Incoming Call
Overview
The CometChatIncomingCall
is a Component that serves as a visual representation when the user receives an incoming call, such as a voice call or video call, providing options to answer or decline the call.
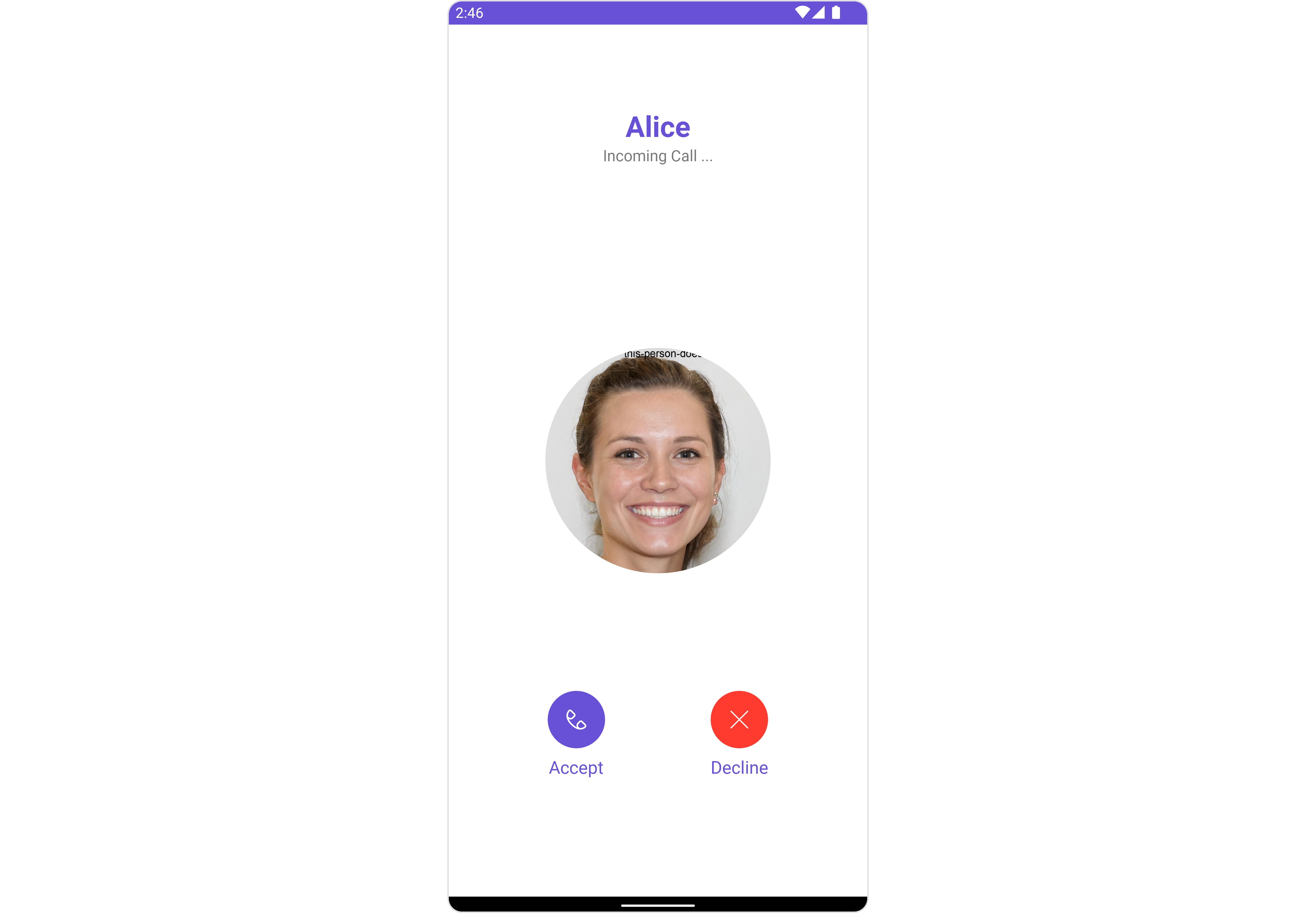
Usage
Integration
CometChatIncomingCall
being a custom component, offers versatility in its integration. It can be seamlessly launched via button clicks or any user-triggered action, enhancing the overall user experience and facilitating smoother interactions within the application.
