Message Header
Overview
MessageHeader
is a Component that showcases the User or Group details in the toolbar. Furthermore, it also presents a typing indicator and a back navigation button for ease of use.
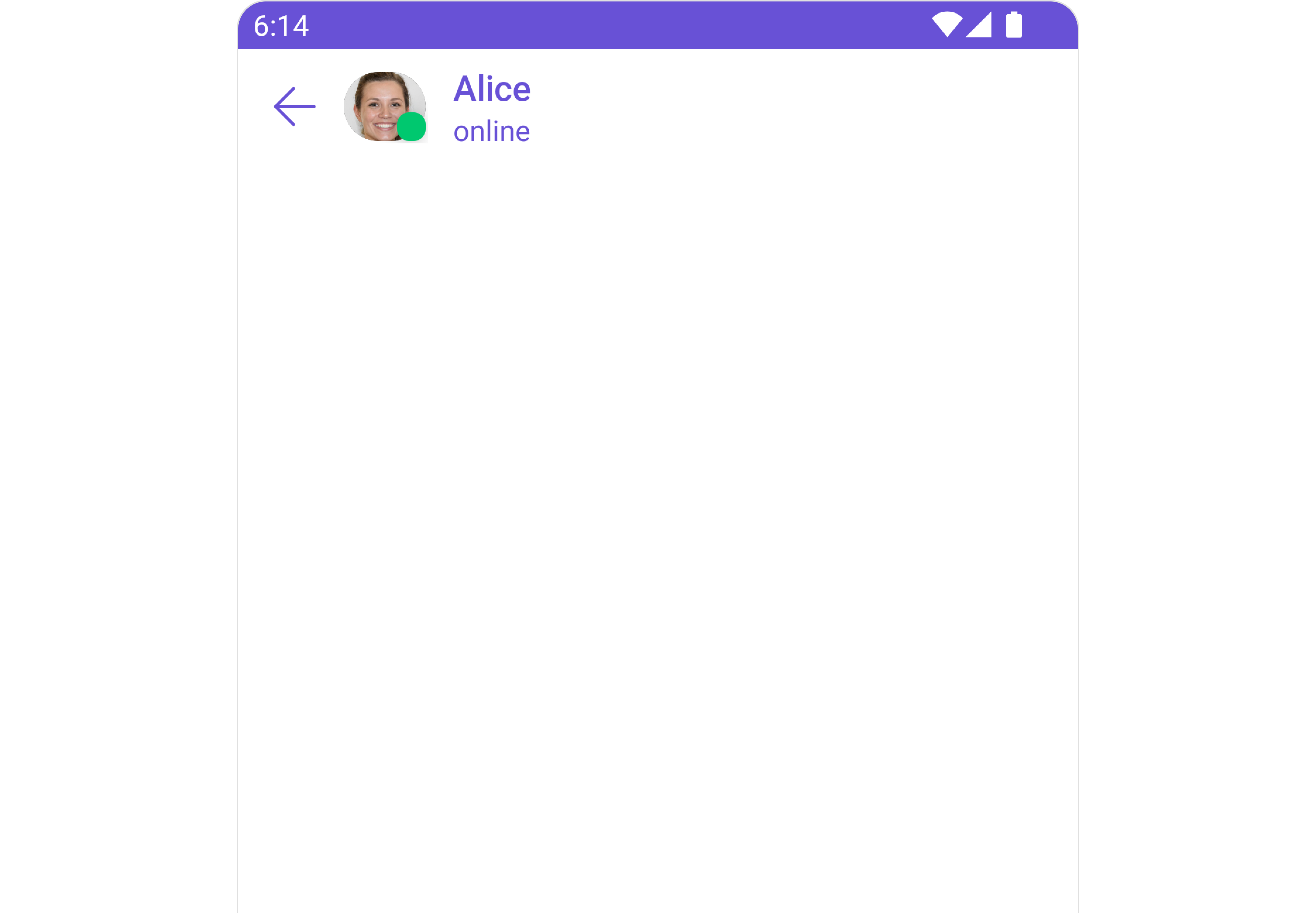
The MessageHeader
is comprised of the following components:
Components | Description |
---|---|
ListItem Component | This component’s view consists of avatar, status indicator , title, and subtitle. The fields are then mapped with the SDK’s user, group class. |
Back Button | BackButton that allows users to navigate back from the current activity or screen to the previous one |
Usage
Integration
You can add MessageHeader
component directly into the `layout.xml`` file.
