Banned Members
Overview
CometChatBannedMembers
is a Component that displays all the users who have been restricted or prohibited from participating in specific groups or conversations. When the user is banned, they are no longer able to access or engage with the content and discussions within the banned group. Group administrators or owners have the authority to ban members from specific groups they manage. They can review user activity, monitor behavior, and take appropriate actions, including banning users when necessary.
- iOS
- Android
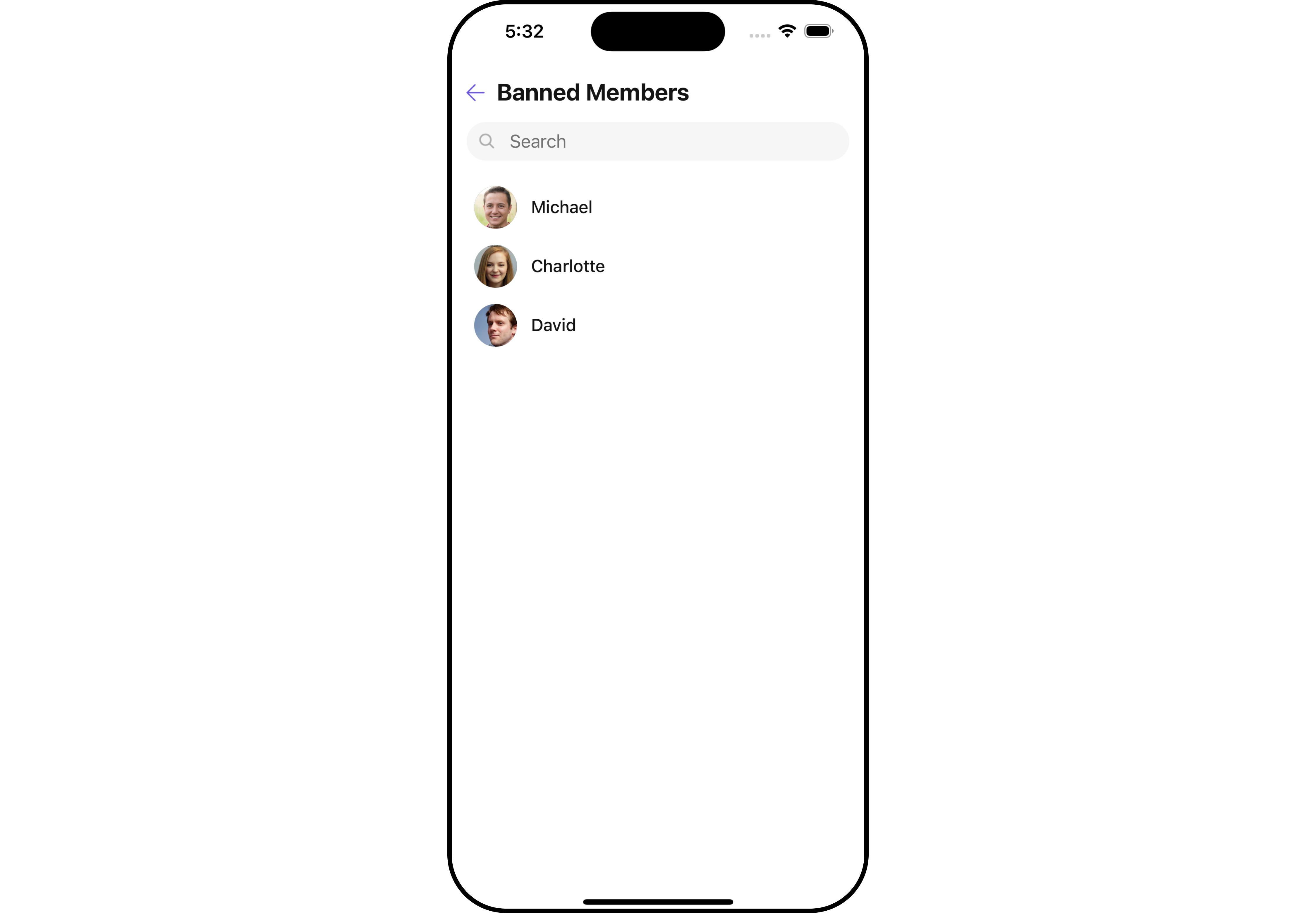
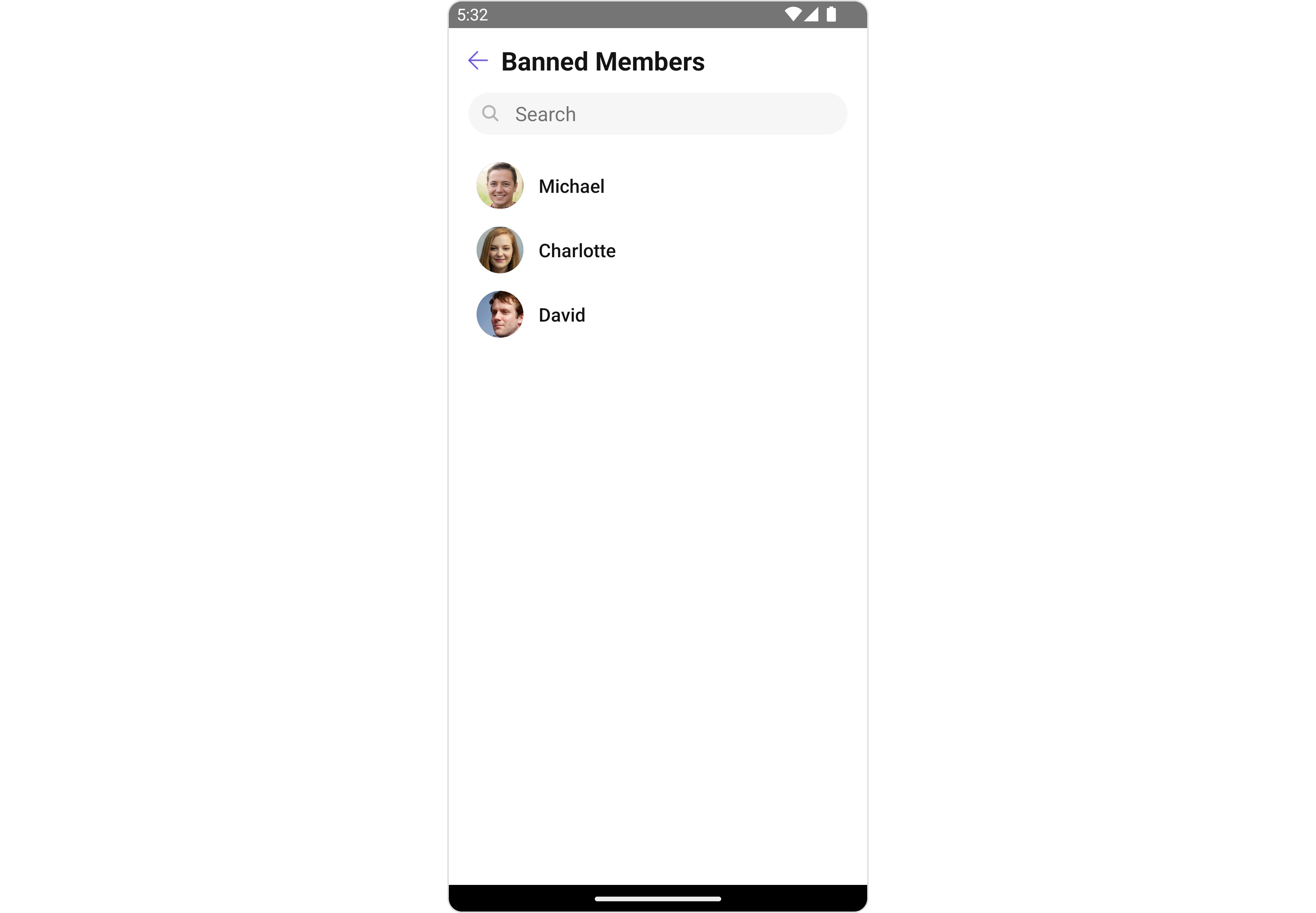
Usage
Integration
The following code snippet illustrates how you can directly incorporate the Banned Members component into your Application.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
return (
<>
{group && <CometChatBannedMembers group={group}></CometChatBannedMembers>}
</>
);
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onSelection
The onSelection
action is activated when you select the done icon while in selection mode. This returns a list of all the banned members that you have selected.
