Groups with Messages
Overview
CometChatGroupsWithMessages
is a Composite Widget encapsulating functionalities from the Groups and Messages widgets. Serving as a versatile wrapper, it seamlessly integrates with CometChatMessages
, enabling users to open the module by clicking on any group within the list. This widget inherits the behavior of Groups, fostering consistency and familiarity in user interactions.
- Android
- iOS
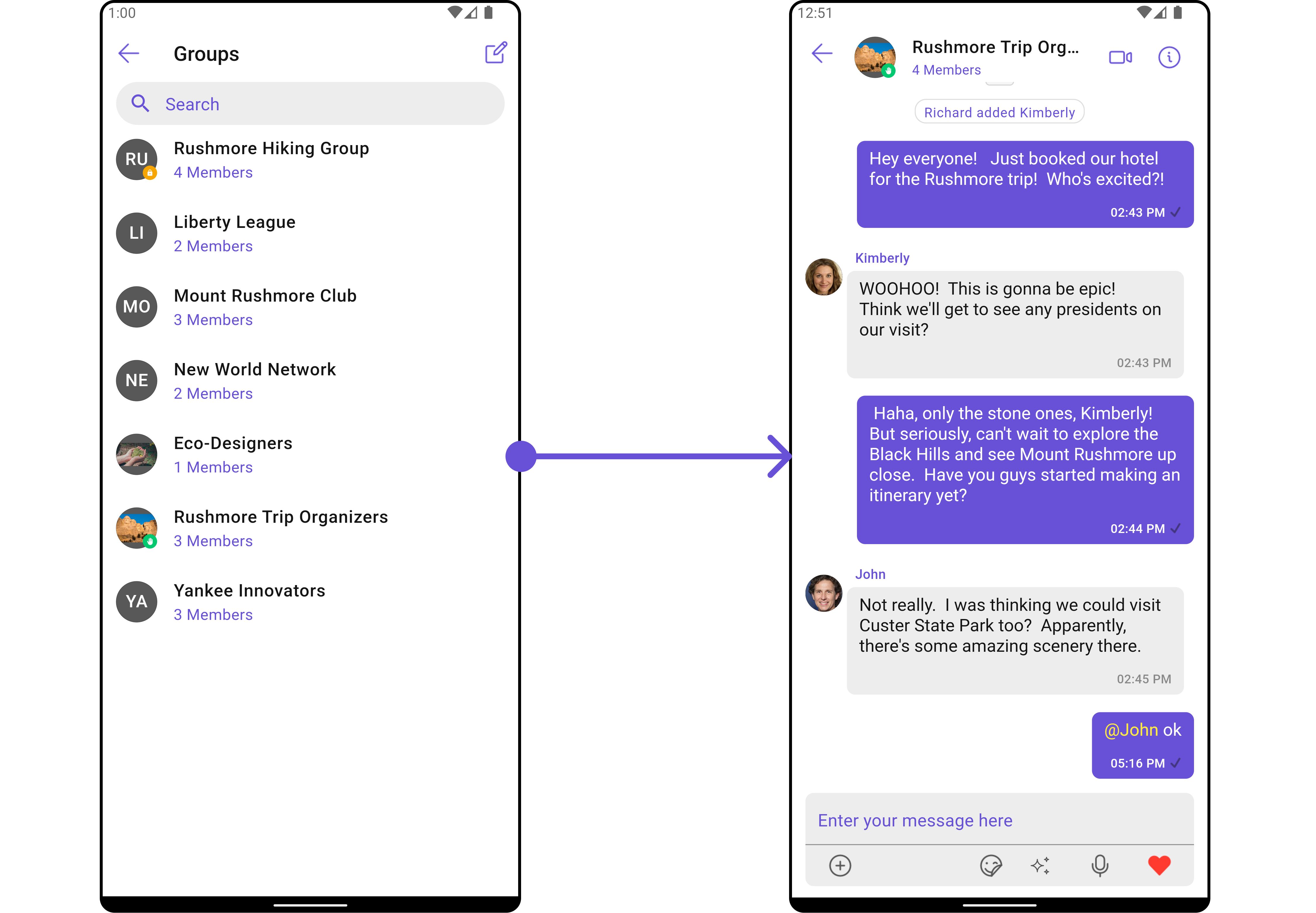
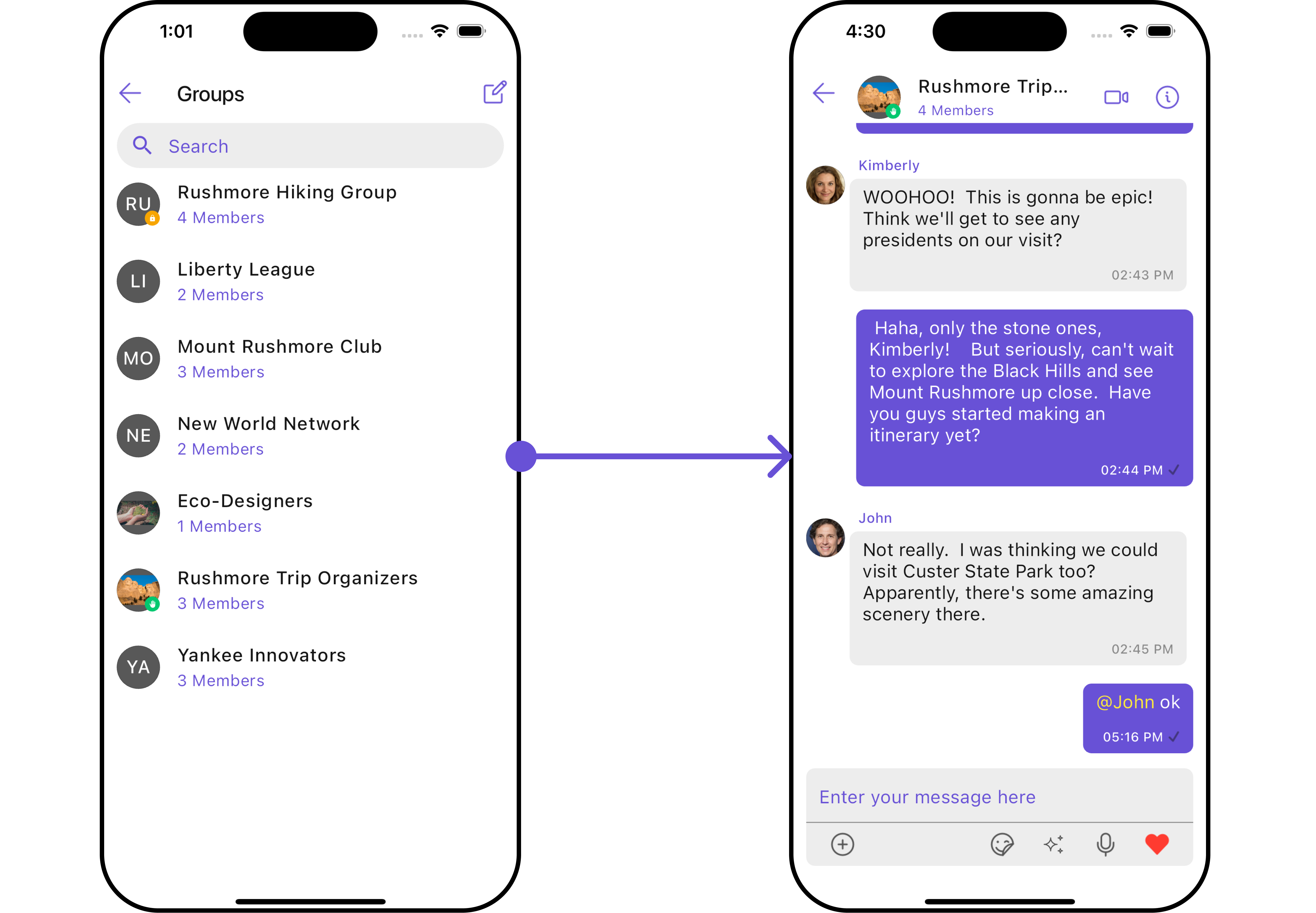
Widgets | Description |
---|---|
Groups | The Groups widget is designed to display a list of Groups . This essentially represents the recent conversation history. |
Messages | The Messages widget is designed to manage the messaging interaction for Group's conversations. |
Usage
Integration
As CometChatGroupsWithMessages
is a widget, it can be effortlessly added directly in response to a button click or any event. Leveraging all the customizable properties and methods inherited from Groups, this widget offers seamless integration and extensive customization capabilities. This makes it a versatile solution for enhancing user interaction within your application.
You can launch CometChatGroupsWithMessages
directly using Navigator.push
, or you can define it as a widget within the build
method of your State
class.
