Message List
Overview
MessageList
is a Composite Component that displays a list of messages and effectively manages real-time operations. It includes various types of messages such as Text Messages, Media Messages, Stickers, and more.
MessageList
is primarily a list of the base component MessageBubble. The MessageBubble Component is utilized to create different types of chat bubbles depending on the message type.
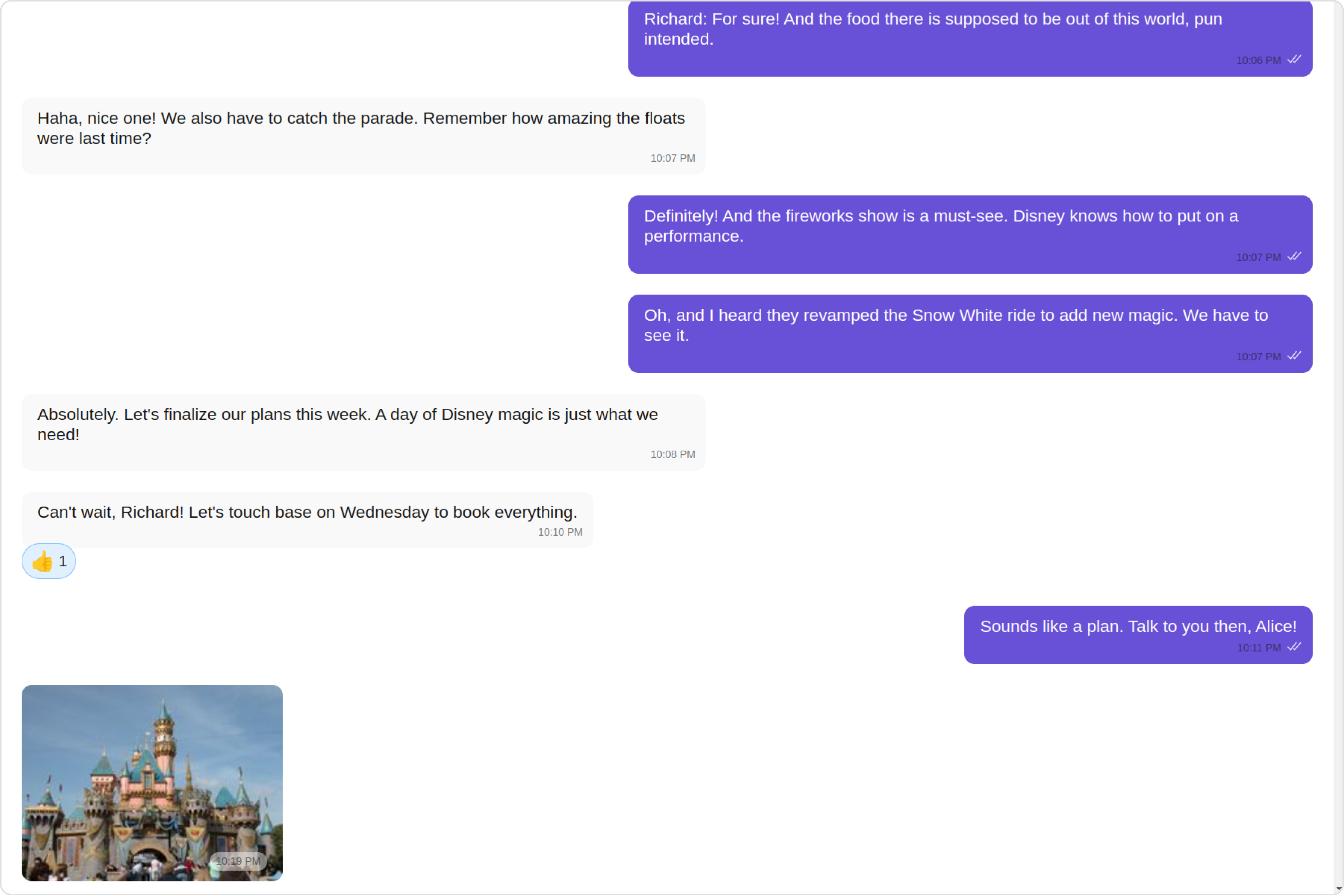
Usage
Integration
The following code snippet illustrates how you can directly incorporate the MessageList component into your Application.
- app.module.ts
- app.component.ts
- app.component.html
import { CUSTOM_ELEMENTS_SCHEMA, NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { CometChatMessageList } from "@cometchat/chat-uikit-angular";
import { AppComponent } from "./app.component";
@NgModule({
imports: [BrowserModule, CometChatMessageList],
declarations: [AppComponent],
providers: [],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA],
})
export class AppModule {}
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
></cometchat-message-list>
</div>
To fetch messages for a specific entity, you need to supplement it with User
or Group
Object.
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onThreadRepliesClick
onThreadRepliesClick
is triggered when you click on the threaded message bubble.
The onThreadRepliesClick
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- app.component.ts
- app.component.html
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public handleOnThreadRepliesClick = ()=>{
console.log("your custom on thread replies click");
};
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[onThreadRepliesClick]="handleOnThreadRepliesClick"
></cometchat-message-list>
</div>
2. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the MessageList component.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public handleOnError = (error: CometChat.CometChatException) => {
console.log("your custom on error action", error);
};
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[onError]="handleOnError"
></cometchat-message-list>
</div>
Filters
You can adjust the MessagesRequestBuilder
in the MessageList Component to customize your message list. Numerous options are available to alter the builder to meet your specific needs. For additional details on MessagesRequestBuilder
, please visit MessagesRequestBuilder.
In the example below, we are applying a filter to the messages based on a search substring and for a specific user. This means that only messages that contain the search term and are associated with the specified user will be displayed
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
messagesRequestBuilder = new CometChat.MessagesRequestBuilder().setLimit(5);
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[messagesRequestBuilder]="messagesRequestBuilder"
></cometchat-message-list>
</div>
The following parameters in messageRequestBuilder will always be altered inside the message list
- UID
- GUID
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The list of events emitted by the Message List component is as follows.
Event | Description |
---|---|
ccOpenChat | this event alerts the listeners if the logged-in user has opened a user or a group chat |
ccMessageEdited | Triggers whenever a loggedIn user edits any message from the list of messages .it will have three states such as: inProgress, success and error |
ccMessageDeleted | Triggers whenever a loggedIn user deletes any message from the list of messages |
ccActiveChatChanged | This event is triggered when the user navigates to a particular chat window. |
ccMessageRead | Triggers whenever a loggedIn user reads any message. |
ccLiveReaction | Triggers whenever a loggedIn clicks on live reaction |
Adding CometChatMessageEvents
Listener's
- TypeScript
import {CometChatMessageEvents} from "@cometchat/chat-uikit-angular";
this.ccOpenChat = CometChatMessageEvents.ccOpenChat.subscribe(
() => {
// Your Code
}
);
this.ccMessageEdited = CometChatMessageEvents.ccMessageEdited.subscribe(
() => {
// Your Code
}
);
this.ccMessageDeleted = CometChatMessageEvents.ccMessageDeleted.subscribe(
() => {
// Your Code
}
);
this.ccActiveChatChanged = CometChatMessageEvents.ccActiveChatChanged.subscribe(
() => {
// Your Code
}
);
this.ccMessageRead = CometChatMessageEvents.ccMessageRead.subscribe(
() => {
// Your Code
}
);
this.ccLiveReaction = CometChatMessageEvents.ccLiveReaction.subscribe(
() => {
// Your Code
}
);
Removing CometChatMessageEvents
Listener's
- TypeScript
this.ccMessageEdited.unsubscribe();
this.ccActiveChatChanged.unsubscribe();
Customization
To fit your app's design requirements, you can customize the appearance of the Message List component. We provide exposed properties that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. MessageList Style
You can set the MessageListStyle to the MessageList Component Component to customize the styling.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { MessageListStyle } from '@cometchat/uikit-shared';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
messageListStyle = new MessageListStyle({
background: "#fdf2ff",
border: "1px solid #d608ff",
borderRadius: "20px",
loadingIconTint: "red",
nameTextColor: "pink",
threadReplyTextColor: "green"
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[messageListStyle]="messageListStyle"
></cometchat-message-list>
</div>
List of properties exposed by MessageListStyle
Property | Description | Code |
---|---|---|
border | Used to set border | border?: string, |
borderRadius | Used to set border radius | borderRadius?: string; |
background | Used to set background colour | background?: string; |
height | Used to set height | height?: string; |
width | Used to set width | width?: string; |
loadingIconTint | used to set loading icon tint | loadingIconTint?: string; |
emptyStateTextFont | used to set empty state text font | emptyStateTextFont?: string; |
errorStateTextFont | used to set error state text font | errorStateTextFont?: string; |
emptyStateTextColor | used to set empty state text color | emptyStateTextColor?: string; |
errorStateTextColor | used to set error state text color | errorStateTextColor?: string; |
nameTextColor | used to set sender/receiver name text color on a message bubble. | nameTextColor?: string; |
nameTextFont | used to set sender/receiver name text font on a message bubble | nameTextFont?: string; |
TimestampTextColor | used to set time stamp text color | TimestampTextColor?: string; |
TimestampTextFont | used to set time stamp text font | TimestampTextFont?: string; |
threadReplyTextColor | used to set thread reply text color | threadReplyTextColor?: string; |
threadReplyTextFont | used to set thread reply text font | threadReplyTextFont?: string; |
threadReplyIconTint | used to set thread reply icon tint | threadReplyIconTint?: string; |
threadReplyUnreadTextColor | used to set thread reply unread text color | threadReplyUnreadTextColor?: string; |
threadReplyUnreadTextFont | used to set thread reply unread text font | threadReplyUnreadTextFont?: string; |
threadReplyUnreadBackground | used to set thread reply unread background | threadReplyUnreadBackground?: string; |
2. Avatar Style
To apply customized styles to the Avatar
component in the Message List
Component, you can use the following code snippet. For further insights on Avatar
Styles refer
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, AvatarStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
avatarStyle = new AvatarStyle({
backgroundColor:"#cdc2ff",
border:"2px solid #6745ff",
borderRadius:"10px",
outerViewBorderColor:"#ca45ff",
outerViewBorderRadius:"5px",
nameTextColor:"#4554ff"
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[avatarStyle]="avatarStyle"
></cometchat-message-list>
</div>
3. DateSeparator Style
To apply customized styles to the DateSeparator
in the Message list
Component, you can use the following code snippet.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, DateStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
dateSeparatorStyle = new DateStyle({
background:'red',
border:'2px solid green',
borderRadius:'15px'
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[dateSeparatorStyle]="dateSeparatorStyle"
></cometchat-message-list>
</div>
4. EmojiKeyboard Style
To apply customized styles to the EmojiKeyBoard
in the Message list
Component, you can use the following code snippet.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, EmojiKeyboardStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
emojiKeyboardStyle = new EmojiKeyboardStyle({
background:'red',
border:'2px solid green',
borderRadius:'15px',
activeIconTint:'yellow',
textColor:'#8830f2'
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[emojiKeyboardStyle]="emojiKeyboardStyle"
></cometchat-message-list>
</div>
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[hideError]="true"
[hideReceipt]="true"
></cometchat-message-list>
</div>
Below is a list of customizations along with corresponding code snippets
Advance
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
Templates
CometChatMessageTemplate is a pre-defined structure for creating message views that can be used as a starting point or blueprint for creating message views often known as message bubbles. For more information, you can refer to CometChatMessageTemplate.
