Threaded Messages Header
Overview
CometChatThreadedMessagePreview is a Component that displays the parent message & number of replies of thread.
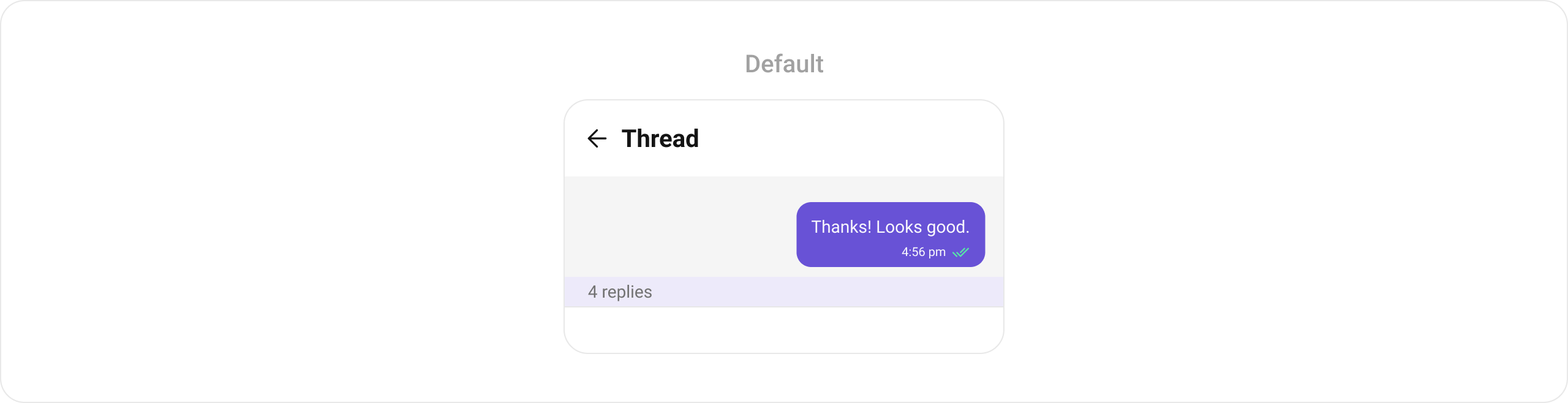
Usage
Integration
The following code snippet illustrates how you can directly incorporate the ThreadedMessages component into your layout.xml
file.
- XML
<com.cometchat.chatuikit.threadheader.CometChatThreadHeader
android:id="@+id/thread_header"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
Customization
To fit your app's design requirements, you can customize the appearance of the conversation component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
Thread Header
The CometChatThreadHeader
is used in threaded message views, displaying information about the parent message and its context. It provides a seamless way to navigate between the thread and the main conversation while maintaining context.
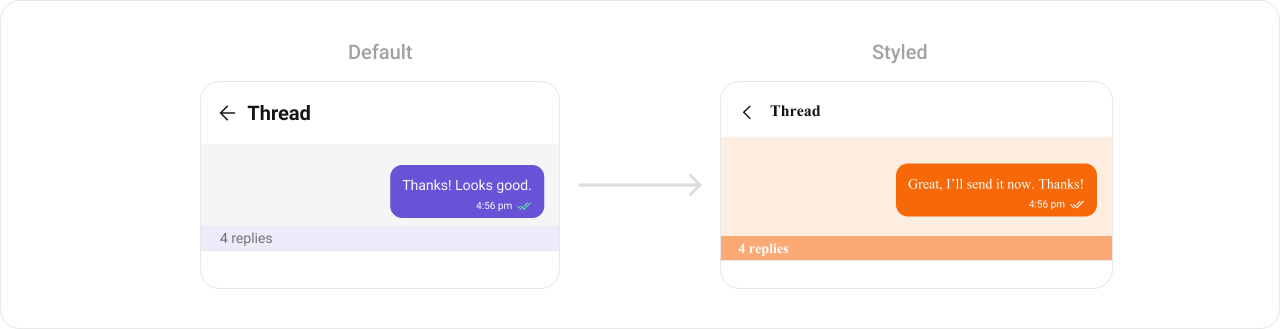
<!-- themes.xml -->
<style name="CustomOutgoingMessageBubbleStyle" parent="CometChatOutgoingMessageBubbleStyle">
<item name="cometchatMessageBubbleBackgroundColor">#F76808</item>
</style>
<style name="CustomIncomingMessageBubbleStyle" parent="CometChatIncomingMessageBubbleStyle">
<item name="cometchatMessageBubbleBackgroundColor">#F76808</item>
</style>
<style name="CustomThreadHeaderStyle" parent="CometChatThreadHeaderStyle">
<item name="cometchatThreadHeaderOutgoingMessageBubbleStyle">@style/CustomOutgoingMessageBubbleStyle</item>
<item name="cometchatThreadHeaderIncomingMessageBubbleStyle">@style/CustomIncomingMessageBubbleStyle</item>
<item name="cometchatThreadHeaderBackgroundColor">#FEEDE1</item>
<item name="cometchatThreadHeaderReplyCountTextColor">#F76808</item>
<item name="cometchatThreadHeaderReplyCountBackgroundColor">#FEEDE1</item>
</style>
- Java
- Kotlin
cometChatThreadHeader.setStyle(R.style.CustomThreadHeaderStyle);
cometChatThreadHeader.setStyle(R.style.CustomThreadHeaderStyle)
To know more such attributes, visit the attributes file.
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- Java
- Kotlin
CometChatThreadHeader cometChatThreadHeader = findViewById(R.id.threaded_header);
val threadedMessages = findViewById<CometChatMessageList>(R.id.threaded_messages)
Below is a list of customizations along with corresponding code snippets
Methods | Description | Code |
---|---|---|
