Outgoing Call
Overview
The CometChatOutgoingCall
Widget is a visual representation of a user-initiated call, whether it's a voice or video call. It serves as an interface for managing outgoing calls, providing users with essential options to control the call experience. This Widget typically includes information about the call recipient, call controls for canceling the call, and feedback on the call status, such as indicating when the call is in progress.
- Android
- iOS
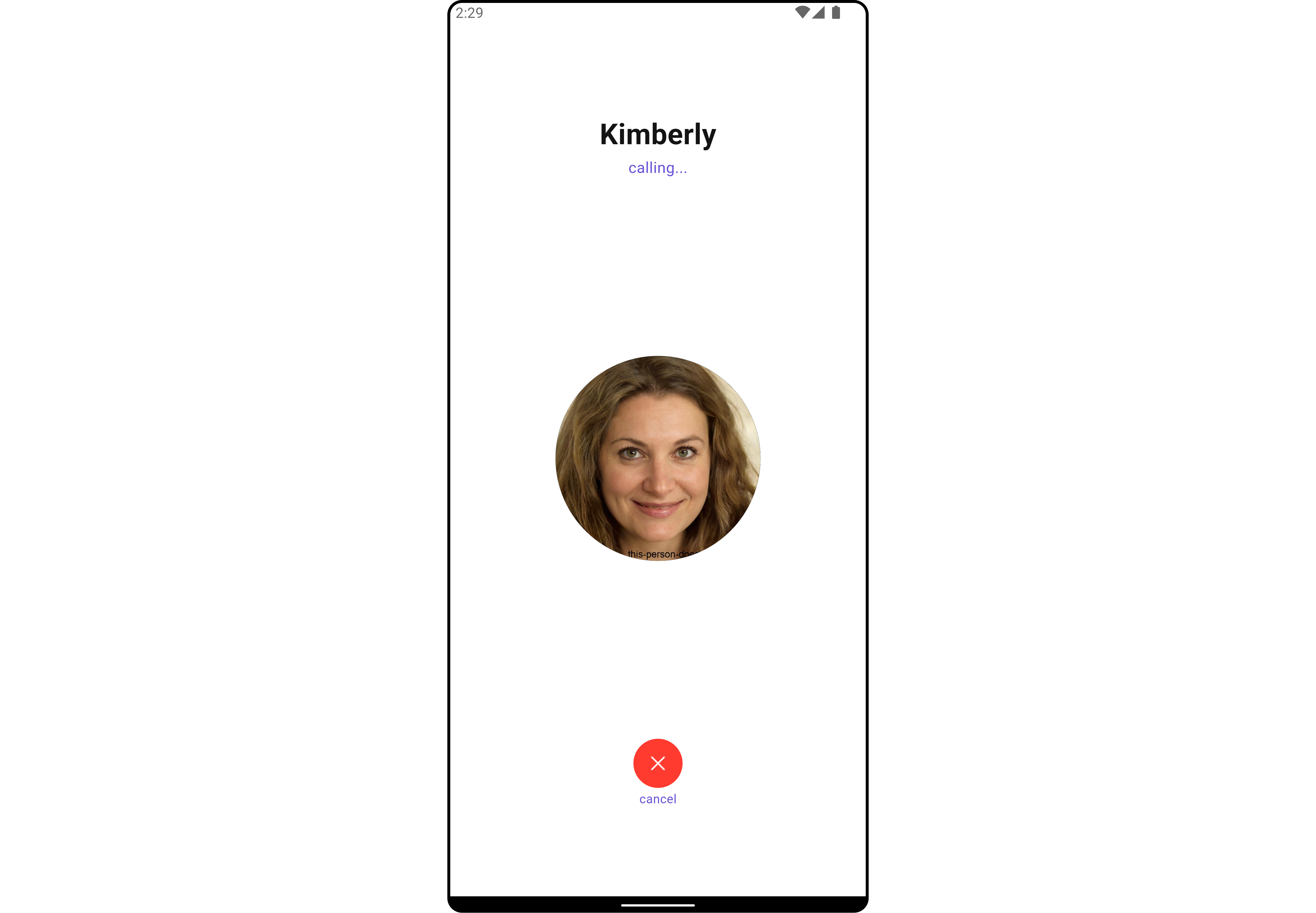
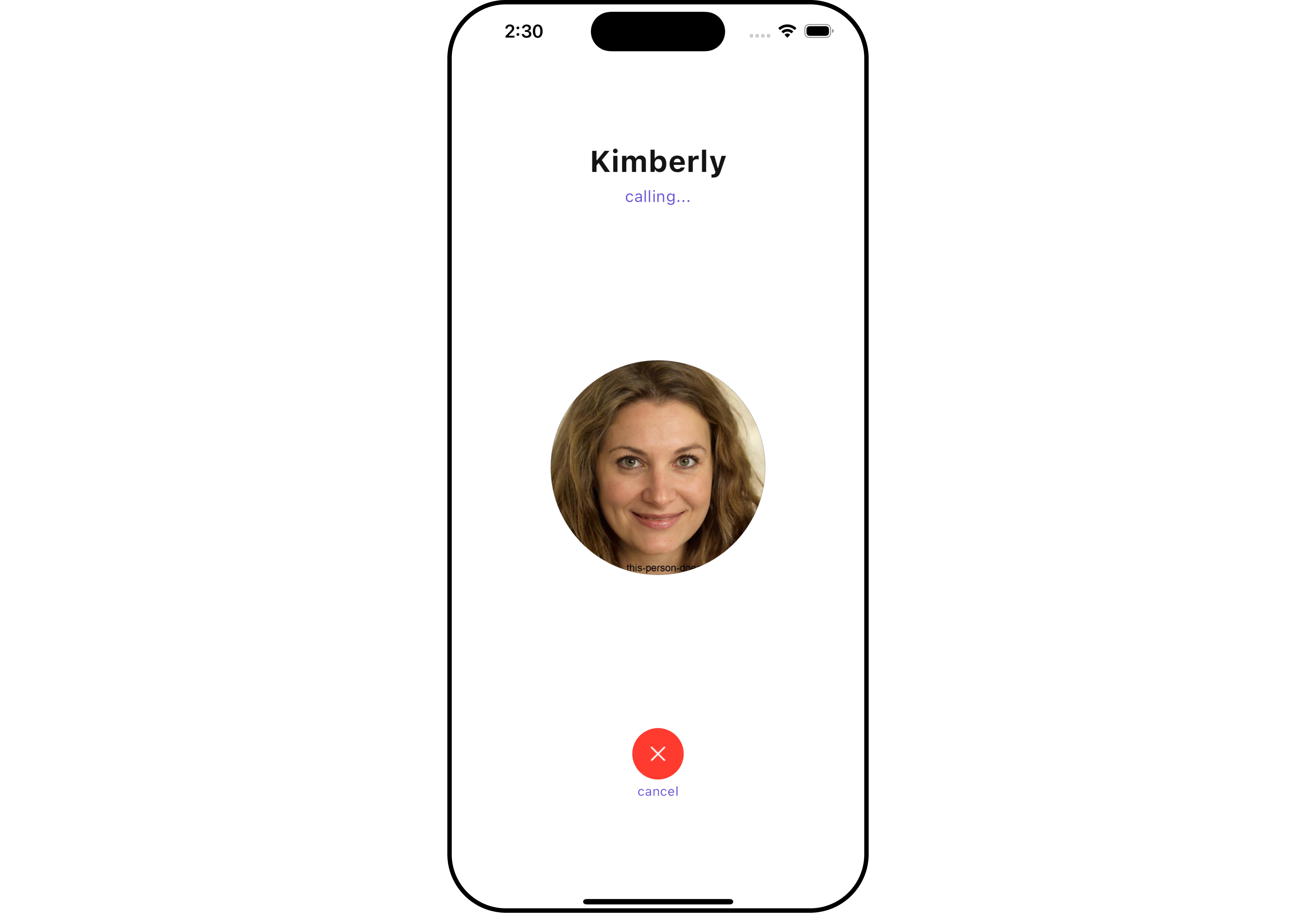
You can launch CometChatOutgoingCall
directly using Navigator.push
, or you can define it as a widget within the build
method of your State
class.
1. Using Navigator to Launch CometChatOutgoingCall
- Dart
Navigator.push(context, MaterialPageRoute(builder: (context) => CometChatOutgoingCall(user: user, call: callObject))); // User object and Call object is required to launch the incoming call widget.
