Message Composer
Overview
MessageComposer is a Component that enables users to write and send a variety of messages, including text, image, video, and custom messages.
Features such as Live Reaction, Attachments, and Message Editing are also supported by it.
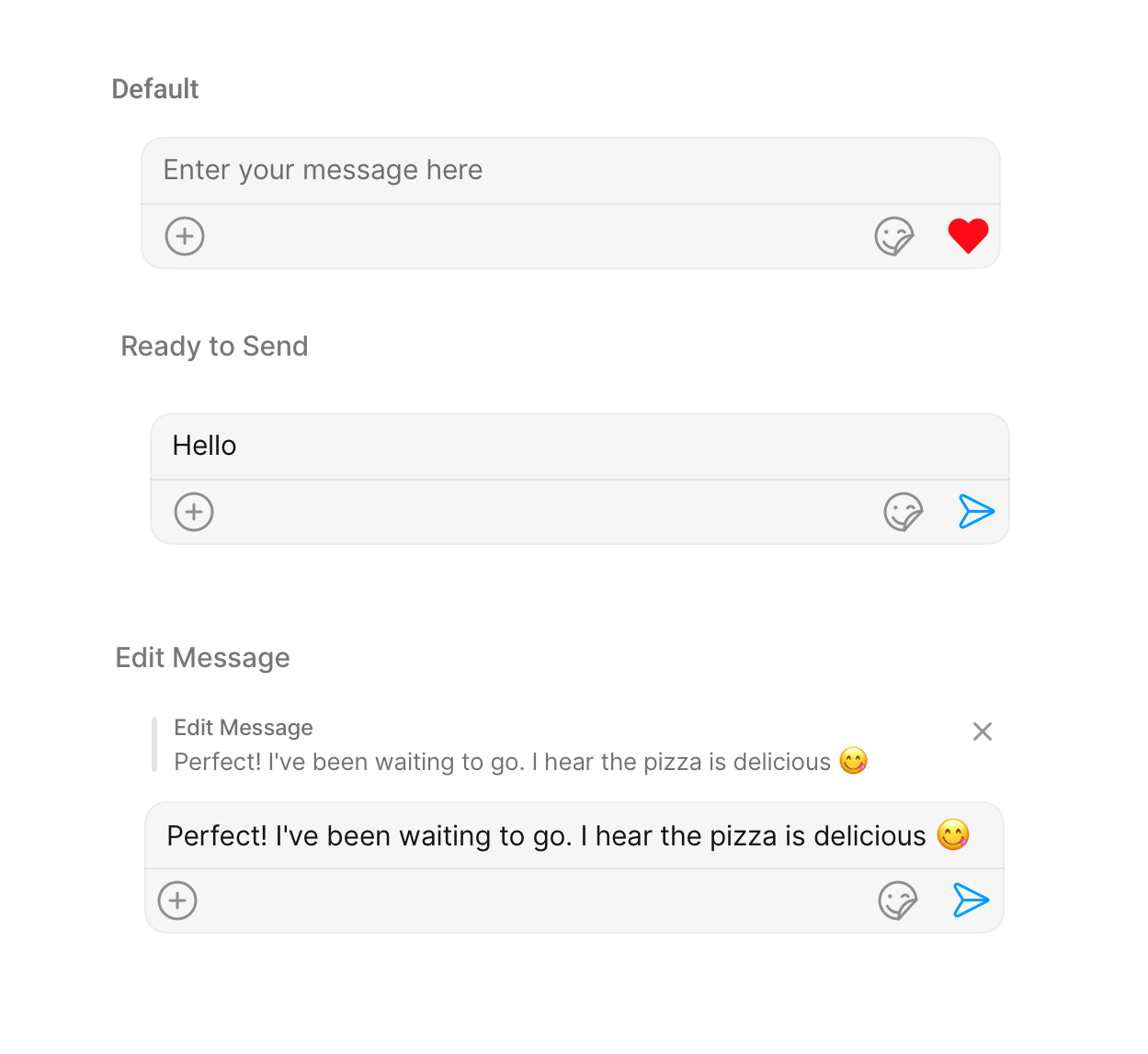
MessageComposer is comprised of the following Base Components:
Base Components | Description |
---|---|
MessageInput | This provides a basic layout for the contents of this component, such as the TextField and buttons |
ActionSheet | The ActionSheet component presents a list of options in either a list or grid mode, depending on the user's preference |
Usage
Integration
The following code snippet illustrates how you can directly incorporate the MessageComposer component into your layout.xml
file.
<com.cometchat.chatuikit.messagecomposer.CometChatMessageComposer
android:id="@+id/composer"
android:layout_width="match_parent"
android:layout_height="wrap_content" />
The MessageComposer is responsible for managing runtime permissions. To ensure the ActivityResultLauncher is properly initialized, its object should be created in the the onCreate state of an activity. To ensure that the composer is loaded within the fragment, it is important to make sure that the fragment is loaded in the onCreate
state of the activity.
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. OnSendButtonClick
The OnSendButtonClick
event gets activated when the send message button is clicked. It has a predefined function of sending messages entered in the composer EditText
. However, you can overide this action with the following code snippet.
- Java
- Kotlin
messageComposer.setOnSendButtonClick(new CometChatMessageComposer.SendButtonClick() {
@Override
public void onClick(Context context, BaseMessage baseMessage) {
Toast.makeText(context, "OnSendButtonClicked ..", Toast.LENGTH_SHORT).show();
}
});
messageComposer.setOnSendButtonClick(object : CometChatMessageComposer.SendButtonClick {
override fun onClick(context: Context, baseMessage: BaseMessage) {
Toast.makeText(context, "OnSendButtonClicked ..", Toast.LENGTH_SHORT).show()
}
})
2. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the MessageList component.
- Java
- Kotlin
messageComposer.setOnError(new OnError() {
@Override
public void onError(Context context, CometChatException e) {
//Your Exception Handling code.
}
});
messageComposer.setOnError(object : OnError {
override fun onError(context: Context, e: CometChatException) {
// Your Exception Handling code.
}
})
Filters
MessageComposer component does not have any available filters.
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The MessageComposer Component does not emit any events of its own.
Customization
To fit your app's design requirements, you can customize the appearance of the MessageComposer component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. MessageComposer Style
To modify the styling, you can apply the MessageComposerStyle to the MessageComposer Component using the setStyle
method.
