Group Members
Overview
CometChatGroupMembers
is a versatile Component designed to showcase all users who are either added to or invited to a group, thereby enabling them to participate in group discussions, access shared content, and engage in collaborative activities. Group members have the capability to communicate in real-time through messaging, voice and video calls, and various other interactions. Additionally, they can interact with each other, share files, and join calls based on the permissions established by the group administrator or owner.
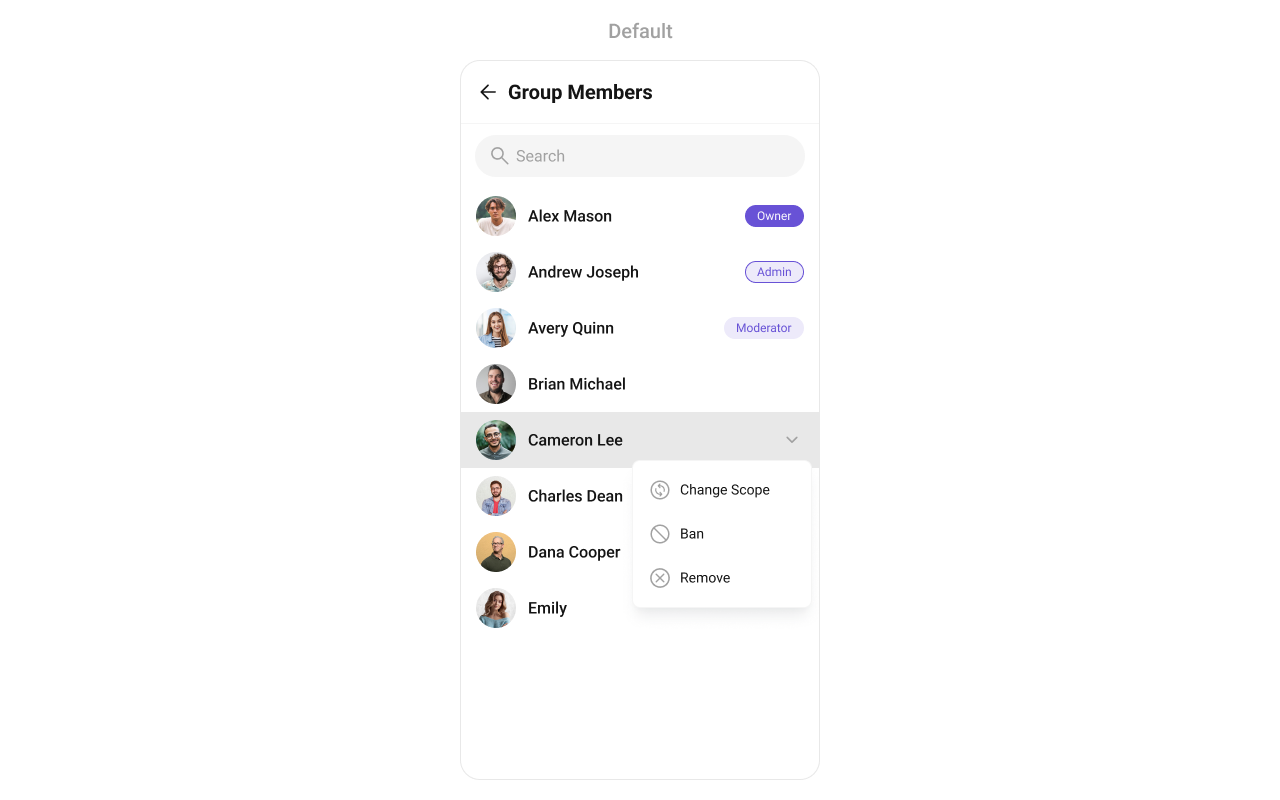
Usage
Integration
CometChatGroupMembers
, as a Composite Component, offers flexible integration options, allowing it to be launched directly via button clicks or any user-triggered action. Additionally, it seamlessly integrates into tab view controllers. With group members, users gain access to a wide range of parameters and methods for effortless customization of its user interface.
The following code snippet exemplifies how you can seamlessly integrate the GroupMembers component into your application.
- XML
<com.cometchat.chatuikit.groupmembers.CometChatGroupMembers
android:id="@+id/group_member"
android:layout_width="match_parent"
android:layout_height="match_parent" />
If you're defining the Group members within the XML code, you'll need to extract them and set them on the Group object using the appropriate method.
- Java
- Kotlin
CometChatGroupMembers cometchatGroupMembers = binding.groupMember;
Group group = new Group();
group.setGuid("GROUP_ID");
group.setName("GROUP_NAME");
cometchatGroupMembers.setGroup(group);
val cometchatGroupMembers: CometChatGroupMembers = binding.groupMember
val group: Group = Group()
group.setGuid("GROUP_ID")
group.setName("GROUP_NAME")
cometchatGroupMembers.setGroup(group)
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
setOnItemClick
Function invoked when a user item is clicked, typically used to open a user profile or chat screen.
- Java
- Kotlin
cometchatUsers.setOnItemClick((view1, position, user) -> {
});
cometchatUsers.onItemClick = OnItemClick { view, position, user ->
}
setOnItemLongClick
Function executed when a user item is long-pressed, allowing additional actions like delete or block.
- Java
- Kotlin
cometchatUsers.setOnItemLongClick((view1, position, user) -> {
});
cometchatUsers.onItemLongClick = OnItemLongClick({ view, position, user ->
})
setOnBackPressListener
OnBackPressListener
is triggered when you press the back button in the app bar. It has a predefined behavior; when clicked, it navigates to the previous activity. However, you can override this action using the following code snippet.
- Java
- Kotlin
cometchatUsers.setOnBackPressListener(() -> {
});
cometchatUsers.onBackPressListener = OnBackPress {
}
setOnSelect
Called when a item from the fetched list is selected, useful for multi-selection features.
- Java
- Kotlin
cometchatUsers.setOnSelect(t -> {
});
cometchatUsers.setOnSelect(object : OnSelection<User?> {
override fun onSelection(t: MutableList<User?>?) {
}
})
setOnError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the Users component.
- Java
- Kotlin
cometchatUsers.setOnError(cometchatException -> {
});
cometchatUsers.setOnError {
}
setOnLoad
Invoked when the list is successfully fetched and loaded, helping track component readiness.
- Java
- Kotlin
cometchatUsers.setOnLoad(list -> {
});
cometchatUsers.setOnLoad(object : OnLoad<User?> {
override fun onLoad(list: MutableList<User?>?) {
}
})
setOnEmpty
Called when the list is empty, enabling custom handling such as showing a placeholder message.
- Java
- Kotlin
cometchatUsers.setOnEmpty(() -> {
});
cometchatUsers.setOnEmpty{
}
Filters
Filters allow you to customize the data displayed in a list within a Component
. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders
of Chat SDK.