1. Using Navigator to Launch CometChatGroupsWithMessages
- Dart
Navigator.push(context, MaterialPageRoute(builder: (context) => const CometChatGroupsWithMessages()));
2. Embedding CometChatGroupsWithMessages
as a Widget in the build Method
- Dart
import 'package:cometchat_chat_uikit/cometchat_chat_uikit.dart';
import 'package:flutter/material.dart';
class CometChatGroupsWithMessages extends StatefulWidget {
const CometChatGroupsWithMessages({super.key});
State<CometChatGroupsWithMessages> createState() => _CometChatGroupsWithMessagesState();
}
class _CometChatGroupsWithMessagesState extends State<CometChatGroupsWithMessages> {
Widget build(BuildContext context) {
return const Scaffold(
body: SafeArea(
child: CometChatGroupsWithMessages()
)
);
}
}
Actions
Actions dictate how a widget functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the widget to fit your specific needs.
1. onItemTap
This method proves valuable when users seek to override onItemTap functionality within CometChatGroupsWithMessages
, empowering them with greater control and customization options.
The onItemTap
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
onItemTap: (context, group) {
// TODO("Not yet implemented")
},
),
)
2. onBack
Enhance your application's functionality by leveraging the onBack
feature. This capability allows you to customize the behavior associated with navigating back within your app. Utilize the provided code snippet to override default behaviors and tailor the user experience according to your specific requirements.
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
onBack: () {
// TODO("Not yet implemented")
},
),
)
3. onError
You can customize this behavior by using the provided code snippet to override the onError
and improve error handling.
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
onError: (e) {
// TODO("Not yet implemented")
},
),
)
4. onItemLongPress
This method becomes invaluable when users seek to override long-click functionality within CometChatGroupsWithMessages
, offering them enhanced control and flexibility in their interactions.
The onItemLongPress
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
onItemLongPress: (context, group) {
// TODO("Not yet implemented")
},
),
)
Filters
Filters allow you to customize the data displayed in a list within a widget. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
While the CometChatGroupsWithMessages
widget does not have filters, its widgets do, For more detail on individual filters of its widget refer to Groups Filters and Messages Filters.
By utilizing the Configurations object of its widgets, you can apply filters.
In the following example, we are applying a filter to the Group List based on only joined groups and setting the limit to 3 using the groupsRequestBuilder
.
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
groupsRequestBuilder: GroupsRequestBuilder()
..limit = 10,
),
)
Events
Events are emitted by a widget
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The CometChatGroupsWithMessages
does not produce any events but its subwidget does.
Customization
To fit your app's design requirements, you have the ability to customize the appearance of the CometChatGroupsWithMessages
widget. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the widget in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the widget.
1. CometChatGroupsWithMessages Style
You can set the groupsWithMessagesStyle
to the CometChatGroupsWithMessages
Widget to customize the styling.
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
groupsStyle: GroupsStyle(
background: Color(0xFFE4EBF5),
titleStyle: TextStyle(color: Colors.red),
backIconTint: Colors.red
),
),
)
- Android
- iOS
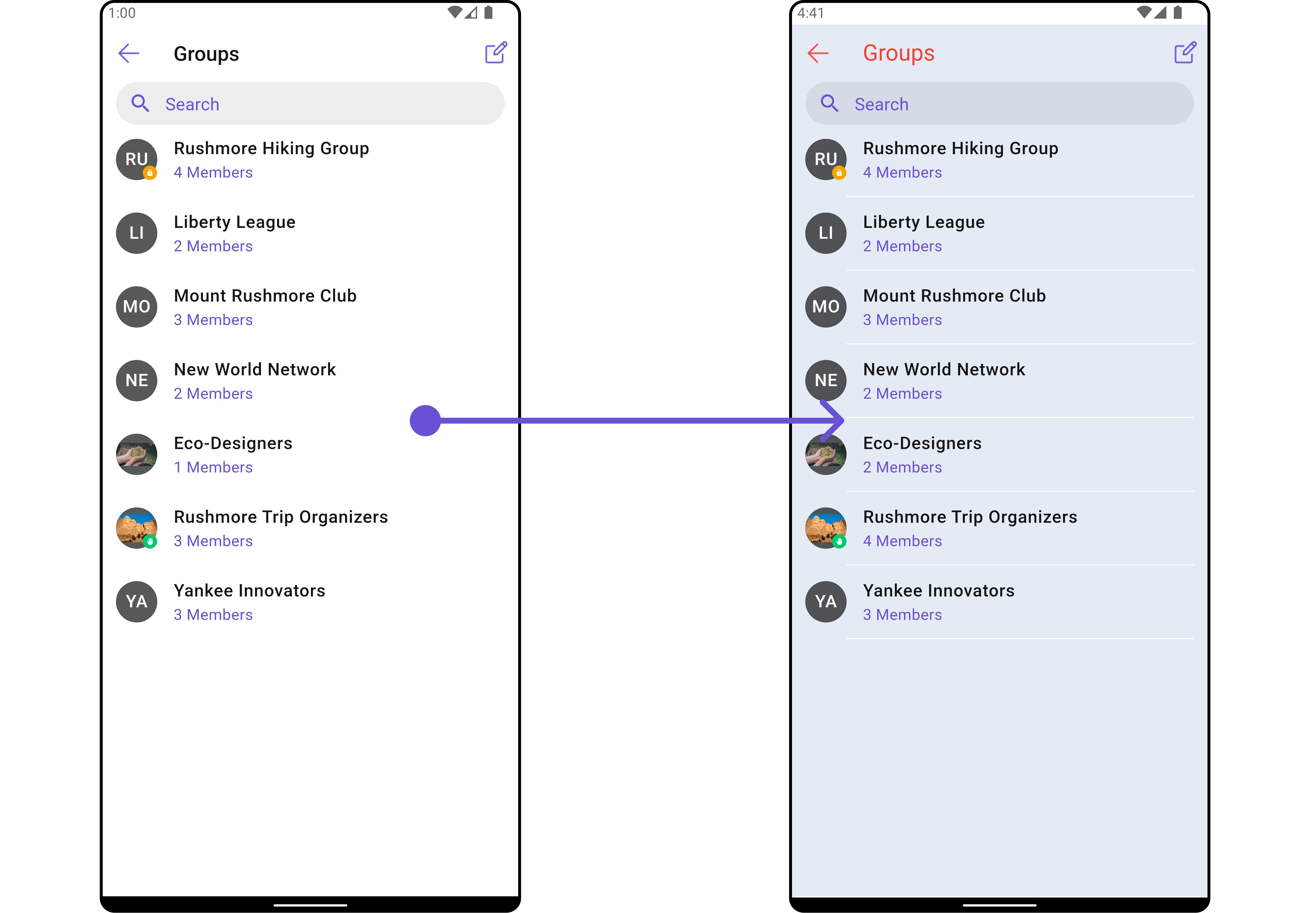
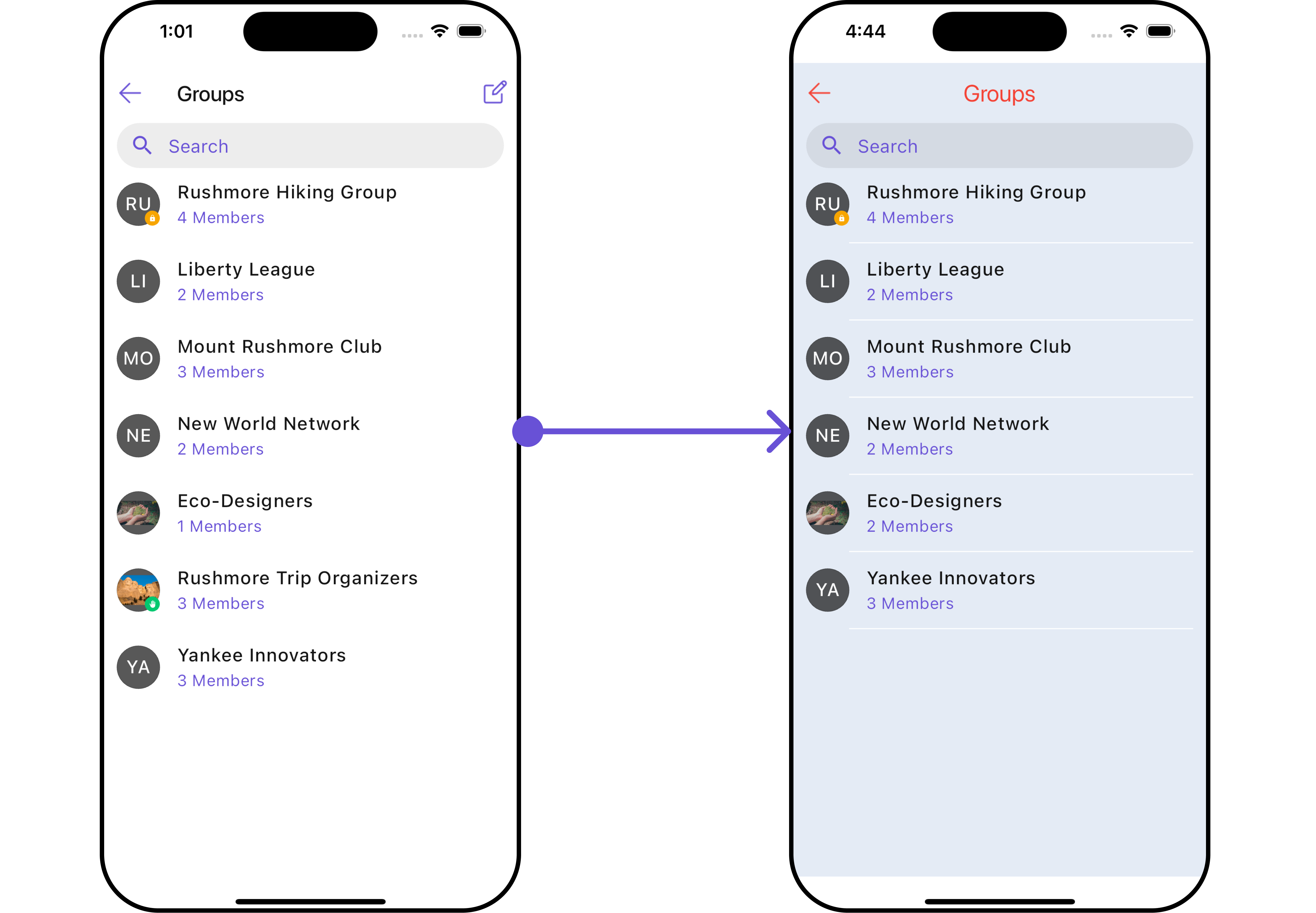
You can also customize its widget styles. For more details on individual widget styles, you can refer Groups Styles and Messages Styles.