- Java
- Kotlin
MessageComposerStyle messageComposerStyle = new MessageComposerStyle();
messageComposer.setStyle(messageComposerStyle);
val messageComposerStyle = MessageComposerStyle()
messageComposer.setStyle(messageComposerStyle)
The following properties are exposed by MessageComposerStyle:
Property | Description | Code |
---|---|---|
Set BorderWidth | Used to set outermost border | .setBorderWidth(int) |
Border Color | Used to set border color | .setBorderColor(@ColorInt int) |
Corner Radius | Used to set corner radius | .setCornerRadius(int) |
Background | This method will set the background color for message list | .setBackground(@ColorInt int) |
Set InputBackgroundColor | Used to set input background color | .setInputBackgroundColor(@ColorInt int) |
Set TextAppearance | Used to set input text style | .setTextAppearance(@StyleRes int) |
Set PlaceHolderTextColor | Used to set placeholder text color | .setPlaceHolderTextColor(@StyleRes int) |
Background | This will set drawable component for list background | .setBackground(@drawable int) |
Set AttachIconTint | Used to set attachment icon tint | .setAttachIconTint(@ColorRes int) |
Set SendIconTint | Used to set send button icon tint | .setSendIconTint(@ColorRes int) |
Set SeparatorTint | Used to set separator color | .setSeparatorTint(@ColorRes int) |
Set VoiceRecordingIconTint | used to set voice recording icon color | .setVoiceRecordingIconTint(@ColorRes int) |
2. MessageInput Style
To customize the styles of the MessageInput component within the MessageComposer Component, use the .setMessageInputStyle()
method. For more details, please refer to MessageInput styles.
- Java
- Kotlin
MessageInputStyle messageInputStyle = new MessageInputStyle();
messageInputStyle.setBackground(Color.BLUE);
messageInputStyle.setCornerRadius(50);
messageComposer.setMessageInputStyle(messageInputStyle);
val messageInputStyle = MessageInputStyle()
messageInputStyle.setBackground(Color.BLUE)
messageInputStyle.setCornerRadius(50)
messageComposer.setMediaRecorderStyle(mediaRecorderStyle)
3. MediaRecorder Style
To customize the styles of the MediaRecorder component within the MessageComposer Component, use the .setMediaRecorderStyle()
method. For more details, please refer to MediaRecorder styles.
- Java
- Kotlin
MediaRecorderStyle mediaRecorderStyle = new MediaRecorderStyle();
mediaRecorderStyle.setBorderColor(Color.BLACK);
mediaRecorderStyle.setRecordedContainerColor(Color.RED);
messageComposer.setMediaRecorderStyle(mediaRecorderStyle);