1. GroupsRequestBuilder
The GroupsRequestBuilder enables you to filter and customize the group list based on available parameters in GroupsRequestBuilder. This feature allows you to create more specific and targeted queries when fetching groups. The following are the parameters available in GroupsRequestBuilder
Property | Description | Code |
---|---|---|
Limit | Sets the number of group members that can be fetched in a single request, suitable for pagination. | .setLimit(int) |
Search Keyword | Used for fetching group members matching the passed string. | .setSearchKeyword(String) |
Scopes | Used for fetching group members having matching scopes which may be of participant, moderator, admin, and owner. | .setScopes(List<String>) |
Example
In the example below, we are applying a filter to the Group List based on limit and scope.
- Java
- Kotlin
GroupMembersRequest.GroupMembersRequestBuilder groupMembersRequestBuilder= new GroupMembersRequest.GroupMembersRequestBuilder(GUID).setLimit(limit);
cometchatGroupMembers.setGroupMembersRequestBuilder(groupMembersRequestBuilder);
val groupMembersRequestBuilder = GroupMembersRequestBuilder(GUID).setLimit(limit)
cometchatGroupMembers.setGroupMembersRequestBuilder(groupMembersRequestBuilder)
2. SearchRequestBuilder
The SearchRequestBuilder uses GroupsRequestBuilder enables you to filter and customize the search list based on available parameters in GroupsRequestBuilder.
This feature allows you to keep uniformity between the displayed Groups List and searched Group List.
Example
- Java
- Kotlin
GroupMembersRequest.GroupMembersRequestBuilder groupMembersRequestBuilder= new GroupMembersRequest.GroupMembersRequestBuilder(GUID).setLimit(LIMIT).setSearchKeyword(SEARCH_KEYWORD);
cometchatGroupMembers.setSearchRequestBuilder(groupMembersRequestBuilder);
val groupMembersRequestBuilder = GroupMembersRequestBuilder(GUID).setLimit(LIMIT).setSearchKeyword(SEARCH_KEYWORD)
cometchatGroupMembers.setSearchRequestBuilder(groupMembersRequestBuilder)
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
Events emitted by the Join Group component is as follows.
Event | Description |
---|---|
ccGroupMemberBanned | Triggers when the group member banned from the group successfully |
ccGroupMemberKicked | Triggers when the group member kicked from the group successfully |
ccGroupMemberScopeChanged | Triggers when the group member scope is changed in the group |
- Java
- Kotlin
CometChatGroupEvents.addGroupListener("LISTENER_ID", new CometChatGroupEvents() {
@Override
public void ccGroupMemberKicked(Action actionMessage, User kickedUser, User kickedBy, Group kickedFrom) {
super.ccGroupMemberKicked(actionMessage, kickedUser, kickedBy, kickedFrom);
}
@Override
public void ccGroupMemberBanned(Action actionMessage, User bannedUser, User bannedBy, Group bannedFrom) {
super.ccGroupMemberBanned(actionMessage, bannedUser, bannedBy, bannedFrom);
}
@Override
public void ccGroupMemberScopeChanged(Action actionMessage, User updatedUser, String scopeChangedTo, String scopeChangedFrom, Group group) {
super.ccGroupMemberScopeChanged(actionMessage, updatedUser, scopeChangedTo, scopeChangedFrom, group);
}
});
CometChatGroupEvents.addGroupListener("LISTENER_ID", object : CometChatGroupEvents() {
override fun ccGroupMemberKicked(
actionMessage: Action,
kickedUser: User,
kickedBy: User,
kickedFrom: Group
) {
super.ccGroupMemberKicked(actionMessage, kickedUser, kickedBy, kickedFrom)
}
override fun ccGroupMemberBanned(
actionMessage: Action,
bannedUser: User,
bannedBy: User,
bannedFrom: Group
) {
super.ccGroupMemberBanned(actionMessage, bannedUser, bannedBy, bannedFrom)
}
override fun ccGroupMemberScopeChanged(
actionMessage: Action,
updatedUser: User,
scopeChangedTo: String,
scopeChangedFrom: String,
group: Group
) {
super.ccGroupMemberScopeChanged(
actionMessage,
updatedUser,
scopeChangedTo,
scopeChangedFrom,
group
)
}
})
Remove the added listener
- Java
- Kotlin
CometChatGroupEvents.removeListener("LISTENER_ID");
CometChatGroupEvents.removeListener("LISTENER_ID")
Customization
To fit your app's design requirements, you can customize the appearance of the Groups component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
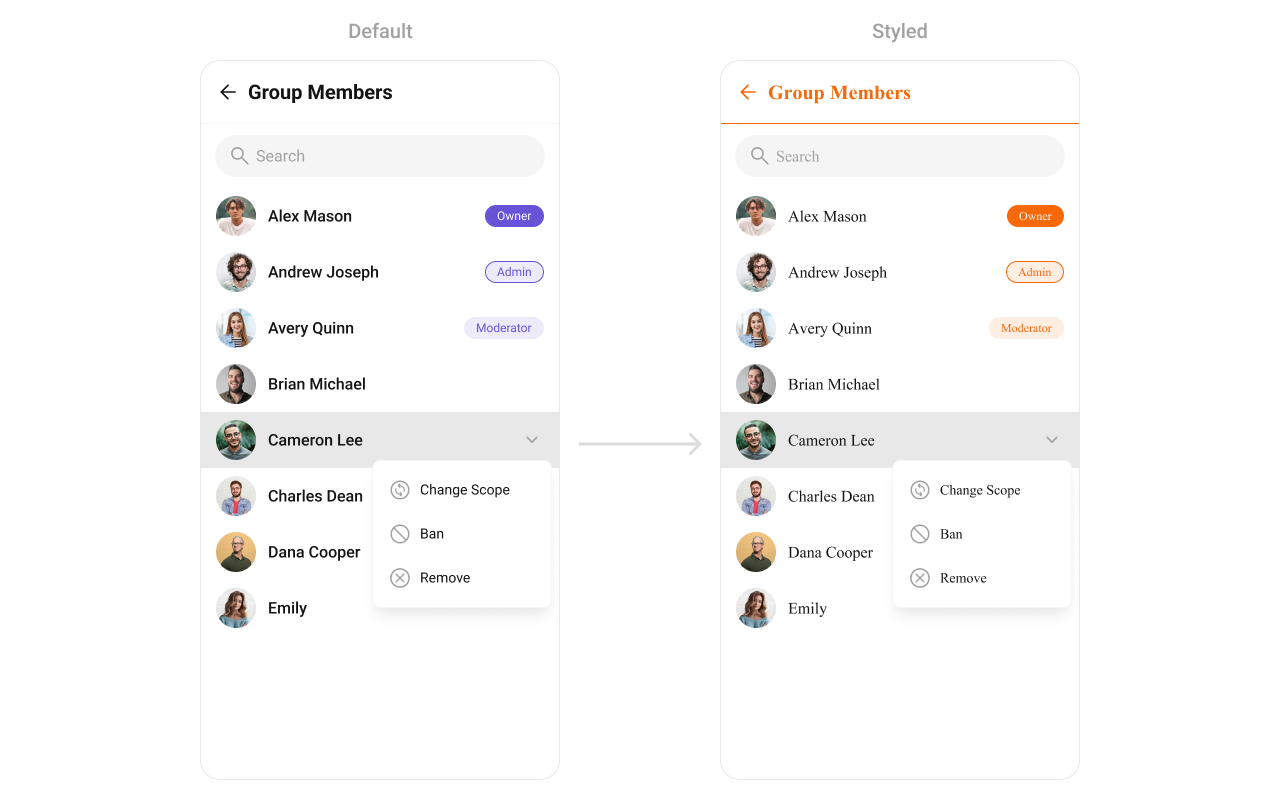
<style name="CustomAvatarStyle" parent="CometChatAvatarStyle">
<item name="cometchatAvatarStrokeRadius">8dp</item>
<item name="cometchatAvatarBackgroundColor">#FBAA75</item>
</style>
<style name="CustomGroupMembersStyle" parent="CometChatGroupMembersStyle">
<item name="cometchatGroupMembersAvatarStyle">@style/CustomAvatarStyle</item>
<item name="cometchatGroupMembersSeparatorColor">#F76808</item>
<item name="cometchatGroupMembersTitleTextColor">#F76808</item>
<item name="cometchatGroupMembersBackIconTint">#F76808</item>
</style>
- Java
- Kotlin
cometchatGroupMembers.setStyle(R.style.CustomGroupMembersStyle);
cometchatGroupMembers.setStyle(R.style.CustomGroupMembersStyle)
To know more such attributes, visit the attributes file.