You can set message Templates to MessageList by using the following code snippet
DateSeparatorPattern
You can customize the date pattern of the message list separator using the DateSeparatorPattern
prop. Choose from predefined options like time, DayDate, DayDateTime, or DateTime.
Example
Default
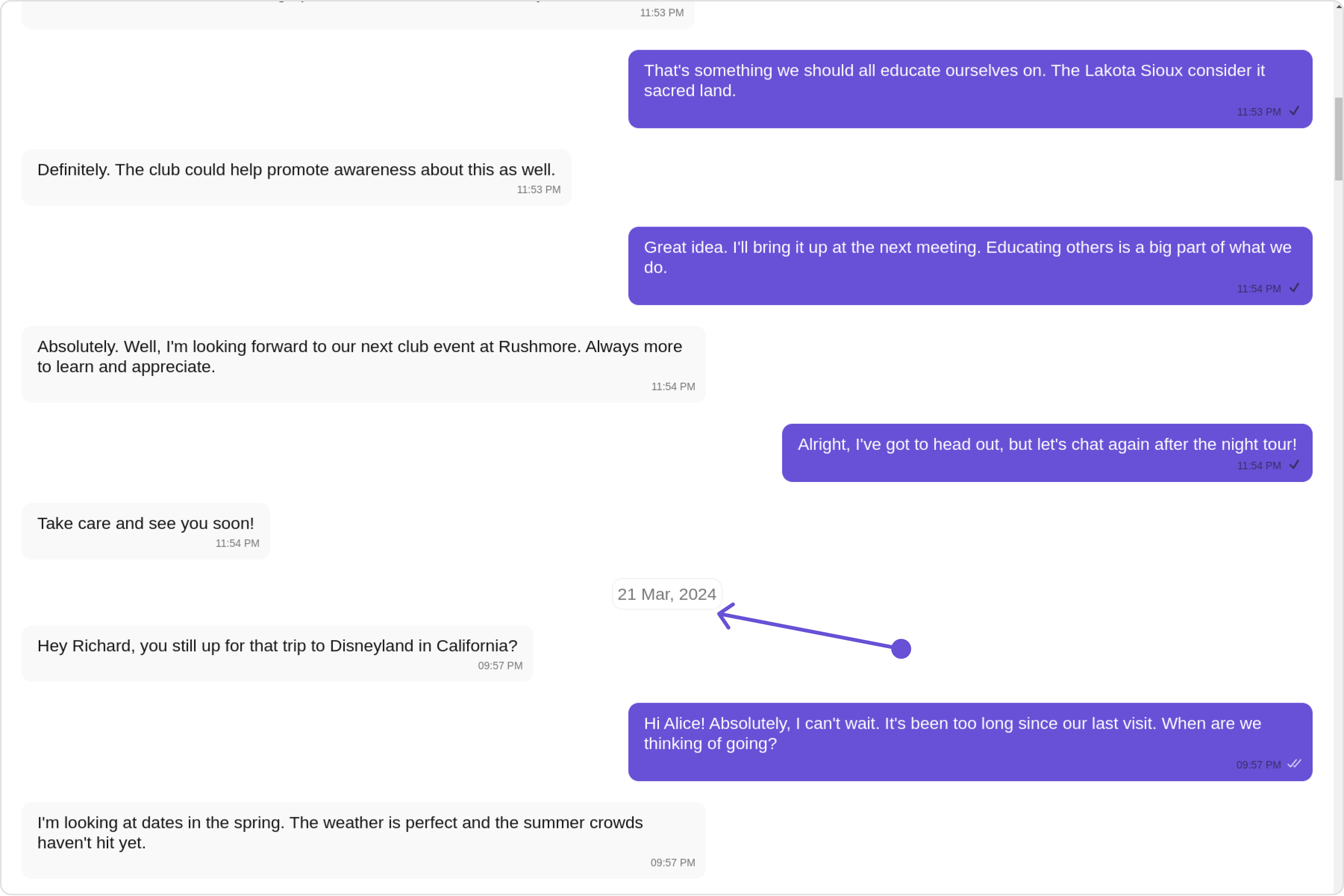
Custom
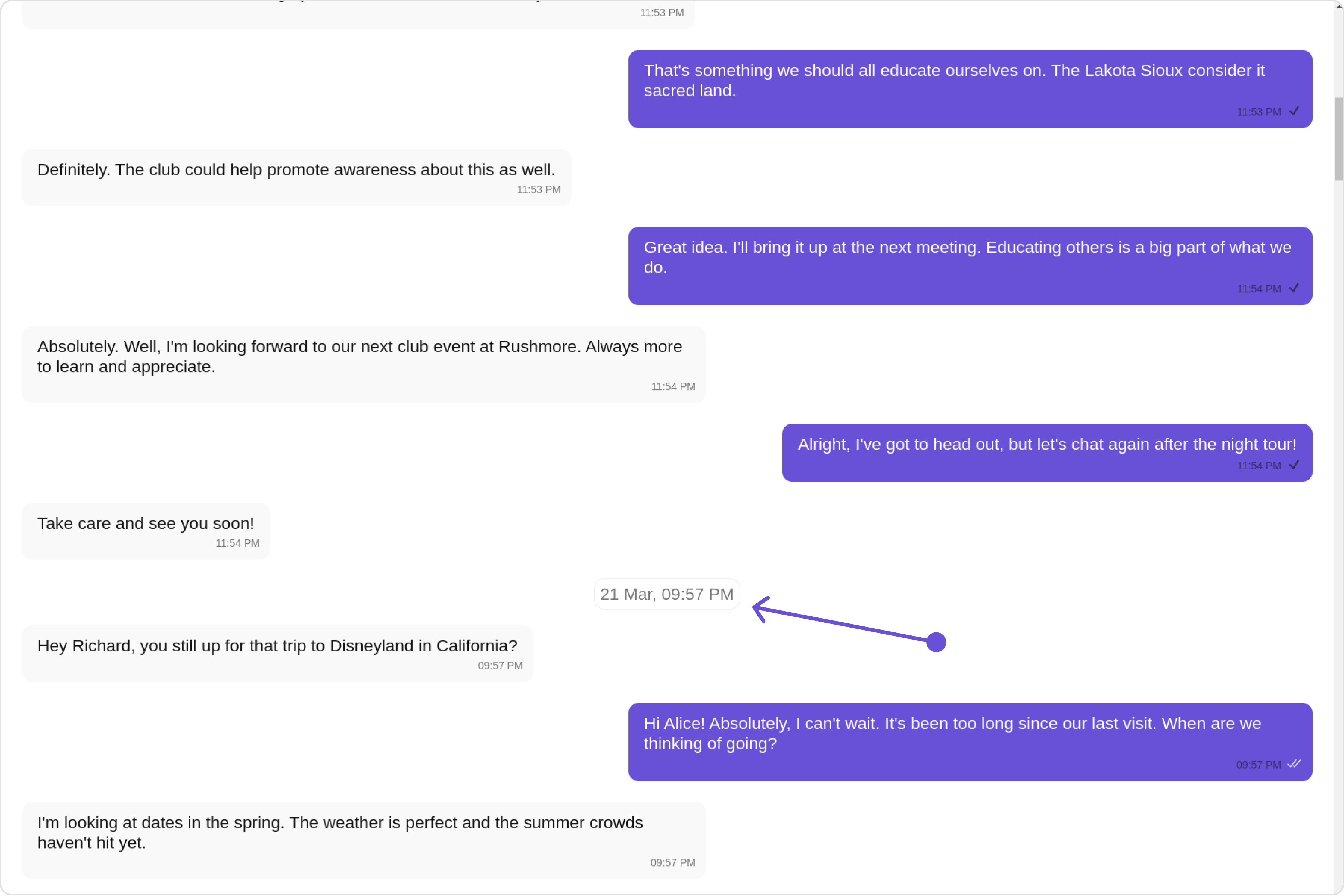
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { DatePattern } from '@cometchat/uikit-resources';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
DateSeparatorPattern = DatePatterns.DateTime;
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[DateSeparatorPattern]="DateSeparatorPattern"
></cometchat-message-list>
</div>
DatePattern
You can modify the date pattern to your requirement using DatePattern. Choose from predefined options like time, DayDate, DayDateTime, or DateTime.
