Group Members
Overview
CometChatGroupMembers
is a versatile Component designed to showcase all users who are either added to or invited to a group, thereby enabling them to participate in group discussions, access shared content, and engage in collaborative activities. Group members have the capability to communicate in real-time through messaging, voice and video calls, and various other interactions. Additionally, they can interact with each other, share files, and join calls based on the permissions established by the group administrator or owner.
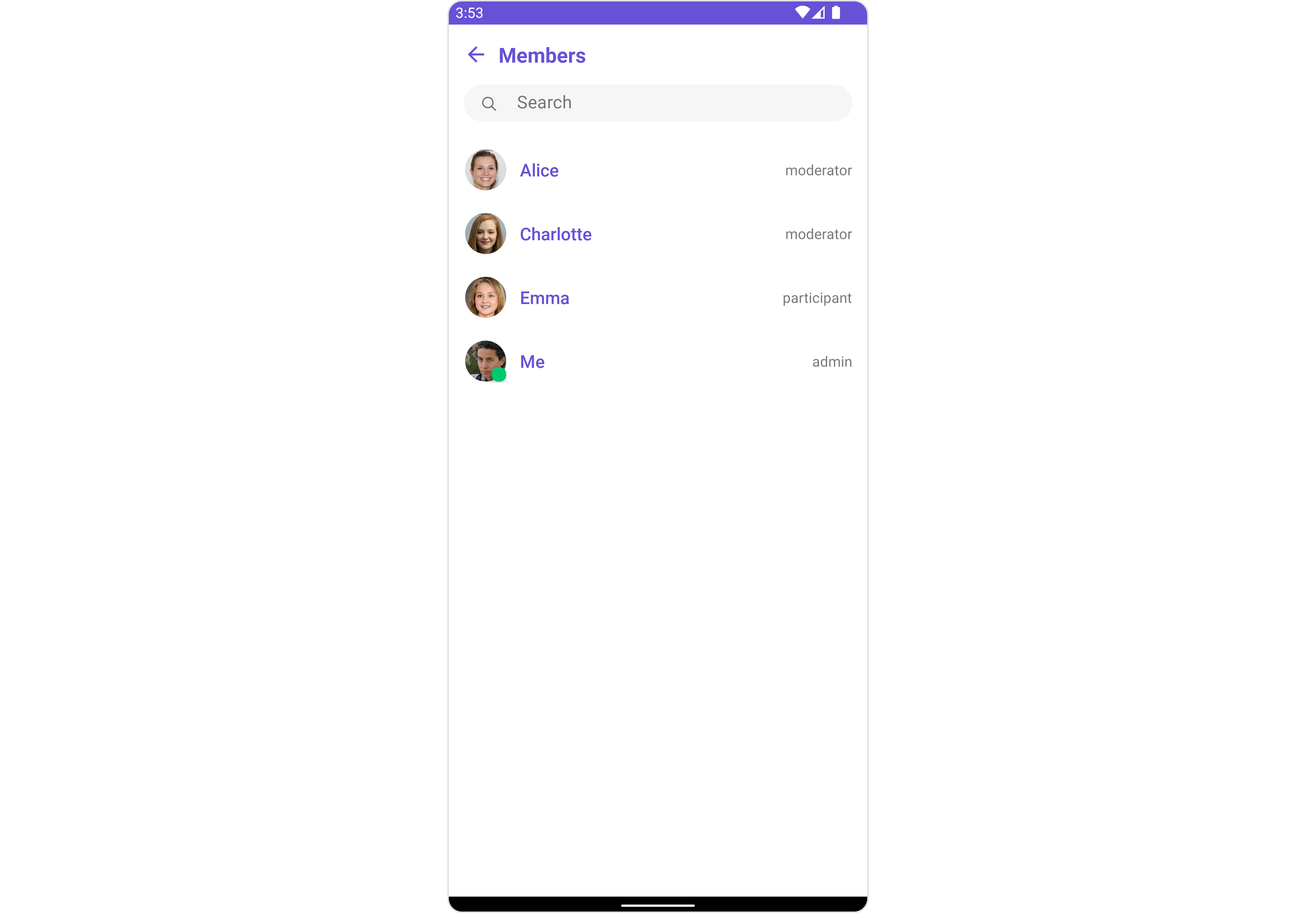
The CometChatGroupMembers
component is composed of the following BaseComponents:
Components | Description |
---|---|
CometChatListBase | CometChatListBase serves as a container component equipped with a title (navigationBar), search functionality (search-bar), background settings, and a container for embedding a list view. |
CometChatListItem | This component renders information extracted from a User object onto a tile, featuring a title, subtitle, leading view, and trailing view. experience, facilitating seamless navigation and interaction within the component. |
Usage
Integration
CometChatGroupMembers
, as a Composite Component, offers flexible integration options, allowing it to be launched directly via button clicks or any user-triggered action. Additionally, it seamlessly integrates into tab view controllers. With group members, users gain access to a wide range of parameters and methods for effortless customization of its user interface.
The following code snippet exemplifies how you can seamlessly integrate the GroupMembers component into your application.
- XML
<com.cometchat.chatuikit.groupmembers.CometChatGroupMembers
android:id="@+id/group_member"
android:layout_width="match_parent"
android:layout_height="match_parent" />
If you're defining the Group members within the XML code, you'll need to extract them and set them on the Group object using the appropriate method.
- Java
- Kotlin
CometChatGroupMembers cometchatGroupMembers = binding.groupMember;
Group group = new Group();
group.setGuid("GROUP_ID");
group.setName("GROUP_NAME");
cometchatGroupMembers.setGroup(group);
val cometchatGroupMembers: CometChatGroupMembers = binding.groupMember
val group: Group = Group()
group.setGuid("GROUP_ID")
group.setName("GROUP_NAME")
cometchatGroupMembers.setGroup(group)
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. SetOnItemClick
This method proves valuable when users seek to override onItemClick functionality within CometChatGroupMembers, empowering them with greater control and customization options.
The setItemClickListener
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- Java
- Kotlin
cometchatGroupMembers.setItemClickListener(new OnItemClickListener<GroupMember>() {
@Override
public void OnItemClick(GroupMember groupMember, int i) {
}
});
cometchatGroupMembers.setItemClickListener(object : OnItemClickListener<GroupMember?>() {
override fun OnItemClick(groupMember: GroupMember?, i: Int) {
}
})
2. SetOnItemLongClick
This method becomes invaluable when users seek to override long-click functionality within CometChatGroupMembers, offering them enhanced control and flexibility in their interactions.
The setOnLongClickListener
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- Java
- Kotlin
cometchatGroupMembers.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View view) {
return false;
}
});
cometchatGroupMembers.setOnLongClickListener(OnLongClickListener {
false
})
3. SetOnError
You can customize this behavior by using the provided code snippet to override the On Error
and improve error handling.
