Call Log With Details
Overview
The CometChatCallLogsWithDetails
is a Composite Widget encompassing widgets such as Call Logs and Call Log Details. Both of these widget contributes to the functionality and structure of the overall CallLogsWithDetails
widget.
- Android
- iOS
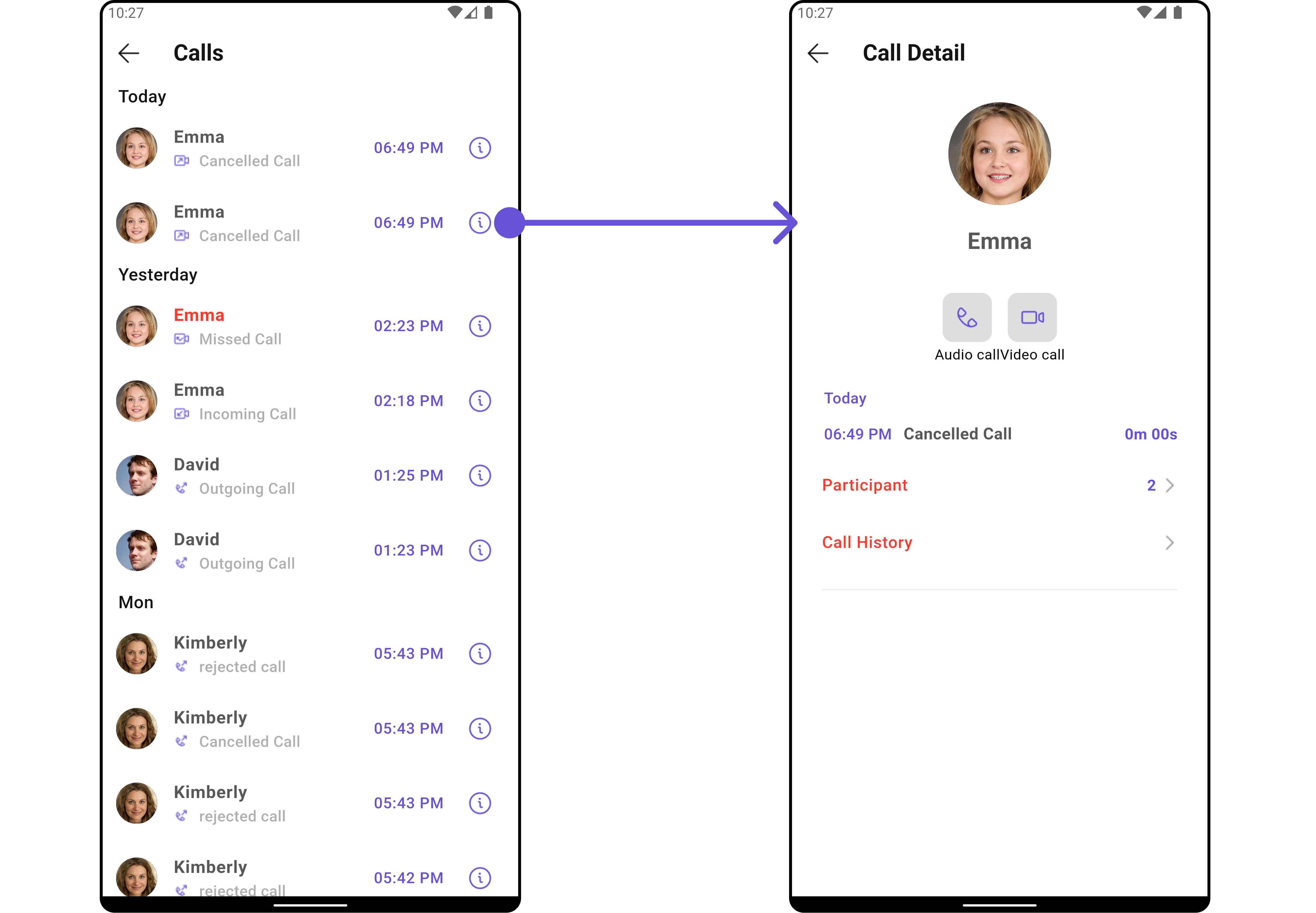
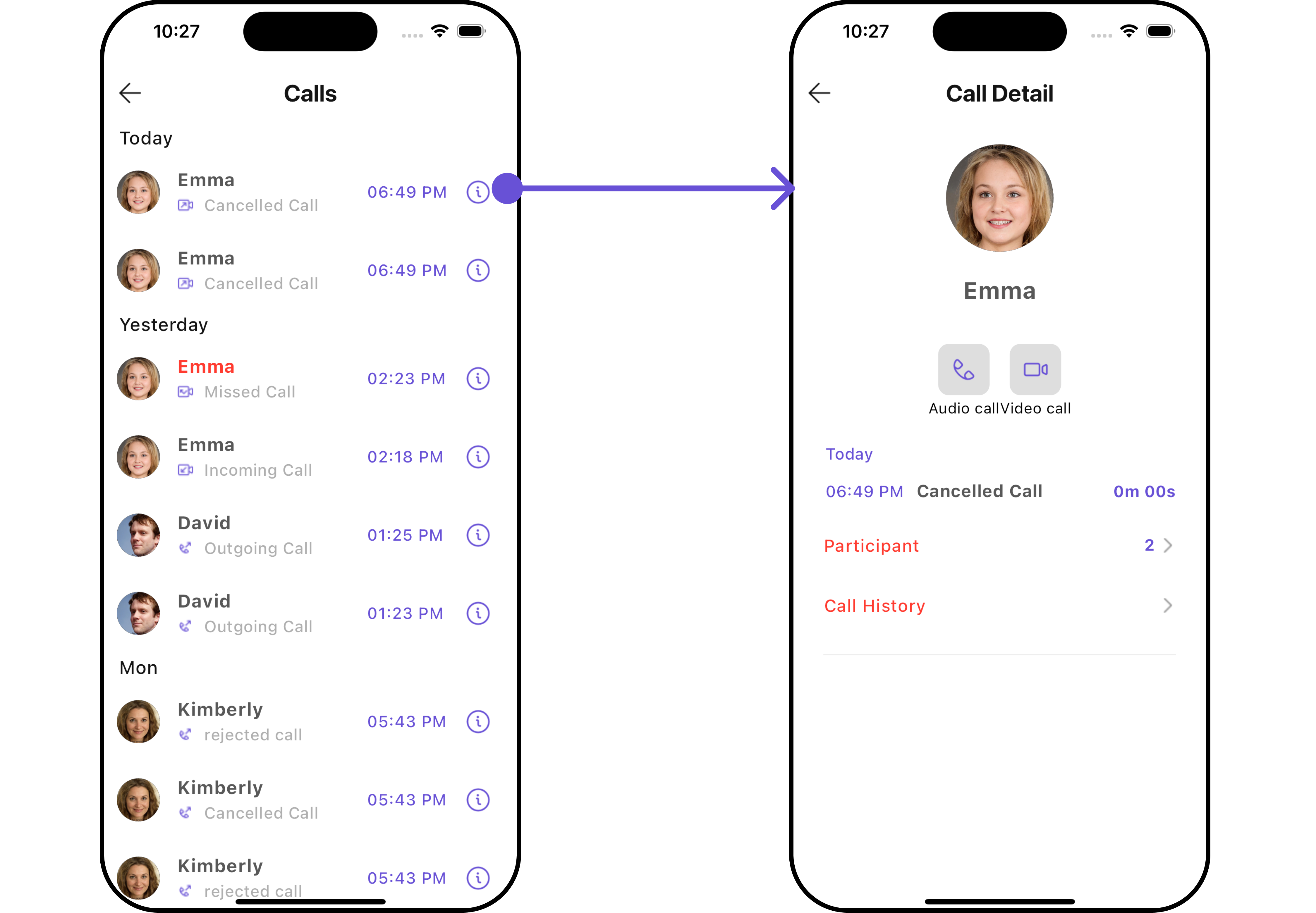
Widgets | Description |
---|---|
Call Logs | The Call Logs widget is designed to show the list of Call Log available . By default, names are shown for all listed users, along with their avatar if available. |
Call Log Details | The Call Log Details widget is designed to displays all the information related to a call. This widget displays information like user/group information, participants of the call, recordings of the call (if available) & history of all the previous calls. |
Usage
Integration
CometChatCallLogsWithDetails
is a composite widget that seamlessly integrates into your application. You can push it onto the navigation stack using a navigation controller. This allows for easy navigation and efficient display of call log details within your application's interface.
You can launch CometChatCallLogsWithDetails
directly using Navigator.push
, or you can define it as a widget within the build
method of your State
class.
1. Using Navigator to Launch CometChatCallLogsWithDetails
- Dart
Navigator.push(context, MaterialPageRoute(builder: (context) => CometChatCallLogsWithDetails()));
2. Embedding CometChatCallLogsWithDetails
as a Widget in the build Method
- Dart
import 'package:cometchat_calls_uikit/cometchat_calls_uikit.dart';
import 'package:flutter/material.dart';
class CallLogWithDetailsExample extends StatefulWidget {
const CallLogWithDetailsExample({super.key});
State<CallLogWithDetailsExample> createState() => _CallLogWithDetailsExampleState();
}
class _CallLogWithDetailsExampleState extends State<CallLogWithDetailsExample> {
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: CometChatCallLogsWithDetails(),
),
);
}
}
Actions
Actions dictate how a widget functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the widget to fit your specific needs.
1. onItemClick
This method proves valuable when users seek to override onItemClick
functionality within CometChatCallLogsWithDetails
, empowering them with greater control and customization options.
The onItemClick
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- Dart
CometChatCallLogsWithDetails(
callLogsConfiguration: CallLogsConfiguration(
onItemClick: (callLog) {
// TODO("Not yet implemented")
},
)
)
2. onError
