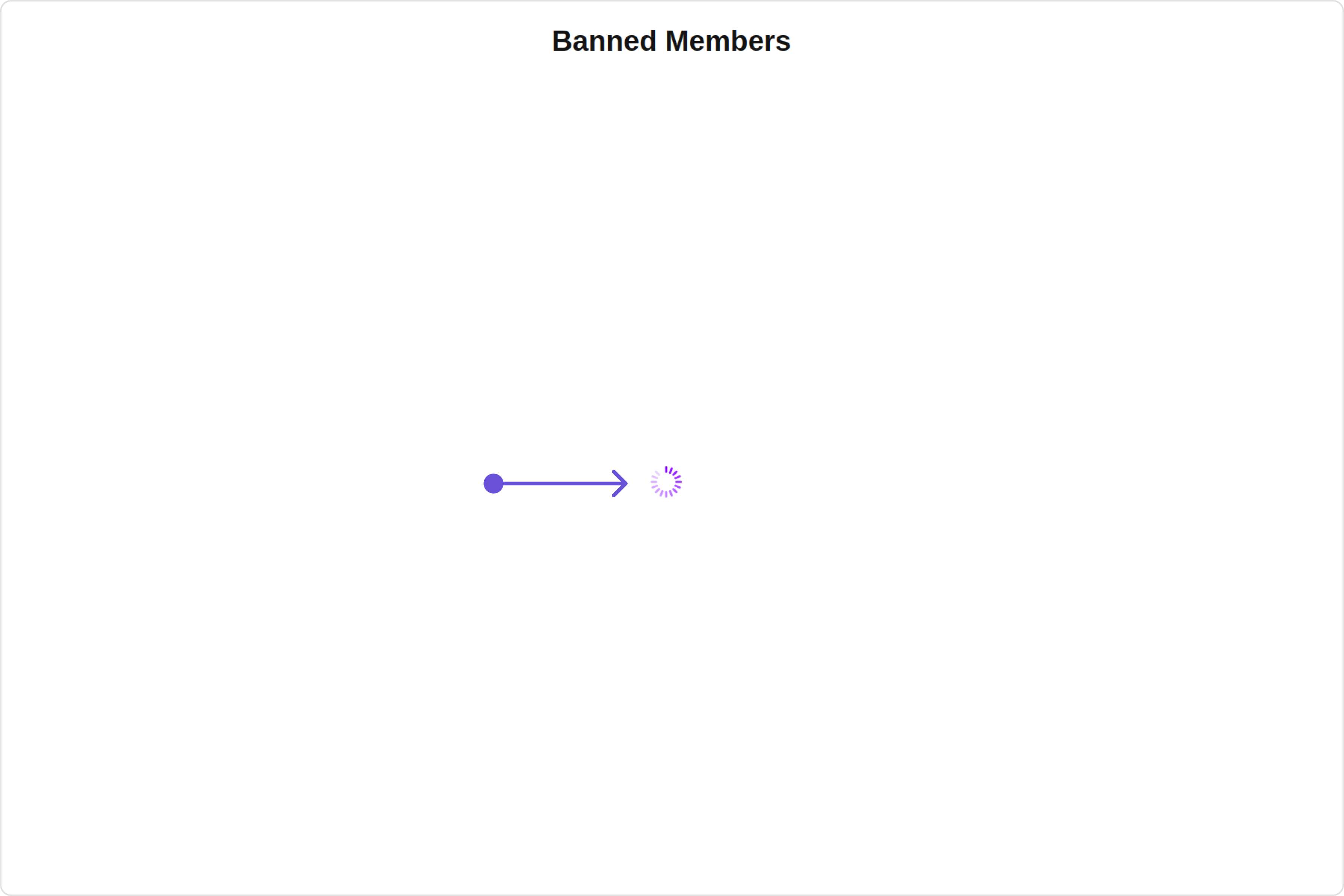
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
LoaderStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getLoadingStateView = () => {
const getLoaderStyle = new LoaderStyle({
iconTint: "#890aff",
background: "transparent",
height: "100vh",
width: "100vw",
});
return (
<cometchat-loader
iconURL="icon"
loaderStyle={JSON.stringify(getLoaderStyle)}
></cometchat-loader>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
loadingStateView={getLoadingStateView()}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
LoaderStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getLoadingStateView = () => {
const getLoaderStyle = new LoaderStyle({
iconTint: "#890aff",
background: "transparent",
height: "100vh",
width: "100vw",
});
return (
<cometchat-loader
iconURL="icon"
loaderStyle={JSON.stringify(getLoaderStyle)}
></cometchat-loader>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
loadingStateView={getLoadingStateView()}
/>
)}
</>
);
};
export default BannedMembersDemo;
EmptyStateView
You can set a custom EmptyStateView
using emptyStateView
to match the empty view of your app.