Since CometChatIncomingCall
can be launched by adding the following code snippet into the XML layout file.
- XML
<com.cometchat.chatuikit.calls.incomingcall.CometChatIncomingCall
android:id="@+id/incoming_call"
android:layout_width="match_parent"
android:layout_height="match_parent" />
If you're defining the CometChatIncomingCall
within the XML code or in your activity or fragment then you'll need to extract them and set them on the User object using the appropriate method.
- Java
- Kotlin
CometChatIncomingCall cometchatIncomingCall = binding.incomingCall; // 'binding' is a view binding instance. Initialize it with `binding = YourXmlFileNameBinding.inflate(getLayoutInflater());` to use views like `binding.incomingCall` after enabling view binding.
User user = new User();
user.setUid(""); //Required
user.setName(""); //Required
user.setAvatar(""); //Required
cometchatIncomingCall.setUser(user); //Required - set the user object
val cometchatIncomingCall: CometChatIncomingCall = binding.incomingCall // 'binding' is a view binding instance. Initialize it with `binding = YourXmlFileNameBinding.inflate(layoutInflater)` to use views like `binding.incomingCall` after enabling view binding.
val user = User()
user.uid = "" //Required
user.name = "" //Required
user.avatar = "" //Required
cometchatIncomingCall.setUser(user) //Required - set the user object
Activity and Fragment
You can integrate CometChatIncomingCall
into your Activity and Fragment by adding the following code snippets into the respective classes.
- Java (Activity)
- Kotlin (Activity)
- Java (Fragment)
- Kotlin (Fragment)
CometChatIncomingCall cometchatIncomingCall;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
cometchatIncomingCall = new CometChatIncomingCall(this);
User user = new User();
user.setUid(""); //Required
user.setName(""); //Required
user.setAvatar(""); //Required
cometchatIncomingCall.setUser(user); //Required - set the user object
setContentView(cometchatIncomingCall);
}
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
cometchatIncomingCall = CometChatIncomingCall(this)
val user = User()
user.uid = "" //Required
user.name = "" //Required
user.avatar = "" //Required
cometchatIncomingCall.setUser(user) //Required - set the user object
setContentView(cometchatIncomingCall)
}
CometChatIncomingCall cometchatIncomingCall;
@Override
public View onCreateView(@NonNull LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
cometchatIncomingCall = new CometChatIncomingCall(requireContext());
User user = new User();
user.setUid(""); //Required
user.setName(""); //Required
user.setAvatar(""); //Required
cometchatIncomingCall.setUser(user); //Required - set the user object
return cometchatIncomingCall;
}
private lateinit var cometchatIncomingCall: CometChatIncomingCall
override fun onCreateView(
inflater: LayoutInflater,
container: ViewGroup?,
savedInstanceState: Bundle?
): View {
cometchatIncomingCall = CometChatIncomingCall(requireContext())
val user = User()
user.uid = "" //Required
user.name = "" //Required
user.avatar = "" //Required
cometchatIncomingCall.setUser(user) //Required - set the user object
return cometchatIncomingCall
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. SetOnAcceptCallClick
The setOnAcceptCallClick
action is typically triggered when the user clicks on the accept button, initiating a predefined action. However, by implementing the following code snippet, you can easily customize or override this default behavior to suit your specific requirements.
- Java
- Kotlin
cometchatIncomingCall.setOnAcceptCallClick(new OnClick() {
@Override
public void onClick() {
//TODO
}
});
cometchatIncomingCall.setOnAcceptCallClick(OnClick {
//TODO
})
2. SetOnDeclineCallClick
The SetOnDeclineCallClick
action is typically triggered when the user clicks on the reject button, initiating a predefined action. However, by implementing the following code snippet, you can easily customize or override this default behavior to suit your specific requirements.
- Java
- Kotlin
cometchatIncomingCall.setOnDeclineCallClick(new OnClick() {
@Override
public void onClick() {
//TODO
}
});
cometchatIncomingCall.setOnDeclineCallClick(OnClick {
//TODO
})
3. SetOnError
You can customize this behavior by using the provided code snippet to override the setOnError
and improve error handling.
- Java
- Kotlin
cometchatIncomingCall.setOnError(new OnError() {
@Override
public void onError(Context context, CometChatException e) {
//TODO
}
});
cometchatIncomingCall.setOnError(OnError { context, e ->
//TODO
})
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
The IncomingCall component does not have any exposed filters.