This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const onSelectionHandler = (list: CometChat.User[]) => {
//code
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
onSelection={onSelectionHandler}
></CometChatBannedMembers>
)}
</>
);
}
2. onItemPress
The onItemPress
event is activated when you click on the Banned Members List item. This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const onItemPresshandler = (user: CometChat.User) => {
//code
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
onItemPress={onItemPresshandler}
></CometChatBannedMembers>
)}
</>
);
}
3. onItemLongPress
The onItemLongPress
event is activated when you Long Press on the Banned Members List item. This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const onItemLongPresshandler = (user: CometChat.User) => {
//code
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
onItemLongPress={onItemLongPresshandler}
></CometChatBannedMembers>
)}
</>
);
}
4. OnBack
OnBack
is triggered when you click on the back button of the Banned Members component. You can override this action using the following code snippet.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const onBackHandler = () => {
//code
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
onBack={onBackHandler}
></CometChatBannedMembers>
)}
</>
);
}
5. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the Banned Members component.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const onErrorHandler = (error: CometChat.CometChatException) => {
//code
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
onError={onErrorHandler}
></CometChatBannedMembers>
)}
</>
);
}
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
1. BannedMembersRequestBuilder
The BannedMembersRequestBuilder enables you to filter and customize the Banned Members list based on available parameters in BannedMembersRequestBuilder. This feature allows you to create more specific and targeted queries when fetching banned members. The following are the parameters available in BannedMembersRequestBuilder
Methods | Type | Description |
---|---|---|
setLimit | number | sets the number of banned members that can be fetched in a single request, suitable for pagination |
setSearchKeyword | String | used for fetching banned members matching the passed string |
setScopes | Array<String> | used for fetching banned members based on multiple scopes |
Example
In the example below, we are applying a filter to the banned members by setting the limit to 2 and setting the scope to show only the moderator.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const bannedMembersRequestBuilder = new CometChat.BannedMembersRequestBuilder(
"guid"
