Group Members
Overview
CometChatGroupMembers
is a versatile Widget designed to showcase all users who are either added to or invited to a group, thereby enabling them to participate in group discussions, access shared content, and engage in collaborative activities. CometChatGroupMembers
have the capability to communicate in real-time through messaging, voice and video calls, and various other interactions. Additionally, they can interact with each other, share files, and join calls based on the permissions established by the group administrator or owner.
- Android
- iOS
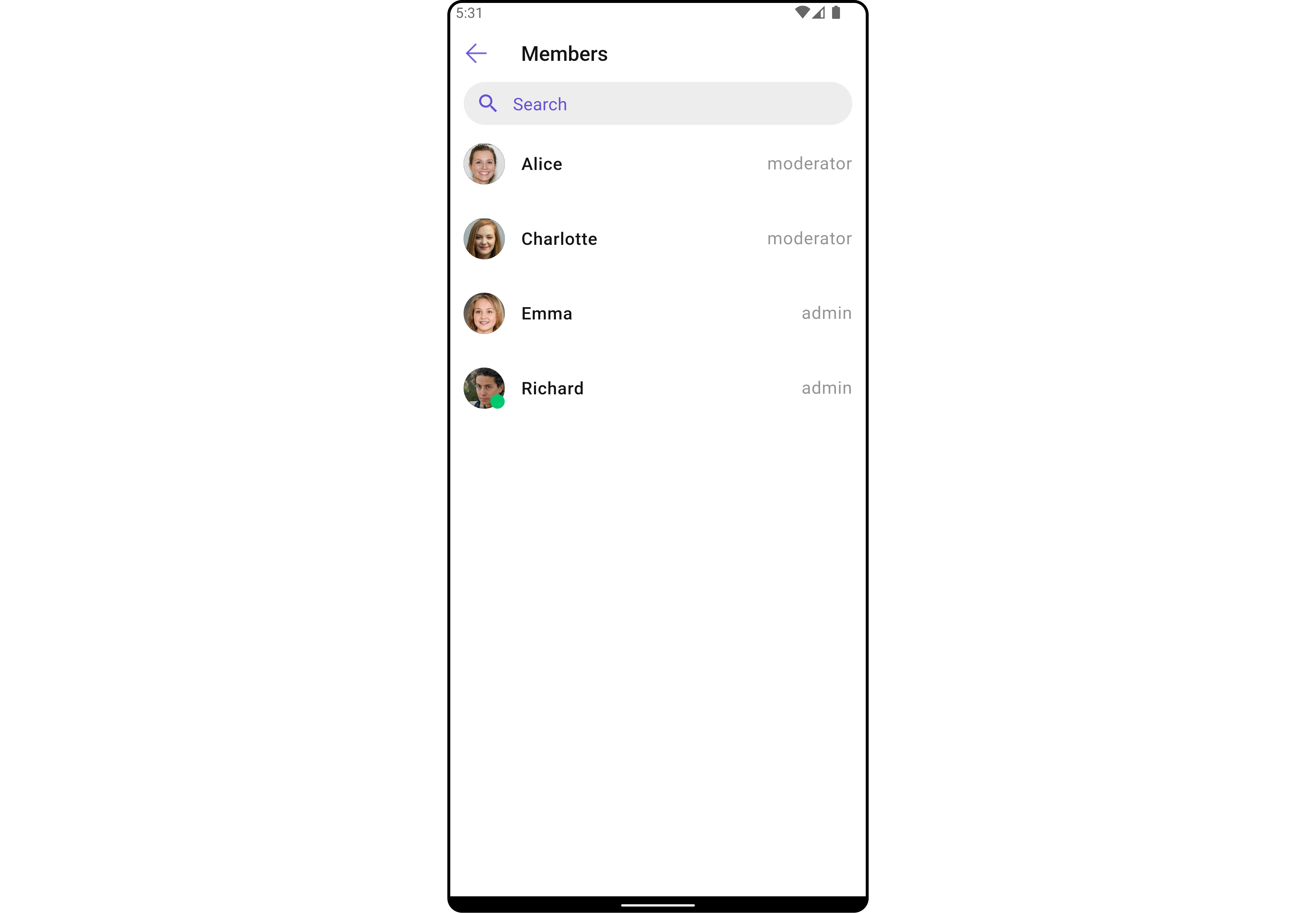
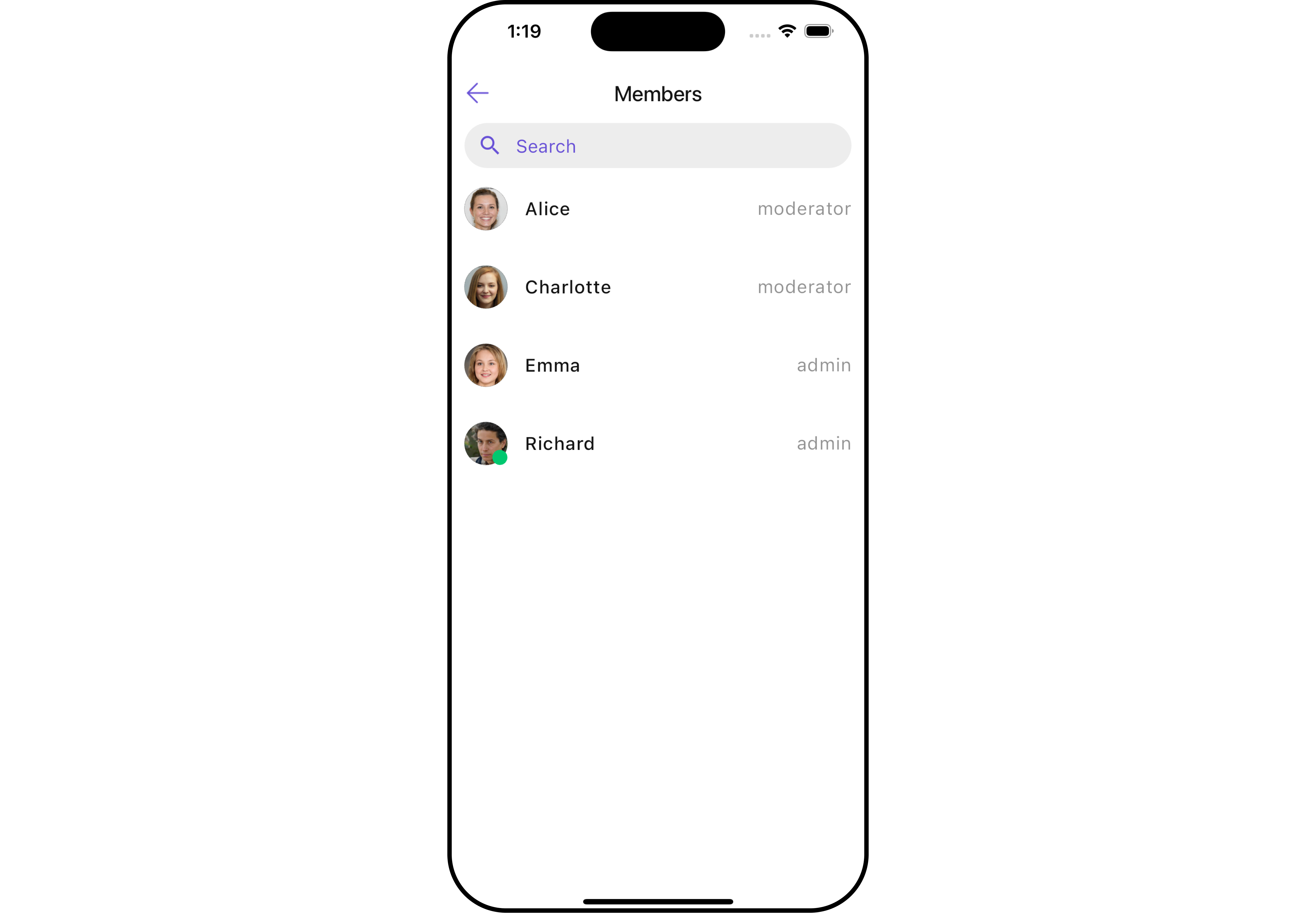
The CometChatGroupMembers
widget is composed of the following BaseWidgets:
Widgets | Description |
---|---|
CometChatListBase | CometChatListBase serves as a container widget equipped with a title (navigationBar), search functionality (search-bar), background settings, and a container for embedding a list widget. |
CometChatListItem | This widget renders information extracted from a User object onto a tile, featuring a title, subtitle, leading widget, and trailing widget. experience, facilitating seamless navigation and interaction within the widget. |
Usage
Integration
CometChatGroupMembers
, as a Composite Widget, offers flexible integration options, allowing it to be launched directly via button clicks or any user-triggered action.
You can launch CometChatGroupMembers
directly using Navigator.push
, or you can define it as a widget within the build
method of your State
class.
1. Using Navigator to Launch CometChatGroupMembers
- Dart
Navigator.push(context, MaterialPageRoute(builder: (context) => CometChatGroupMembers(group: Group(guid: "", name: "", type: "")))); // A group object is required to launch this widget.
2. Embedding CometChatGroupMembers
as a Widget in the build Method
- Dart
import 'package:cometchat_chat_uikit/cometchat_chat_uikit.dart';
import 'package:flutter/material.dart';
class GroupMembers extends StatefulWidget {
const GroupMembers({super.key});
State<GroupMembers> createState() => _GroupMembersState();
}
class _GroupMembersState extends State<GroupMembers> {
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""),
) // A group object is required to launch this widget.
)
);
}
}
Actions
Actions dictate how a widget functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the widget to fit your specific needs.
1. onItemTap
This method proves valuable when users seek to override onItemClick functionality within CometChatGroupMembers
, empowering them with greater control and customization options.
The onItemTap
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
onItemTap: (groupMember) {
// TODO("Not yet implemented")
},
)
2. onItemLongPress
This method becomes invaluable when users seek to override long-click functionality within CometChatGroupMembers
, offering them enhanced control and flexibility in their interactions.
The onItemLongPress
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
onItemLongPress: (groupMember) {
// TODO("Not yet implemented")
},
)
3. onBack
Enhance your application's functionality by leveraging the onBack
feature. This capability allows you to customize the behavior associated with navigating back within your app. Utilize the provided code snippet to override default behaviors and tailor the user experience according to your specific requirements.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
onBack: () {
// TODO("Not yet implemented")
},
)
4. onError
You can customize this behavior by using the provided code snippet to override the onError
and improve error handling.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
onError: (e) {
// TODO("Not yet implemented")
},
)
5. onSelection
When the onSelection
event is triggered, it furnishes the list of selected members. This event can be invoked by any button or action within the interface. You have the flexibility to implement custom actions or behaviors based on the selected members.
This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
selectionMode: SelectionMode.multiple,
activateSelection: ActivateSelection.onClick,
onSelection: (groupMembersList) {
// TODO("Not yet implemented")
},
)
Filters
Filters allow you to customize the data displayed in a list within a Widget
. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders
of Chat SDK.
1. GroupMembersRequestBuilder
Property | Description | Code |
---|---|---|
GUID | Group ID for the group whose members are to be fetched. | guid: String |
Limit | Number of results to limit the query. | limit: int? |
Search Keyword | Keyword for searching members within the group. | searchKeyword: String? |
Scopes | List of scopes for filtering members (e.g., moderators). | scopes: List<String>? |
Example
In the example below, we are applying a filter to the Group List based on limit and scope.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
groupMembersRequestBuilder: GroupMembersRequestBuilder("")
..limit = 10
)
2. GroupMembersProtocol
The GroupMembersProtocol
uses GroupsRequestBuilder enables you to filter and customize the search list based on available parameters in GroupsRequestBuilder.
This feature allows you to keep uniformity between the displayed Group Members List and Searched Group Members List.