- Java
- Kotlin
cometchatGroupMembers.setOnError(new OnError() {
@Override
public void onError(Context context, CometChatException e) {
}
});
cometchatGroupMembers.setOnError(OnError { context, e ->
})
4. AddOnBackPressListener
You can customize this behavior by using the provided code snippet to override the addOnBackPressListener
and improve error handling.
- Java
- Kotlin
cometchatGroupMembers.addOnBackPressListener(new CometChatListBase.OnBackPress() {
@Override
public void onBack() {
}
});
cometchatGroupMembers.addOnBackPressListener(CometChatListBase.OnBackPress {
})
5. OnSelection
The OnSelection
event is triggered upon the completion of a selection in SelectionMode
. It does not have a default behavior. However, you can override its behavior using the following code snippet.
- Java
- Kotlin
cometchatGroupMembers.setSelectionMode(UIKitConstants.SelectionMode.MULTIPLE);
cometchatGroupMembers.setOnSelection(new CometChatGroupMembers.OnSelection() {
@Override
public void onSelection(List<GroupMember> list) {
// Handle selected group members here
}
});
cometchatGroupMembers.setSelectionMode(UIKitConstants.SelectionMode.MULTIPLE)
cometchatGroupMembers.setOnSelection { list ->
// Handle selected group members here
}
Filters
Filters allow you to customize the data displayed in a list within a Component
. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders
of Chat SDK.
1. GroupsRequestBuilder
The GroupsRequestBuilder enables you to filter and customize the group list based on available parameters in GroupsRequestBuilder. This feature allows you to create more specific and targeted queries when fetching groups. The following are the parameters available in GroupsRequestBuilder
Property | Description | Code |
---|---|---|
Limit | Sets the number of group members that can be fetched in a single request, suitable for pagination. | .setLimit(int) |
Search Keyword | Used for fetching group members matching the passed string. | .setSearchKeyword(String) |
Scopes | Used for fetching group members having matching scopes which may be of participant, moderator, admin, and owner. | .setScopes(List<String>) |
Example
In the example below, we are applying a filter to the Group List based on limit and scope.
- Java
- Kotlin
GroupMembersRequest.GroupMembersRequestBuilder groupMembersRequestBuilder= new GroupMembersRequest.GroupMembersRequestBuilder(GUID).setLimit(limit);
cometchatGroupMembers.setGroupMembersRequestBuilder(groupMembersRequestBuilder);
val groupMembersRequestBuilder = GroupMembersRequestBuilder(GUID).setLimit(limit)
cometchatGroupMembers.setGroupMembersRequestBuilder(groupMembersRequestBuilder)
2. SearchRequestBuilder
The SearchRequestBuilder uses GroupsRequestBuilder enables you to filter and customize the search list based on available parameters in GroupsRequestBuilder.
This feature allows you to keep uniformity between the displayed Groups List and searched Group List.
Example
- Java
- Kotlin
GroupMembersRequest.GroupMembersRequestBuilder groupMembersRequestBuilder= new GroupMembersRequest.GroupMembersRequestBuilder(GUID).setLimit(LIMIT).setSearchKeyword(SEARCH_KEYWORD);
cometchatGroupMembers.setSearchRequestBuilder(groupMembersRequestBuilder);
val groupMembersRequestBuilder = GroupMembersRequestBuilder(GUID).setLimit(LIMIT).setSearchKeyword(SEARCH_KEYWORD)
cometchatGroupMembers.setSearchRequestBuilder(groupMembersRequestBuilder)
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
Events emitted by the Join Group component is as follows.
Event | Description |
---|---|
ccGroupMemberBanned | Triggers when the group member banned from the group successfully |
ccGroupMemberKicked | Triggers when the group member kicked from the group successfully |
ccGroupMemberScopeChanged | Triggers when the group member scope is changed in the group |
- Java
- Kotlin
CometChatGroupEvents.addGroupListener("LISTENER_ID", new CometChatGroupEvents() {
@Override
public void ccGroupMemberKicked(Action actionMessage, User kickedUser, User kickedBy, Group kickedFrom) {
super.ccGroupMemberKicked(actionMessage, kickedUser, kickedBy, kickedFrom);
}
@Override
public void ccGroupMemberBanned(Action actionMessage, User bannedUser, User bannedBy, Group bannedFrom) {
super.ccGroupMemberBanned(actionMessage, bannedUser, bannedBy, bannedFrom);
}
@Override
public void ccGroupMemberScopeChanged(Action actionMessage, User updatedUser, String scopeChangedTo, String scopeChangedFrom, Group group) {
super.ccGroupMemberScopeChanged(actionMessage, updatedUser, scopeChangedTo, scopeChangedFrom, group);
}
});
CometChatGroupEvents.addGroupListener("LISTENER_ID", object : CometChatGroupEvents() {
override fun ccGroupMemberKicked(
actionMessage: Action,
kickedUser: User,
kickedBy: User,
kickedFrom: Group
) {
super.ccGroupMemberKicked(actionMessage, kickedUser, kickedBy, kickedFrom)
}
override fun ccGroupMemberBanned(
actionMessage: Action,
bannedUser: User,
bannedBy: User,
bannedFrom: Group
) {
super.ccGroupMemberBanned(actionMessage, bannedUser, bannedBy, bannedFrom)
}
override fun ccGroupMemberScopeChanged(
actionMessage: Action,
updatedUser: User,
scopeChangedTo: String,
scopeChangedFrom: String,
group: Group
) {
super.ccGroupMemberScopeChanged(
actionMessage,
updatedUser,
scopeChangedTo,
scopeChangedFrom,
group
)
}
})
Remove the added listener
- Java
- Kotlin
CometChatGroupEvents.removeListener("LISTENER_ID");
CometChatGroupEvents.removeListener("LISTENER_ID")
Customization
To fit your app's design requirements, you can customize the appearance of the Groups component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. GroupMembers Style
You can set the GroupMembersStyle
to the Group Memebers
Component to customize the styling.