DatePatterns describes a specific format or arrangement used to represent dates in a human-readable form.
Name | Description |
---|---|
time | Date format displayed in the format hh:mm a |
DayDate | Date format displayed in the following format.
|
DayDateTime | Date format displayed in the following format.
|
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { DatePattern } from '@cometchat/uikit-resources';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
DatePattern = DatePatterns.DateTime;
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[DatePattern]="DatePattern"
></cometchat-message-list>
</div>
Headerview
You can set custom headerView to the Message List component using the following method.
Example
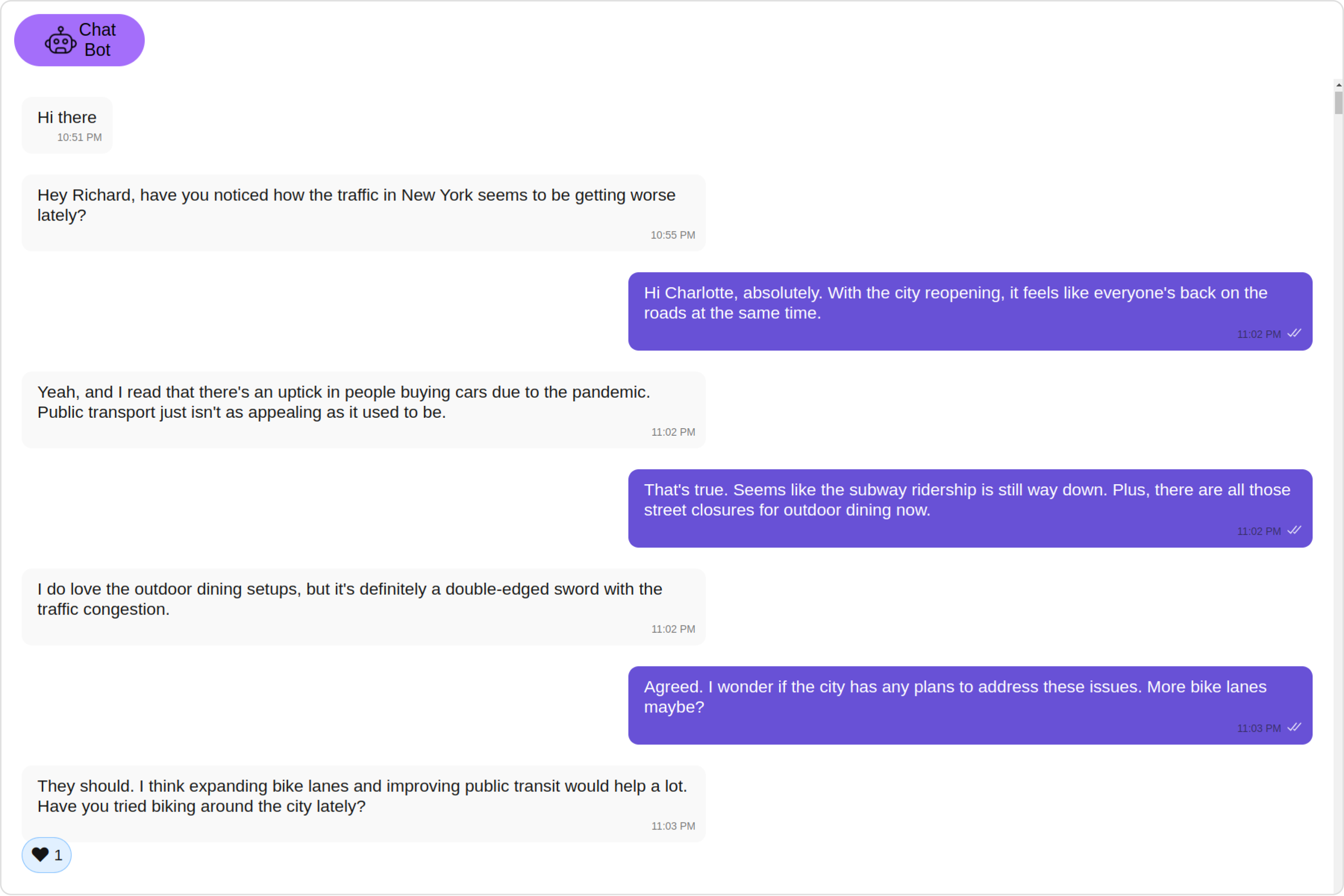
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[headerView]="headerView"
></cometchat-message-list>
</div>
<ng-template #headerView>
<div
[ngStyle]="{ height: '40px', width: '100px', background: '#a46efa', borderRadius: '20px', display: 'flex', justifyContent: 'center', alignItems: 'center', margin: '10px' }"
>
<button
[ngStyle]="{ height: '40px', width: '40px', background: '#a46efa', border: 'none', display: 'flex', justifyContent: 'center', alignItems: 'center', cursor: 'pointer' }"
>
<img
src="img"
[ngStyle]="{ height: 'auto', width: '100%', maxWidth: '100%', maxHeight: '100%', borderRadius: '50%' }"
alt="bot"
/>
<span>Chat Bot</span>
</button>
</div>
</ng-template>
FooterView
You can set custom footerview to the Message List component using the following method.