)
.setLimit(2)
.setScopes(["moderator"]);
return (
<>
{group && (
<CometChatBannedMembers
group={group}
bannedMembersRequestBuilder={bannedMembersRequestBuilder}
></CometChatBannedMembers>
)}
</>
);
}
2. SearchRequestBuilder
The SearchRequestBuilder uses BannedMembersRequestBuilder enables you to filter and customize the search list based on available parameters in BannedMembersRequestBuilder.
Example
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const searchBannedMembersRequestBuilder =
new CometChat.BannedMembersRequestBuilder("guid")
.setLimit(2)
.setSearchKeyword("some-keyword");
return (
<>
{group && (
<CometChatBannedMembers
group={group}
searchRequestBuilder={searchBannedMembersRequestBuilder}
></CometChatBannedMembers>
)}
</>
);
}
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
Events emitted by the Add Members component is as follows.
Event | Description |
---|---|
ccGroupMemberUnBanned | Triggers when a user is unbanned from a group |
- Adding Listeners
import { CometChatUIEventHandler } from "@cometchat/chat-uikit-react-native";
CometChatUIEventHandler.addGroupListener("GROUP_LISTENER_ID", {
ccGroupMemberUnBanned: ({ message }: { message: CometChat.BaseMessage }) => {
//code
},
});
- Removing Listeners
import { CometChatUIEventHandler } from "@cometchat/chat-uikit-react-native";
CometChatUIEventHandler.removeGroupListener("GROUP_LISTENER_ID");
Customization
To fit your app's design requirements, you can customize the appearance of the Groups component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. BannedMembers Style
You can set the BannedMembersStyle
to the Banned Members Component to customize the styling.
- iOS
- Android
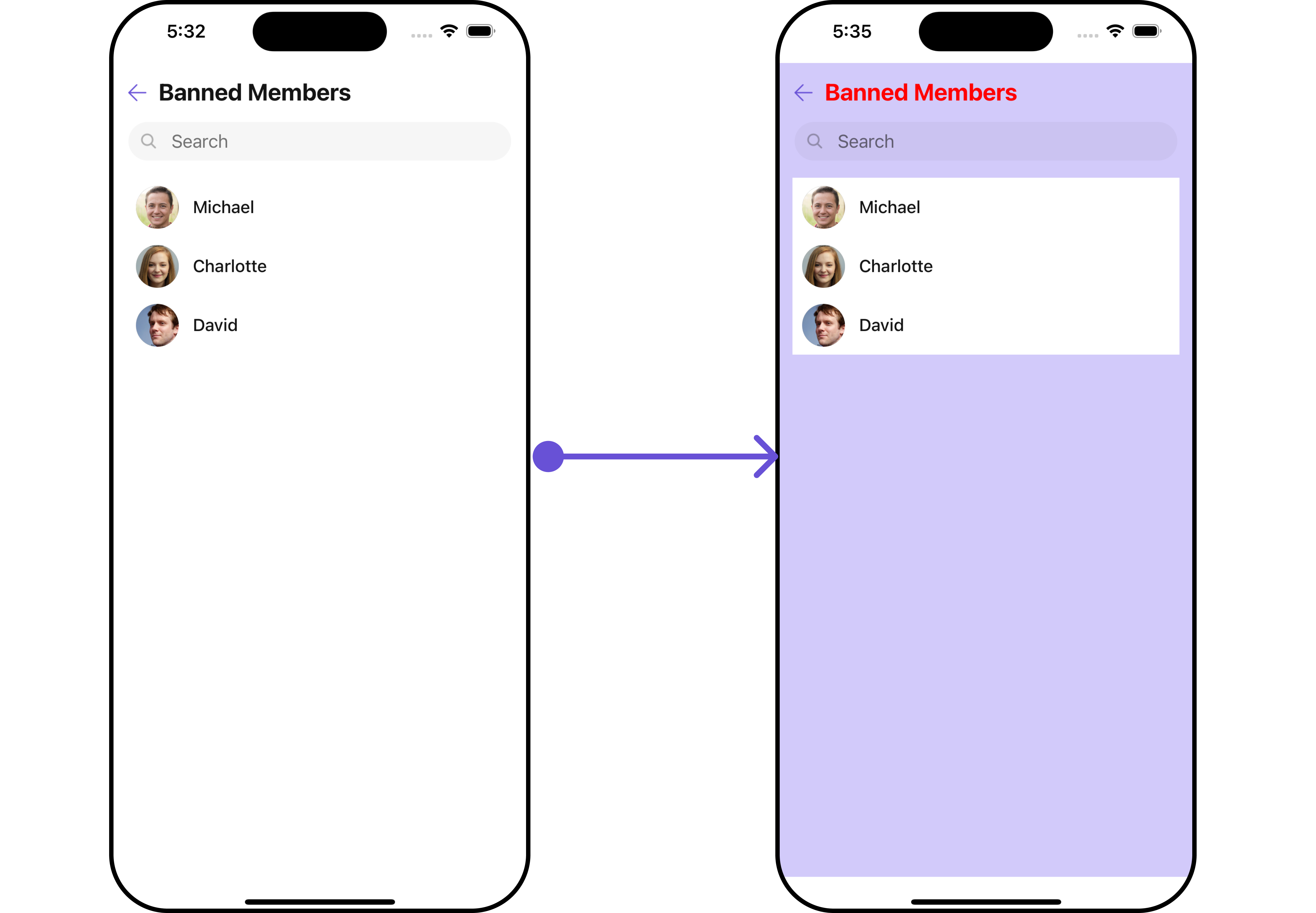
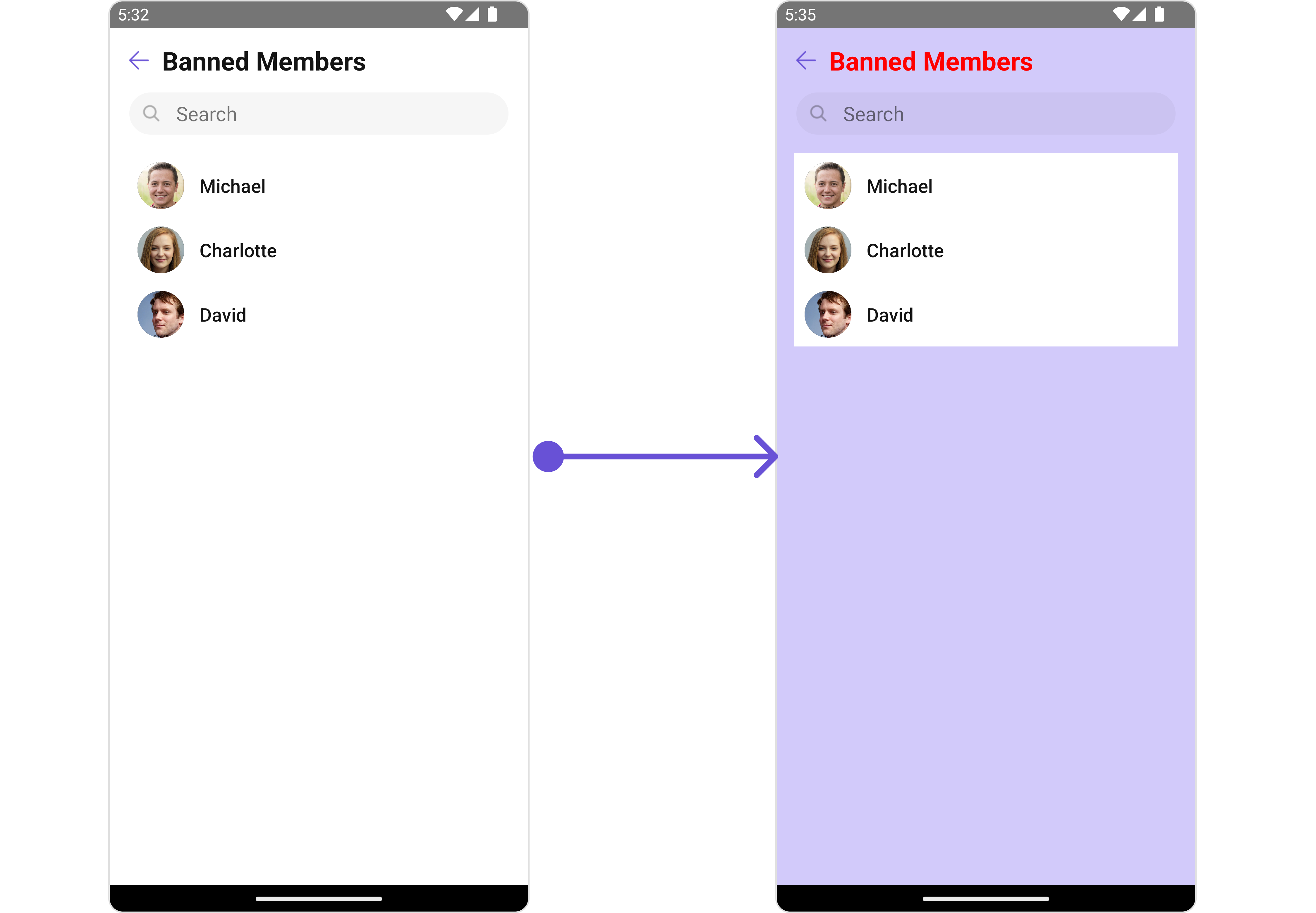
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatBannedMembers,
CometChatListStylesInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const bannedMemberStyle: CometChatListStylesInterface = {
background: "#d2cafa",
titleColor: "red",
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
bannedMemberStyle={bannedMemberStyle}
></CometChatBannedMembers>
)}
</>
);
}
List of properties exposed by BannedMembersStyle:
Property | Description | Code |
---|---|---|
border | Used to set border | border?: BorderStyleInterface, |
borderRadius | Used to set border radius | borderRadius?: number; |
background | Used to set background colour | background?: string; |
height | Used to set height | height?: string | number ; |
width | Used to set width | width?: string | number ; |
titleFont | Used to set title text font | titleFont?: FontStyleInterface, |
titleColor | Used to set title text color | titleColor?: string; |