- Java
- Kotlin
GroupMembersStyle groupMembersStyle = new GroupMembersStyle();
groupMembersStyle.setBackground(getResources().getColor(R.color.white_300));
groupMembersStyle.setTitleColor(getResources().getColor(R.color.red));
cometchatGroupMembers.setStyle(groupMembersStyle);
val groupMembersStyle = GroupMembersStyle()
groupMembersStyle.setBackground(getResources().getColor(android.R.color.holo_red_dark))
groupMembersStyle.setTitleColor(getResources().getColor(R.color.red))
cometchatGroupMembers.setStyle(groupMembersStyle)
List of properties exposed by GroupMemberStyle
Property | Description | Code |
---|---|---|
Background | Used to set the background color | .setBackground(@ColorInt int) |
Border Width | Used to set border | .setBorderWidth(int) |
Border Color | Used to set border color | .setBorderColor(@ColorInt int) |
Corner Radius | Used to set border radius | .setCornerRadius(float) |
Background | Used to set background Drawable | .setBackground(Drawable) |
Title Appearance | Used to customise the appearance of the title in the app bar | .setTitleAppearance(@StyleRes int) |
BackIcon Tint | Used to set the color of the back icon in the app bar | .setBackIconTint(@ColorInt int) |
Search Background | Used to set the background color of the search box | .setSearchBackground(@ColorInt int) |
Search Border Radius | Used to set the border radius of the search box | .setSearchBorderRadius(int) |
Search Icon Tint | Used to set the color of the search icon in the search box | .setSearchIconTint(@ColorInt int) |
Search Border Width | Used to set the border width of the search box | .setSearchBorderWidth(int) |
Search Text Appearance | Used to set the style of the text in the search box | .setSearchTextAppearance(@StyleRes int) |
Loading Icon Tint | Used to set the color of the icon shown while the list of group members is being fetched | .setLoadingIconTint(@ColorInt int) |
Empty Text Appearance | Used to set the style of the response text shown when fetching the list of group members has returned an empty list | .setEmptyTextAppearance(@StyleRes int) |
Error Text Appearance | Used to set the style of the response text shown in case some error occurs while fetching the list of group members | .setErrorTextAppearance(@StyleRes int) |
Online Status Color | Used to set the color of the status indicator shown if a group member is online | .setOnlineStatusColor(@ColorInt int) |
Separator Color | Used to set the color of the divider separating the group member items | .setSeparatorColor(@ColorInt int) |
2. Avatar Style
To apply customized styles to the Avatar
component in the Group Member Component, you can use the following code snippet. For further insights on Avatar
Styles refer
- Java
- Kotlin
AvatarStyle avatarStyle = new AvatarStyle();
avatarStyle.setBorderWidth(10);
avatarStyle.setBorderColor(Color.BLACK);
cometchatGroupMembers.setAvatarStyle(avatarStyle);
val avatarStyle = AvatarStyle()
avatarStyle.borderWidth = 10
avatarStyle.borderColor = Color.BLACK
cometchatGroupMembers.setAvatarStyle(avatarStyle)
3. StatusIndicator Style
To apply customized styles to the Status Indicator component in the Group Member Component, You can use the following code snippet. For further insights on Status Indicator Styles refer
- Java
- Kotlin
StatusIndicatorStyle statusIndicatorStyle = new StatusIndicatorStyle();
statusIndicatorStyle.setCornerRadius(3.5f);
statusIndicatorStyle.setBorderColor(Color.YELLOW);
cometchatGroupMembers.setStatusIndicatorStyle(statusIndicatorStyle);
val statusIndicatorStyle = StatusIndicatorStyle()
statusIndicatorStyle.cornerRadius = 3.5f
statusIndicatorStyle.borderColor = Color.YELLOW
cometchatGroupMembers.setStatusIndicatorStyle(statusIndicatorStyle)
4. ListItem Style
To apply customized styles to the List Item
component in the Group Member
Component, you can use the following code snippet. For further insights on List Item
Styles refer
- Java
- Kotlin
ListItemStyle listItemStyle = new ListItemStyle();
listItemStyle.setBackground(R.color.purple_200);
listItemStyle.setBorderWidth(2);
listItemStyle.setBorderColor(R.color.purple_700);
listItemStyle.setCornerRadius(20);
cometchatGroupMembers.setListItemStyle(listItemStyle);
val listItemStyle = ListItemStyle()
listItemStyle.setBackground(R.color.purple_200)
listItemStyle.setBorderWidth(2)
listItemStyle.setBorderColor(R.color.purple_700)
listItemStyle.setCornerRadius(20f)
cometchatGroupMembers.setListItemStyle(listItemStyle)
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- Java
- Kotlin
cometchatGroupMembers.setTitle("Your Title");
cometchatGroupMembers.disableUsersPresence(true);
cometchatGroupMembers.hideSeparator(true);
cometchatGroupMembers.setTitle("Your Title")
cometchatGroupMembers.disableUsersPresence(true)
cometchatGroupMembers.hideSeparator(true)
Property | Description | Code |
---|---|---|
Group | The group for which the group members will be listed. A required parameter. | .setGroup(Group) |
Title | Used to set title in the app bar | .setTitle(String) |
Style | Used to set styling properties | .setStyle(GroupMembersStyle) |
Avatar Style | Used to customise the Avatar of the group member | .setAvatarStyle(AvatarStyle) |
Status Indicator Style | Used to customise the status indicator shown if a group member is online | .setStatusIndicatorStyle(StatusIndicatorStyle) |
Search Placeholder Text | Used to set search placeholder text | .setSearchPlaceholderText(String) |
Back Icon | Used to set back button widget | .backIcon(@DrawableRes int res) |
Show Back Button | Used to toggle visibility for back button | .showBackButton(boolean) |
Search Box Icon | Used to set search Icon in the search field | .setSearchBoxIcon(@DrawableRes int res) |
Hide Search | Used to toggle visibility for search box | .hideSearch(boolean) |
Hide Error | Used to hide error on fetching group members | .hideError(boolean) |
Hide Separator | Used to hide the divider separating the group member items | .hideSeparator(boolean) |
Disable Users Presence | Used to control visibility of group member indicator shown if group member is online | .disableUsersPresence(boolean) |
List Item Style | Used to set style to Tile which displays data obtained from a GroupMember object | .setListItemStyle(ListItemStyle) |
Selection Icon | Used to override the of the default item selection icon | .setSelectionIcon(@DrawableRes int selectionIcon) |
Submit Icon | Used to override the default selection complete icon | .setSubmitIcon(@DrawableRes int submitIcon) |
Selection Mode | Used to set the number of group members that can be selected if activateSelection is not null. SelectionMode can be single, multiple or none. | .setSelectionMode(SelectionMode) |
Error State Text | Used to set a custom text response when some error occurs on fetching the list of group members | .errorStateText(String) |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your own views, layouts, and UI elements and then incorporate those into the component.
The Group Memebers
component does not provide additional functionalities beyond this level of customization.
SetOptions
You can set a List of CometChatOption
for a Members to add your custom actions to the Members. These options will be visible when swiping any Members item.