<com.cometchat.chatuikit.messageheader.CometChatMessageHeader
android:id="@+id/header"
android:layout_width="match_parent"
android:layout_height="56dp" />
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
The MessageHeader
component does not have any exposed actions.
Filters
Filters allow you to customize the data displayed in a list within a Component
. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders
of Chat SDK.
The MessageHeader
component does not have any exposed filters.
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The MessageHeader
component does not produce any events.
Customization
To fit your app's design requirements, you can customize the appearance of the conversation component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. MessageHeader Style
To customize the appearance, you can assign a MessageHeaderStyle
object to the MessageHeader
component.
- Java
- Kotlin
MessageHeaderStyle style = new MessageHeaderStyle();
style.setTypingIndicatorTextColor(Color.parseColor("#ff0000"));
messageHeader.setStyle(style);
val style = MessageHeaderStyle()
style.typingIndicatorTextColor = Color.parseColor("#ff0000")
messageHeader.setStyle(style)
The properties exposed by MessageHeaderStyle
are as follows:
Property | Description | Code |
---|---|---|
Border Width | Used to set border | .setBorderWidth(int) |
Border Color | Used to set border color | .setBorderColor(@ColorInt int) |
Corner Radius | Used to set corner radius | .setCornerRadius(float) |
Background | Used to set background colour | .setBackground(@ColorInt int) |
Background | Used to set Drawable in background | .setBackground(@Drawable ) |
BackIcon Tint | Used to set back button icon tint | .setBackIconTint(@ColorInt int) |
TypingIndicator TextAppearance | Used to set typing indicator textStyle | .setTypingIndicatorTextAppearance(@StyleRes int) |
TypingIndicator TextColor | Used to set typing indicator text color | .setTypingIndicatorTextColor(@ColorInt int) |
SubtitleText Appearance | Used to set subtitle text style | .setSubtitleTextAppearance(@StyleRes int) |
SubtitleText Color | Used to set subtitle text color | .setSubtitleTextColor(@ColorInt int) |
OnlineStatus Color | Used to set online status colour | .setOnlineStatusColor(@ColorInt int) |
2. Avatar Style
If you want to apply customized styles to the Avatar
component within the MessageHeader
Component, you can use the following code snippet. For more information you can refer Avatar Styles.
- Java
- Kotlin
AvatarStyle avatarStyle = new AvatarStyle();
avatarStyle.setBorderWidth(10);
avatarStyle.setBorderColor(Color.BLACK);
messageHeader.setAvatarStyle(avatarStyle);
val avatarStyle = AvatarStyle()
avatarStyle.borderWidth = 10
avatarStyle.borderColor = Color.BLACK
messageHeader.setAvatarStyle(avatarStyle)
3. ListItem Style
If you want to apply customized styles to the ListItemStyle
component within the MessageHeader
Component, you can use the following code snippet. For more information, you can refer ListItem Styles.
- Java
- Kotlin
ListItemStyle listItemStyle = new ListItemStyle();
listItemStyle.setTitleColor(Color.BLACK);
messageHeader.setListItemStyle(listItemStyle);
val listItemStyle = ListItemStyle()
listItemStyle.setTitleColor(Color.BLACK)
messageHeader.setListItemStyle(listItemStyle)
4. StatusIndicator Style
If you want to apply customized styles to the Status Indicator
component within the MessageHeader
Component, you can use the following code snippet. For more information you can refer StatusIndicator Styles.
- Java
- Kotlin
StatusIndicatorStyle statusIndicatorStyle = new StatusIndicatorStyle();
statusIndicatorStyle.setCornerRadius(3.5f);
statusIndicatorStyle.setBorderColor(Color.GREEN);
messageHeader.setStatusIndicatorStyle(statusIndicatorStyle);
val statusIndicatorStyle = StatusIndicatorStyle()
statusIndicatorStyle.cornerRadius = 3.5f
statusIndicatorStyle.borderColor = Color.GREEN
messageHeader.setStatusIndicatorStyle(statusIndicatorStyle)
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
Here is a code snippet demonstrating how you can customize the functionality of the Message Header component.