backIconTint | Used to set back button icon tint | backIconTint?: string; |
searchIconTint | Used to set search icon tint | searchIconTint?: string; |
searchBorder | Used to set search border | searchBorder?: BorderStyleInterface; |
searchBorderRadius | Used to set search border radius | searchBorderRadius?: number; |
searchBackground | Used to set search background color | searchBackground?: string; |
searchTextFont | Used to set search text font | searchTextFont?: FontStyleInterface; |
searchTextColor | Used to set search text color | searchTextColor?: string; |
onlineStatusColor | Used to set online status color | onlineStatusColor?: string; |
loadingIconTint | Used to set loading icon tint | loadingIconTint?: string; |
separatorColor | Used to set separator color | separatorColor?: string; |
emptyTextColor | Used to set empty state text color | emptyTextColor?: string; |
emptyStateTextFont | Used to set empty state text font | emptyStateTextFont?: FontStyleInterface; |
errorTextFont | Used to set error state text font | errorStateTextFont?: FontStyleInterface; |
errorTextColor | Used to set error state text color | errorStateTextColor?: string; |
sectionHeaderTextFont | Used to set section header text font | sectionHeaderTextFont?: FontStyleInterface; |
sectionHeaderTextColor | Used to set section header text color | sectionHeaderTextColor?: string; |
2. Avatar Style
To apply customized styles to the Avatar
component in the Add Members Component, you can use the following code snippet. For further insights on Avatar
Styles refer
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatBannedMembers,
BorderStyleInterface,
AvatarStyleInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const borderStyle: BorderStyleInterface = {
borderWidth: 1,
borderStyle: "solid",
borderColor: "red",
};
const avatarStyle: AvatarStyleInterface = {
outerViewSpacing: 5,
outerView: {
borderWidth: 2,
borderStyle: "dotted",
borderColor: "blue",
},
border: borderStyle,
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
avatarStyle={avatarStyle}
></CometChatBannedMembers>
)}
</>
);
}
3. ListItem Style
To apply customized styles to the ListItemStyle
component in the Add Members
Component, you can use the following code snippet. For further insights on ListItemStyle
Styles refer
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatBannedMembers,
ListItemStyleInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const listItemStyle: ListItemStyleInterface = {
titleColor: "red",
backgroundColor: "#d2cafa",
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
listItemStyle={listItemStyle}
></CometChatBannedMembers>
)}
</>
);
}
4. StatusIndicator Style