Styles can be applied to Sub widgets using their respective configurations.
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the widget. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
CometChatGroupsWithMessages
widget does not have any available functionality. You can use Functional customisation its Widgets. For more details on individual widget functionalities, you can refer Groups Functionalities and Messages functionalities.
Advanced
For advanced-level customization, you can set custom widget to the widget. This lets you tailor each aspect of the widget to fit your exact needs and application aesthetics. You can create and define your own widget and then incorporate those into the widget.
CometChatGroupsWithMessages
widget does not have any advanced-level customization . You can use Advanced customisation its Widgets. For more details on individual widget functionalities, you can refer Groups Advanced and Messages Advanced.
CometChatGroupsWithMessages
uses advanced-level customization of both Groups & Messages widgets to achieve its default behavior.
1. SubtitleView
You can customize the subtitle widget for each CometChatGroupsWithMessages
item to meet your requirements
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
subtitleView: (context, conversation) {
return const Row(
children: [
Icon(Icons.call, color: Color(0xFF6851D6), size: 25,),
SizedBox(width: 10),
Icon(Icons.video_call, color: Color(0xFF6851D6), size: 25,),
],
);
},
),
)
- Android
- iOS
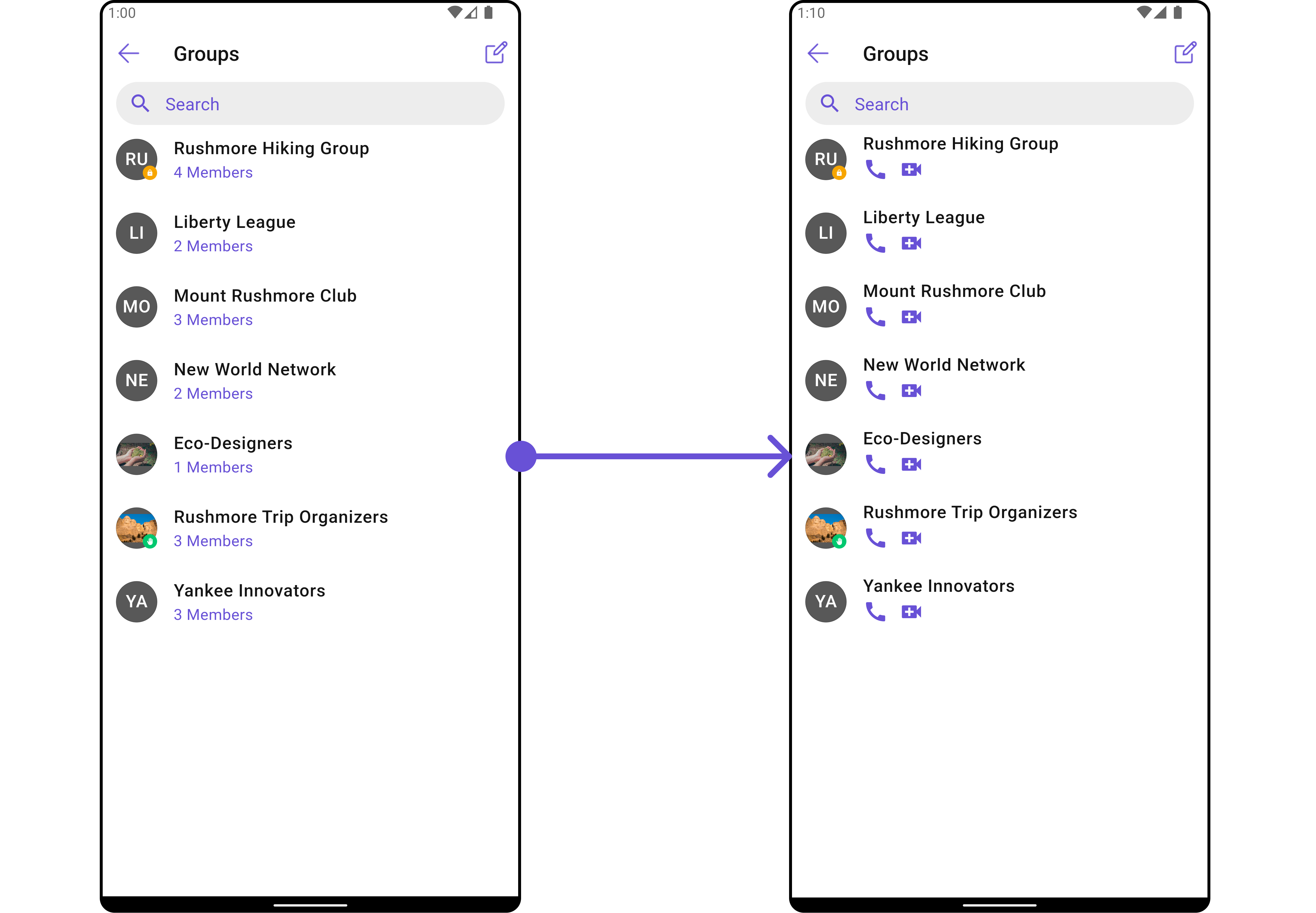
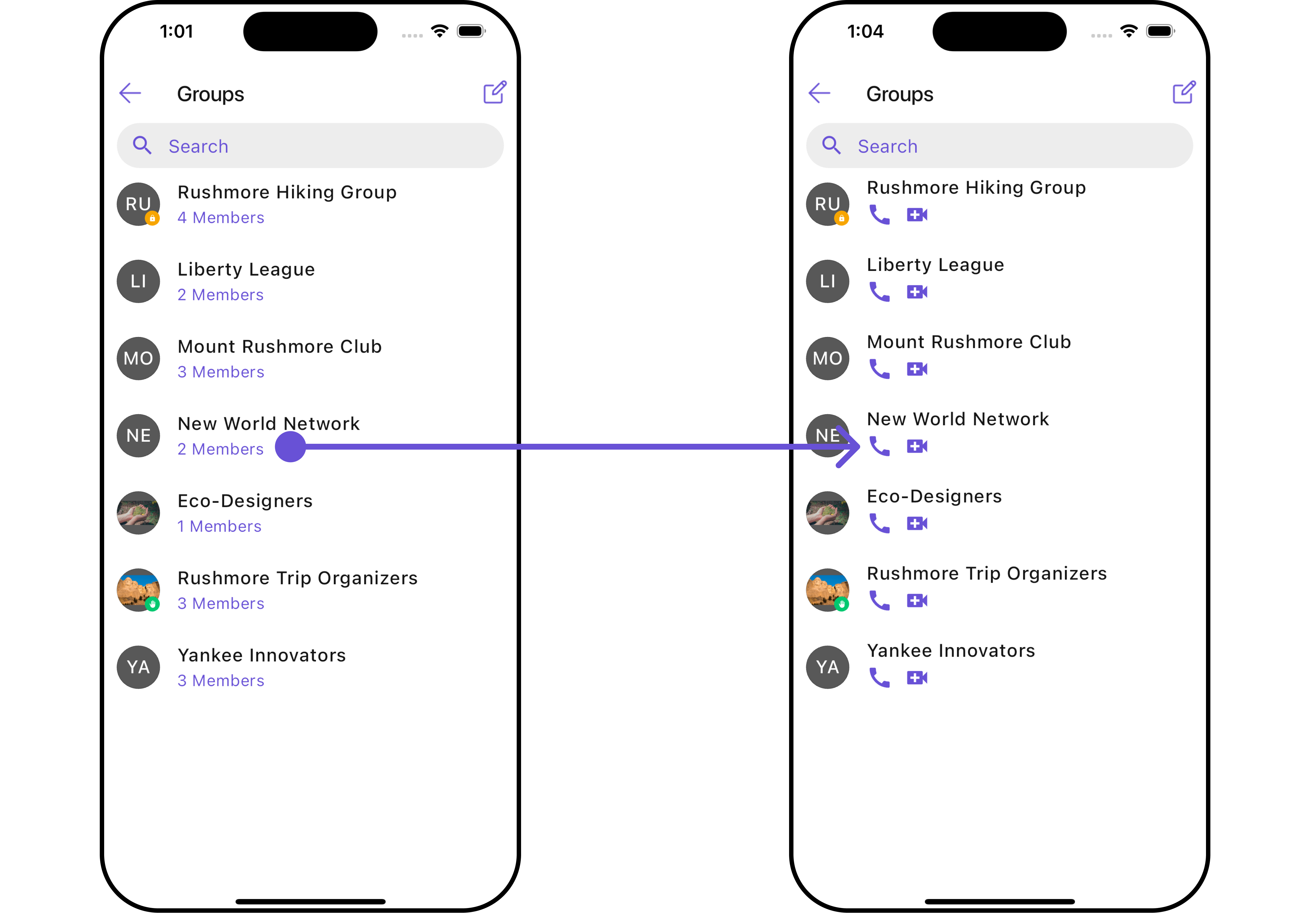
2. AppBarOptions
You can set the Custom AppBarOptions to the CometChatGroupsWithMessages
widget.