val mediaRecorderStyle = MediaRecorderStyle()
mediaRecorderStyle.setBorderColor(Color.BLACK)
mediaRecorderStyle.setRecordedContainerColor(Color.RED)
messageComposer.setMediaRecorderStyle(mediaRecorderStyle)
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- Java
- Kotlin
CometChatMessageComposer messageComposer = findViewById(R.id.composer);
messageComposer.hideLiveReaction(true);
messageComposer.disableTypingEvents(true);
val messageComposer: CometChatMessageComposer = findViewById(R.id.composer)
messageComposer.hideLiveReaction(true)
messageComposer.disableTypingEvents(true)
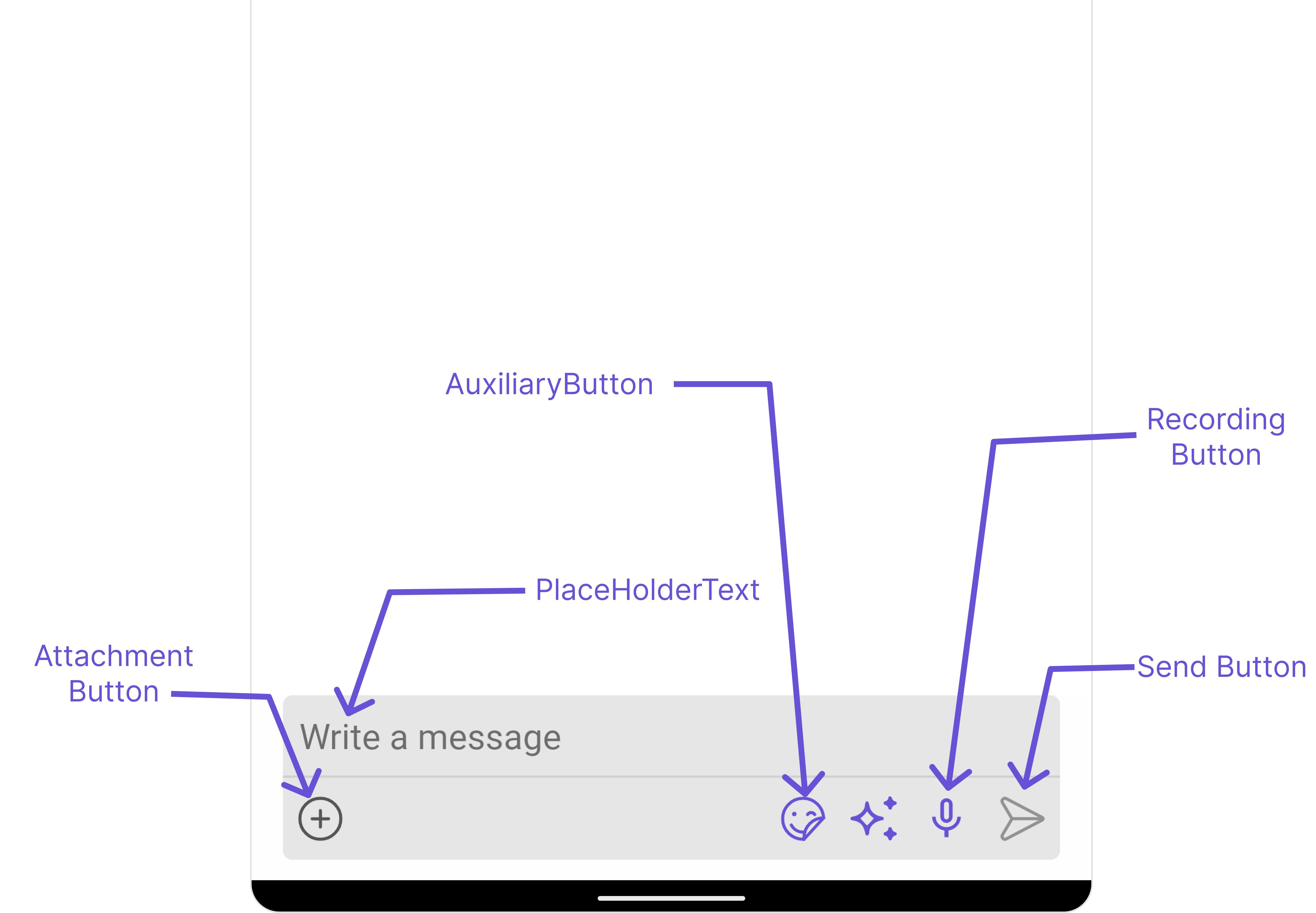
Below is a list of customizations along with corresponding code snippets
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
setTextFormatters
Assigns the list of text formatters. If the provided list is not null, it sets the list. Otherwise, it assigns the default text formatters retrieved from the data source. To configure the existing Mentions look and feel check out CometChatMentionsFormatter
Example
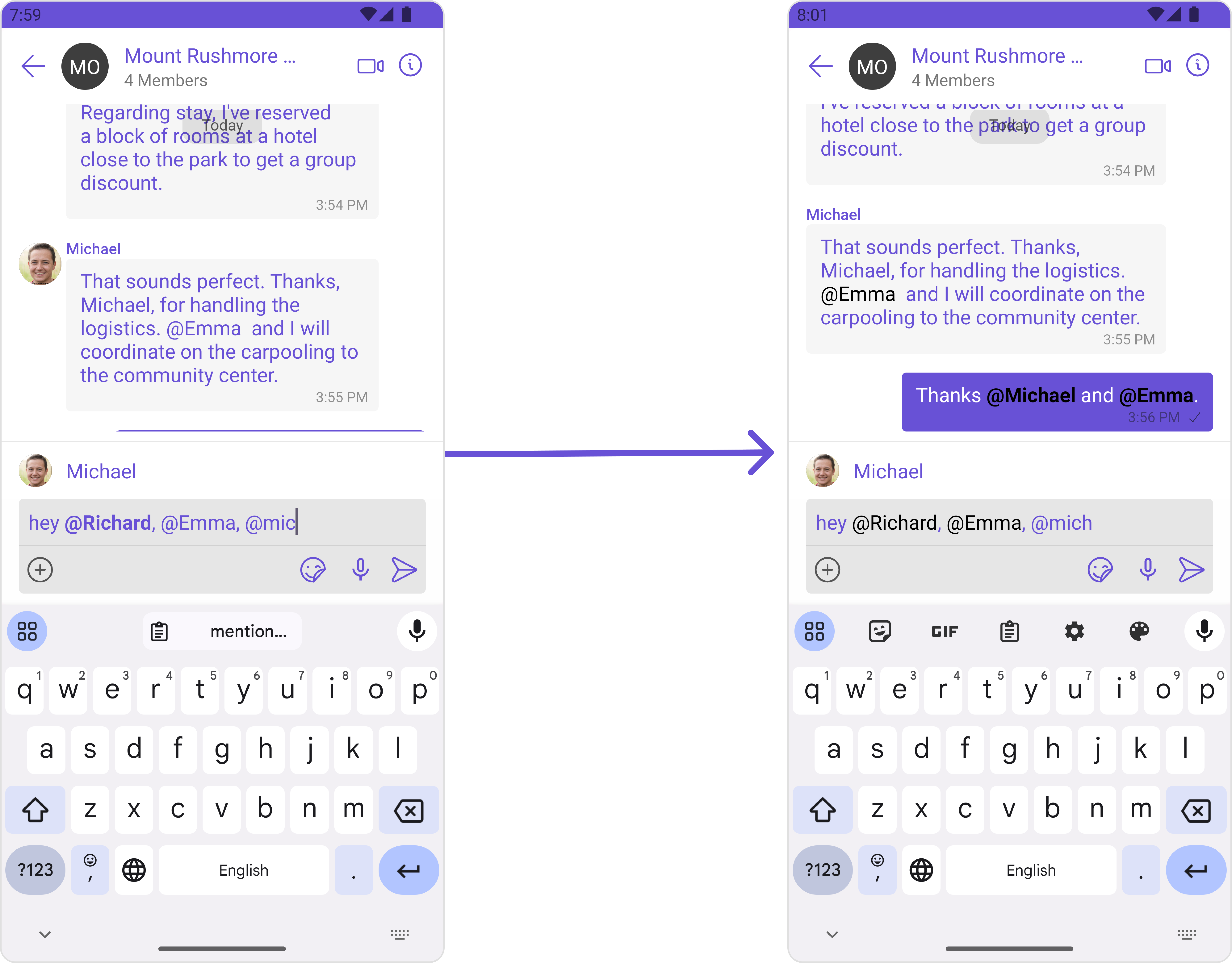
- Java
- Kotlin
// Initialize CometChatMentionsFormatter
CometChatMentionsFormatter mentionFormatter = new CometChatMentionsFormatter(context);
// set style to customize composer mention text
mentionFormatter.setComposerMentionTextStyle(new MentionTextStyle()
.setLoggedInUserTextStyle(Typeface.defaultFromStyle(Typeface.BOLD))
.setTextColor(Color.parseColor("#000000")));
// This can be passed as an array of formatter in CometChatMessageComposer by using setTextFormatters method.
List<CometChatTextFormatter> textFormatters = new ArrayList<>();
textFormatters.add(mentionFormatter);
messageComposer.setTextFormatters(textFormatters);
// Initialize CometChatMentionsFormatter
val mentionFormatter = CometChatMentionsFormatter(context)
// set style to customize composer mention text
mentionFormatter.composerMentionTextStyle = MentionTextStyle()
.setLoggedInUserTextStyle(Typeface.defaultFromStyle(Typeface.BOLD))
.setTextColor(Color.parseColor("#000000"))
// This can be passed as an array of formatter in CometChatMessageComposer by using setTextFormatters method.
val textFormatters: MutableList<CometChatTextFormatter> = ArrayList()
textFormatters.add(mentionFormatter)
messageComposer.setTextFormatters(textFormatters)
AttachmentOptions
By using setAttachmentOptions()
, you can set a list of custom MessageComposerActions
for the MessageComposer Component. This will override the existing list of MessageComposerActions
.