To apply customized styles to the Status Indicator component in the Add Members Component, You can use the following code snippet. For further insights on Status Indicator Styles refer
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import {
CometChatBannedMembers,
StatusIndicatorStyleInterface,
} from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const statusIndicatorStyle: StatusIndicatorStyleInterface = {
backgroundColor: "grey",
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
statusIndicatorStyle={statusIndicatorStyle}
></CometChatBannedMembers>
)}
</>
);
}
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
return (
<>
{group && (
<CometChatBannedMembers
group={group}
title="Custom Title"
></CometChatBannedMembers>
)}
</>
);
}
- iOS
- Android
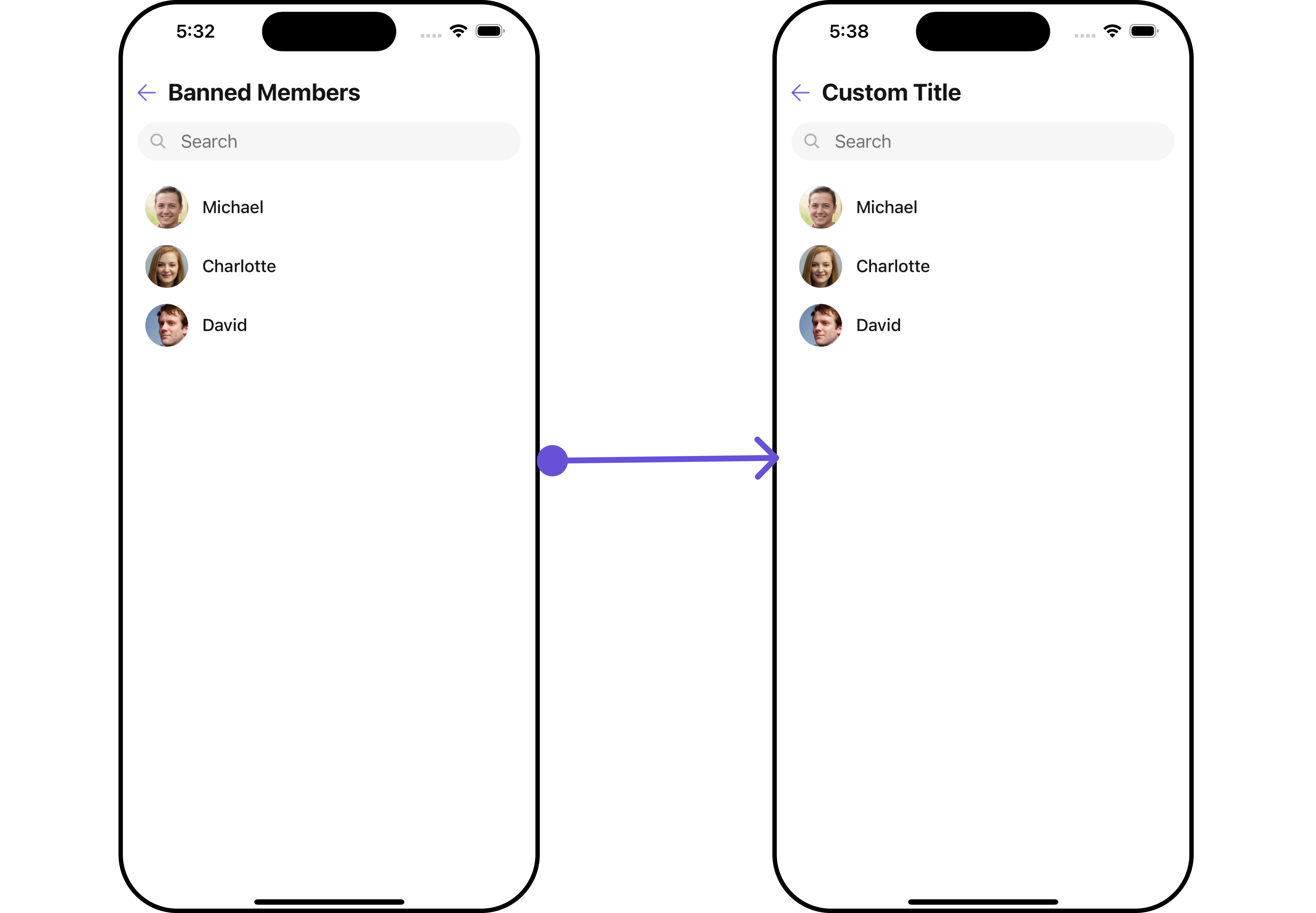
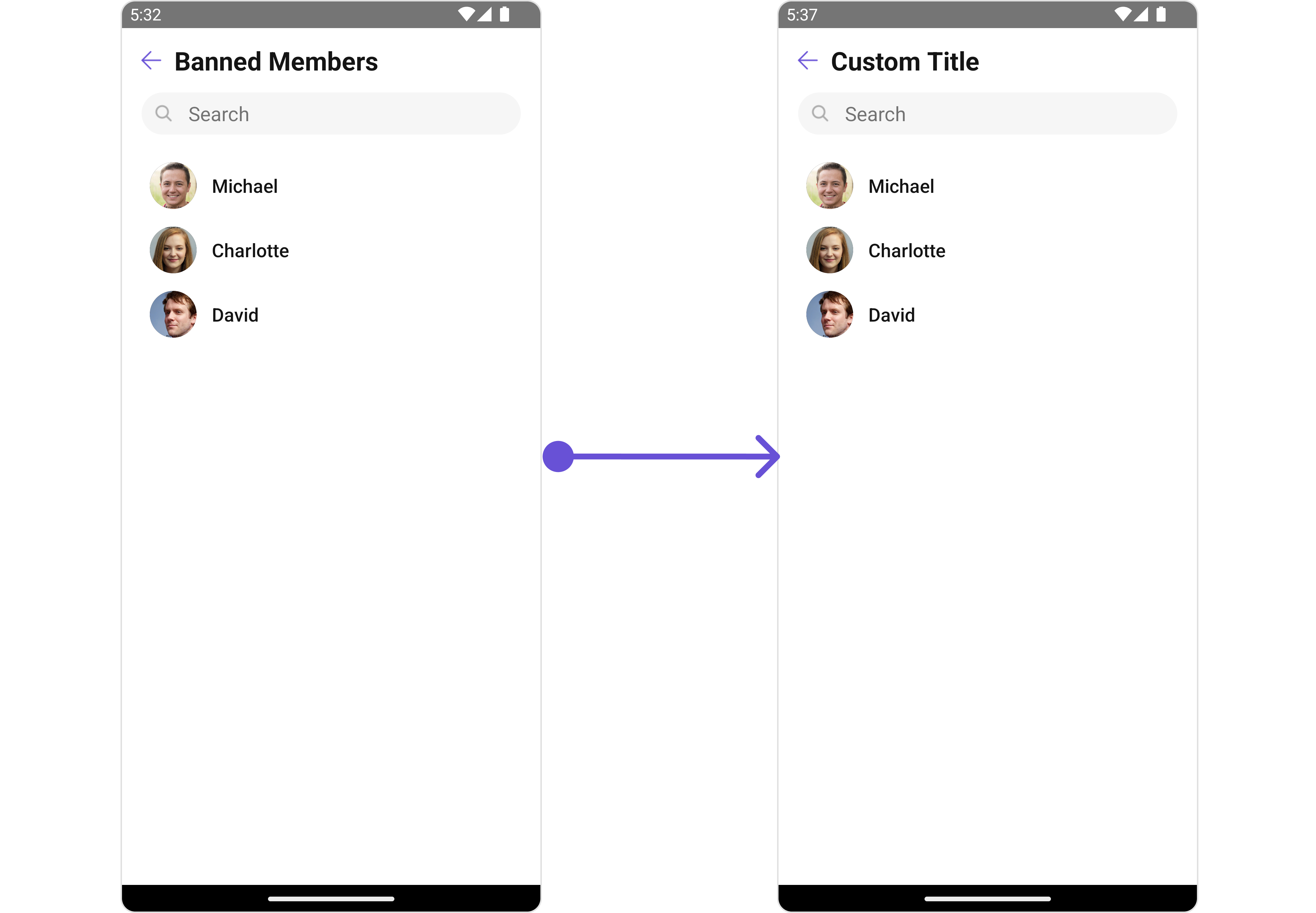
Below is a list of customizations along with corresponding code snippets
Advance
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
ListItemView
With this property, you can assign a custom ListItem to the Add Members Component.
Example
- iOS
- Android
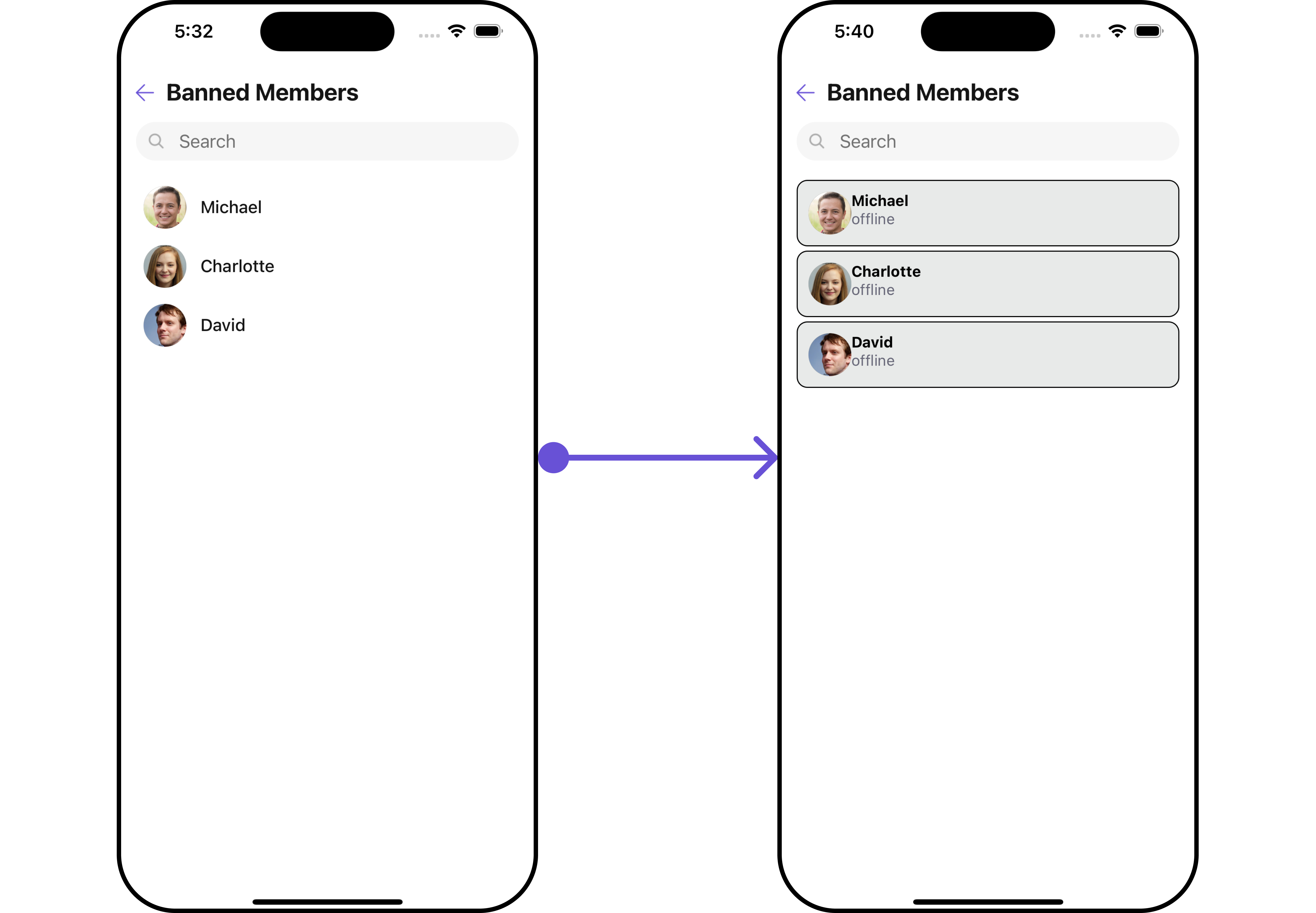
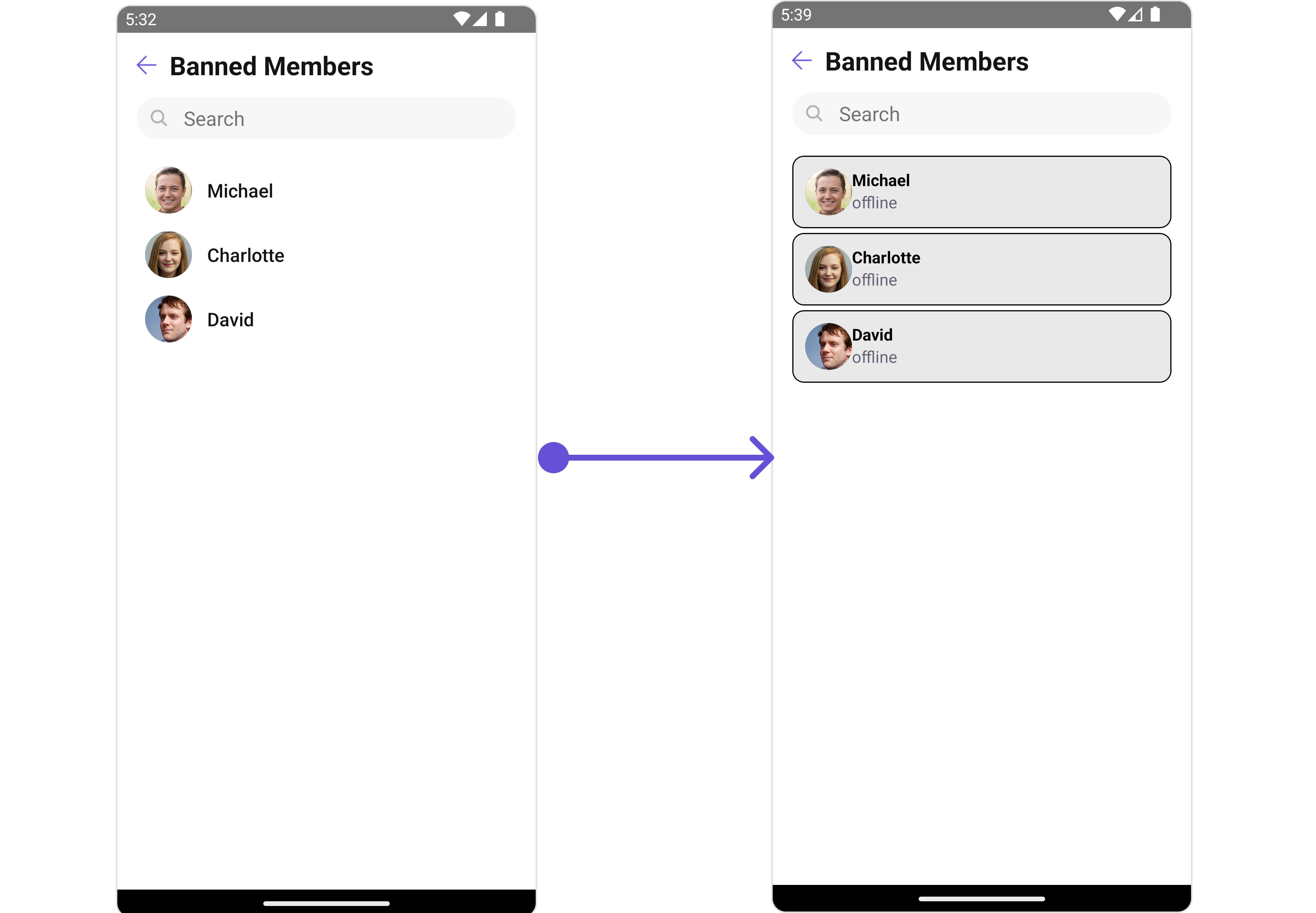
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const viewStyle: StyleProp<ViewStyle> = {
flex: 1,
flexDirection: "row",
alignItems: "flex-start",
padding: 10,
borderColor: "black",
borderWidth: 1,
backgroundColor: "#E8EAE9",
borderRadius: 10,
margin: 2,
};
const getListItemView = (info: { item: CometChat.User; index: number }) => {
let user = info.item;
return (
<View style={viewStyle}>
<CometChatAvatar
image={user.getAvatar() ? { uri: user.getAvatar() } : undefined}
name={user.getName()}
/>
<View>
<Text style={{ color: "black", fontWeight: "bold" }}>
{user.getName()}
</Text>
<Text style={{ color: "#667" }}>{user.getStatus()}</Text>
</View>
</View>
);
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
title="Custom Title"
ListItemView={getListItemView}
></CometChatBannedMembers>
)}
</>
);
}
SubtitleView
You can customize the subtitle view for each users to meet your requirements
- iOS
- Android
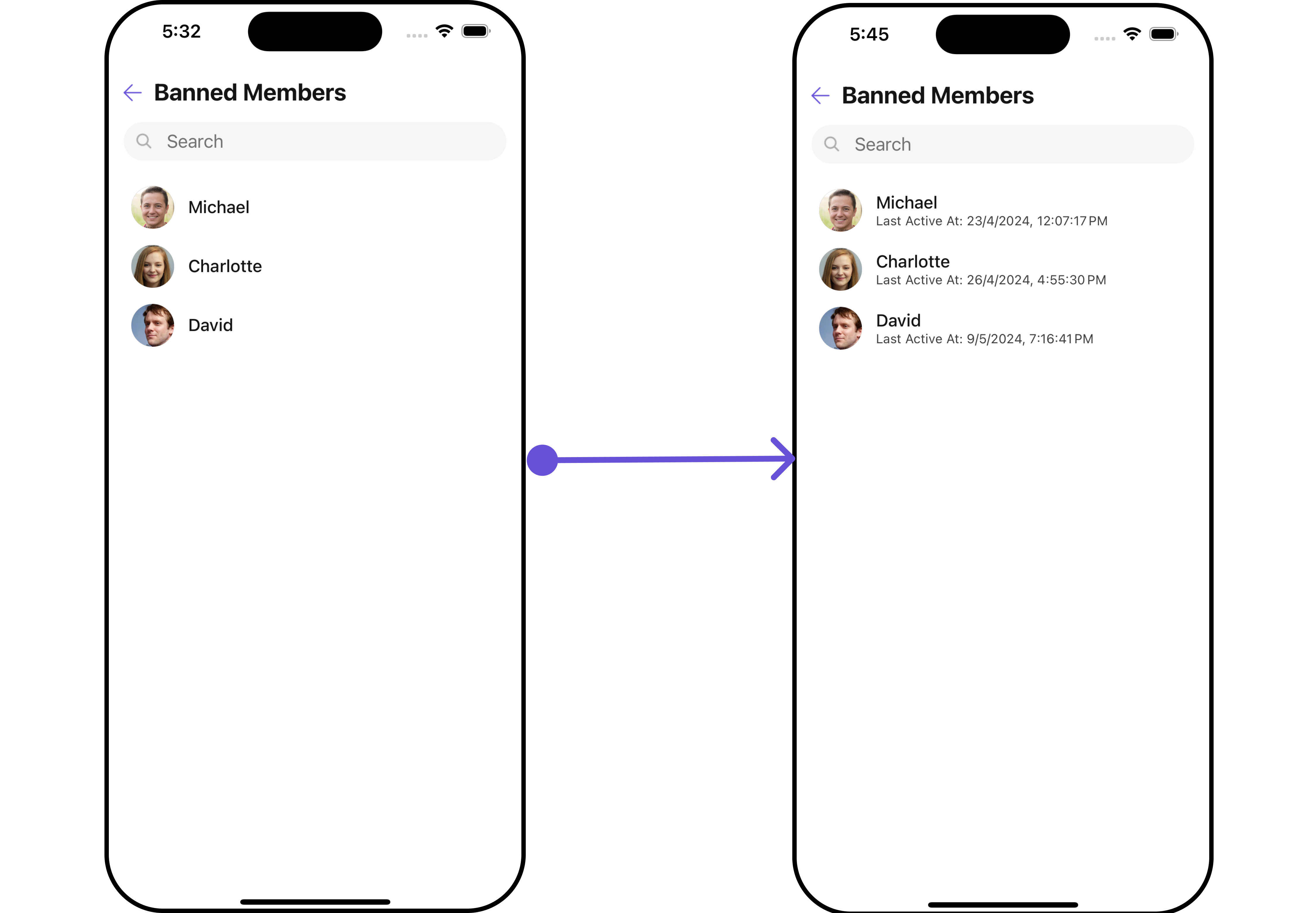
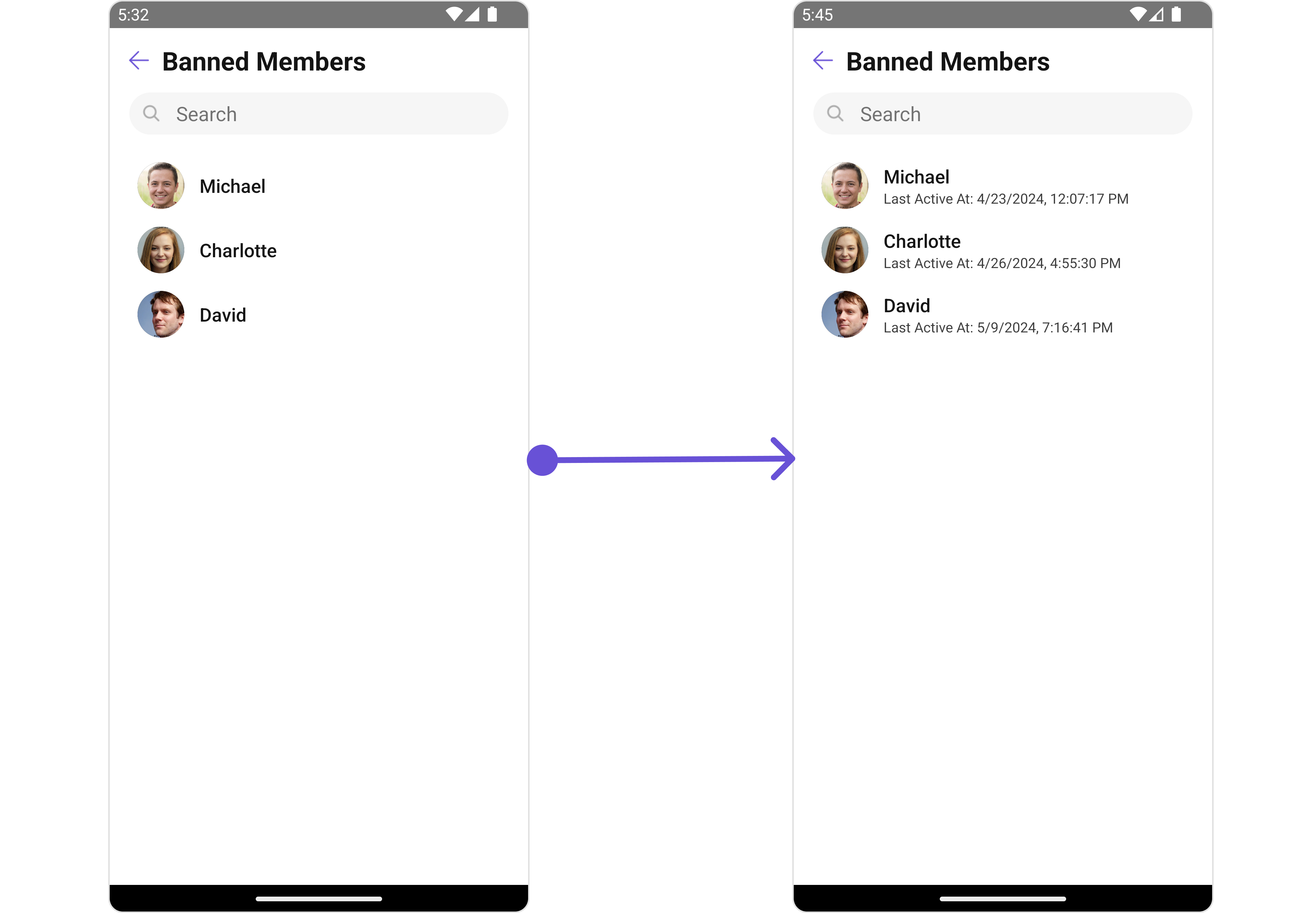
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const getSubtitleView = (user) => {
return (
<Text
style={{
fontSize: 12,
color: theme.palette.getAccent800(),
}}
>
Last Active At:{" "}
{user?.lastActiveAt ? formatTime(user?.lastActiveAt) : "--"}
</Text>
);
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
title="Custom Title"
SubtitleView={getSubtitleView}
></CometChatBannedMembers>
)}
</>
);
}
LoadingStateView
You can set a custom loader view using LoadingStateView
to match the loading view of your app.