Example
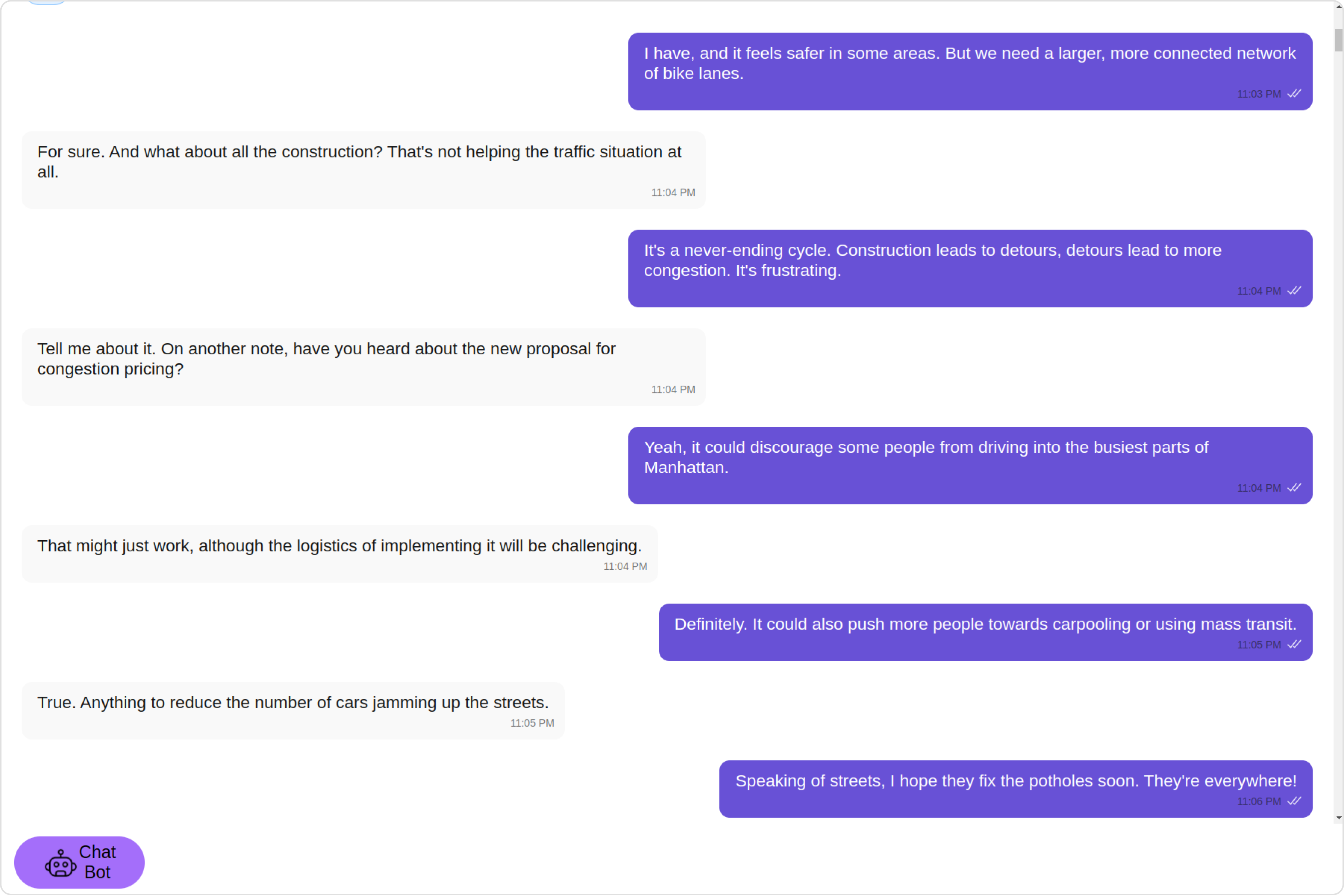
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[footerView]="footerView"
></cometchat-message-list>
</div>
<ng-template #footerView>
<div
[ngStyle]="{ height: '40px', width: '100px', background: '#a46efa', borderRadius: '20px', display: 'flex', justifyContent: 'center', alignItems: 'center', margin: '10px' }"
>
<button
[ngStyle]="{ height: '40px', width: '40px', background: '#a46efa', border: 'none', display: 'flex', justifyContent: 'center', alignItems: 'center', cursor: 'pointer' }"
>
<img
src="img"
[ngStyle]="{ height: 'auto', width: '100%', maxWidth: '100%', maxHeight: '100%', borderRadius: '50%' }"
alt="bot"
/>
<span>Chat Bot</span>
</button>
</div>
</ng-template>
ErrorStateView
You can set a custom errorStateView
to match the error view of your app.
Example
Default
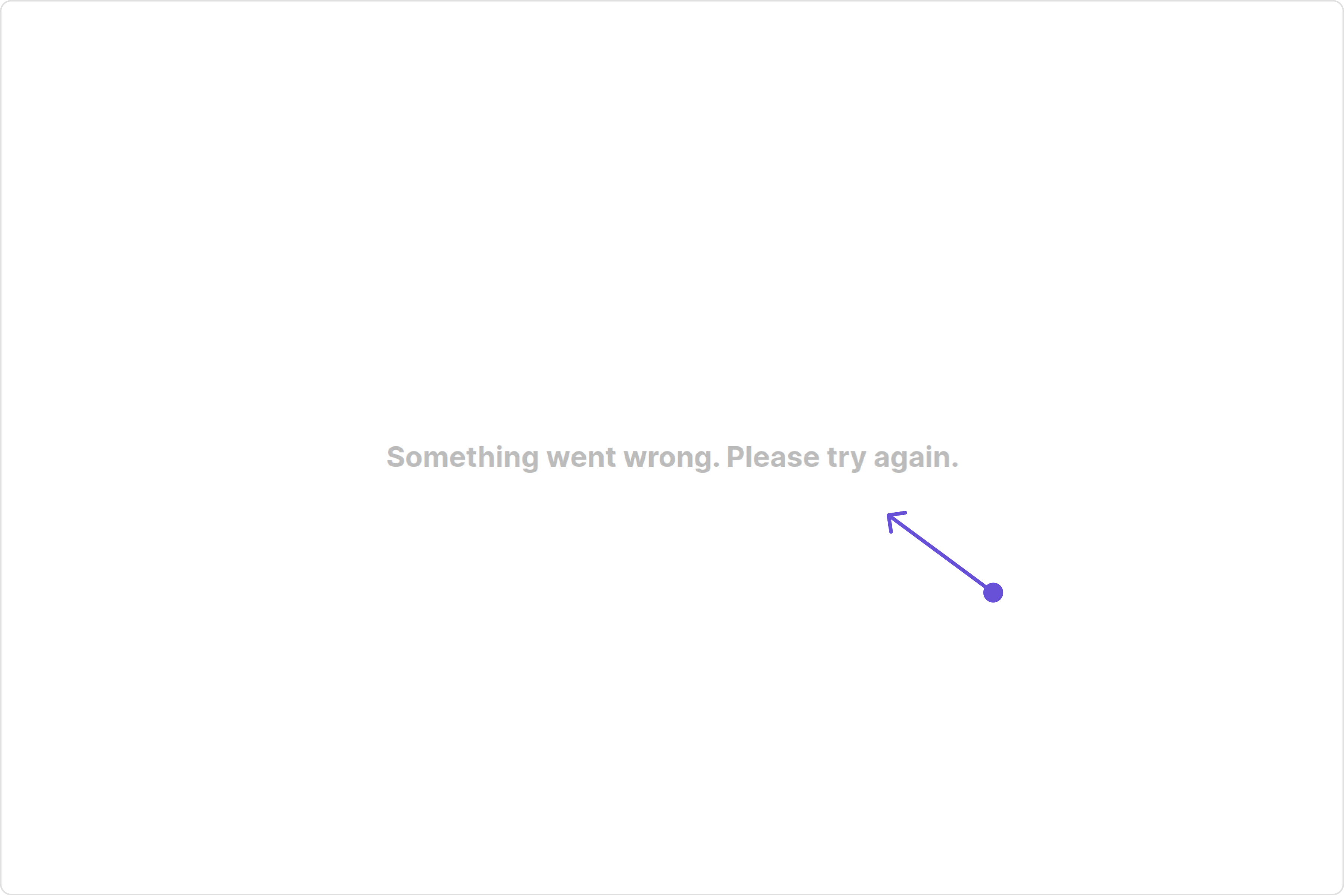
Custom
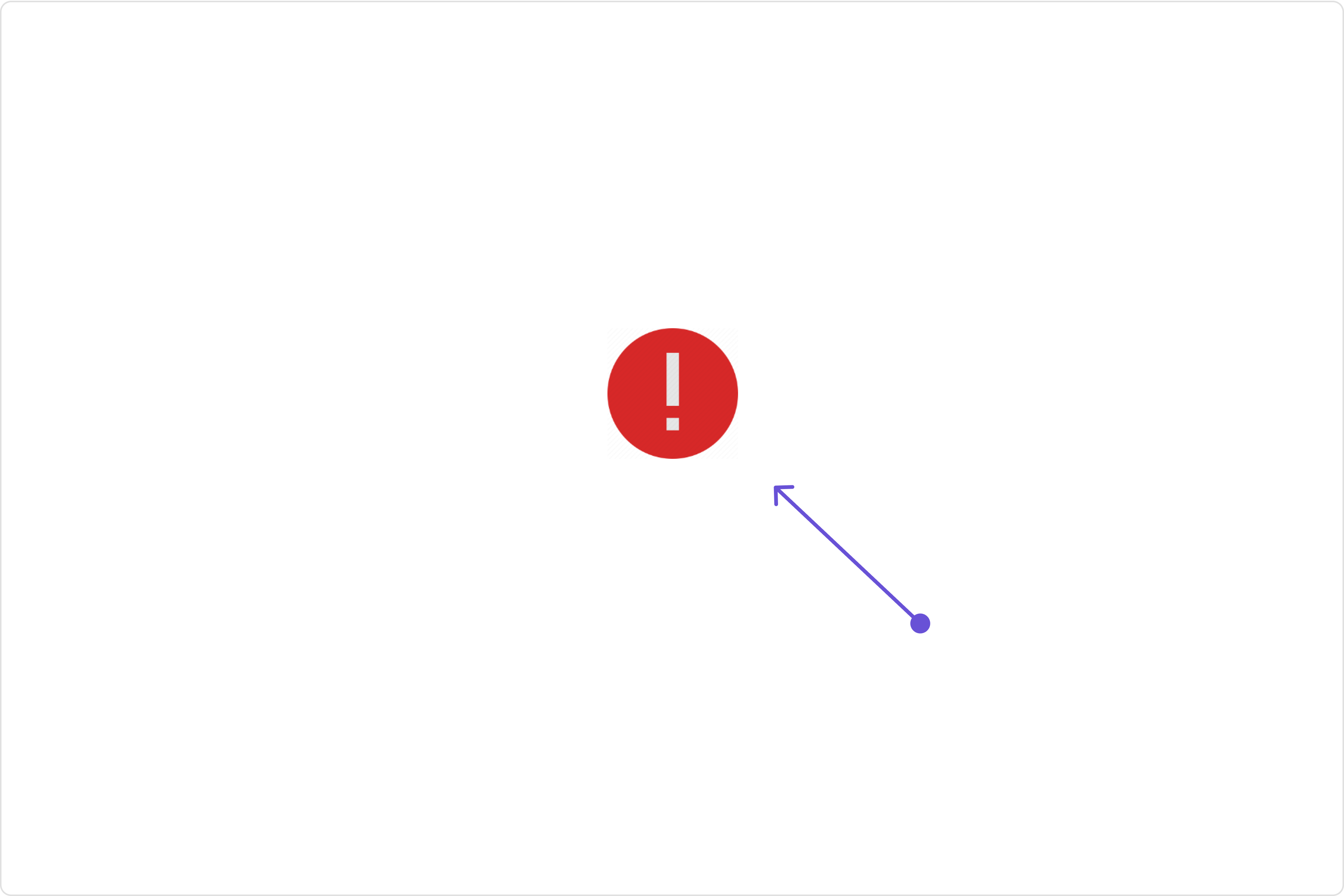
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[errorStateView]="errorStateView"
></cometchat-message-list>
</div>
<ng-template #errorStateView>
<div style="height: 100vh; width: 100vw">
<img
src="https://cdn0.iconfinder.com/data/icons/shift-interfaces/32/Error-512.png"
alt="error icon"
style="height:100px; width: 100px; justify-content: center"
/>
</div>
</ng-template>
EmptyStateView
The emptyStateView
method provides the ability to set a custom empty state view in your app. An empty state view is displayed when there are no messages for a particular user.