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
appBarOptions: (context) {
return [
InkWell(
onTap: () {
// TODO("Not yet implemented")
},
child: const Icon(Icons.ac_unit, color: Color(0xFF6851D6)),
),
const SizedBox(width: 10)
];
},
),
)
- Android
- iOS
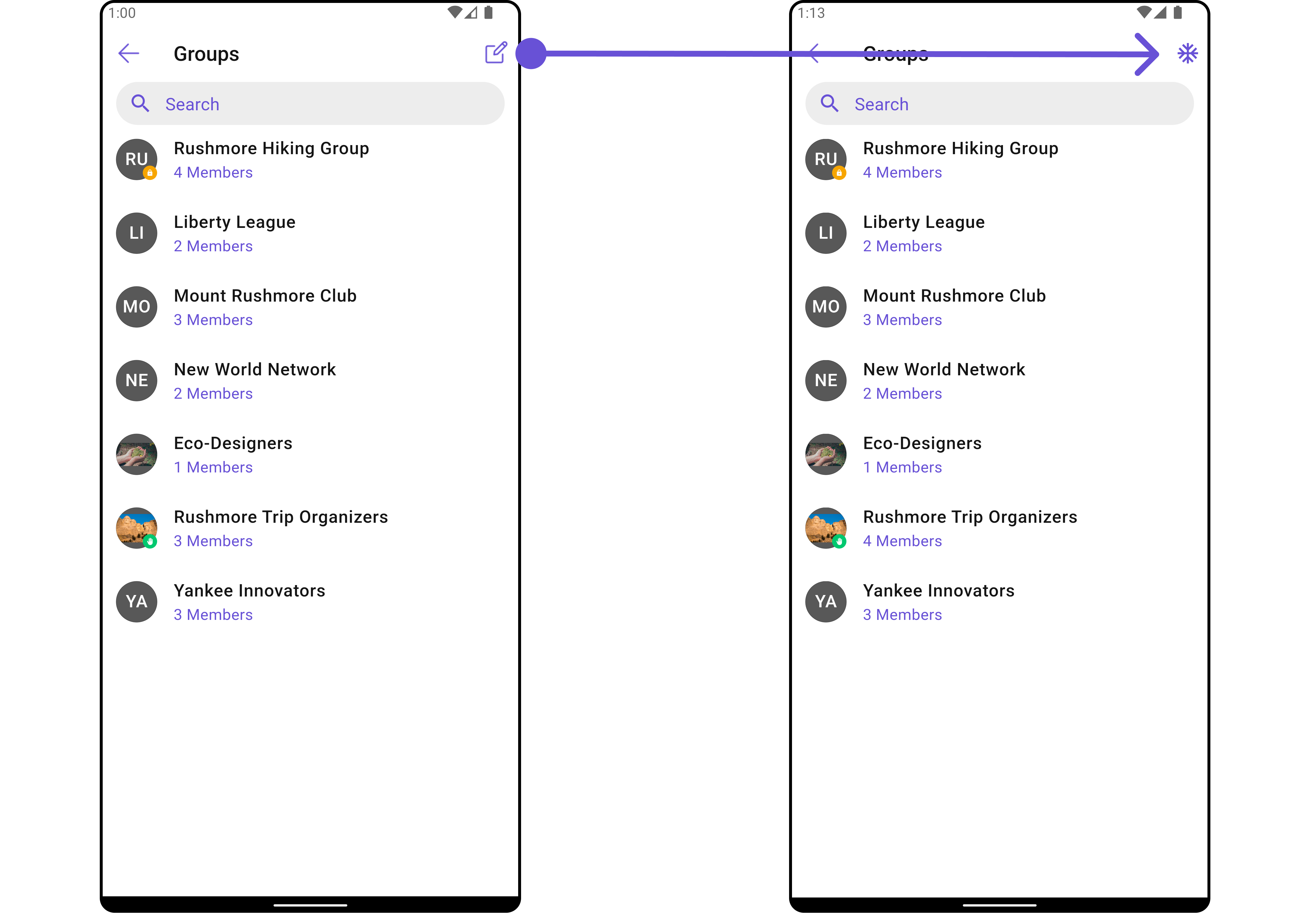
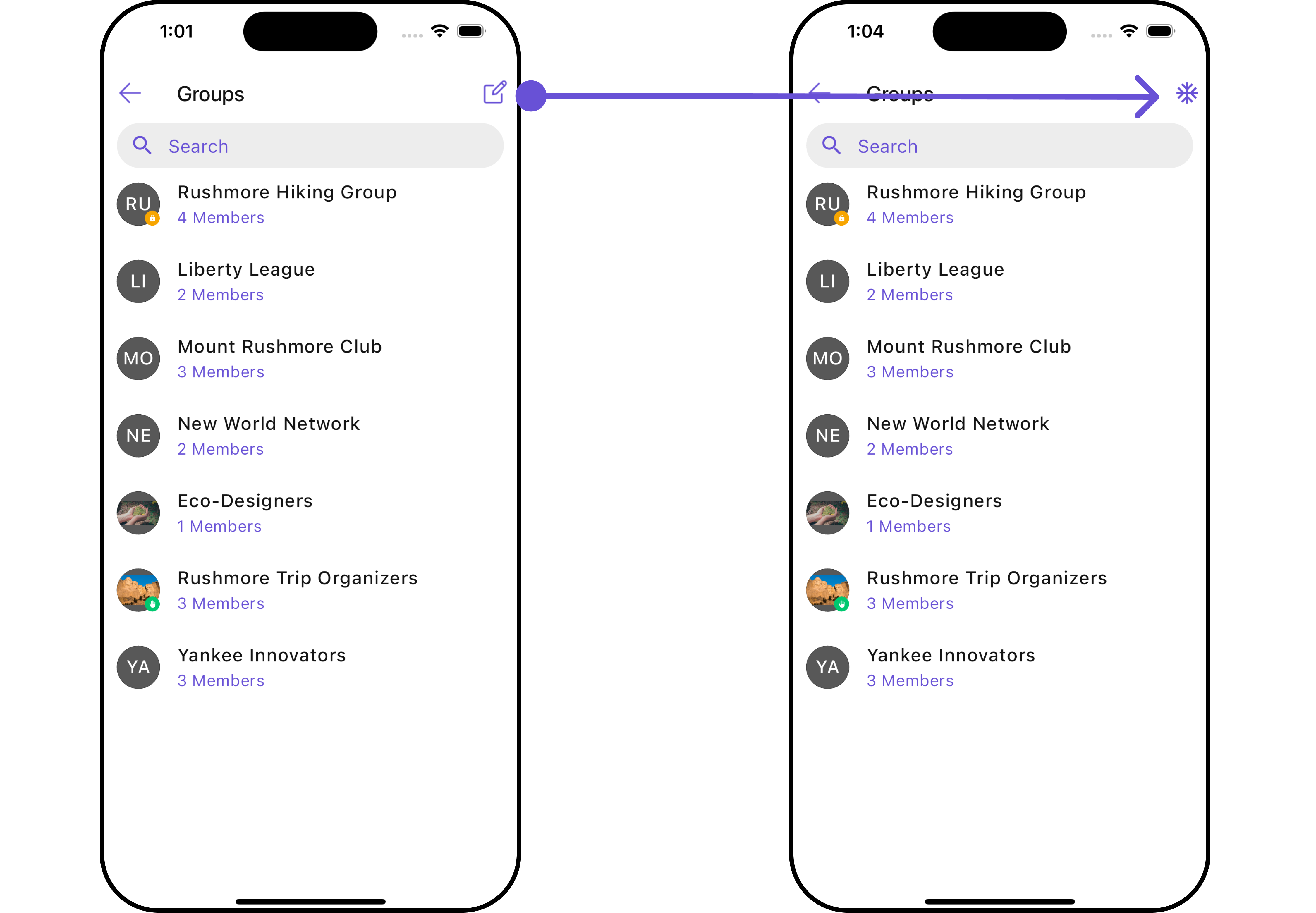
Configurations
Configurations offer the ability to customize the properties of each widget within a Composite widget.