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can toggle the visibility of UI elements.
Below is a list of customizations along with corresponding code snippets
Methods | Description | Code |
---|---|---|
setGroup | Sets the group whose members need to be fetched. This is a required property for the component to function properly. | .setGroup(group); |
setBackIconVisibility | Used to toggle visibility for back button in the app bar | .setBackIconVisibility(View.VISIBLE); |
setToolbarVisibility | Used to toggle visibility for back button in the app bar | .setToolbarVisibility(View.GONE); |
setErrorStateVisibility | Used to hide error state on fetching Users | .setErrorStateVisibility(View.GONE); |
setEmptyStateVisibility | Used to hide empty state on fetching Users | .setEmptyStateVisibility(View.GONE); |
setLoadingStateVisibility | Used to hide loading state while fetching Users | .setLoadingStateVisibility(View.GONE); |
setSeparatorVisibility | Used to control visibility of Separators in the list view | .setSeparatorVisibility(View.GONE); |
setUsersStatusVisibility | Used to control visibility of status indicator shown if user is online | .setUsersStatusVisibility(View.GONE); |
setSelectionMode | This method determines the selection mode for members, enabling users to select either a single or multiple members at once. | .setSelectionMode(UIKitConstants.SelectionMode.MULTIPLE); |
setSearchkeyword | Used for fetching members matching the passed keywords | .setSearchkeyword("anything"); |
setSearchBoxVisibility | Used to hide search box shown in the tool bar | .setSearchBoxVisibility(View.GONE); |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your own views, layouts, and UI elements and then incorporate those into the component.
The Group Memebers
component does not provide additional functionalities beyond this level of customization.
setOptions
Defines a set of available actions that users can perform when they interact with a group member item.
Use Cases:
- Provide actions like "View Profile", "Send Message", "Remove from Group".
- Restrict certain actions to admins (e.g., "Promote to Admin", "Ban User").
- Java
- Kotlin
cometchatUsers.setOptions((context, user) -> Collections.emptyList());
cometchatUsers.options = Function2<Context?, User?, List<CometChatPopupMenu.MenuItem?>?> { context, user -> emptyList<CometChatPopupMenu.MenuItem?>() }
addOptions
Adds custom actions to the existing options available when interacting with a group member.
Use Cases:
- Extend functionality by adding "Block User", "Report User", or "View Activity".
- Customize actions based on member roles.
- Java
- Kotlin
cometchatUsers.addOptions((context, user) -> Collections.emptyList());
cometchatUsers.addOptions { context, user -> emptyList<CometChatPopupMenu.MenuItem?>() }
setLoadingView
Displays a custom loading view while group members are being fetched.
Use Cases:
- Show a loading spinner with "Fetching group members...".
- Implement a skeleton loader for a smoother UI experience.
- Java
- Kotlin
cometchatUsers.setLoadingView(R.layout.your_loading_view);
cometchatUsers.loadingView = R.layout.your_loading_view
setEmptyView
Configures a view to be displayed when no group members are found.
Use Cases:
- Display a message like "No members in this group yet.".
- Show a button to Invite Members.
- Java
- Kotlin
cometchatUsers.setEmptyView(R.layout.your_empty_view);
cometchatUsers.emptyView = R.layout.your_empty_view
setErrorView
Defines a custom error state view when there is an issue loading group members.
Use Cases:
- Display a retry button with "Failed to load members. Tap to retry.".
- Show an illustration for a better user experience.
- Java
- Kotlin
cometchatUsers.setErrorView(R.layout.your_empty_view);
cometchatUsers.errorView = R.layout.your_error_view
setLeadingView
Sets a custom leading view for each group member item, usually used for profile images or avatars.
Use Cases:
- Show a circular avatar with an online/offline indicator.
- Add a role-based badge (Admin, Moderator, Member).
- Java
- Kotlin
cometchatUsers.setLeadingView(new UsersViewHolderListener() {
@Override
public View createView(Context context, CometchatListBaseItemsBinding listItem) {
return null;
}
@Override
public void bindView(Context context, View createdView, User user, RecyclerView.ViewHolder holder, List<User> userList, int position) {
}
});
cometchatUsers.setLeadingView(object: UsersViewHolderListener {
override fun createView(context: Context, cometChatListItem: CometChatListItem): View? {
return null
}
override fun bindView(context: Context, view: View, user: User, viewHolder: RecyclerView.ViewHolder, list: List<User>, i: Int) {
}
})

You can indeed create a custom layout file named custom_title_view.xml
for more complex or unique list items.