Example
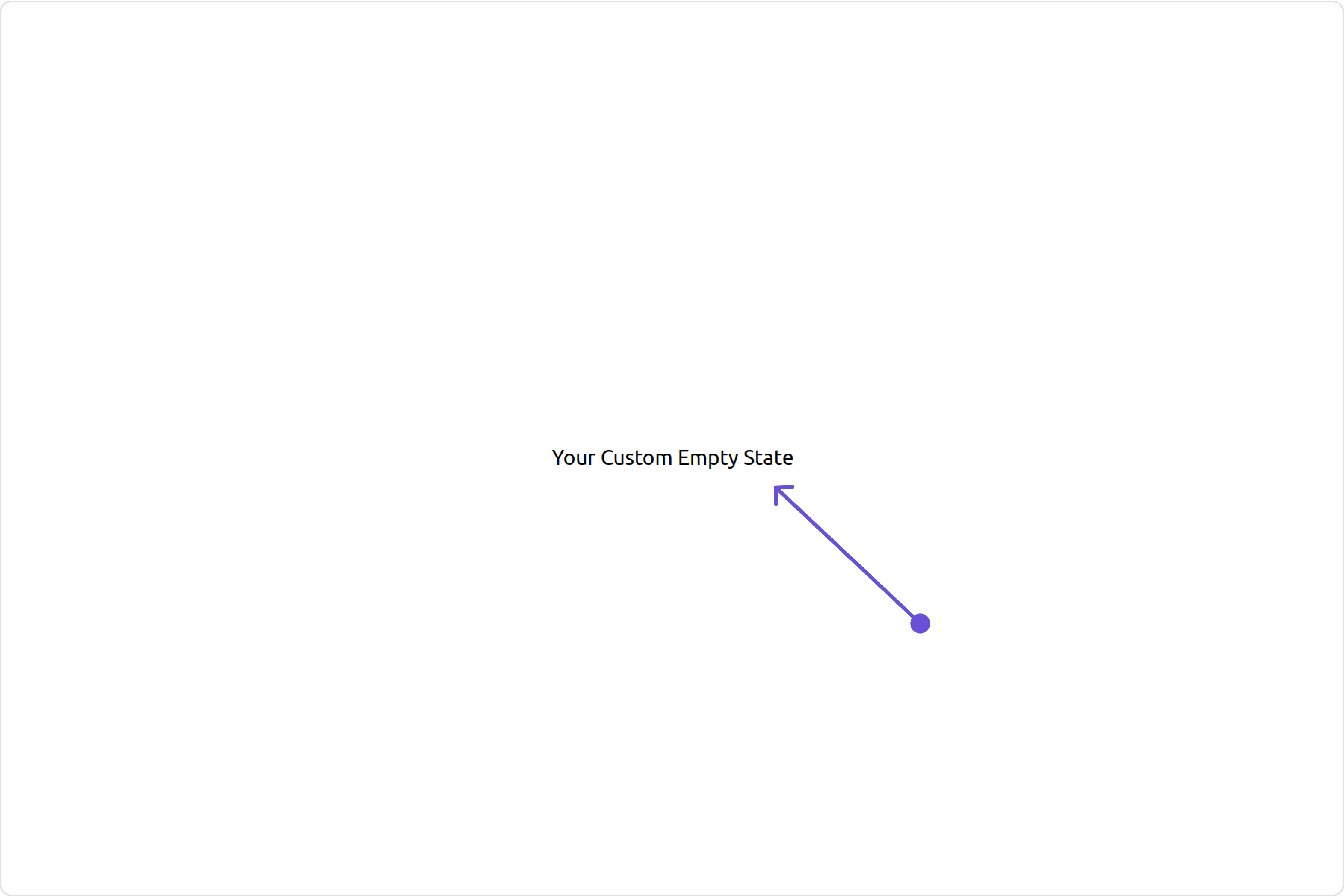
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[emptyStateView]="emptyStateView"
></cometchat-message-list>
</div>
<ng-template #emptyStateView>
<div>Your Custom Empty State</div>
</ng-template>
LoadingStateView
The loadingStateView
property allows you to set a custom loading view in your app. This feature enables you to maintain a consistent look and feel throughout your application,
Example
Default
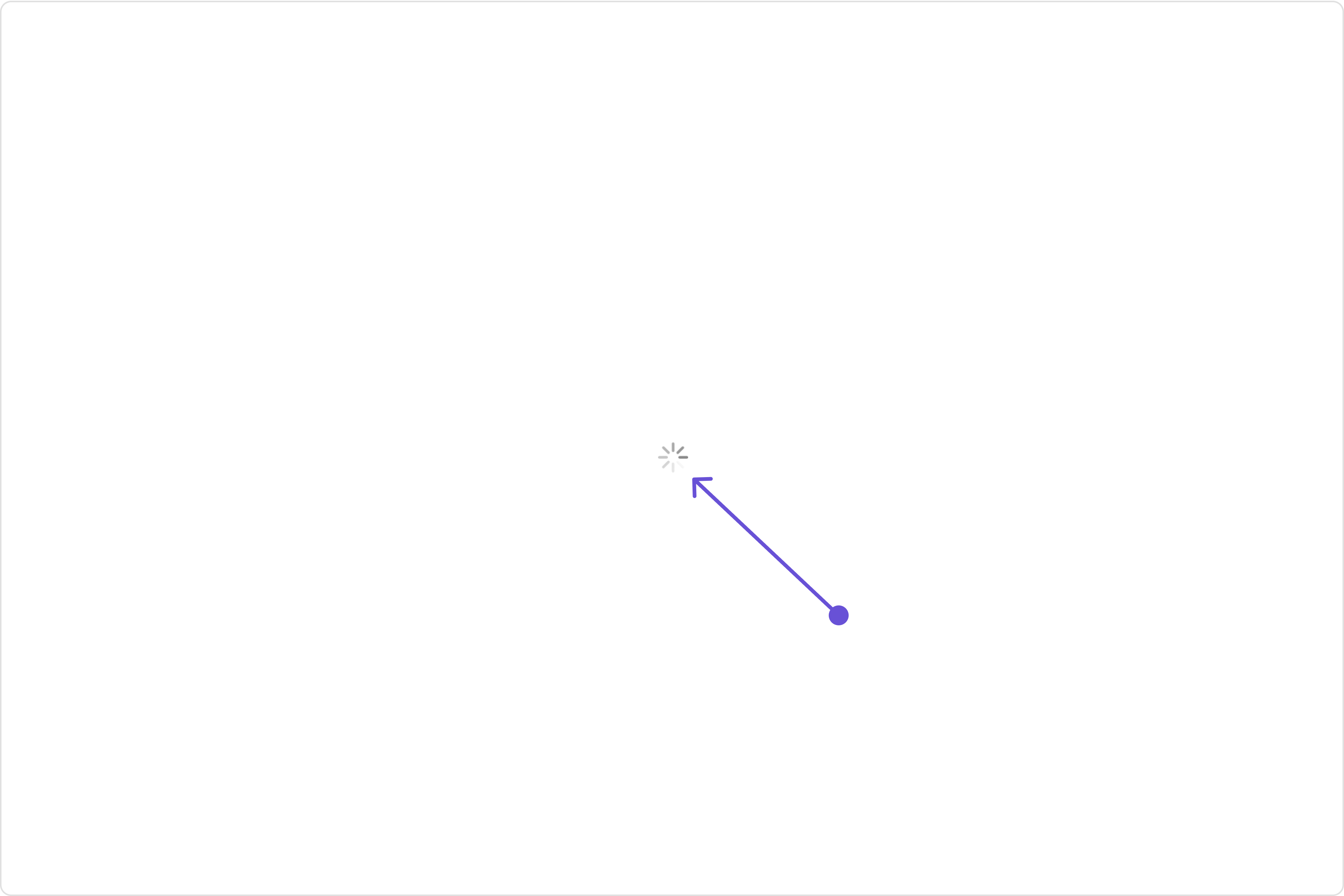
Custom
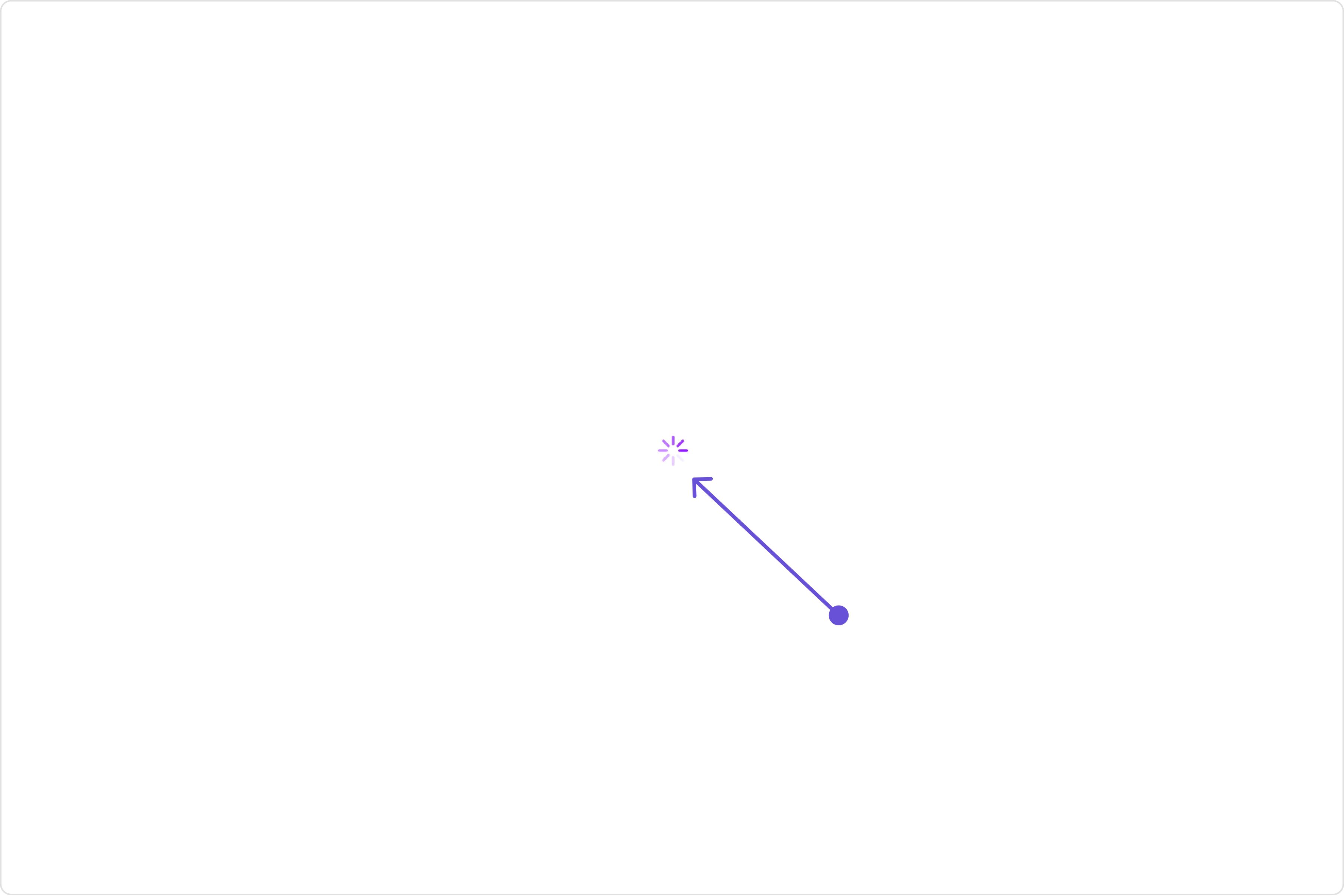
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, LoaderStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
getLoaderStyle: LoaderStyle = new LoaderStyle({
iconTint: "red",
background: "transparent",
height: "20px",
width: "20px",
border: "none",
borderRadius: "0",
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[loadingStateView]="loadingStateView"
></cometchat-message-list>
</div>
<ng-template #loadingStateView>
<cometchat-loader
iconURL="icon"
loaderStyle="getLoaderStyle()"
></cometchat-loader>
</ng-template>
TextFormatters
Assigns the list of text formatters. If the provided list is not null, it sets the list. Otherwise, it assigns the default text formatters retrieved from the data source. To configure the existing Mentions look and feel check out CometChatMentionsFormatter
- app.component.ts
- HashTagTextFormatter.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
import HashTagTextFormatter from '../HashTagTextFormatter';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
public textFormatters = [new HashTagTextFormatter];
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
import { CometChatTextFormatter } from "@cometchat/uikit-shared";
class HashTagTextFormatter extends CometChatTextFormatter {
constructor() {
super();
// Set the tracking character to #
this.setTrackingCharacter("#");
// Define regex patterns to find specific text patterns
this.setRegexPatterns([
/\B#(\w+)\b/g, // Matches hashtags starting with #
]);
// Define regex patterns to replace formatter text with original text
this.setRegexToReplaceFormatting([
/#(\w+)/g, // Replace hashtags without formatting
]);
// Set callback functions for key up and key down events
this.setKeyUpCallBack(this.onKeyUp.bind(this));
this.setKeyDownCallBack(this.onKeyDown.bind(this));
// Set the re-render callback function
this.setReRender(() => {
// Trigger re-rendering of the message composer component
// This could involve updating the UI with the formatted text
console.log("Re-rendering message composer to update text content.");
});
// Initialize composer tracking
this.initializeComposerTracking();
}
initializeComposerTracking() {
// Get the reference to the input field (composer)
const composerInput = document.getElementById("yourComposerInputId");
// Set the reference to the input field
this.setInputElementReference(composerInput);
}
getCaretPosition(): number {