CometChatGroupsWithMessages has Groups
and Messages
widget. Hence, each of these widgets will have its individual Configuration
. Configurations
expose properties that are available in its individual widgets.
Groups
You can customize the properties of the Groups widget by making use of the GroupsConfiguration. You can accomplish this by employing the following method as demonstrated below:
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
// TODO("Not yet implemented")
),
)
All exposed properties of GroupsConfiguration
can be found under Groups. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Groups sub widget
You can modify the style using the GroupsStyle
method.
- Dart
CometChatGroupsWithMessages(
groupsConfiguration: GroupsConfiguration(
groupsStyle: GroupsStyle(
background: Color(0xFFFAE6FA),
titleStyle: TextStyle(color: Colors.red),
backIconTint: Colors.red
),
),
)
- Android
- iOS
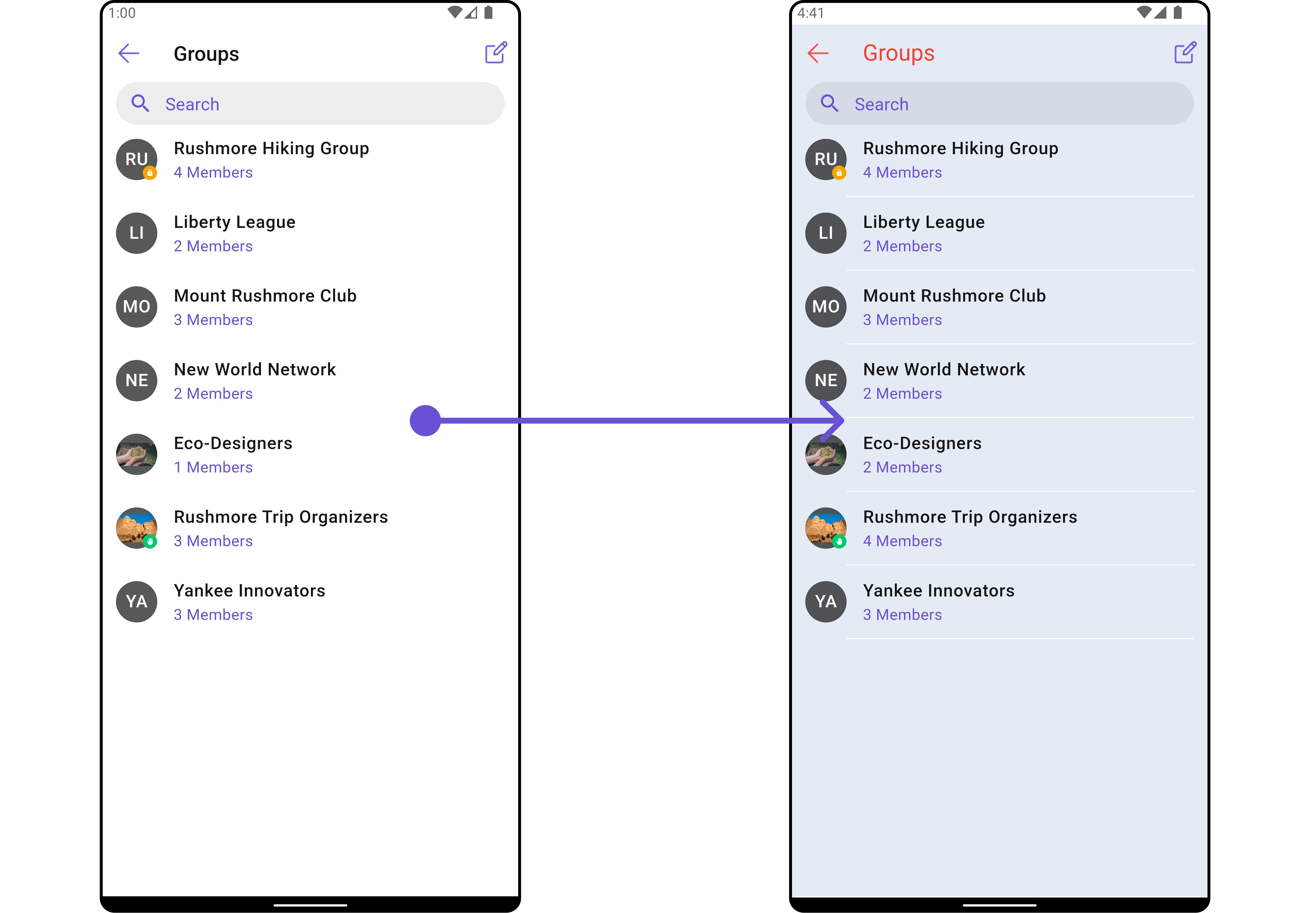
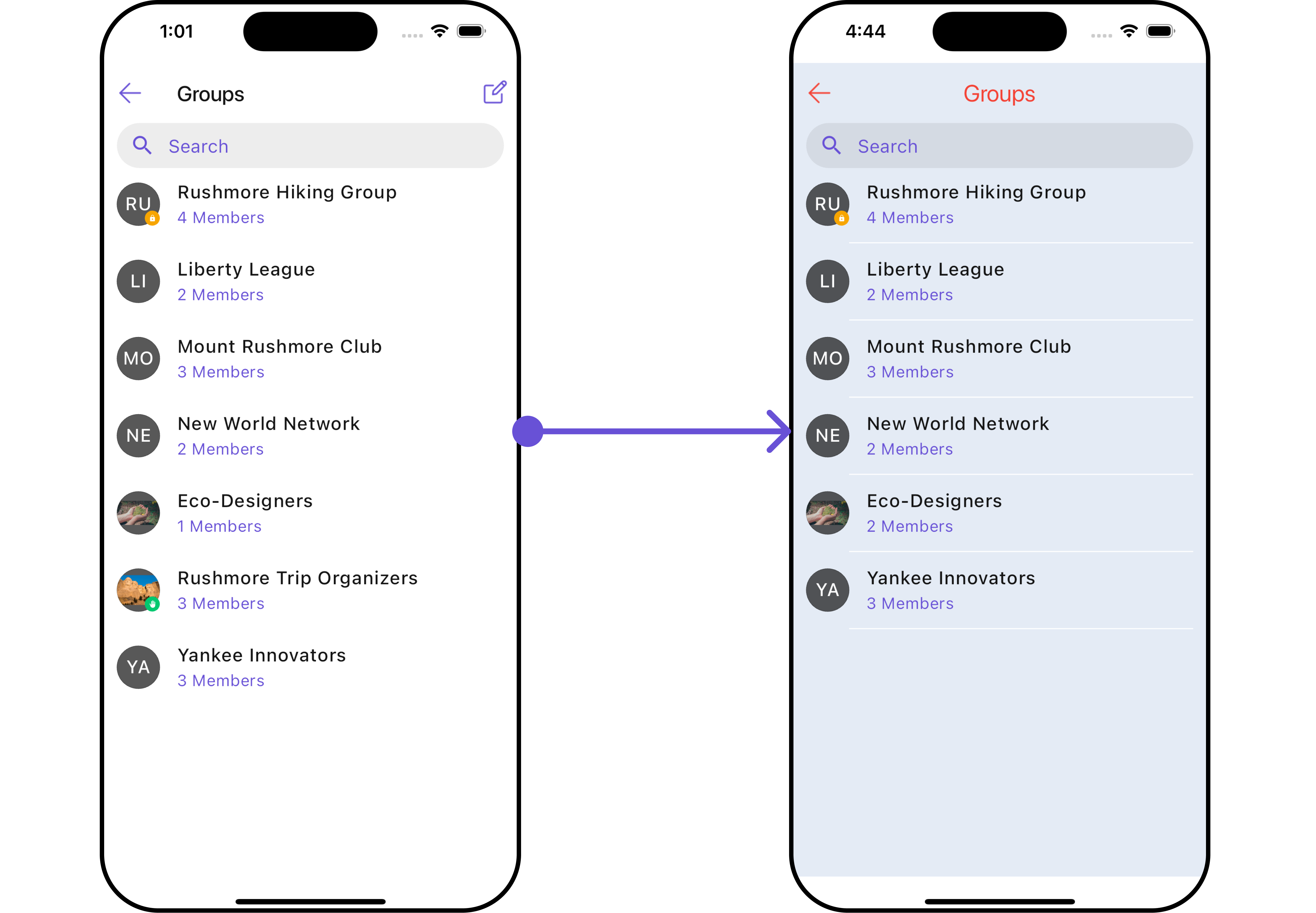
Messages
You can customize the properties of the Messages widget by making use of the messagesConfiguration
. You can accomplish this by employing the messagesConfiguration
as demonstrated below:
- Dart
CometChatGroupsWithMessages(
messageConfiguration: MessageConfiguration(
// TODO("Not yet implemented")
),
)
All exposed properties of MessagesConfiguration
can be found under Messages. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Messages sub widget and, in addition, you want to hide message composer.