- Java
- Kotlin
cometchatGroupMembers.setOptions((context, groupMember, group) -> {
// Handle banned member options here (return null for default behavior)
return null;
});
cometchatGroupMembers.setOptions { context, groupMember, group ->
// Handle banned member options here (return null for default behavior)
return null
}
Example
- Java
- Kotlin
cometchatGroupMembers.setOptions((context, groupMember, group) -> {
List<CometChatOption> optionList = new ArrayList<>();
CometChatOption option = new CometChatOption();
option.setId("option_send_photo");
option.setIcon(R.drawable.ic_camera);
option.setBackgroundColor(Color.CYAN);
option.setOnClick(() -> {
// Your Action onClick of option
});
optionList.add(option);
CometChatOption option2 = new CometChatOption();
option2.setId("option_audio_call");
option2.setIcon(R.drawable.ic_call);
option2.setBackgroundColor(Color.BLUE);
option2.setOnClick(() -> {
// Your Action onClick of option
});
optionList.add(option2);
CometChatOption option3 = new CometChatOption();
option3.setId("option_video_call");
option3.setIcon(R.drawable.ic_video);
option3.setBackgroundColor(Color.RED);
option3.setOnClick(() -> {
// Your Action onClick of option
});
optionList.add(option3);
return optionList;
});
cometchatGroupMembers.setOptions { context, groupMember, group ->
val optionList = ArrayList<CometChatOption>()
val option1 = CometChatOption().apply {
id = "option_send_photo"
icon = context.resources.getDrawable(R.drawable.ic_camera) // Access drawable using resources
backgroundColor = Color.CYAN
setOnClick {
// Your action on click of option1
}
}
optionList.add(option1)
val option2 = CometChatOption().apply {
id = "option_audio_call"
icon = context.resources.getDrawable(R.drawable.ic_call)
backgroundColor = Color.BLUE
setOnClick {
// Your action on click of option2
}
}
optionList.add(option2)
val option3 = CometChatOption().apply {
id = "option_video_call"
icon = context.resources.getDrawable(R.drawable.ic_video)
backgroundColor = Color.RED
setOnClick {
// Your action on click of option3
}
}
optionList.add(option3)
return optionList
}
SetListItemView
With this function, you can assign a custom ListItem to the Conversations Component.
- Java
- Kotlin
cometchatGroupMembers.setListItemView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometChatListItem cometChatListItem) {
return null;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
}
});
cometchatGroupMembers.setListItemView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometChatListItem): View? {
return null
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
}
})
Example
You can indeed create a custom layout file named item_list.xml
for more complex or unique list items.
Once this layout file is made, you would inflate it inside the createView()
method of the GroupsViewHolderListener
. The inflation process prepares the layout for use in your application:
Following this, you would use the bindView()
method to initialize and assign values to your individual views. This could include setting text on TextViews, images on ImageViews, and so on based on the properties of the Group object:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent">
<androidx.cardview.widget.CardView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_margin="5dp"
android:elevation="10dp"
app:cardBackgroundColor="@color/purple_500"
app:cardCornerRadius="10dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<com.cometchat.chatuikit.shared.views.CometChatAvatar.CometChatAvatar
android:id="@+id/item_avatar"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_centerVertical="true"
android:layout_margin="10dp"
android:padding="10dp" />
<TextView
android:id="@+id/txt_item_name"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_centerVertical="true"
android:layout_toRightOf="@+id/item_avatar"
android:text="name"
android:textSize="17sp" />
<TextView
android:id="@+id/txt_item_date"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true"
android:layout_centerVertical="true"
android:layout_margin="10dp"
android:text="date"
android:textSize="12sp" />
</RelativeLayout>
</androidx.cardview.widget.CardView>
</RelativeLayout>
- Java
- Kotlin
cometchatGroupMembers.setListItemView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometChatListItem cometChatListItem) {
View view = getLayoutInflater().inflate(R.layout.item_list, null);
return view;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
CometChatAvatar avatarView = view.findViewById(R.id.item_avatar);
avatarView.setRadius(100);
TextView nameView = view.findViewById(R.id.txt_item_name);
nameView.setText(groupMember.getName());
avatarView.setImage(groupMember.getAvatar(), groupMember.getName());
}
});
cometchatGroupMembers.setListItemView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometChatListItem): View {
val view: View = getLayoutInflater().inflate(R.layout.item_list, null)
return view
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
val avatarView = view.findViewById<CometChatAvatar>(R.id.item_avatar)
avatarView.radius = 100f
val nameView = view.findViewById<TextView>(R.id.txt_item_name)
nameView.text = groupMember.name
avatarView.setImage(groupMember.avatar, groupMember.name)
}
})
SetSubTitleView
You can customize the subtitle view for each conversation item to meet your requirements
- Java
- Kotlin
cometchatGroupMembers.setSubtitleView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometChatListItem cometChatListItem) {
return null;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
}
});
cometchatGroupMembers.setSubtitleView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometChatListItem): View? {
return null
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
}
})
Example
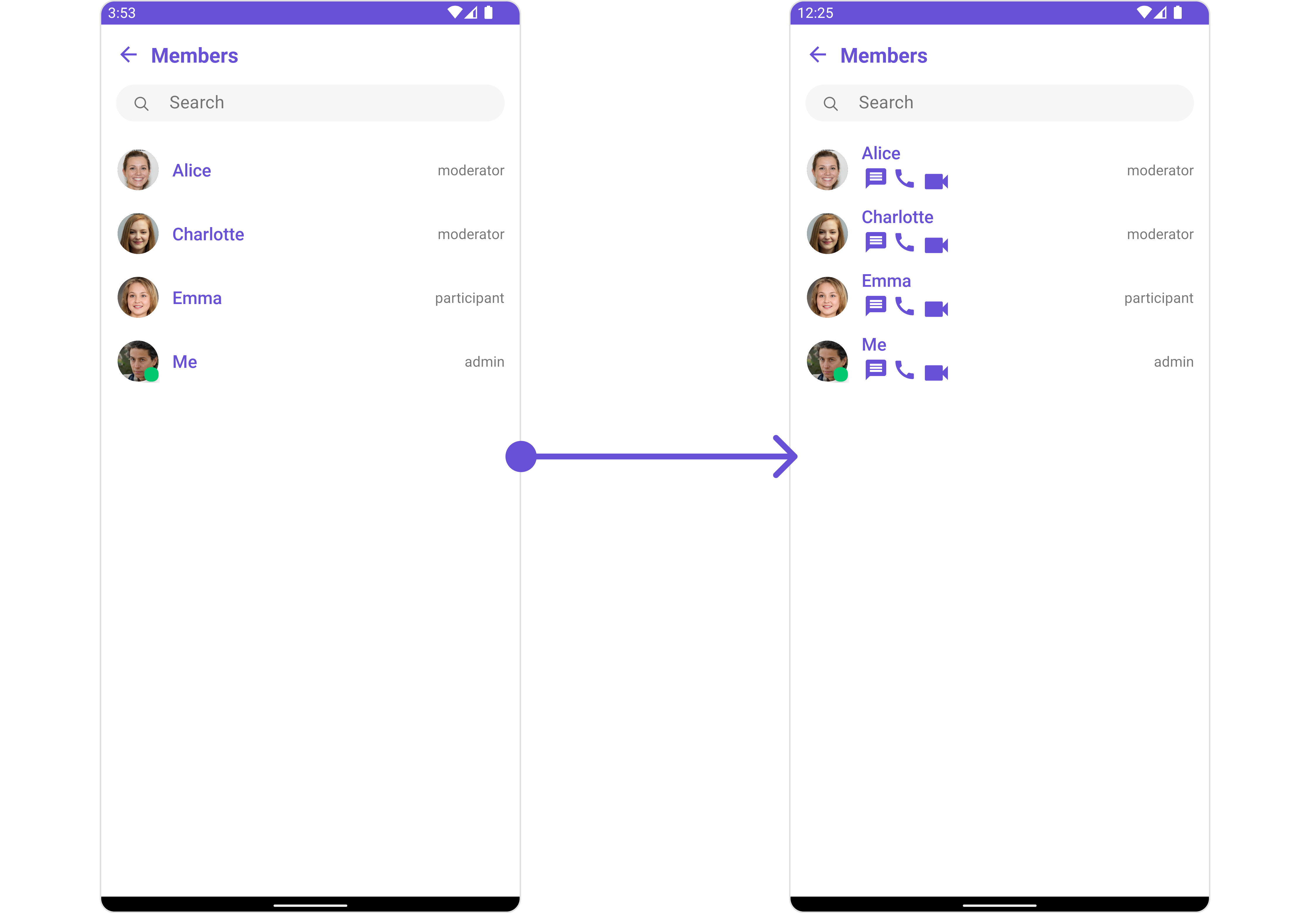
You can indeed create a custom layout file named subtitle_layout.xml
for more complex or unique list items.