emptyStateView={getEmptyStateView()}
Default:
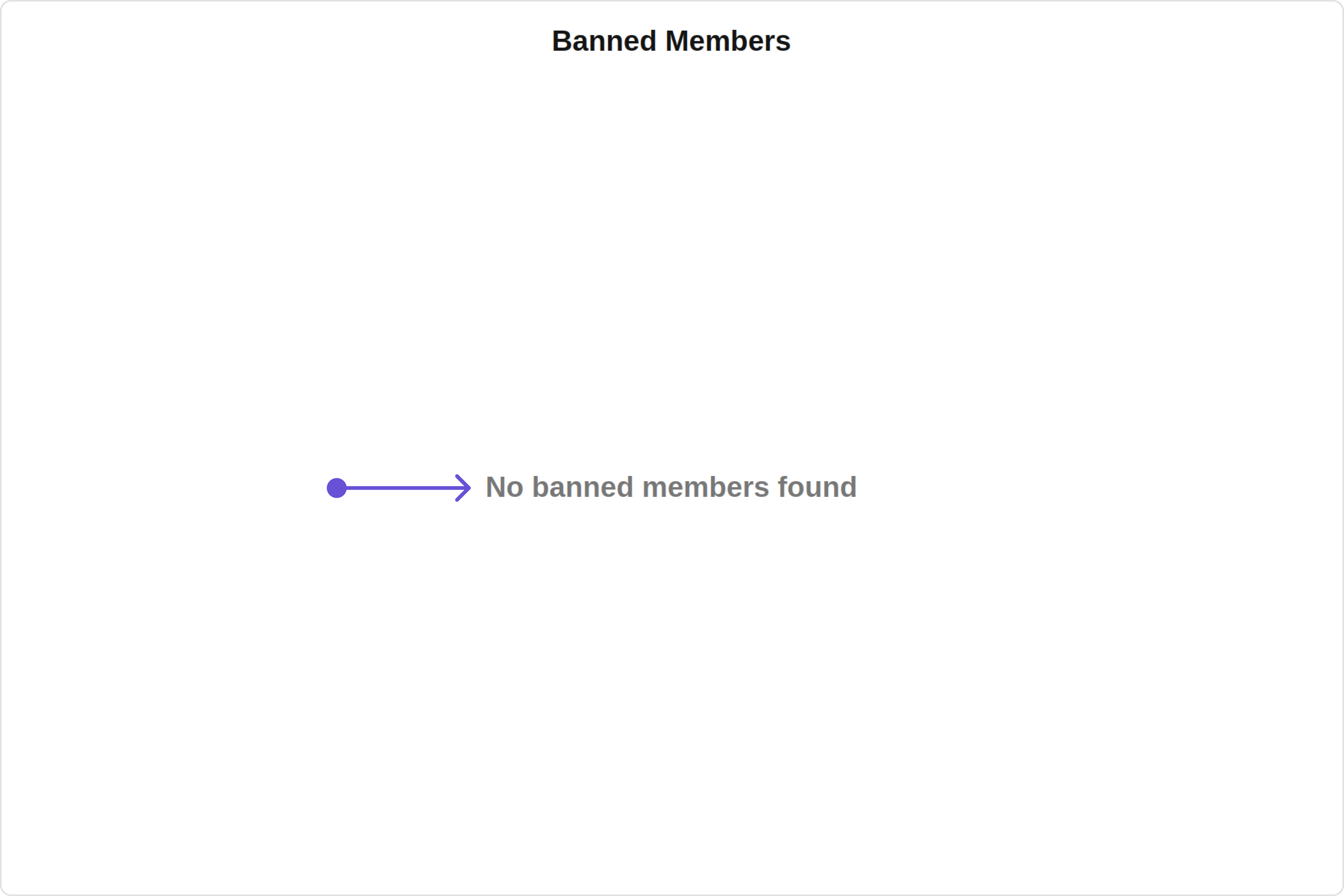
Custom:
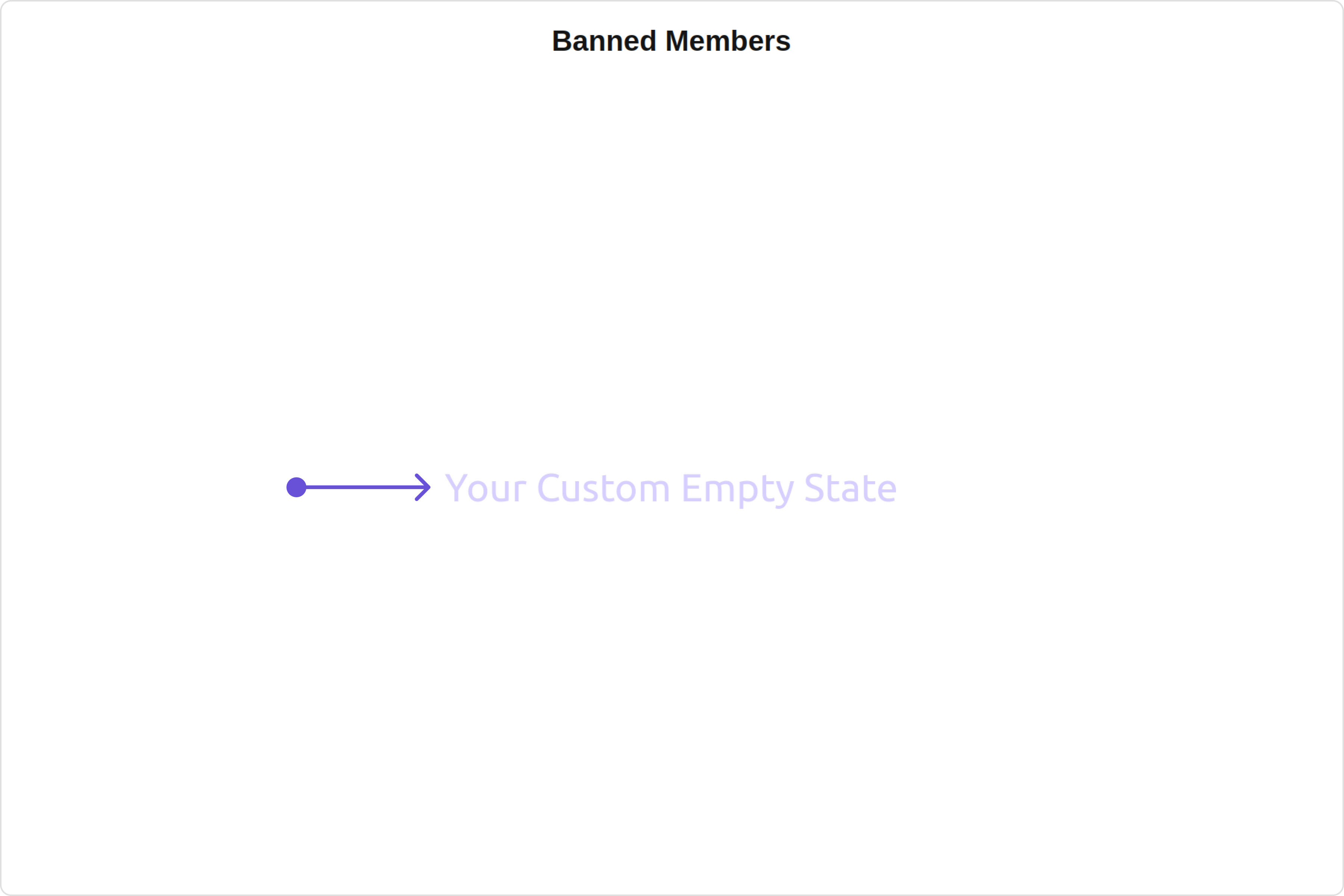
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getEmptyStateView = () => {
return (
<div style={{ color: "#d6cfff", fontSize: "30px", font: "bold" }}>
Your Custom Empty State
</div>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
emptyStateView={getEmptyStateView()}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getEmptyStateView = () => {
return (
<div style={{ color: "#d6cfff", fontSize: "30px", font: "bold" }}>
Your Custom Empty State
</div>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
emptyStateView={getEmptyStateView()}
/>
)}
</>
);
};
export default BannedMembersDemo;
ErrorStateView
You can set a custom ErrorStateView
using errorStateView
to match the error view of your app.