You can customize this behavior by using the provided code snippet to override the onError
and improve error handling.
- Dart
CometChatCallLogsWithDetails(
callLogsConfiguration: CallLogsConfiguration(
onError: (e) {
// TODO("Not yet implemented")
},
),
callLogDetailConfiguration: CallLogDetailsConfiguration(
onError: (e) {
// TODO("Not yet implemented")
},
),
)
2. onBack
You can customize this behavior by using the provided code snippet to override the onBack
and improve error handling.
- Dart
CometChatCallLogsWithDetails(
callLogsConfiguration: CallLogsConfiguration(
onBack: () {
// TODO("Not yet implemented")
},
),
callLogDetailConfiguration: CallLogDetailsConfiguration(
onBack: () {
// TODO("Not yet implemented")
},
),
)
Filters
Filters allow you to customize the data displayed in a list within a Widget. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of ChatSDK.
While the CometChatCallLogsWithDetails
widget does not have filters, its widgets do, For more detail on individual filters of its widget refer to Call Logs and CometChatCallLogsWithDetails.
By utilizing the Configurations object of its widgets, you can apply filters.
1. CallLogRequestBuilder
The CallLogRequestBuilder enables you to filter and customize the call list based on available parameters in CallLogRequestBuilder. This feature allows you to create more specific and targeted queries during the call. The following are the parameters available in CallLogRequestBuilder
- Dart
CometChatCallLogsWithDetails(
callLogsConfiguration: CallLogsConfiguration(
callLogsRequestBuilder: CallLogRequestBuilder()
..limit = 10
..hasRecording = true,
),
)
List of properties exposed by CallLogRequestBuilder
Property | Description | Code |
---|---|---|
Auth Token | Sets the authentication token. | authToken: String? |
Call Category | Sets the category of the call. | callCategory: String? |
Call Direction | Sets the direction of the call. | callDirection: String? |
Call Status | Sets the status of the call. | callStatus: String? |
Call Type | Sets the type of the call. | callType: String? |
Guid | Sets the unique ID of the group involved in the call. | guid: String? |
Has Recording | Indicates if the call has a recording. | hasRecording: bool |
Limit | Sets the maximum number of call logs to return per request. | limit: int |
Uid | Sets the unique ID of the user involved in the call. | uid: String? |
Events
Events are emitted by a Widget
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The CometChatCallLogsWithDetails
does not produce any events but its subwidget does.