Once this layout file is made, you would inflate it inside the createView()
method of the GroupsViewHolderListener
. The inflation process prepares the layout for use in your application:
Following this, you would use the bindView()
method to initialize and assign values to your individual views. This could include setting text on TextViews, images on ImageViews, and so on based on the properties of the Group object:
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="@dimen/cometchat_45dp"
android:layout_height="@dimen/cometchat_45dp"
android:orientation="vertical">
<com.cometchat.chatuikit.shared.views.avatar.CometChatAvatar
android:id="@+id/avatar"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
<View
android:id="@+id/batch_view"
android:layout_width="match_parent"
android:layout_height="@dimen/cometchat_12dp"
android:layout_alignParentBottom="true"
android:background="@drawable/admin_batch"
android:visibility="gone" />
</RelativeLayout>
- Java
- Kotlin
cometchatGroupMembers.setTitleView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometchatGroupMemberListItemBinding listItem) {
return LayoutInflater.from(context).inflate(R.layout.header_leading_view, null, false);
}
@Override
public void bindView(Context context, View createdView, GroupMember groupMember, Group group, RecyclerView.ViewHolder holder, List<GroupMember> groupMemberList, int position) {
CometChatAvatar avatar = createdView.findViewById(R.id.avatar);
avatar.setAvatar(groupMember.getName(), groupMember.getAvatar());
View role = createdView.findViewById(R.id.batch_view);
if (UIKitConstants.GroupMemberScope.ADMIN.equals(groupMember.getScope())) {
role.setVisibility(View.VISIBLE);
role.setBackground(ResourcesCompat.getDrawable(getResources(), R.drawable.marketing_head, null));
} else if (UIKitConstants.GroupMemberScope.MODERATOR.equals(groupMember.getScope())) {
role.setVisibility(View.VISIBLE);
role.setBackground(ResourcesCompat.getDrawable(getResources(), R.drawable.sr_manager, null));
} else if (UIKitConstants.GroupMemberScope.PARTICIPANTS.equals(groupMember.getScope())) {
role.setVisibility(View.VISIBLE);
role.setBackground(ResourcesCompat.getDrawable(getResources(), R.drawable.content_manager, null));
} else {
role.setBackground(ResourcesCompat.getDrawable(getResources(), R.drawable.team_member, null));
}
LinearLayout.LayoutParams layoutParams = new LinearLayout.LayoutParams(Utils.convertDpToPx(context, 40),
Utils.convertDpToPx(context, 40));
createdView.setLayoutParams(layoutParams);
}
});
cometchatGroupMembers.setLeadingView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context?, listItem: CometchatGroupMemberListItemBinding?): View {
return LayoutInflater.from(context).inflate(R.layout.header_leading_view, null, false)
}
override fun bindView(
context: Context,
createdView: View,
groupMember: GroupMember,
group: Group,
holder: RecyclerView.ViewHolder,
groupMemberList: List<GroupMember>,
position: Int
) {
val avatar = createdView.findViewById<CometChatAvatar>(R.id.avatar)
avatar.setAvatar(groupMember.name, groupMember.avatar)
val role = createdView.findViewById<View>(R.id.batch_view)
if (UIKitConstants.GroupMemberScope.ADMIN == groupMember.scope) {
role.visibility = View.VISIBLE
role.background = ResourcesCompat.getDrawable(resources, R.drawable.marketing_head, null)
} else if (UIKitConstants.GroupMemberScope.MODERATOR == groupMember.scope) {
role.visibility = View.VISIBLE
role.background = ResourcesCompat.getDrawable(resources, R.drawable.sr_manager, null)
} else if (UIKitConstants.GroupMemberScope.PARTICIPANTS == groupMember.scope) {
role.visibility = View.VISIBLE
role.background = ResourcesCompat.getDrawable(resources, R.drawable.content_manager, null)
} else {
role.background = ResourcesCompat.getDrawable(resources, R.drawable.team_member, null)
}
val layoutParams = LinearLayout.LayoutParams(
Utils.convertDpToPx(context, 40),
Utils.convertDpToPx(context, 40)
)
createdView.layoutParams = layoutParams
}
})
setTitleView
Customizes the title view, typically displaying the member's name.
Use Cases:
- Customize fonts, colors, or styles for usernames.
- Add role-specific indicators like "(Group Admin)".
- Java
- Kotlin
cometchatUsers.setTitleView(new UsersViewHolderListener() {
@Override
public View createView(Context context, CometchatListBaseItemsBinding listItem) {
return null;
}
@Override
public void bindView(Context context, View createdView, User user, RecyclerView.ViewHolder holder, List<User> userList, int position) {
}
});
cometchatUsers.setTitleView(object: UsersViewHolderListener {
override fun createView(context: Context, cometChatListItem: CometChatListItem): View? {
return null
}
override fun bindView(context: Context, view: View, user: User, viewHolder: RecyclerView.ViewHolder, list: List<User>, i: Int) {
}
})
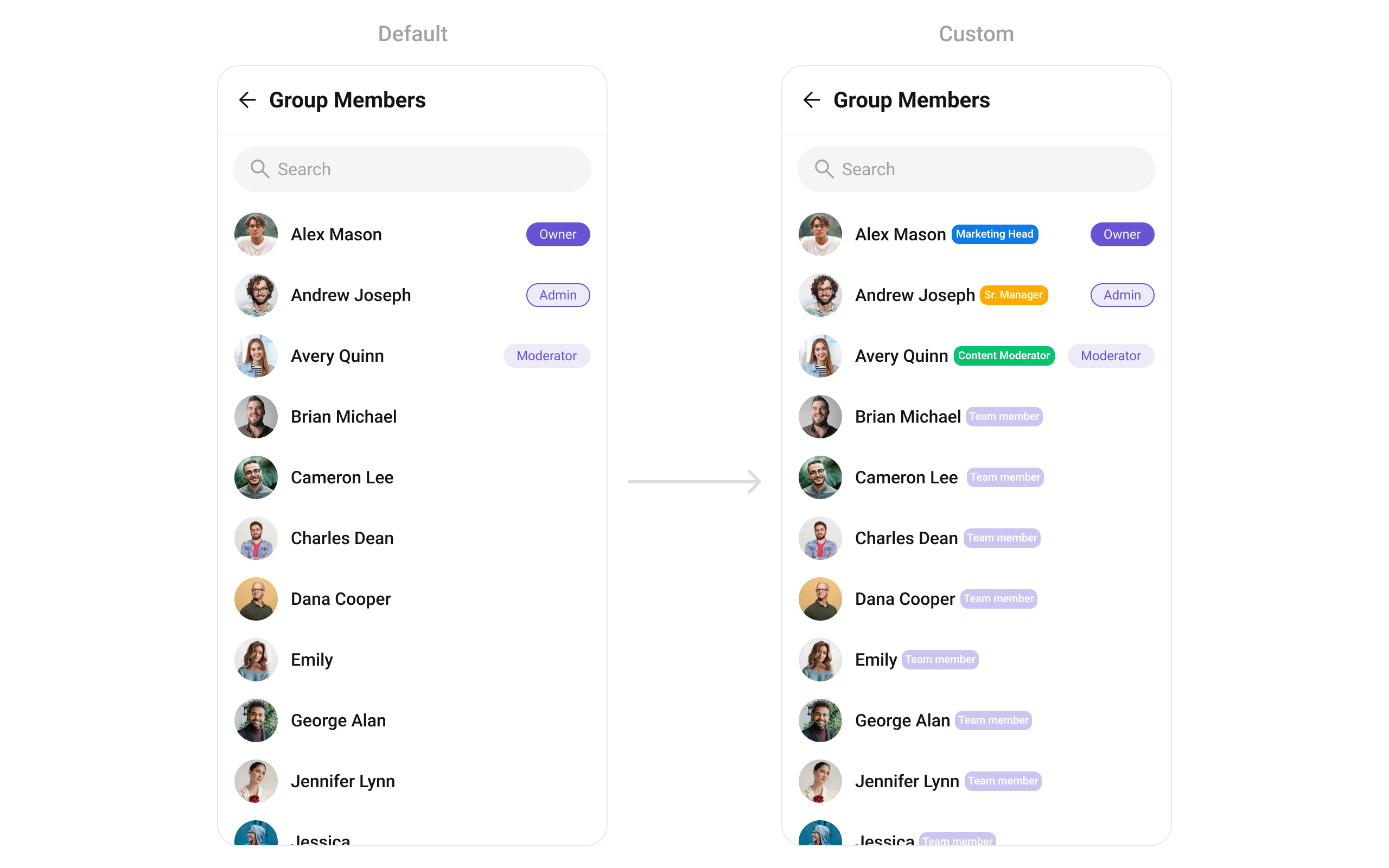
You can indeed create a custom layout file named custom_title_view.xml
for more complex or unique list items.
