Conversations with Messages
Overview
The ConversationsWithMessages is a Composite Component encompassing components such as Conversations, Messages, and Contacts. Each of these component contributes to the functionality and structure of the overall ConversationsWithMessages component.
- iOS
- Android
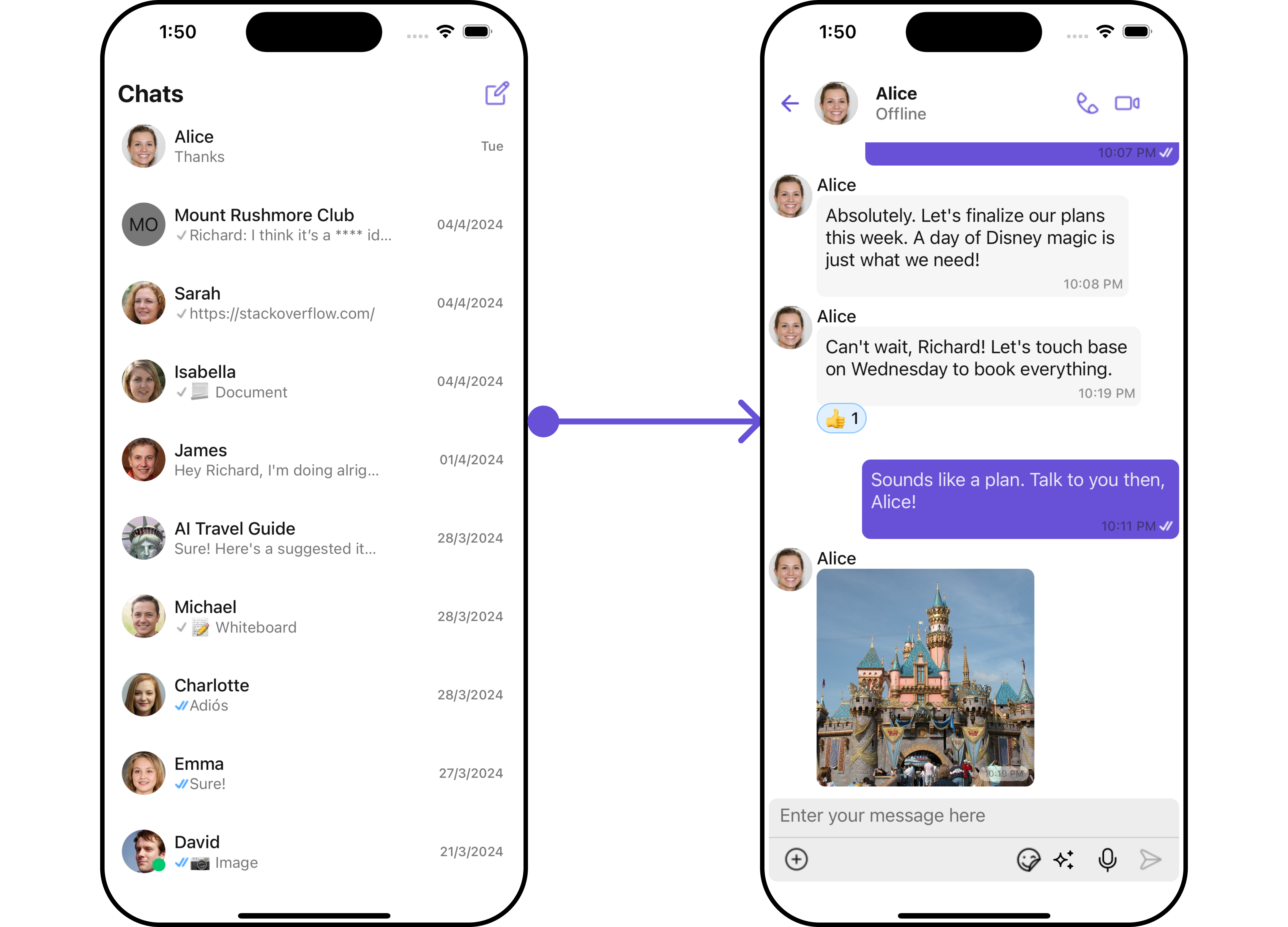
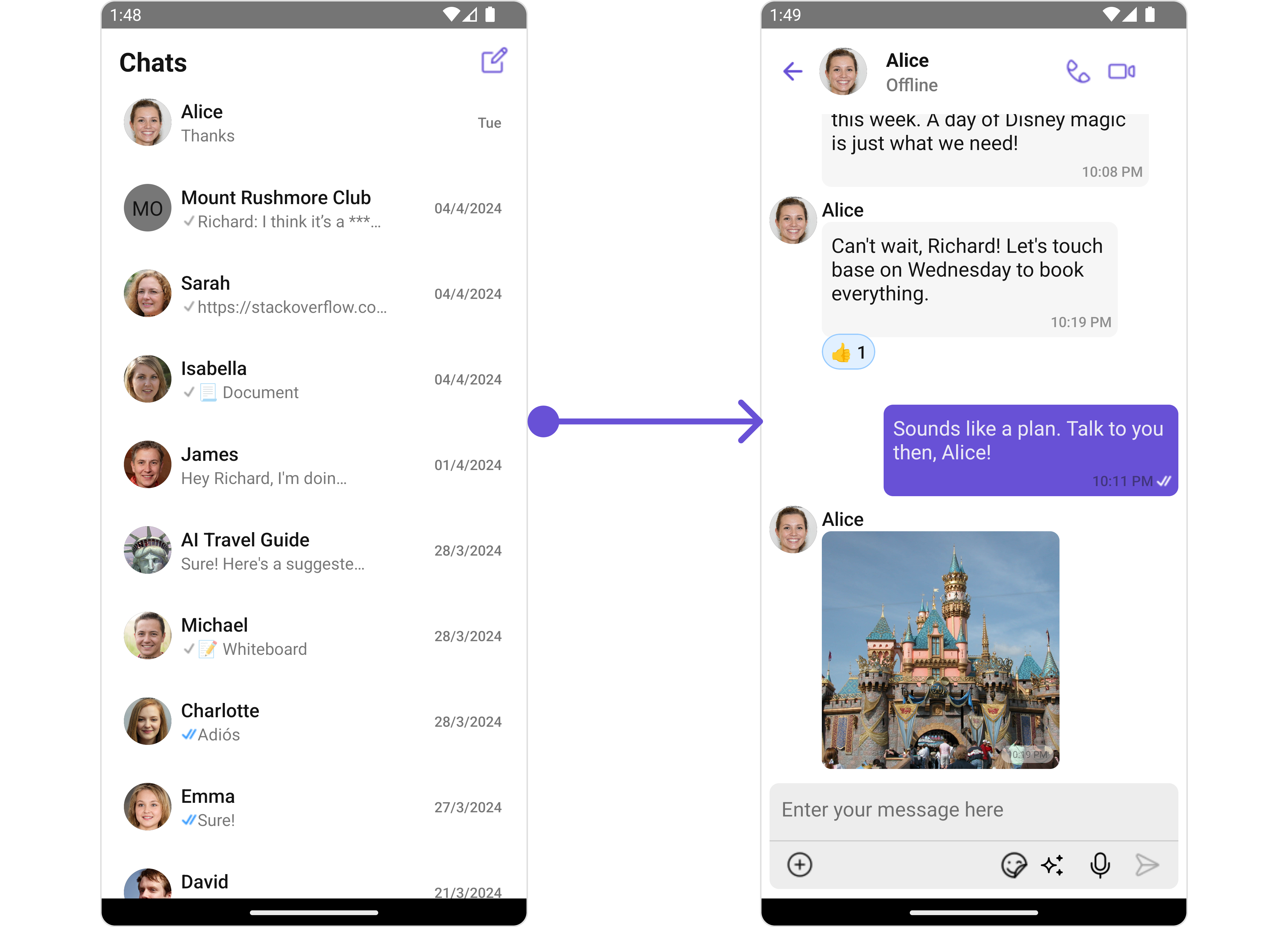
Components | Description |
---|---|
Conversations | The Conversations component is designed to display a list of either User or Group . This essentially represents your recent conversation history. |
Messages | The Messages component is designed to manage the messaging interaction for either individual User or Group conversations. |
Contacts | The CometChatContacts component is specifically designed to facilitate the display and management of both User and Groups . |