Here is the complete example for reference:
Example
- Dart
import 'package:cometchat_chat_uikit/cometchat_chat_uikit.dart';
class CustomProtocolBuilder extends GroupMembersBuilderProtocol {
const CustomProtocolBuilder(super.builder);
GroupMembersRequest getRequest() {
return requestBuilder.build();
}
GroupMembersRequest getSearchRequest(String val) {
requestBuilder.searchKeyword = val;
return requestBuilder.build();
}
}
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
groupMembersProtocol: CustomProtocolBuilder(GroupMembersRequestBuilder("")..searchKeyword = "searchKeyword"),
)
Events
Events are emitted by a Widget
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
Events emitted by the Join Group widget is as follows.
Event | Description |
---|---|
ccGroupMemberBanned | Triggers when the group member banned from the group successfully |
ccGroupMemberKicked | Triggers when the group member kicked from the group successfully |
ccGroupMemberScopeChanged | Triggers when the group member scope is changed in the group |
Example
- Dart
import 'package:cometchat_chat_uikit/cometchat_chat_uikit.dart';
import 'package:cometchat_sdk/models/action.dart' as cc;
import 'package:flutter/material.dart';
class YourScreen extends StatefulWidget {
const YourScreen({super.key});
State<YourScreen> createState() => _YourScreenState();
}
class _YourScreenState extends State<YourScreen> with CometChatGroupEventListener {
void initState() {
super.initState();
CometChatGroupEvents.addGroupsListener("listenerId", this); // Add the listener
}
void dispose(){
super.dispose();
CometChatGroupEvents.removeGroupsListener("listenerId"); // Remove the listener
}
void ccGroupMemberScopeChanged(cc.Action message, User updatedUser, String scopeChangedTo, String scopeChangedFrom, Group group) {
// TODO("Not yet implemented")
}
void ccGroupMemberBanned(cc.Action message, User bannedUser, User bannedBy, Group bannedFrom) {
// TODO("Not yet implemented")
}
void ccGroupMemberKicked(cc.Action message, User kickedUser, User kickedBy, Group kickedFrom) {
// TODO("Not yet implemented")
}
Widget build(BuildContext context) {
return const Placeholder();
}
}
Customization
To fit your app's design requirements, you can customize the appearance of the Groups widget. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the widget in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the widget.
1. GroupMembers Style
You can set the GroupMembersStyle
to the CometChatGroupMembers
Widget to customize the styling.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
groupMemberStyle: GroupMembersStyle(
background: Color(0xFFE4EBF5),
titleStyle: TextStyle(color: Colors.red),
backIconTint: Colors.red
),
)
- Android
- iOS
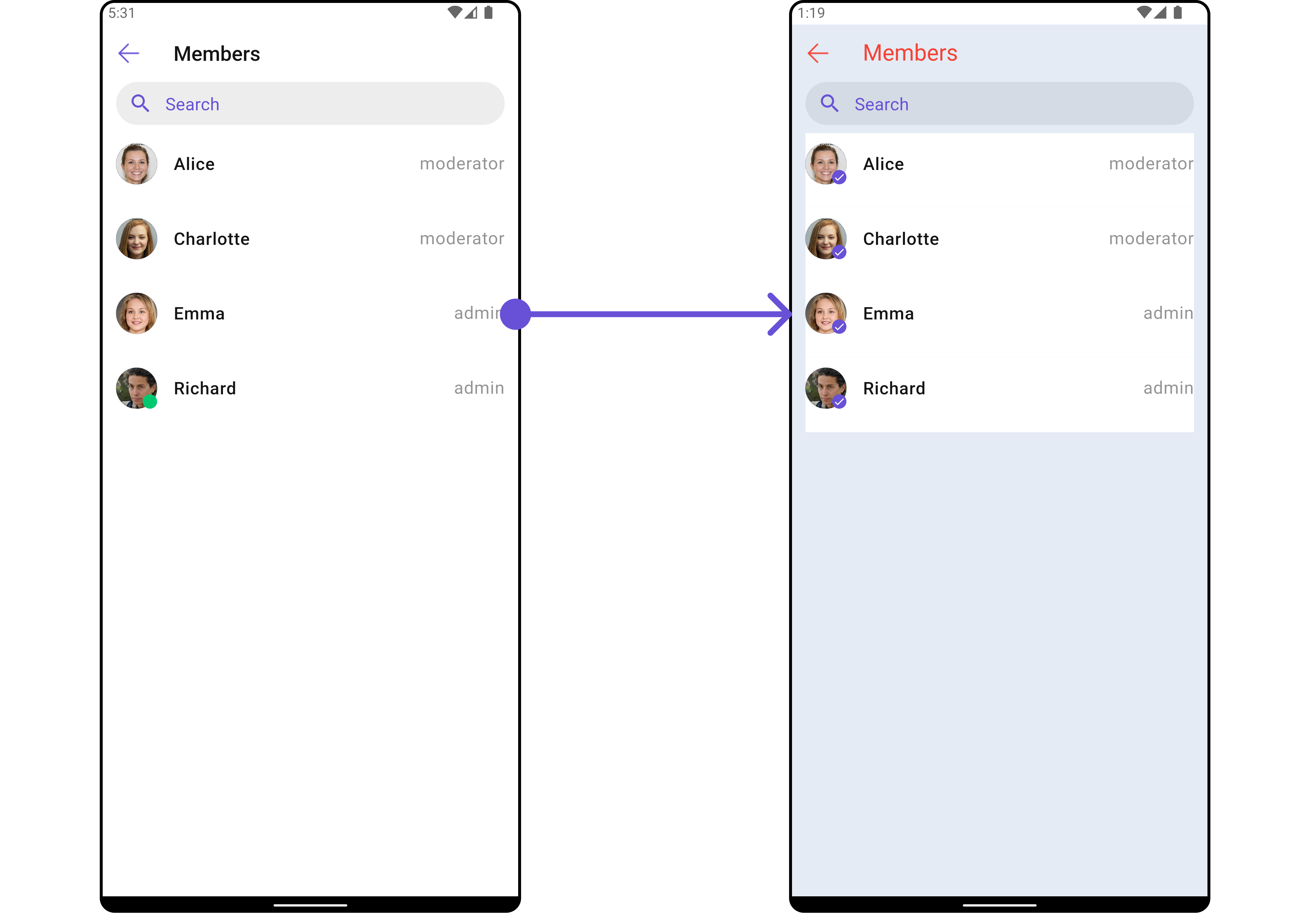
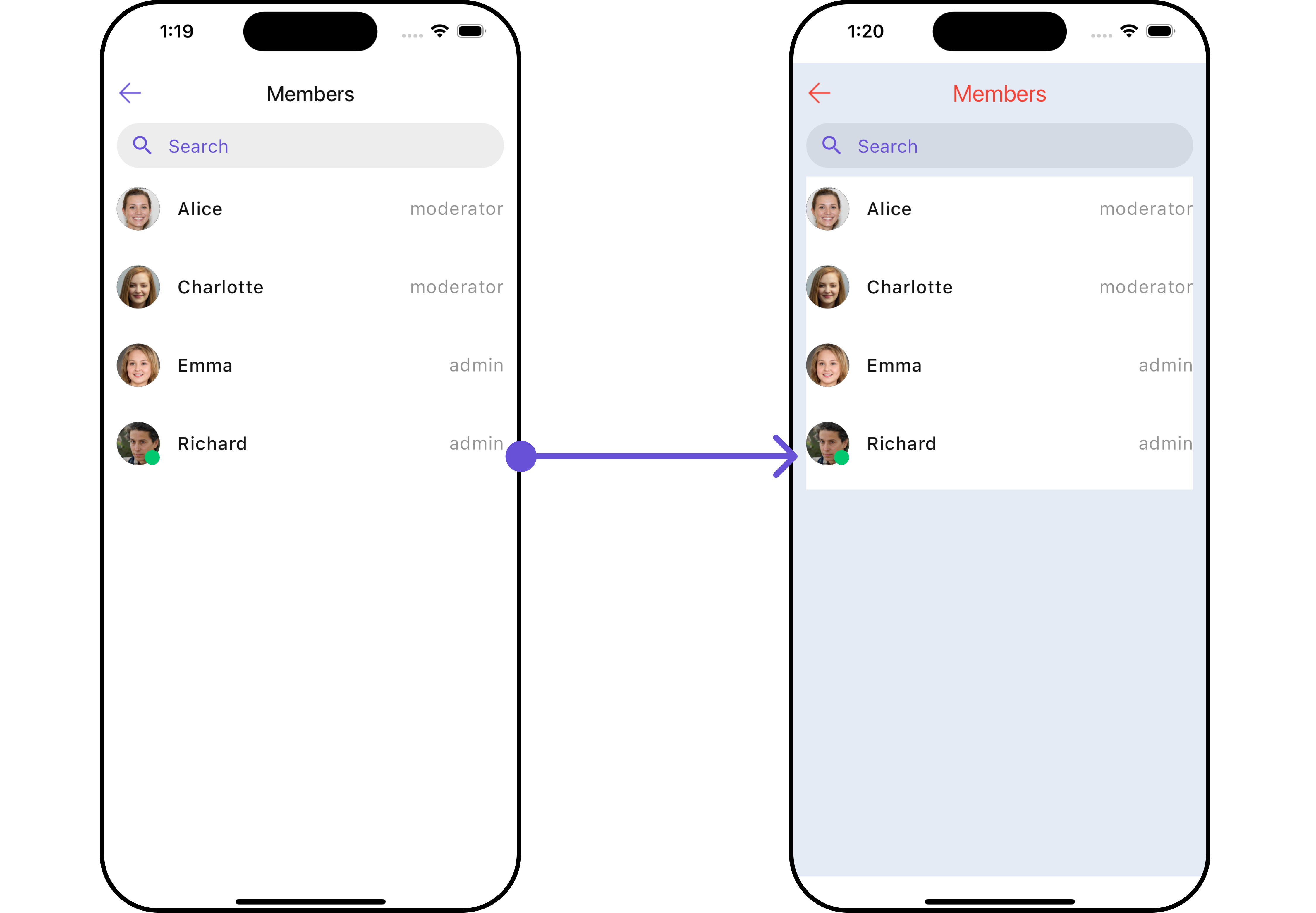
List of properties exposed by GroupMemberStyle
Property | Description | Code |
---|---|---|
Background | Inherits from BaseStyles . Sets the background color or image of the widget. | background: Color? (inherited from BaseStyles ) |
Border | Inherits from BaseStyles . Sets the border of the widget. | border: Border? (inherited from BaseStyles ) |
Border Radius | Inherits from BaseStyles . Sets the border radius of the widget. | borderRadius: BorderRadius? (inherited from BaseStyles ) |
Empty Text Style | Style for the text displayed when the member list is empty. | emptyTextStyle: TextStyle? |
Error Text Style | Style for the text displayed when an error occurs. | errorTextStyle: TextStyle? |
Gradient | Inherits from BaseStyles . Sets a gradient for the background of the widget. | gradient: Gradient? (inherited from BaseStyles ) |
Height | Inherits from BaseStyles . Sets the height of the widget. | height: double? (inherited from BaseStyles ) |
List Padding | Padding applied to the list of group members. | listPadding: EdgeInsets? |
Loading Icon Tint | Tint color for the loading indicator icon. | loadingIconTint: Color? |
Member Scope Text Style | Style applied to the text displaying the member's scope (e.g., moderator). | memberScopeTextStyle: TextStyle? |
Online Status Color | Color for the online status indicator of group members. | onlineStatusColor: Color? |
Search Background | Background color for the search bar. | searchBackground: Color? |
Search Border Color | Color for the border of the search bar. | searchBorderColor: Color? |
Search Border Radius | Border radius applied to the search bar. | searchBorderRadius: BorderRadius? |
Search Icon Tint | Tint color for the search icon. | searchIconTint: Color? |