Once this layout file is made, you would inflate it inside the createView()
method of the GroupsViewHolderListener
. The inflation process prepares the layout for use in your application:
Following this, you would use the bindView()
method to initialize and assign values to your individual views. This could include setting text on TextViews, images on ImageViews, and so on based on the properties of the Group object:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/txt_subtitle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Subtitle" />
<ImageView
android:id="@+id/img_conversation"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txt_subtitle"
android:layout_margin="2dp"
android:src="@drawable/ic_message_grey" />
<ImageView
android:id="@+id/img_audio_call"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txt_subtitle"
android:layout_margin="2dp"
android:layout_toEndOf="@+id/img_conversation"
android:src="@drawable/ic_call" />
<ImageView
android:id="@+id/img_video_call"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txt_subtitle"
android:layout_margin="2dp"
android:layout_toEndOf="@+id/img_audio_call"
android:src="@drawable/ic_video" />
</RelativeLayout>
- Java
- Kotlin
cometchatGroupMembers.setSubtitleView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometChatListItem cometChatListItem) {
View view = getLayoutInflater().inflate(R.layout.subtitle_layout, null);
return view;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
TextView txtSubtitle = view.findViewById(R.id.txt_subtitle);
ImageView imgConversation = view.findViewById(R.id.img_conversation);
ImageView imgAudioCall = view.findViewById(R.id.img_audio_call);;
ImageView imgVideCall = view.findViewById(R.id.img_video_call);;
txtSubtitle.setText(group.getName());
imgConversation.setOnClickListener(v -> {
Toast.makeText(context, "Conversation Clicked", Toast.LENGTH_SHORT).show();
});
imgAudioCall.setOnClickListener(v -> {
Toast.makeText(context, "Audio Call Clicked", Toast.LENGTH_SHORT).show();
});
imgVideCall.setOnClickListener(v -> {
Toast.makeText(context, "Video Call Clicked", Toast.LENGTH_SHORT).show();
});
}
});
cometchatGroupMembers.setSubtitleView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometChatListItem): View {
val view: View = layoutInflater.inflate(R.layout.subtitle_layout, null)
return view
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
val txtSubtitle = view.findViewById<TextView>(R.id.txt_subtitle)
val imgConversation = view.findViewById<ImageView>(R.id.img_conversation)
val imgAudioCall = view.findViewById<ImageView>(R.id.img_audio_call)
val imgVideCall = view.findViewById<ImageView>(R.id.img_video_call)
txtSubtitle.text = group.name
imgConversation.setOnClickListener { v: View? ->
Toast.makeText(context, "Conversation Clicked", Toast.LENGTH_SHORT).show()
}
imgAudioCall.setOnClickListener { v: View? ->
Toast.makeText(context, "Audio Call Clicked", Toast.LENGTH_SHORT).show()
}
imgVideCall.setOnClickListener { v: View? ->
Toast.makeText(context, "Video Call Clicked", Toast.LENGTH_SHORT).show()
}
}
})
SetTailView
Used to generate a custom trailing view for the GroupList item. You can add a Tail view using the following method.
- Java
- Kotlin
cometchatGroupMembers.setTailView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometChatListItem cometChatListItem) {
return null;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
}
});
cometchatGroupMembers.setTailView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometChatListItem): View? {
return null
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
}
})
Example
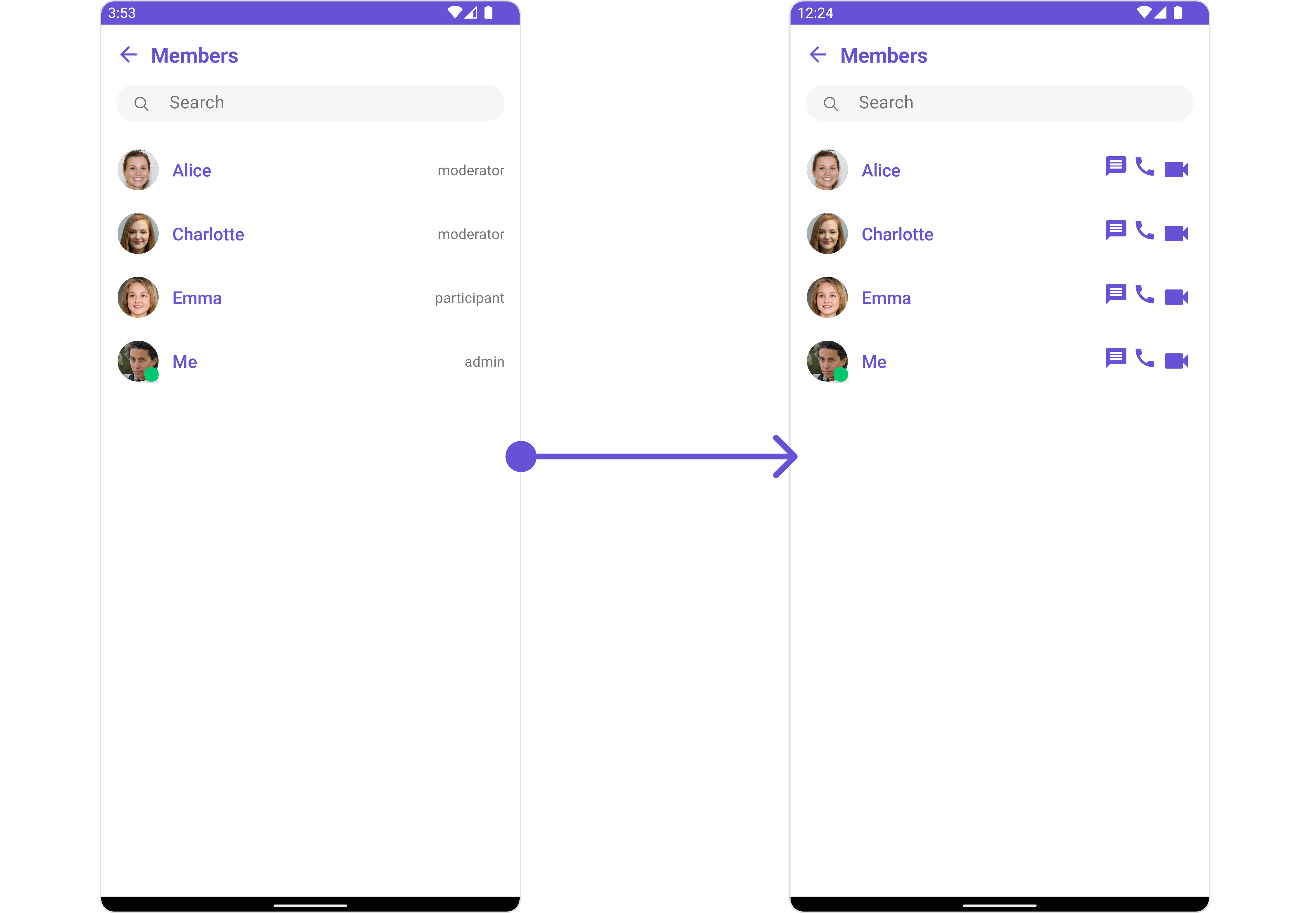
You can indeed create a custom layout file named tail_view_layout.xml
for more complex or unique list items.