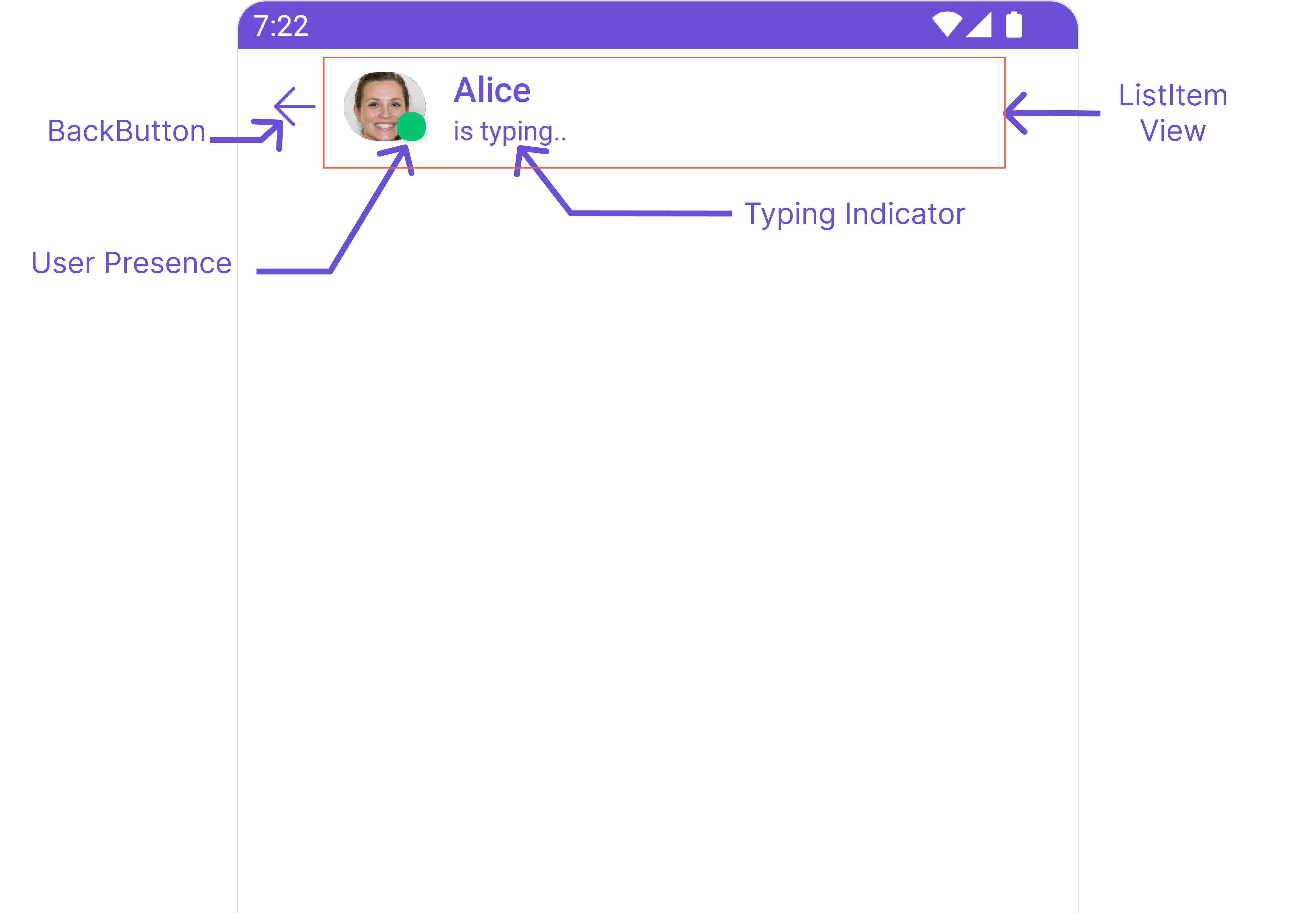
- Java
- Kotlin
CometChatMessageHeader header = findViewById(R.id.header);
header.setUser(user);
header.hideBackIcon(false);
val header = findViewById<CometChatMessageHeader>(R.id.header)
header.setUser(user)
header.hideBackIcon(false)
Following is a list of customizations along with their corresponding code snippets:
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
SetListItemView
The MessageHeader
component consists of a ListItemView
. You can customize the ListItem according to your requirements by using the .setListItemView
method.
