Groups
Overview
The Groups is a Component, showcasing an accessible list of all available groups. It provides an integral search functionality, allowing you to locate any specific groups swiftly and easily. For each group listed, the group name is displayed by default, in conjunction with the group icon when available. Additionally, it provides a useful feature by displaying the number of members in each group as a subtitle, offering valuable context about group size and activity level.
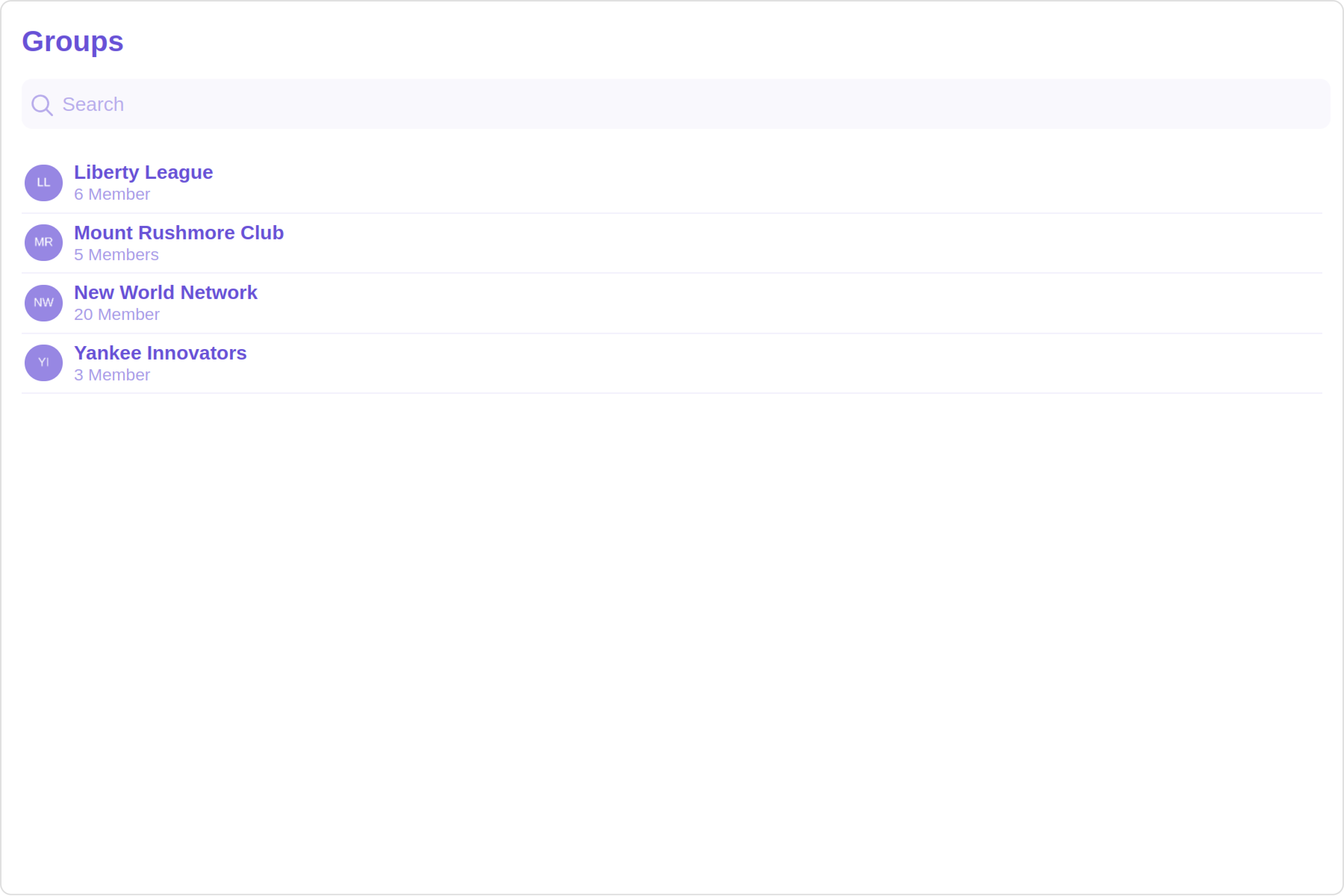
The Groups component is composed of the following BaseComponents:
Components | Description |
---|---|
CometChatList | a reusable container component having title, search box, customisable background and a List View |
CometChatListItem | a component that renders data obtained from a Group object on a Tile having a title, subtitle, leading and trailing view |
Usage
Integration
The following code snippet illustrates how you can directly incorporate the Groups component into your Application.
- app.module.ts
- app.component.ts
- app.component.html
import { CUSTOM_ELEMENTS_SCHEMA, NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { CometChatGroups } from "@cometchat/chat-uikit-angular";
import { AppComponent } from "./app.component";
@NgModule({
imports: [BrowserModule, CometChatGroups],
declarations: [AppComponent],
providers: [],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA],
})
export class AppModule {}
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
title = 'angular-app';
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups></cometchat-groups>
</div>
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onSelect
The onSelect
action is activated when you select the done icon while in selection mode. This returns the group
object along with the boolean flag selected
to indicate if the group was selected or unselected.