Search Placeholder Style | Style applied to the placeholder text within the search bar. | searchPlaceholderStyle: TextStyle? |
Search Text Style | Style applied to the text entered in the search bar. | searchTextStyle: TextStyle? |
Separator Color | Color for the separator between group members in the list. | separatorColor: Color? |
Title Style | Style applied to the title text displayed at the top of the widget. | titleStyle: TextStyle? |
Width | Inherits from BaseStyles . Sets the width of the widget. | width: double? (inherited from BaseStyles ) |
2. Avatar Style
To apply customized styles to the Avatar
widget in the CometChatGroupMembers
Widget, you can use the following code snippet. For further insights on Avatar
Styles refer
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
avatarStyle: AvatarStyle(
border: Border.all(width: 5),
borderRadius: 20,
background: Colors.red
),
)
3. StatusIndicator Style
To apply customized styles to the Status Indicator widget in the CometChatGroupMembers
Widget, You can use the following code snippet. For further insights on Status Indicator Styles refer
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
statusIndicatorStyle: StatusIndicatorStyle(
borderRadius: 10,
gradient: LinearGradient(colors: [Colors.red, Colors.orange], begin: Alignment.topLeft, end: Alignment.bottomRight)
),
)
4. ListItem Style
To apply customized styles to the List Item
widget in the CometChatGroupMembers
Widget, you can use the following code snippet. For further insights on List Item
Styles refer
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
hideSeparator: true,
listItemStyle: ListItemStyle(
background: Color(0xFFE4EBF5),
borderRadius: 20,
border: Border.all(width: 2),
margin: EdgeInsets.only(top: 10),
padding: EdgeInsets.only(left: 10)
),
)
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the widget. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
title: "Your Title",
hideSeparator: true,
hideSearch: true,
showBackButton: false
)
- Android
- iOS
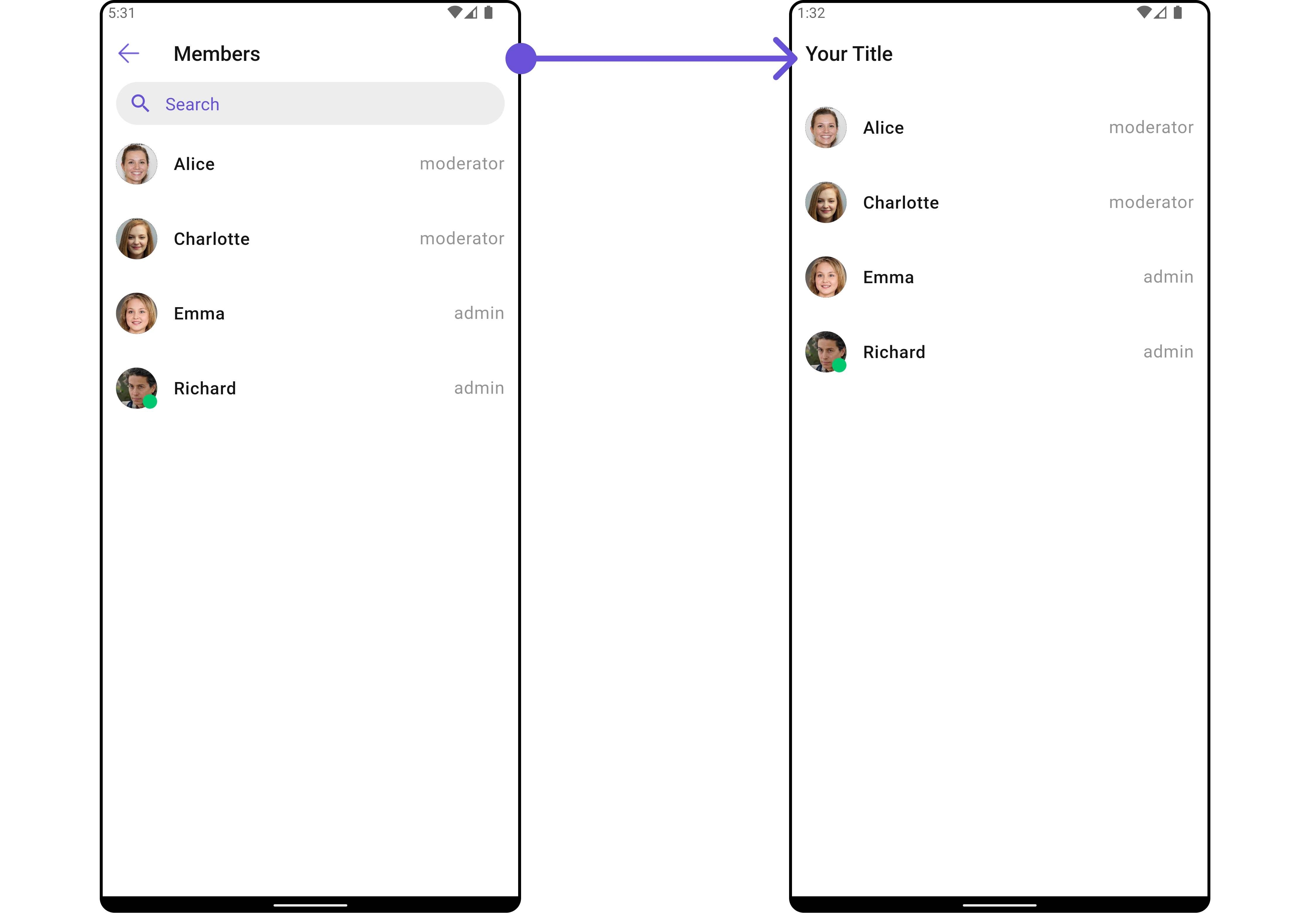
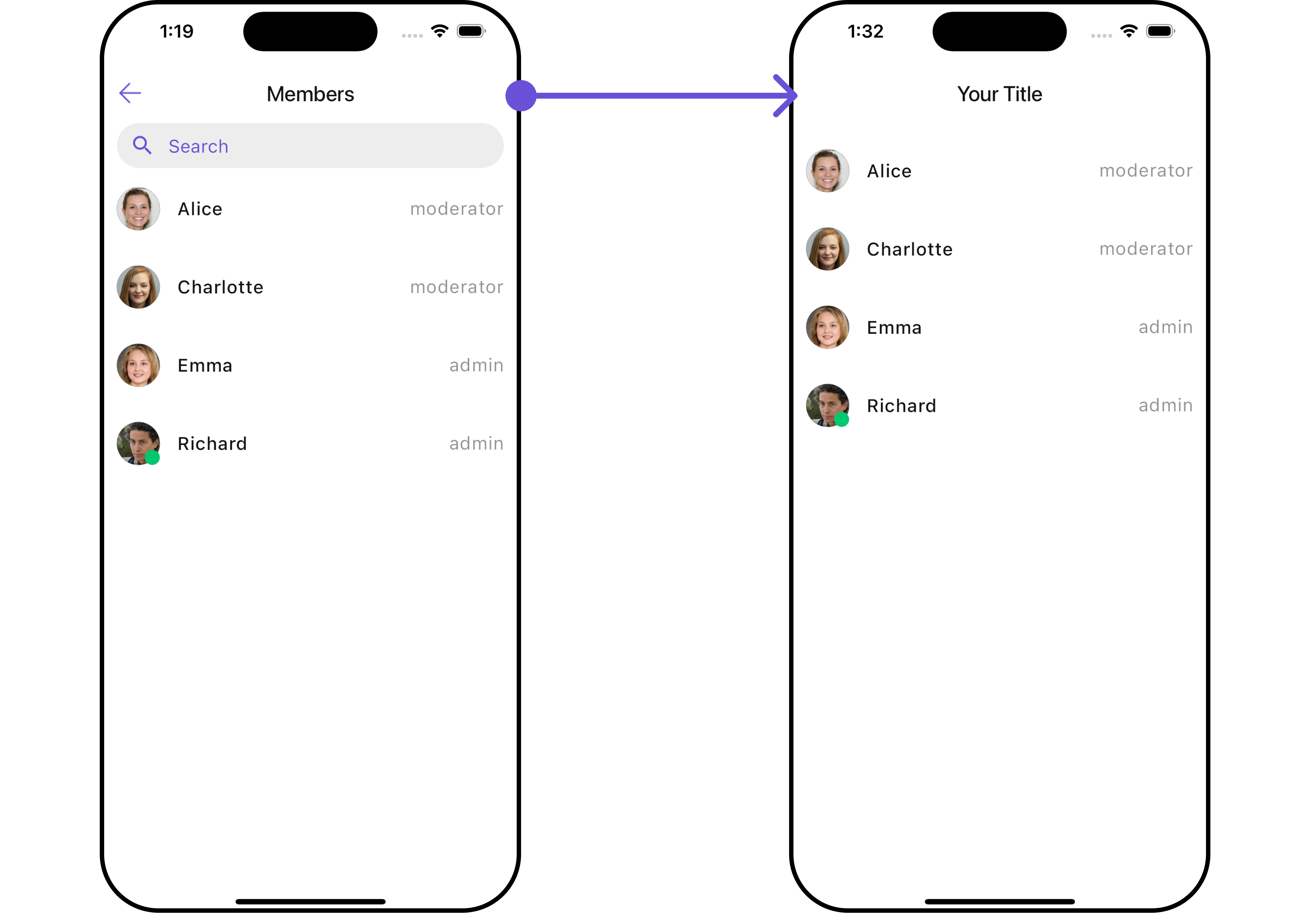
List of properties exposed by CometChatGroupMembers
Property | Description | Code |
---|---|---|
Back Button | A custom widget to replace the default back button. | backButton: Widget? |
Hide Search | Hides the search bar for filtering group members (default: false). | hideSearch: bool (default: false) |
Hide Separator | Hides the separator between group members in the list. | hideSeparator: bool |
Search Box Icon | A custom icon to display in the search bar. | searchBoxIcon: Widget? |
Search Placeholder | Text displayed as a placeholder in the search bar. | searchPlaceholder: String? |
Selection Mode | Mode for selecting group members (e.g., single, multiple). | selectionMode: CometChatSelectionMode? |
Show Back Button | A boolean value indicating whether to show the back button (default: true). | showBackButton: bool (default: true) |
Empty State Text | Text displayed when the group member list is empty. | emptyStateText: String? |
Error State Text | Text displayed when an error occurs while fetching group members. | errorStateText: String? |
Hide Error | Hides the error state view when an error occurs. | hideError: bool |
Title | Title displayed at the top of the widget. | title: String? |
Disable Users Presence | A boolean value indicating whether to disable displaying the presence of group members (default: false). | disableUsersPresence: bool (default: false) |
Select Icon | A custom icon to display for the selection mode. | this.selectIcon: Widget? |
Submit Icon | A custom icon to display for the submit button. | this.submitIcon: Widget? |
Advanced
For advanced-level customization, you can set custom widgets to the widget. This lets you tailor each aspect of the widget to fit your exact needs and application aesthetics. You can create and define your own widgets and then incorporate those into the widget.
The CometChatGroupMembers
widget does not provide additional functionalities beyond this level of customization.
ListItemView
With this function, you can assign a custom ListItem to the CometChatGroupMembers
Widget.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
listItemView: (groupMember) {
return Placeholder(); // Replace this placeholder with your custom widget.
},
)
Here is the complete example for reference:
Example
You can indeed create a custom widget named custom_list_item.dart
for more complex or unique list items.