Customization
To fit your app's design requirements, you can customize the appearance of the conversation widget. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the widget in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the widget.
It's important to note that while CometChatCallLogsWithDetails
does not provide its own specific styling options, each individual widget contained within it offers its own set of styling attributes.
You can also customize its widget styles. For more details on individual widget styles, you can refer Call Logs Styles and Call Log Details Styles.
- Dart
CometChatCallLogsWithDetails(
callLogsConfiguration: CallLogsConfiguration(
callLogsStyle: CallLogsStyle(
// Add here CallLog Style
),
),
callLogDetailConfiguration: CallLogDetailsConfiguration(
detailStyle: CallLogDetailsStyle(
// Add here CallLogDetails Style
),
),
)
- Android (Call Logs Style)
- Android (Call Log Details Style)
- iOS (Call Logs Style)
- iOS (Call Log Details Style)
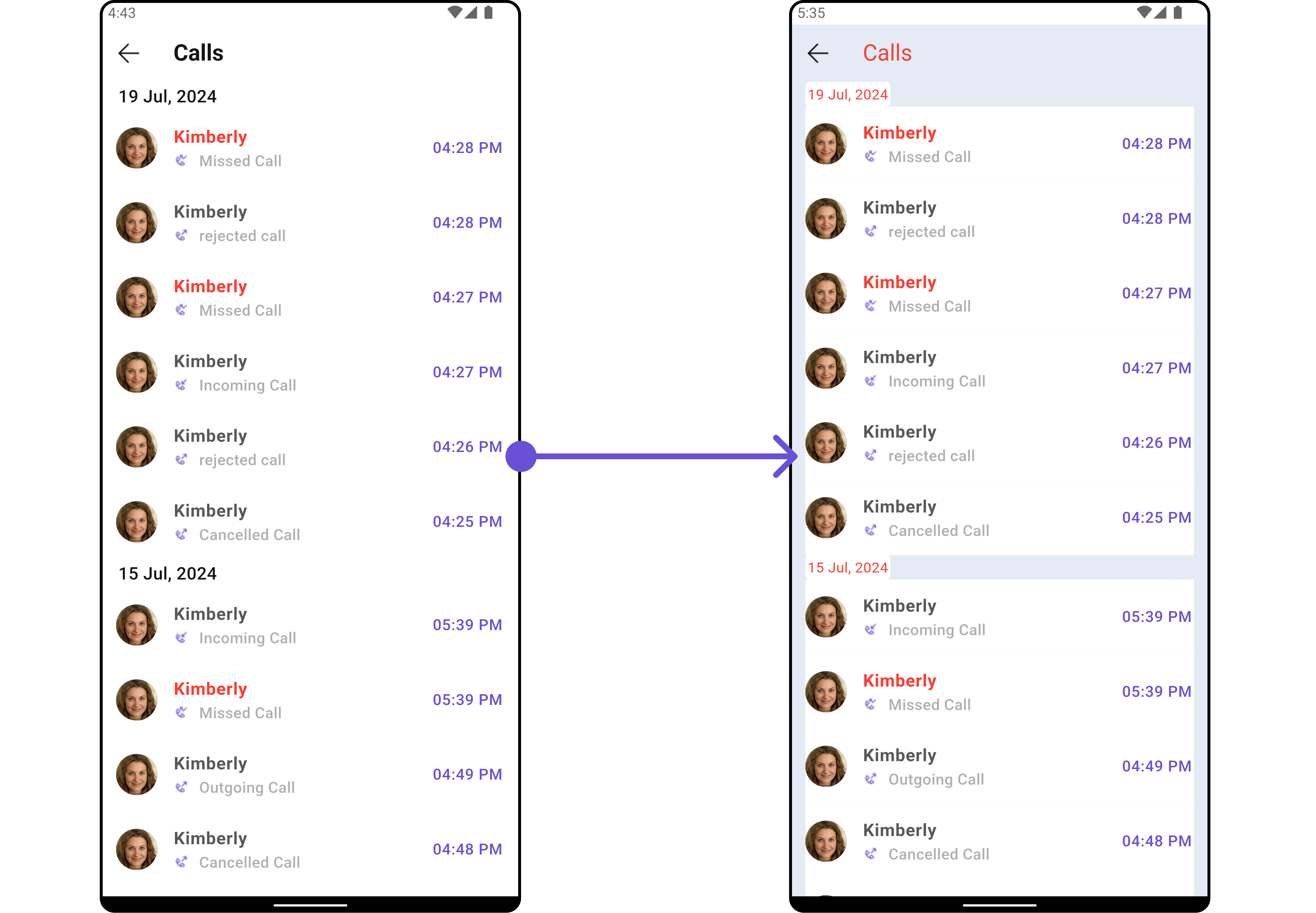