This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { SelectionMode } from '@cometchat/uikit-resources';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
public selectionMode: SelectionMode = SelectionMode.multiple;
public handleOnSelectGroup = (group: CometChat.Group, selected: boolean): void => {
console.log("Custom on select action", group, selected);
};
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups
[selectionMode]="selectionMode"
[onSelect]="handleOnSelectGroup"
></cometchat-groups>
</div>
2. onItemClick
The onItemClick
event is activated when you click on the Group List item. This action does not come with any predefined behavior. However, you have the flexibility to override this event and tailor it to suit your needs using the following code snippet.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
public handleOnItemClickGroup = (group: CometChat.Group)=> {
console.log("your custom on item click action", group);
};
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [onItemClick]="handleOnItemClickGroup"></cometchat-groups>
</div>
3. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the Groups component.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
public handleOnError = (error: CometChat.CometChatException) => {
console.log("your custom on error action", error);
};
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [onError]="handleOnError"></cometchat-groups>
</div>
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
1. GroupsRequestBuilder
The GroupsRequestBuilder enables you to filter and customize the group list based on available parameters in GroupsRequestBuilder. This feature allows you to create more specific and targeted queries when fetching groups. The following are the parameters available in GroupsRequestBuilder
Methods | Type | Description |
---|---|---|
setLimit | number | sets the number groups that can be fetched in a single request, suitable for pagination |
setSearchKeyword | String | used for fetching groups matching the passed string |
joinedOnly | boolean | to fetch only joined groups. |
setTags | Array<String> | used for fetching groups containing the passed tags |
withTags | boolean | used to fetch tags data along with the list of groups |
Example
In the example below, we are applying a filter to the Group List based on only joined groups and setting the limit to two.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
groupsRequestBuilder = new CometChat.GroupsRequestBuilder().setLimit(2).joinedOnly(true);
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups
[groupsRequestBuilder]="groupsRequestBuilder"
></cometchat-groups>
</div>
2. SearchRequestBuilder
The SearchRequestBuilder uses GroupsRequestBuilder enables you to filter and customize the search list based on available parameters in GroupsRequestBuilder. This feature allows you to keep uniformity between the displayed Groups List and searched Group List.
Example
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
searchRequestBuilder = new CometChat.GroupsRequestBuilder().setLimit(4).setSearchKeyword("your search keyword")
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups
[searchRequestBuilder]="searchRequestBuilder"
></cometchat-groups>
</div>
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
To handle events supported by Groups you have to add corresponding listeners by using CometChatGroupEvents
The Groups
component does not produce any events directly.
Customization
To fit your app's design requirements, you can customize the appearance of the Groups component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. Groups Style
You can set the GroupsStyle
to the Groups Component to customize the styling.
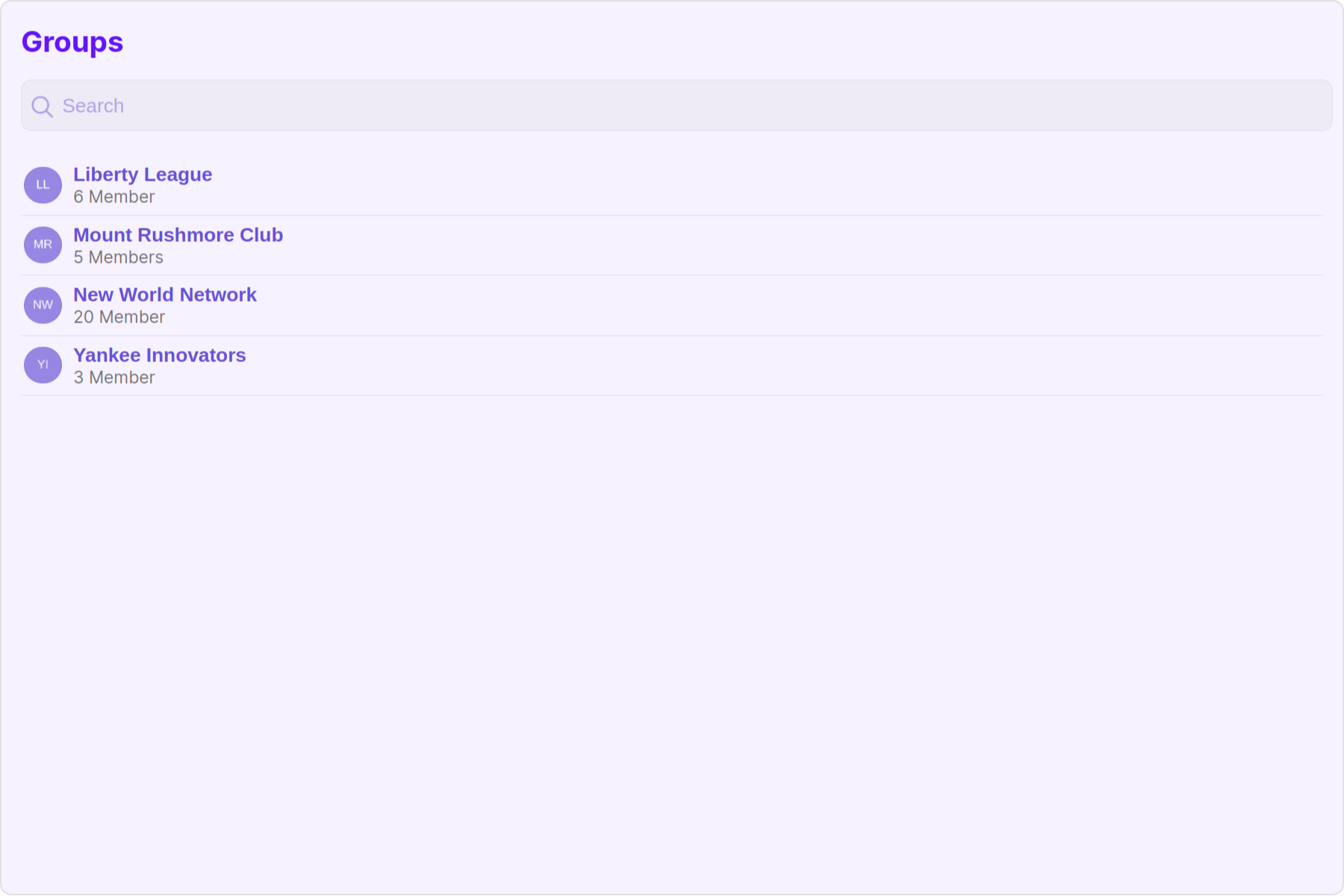