if (!this.inputElementReference) return 0;
const selection = window.getSelection();
if (!selection || selection.rangeCount === 0) return 0;
const range = selection.getRangeAt(0);
const clonedRange = range.cloneRange();
clonedRange.selectNodeContents(this.inputElementReference);
clonedRange.setEnd(range.endContainer, range.endOffset);
return clonedRange.toString().length;
}
setCaretPosition(position: number) {
if (!this.inputElementReference) return;
const range = document.createRange();
const selection = window.getSelection();
if (!selection) return;
range.setStart(
this.inputElementReference.childNodes[0] || this.inputElementReference,
position
);
range.collapse(true);
selection.removeAllRanges();
selection.addRange(range);
}
override onKeyUp(event: KeyboardEvent) {
if (event.key === this.trackCharacter) {
this.startTracking = true;
}
// Custom logic to format hashtags as users type in the composer
if (this.startTracking && (event.key === " " || event.key === "Enter")) {
const caretPosition = this.getCaretPosition();
this.formatText();
this.setCaretPosition(caretPosition);
}
// Check if the last character typed was not a space or enter
// and if the caret position is at the end, then we need to set start tracking to false
if (
this.startTracking &&
event.key !== " " &&
event.key !== "Enter" &&
this.getCaretPosition() === this.inputElementReference?.innerText?.length
) {
this.startTracking = false;
}
}
formatText() {
const inputValue =
this.inputElementReference?.innerText ||
this.inputElementReference?.textContent ||
"";
const formattedText = this.getFormattedText(inputValue);
// Update the composer with formatted text
if (this.inputElementReference) {
this.inputElementReference.innerHTML = formattedText || "";
// Trigger re-render of the composer
this.reRender();
}
}
override onKeyDown(event: KeyboardEvent) {
// Custom onKeyDown logic can be added here
}
override getFormattedText(inputText: string) {
if (!inputText) {
// Edit at cursor position and return void
return;
}
// Perform custom logic to format text (e.g., formatting hashtags)
return this.customLogicToFormatText(inputText);
}
customLogicToFormatText(inputText: string) {
// Replace hashtags with HTML span elements to change color to green
return inputText.replace(
/\B#(\w+)\b/g,
'<span style="color: #5dff05;">#$1</span>'
);
}
override getOriginalText(inputText: string) {
// Sample implementation
if (!inputText) {
return "";
}
// Remove formatting and return original text
for (let i = 0; i < this.regexToReplaceFormatting.length; i++) {
let regexPattern = this.regexToReplaceFormatting[i];
if (inputText) {
inputText = inputText.replace(regexPattern, "#$1");
}
}
return inputText;
}
}
export default HashTagTextFormatter;
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[textFormatters]="textFormatters"
></cometchat-message-list>
</div>
Configuration
Configurations offer the ability to customize the properties of each component within a Composite Component.