2. Embedding CometChatOutgoingCall
as a Widget in the build Method
- Dart
import 'package:cometchat_calls_uikit/cometchat_calls_uikit.dart';
import 'package:flutter/material.dart';
class OutgoingCallExample extends StatefulWidget {
const OutgoingCallExample({super.key});
State<OutgoingCallExample> createState() => _OutgoingCallExampleState();
}
class _OutgoingCallExampleState extends State<OutgoingCallExample> {
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: CometChatOutgoingCall(
user: user, // User Object
call: callObject
), // User object and Call object is required to launch the incoming call widget.
),
);
}
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onDecline
The onDecline
action is typically triggered when the call is ended, carrying out default actions. However, with the following code snippet, you can effortlessly customize or override this default behavior to meet your specific needs.
- Dart
CometChatOutgoingCall(
user: user, // User Object
call: callObject, // Call Object
onDecline: (BuildContext context, Call call) {
// TODO("Not yet implemented")
},
)
2. onError
You can customize this behavior by using the provided code snippet to override the onError
and improve error handling.
- Dart
CometChatOutgoingCall(
user: user, // User Object
call: callObject, // Call Object
onError: (e) {
// TODO("Not yet implemented")
},
)
Filters
Filters allow you to customize the data displayed in a list within a Widget. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
The CometChatOutgoingCall
Widget does not have any exposed filters.
Events
Events are emitted by a Widget
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
Events emitted by the Outgoing call Widget are as follows.
Event | Description |
---|---|
ccCallAccepted | Triggers when the outgoing call is accepted. |
ccCallRejected | Triggers when the outgoing call is rejected. |
Example
Here is the complete example for reference:
- Dart
import 'package:cometchat_chat_uikit/cometchat_chat_uikit.dart';
import 'package:flutter/material.dart';
class YourScreen extends StatefulWidget {
const YourScreen({super.key});
State<YourScreen> createState() => _YourScreenState();
}
class _YourScreenState extends State<YourScreen> with CometChatCallEventListener {
void initState() {
super.initState();
CometChatCallEvents.addCallEventsListener("unique_listener_ID", this); // Add the listener
}
void dispose(){
super.dispose();
CometChatCallEvents.removeCallEventsListener("unique_listener_ID"); // Remove the listener
}
void ccCallAccepted(Call call) {
// TODO("Not yet implemented")
}
void ccCallRejected(Call call) {
// TODO("Not yet implemented")
}
Widget build(BuildContext context) {
return const Placeholder();
}
}
Customization
To fit your app's design requirements, you can customize the appearance of the conversation widget. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the widget in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the widget.
1. OutgoingCall Style
You can customize the appearance of the OutgoingCallStyle
Widget by applying the OutgoingCallStyle
to it using the following code snippet.
Example
Here is the complete example for reference:
- Dart
CometChatOutgoingCall(
user: user, // User Object
call: callObject, // Call Object
outgoingCallStyle: OutgoingCallStyle(
background: Color(0xFFE4EBF5),
declineButtonTextStyle: const TextStyle(
color: Colors.red,
fontSize: 16,
backgroundColor: Colors.black12,
),
border: Border.all(color: Colors.grey, width: 5),
borderRadius: 50,
),
)
- Android
- iOS
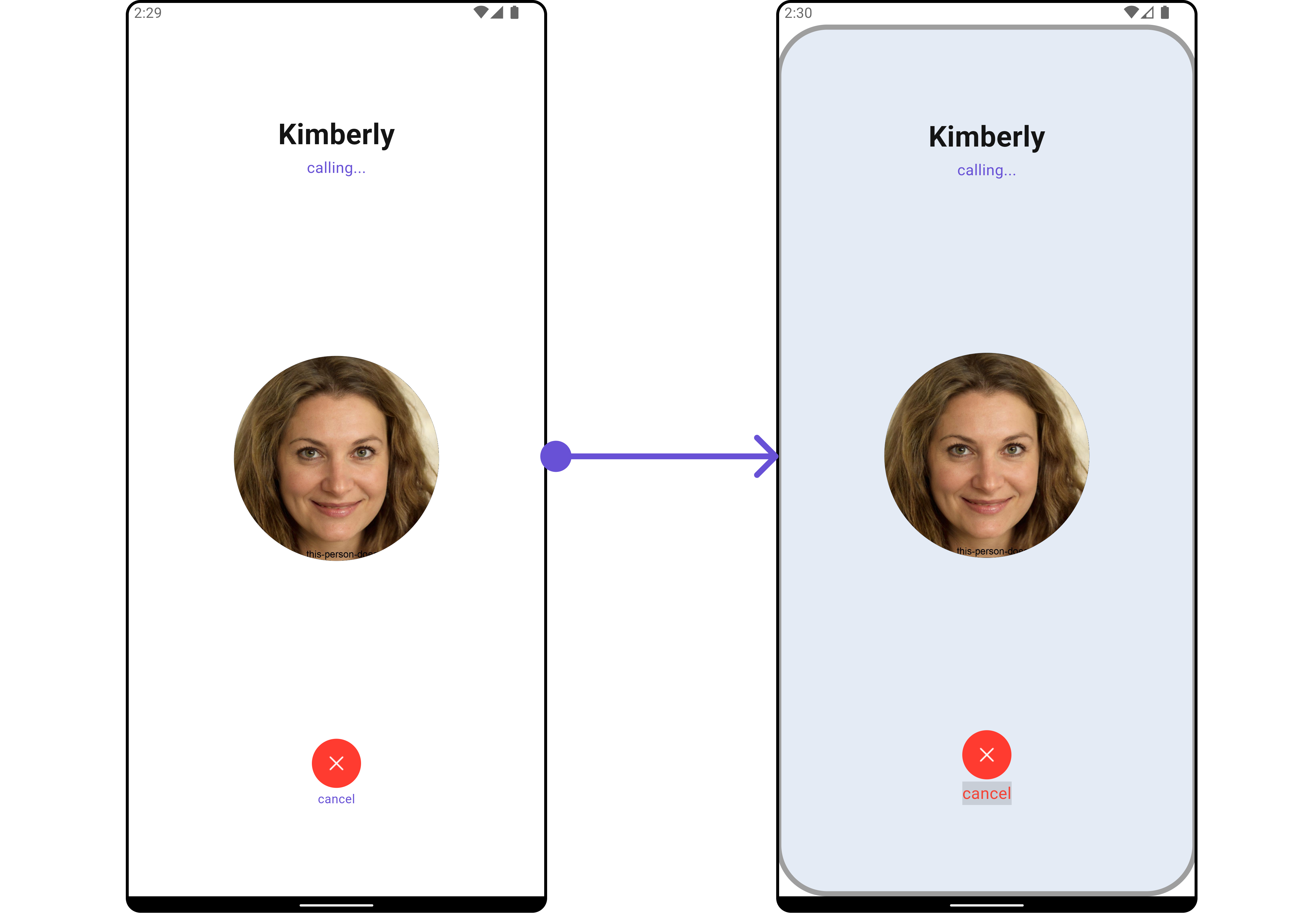
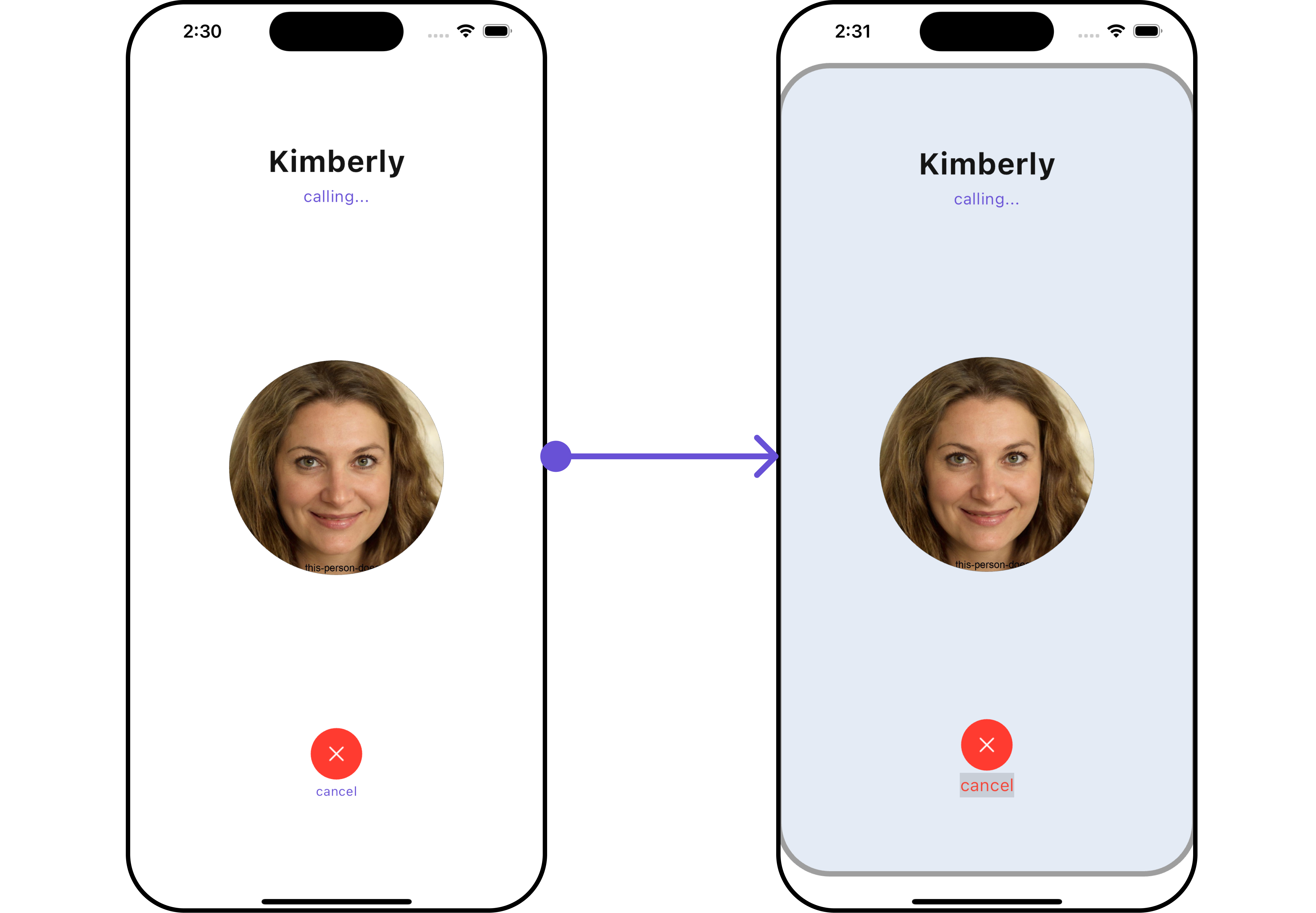
List of properties exposed by OutgoingCallStyle
Property | Description | Code |
---|---|---|
Background | Sets the background color of the outgoing call style. | background: Color? |
Border | Sets the border properties of the outgoing call style. | border: BoxBorder? |