- Dart
import 'package:flutter/material.dart';
import 'package:intl/intl.dart';
import '../helper/utils/custom_colors.dart';
class CustomListItems extends StatelessWidget {
final String name;
final DateTime? lastMessageTime;
final String? avatarUrl;
const CustomListItems({
super.key,
required this.name,
this.lastMessageTime,
this.avatarUrl,
});
String formatDateTime(DateTime dateTime) {
final now = DateTime.now();
final difference = now.difference(dateTime);
if (difference.inDays == 0) {
return DateFormat('HH:mm').format(dateTime);
} else if (difference.inDays == 1) {
return 'Yesterday';
} else {
return DateFormat('yyyy-MM-dd').format(dateTime);
}
}
Widget build(BuildContext context) {
return Container(
margin: const EdgeInsets.only(top: 5, bottom: 5),
padding: const EdgeInsets.all(8.0),
decoration: BoxDecoration(
border: Border.all(color: Color(0xFF6851D6), width: 1), // Example border color
borderRadius: BorderRadius.circular(8.0),
color: Color(0xFFEEEEEE)
),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
Expanded(
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
name,
style: const TextStyle(
fontWeight: FontWeight.bold,
),
),
lastMessageTime == null ? Container() : Text(formatDateTime(lastMessageTime!)),
],
),
),
const SizedBox(width: 8.0),
if (avatarUrl != null)
ClipOval(
child: Image.network(
avatarUrl!,
width: 40.0,
height: 40.0,
fit: BoxFit.cover,
errorBuilder: (context, error, stackTrace) {
return const Icon(
Icons.person,
size: 40.0,
);
},
),
)
else
const Icon(
Icons.person,
size: 40.0,
),
],
),
);
}
}
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
listItemView: (groupMember) {
return CustomListItems(
name: groupMember.name,
avatarUrl: groupMember.avatar,
); // Replaced Placeholder with CustomListItems widget.
},
)
- Android
- iOS
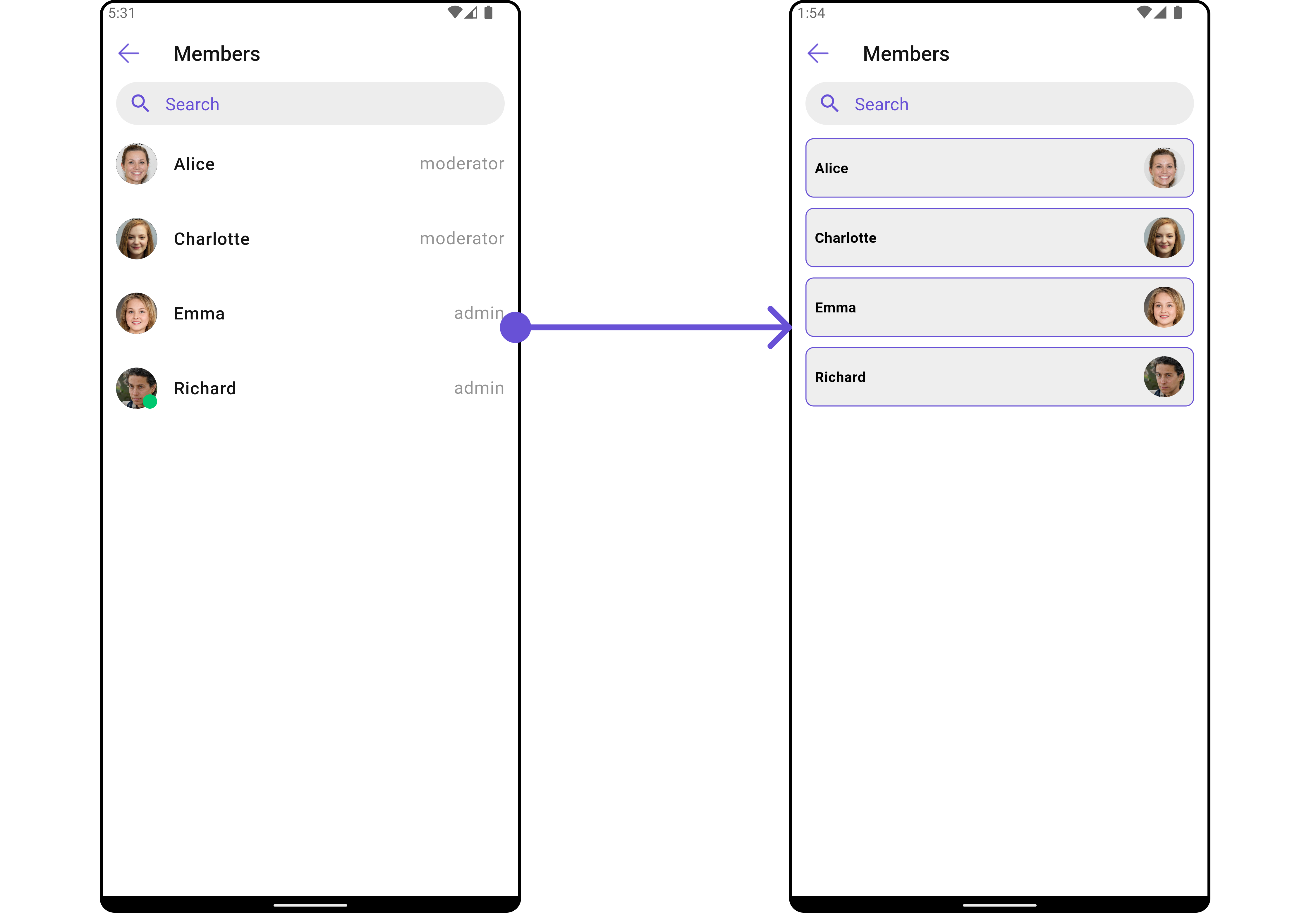
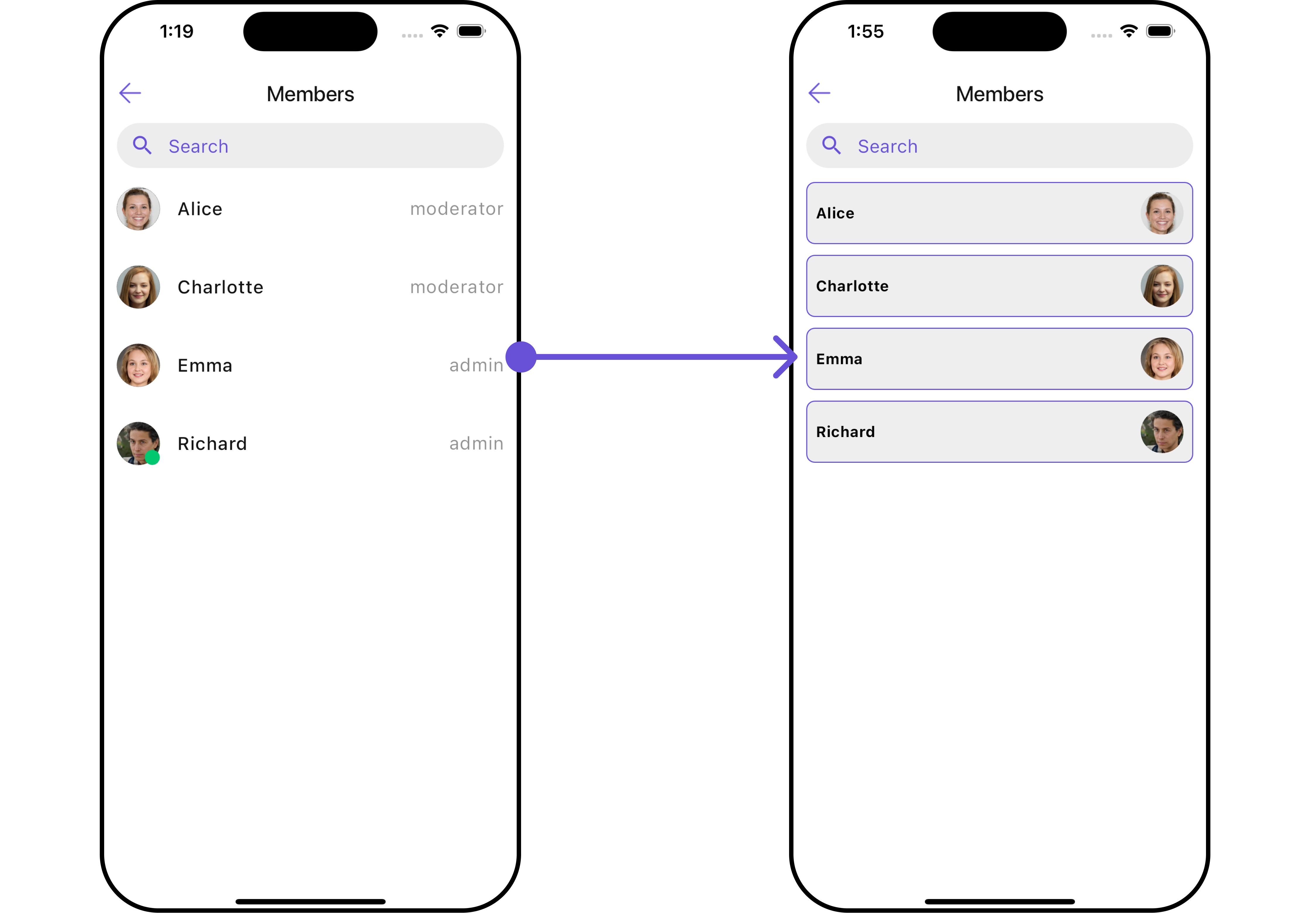
SubtitleView
You can customize the subtitle view for each item to meet your specific preferences and needs.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
subtitleView: (context, groupMember) {
return Placeholder(); // Replace this placeholder with your custom widget.
},
)
Here is the complete example for reference:
Example
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
subtitleView: (context, groupMember) {
return const Row(
children: [
Icon(Icons.call, color: Color(0xFF6851D6), size: 25,),
SizedBox(width: 10),
Icon(Icons.video_call, color: Color(0xFF6851D6), size: 25,),
],
); // Replaced the placeholder with a custom widget.