Once this layout file is made, you would inflate it inside the createView()
method of the GroupsViewHolderListener
. The inflation process prepares the layout for use in your application:
Following this, you would use the bindView()
method to initialize and assign values to your individual views. This could include setting text on TextViews, images on ImageViews, and so on based on the properties of the Group object:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/txt_subtitle"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Subtitle" />
<ImageView
android:id="@+id/img_conversation"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txt_subtitle"
android:layout_margin="2dp"
android:src="@drawable/ic_message_grey" />
<ImageView
android:id="@+id/img_audio_call"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txt_subtitle"
android:layout_margin="2dp"
android:layout_toRightOf="@+id/img_conversation"
android:src="@drawable/ic_call" />
<ImageView
android:id="@+id/img_video_call"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/txt_subtitle"
android:layout_margin="2dp"
android:layout_toRightOf="@+id/img_audio_call"
android:src="@drawable/ic_video" />
</RelativeLayout>
- Java
- Kotlin
cometchatGroupMembers.setTailView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometChatListItem cometChatListItem) {
View view = getLayoutInflater().inflate(R.layout.subtitle_layout, null);
return view;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
TextView txtSubtitle = view.findViewById(R.id.txt_subtitle);
ImageView imgConversation = view.findViewById(R.id.img_conversation);
ImageView imgAudioCall = view.findViewById(R.id.img_audio_call);;
ImageView imgVideCall = view.findViewById(R.id.img_video_call);;
txtSubtitle.setText(group.getName());
imgConversation.setOnClickListener(v -> {
Toast.makeText(context, "Conversation Clicked", Toast.LENGTH_SHORT).show();
});
imgAudioCall.setOnClickListener(v -> {
Toast.makeText(context, "Audio Call Clicked", Toast.LENGTH_SHORT).show();
});
imgVideCall.setOnClickListener(v -> {
Toast.makeText(context, "Video Call Clicked", Toast.LENGTH_SHORT).show();
});
}
});
cometchatGroupMembers.setTailView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometChatListItem): View {
val view: View = layoutInflater.inflate(R.layout.subtitle_layout, null)
return view
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
val txtSubtitle = view.findViewById<TextView>(R.id.txt_subtitle)
val imgConversation = view.findViewById<ImageView>(R.id.img_conversation)
val imgAudioCall = view.findViewById<ImageView>(R.id.img_audio_call)
val imgVideCall = view.findViewById<ImageView>(R.id.img_video_call)
txtSubtitle.text = group.name
imgConversation.setOnClickListener { v: View? ->
Toast.makeText(context, "Conversation Clicked", Toast.LENGTH_SHORT).show()
}
imgAudioCall.setOnClickListener { v: View? ->
Toast.makeText(context, "Audio Call Clicked", Toast.LENGTH_SHORT).show()
}
imgVideCall.setOnClickListener { v: View? ->
Toast.makeText(context, "Video Call Clicked", Toast.LENGTH_SHORT).show()
}
}
})
SetMenu
You can set the Custom Menu view to add more options to the Groups component.