setParentMessage | Used to to set the message for which the replies need to be fetched | .setParentMessage(BaseMessage) |
setIncomingMessageBubbleStyle | Used to set Incoming Bubble style | .setIncomingMessageBubbleStyle(@StyleRes int) |
setOutgoingMessageBubbleStyle | Used to set Outgoing Bubble style | .setOutgoingMessageBubbleStyle(@StyleRes int) |
setReceiptsVisibility | Used to hide Receipt from Message Bubble | setReceiptsVisibility(View.GONE) |
setReplyCountVisibility | Used to hide reply count from the component | setReplyCountVisibility(View.GONE) |
setReplyCountBarVisibility | Used to hide reply count bar from component | setReplyCountBarVisibility(View.GONE) |
setAlignment | Used to set the bubble alignment: either leftAligned or standard. If set to standard, the bubble will appear on the left or right based on the message type (incoming or outgoing). If set to leftAligned, all message bubbles will appear on the left regardless of their type. | setAlignment(UIKitConstants.MessageListAlignment alignment) |
setAvatarVisibility | Shows or hides the avatar in the Thread Header. | setAvatarVisibility(true) |
setDateFormat | Sets the date pattern for displaying message dates in the Thread Header. | setDateFormat(new SimpleDateFormat("MMM dd, yyyy",Locale.getDefault())) |
setTimeFormat | Sets the date pattern for displaying message dates in the Thread Header. | setDateFormat(new SimpleDateFormat("hh:mm a",Locale.getDefault())) |
addTemplate | Adds a new message template to the existing list of templates to the Thread Header. | addTemplate(CometChatMessageTemplate template) |
setTemplates | Replace the default list of message template and sets a new list. | setTemplates(List<CometChatMessageTemplate> cometchatMessageTemplates) |
setTextFormatters | This method allows the addition of custom text formatters to the current list of formatters used in the Thread Header. | setTextFormatters(List<CometChatTextFormatter> cometchatTextFormatters) |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
setTemplates
The setTemplates method is used to configure and set a list of templates for message bubbles. It allows for dynamic customization of message appearance, content, or other predefined settings based on the templates provided. This method can be called to update or apply a new set of templates to the message handling system.