You can modify the style using the messagesStyle
method and hide using hideMessageComposer
method.
- Dart
CometChatGroupsWithMessages(
messageConfiguration: MessageConfiguration(
disableTyping: true,
hideMessageComposer: true,
messagesStyle: MessagesStyle(
background: Color(0xFFE4EBF5),
)
),
)
- Android
- iOS
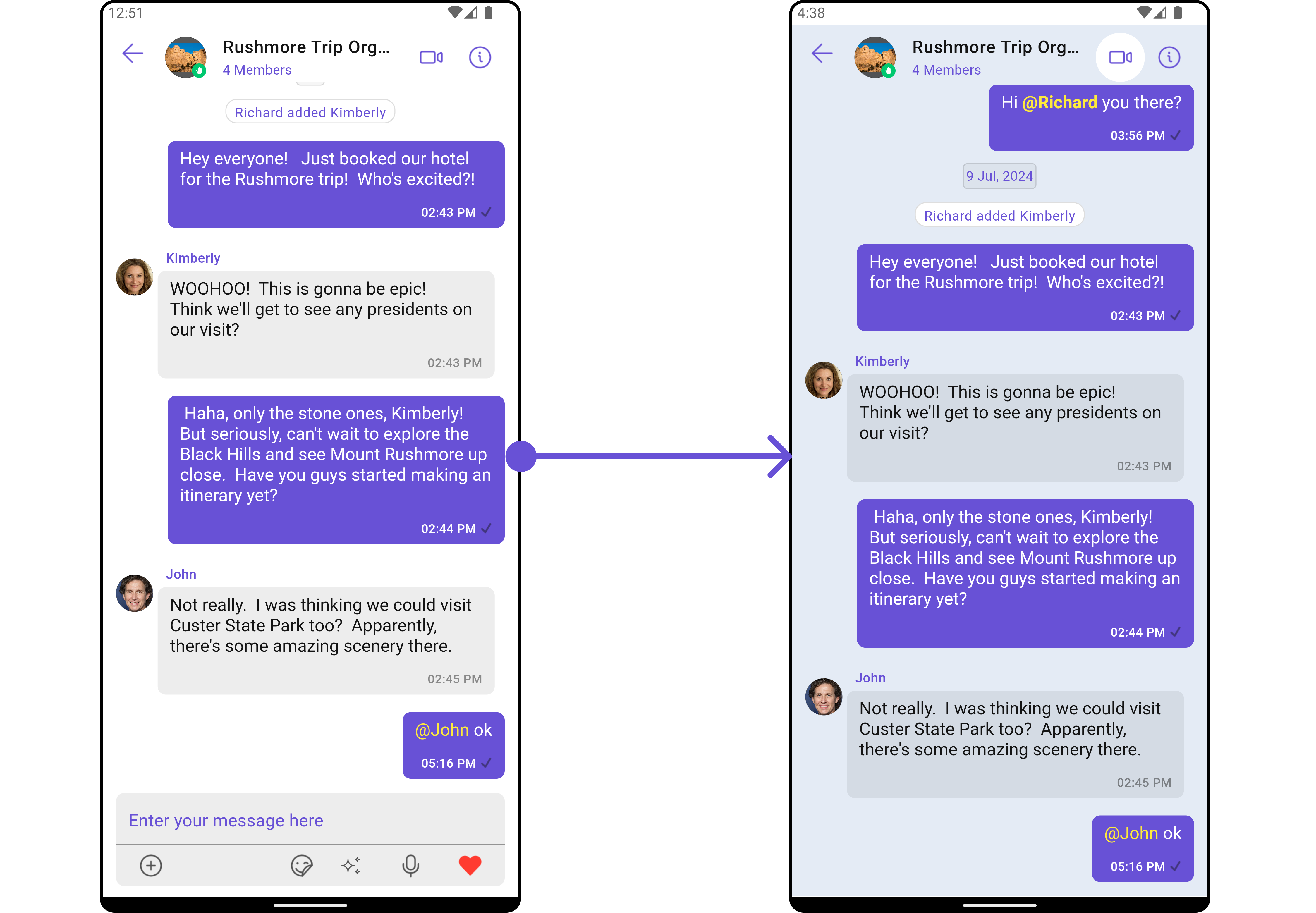
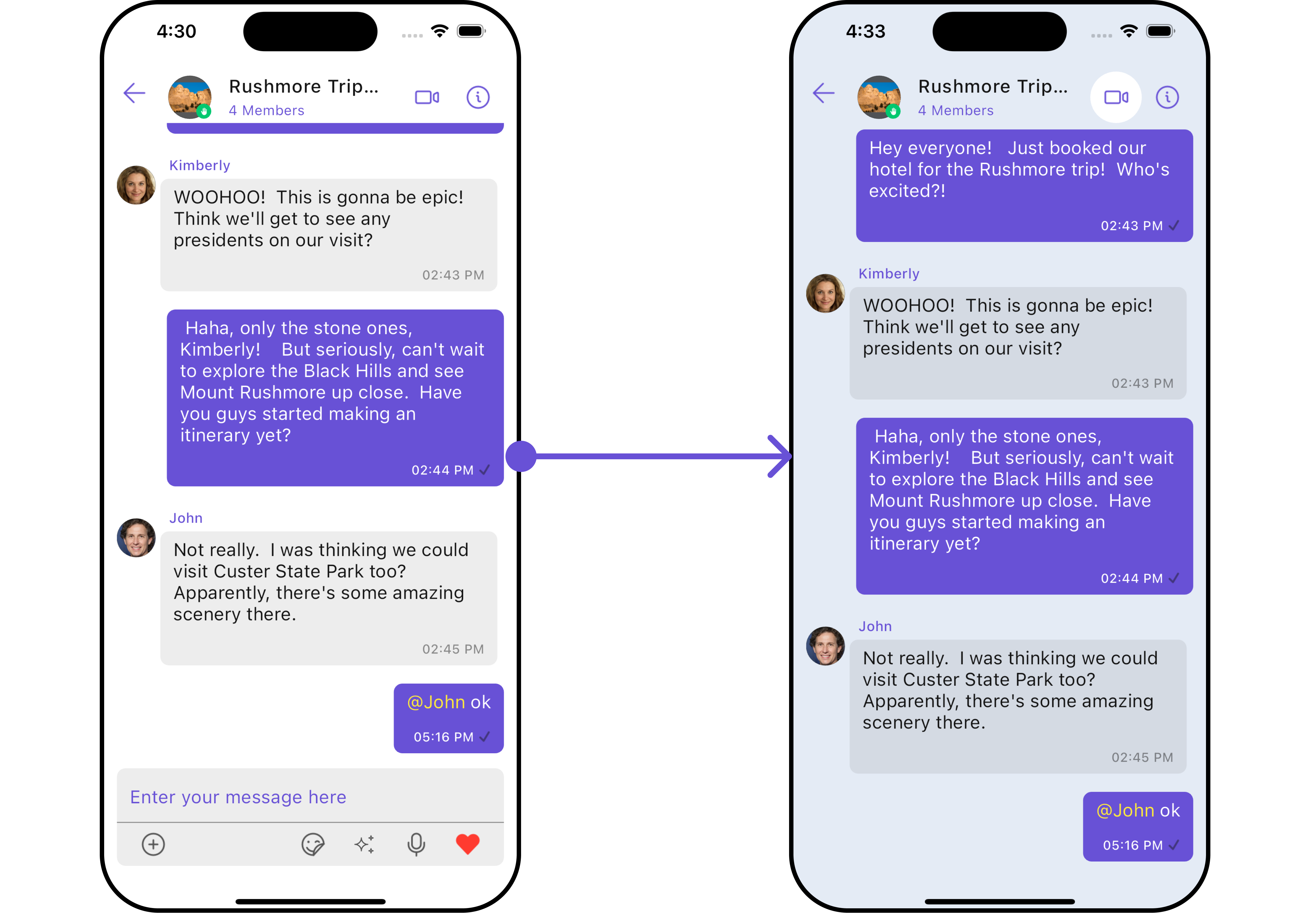
Join Protected Group
You can customize the properties of the Join Group widget by making use of the JoinGroupConfiguration. You can accomplish this by employing the JoinProtectedGroupConfiguration
class as demonstrated below:
- Dart
CometChatGroupsWithMessages(
joinProtectedGroupConfiguration: JoinProtectedGroupConfiguration(
// TODO("Not yet implemented")
),
)
All exposed properties of JoinProtectedGroupConfiguration
can be found under Join Group. Properties marked with the report symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Join Group sub widget.