errorSateView={getErrorStateView()}
Default:
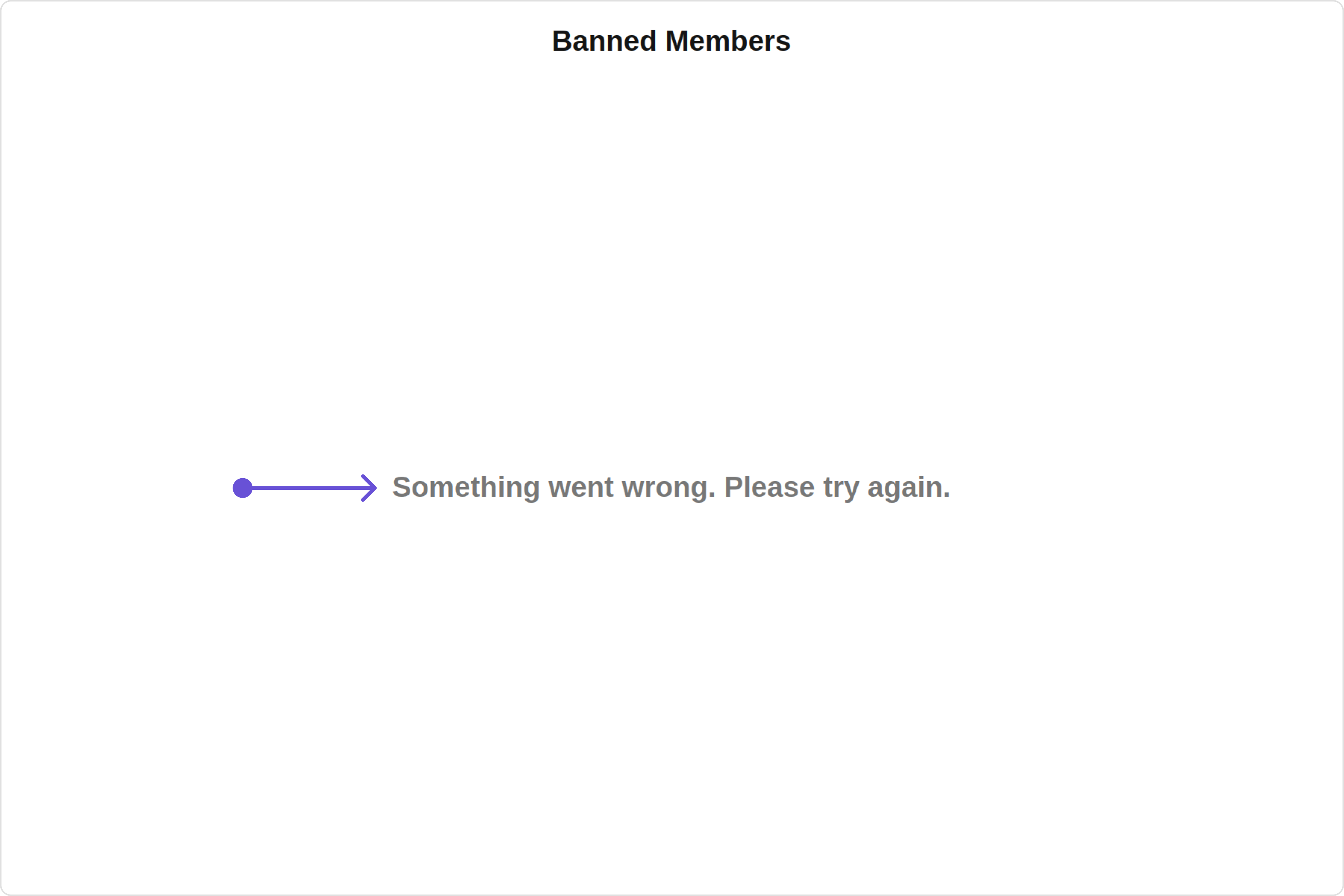
Custom:
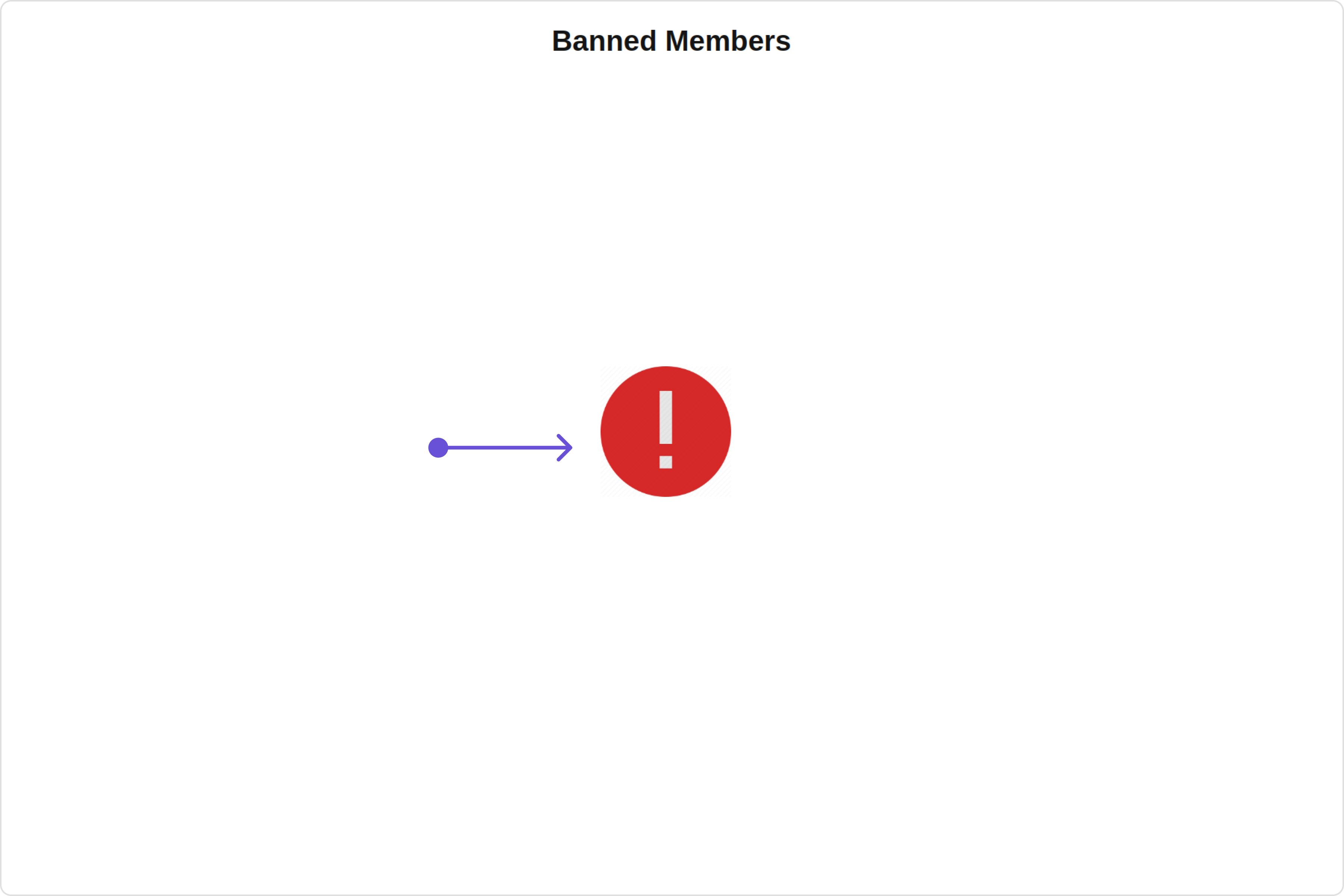
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getErrorStateView = () => {
return (
<div style={{ height: "100vh", width: "100vw" }}>
<img
src="image"
alt="error icon"
style={{
height: "100px",
width: "100px",
marginTop: "250px",
justifyContent: "center",
}}
></img>
</div>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
errorStateView={getErrorStateView()}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getErrorStateView = () => {