Once this layout file is made, you would inflate it inside the createView()
method of the GroupsViewHolderListener
. The inflation process prepares the layout for use in your application:
Following this, you would use the bindView()
method to initialize and assign values to your individual views. This could include setting text on TextViews, images on ImageViews, and so on based on the properties of the Group object:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:id="@+id/user_layout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:gravity="center_vertical"
android:orientation="horizontal">
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_weight="1"
android:text="teacher"
android:textAppearance="?attr/cometchatTextAppearanceHeading4Medium"
android:textColor="?attr/cometchatTextColorPrimary" />
<View
android:id="@+id/role"
android:layout_width="50dp"
android:layout_height="15dp"
android:layout_marginStart="@dimen/cometchat_16dp"
android:background="@drawable/team_member" />
</LinearLayout>
- Java
- Kotlin
cometchatGroupMembers.setTitleView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometchatGroupMemberListItemBinding listItem) {
return LayoutInflater.from(context).inflate(R.layout.custom_title_view, null, false);
}
@Override
public void bindView(Context context, View createdView, GroupMember groupMember, Group group, RecyclerView.ViewHolder holder, List<GroupMember> groupMemberList, int position) {
LinearLayout layout = createdView.findViewById(R.id.user_layout);
LinearLayout.LayoutParams layoutParams = new LinearLayout.LayoutParams(ViewGroup.LayoutParams.WRAP_CONTENT,
ViewGroup.LayoutParams.WRAP_CONTENT);
layout.setLayoutParams(layoutParams);
TextView name = createdView.findViewById(R.id.title);
name.setText(groupMember.getName());
View role = createdView.findViewById(R.id.role);
if (UIKitConstants.GroupMemberScope.ADMIN.equals(groupMember.getScope())) {
role.setVisibility(View.VISIBLE);
role.setBackground(ResourcesCompat.getDrawable(getResources(), R.drawable.marketing_head, null));
} else if (UIKitConstants.GroupMemberScope.MODERATOR.equals(groupMember.getScope())) {
role.setVisibility(View.VISIBLE);
role.setBackground(ResourcesCompat.getDrawable(getResources(), R.drawable.sr_manager, null));
} else if (UIKitConstants.GroupMemberScope.PARTICIPANTS.equals(groupMember.getScope())) {
role.setVisibility(View.VISIBLE);
role.setBackground(ResourcesCompat.getDrawable(getResources(), R.drawable.content_manager, null));
} else {
role.setBackground(ResourcesCompat.getDrawable(getResources(), R.drawable.team_member, null));
}
}
});
cometchatGroupMembers.setTitleView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context?, listItem: CometchatGroupMemberListItemBinding?): View {
return LayoutInflater.from(context).inflate(R.layout.custom_title_view, null, false)
}
override fun bindView(
context: Context,
createdView: View,
groupMember: GroupMember,
group: Group,
holder: RecyclerView.ViewHolder,
groupMemberList: List<GroupMember>,
position: Int
) {
val layout = createdView.findViewById<LinearLayout>(R.id.user_layout)
val layoutParams = LinearLayout.LayoutParams(
ViewGroup.LayoutParams.WRAP_CONTENT,
ViewGroup.LayoutParams.WRAP_CONTENT
)
layout.layoutParams = layoutParams
val name = createdView.findViewById<TextView>(R.id.title)
name.text = groupMember.name
val role = createdView.findViewById<View>(R.id.role)
if (UIKitConstants.GroupMemberScope.ADMIN == groupMember.scope) {
role.visibility = View.VISIBLE
role.background = ResourcesCompat.getDrawable(resources, R.drawable.marketing_head, null)
} else if (UIKitConstants.GroupMemberScope.MODERATOR == groupMember.scope) {
role.visibility = View.VISIBLE
role.background = ResourcesCompat.getDrawable(resources, R.drawable.sr_manager, null)
} else if (UIKitConstants.GroupMemberScope.PARTICIPANTS == groupMember.scope) {
role.visibility = View.VISIBLE
role.background = ResourcesCompat.getDrawable(resources, R.drawable.content_manager, null)
} else {
role.background = ResourcesCompat.getDrawable(resources, R.drawable.team_member, null)
}
}
})
setItemView
Assigns a fully custom ListItem layout to the Group Members Component, replacing the default structure.
Use Cases:
- Include additional member details like joined date, bio, or status.
- Modify layout based on user roles.