- iOS
- Android
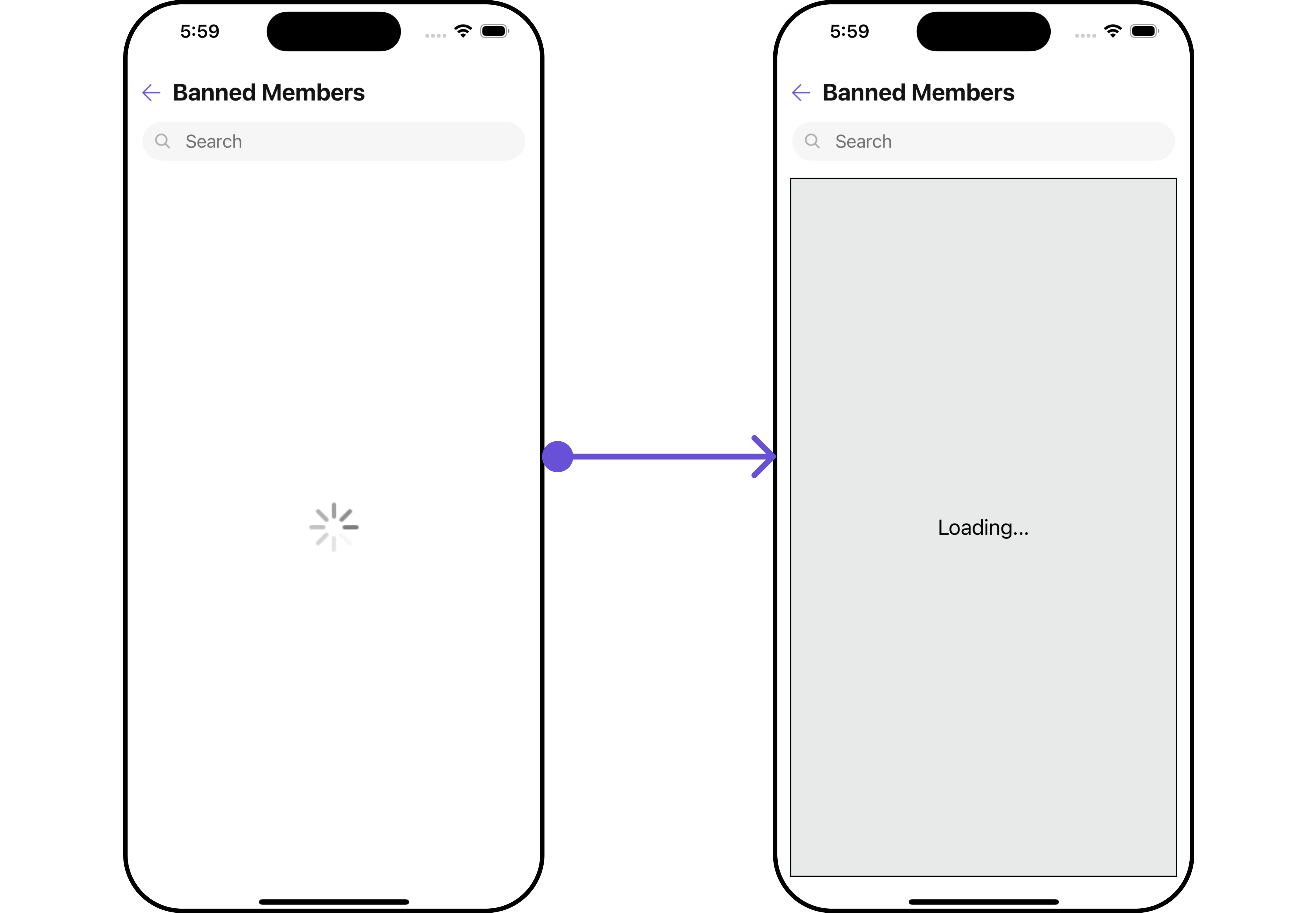
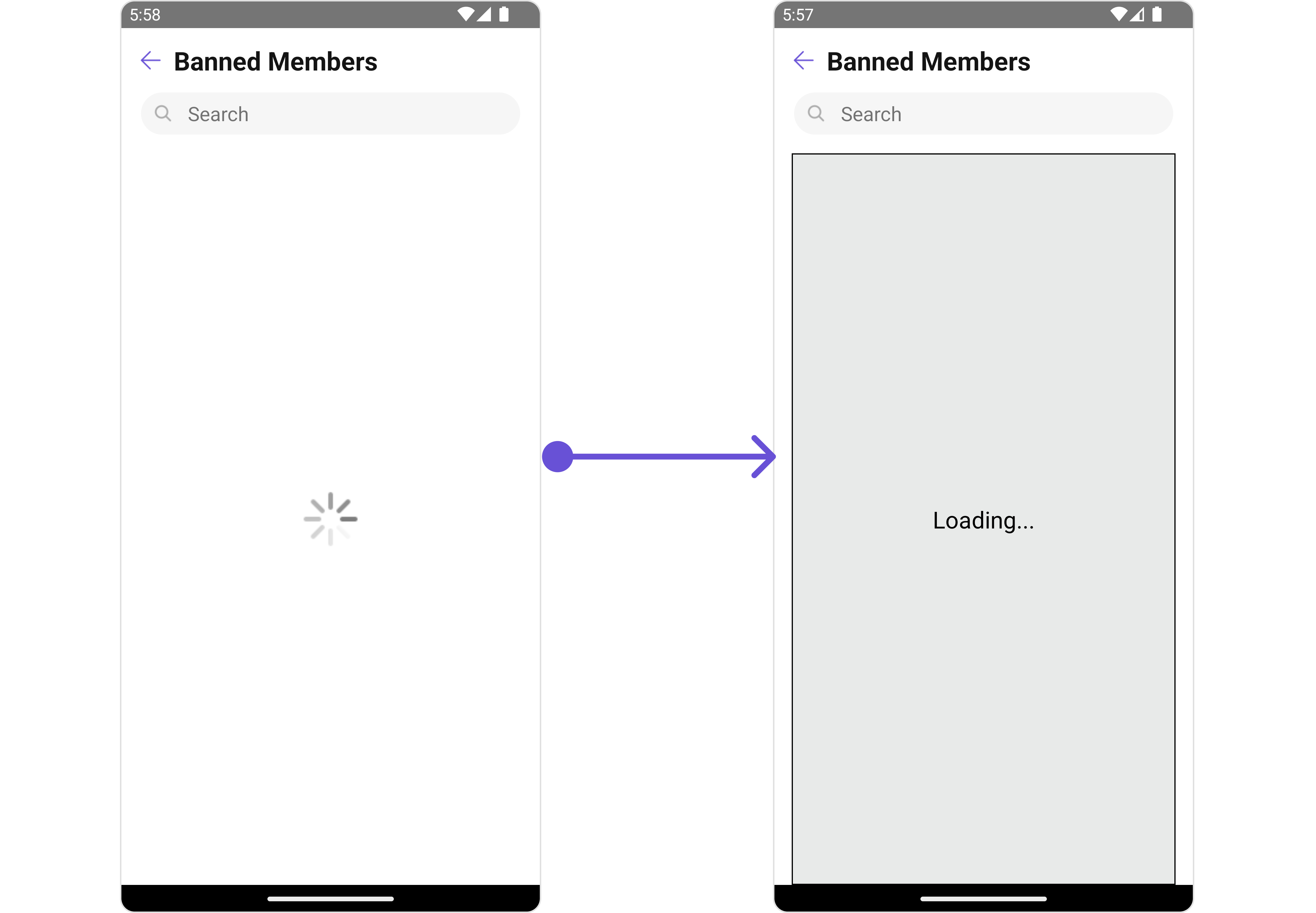
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const loadingViewStyle: StyleProp<ViewStyle> = {
flex: 1,
alignItems: "center",
justifyContent: "center",
padding: 10,
borderColor: "black",
borderWidth: 1,
backgroundColor: "#E8EAE9",
};
const getLoadingStateView = () => {
return (
<View style={loadingViewStyle}>
<Text style={{ fontSize: 20, color: "black" }}>Loading...</Text>
</View>
);
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
LoadingStateView={getLoadingStateView}
></CometChatBannedMembers>
)}
</>
);
}
EmptyStateView
You can set a custom EmptyStateView
using EmptyStateView
to match the empty view of your app.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const searchRequestBuilder = new CometChat.UsersRequestBuilder()
.setLimit(100)
.setSearchKeyword("does-not-exist");
const emptyViewStyle: StyleProp<ViewStyle> = {
flex: 1,
alignItems: "center",
justifyContent: "center",
padding: 10,
borderColor: "black",
borderWidth: 1,
backgroundColor: "#E8EAE9",
};
const getEmptyStateView = () => {
return (
<View style={emptyViewStyle}>
<Text style={{ fontSize: 80, color: "black" }}>Empty</Text>
</View>
);
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
searchRequestBuilder={searchRequestBuilder}
EmptyStateView={getEmptyStateView}
></CometChatBannedMembers>
)}
</>
);
}
AppBarOptions
You can set the Custom Menu view to add more options to the Add Members component.