header.setListItemView(View);
Example
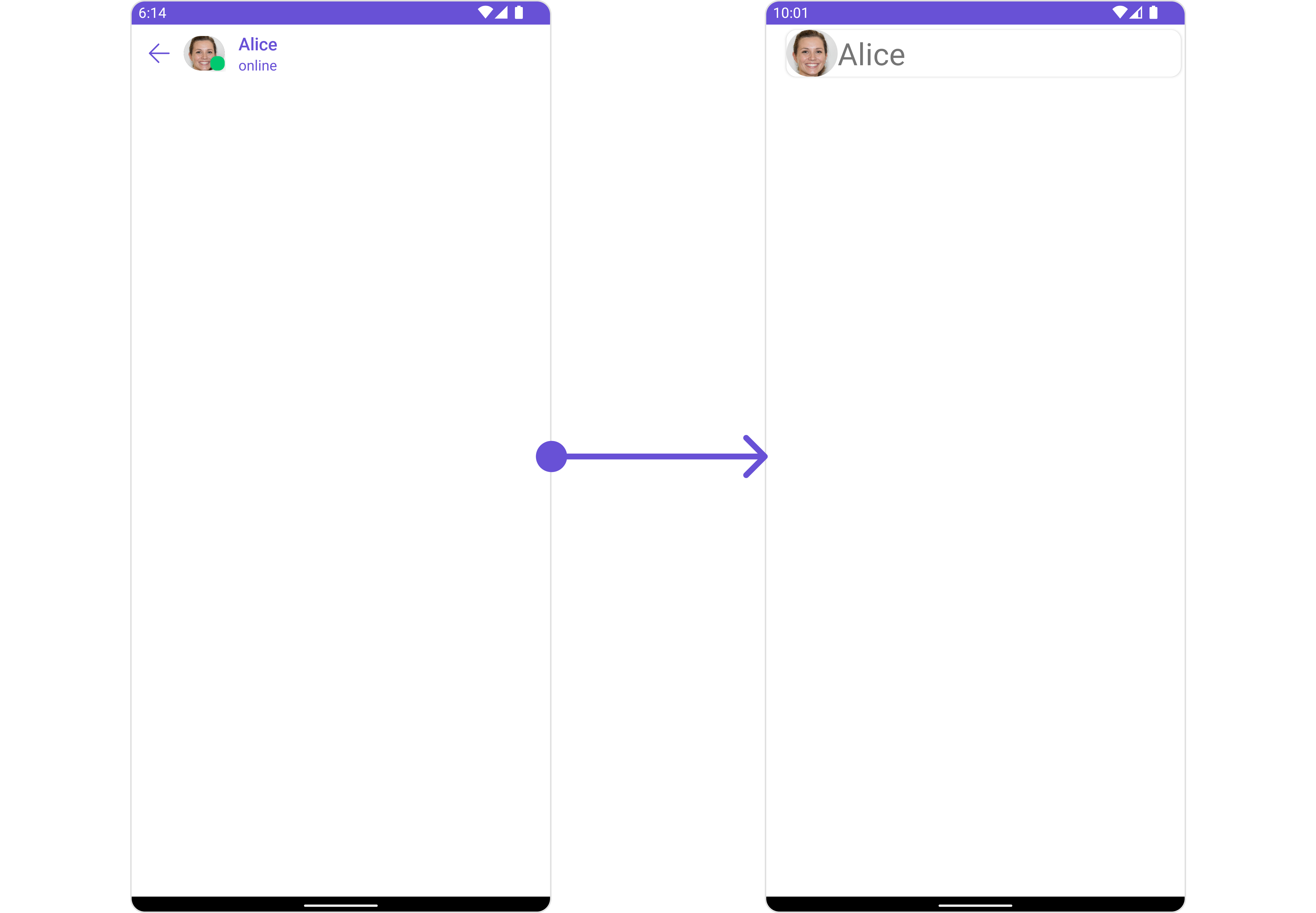
You have to create a custom_header_item.xml
as a custom layout file. This file can then be inflated and passed to setListItemView()
method.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.cardview.widget.CardView
android:layout_width="match_parent"
android:layout_height="54dp"
app:cardBackgroundColor="@color/white"
app:cardCornerRadius="10dp"
android:layout_margin="5dp"
android:elevation="20dp"
>
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<com.cometchat.chatuikit.shared.views.CometChatAvatar.CometChatAvatar
android:id="@+id/avatar_view"
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_centerVertical="true"
app:cardCornerRadius="10dp"
/>
<TextView
android:id="@+id/txt_name"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Name"
android:layout_toRightOf="@+id/avatar_view"
android:textSize="30sp"
android:layout_centerVertical="true"
/>
</RelativeLayout>
</androidx.cardview.widget.CardView>
</RelativeLayout>
- Java
- Kotlin
View view = getLayoutInflater().inflate(R.layout.custom_header_item, null);
CometChatAvatar avatar = view.findViewById(R.id.avatar_view);
TextView name = view.findViewById(R.id.txt_name);
avatar.setImage(user.getAvatar());
name.setText(user.getName());
header.setListItemView(view);
val view = layoutInflater.inflate(R.layout.custom_header_item, null)
val avatar = view.findViewById<CometChatAvatar>(R.id.avatar_view)
val name = view.findViewById<TextView>(R.id.txt_name)
avatar.setImage(user.avatar)
name.text = user.name
header.setListItemView(view)
SetSubtitleView
By using the .setSubtitleView
method, you can modify the SubtitleView to meet your specific needs.