Border Radius | Sets the border radius of the outgoing call style. | borderRadius: double? |
Decline Button Text Style | Sets the text style for the decline button. | declineButtonTextStyle: TextStyle? |
Gradient | Sets the gradient applied to the outgoing call style. | gradient: Gradient? |
Height | Sets the height of the outgoing call style. | height: double? |
Width | Sets the width of the outgoing call style. | width: double? |
2. Avatar Styles
To apply customized styles to the Avatar
widget in the CometChatIncomingCall
Widget, you can use the following code snippet. For further insights on Avatar
Styles refer
Example
Here is the complete example for reference:
- Dart
CometChatOutgoingCall(
user: user, // User Object
call: callObject, // Call Object
avatarStyle: AvatarStyle(
border: Border.all(width: 5),
borderRadius: 20,
background: Colors.red
),
)
3. Button Style
You can customize the appearance of the Decline Button Widget by applying the buttonStyle
to it using the following code snippet.
Example
Here is the complete example for reference:
- Dart
import 'package:cometchat_calls_uikit/cometchat_calls_uikit.dart' as uikit;
CometChatOutgoingCall(
user: user, // User Object
call: callObject, // Call Object
buttonStyle: uikit.ButtonStyle(
background: Colors.brown,
border: Border.all(color: Colors.black),
borderRadius: 10,
textStyle: const TextStyle(color: Colors.red),
iconTint: Colors.white,
),
)
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the widget. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
Example
In this example, we're enhancing the interface by customizing the decline button icons. By setting custom icons for decline buttons, users can enjoy a more visually appealing and personalized experience.
This level of customization allows developers to tailor the user interface to match the overall theme and branding of their application.