- Java
- Kotlin
messageComposer.setAttachmentOptions()
messageComposer.setAttachmentOptions()
Example
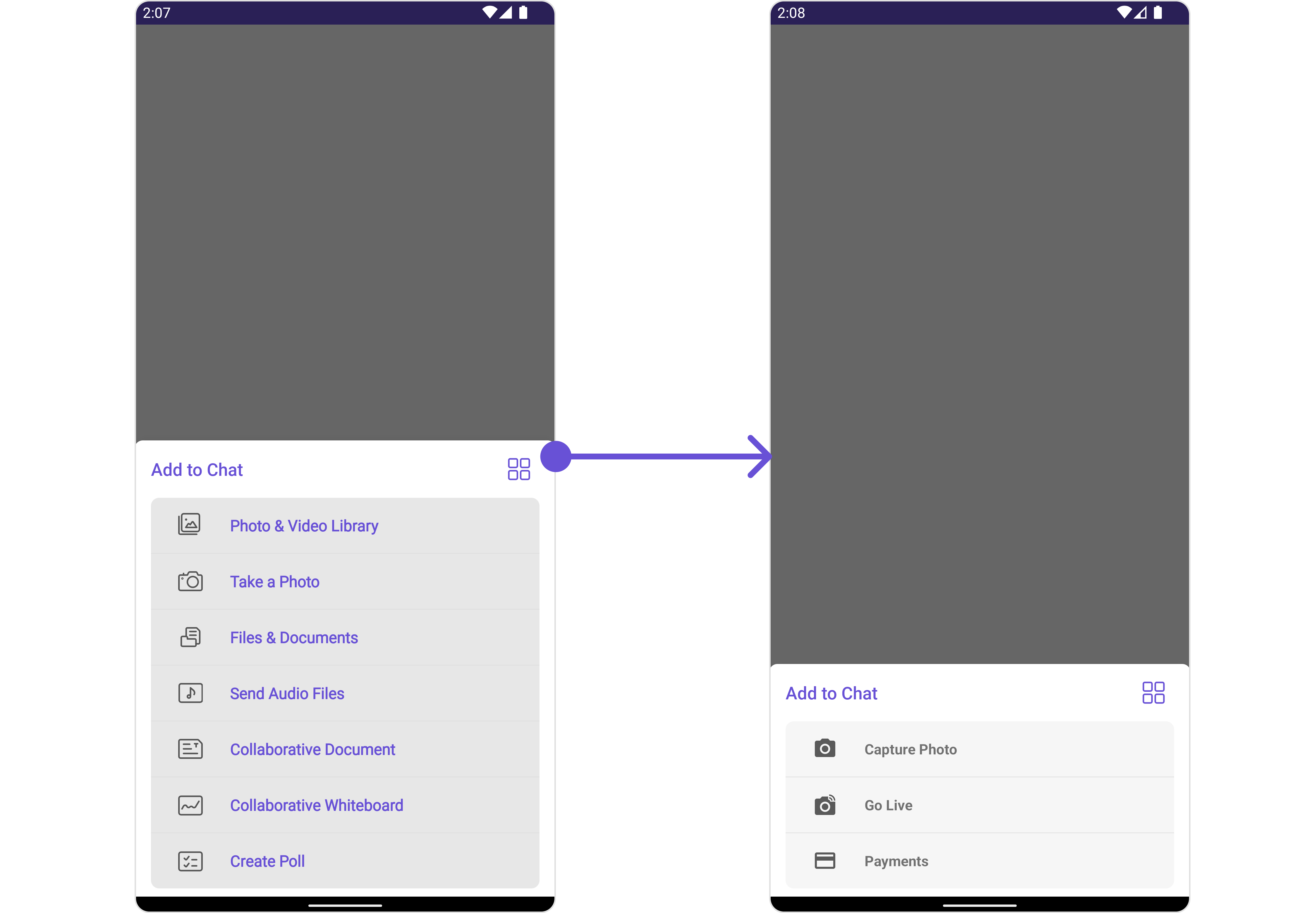
In this example, we are overriding the existing MessageComposerActions List with Capture Photo, Go Live & Payments actions.
- Java
- Kotlin
messageComposer.setAttachmentOptions(new Function4<Context, User, Group, HashMap<String, String>, List<CometChatMessageComposerAction>>() {
@Override
public List<CometChatMessageComposerAction> invoke(Context context, User user, Group group, HashMap<String, String> stringStringHashMap) {
List<CometChatMessageComposerAction> actionList = new ArrayList<>();
CometChatMessageComposerAction action1 = new CometChatMessageComposerAction();
action1.setTitle("Capture Photo");
action1.setIcon(com.cometchat.chatuikit.R.drawable.ic_camera);
action1.setOnClick(new OnClick() {
@Override
public void onClick() {
Toast.makeText(context, "Capture Photo option Clicked !!!", Toast.LENGTH_SHORT).show();
}
});
actionList.add(action1);
CometChatMessageComposerAction action2 = new CometChatMessageComposerAction();
action2.setTitle("Go Live");
action2.setIcon(R.drawable.broadcast_camera);
action2.setOnClick(new OnClick() {
@Override
public void onClick() {
Toast.makeText(context, "Live Option Clicked !!!", Toast.LENGTH_SHORT).show();
}
});
actionList.add(action2);
CometChatMessageComposerAction action3 = new CometChatMessageComposerAction();
action3.setTitle("Payments");
action3.setIcon(R.drawable.ic_payment);
action3.setOnClick(new OnClick() {
@Override
public void onClick() {
Toast.makeText(context, "Payment Option Clicked !!!", Toast.LENGTH_SHORT).show();
}
});
actionList.add(action3);
return actionList;
}
});
messageComposer.setAttachmentOptions { context, user, group, stringStringHashMap ->
val actionList = ArrayList<CometChatMessageComposerAction>()
val action1 = CometChatMessageComposerAction()
action1.title = "Capture Photo"
action1.icon = com.cometchat.chatuikit.R.drawable.ic_camera
action1.onClick = {
Toast.makeText(context, "Capture Photo option Clicked !!!", Toast.LENGTH_SHORT).show()
}
actionList.add(action1)
val action2 = CometChatMessageComposerAction()
action2.title = "Go Live"
action2.icon = R.drawable.broadcast_camera
action2.onClick = {
Toast.makeText(context, "Live Option Clicked !!!", Toast.LENGTH_SHORT).show()
}
actionList.add(action2)
val action3 = CometChatMessageComposerAction()
action3.title = "Payments"
action3.icon = R.drawable.ic_payment
action3.onClick = {
Toast.makeText(context, "Payment Option Clicked !!!", Toast.LENGTH_SHORT).show()
}
actionList.add(action3)
actionList
}
AuxiliaryButtonView
You can insert a custom view into the MessageComposer component to add additional functionality using the following method.