header.setSubtitleView()
Example
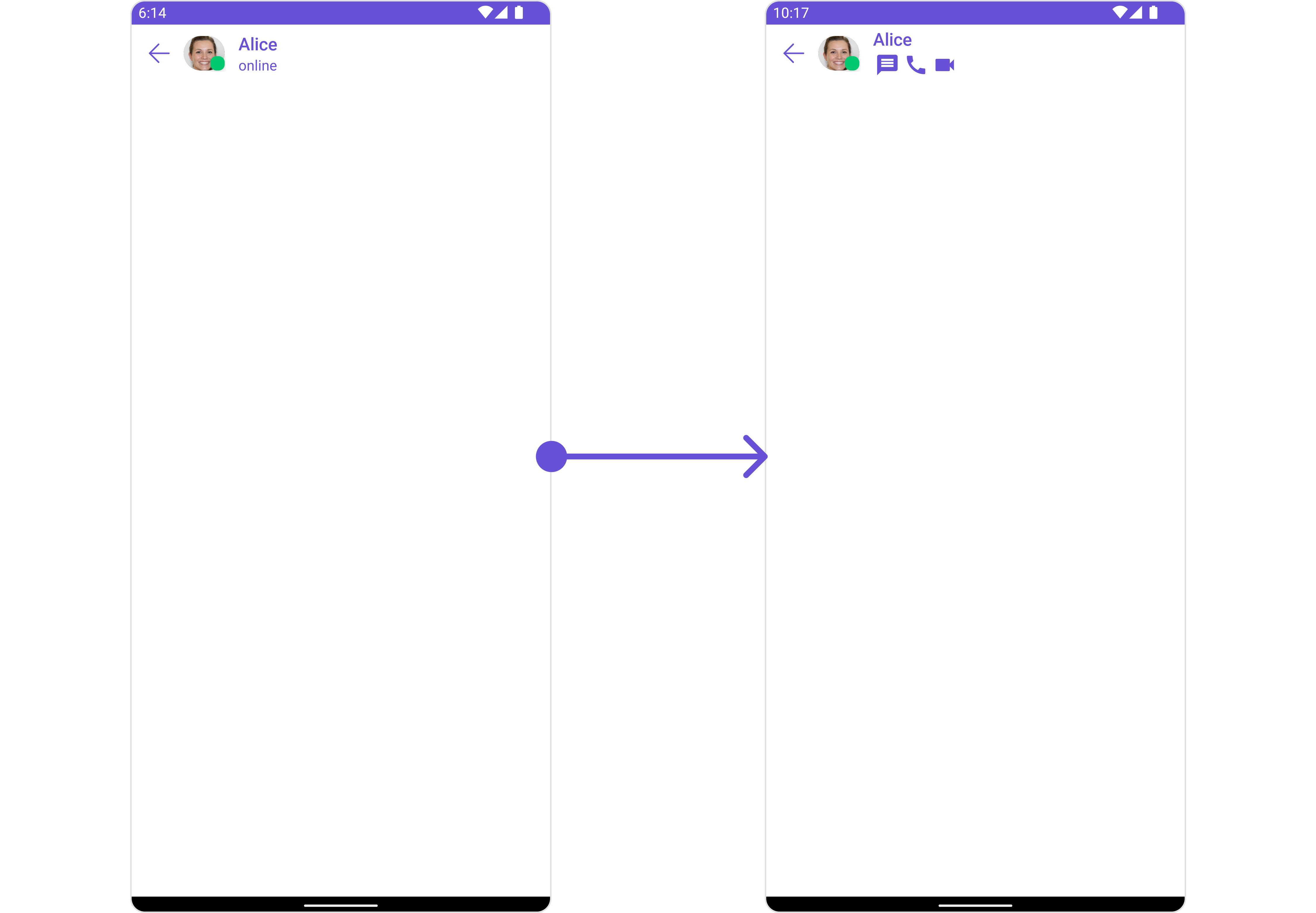
You should create a subtitle_layout.xml
file and inflate it inside apply
function.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<ImageView
android:id="@+id/img_conversation"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_message_grey"
android:layout_margin="2dp"
/>
<ImageView
android:id="@+id/img_audio_call"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_call"
android:layout_margin="2dp"
android:layout_toRightOf="@+id/img_conversation"
/>
<ImageView
android:id="@+id/img_video_call"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_video"
android:layout_margin="2dp"
android:layout_toRightOf="@+id/img_audio_call"
/>
</RelativeLayout>
- Java
- Kotlin
header.setSubtitleView(new Function3<Context, User, Group, View>() {
@Override
public View apply(Context context, User user, Group group) {
View view = getLayoutInflater().inflate(R.layout.subtitle_layout, null);
ImageView imgConversation = view.findViewById(R.id.img_conversation);
ImageView imgAudioCall = view.findViewById(R.id.img_audio_call);;
ImageView imgVideCall = view.findViewById(R.id.img_video_call);
imgConversation.setOnClickListener(v -> {