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The CometChatIncomingCall
component does not have any exposed events.
Customization
To fit your app's design requirements, you can customize the appearance of the conversation component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. IncomingCall Style
You can customize the appearance of the IncomingCall
Component by applying the IncomingCallStyle
to it using the following code snippet.
- Java
- Kotlin
IncomingCallStyle incomingCallStyle = new IncomingCallStyle();
incomingCallStyle.setTitleColor(R.color.yellow);
incomingCallStyle.setBackground(R.color.yellow);
incomingCallStyle.setBorderColor(R.color.yellow);
incomingCallStyle.setSubTitleColor(R.color.black);
incomingCallStyle.setBorderWidth(20);
cometchatIncomingCall.setStyle(incomingCallStyle);
val incomingCallStyle = IncomingCallStyle()
incomingCallStyle.setTitleColor(R.color.yellow)
incomingCallStyle.setBackground(R.color.yellow)
incomingCallStyle.setBorderColor(R.color.yellow)
incomingCallStyle.setSubTitleColor(R.color.black)
incomingCallStyle.setBorderWidth(20)
cometchatIncomingCall.setStyle(incomingCallStyle)
List of properties exposed by IncomingCallStyle
Property | Description | Code |
---|---|---|
Background | Used to set the background color | .setBackground(@ColorInt int) |
Background | Used to set background gradient | .setBackground(Drawable) |
Border Width | Used to set border | .setBorderWidth(int) |
Corner Radius | Used to set border radius | .setCornerRadius(float) |
Border Color | Used to set border color | .setBorderColor(@ColorInt int) |
Title Appearance | Used to customise the appearance of the title | .setTitleAppearance(@StyleRes int) |
Subtitle Appearance | Used to customise the appearance of the subtitle | .setSubTitleAppearance(@StyleRes int) |
2. Avatar Styles
To apply customized styles to the Avatar
component in the IncomingCall Component, you can use the following code snippet. For further insights on Avatar
Styles refer
- Java
- Kotlin
AvatarStyle avatarStyle = new AvatarStyle();
avatarStyle.setCornerRadius(10);
avatarStyle.setBorderWidth(20);
avatarStyle.setBorderColor(R.color.yellow);
cometchatIncomingCall.setAvatarStyle(avatarStyle);
val avatarStyle = AvatarStyle()
avatarStyle.setCornerRadius(10f)
avatarStyle.setBorderWidth(20)
avatarStyle.setBorderColor(R.color.yellow)
cometchatIncomingCall.setAvatarStyle(avatarStyle)
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
Example
In this example, we're enhancing the interface by customizing the accept and decline button icons. By setting custom icons for both the accept and decline buttons, users can enjoy a more visually appealing and personalized experience.
This level of customization allows developers to tailor the user interface to match the overall theme and branding of their application.
- Java
- Kotlin
cometchatIncomingCall.setDeclineButtonIcon(R.drawable.ic_call);
cometchatIncomingCall.setOnDeclineCallClick(new OnClick() {
@Override
public void onClick() {
//TODO
}
});
cometchatIncomingCall.setDeclineButtonIcon(R.drawable.ic_call)
cometchatIncomingCall.setOnDeclineCallClick(OnClick {
//TODO
})
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
Accept Button Icon | Sets a custom accept button icon url for the incoming call screen. | .setAcceptButtonIcon(@DrawableRes int) |
Accept Button Text | Sets custom text for the accept button on the incoming call screen. | .setAcceptButtonText(String) |
Call | Sets the Call object for which the incoming call screen is displayed. Required for call actions. | .setCall(Call) |
Custom Sound For Calls | Defines the path for custom sound for calls on the incoming call screen. | .setCustomSoundForCalls(@RawRes int) |
Decline Button Icon | Sets a custom decline button icon for the incoming call screen. | .setDeclineButtonIcon(@DrawableRes int) |
Decline Button Text | Sets custom text for the decline button on the incoming call screen. | .setDeclineButtonText(String) |
Disable Sound For Call | Defines whether to disable sound for the call on the incoming call screen. | .disableSoundForCall(boolean) |
Set User | Sets the User object of the user who initiated the call on the incoming call screen. | .setUser(User) |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
The CometChatIncomingCall
component does not provide additional functionalities beyond this level of customization.