You can modify the style using the JoinProtectedGroupStyle
property.
- Dart
CometChatGroupsWithMessages(
joinProtectedGroupConfiguration: JoinProtectedGroupConfiguration(
joinProtectedGroupStyle: JoinProtectedGroupStyle(
background: Color(0xFFE4EBF5),
titleStyle: TextStyle(color: Colors.red)
)
),
)
- Android
- iOS
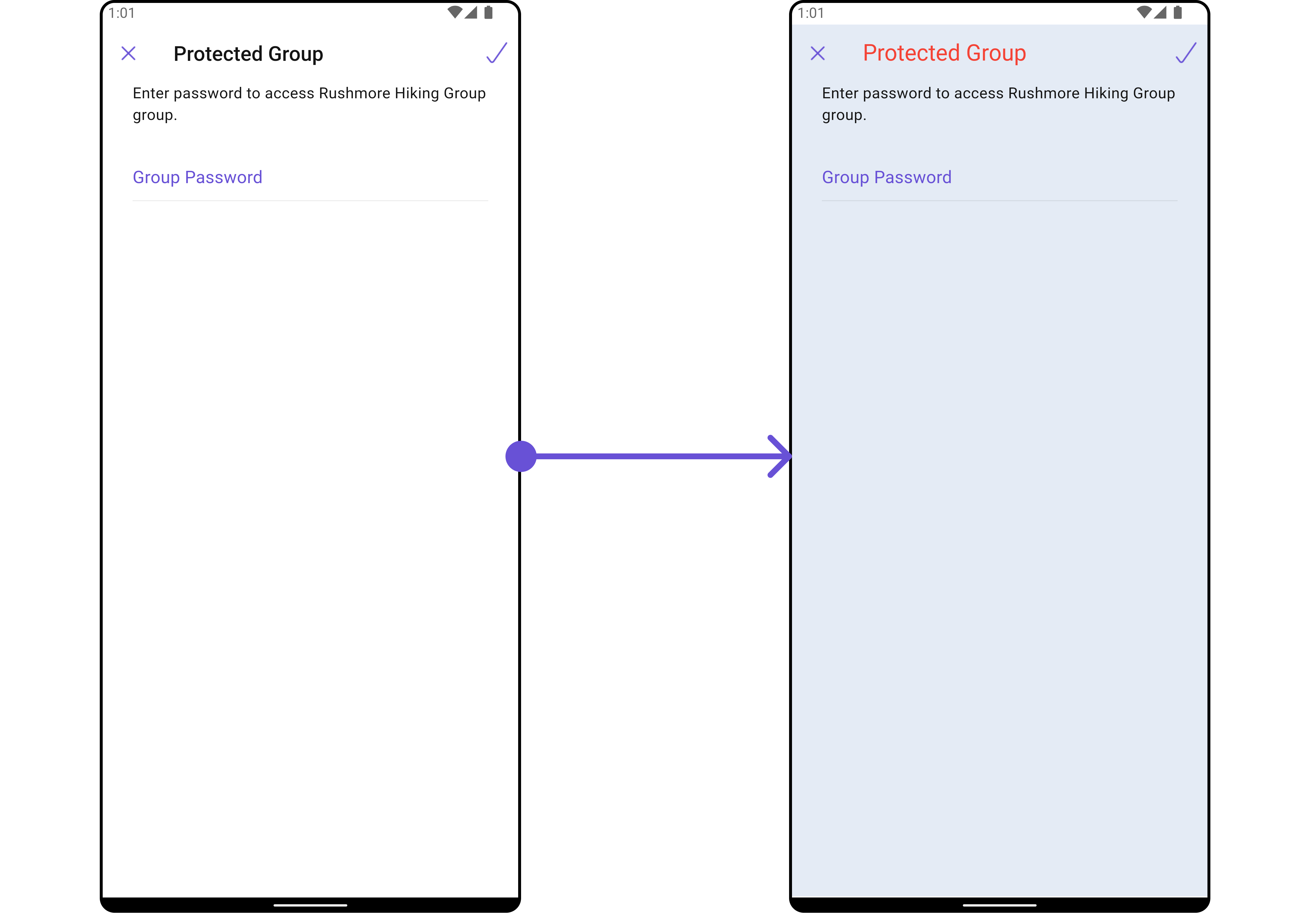
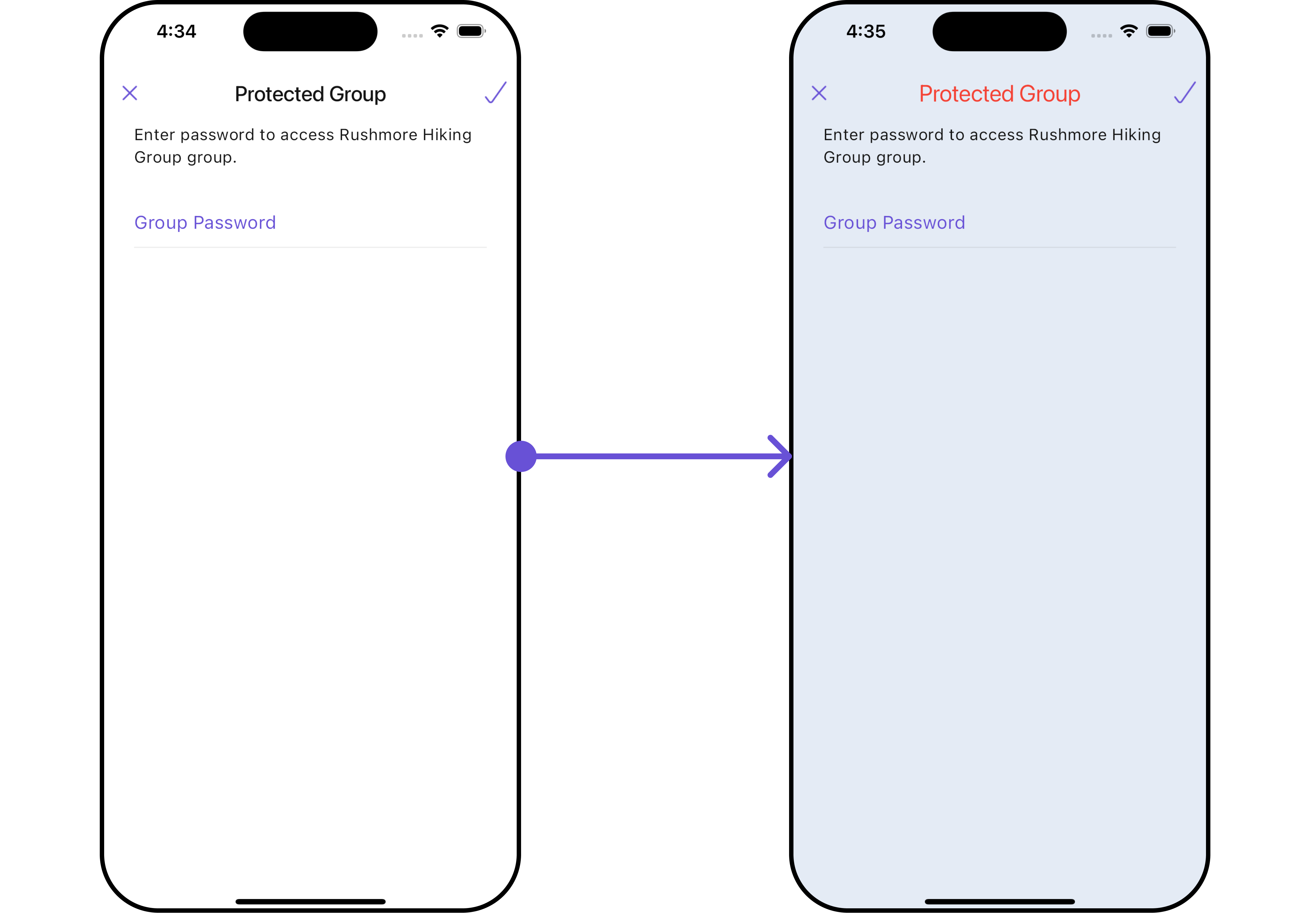
Create Group
You can customize the properties of the Create Group widget by making use of the CreateGroupConfiguration. You can accomplish this by employing the createGroupConfiguration
props as demonstrated below:
- Dart
CometChatGroupsWithMessages(
createGroupConfiguration: CreateGroupConfiguration(
// TODO("Not yet implemented")
),
)
All exposed properties of CreateGroupConfiguration
can be found under Create Group. Properties marked with the symbol are not accessible within the Configuration Object.