},
)
- Android
- iOS
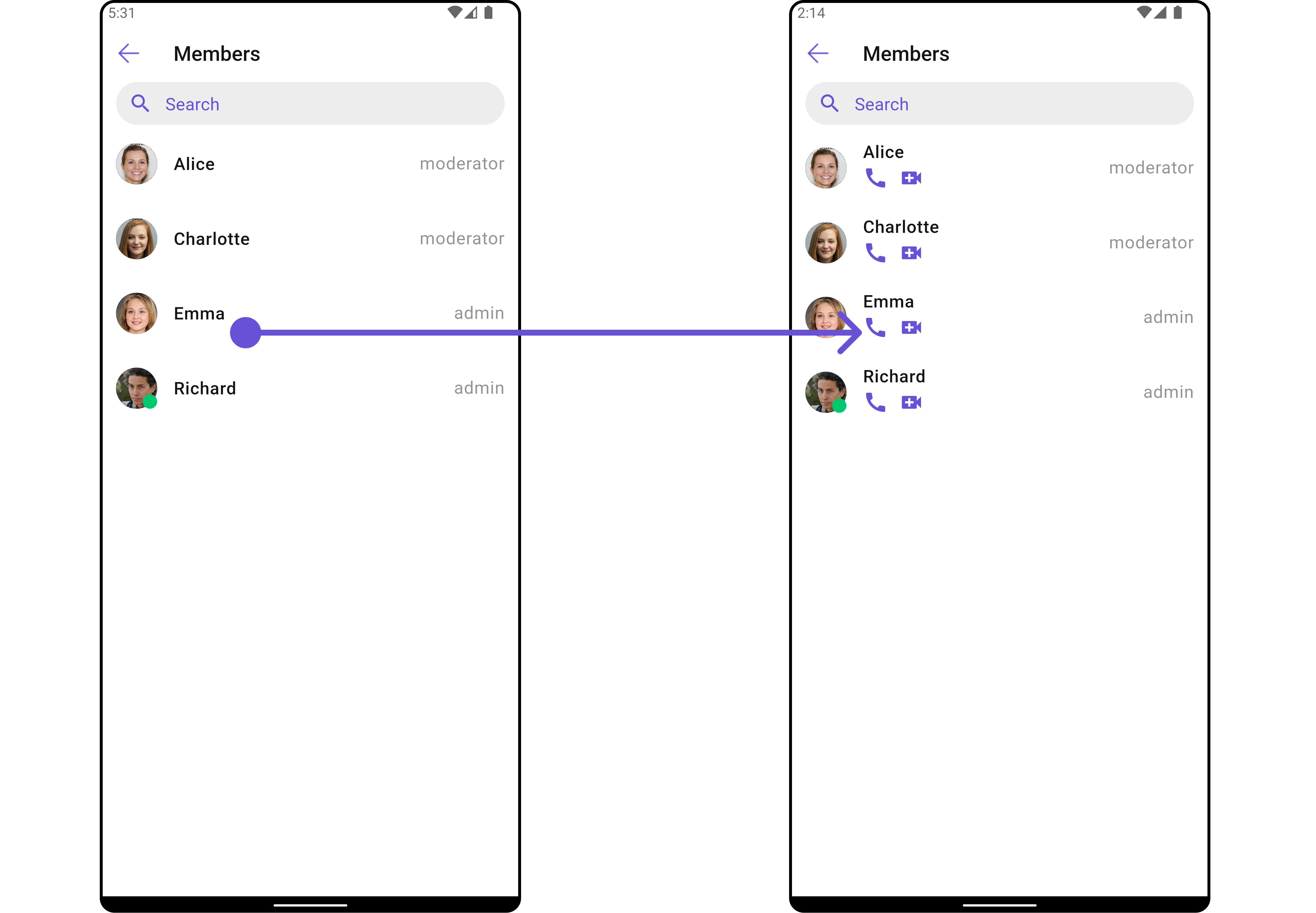
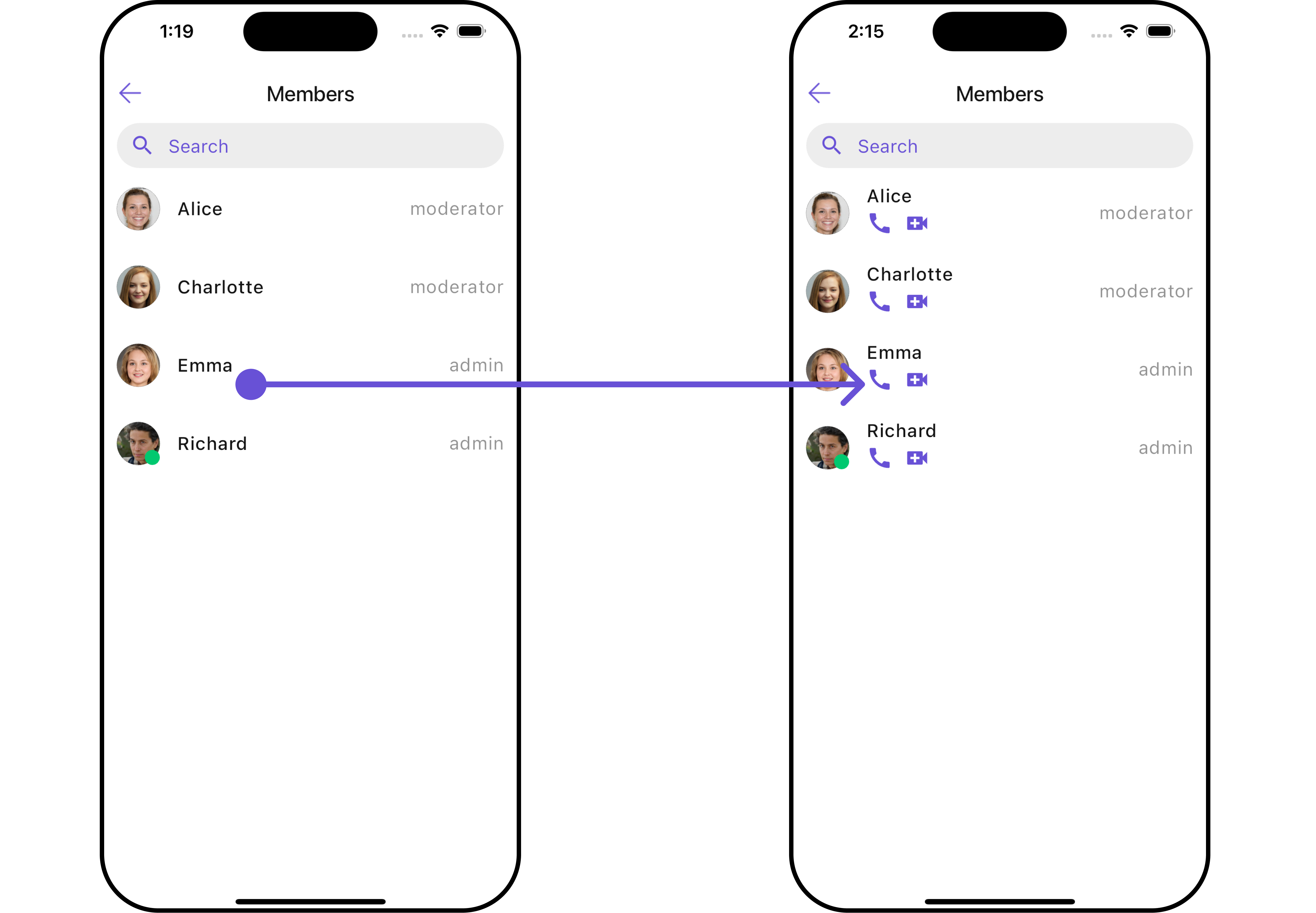
AppBarOptions
You can set the Custom appBarOptions
to the CometChatGroupMembers
widget.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
appBarOptions: [
Placeholder(),
Placeholder(),
Placeholder()
] // Replace this list of placeholder widgets with your list of custom widgets.
)
Here is the complete example for reference:
Example
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
appBarOptions: [
InkWell(
onTap: () {
// TODO("Not yet implemented")
},
child: const Icon(Icons.ac_unit, color: Color(0xFF6851D6)),
),
const SizedBox(width: 10)
], // Replaced the list of placeholder widgets with a list of custom widgets.
)
- Android
- iOS
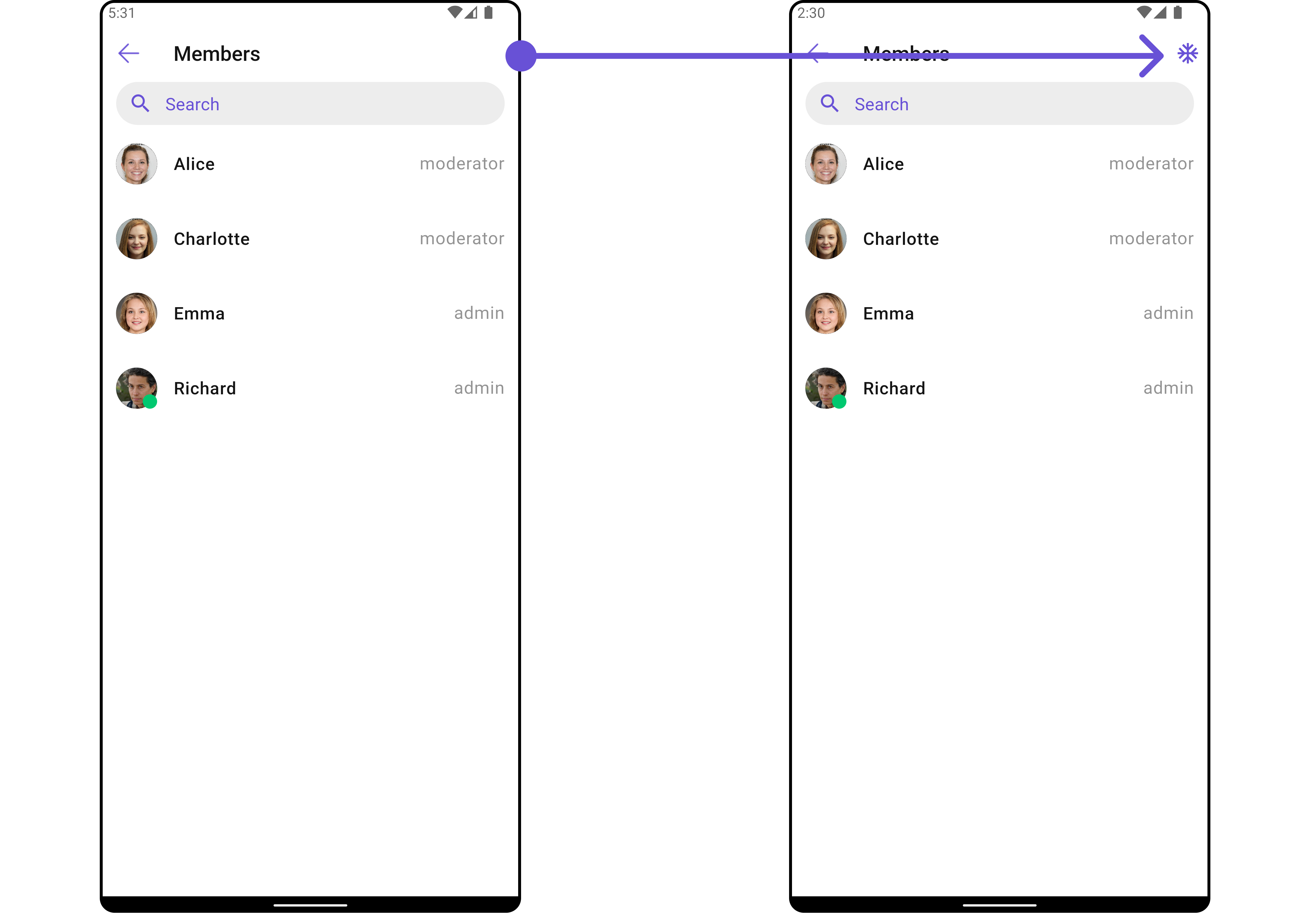
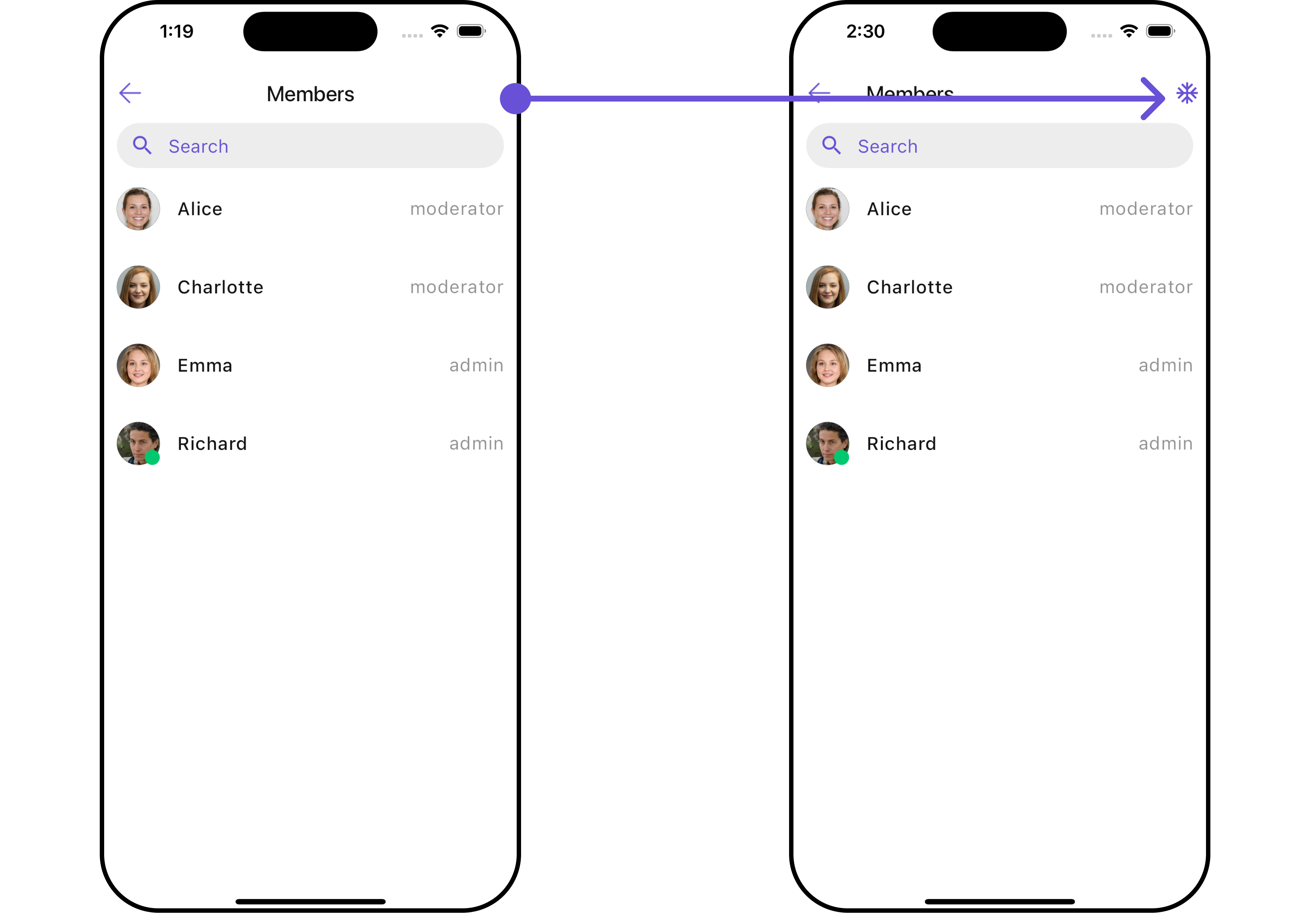
TailView
Used to generate a custom trailing widget for the CometChatGroupMembers
widget. You can add a Tail widget using the following method.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
tailView: (context, groupMembers) {
return Placeholder(); // Replace this placeholder with your custom widget.