return (
<div style={{ height: "100vh", width: "100vw" }}>
<img
src="image"
alt="error icon"
style={{
height: "100px",
width: "100px",
marginTop: "250px",
justifyContent: "center",
}}
></img>
</div>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
errorStateView={getErrorStateView()}
/>
)}
</>
);
};
export default BannedMembersDemo;
Menus
You can set the Custom Menu view to add more options to the Banned Members component.
menus={getMenus()}
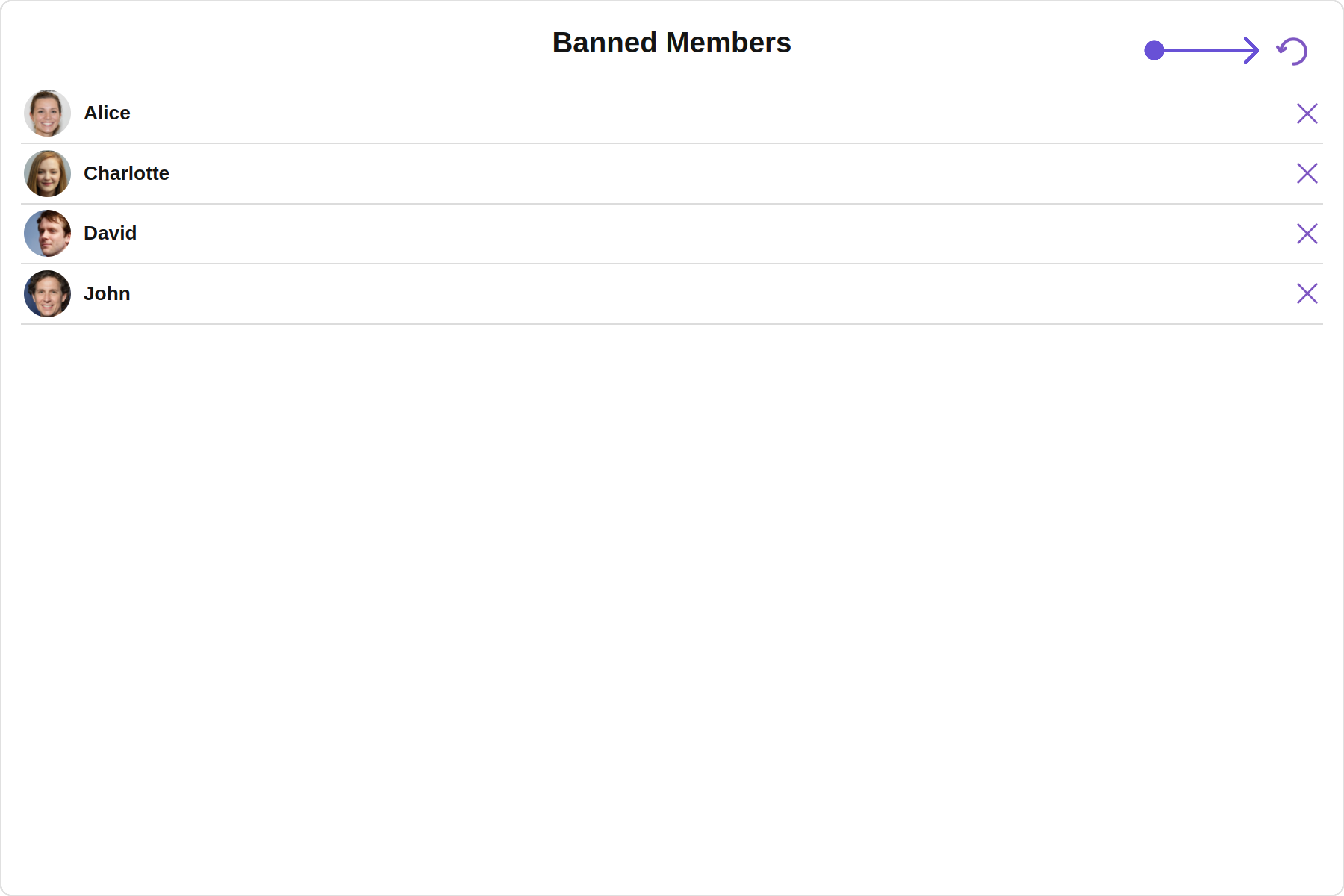
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getMenus = () => {
const handleReload = () => {
window.location.reload();
};
const getButtonStyle = () => {
return {
height: "20px",
width: "20px",
border: "none",
borderRadius: "0",
background: "transparent",
buttonIconTint: "#7E57C2",
};
};
return (
<div style={{ marginRight: "20px" }}>
<cometchat-button
iconURL="icon"
buttonStyle={JSON.stringify(getButtonStyle())}
onClick={handleReload}
>
{" "}
</cometchat-button>
</div>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} menus={getMenus()} />
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
const getMenus = () => {
const handleReload = () => {
window.location.reload();
};
const getButtonStyle = () => {
return {
height: "20px",
width: "20px",
border: "none",
borderRadius: "0",
background: "transparent",
buttonIconTint: "#7E57C2",
};
};
return (
<div style={{ marginRight: "20px" }}>
<cometchat-button
iconURL="icon"
buttonStyle={JSON.stringify(getButtonStyle())}
onClick={handleReload}
>
{" "}
</cometchat-button>
</div>
);
};
return (
<>
{chatGroup && (
<CometChatBannedMembers group={chatGroup} menus={getMenus()} />
)}
</>
);
};
export default BannedMembersDemo;
Options
You can set the Custom options to the Banned Members component.