- Java
- Kotlin
cometchatGroupMembers.setItemView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometchatGroupMemberListItemBinding cometChatListItem) {
return null;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
}
});
cometchatGroupMembers.setItemView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometchatGroupMemberListItemBinding): View? {
return null
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
}
})
Example
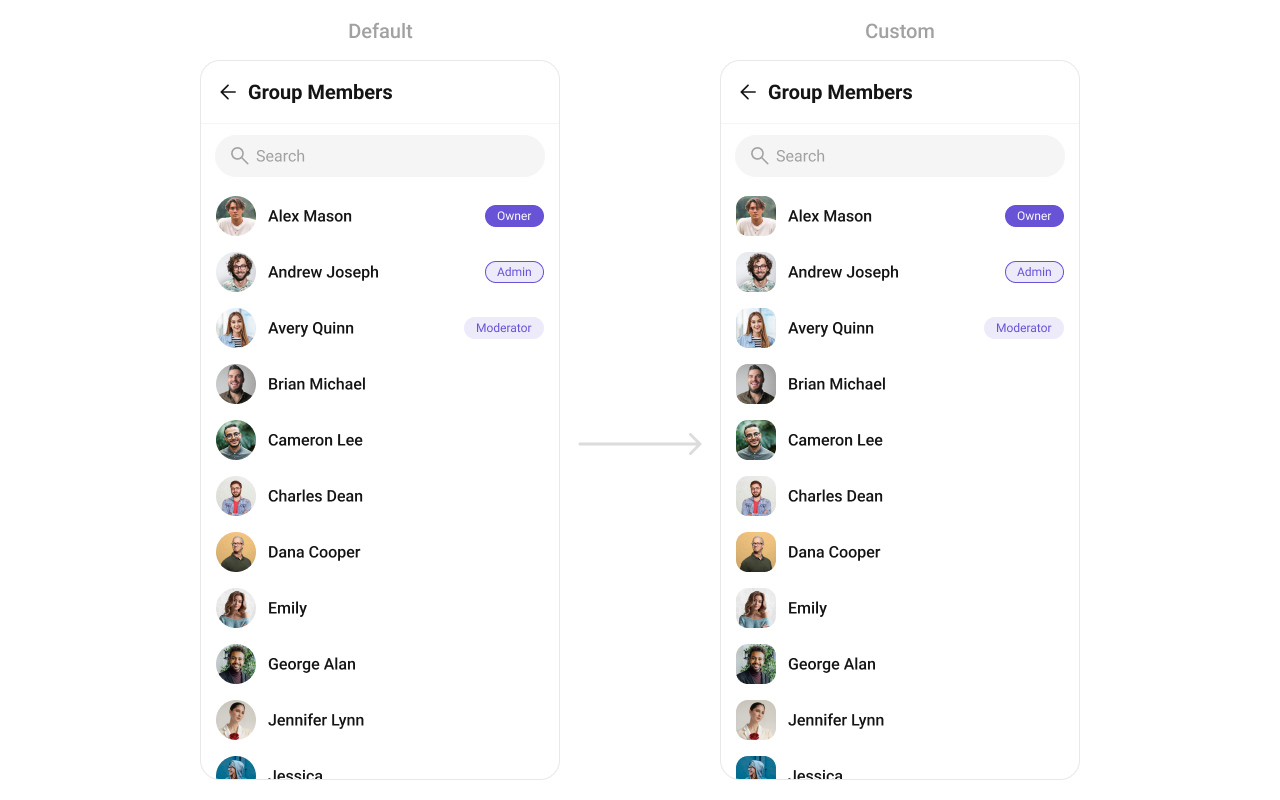
You can indeed create a custom layout file named item_list.xml
for more complex or unique list items.
Once this layout file is made, you would inflate it inside the createView()
method of the GroupsViewHolderListener
. The inflation process prepares the layout for use in your application:
Following this, you would use the bindView()
method to initialize and assign values to your individual views. This could include setting text on TextViews, images on ImageViews, and so on based on the properties of the Group object:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:id="@+id/tvName"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:maxLines="1"
android:textAppearance="?attr/cometchatTextAppearanceHeading4Bold"
android:textColor="?attr/cometchatTextColorPrimary" />
<TextView
android:id="@+id/tvSubtitle"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginTop="@dimen/cometchat_margin_1"
android:textAppearance="?attr/cometchatTextAppearanceBodyRegular"
android:textColor="?attr/cometchatTextColorSecondary" />
</LinearLayout>
- Java
- Kotlin
cometchatGroupMembers.setItemView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometchatGroupMemberListItemBinding listItem) {
return View.inflate(context, R.layout.custom_group_list_item, null);
}
@Override
public void bindView(Context context, View createdView, GroupMember groupMember, Group group, RecyclerView.ViewHolder holder, List<GroupMember> groupMemberList, int position) {
TextView tvName = createdView.findViewById(R.id.tvName);
TextView tvScope = createdView.findViewById(R.id.tvSubtitle);
tvName.setText(groupMember.getName());
tvScope.setText(groupMember.getScope());
}
});
cometchatGroupMembers.setItemView(object :
GroupMembersViewHolderListeners() {
override fun createView(
context: Context?,
listItem: CometchatGroupMemberListItemBinding?
): View {
return View.inflate(context, R.layout.custom_group_list_item, null)
}
override fun bindView(
context: Context,
createdView: View,
groupMember: GroupMember,
group: Group,
holder: RecyclerView.ViewHolder,
groupMemberList: List<GroupMember>,
position: Int
) {
val tvName = createdView.findViewById<TextView>(R.id.tvName)
val tvScope = createdView.findViewById<TextView>(R.id.tvSubtitle)
tvName.text = groupMember.name
tvScope.text = groupMember.scope
}
})
setSubTitleView
Customizes the subtitle view for each group member, typically used for extra details.
Use Cases:
- Show "Last Active" time.
- Display a custom status message.
- Java
- Kotlin
cometchatGroupMembers.setSubtitleView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometchatGroupMemberListItemBinding cometChatListItem) {
return null;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
}
});
cometchatGroupMembers.setSubtitleView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometchatGroupMemberListItemBinding?): View? {
return null
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
}
})
Example
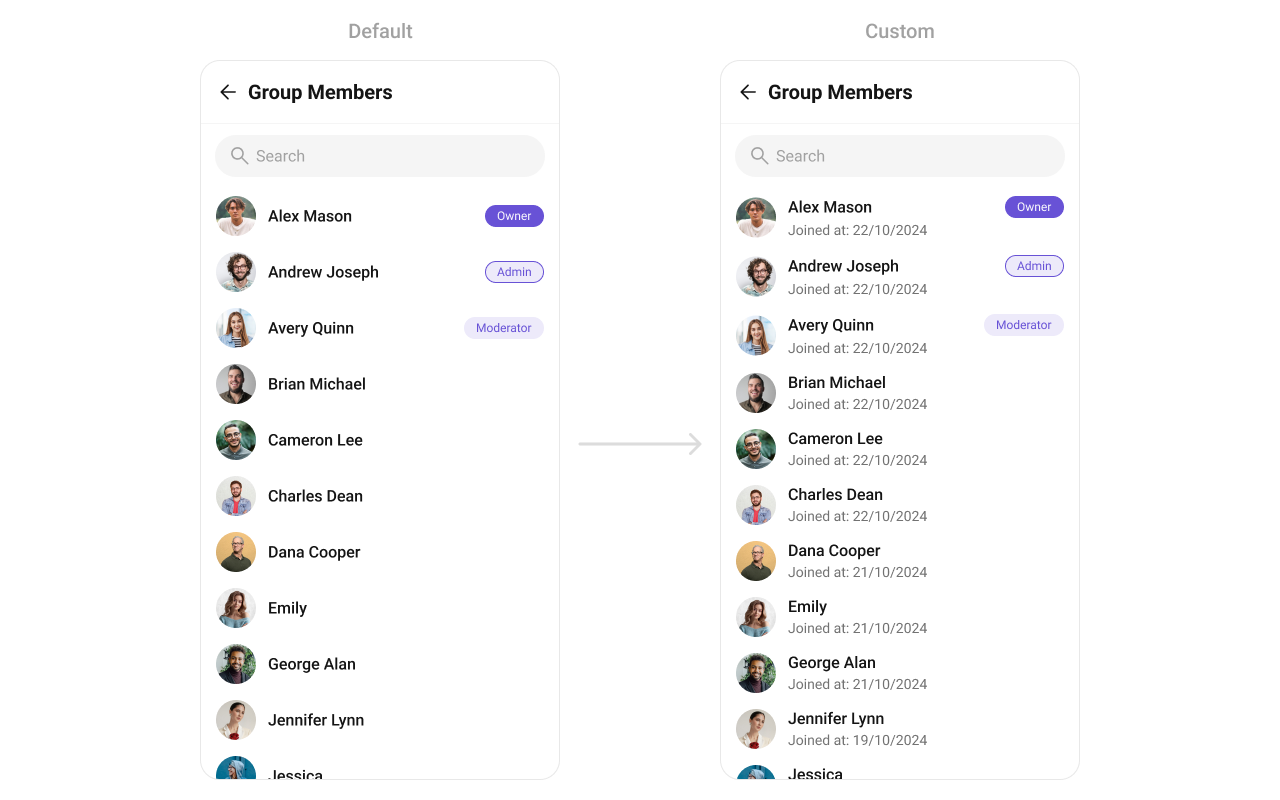
You can indeed create a custom layout file named subtitle_layout.xml
for more complex or unique list items.