Toast.makeText(context, "Conversation Clicked", Toast.LENGTH_SHORT).show();
})
imgAudioCall.setOnClickListener(v -> {
Toast.makeText(context, "Audio Call Clicked", Toast.LENGTH_SHORT).show();
})
imgVideCall.setOnClickListener(v -> {
Toast.makeText(context, "Video Call Clicked", Toast.LENGTH_SHORT).show();
});
return view;
}
});
header.setSubtitleView { context, user, group ->
val view = layoutInflater.inflate(R.layout.subtitle_layout, null)
val imgConversation = view.findViewById<ImageView>(R.id.img_conversation)
val imgAudioCall = view.findViewById<ImageView>(R.id.img_audio_call)
val imgVideoCall = view.findViewById<ImageView>(R.id.img_video_call)
imgConversation.setOnClickListener {
Toast.makeText(context, "Conversation Clicked", Toast.LENGTH_SHORT).show()
}
imgAudioCall.setOnClickListener {
Toast.makeText(context, "Audio Call Clicked", Toast.LENGTH_SHORT).show()
}
imgVideoCall.setOnClickListener {
Toast.makeText(context, "Video Call Clicked", Toast.LENGTH_SHORT).show()
}
view
}
SetBackIcon
You can customize the Back Icon according to your specific requirements by using the .setBackIcon()
method.
header.setBackIcon(view);
Example
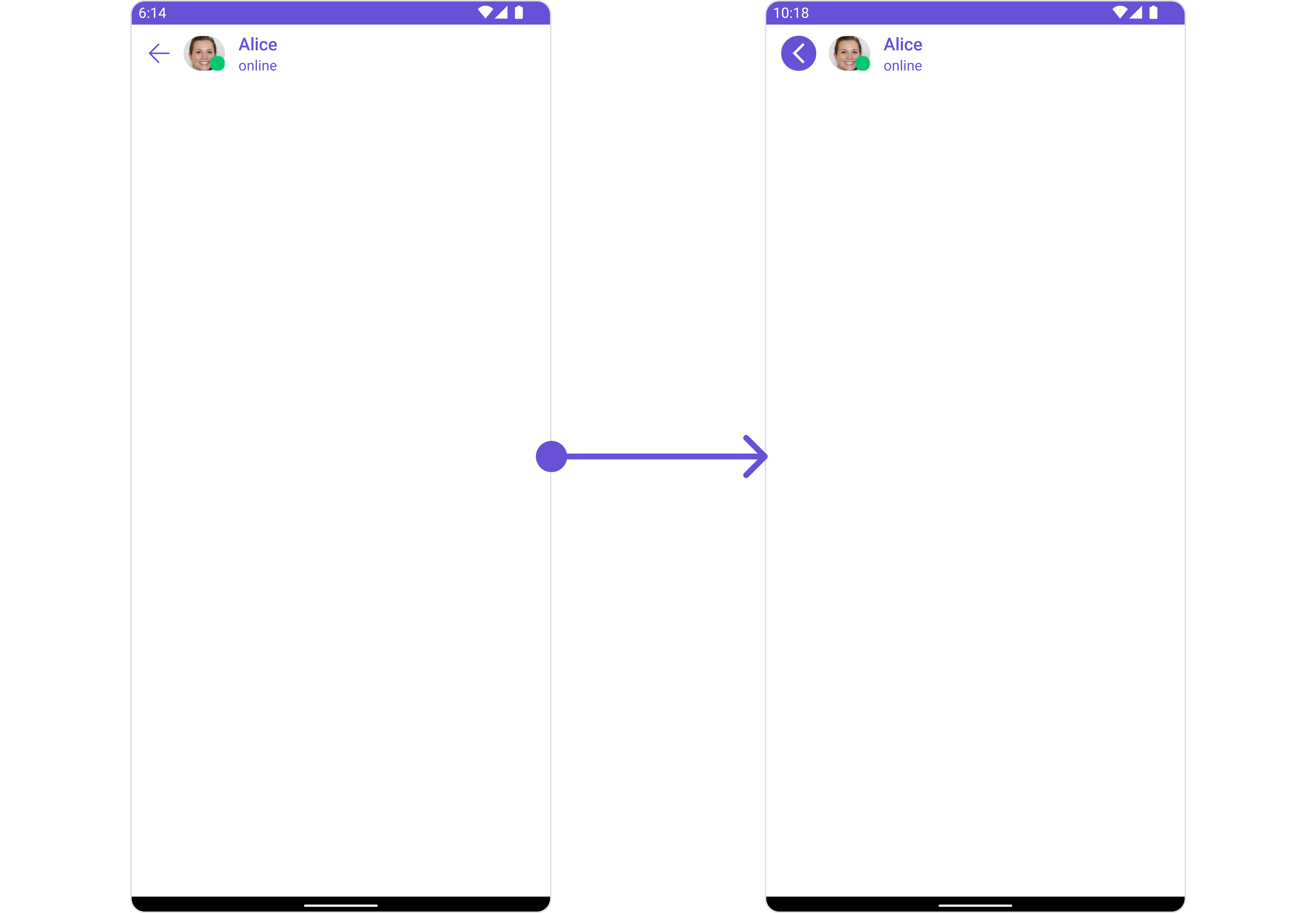
You need to create a custom_header_back_icon.xml
file, inflate it, and then pass it to the .setBackIcon(view)
method.
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto">
<androidx.cardview.widget.CardView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:elevation="20dp"
android:backgroundTint="#6851D6"
app:cardCornerRadius="20dp"
>
<ImageView
android:id="@+id/img_back"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:src="@drawable/ic_back_ios"
android:padding="5dp"
/>
</androidx.cardview.widget.CardView>
</RelativeLayout>
- Java
- Kotlin
View view = getLayoutInflater().inflate(R.layout.custom_header_back_icon, null);
ImageView imgBack = view.findViewById(R.id.img_back);
imgBack.setOnClickListener(v -> {
Toast.makeText(this, "BackIcon Clicked !!", Toast.LENGTH_SHORT).show();
});
header.setBackIcon(view);
val view = layoutInflater.inflate(R.layout.custom_header_back_icon, null)
val imgBack = view.findViewById<ImageView>(R.id.img_back)
imgBack.setOnClickListener {
Toast.makeText(this, "BackIcon Clicked !!", Toast.LENGTH_SHORT).show()
}
header.setBackIcon(view)
SetMenu
You can customize the Menu options to meet your specific needs by using the .setMenu()
method.