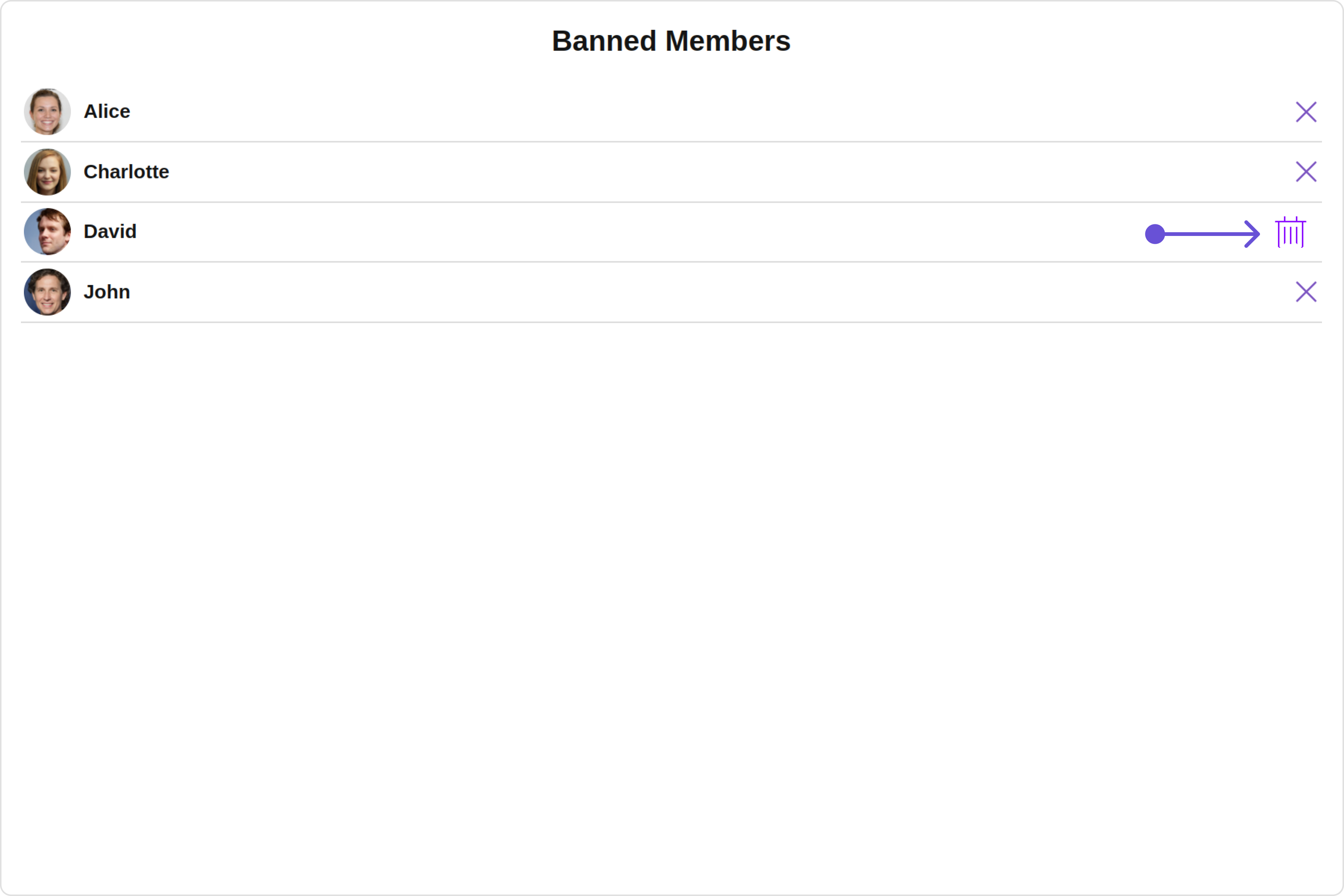
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
CometChatOption,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = React.useState<
CometChat.Group | undefined
>();
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
options={(bannedMember: CometChat.GroupMember) => {
const customOptions = [
new CometChatOption({
id: "1",
title: "Title",
iconURL: "icon",
backgroundColor: "transparent",
onClick: () => {
console.log("Custom option clicked:", bannedMember);
},
iconTint: "#890aff",
titleColor: "blue",
}),
];
return customOptions;
}}
/>
)}
</>
);
};
export default BannedMembersDemo;
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatBannedMembers,
CometChatOption,
} from "@cometchat/chat-uikit-react";
import React from "react";
const BannedMembersDemo = () => {
const [chatGroup, setChatGroup] = useState(null);
React.useEffect(() => {
CometChat.getGroup("uid").then((group) => {
setChatGroup(group);
});
}, []);
return (
<>
{chatGroup && (
<CometChatBannedMembers
group={chatGroup}
options={(bannedMember) => {
const customOptions = [
new CometChatOption({
id: "1",
title: "Title",
iconURL: "icon",
backgroundColor: "transparent",
onClick: () => {
console.log("Custom option clicked:", bannedMember);
},
iconTint: "#890aff",
titleColor: "blue",
}),
];
return customOptions;
}}
/>
)}
</>
);
};
export default BannedMembersDemo;