Once this layout file is made, you would inflate it inside the createView()
method of the GroupsViewHolderListener
. The inflation process prepares the layout for use in your application:
Following this, you would use the bindView()
method to initialize and assign values to your individual views. This could include setting text on TextViews, images on ImageViews, and so on based on the properties of the Group object:
- Java
- Kotlin
cometchatGroupMembers.setSubtitleView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometchatGroupMemberListItemBinding listItem) {
return new TextView(context);
}
@Override
public void bindView(Context context, View createdView, GroupMember groupMember, Group group, RecyclerView.ViewHolder holder, List<GroupMember> groupMemberList, int position) {
((TextView) createdView).setText("Joined at: "+new SimpleDateFormat("dd/mm/yyyy").format(groupMember.getJoinedAt()*1000));
}
});
cometchatGroupMembers.setSubtitleView(object :
GroupMembersViewHolderListeners() {
override fun createView(
context: Context?,
listItem: CometchatGroupMemberListItemBinding?
): View {
return TextView(context)
}
override fun bindView(
context: Context,
createdView: View,
groupMember: GroupMember,
group: Group,
holder: RecyclerView.ViewHolder,
groupMemberList: List<GroupMember>,
position: Int
) {
(createdView as TextView).text =
"Joined at: " + SimpleDateFormat("dd/mm/yyyy").format(groupMember.joinedAt * 1000)
}
})
setTrailingView
Customizes the trailing (right-end) section of each member item, typically used for action buttons.
Use Cases:
- Show quick actions like Mute, Remove, or Promote.
- Display a "Last Active" timestamp.
- Java
- Kotlin
cometchatGroupMembers.setTrailingView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometchatGroupMemberListItemBinding cometChatListItem) {
return null;
}
@Override
public void bindView(Context context, View view, GroupMember groupMember, Group group, RecyclerView.ViewHolder viewHolder, List<GroupMember> list, int i) {
}
});
cometchatGroupMembers.setTrailingView(object : GroupMembersViewHolderListeners() {
override fun createView(context: Context, cometChatListItem: CometchatGroupMemberListItemBinding?): View? {
return null
}
override fun bindView(
context: Context,
view: View,
groupMember: GroupMember,
group: Group,
viewHolder: RecyclerView.ViewHolder,
list: List<GroupMember>,
i: Int
) {
}
})
Example
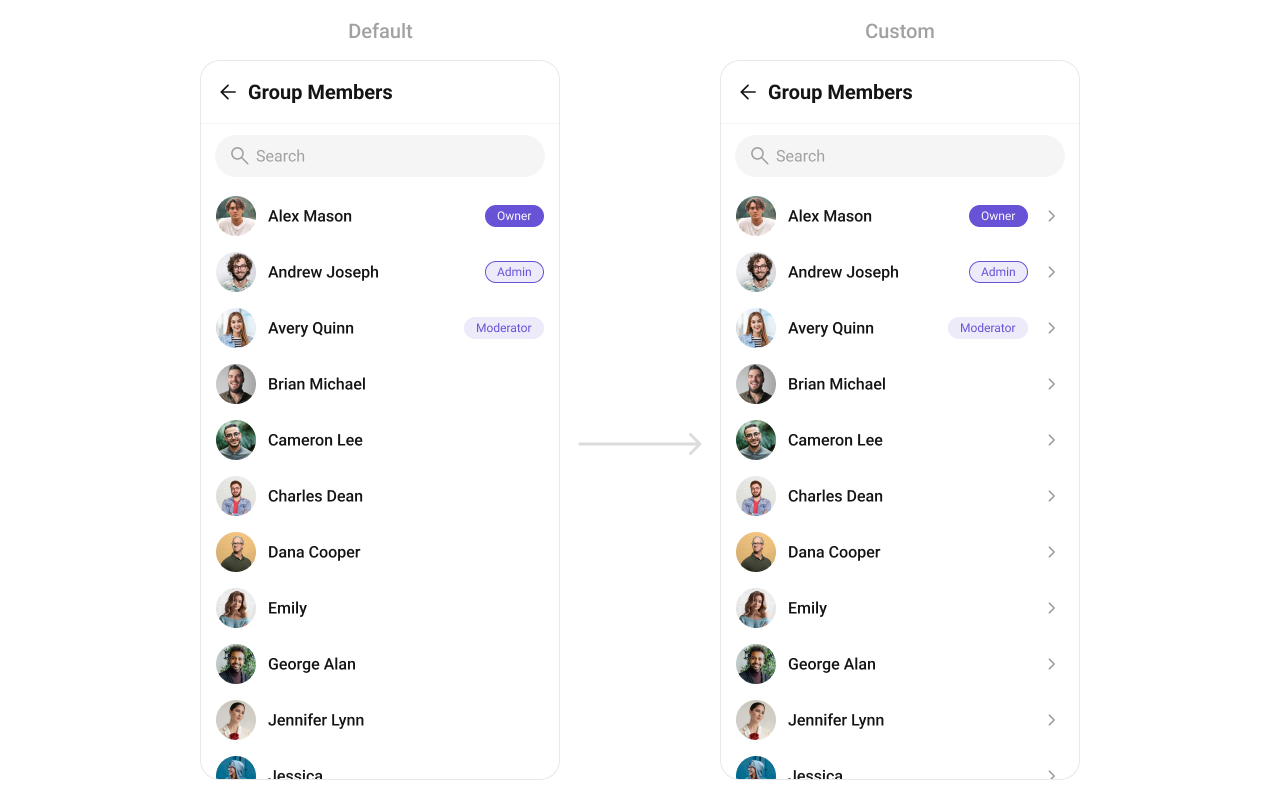
You can indeed create a custom layout file named tail_view_layout.xml
for more complex or unique list items.