header.setMenu()
Example
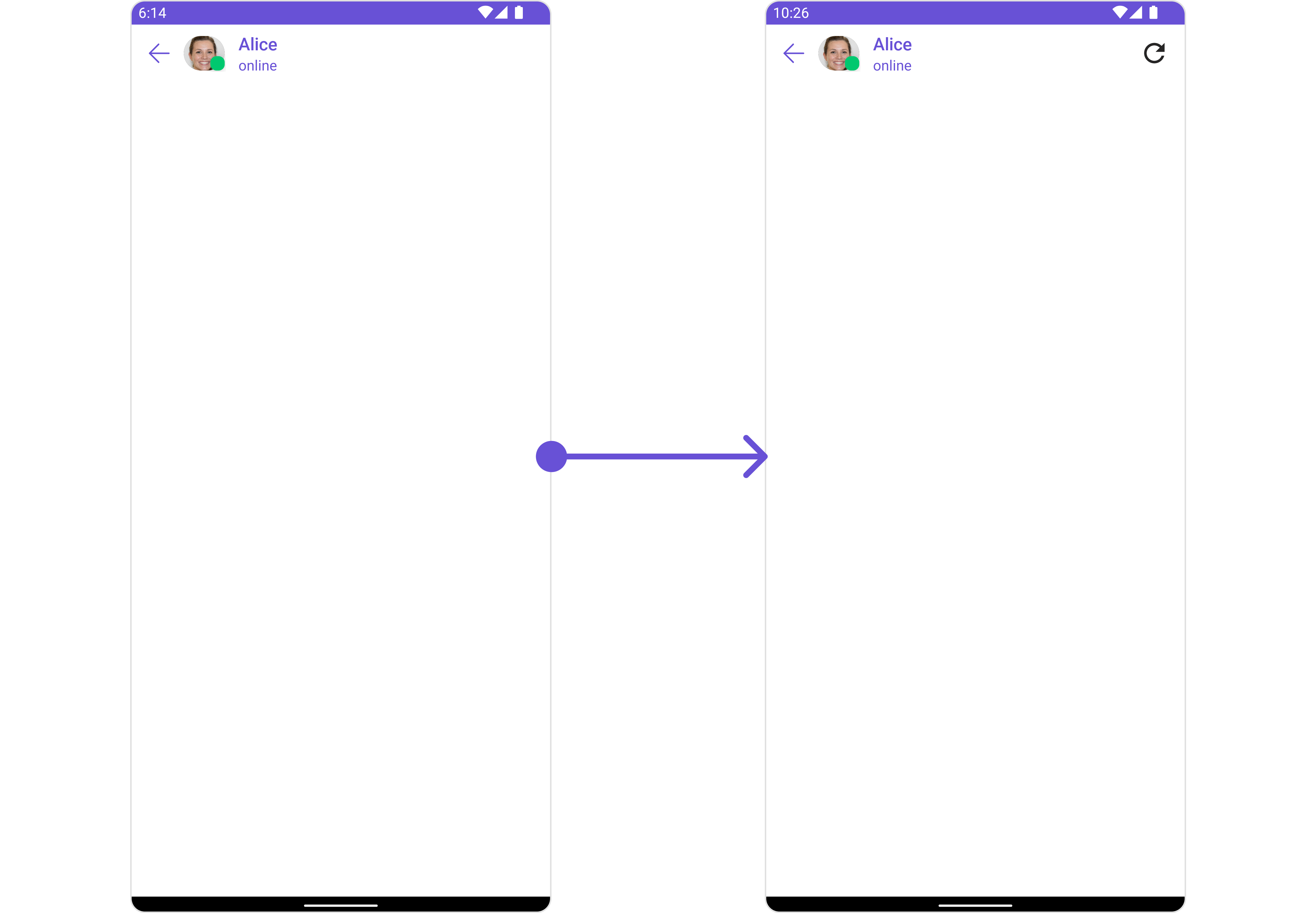
You need to create a view_menu.xml
file, inflate it, and then return it from .setBackIcon(view)
method.
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="horizontal"
tools:context=".MainActivity">
<ImageView
android:id="@+id/img_refresh"
android:layout_width="30dp"
android:layout_height="30dp"
android:src="@drawable/ic_refresh_black"
/>
</LinearLayout>
- Java
- Kotlin
header.setMenu(new Function3<Context, User, Group, View>() {
@Override
public View apply(Context context, User user, Group group) {
View view = getLayoutInflater().inflate(R.layout.view_menu, null);
ImageView imgRefresh = view.findViewById(R.id.img_refresh);
imgRefresh.setOnClickListener(v -> {
//Your Action
});
return view;
}
});
header.setMenu { context, user, group ->
val view = layoutInflater.inflate(R.layout.view_menu, null)
val imgRefresh = view.findViewById<ImageView>(R.id.img_refresh)
imgRefresh.setOnClickListener {
// Your Action
}
view
}