- Java
- Kotlin
messageComposer.setAuxiliaryButtonView()
messageComposer.setAuxiliaryButtonView()
Please note that the MessageComposer Component utilizes the AuxiliaryButton to provide sticker functionality. Overriding the AuxiliaryButton will subsequently replace the sticker functionality.
Example
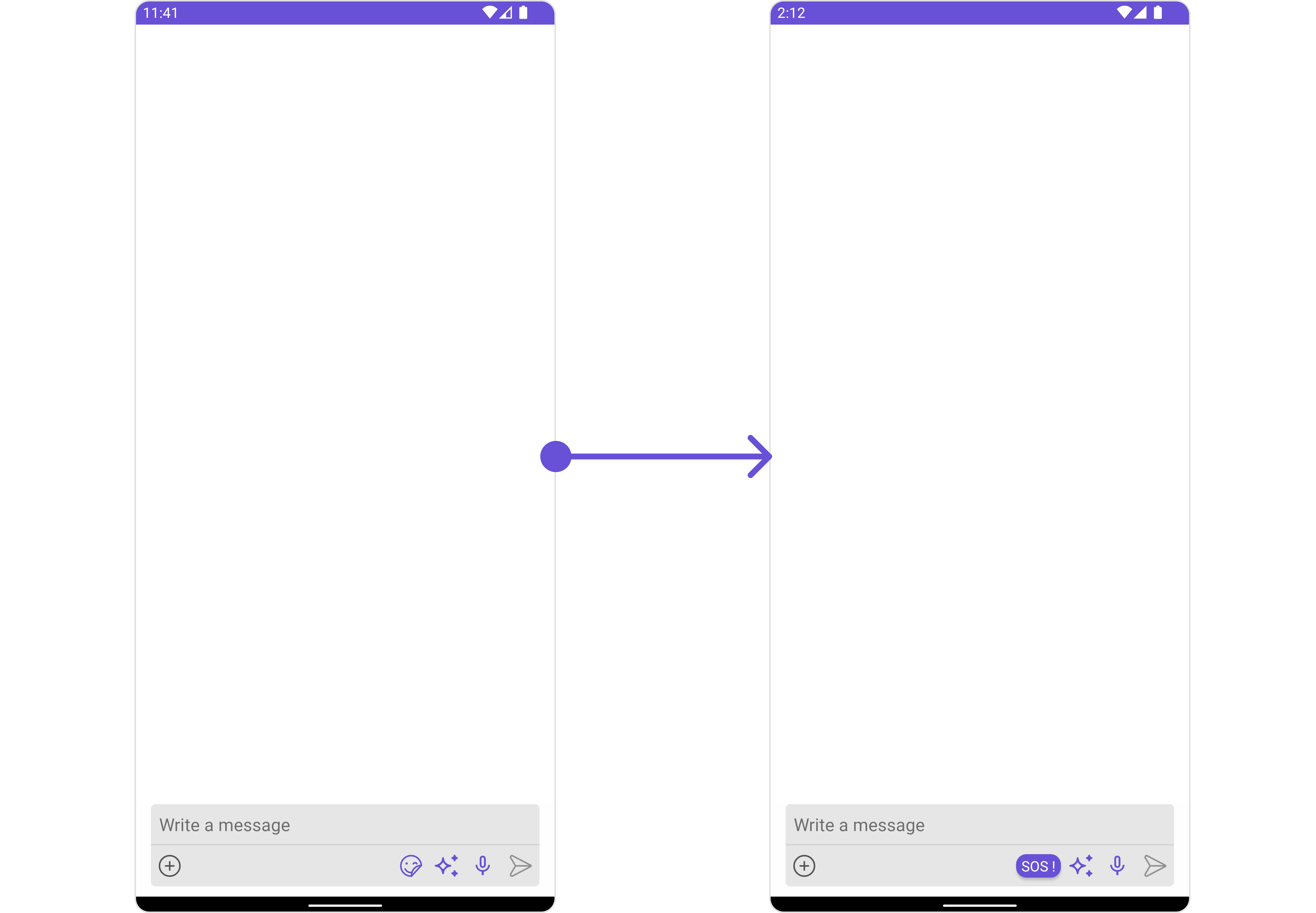
In this example, we'll be adding a custom SOS button with click functionality. You'll first need to create a layout file and then inflate it inside the .setAuxiliaryButtonView()
function.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.cardview.widget.CardView
android:id="@+id/card_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="SOS !"
android:padding="2dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
</RelativeLayout>
- Java
- Kotlin
messageComposer.setAuxiliaryButtonView((context, user, group, stringStringHashMap) -> {
View view = getLayoutInflater().inflate(R.layout.auxiliary_button_layout, null);
CardView cardView = view.findViewById(R.id.card_button);
cardView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(context, "Clicked on SOS", Toast.LENGTH_SHORT).show();
}
});
return view;
});
messageComposer.setAuxiliaryButtonView { context, user, group, stringStringHashMap ->
val view: View = LayoutInflater.from(context).inflate(R.layout.auxiliary_button_layout, null)
val cardView: CardView = view.findViewById(R.id.card_button)
cardView.setOnClickListener {
Toast.makeText(context, "Clicked on SOS", Toast.LENGTH_SHORT).show()
}
view
}
SecondaryButtonView
You can add a custom view into the SecondaryButton component for additional functionality using the below method.
- Java
- Kotlin
messageComposer.setSecondaryButtonView()
messageComposer.setSecondaryButtonView()
Please note that the MessageComposer Component uses the SecondaryButton to open the ComposerActionsList. Overriding the SecondaryButton will replace the ComposerActionsList functionality.