},
)
Here is the complete example for reference:
Example
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
tailView: (context, groupMembers) {
return Container(
width: MediaQuery.of(context).size.width/6,
child: const Row(
children: [
Icon(Icons.call, color: Color(0xFF6851D6), size: 25,),
SizedBox(width: 10),
Icon(Icons.video_call, color: Color(0xFF6851D6), size: 25,),
],
),
); // Replaced the placeholder with a custom widget.
},
)
- Android
- iOS
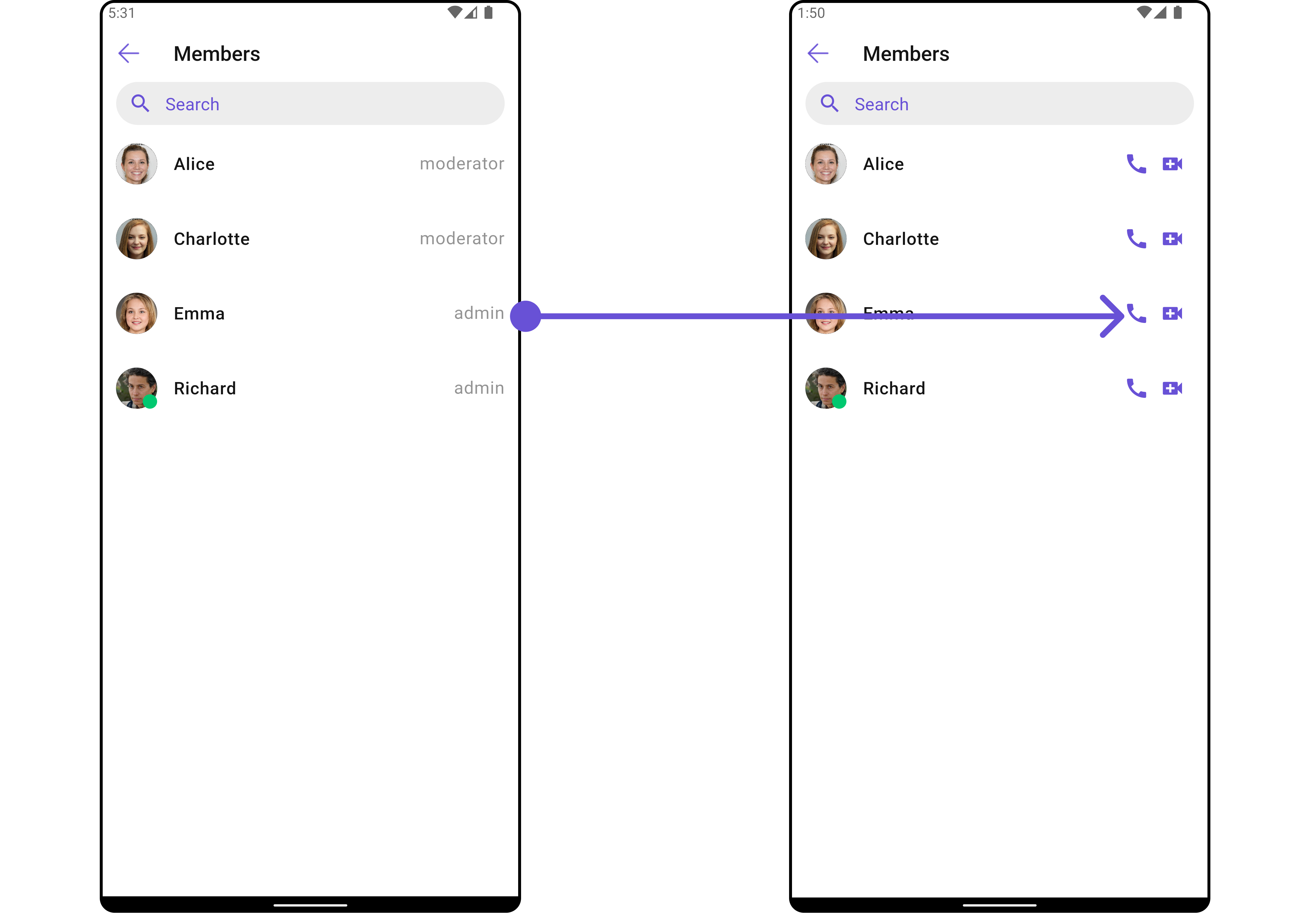
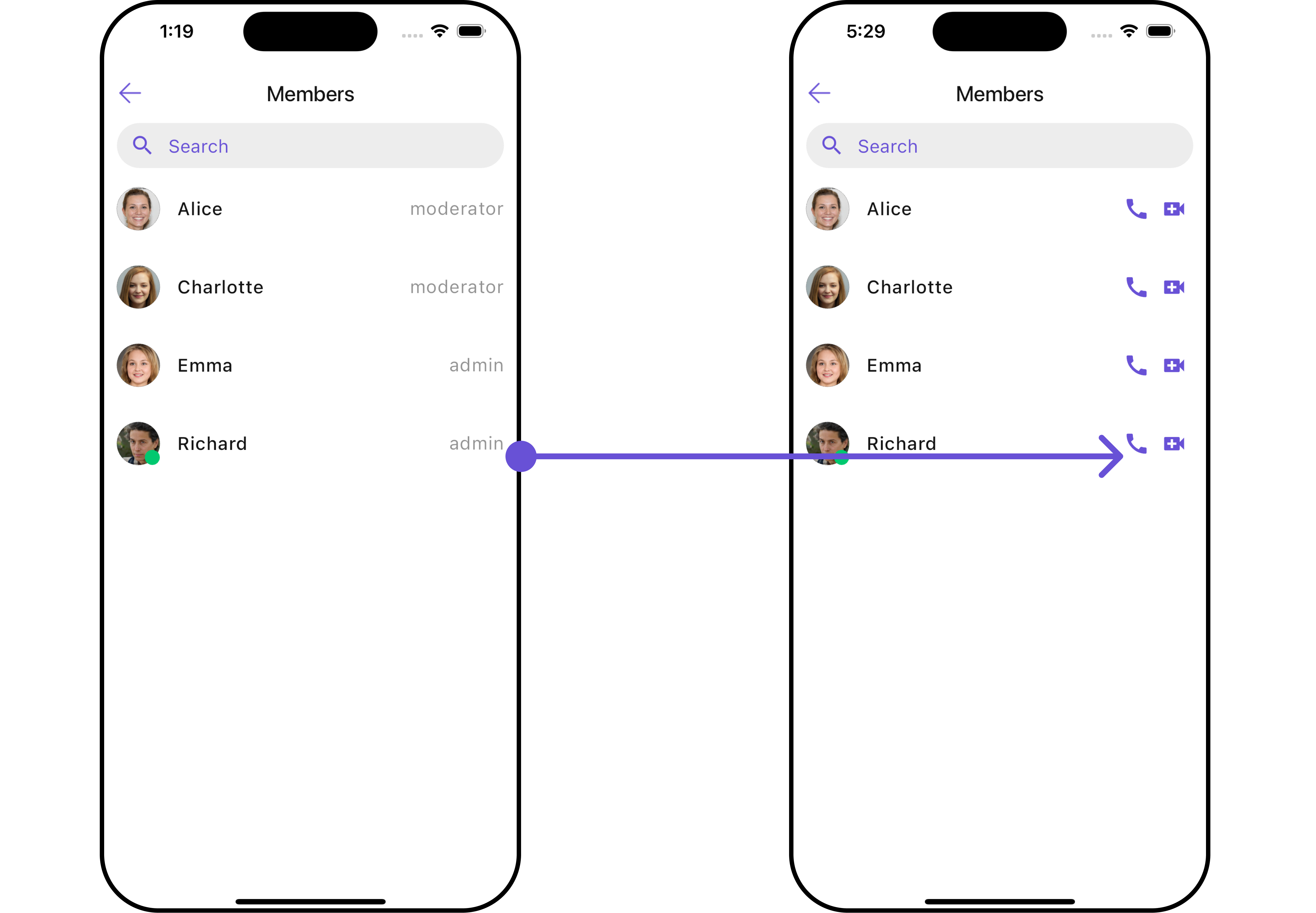
LoadingStateView
You can set a custom loader widget using loadingStateView
to match the loading UI of your app.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
loadingStateView: (context) {
return Placeholder(); // Replace this placeholder with your custom widget.
},
)
Here is the complete example for reference:
Example
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
loadingStateView: (context) {
return Container(
width: MediaQuery.of(context).size.width,
child: Center(
child: CircularProgressIndicator()
),
); // Replaced the placeholder with a custom widget.