Example
Let's say you want to change the style of the Create Group sub widget.
You can modify the style using the createGroupStyle
property.
- Dart
CometChatGroupsWithMessages(
createGroupConfiguration: CreateGroupConfiguration(
createGroupStyle: CreateGroupStyle(
background: Color(0xFFE4EBF5),
titleTextStyle: TextStyle(color: Colors.red, fontFamily: "PlaywritePL")
)
),
)
- Android
- iOS
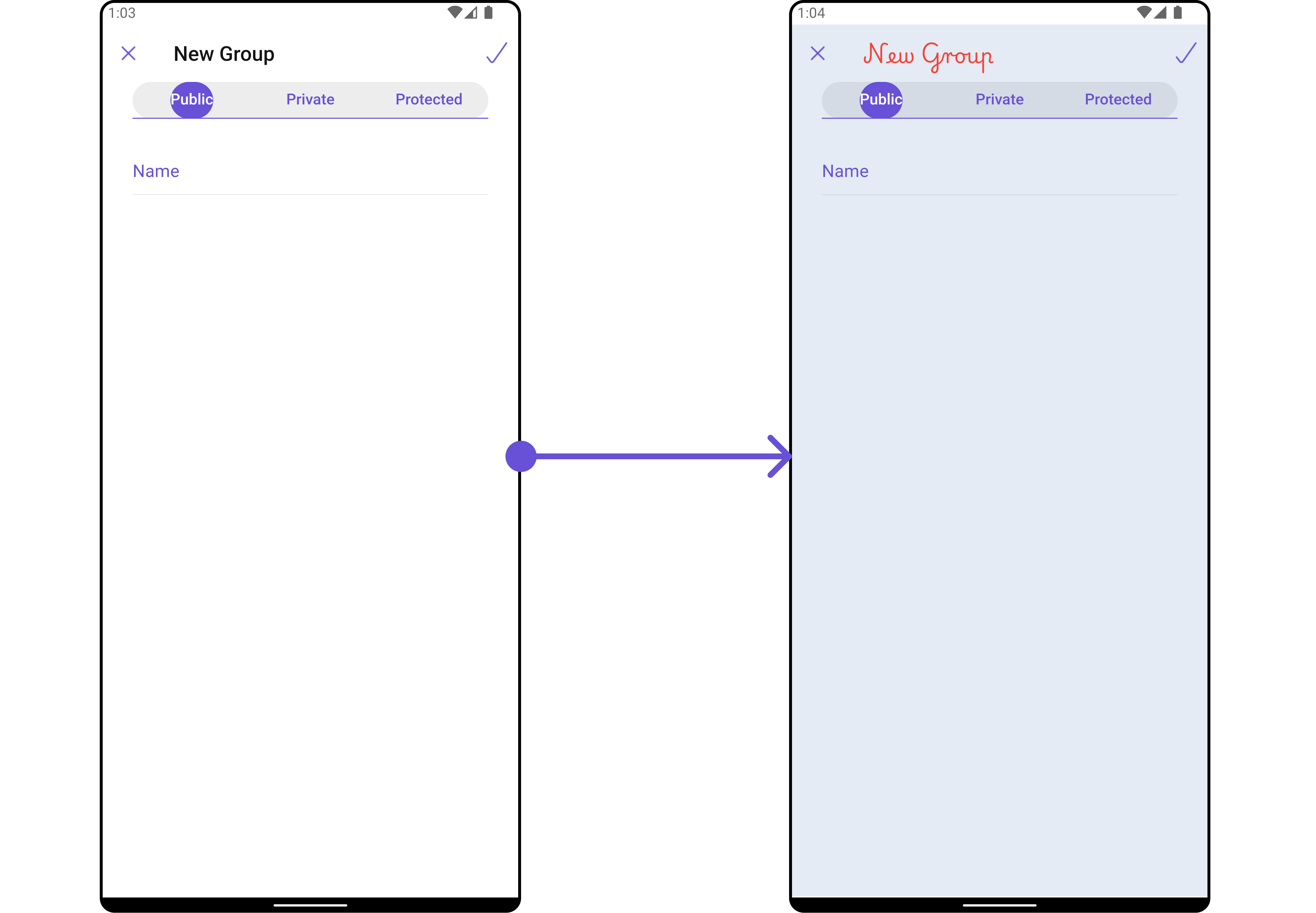
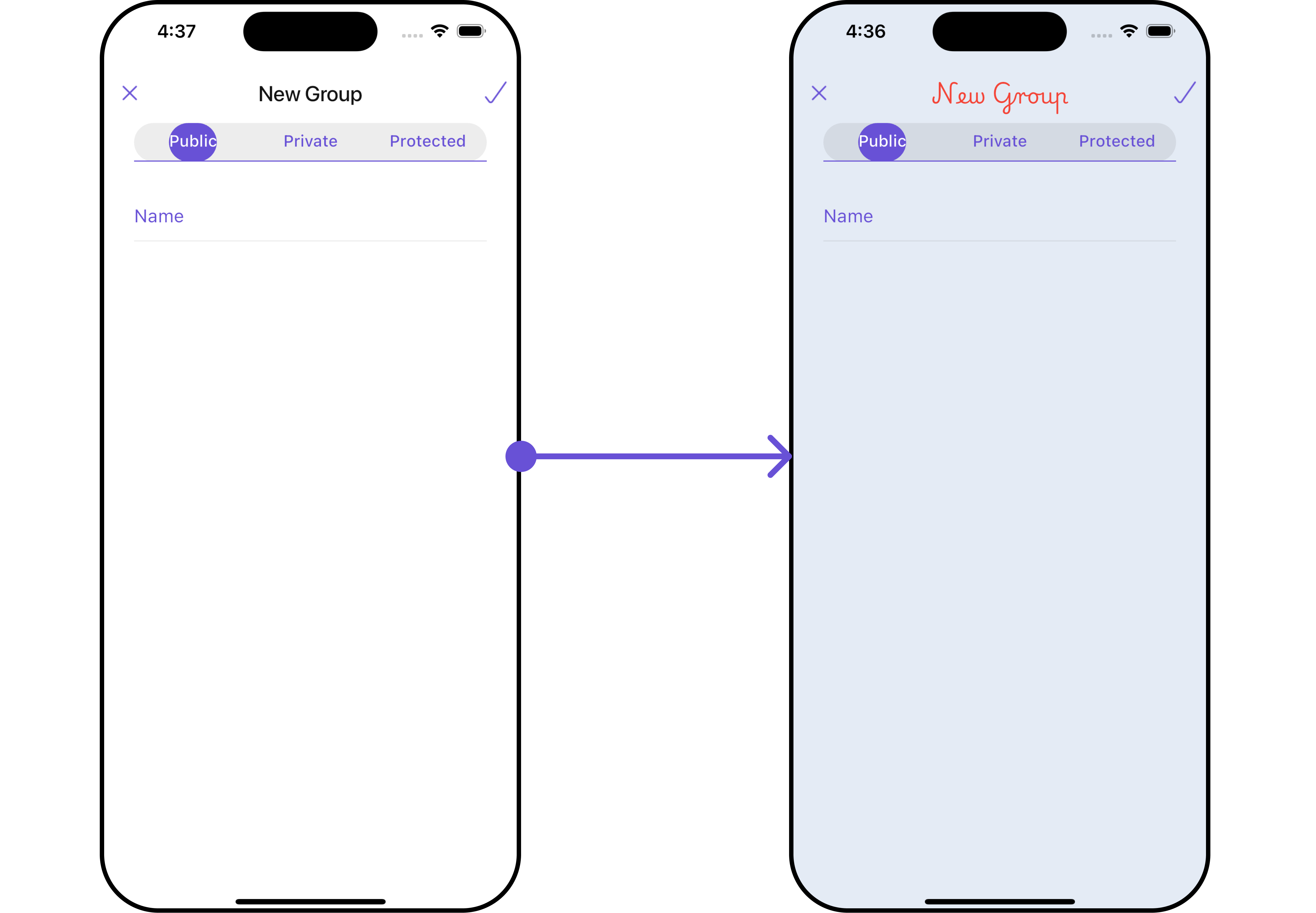