Example
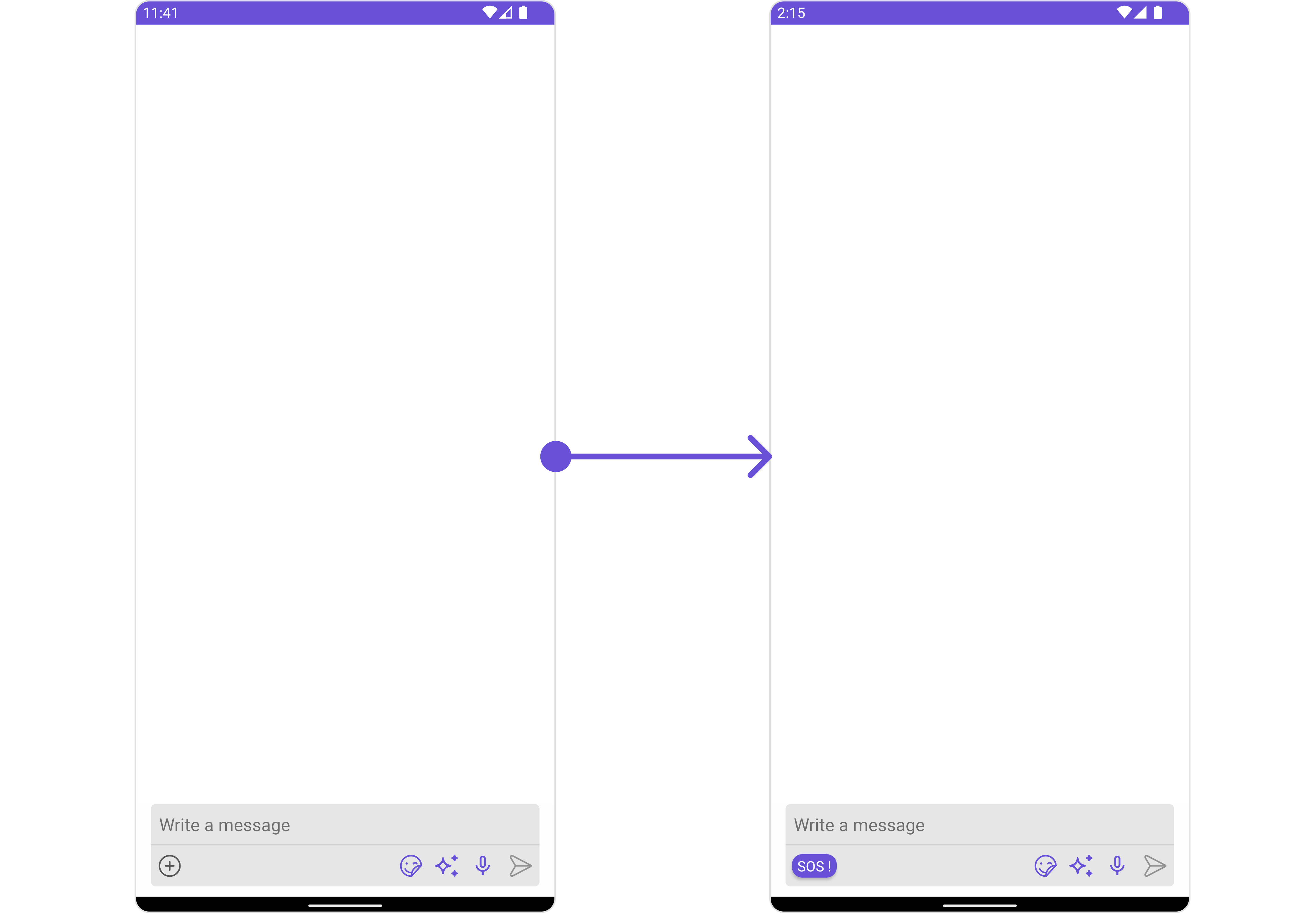
In this example, we'll be adding a custom SOS button with click functionality. You'll first need to create a layout file and then inflate it inside the .setSecondaryButtonView()
function.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.cardview.widget.CardView
android:id="@+id/card_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="SOS !"
android:padding="2dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
</RelativeLayout>
- Java
- Kotlin
messageComposer.setSecondaryButtonView((context, user, group, stringStringHashMap) -> {
View view = getLayoutInflater().inflate(R.layout.secondary_button_layout, null);
CardView cardView = view.findViewById(R.id.card_button);
cardView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Toast.makeText(context, "Clicked on SOS", Toast.LENGTH_SHORT).show();
}
});
return view;
});
messageComposer.setSecondaryButtonView { context, user, group, stringStringHashMap ->
val view: View = LayoutInflater.from(context).inflate(R.layout.secondary_button_layout, null)
val cardView: CardView = view.findViewById(R.id.card_button)
cardView.setOnClickListener {
Toast.makeText(context, "Clicked on SOS", Toast.LENGTH_SHORT).show()
}
view
}
Set SendButtonView
You can set a custom view in place of the already existing send button view. Using the following method.