MessageInformation
From the MessageList, you can navigate to the MesssageInformation component as shown in the image.
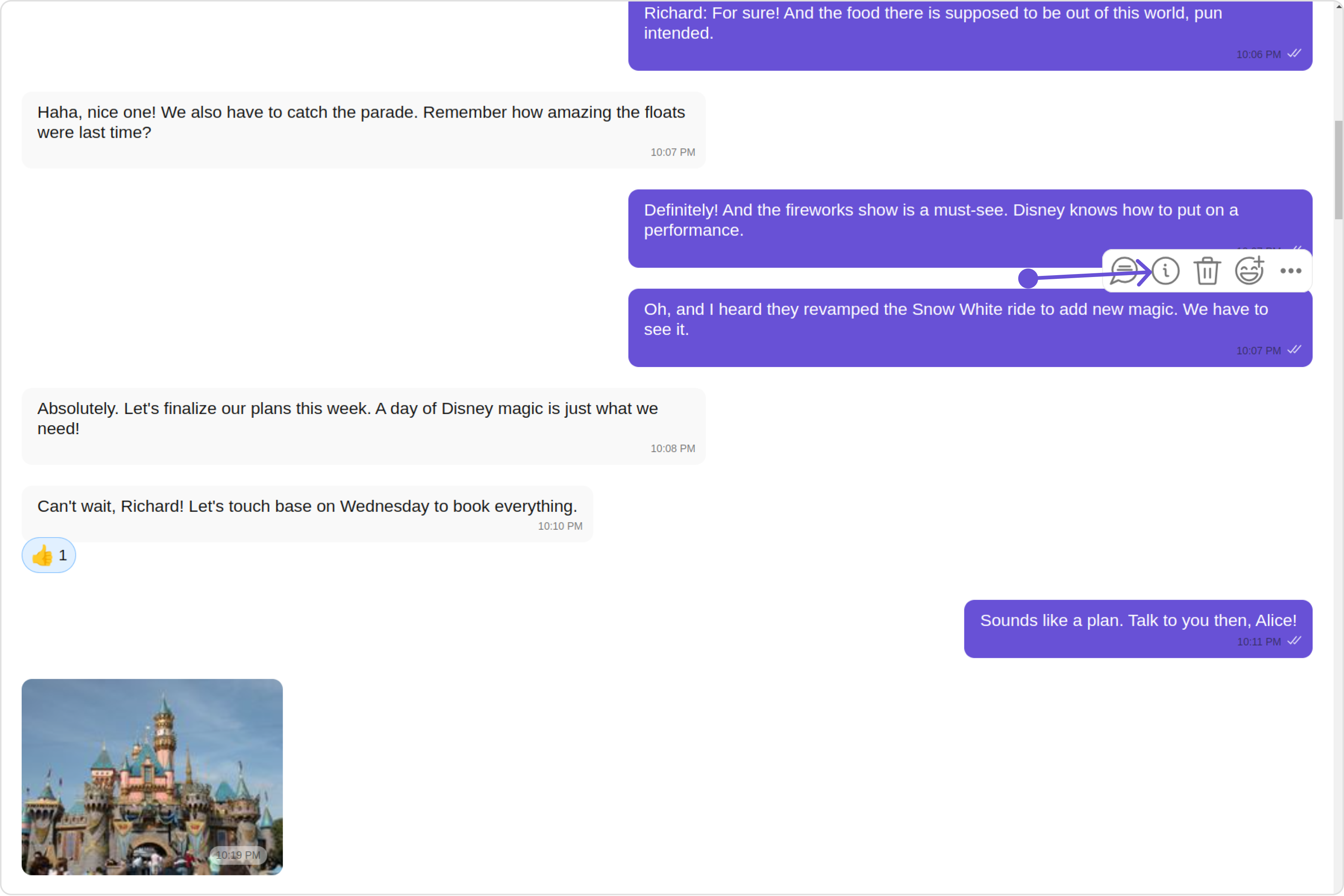
If you wish to modify the properties of the MesssageInformation Component, you can use the MessageInformationConfiguration
object.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { MessageInformationConfiguration, MessageInformationStyle } from '@cometchat/uikit-shared';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public messageInformationConfiguration = new MessageInformationConfiguration({
messageInformationStyle: new MessageInformationStyle({
background: "#f7f2fa",
border: "2px solid #d895fc",
borderRadius: "20px",
captionTextColor: "#8629e3",
titleTextColor: "#908deb",
})
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[messageInformationConfiguration]="messageInformationConfiguration"
></cometchat-message-list>
</div>
The MessageInformationConfiguration
indeed provides access to all the Action, Filters, Styles, Functionality, and Advanced properties of the MesssageInformation component.
Please note that the properties marked with the symbol are not accessible within the Configuration Object.
Example
Default