Example
Here is the example for reference:
- Dart
CometChatOutgoingCall(
user: user, // User Object
call: callObject, // Call Object
subtitle: "VOIP Call",
disableSoundForCalls: true,
declineButtonText: "Decline",
)
- Android
- iOS
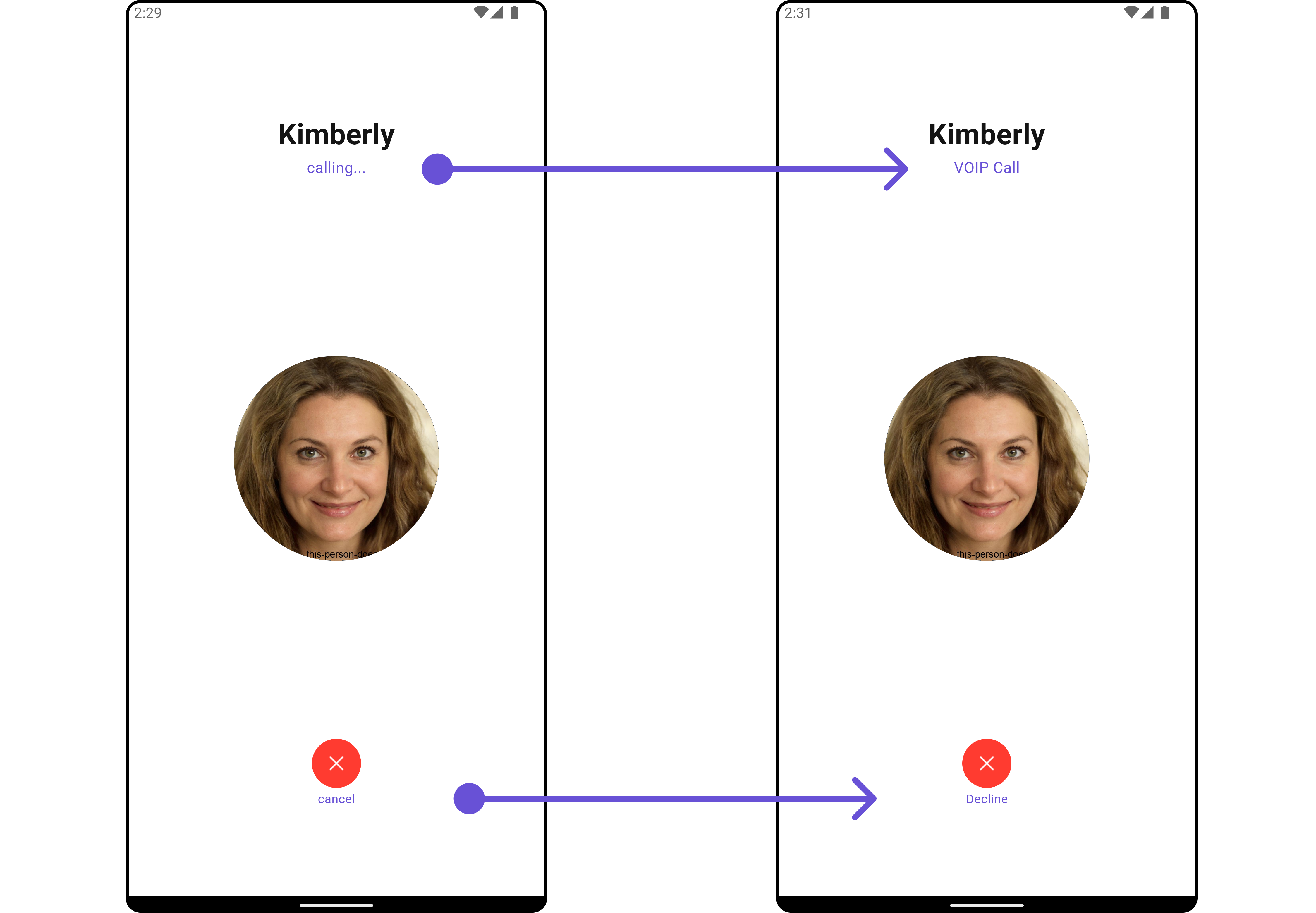
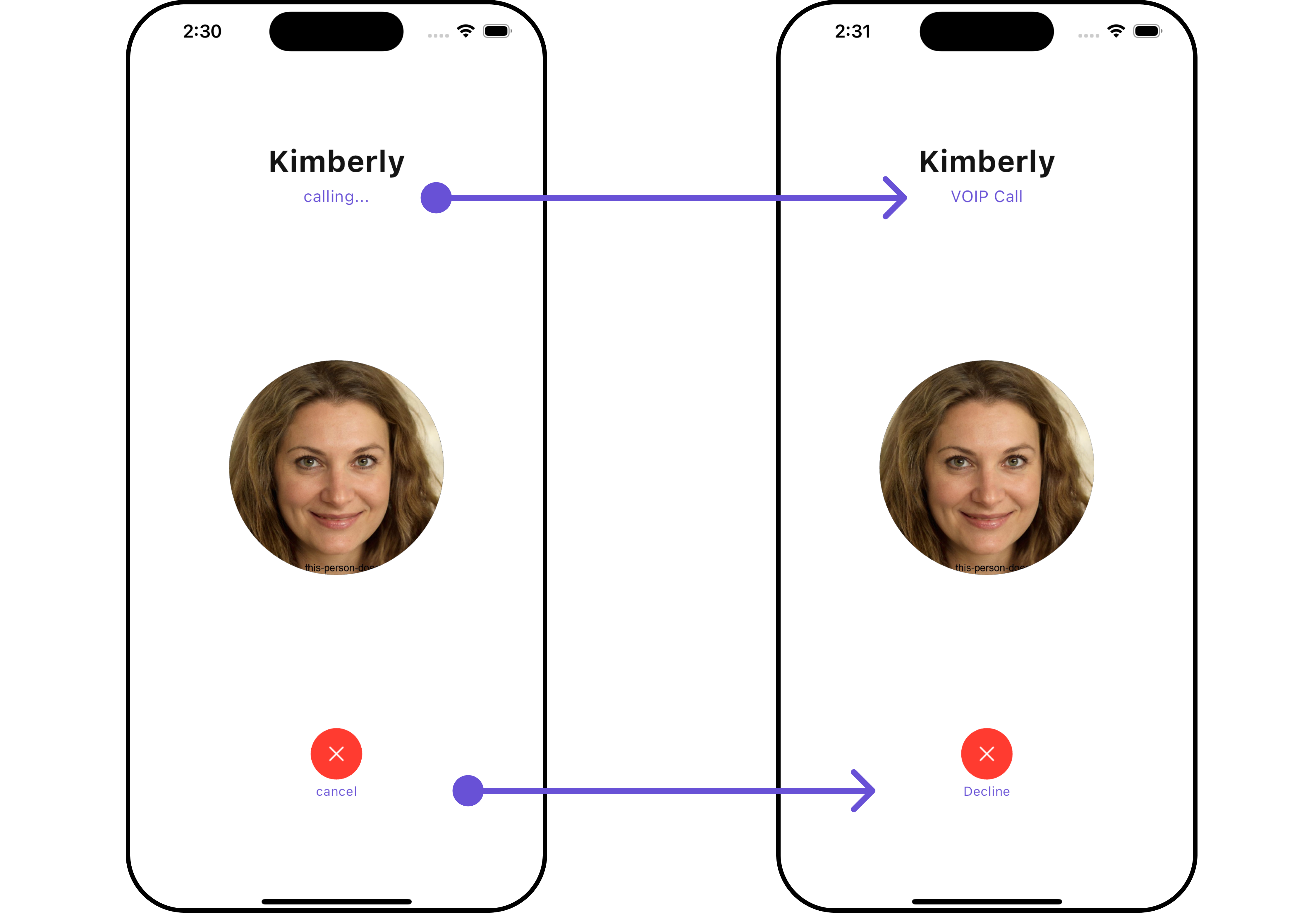
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
Custom Sound For Calls | Sets the custom sound for outgoing calls. | customSoundForCalls: String? |
Custom Sound For Calls Package | Sets the package for the custom sound for outgoing calls. | customSoundForCallsPackage: String? |
Decline Button Icon Url | Sets the URL for the decline button icon. | declineButtonIconUrl: String? |
Decline Button Icon Url Package | Sets the package for the decline button icon URL. | declineButtonIconUrlPackage: String? |
Decline Button Text | Sets the text for the decline button. | declineButtonText: String? |
Disable Sound For Calls | Disables sound for outgoing calls. | disableSoundForCalls: bool? |
Subtitle | Sets the subtitle for the outgoing call screen. | subtitle: String? |
Advanced
For advanced-level customization, you can set custom widgets to the widget. This lets you tailor each aspect of the widget to fit your exact needs and application aesthetics. You can create and define your widgets, layouts, and UI elements and then incorporate those into the widget.
The CometChatOutgoingCall
widget does not provide additional functionalities beyond this level of customization.