- Java
- Kotlin
cometchatGroupMembers.setMenu(View v);
cometchatGroupMembers.setMenu(v)
Example
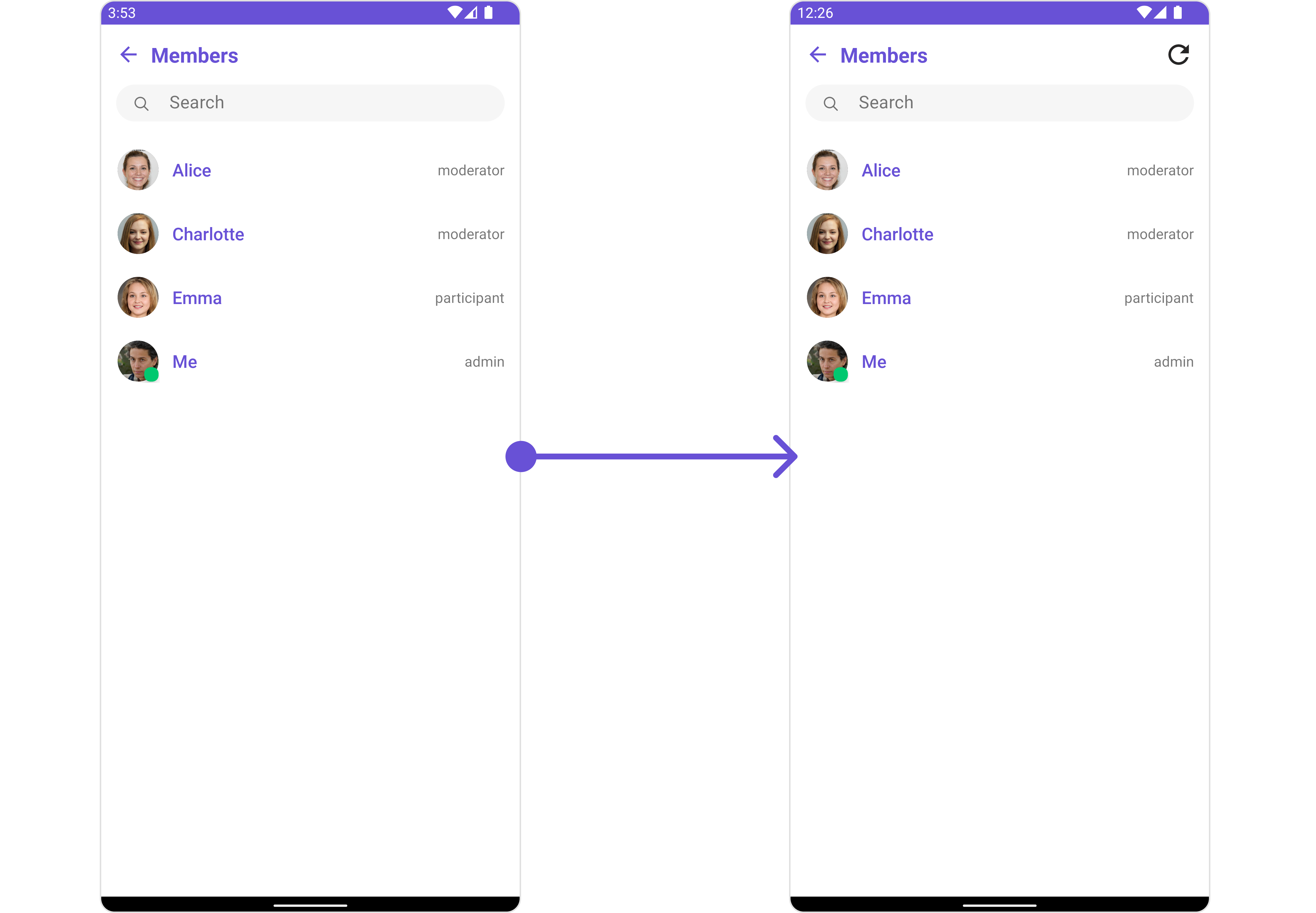
You need to create a view_menu.xml
as a custom view file. Which we will inflate and pass to .setMenu()
.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
tools:context=".MainActivity">
<ImageView
android:id="@+id/img_refresh"
android:layout_width="30dp"
android:layout_height="30dp"
android:src="@drawable/ic_refresh_black" />
</LinearLayout>
You inflate the view and pass it to setMenu
. You can get the child view reference and can handle click actions.
- Java
- Kotlin
View view = getLayoutInflater().inflate(R.layout.view_menu, null);
ImageView imgRefresh = view.findViewById(R.id.img_refresh);
imgRefresh.setOnClickListener(v -> {
Toast.makeText(requireContext(), "Clicked on Refresh", Toast.LENGTH_SHORT).show();
});
cometchatGroupMembers.setMenu(view);
val view: View = layoutInflater.inflate(R.layout.view_menu, null)
val imgRefresh = view.findViewById<ImageView>(R.id.img_refresh)
imgRefresh.setOnClickListener { v: View? ->
Toast.makeText(requireContext(), "Clicked on Refresh", Toast.LENGTH_SHORT).show()
}
cometchatGroupMembers.setMenu(view)
SetLoadingStateView
You can set a custom loader view using setLoadingStateView
to match the loading view of your app.
- Java
- Kotlin
cometchatGroupMembers.setLoadingStateView();
cometchatGroupMembers.setLoadingStateView()
Example
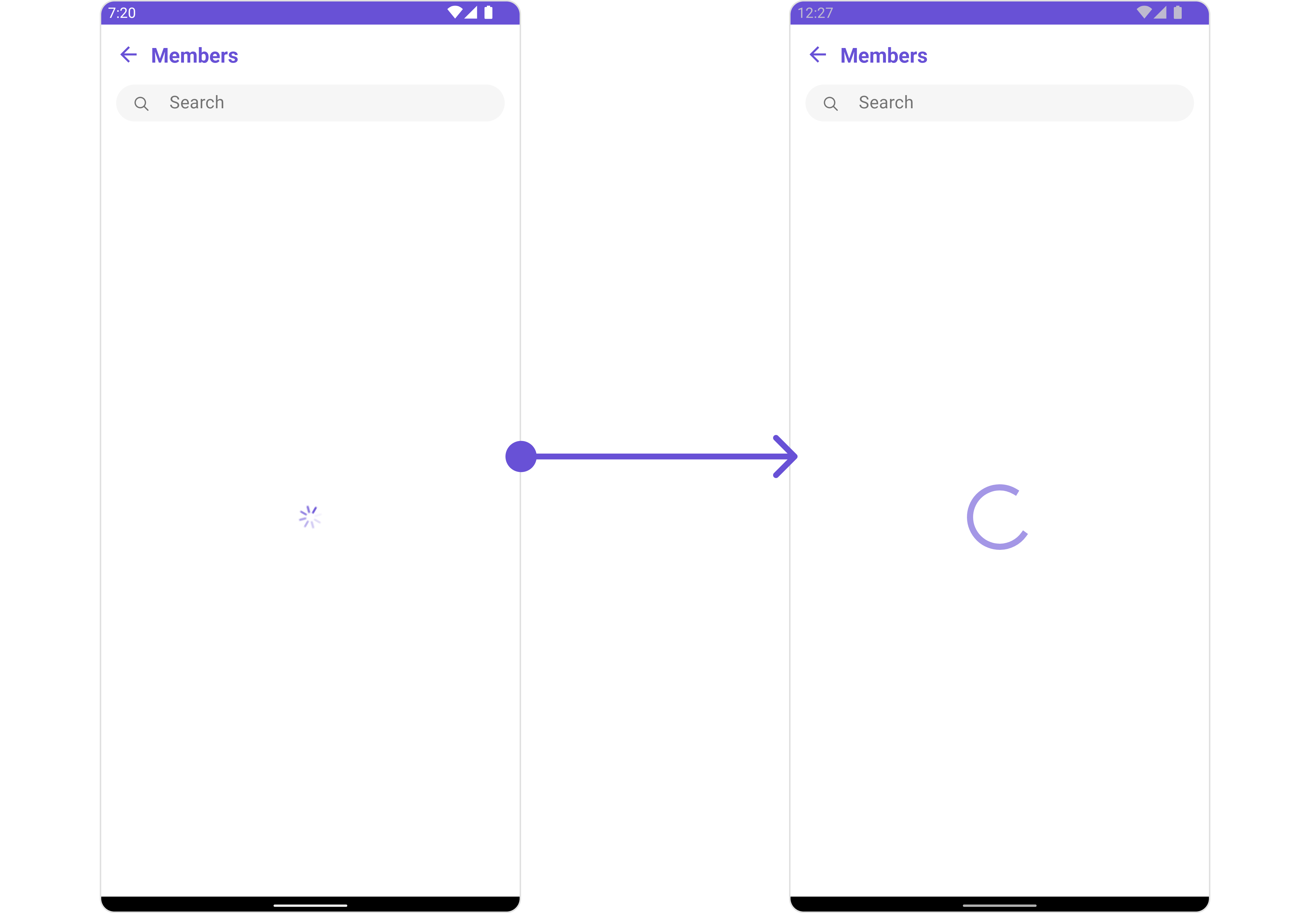
We have added a ContentLoadingProgressBar
to loading_view_layout.xml
. You can choose any view you prefer. This view should be inflated and passed to the setLoadingStateView()
method.