},
)
- Android
- iOS
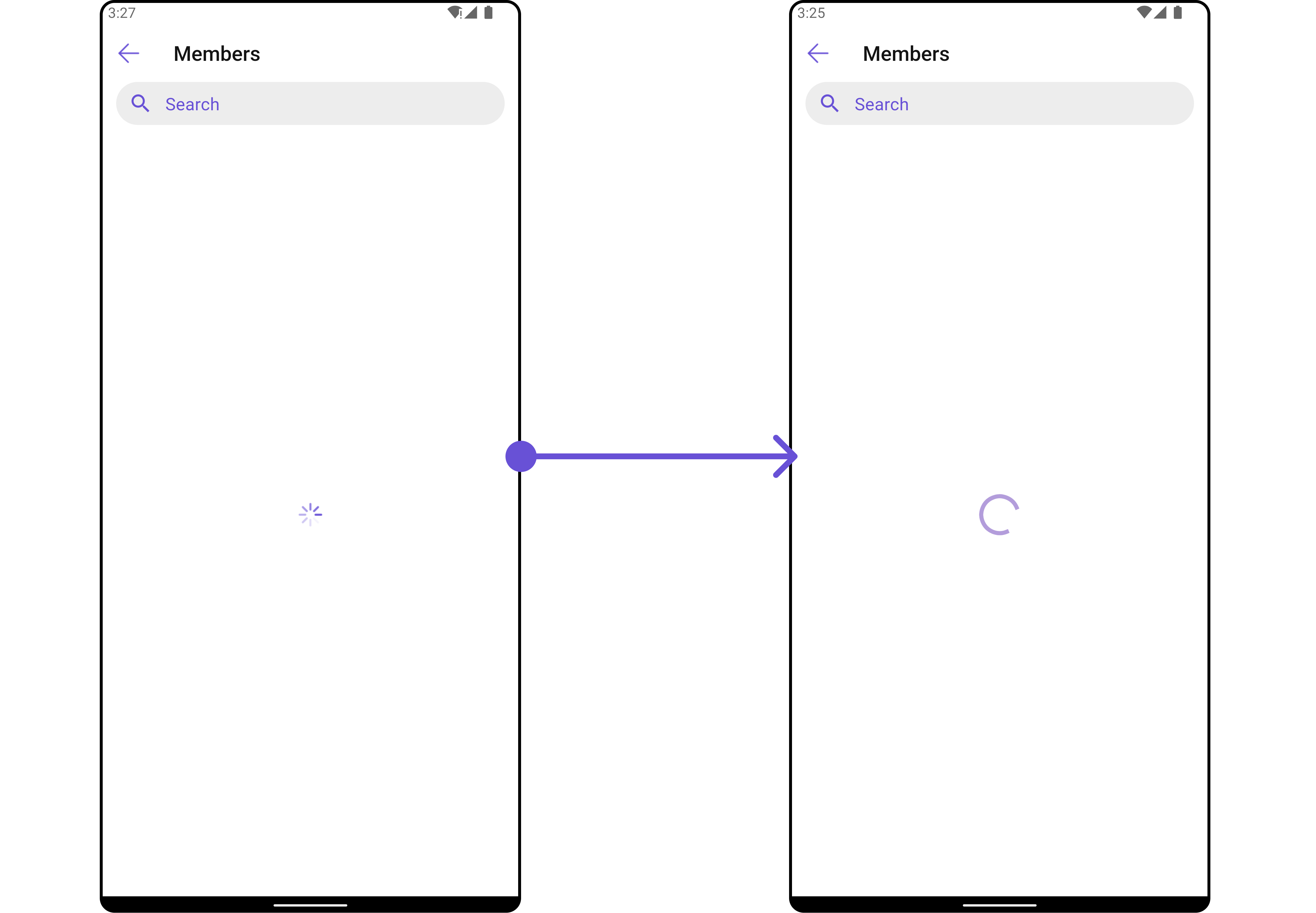
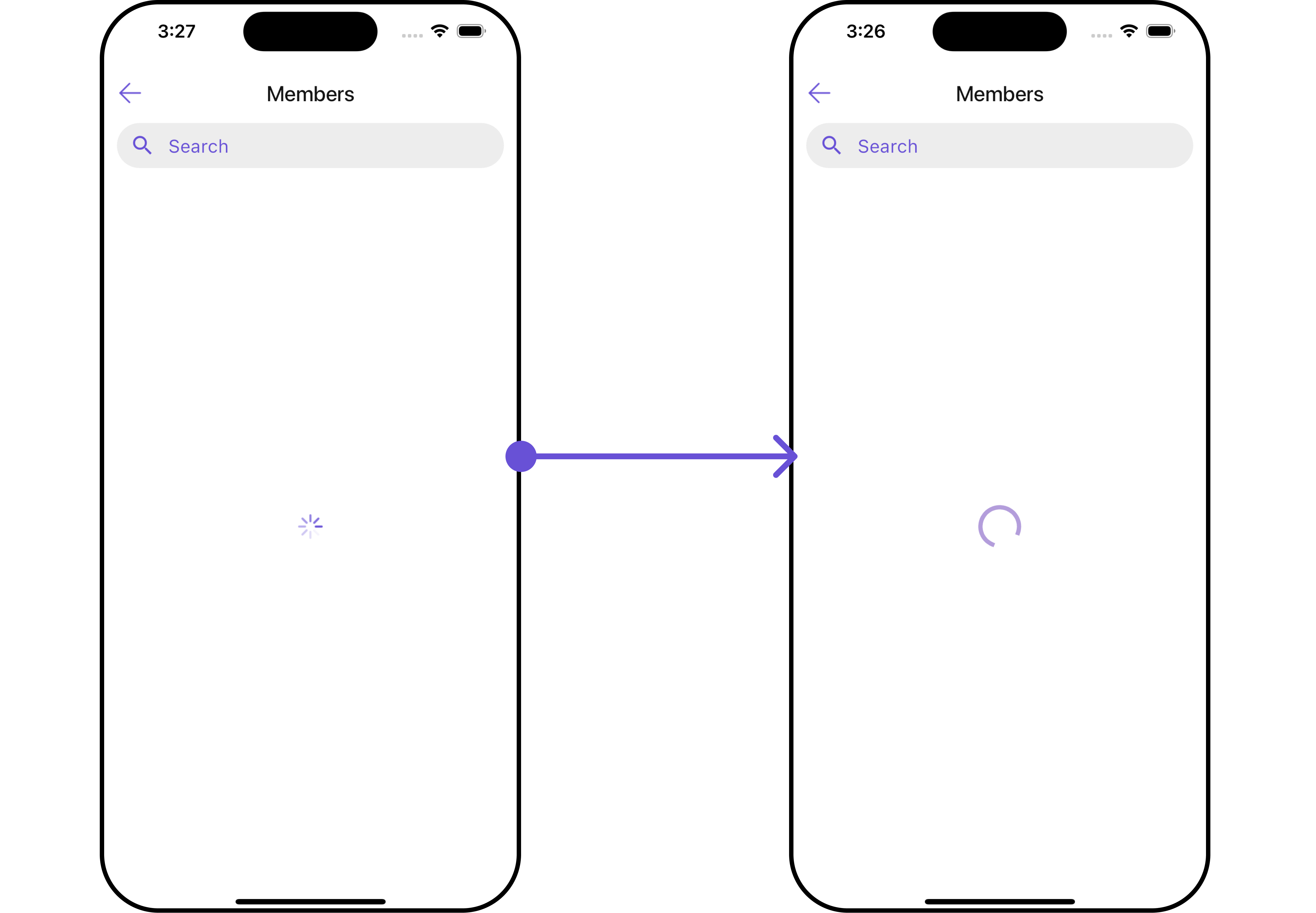
EmptyStateView
You can set a custom EmptyStateView
using emptyStateView
to match the error UI of your app.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
emptyStateView: (context) {
return Placeholder(); // Replace this placeholder with your custom widget.
},
)
Here is the complete example for reference:
Example
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
emptyStateView: (context) {
return Container(
width: MediaQuery.of(context).size.width,
child: Center(
child: const Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Spacer(),
Icon(Icons.sms_failed_outlined, color: Colors.red, size: 100,),
SizedBox(height: 20,),
Text("Your Custom Message"),
Spacer(),
],
)
),
); // Replaced the placeholder with a custom widget.
},
)
- Android
- iOS
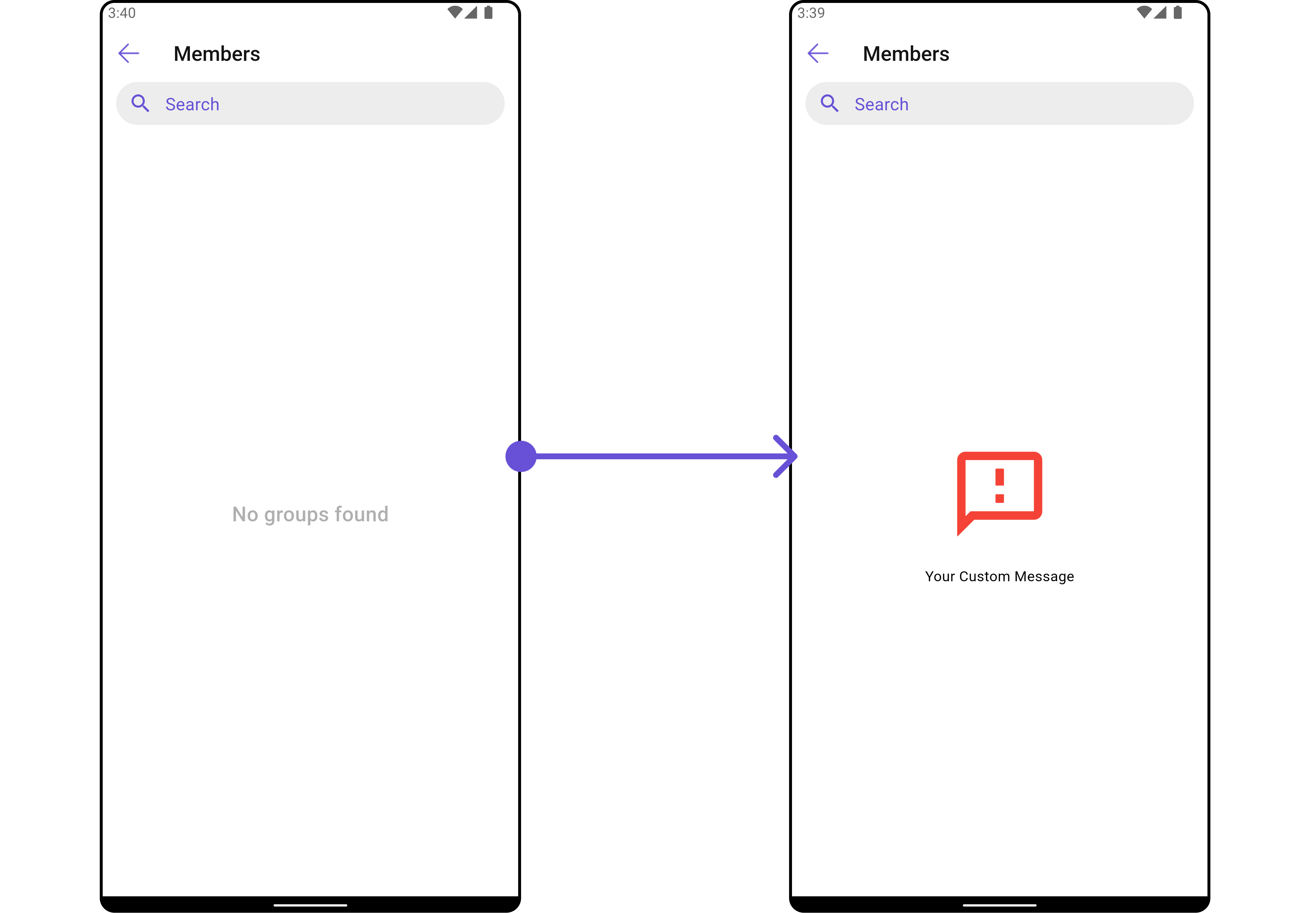
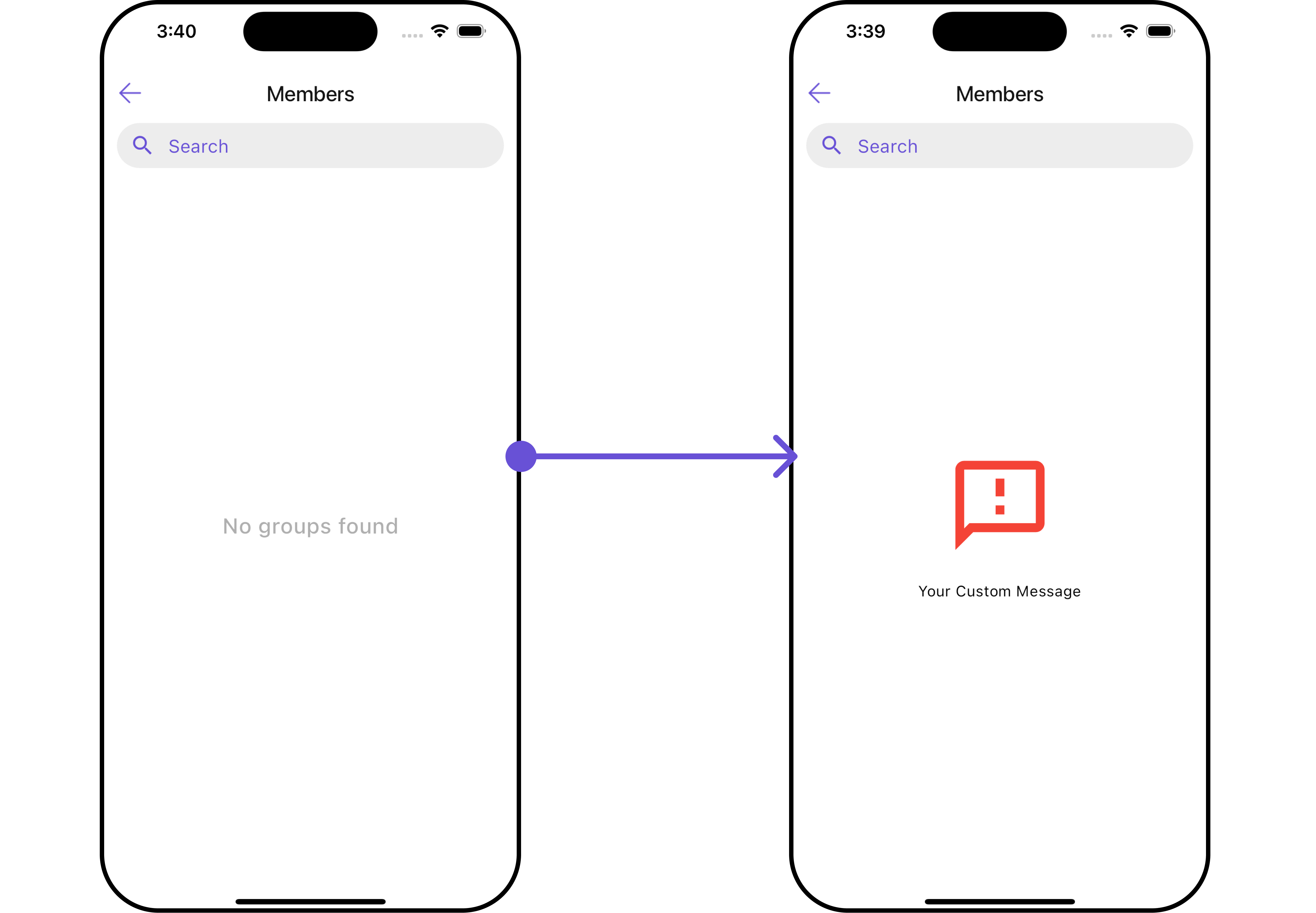
ErrorStateView
You can set a custom ErrorStateView
using errorStateView
to match the error UI of your app.