- iOS
- Android
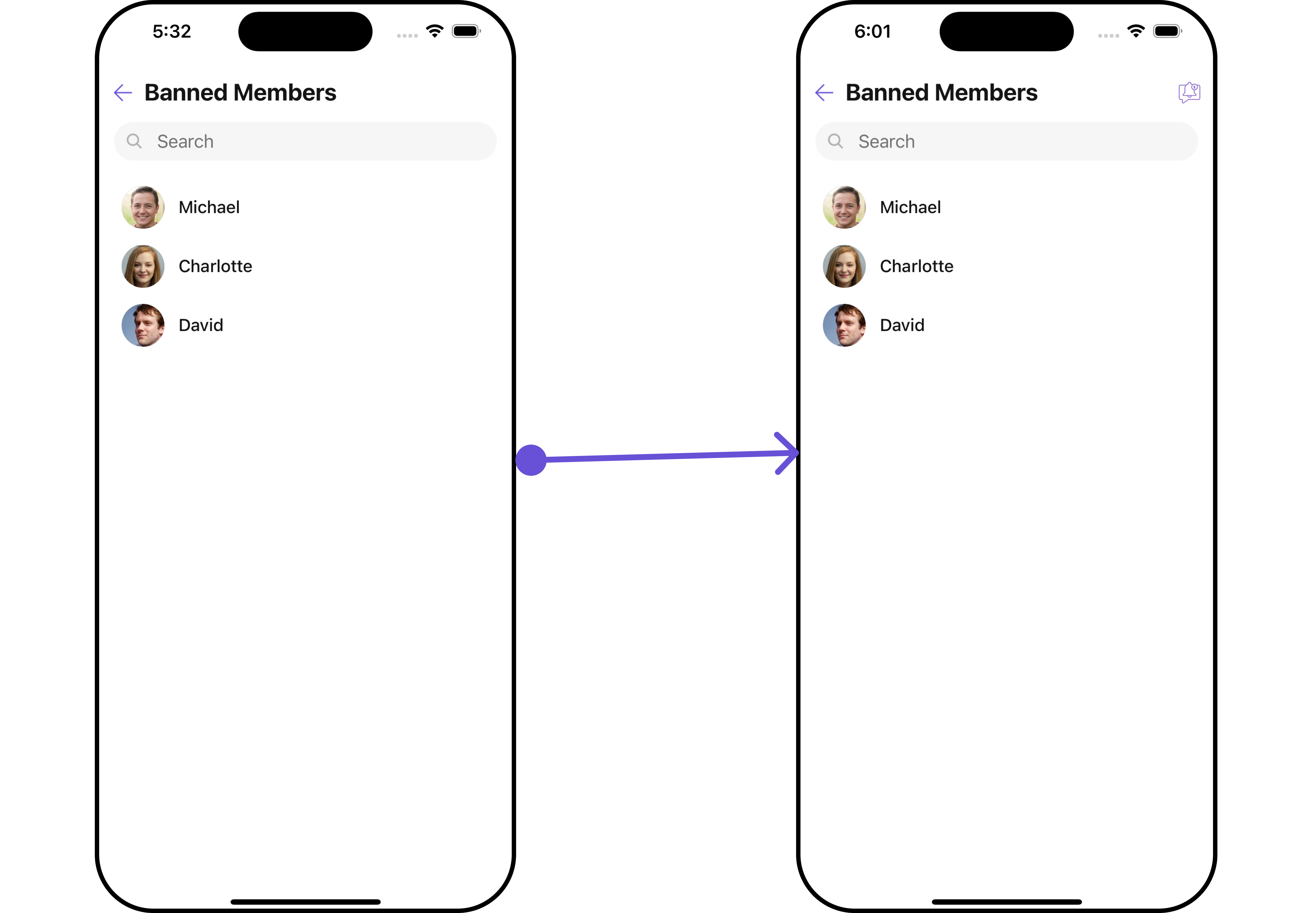
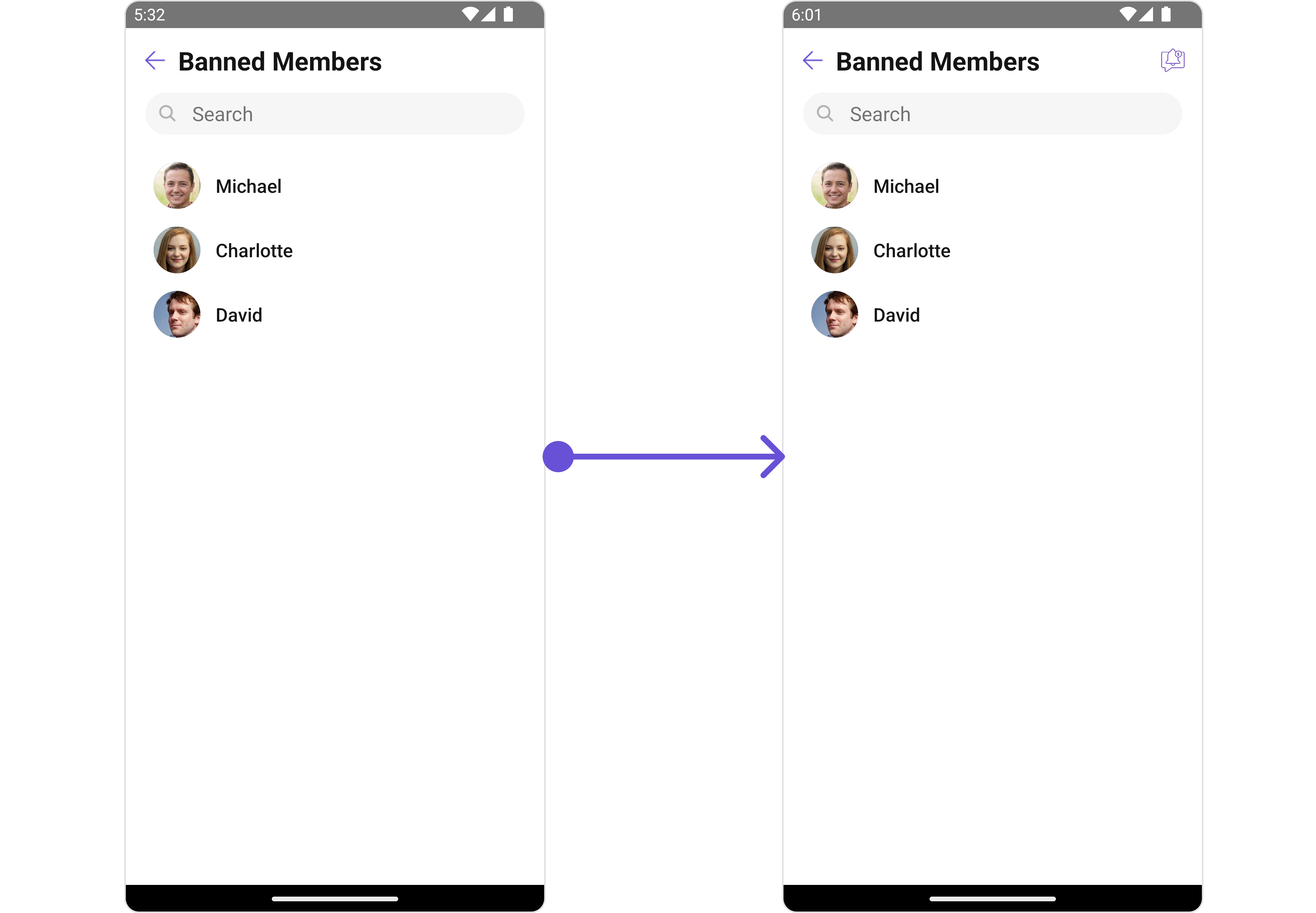
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const getAppBarOptions = () => {
return (
<TouchableOpacity
style={styles.button}
onPress={() => {
/*code*/
}}
>
<Image source={Notification} style={styles.image} />
</TouchableOpacity>
);
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
AppBarOptions={getAppBarOptions}
selectionMode="none"
></CometChatBannedMembers>
)}
</>
);
}
Swipe Options
You can set the Custom Swipe options to the Add Members component.