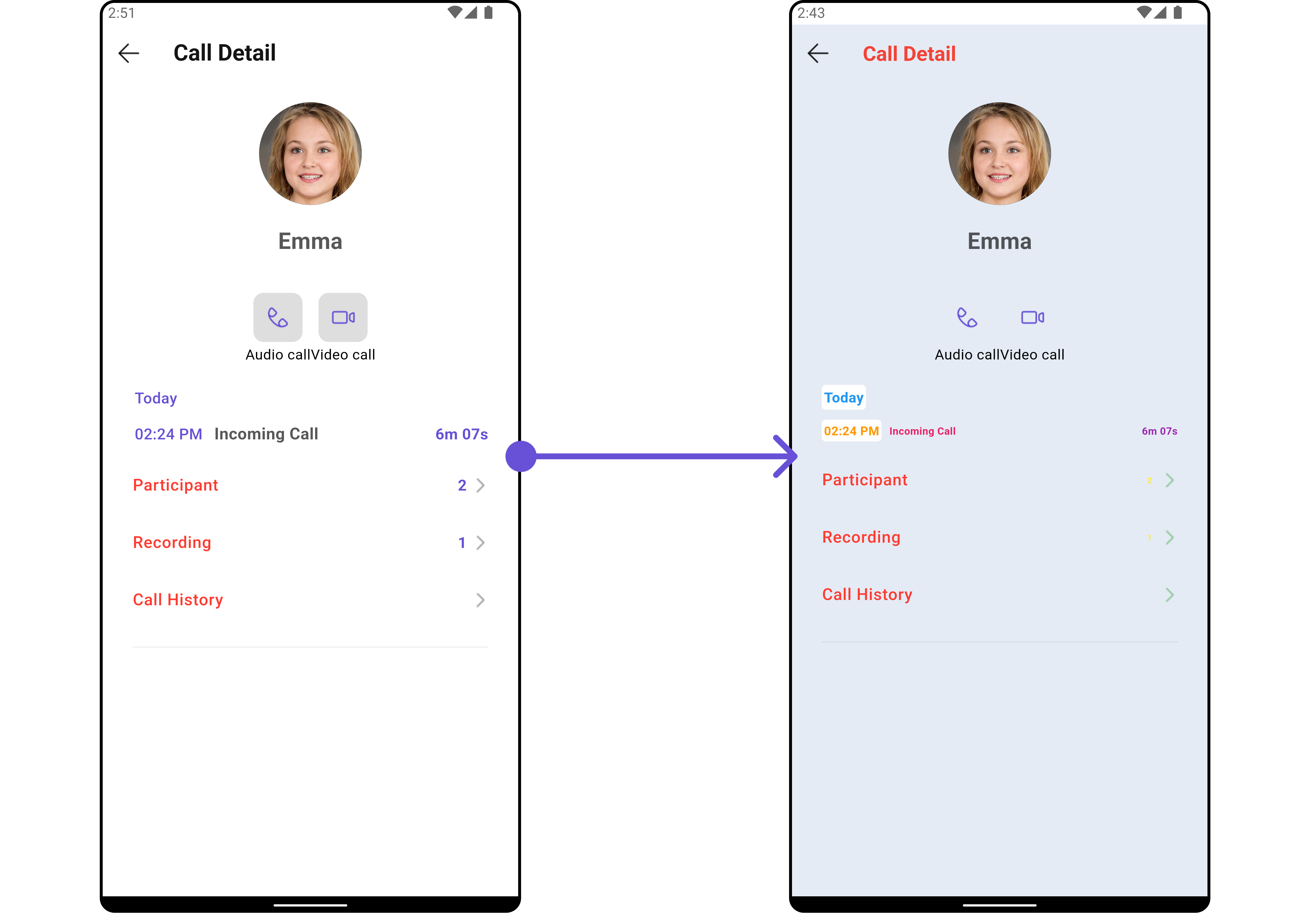
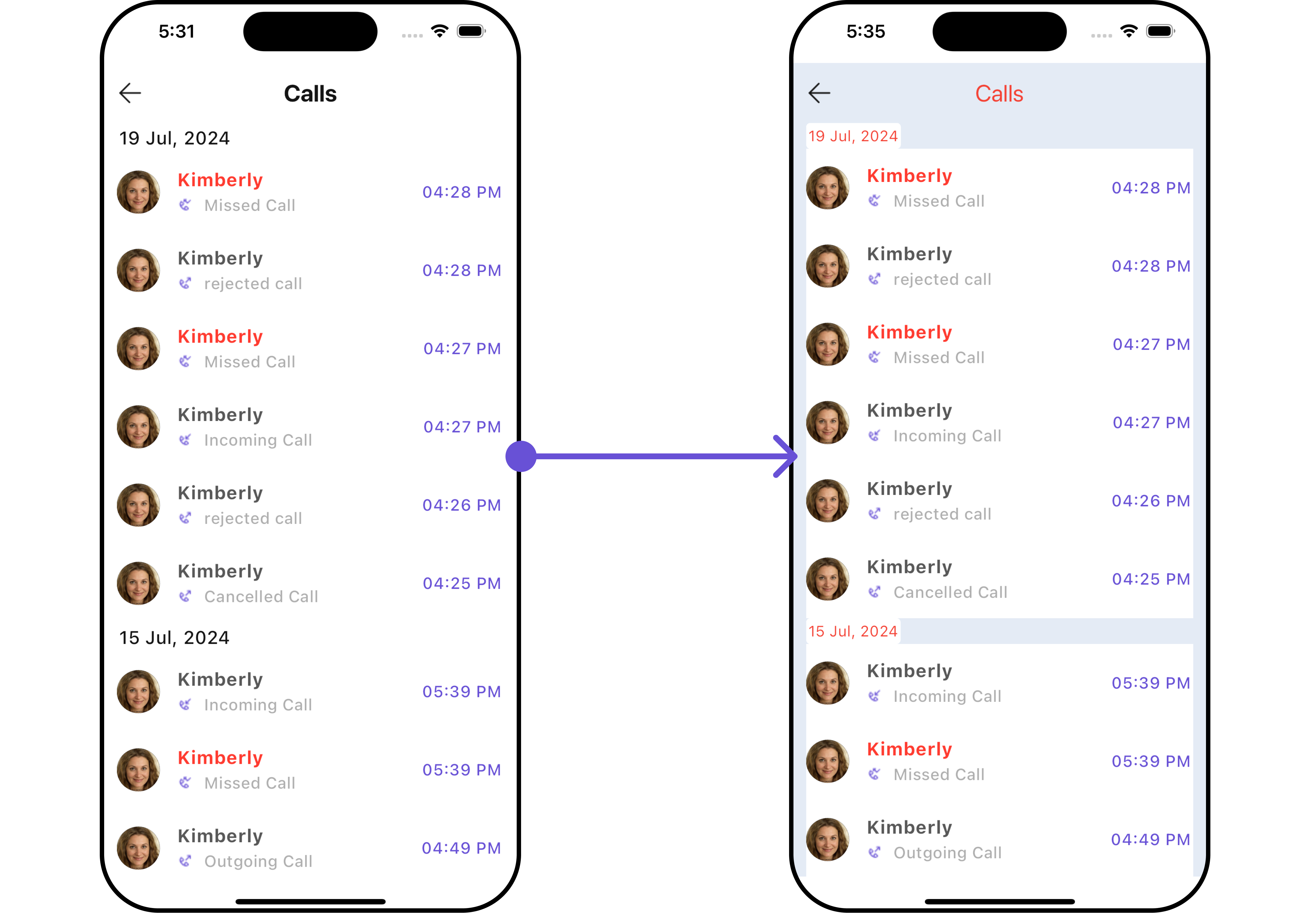
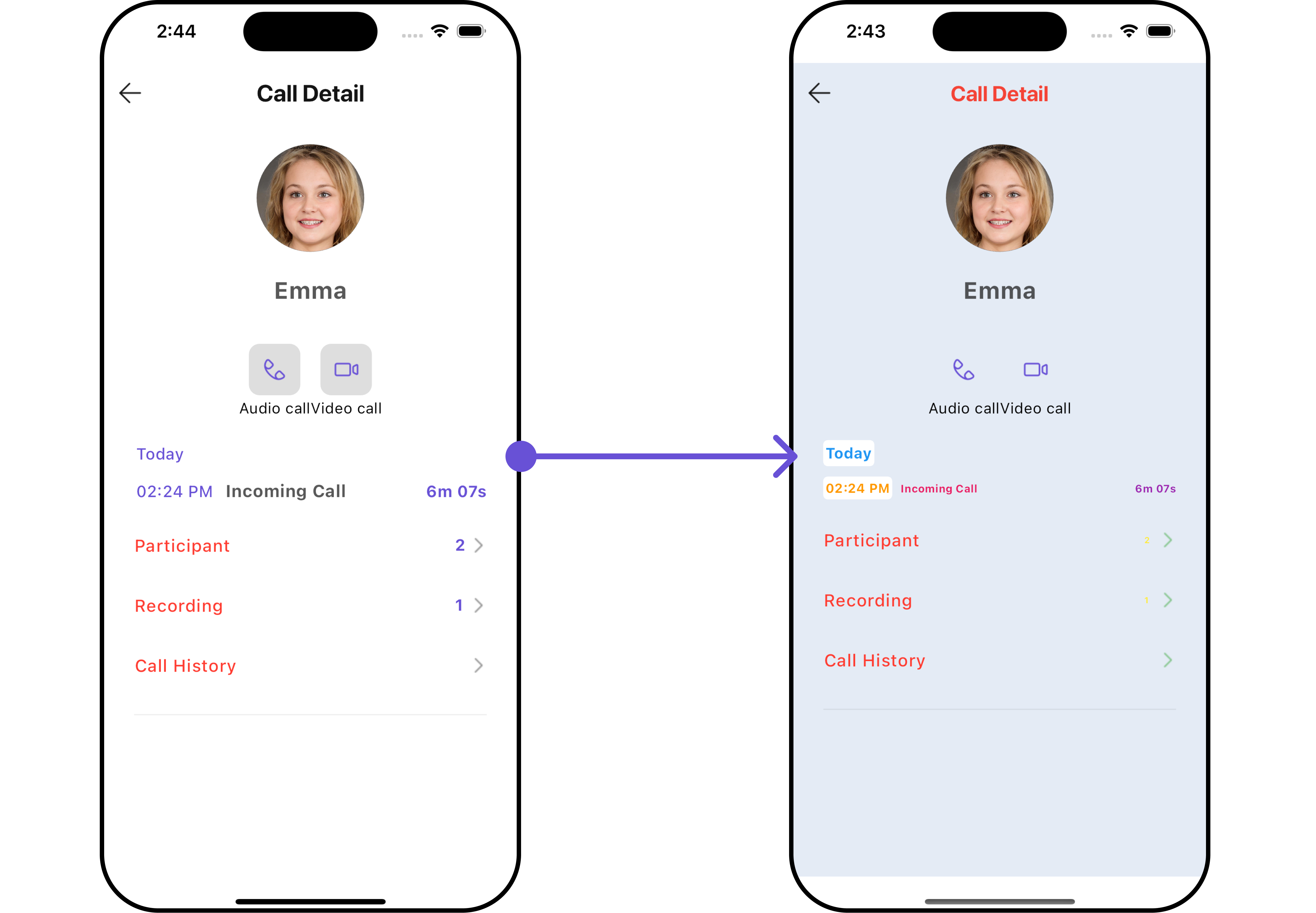
Styles can be applied to SubWidgets using their respective configurations.
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the widget. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
You can also customize its widget functionality. For more details on individual widget functionality, you can refer Call Logs Functionality and Call Log Details Functionality.