- Java
- Kotlin
messageComposer.setSendButtonView();
messageComposer.setSendButtonView();
Example
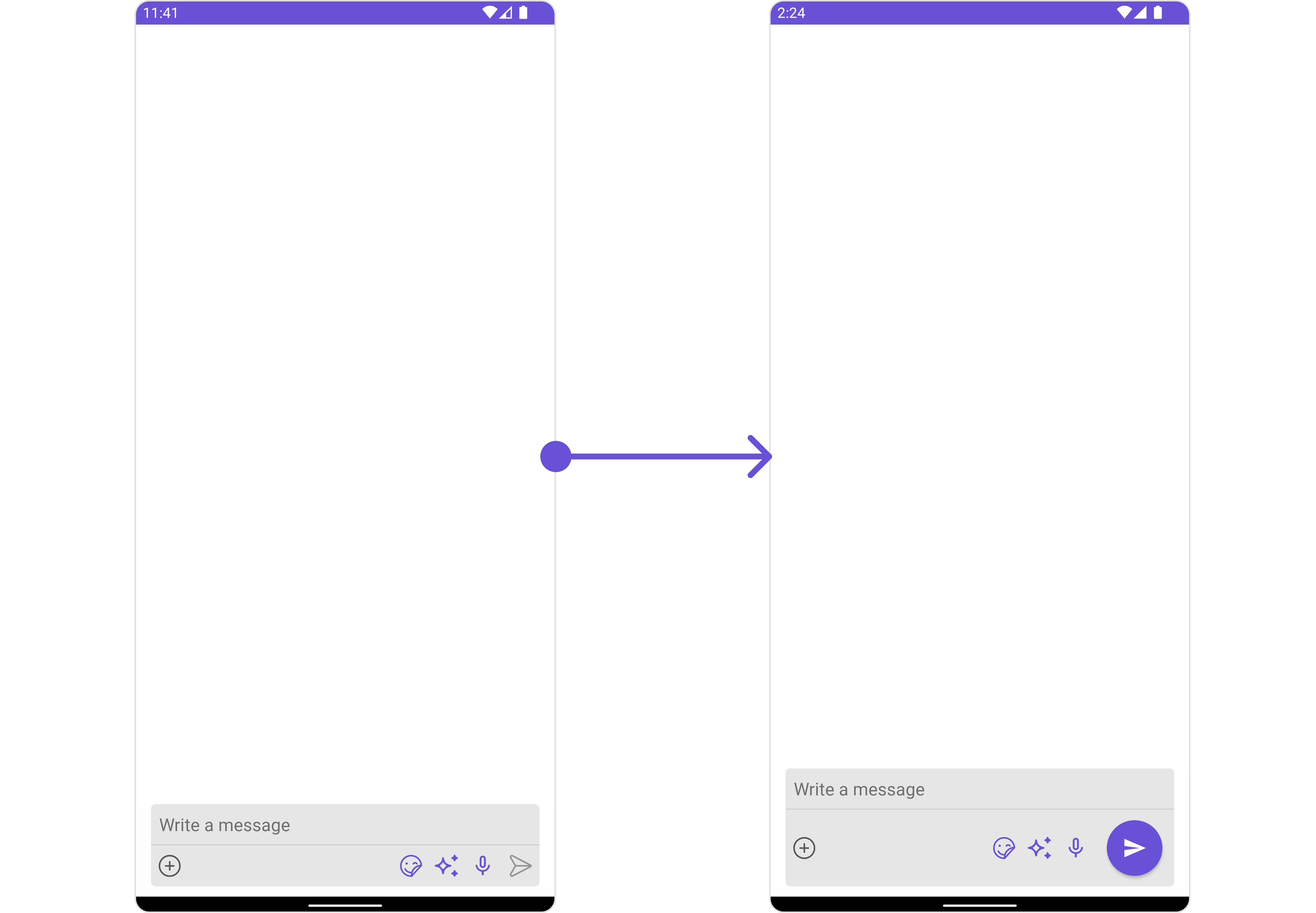
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.cardview.widget.CardView
android:id="@+id/card_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="5dp"
app:cardCornerRadius="30dp"
>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="15dp"
android:src="@drawable/ic_send"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
</RelativeLayout>
- Java
- Kotlin
View view = getLayoutInflater().inflate(R.layout.send_button_layout, null);
messageComposer.setSendButtonView(view);
View view = getLayoutInflater().inflate(R.layout.send_button_layout, null);
messageComposer.setSendButtonView(view);
HeaderView
You can set custom headerView to the MessageComposer component using the following method
- Java
- Kotlin
messageComposer.setHeaderView();
messageComposer.setHeaderView();
Example
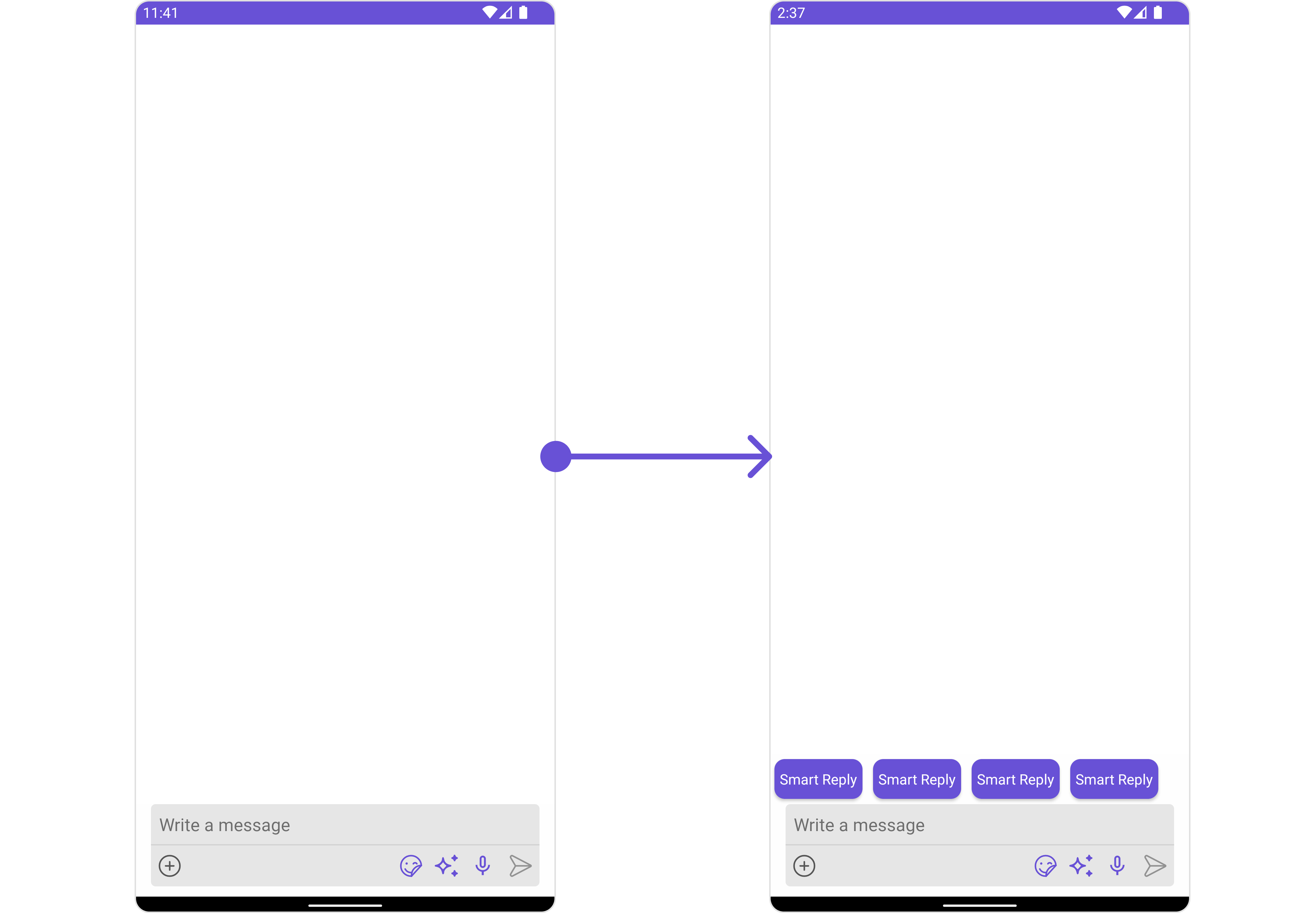
In the following example, we're going to apply a mock smart reply view to the MessageComposer Component using the .setHeaderView()
method.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:orientation="horizontal"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.cardview.widget.CardView
android:id="@+id/card1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Smart Reply"
android:padding="10dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
<androidx.cardview.widget.CardView
android:id="@+id/card2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Smart Reply"
android:padding="10dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
<androidx.cardview.widget.CardView
android:id="@+id/card3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Smart Reply"
android:padding="10dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
<androidx.cardview.widget.CardView
android:id="@+id/card4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Smart Reply"
android:padding="10dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
</LinearLayout>
- Java
- Kotlin