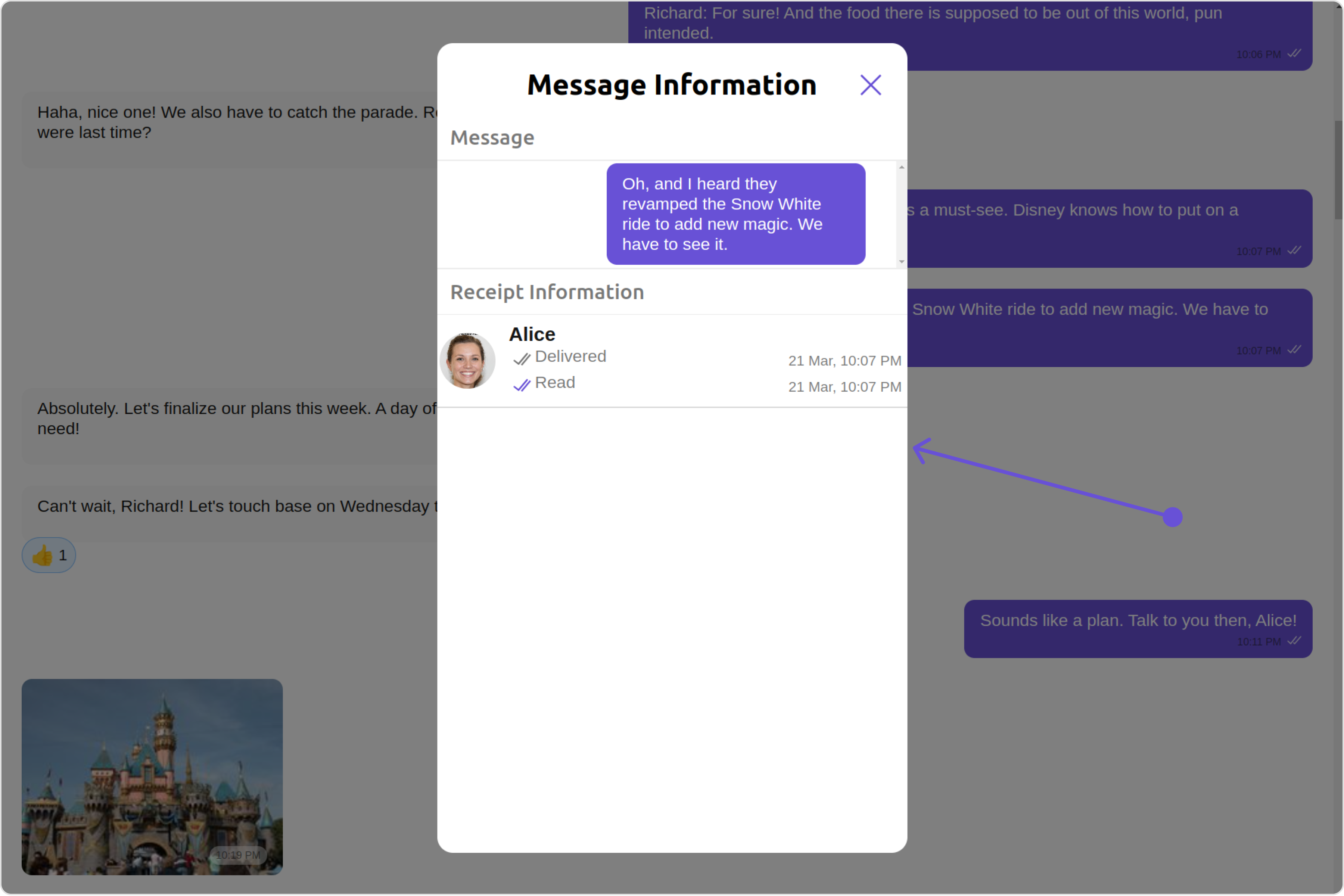
Custom
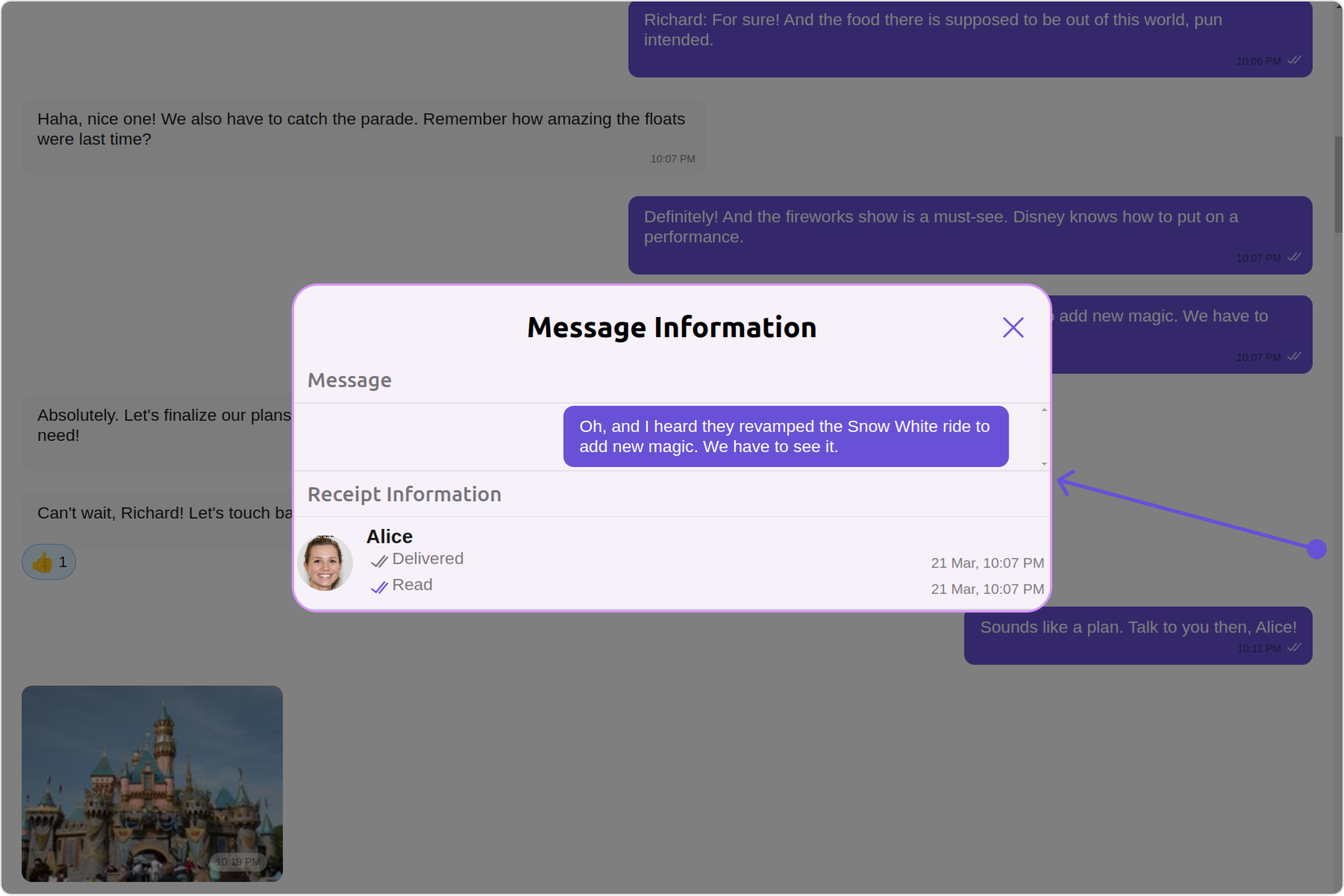
In the above example, we are styling a few properties of the MesssageInformation component using MessageInformationConfiguration
.
Reaction
If you wish to modify the properties of the Reaction Component, you can use the reactionsConfiguration
object.
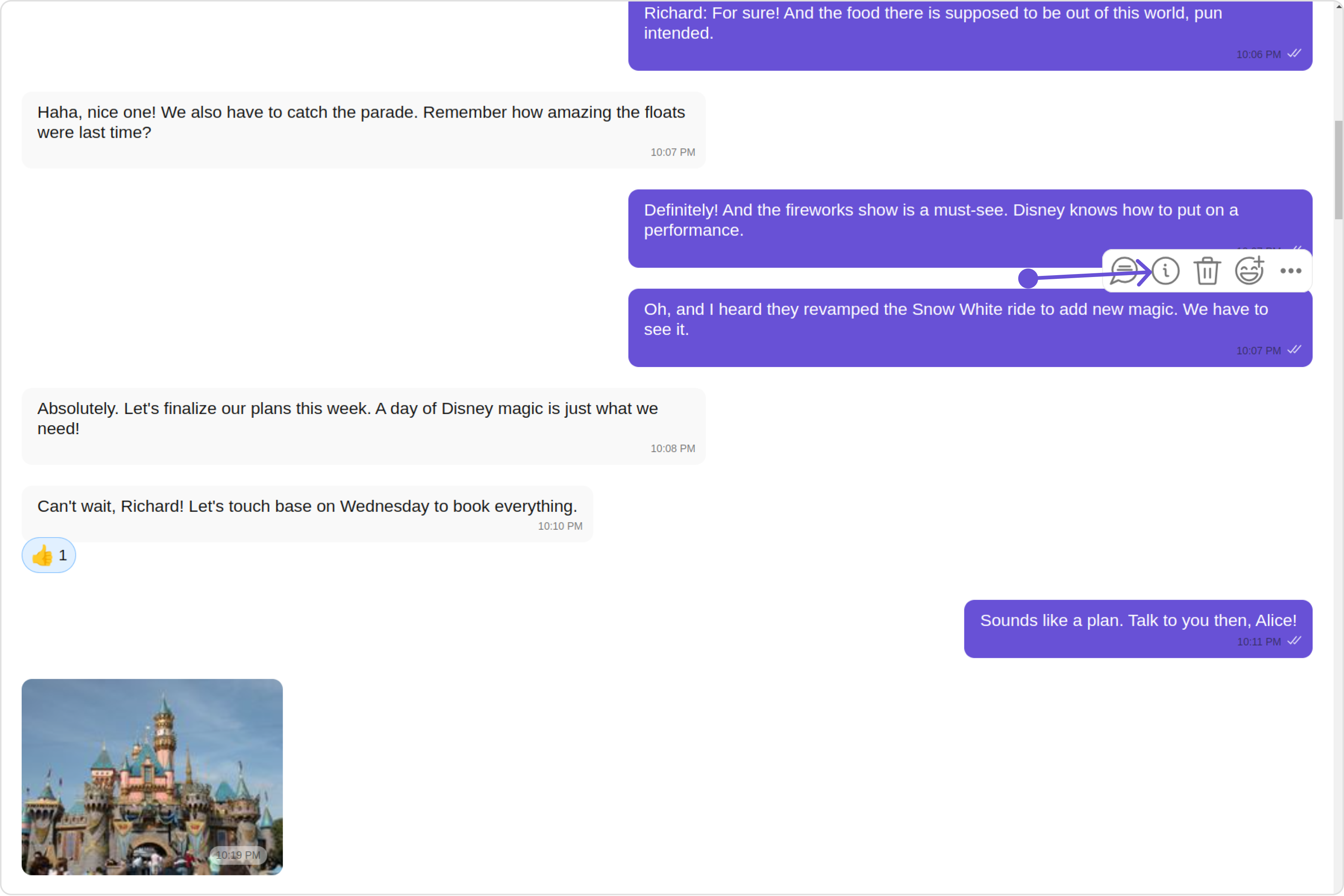
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { ReactionsConfiguration, ReactionsStyle } from '@cometchat/uikit-shared';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
ngOnInit(): void {
CometChat.getUser("uid").then((user:CometChat.User)=>{
this.userObject=user;
});
}
public userObject!: CometChat.User;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public reactionsConfiguration = new ReactionsConfiguration({
reactionsStyle: new ReactionsStyle({
border:'2px solid #8742f5',
activeReactionBackground:'#b88cff',
background:'#7b34ed',
baseReactionBackground:'#ebe3ff',
borderRadius:'20px'
})
//properties of reactions
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-message-list
*ngIf="userObject"
[user]="userObject"
[reactionsConfiguration]="reactionsConfiguration"
></cometchat-message-list>
</div>