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center_vertical">
<androidx.core.widget.ContentLoadingProgressBar
style="?android:attr/progressBarStyleLarge"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center_horizontal" />
</FrameLayout>
- Java
- Kotlin
cometchatGroupMembers.setLoadingStateView(R.layout.loading_view_layout);
cometchatGroupMembers.setLoadingStateView(R.layout.loading_view_layout)
SetEmptyStateView
You can set a custom EmptyStateView
using setEmptyStateView
to match the empty view of your app.
- Java
- Kotlin
cometchatGroupMembers.setEmptyStateView();
cometchatGroupMembers.setEmptyStateView()
Examples
We have added an error view to empty_view_layout.xml
. You can choose any view you prefer. This view should be inflated and passed to the setEmptyStateView()
method.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center_vertical">
<TextView
android:id="@+id/txt_title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_centerInParent="true"
android:text="No Friends available"
android:textColor="@color/cometchat_grey"
android:textSize="20sp"
android:textStyle="bold" />
</RelativeLayout>
- Java
- Kotlin
cometchatGroupMembers.setEmptyStateView(R.layout.empty_view_layout);
cometchatGroupMembers.setEmptyStateView(R.layout.empty_view_layout)
SetErrorStateView
You can set a custom ErrorStateView
using setErrorStateView
to match the error view of your app.
- Java
- Kotlin
cometchatGroupMembers.setErrorStateView();
cometchatGroupMembers.setErrorStateView()
Example
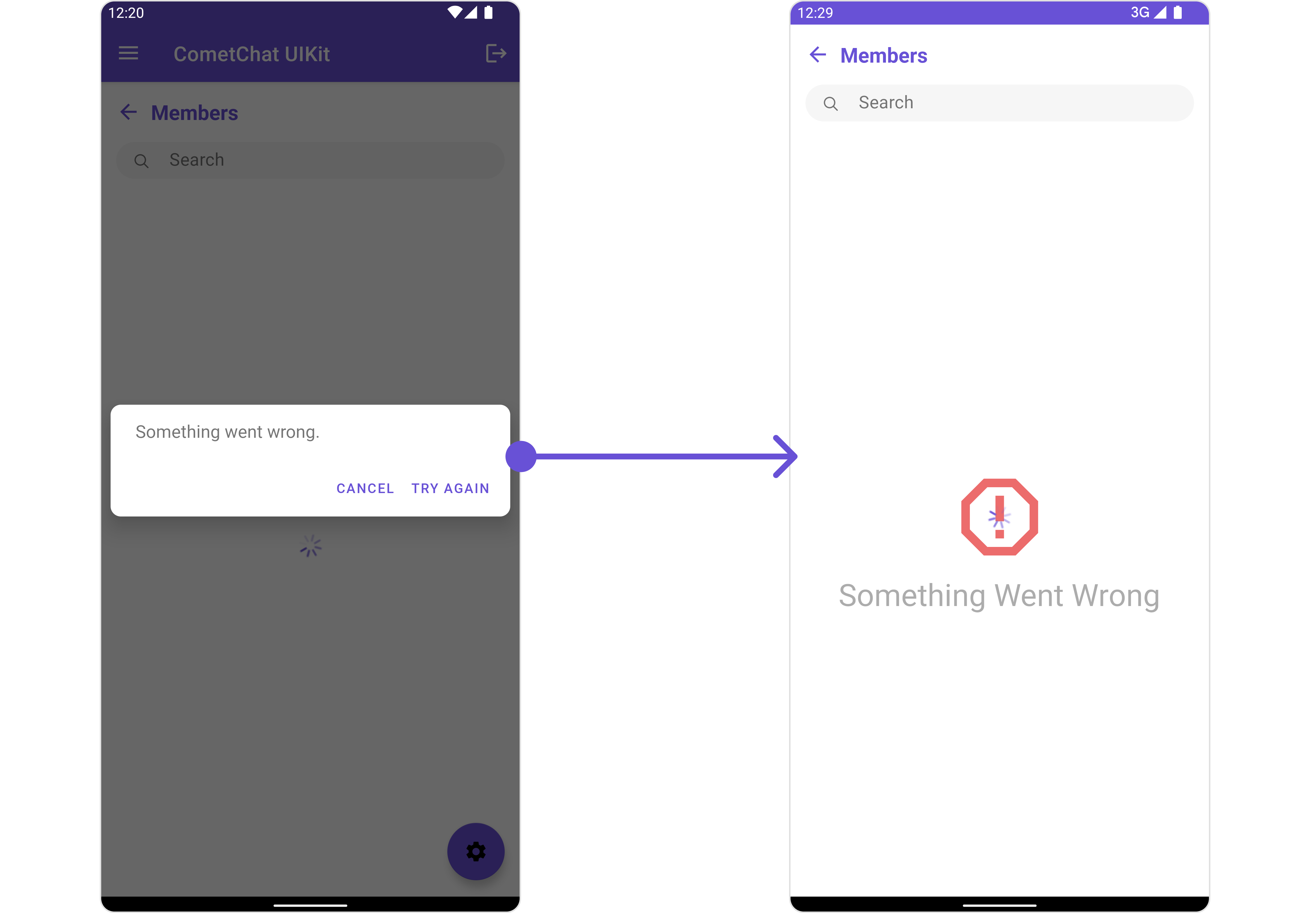
We have added an error view to error_state_view_layout.xml
. You can choose any view you prefer. This view should be inflated and passed to the setErrorStateView()
method.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/img_error"
android:layout_width="100dp"
android:layout_height="100dp"
android:layout_centerInParent="true"
android:src="@drawable/ic_error" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/img_error"
android:layout_centerHorizontal="true"
android:layout_marginTop="50dp"
android:text="Something Went Wrong"
android:textSize="30sp" />
</RelativeLayout>
- Java
- Kotlin
cometchatGroupMembers.setErrorStateView(R.layout.error_state_view_layout);
cometchatGroupMembers.setErrorStateView(R.layout.error_state_view_layout);