- Dart
CometChatCallLogsWithDetails(
callLogsConfiguration: CallLogsConfiguration(
// CallLog functionality
),
callLogDetailConfiguration: CallLogDetailsConfiguration(
// CallLogDetails functionality
),
)
- Android (Call Logs Functionality)
- Android Functionality
- iOS (Call Logs Functionality)
- iOS (Call Log Details Functionality)
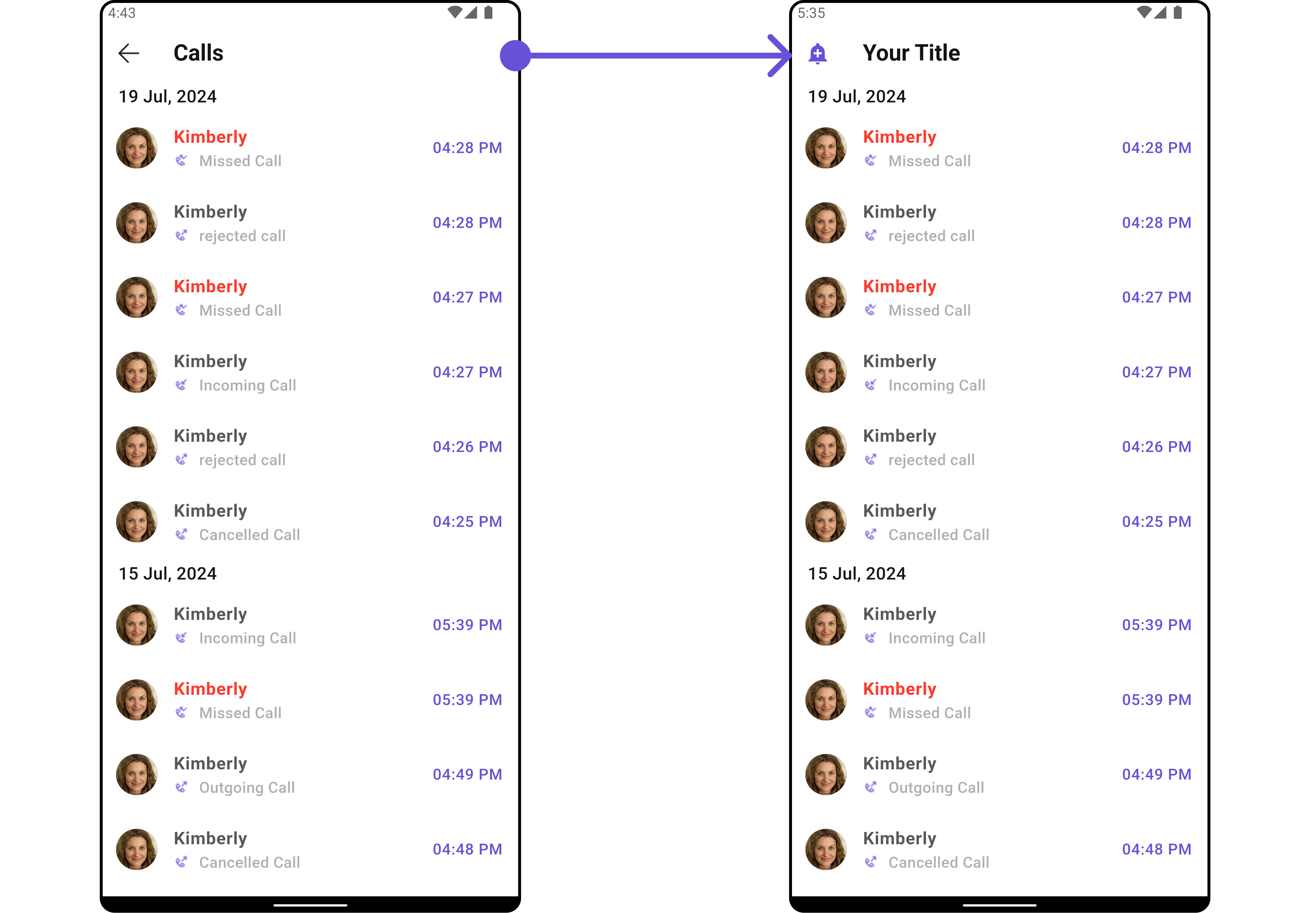
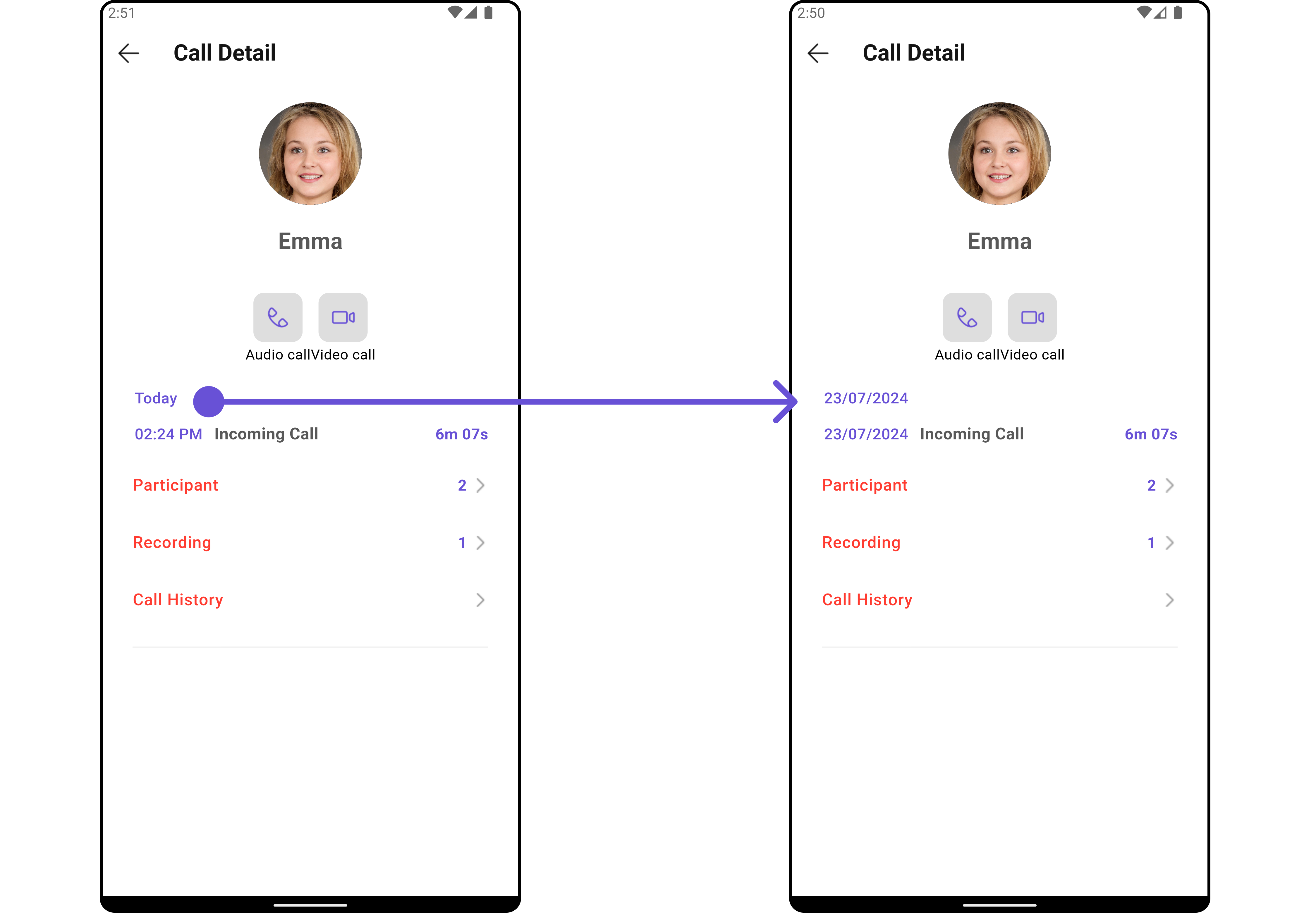
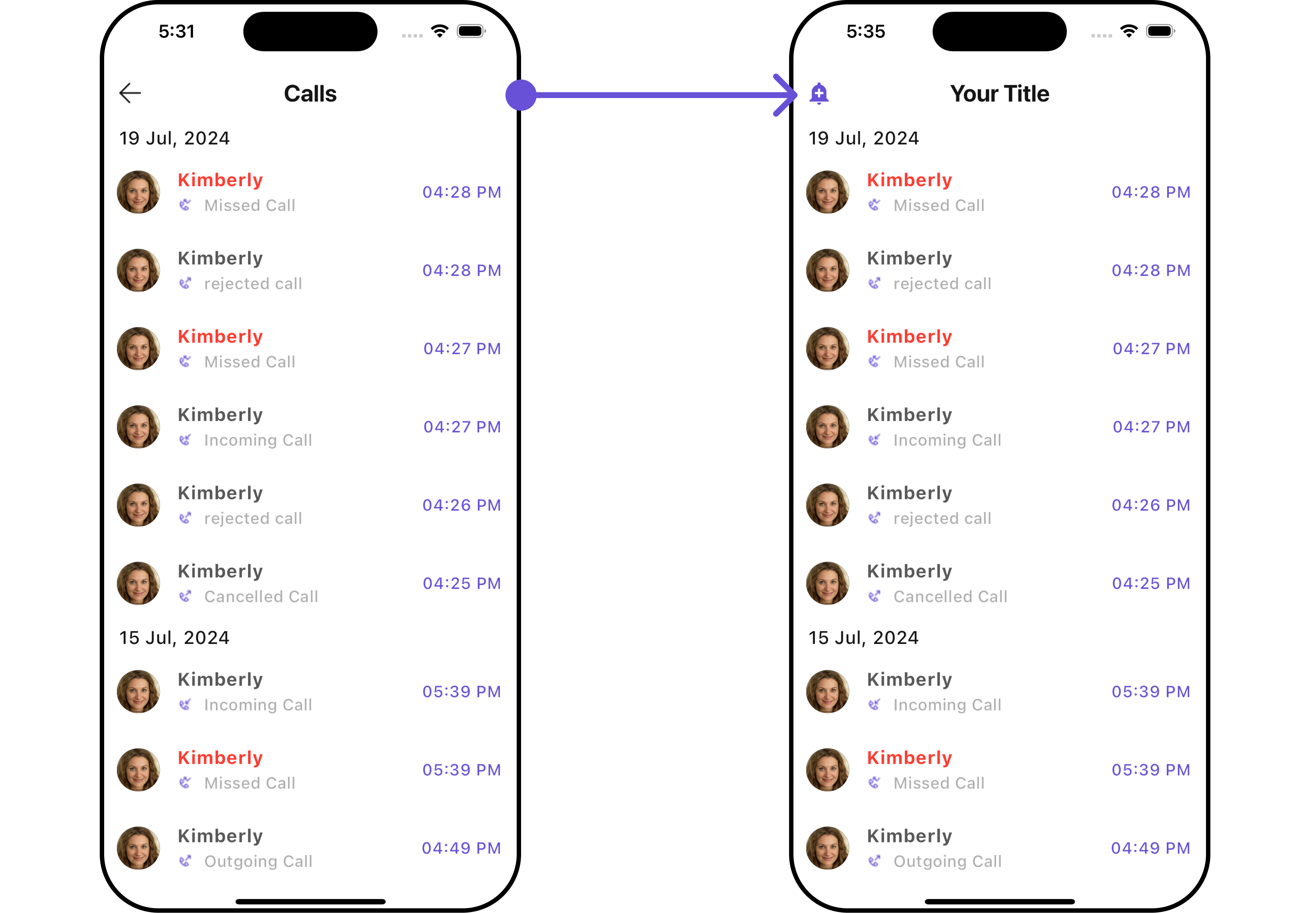
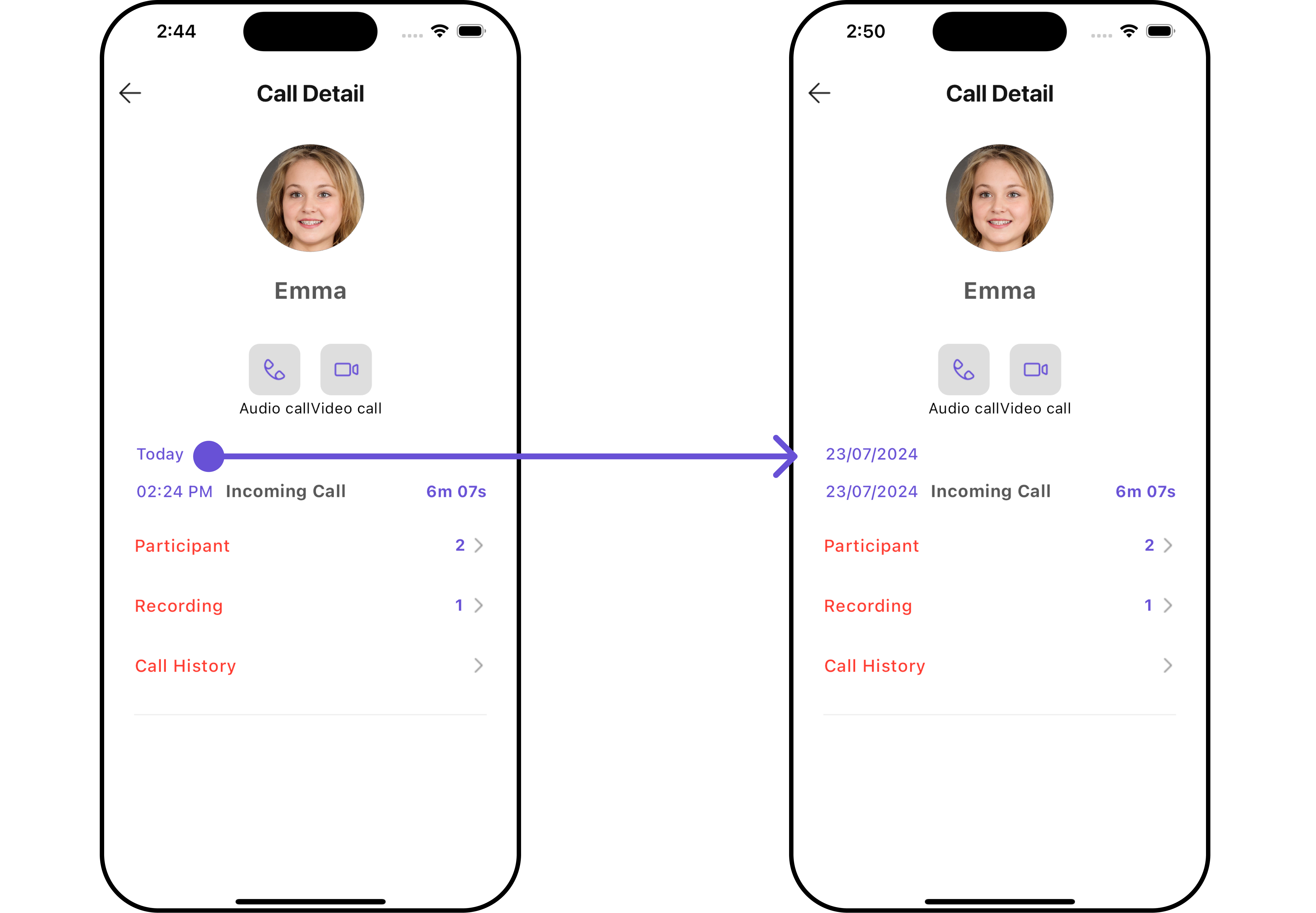
Functionality can be applied to SubWidgets using their respective configurations.
Advanced
For advanced-level customization, you can set custom views to the widget. This lets you tailor each aspect of the widget to fit your exact needs and application aesthetics. You can create and define your own views, layouts, and UI elements and then incorporate those into the widget.