View view = getLayoutInflater().inflate(R.layout.custom_header_view_layout, null);
messageComposer.setHeaderView(view);
val view: View = LayoutInflater.from(context).inflate(R.layout.custom_header_view_layout, null)
messageComposer.setHeaderView(view)
FooterView
You can set a custom footer view to the MessageComposer component using the following method:
- Java
- Kotlin
messageComposer.setFooterView();
messageComposer.setFooterView();
Example
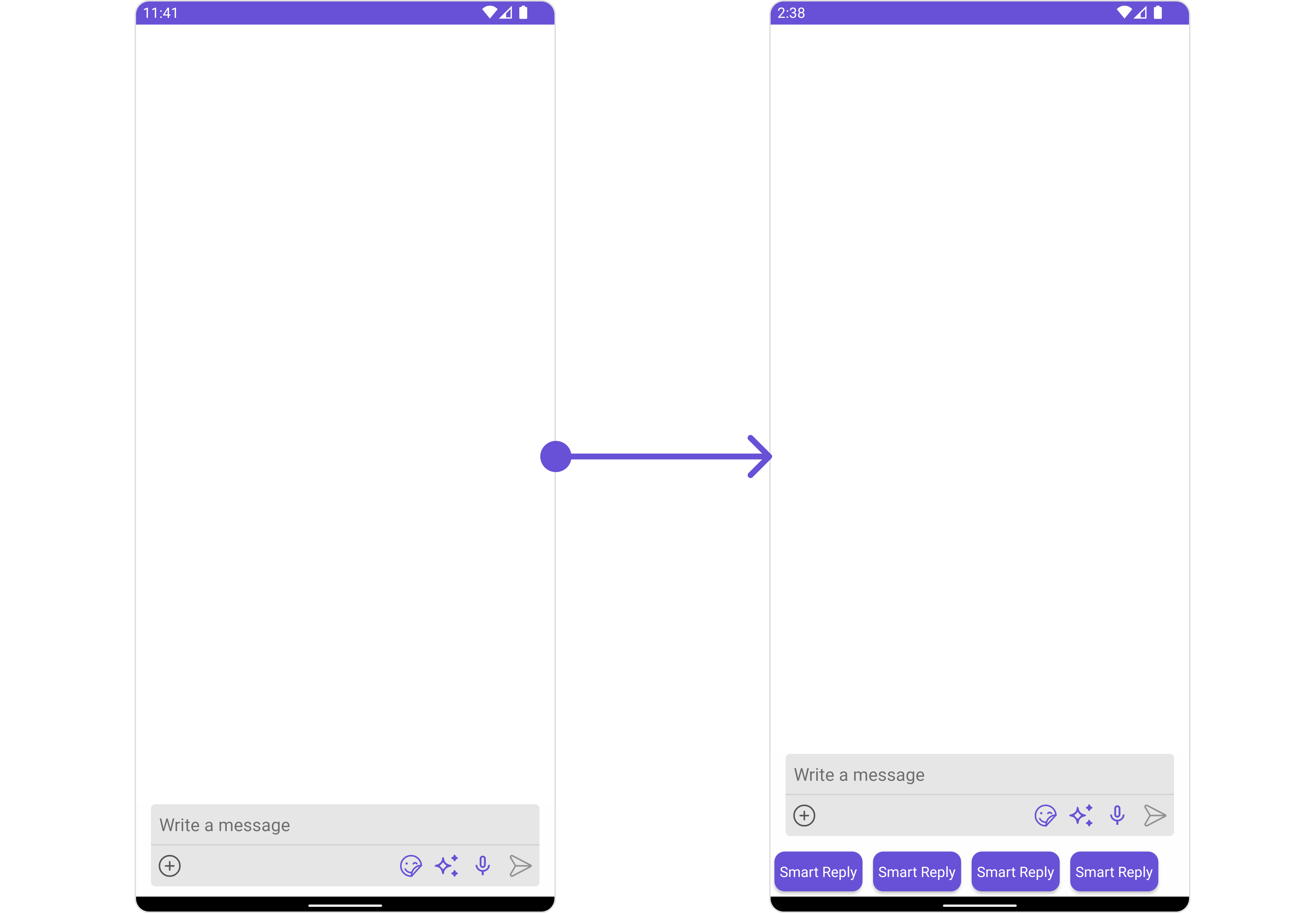
In the following example, we're going to apply a mock smart reply view to the MessageComposer Component using the .setFooterView()
method.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center_vertical"
android:orientation="horizontal"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.cardview.widget.CardView
android:id="@+id/card1"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Smart Reply"
android:padding="10dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
<androidx.cardview.widget.CardView
android:id="@+id/card2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Smart Reply"
android:padding="10dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
<androidx.cardview.widget.CardView
android:id="@+id/card3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Smart Reply"
android:padding="10dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
<androidx.cardview.widget.CardView
android:id="@+id/card4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:padding="10dp"
android:layout_margin="5dp"
app:cardCornerRadius="10dp"
>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Smart Reply"
android:padding="10dp"
android:paddingEnd="5dp"
android:paddingStart="5dp"
android:textColor="@color/white"
android:background="@color/purple_700"
/>
</androidx.cardview.widget.CardView>
</LinearLayout>
- Java
- Kotlin
View view = getLayoutInflater().inflate(R.layout.custom_footer_view_layout, null);
messageComposer.setHeaderView(view);
val view: View = LayoutInflater.from(context).inflate(R.layout.custom_footer_view_layout, null)
messageComposer.setHeaderView(view)