- iOS
- Android
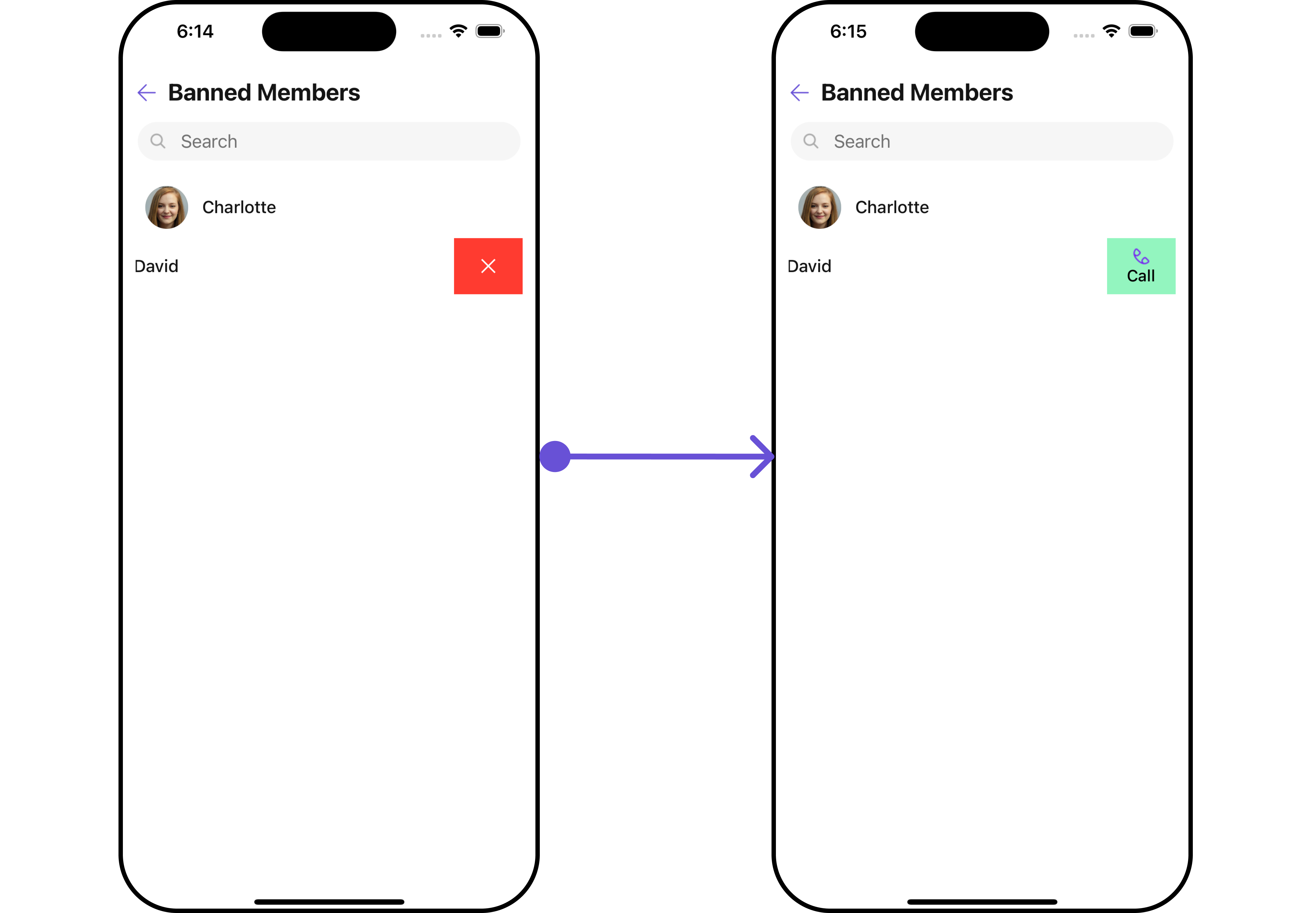
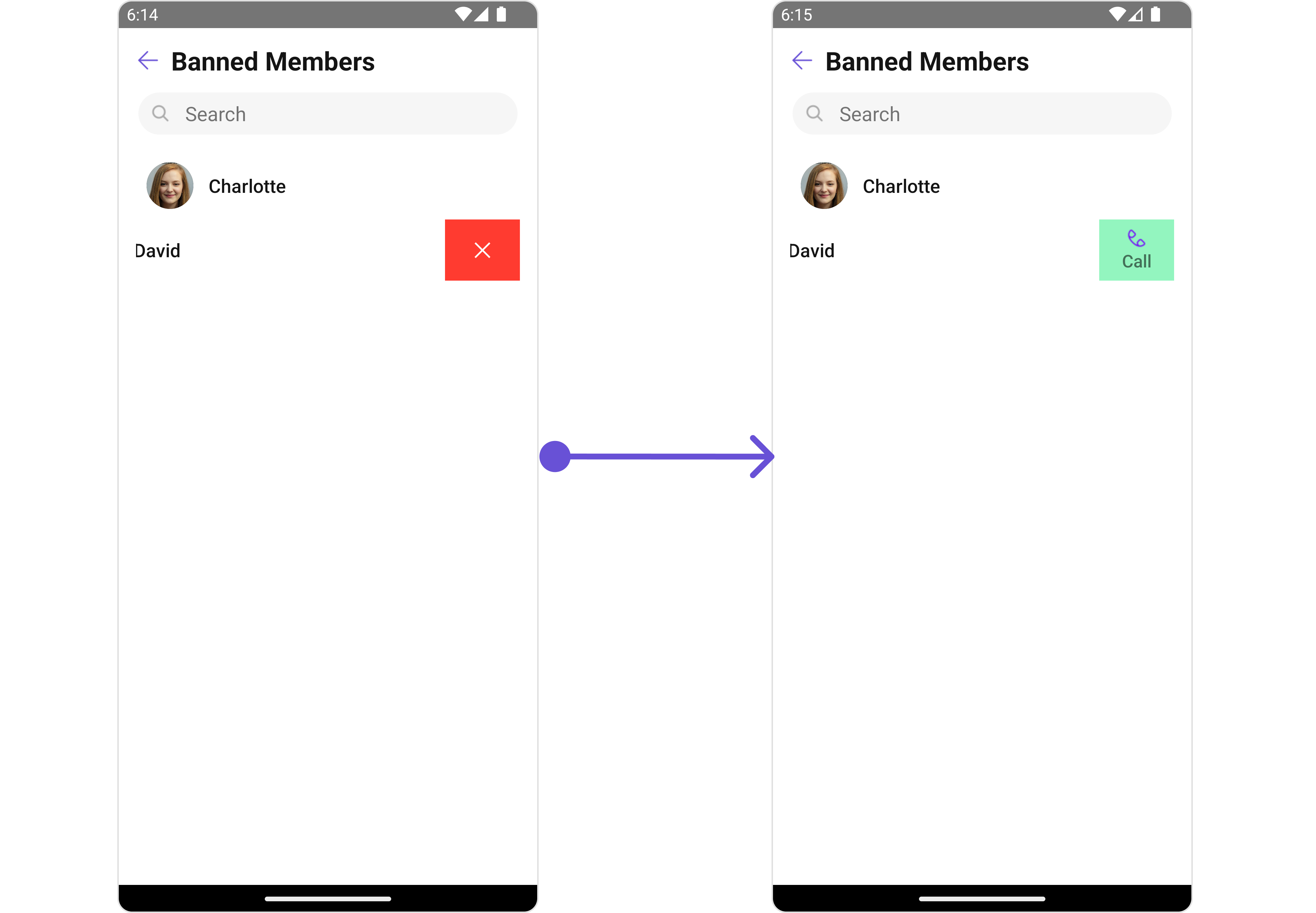
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatBannedMembers } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const [group, setGroup] = useState<CometChat.Group | undefined>(undefined);
const getGroup = async () => {
const group = await CometChat.getGroup("guid");
setGroup(group);
};
useEffect(() => {
//login
getGroup();
});
const getCustomOptions = (user: CometChat.User) => {
const customOption: CometChatOptions = {
id: "custom id",
title: "Call",
icon: Call,
iconTint: "#7316f5",
backgroundColor: "#93f5bf",
onPress: () => console.log("custom action"),
};
return [customOption];
};
return (
<>
{group && (
<CometChatBannedMembers
group={group}
options={getCustomOptions}
></CometChatBannedMembers>
)}
</>
);
}