For the details of individual widgets' advanced-level customization, you can refer to Call Logs Advanced Section and Call Log Details Advanced Section.
- Dart
CometChatCallLogsWithDetails(
callLogsConfiguration: CallLogsConfiguration(
// CallLog advanced-level customization
),
callLogDetailConfiguration: CallLogDetailsConfiguration(
// CallLogDetails advanced-level customization
),
)
By utilizing the Configuration object of each widget, you can apply advanced-level customizations to the GroupsWithMessages.
Configurations
Configurations offer the ability to customize the properties of each widget within a Composite Widget.
CometChatCallLogsWithDetails
has Call Logs and Call Log Details widget. Hence, each of these widgets will have its individual Configuration
.
Configurations
expose properties that are available in its individual widgets.
Call Logs
You can customize the properties of the Call Logs by making use of the CallLogsConfiguration
. You can accomplish this by employing the callLogsConfiguration
props as demonstrated below:
- Dart
CometChatCallLogsWithDetails(
callLogsConfiguration: CallLogsConfiguration(
// Override the properties of CallLog
)
)
All exposed properties of CallLogsConfiguration
can be found under Call Logs. Properties marked with the symbol are not accessible within the Configuration Object.
Call Log Details
You can customize the properties of the Call Log Details widget by making use of the CallLogDetailsConfiguration
. You can accomplish this by employing the callLogDetailConfiguration
props as demonstrated below:
- Dart
CometChatCallLogsWithDetails(
callLogDetailConfiguration: CallLogDetailsConfiguration(
// Override the properties of CallLogDetails
)
)
All exposed properties of CallLogDetailsConfiguration
can be found under Call Log Details. Properties marked with the symbol are not accessible within the Configuration Object.