Once this layout file is made, you would inflate it inside the createView()
method of the GroupsViewHolderListener
. The inflation process prepares the layout for use in your application:
Following this, you would use the bindView()
method to initialize and assign values to your individual views. This could include setting text on TextViews, images on ImageViews, and so on based on the properties of the Group object:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="wrap_content"
android:layout_height="wrap_content">
<com.google.android.material.card.MaterialCardView
android:id="@+id/scope_card"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginStart="16dp"
app:cardBackgroundColor="?attr/cometchatPrimaryColor"
app:cardCornerRadius="@dimen/cometchat_radius_max"
app:cardElevation="@dimen/cometchat_0dp"
>
<TextView
android:id="@+id/tv_scope"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_gravity="center"
android:paddingStart="@dimen/cometchat_padding_3"
android:paddingTop="@dimen/cometchat_padding_1"
android:paddingEnd="@dimen/cometchat_padding_3"
android:paddingBottom="@dimen/cometchat_padding_1"
android:text="@string/cometchat_owner"
android:textAppearance="?attr/cometchatTextAppearanceCaption1Regular"
android:textColor="?attr/cometchatPrimaryColor" />
</com.google.android.material.card.MaterialCardView>
</LinearLayout>
- Java
- Kotlin
cometchatGroupMembers.setTrailingView(new GroupMembersViewHolderListeners() {
@Override
public View createView(Context context, CometchatGroupMemberListItemBinding listItem) {
return View.inflate(context, R.layout.custom_tail_view, null);
}
@Override
public void bindView(Context context, View createdView, GroupMember groupMember, Group group, RecyclerView.ViewHolder holder, List<GroupMember> groupMemberList, int position) {
MaterialCardView cardView = createdView.findViewById(R.id.scope_card);
TextView tvName = createdView.findViewById(R.id.tv_scope);
cardView.setCardBackgroundColor(CometChatTheme.getExtendedPrimaryColor100(context));
tvName.setText(groupMember.getScope());
createdView.setVisibility(groupMember.getScope().equals(UIKitConstants.GroupMemberScope.PARTICIPANTS) ? View.VISIBLE : View.GONE);
}
});
cometchatGroupMembers.setTrailingView(object :
GroupMembersViewHolderListeners() {
override fun createView(
context: Context?,
listItem: CometchatGroupMemberListItemBinding?
): View {
return View.inflate(context, R.layout.custom_tail_view, null)
}
override fun bindView(
context: Context,
createdView: View,
groupMember: GroupMember,
group: Group,
holder: RecyclerView.ViewHolder,
groupMemberList: List<GroupMember>,
position: Int
) {
val cardView = createdView.findViewById<MaterialCardView>(R.id.scope_card)
val tvName = createdView.findViewById<TextView>(R.id.tv_scope)
cardView.setCardBackgroundColor(CometChatTheme.getExtendedPrimaryColor100(context))
tvName.text = groupMember.scope
createdView.visibility =
if (groupMember.scope == UIKitConstants.GroupMemberScope.PARTICIPANTS) View.VISIBLE else View.GONE
}
})
setOverflowMenu
Allows customization of the overflow menu (three-dot ⋮ icon) with additional options.
Use Cases:
- Add extra actions like "Report Member", "Restrict from Posting".
- Provide group admins with moderation options.
- Java
- Kotlin
cometchatGroupMembers.setOverflowMenu(v);
cometchatGroupMembers.setOverflowMenu(v)
Example:
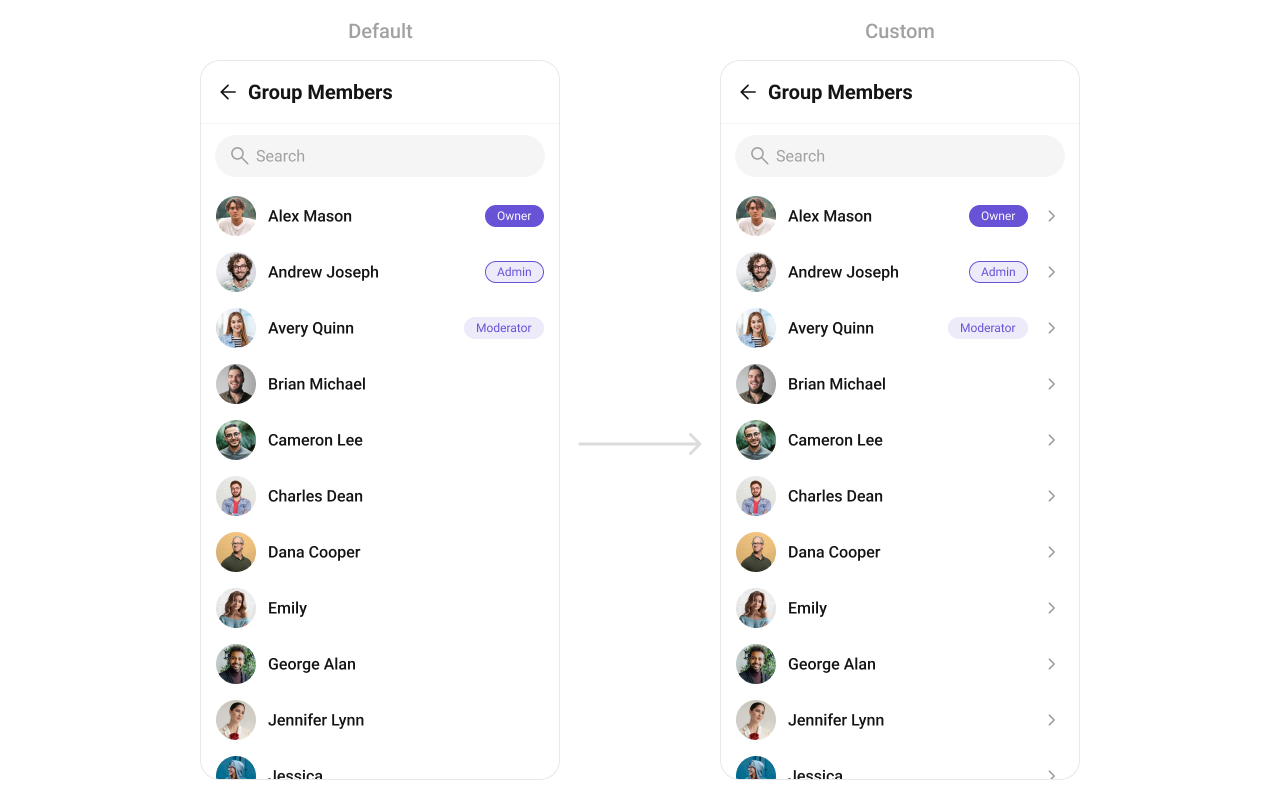
- Java
- Kotlin
ImageView addMemberIv = new ImageView(this);
addMemberIv.setImageDrawable(ResourcesCompat.getDrawable(getResources(), R.drawable.ic_add, null));
cometchatGroupMembers.setOverflowMenu(addMemberIv);
val addMemberIv = ImageView(this)
addMemberIv.setImageDrawable(ResourcesCompat.getDrawable(getResources(), R.drawable.ic_add, null))
cometchatGroupMembers.setOverflowMenu(addMemberIv)