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
errorStateView: (context) {
return Placeholder(); // Replace this placeholder with your custom widget.
},
)
Here is the complete example for reference:
Example
- Dart
CometChatGroupMembers(
group: Group(guid: "", name: "", type: ""), // A group object is required to launch this widget.
errorStateView: (context) {
return Container(
width: MediaQuery.of(context).size.width,
child: Center(
child: const Column(
crossAxisAlignment: CrossAxisAlignment.center,
mainAxisAlignment: MainAxisAlignment.center,
children: [
Spacer(),
Icon(Icons.error_outline, color: Colors.red, size: 100,),
SizedBox(height: 20,),
Text("Your Custom Error Message"),
Spacer(),
],
),
),
); // Replaced the placeholder with a custom widget.
},
)
- Android
- iOS
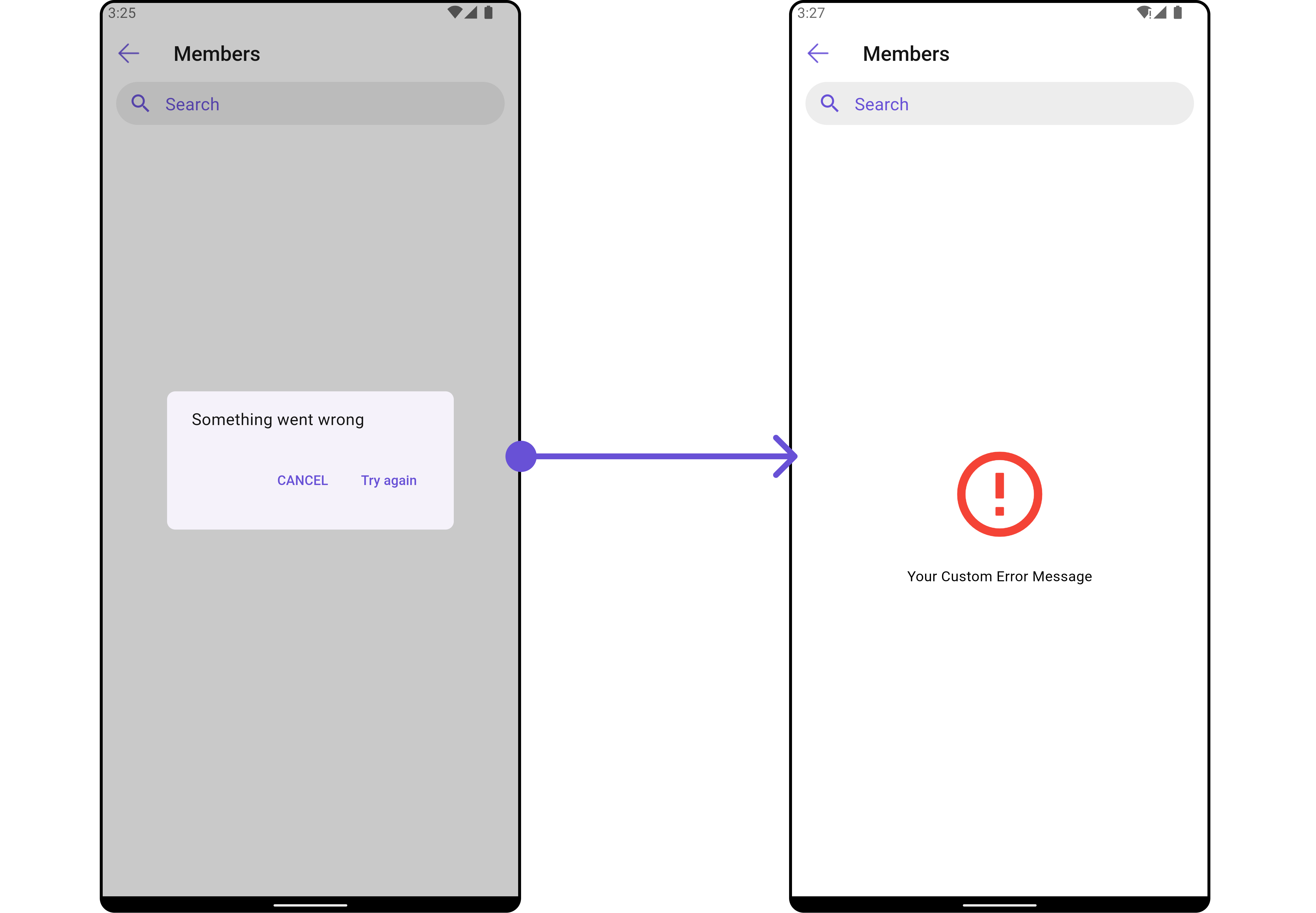
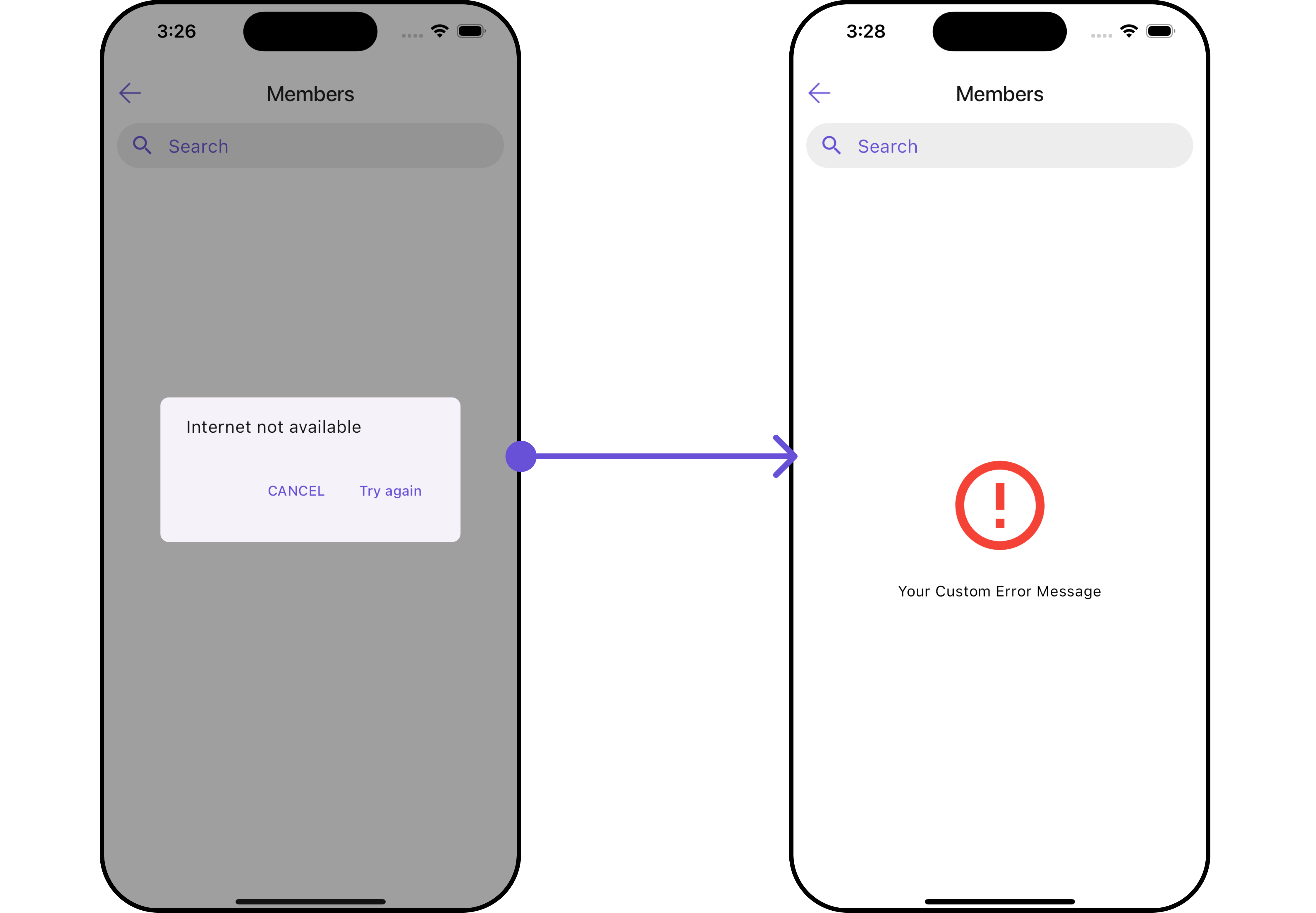