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { GroupsStyle } from '@cometchat/uikit-shared';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
groupsStyle = new GroupsStyle({
background: "#f7f2ff",
titleTextColor: "#6414fa",
searchTextColor: "#940be3",
separatorColor: "#ffffff",
});
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [groupsStyle]="groupsStyle"></cometchat-groups>
</div>
List of properties exposed by GroupsStyle
Property | Description | Code |
---|---|---|
border | Used to set border | border?: string, |
borderRadius | Used to set border radius | borderRadius?: string; |
background | Used to set background colour | background?: string; |
height | Used to set height | height?: string; |
width | Used to set width | width?: string; |
titleTextFont | Used to customise the font of the title in the app bar | titleTextFont?: string; |
titleTextColor | Used to customise the color of the title in the app bar | titleTextColor?: string; |
emptyStateTextFont | Used to set the font style of the response text shown when fetchig the list of groups has returned an empty list | emptyStateTextFont?: string; |
emptyStateTextColor | Used to set the color of the response text shown when fetchig the list of groups has returned an empty list | emptyStateTextColor?: string; |
errorStateTextFont | Used to set the font style of the response text shown in case some error occurs while fetching the list of groups | errorStateTextFont?: string; |
errorStateTextColor | Used to set the font style of the response text shown in case some error occurs while fetching the list of groups | errorStateTextColor?: string; |
loadingIconTint | Used to set the color of the icon shown while the list of groups is being fetched | loadingIconTint?: string; |
separatorColor | Used to set the color of the divider separating the group member items | separatorColor?: string; |
boxShadow | Sets shadow effects around the element | boxShadow?: string; |
privateGroupIconBackground | Sets background color of private group Icon | privateGroupIconBackground?: string; |
passwordGroupIconBackground | Sets background color of protected group Icon | passwordGroupIconBackground?: string; |
searchIconTint | Used to set the color of the search icon in the search box | searchIconTint?: string; |
searchBorder | Used to set the border of the search box | searchBorder?: string; |
searchBorderRadius | Used to set the border radius of the search box | searchBorderRadius?: string; |
searchBackground | Used to set the background color of the search box | searchBackground?: string; |
searchPlaceholderTextFont | Used to set the font of the placeholder text in the search box | searchPlaceholderTextFont?: string; |
searchPlaceholderTextColor | Used to set the color of the placeholder text in the search box | searchPlaceholderTextColor?: string; |
searchTextFont | Used to set the font of the text in the search box | searchTextFont?: string; |
searchTextColor | Used to set the color of the text in the search box | searchTextColor?: string; |
subTitleTextFont | Used to customise the font of the subtitle text. | subTitleTextFont?: string; |
subTitleTextColor | Used to customise the color of the subtitle text. | subTitleTextColor?: string; |
2. Avatar Style
To apply customized styles to the Avatar
component in the Groups Component, you can use the following code snippet. For further insights on Avatar
Styles refer
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, AvatarStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
avatarStyle = new AvatarStyle({
backgroundColor: "#cdc2ff",
border: "2px solid #6745ff",
borderRadius: "10px",
outerViewBorderColor: "#ca45ff",
outerViewBorderRadius: "5px",
nameTextColor: "#4554ff"
});
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [avatarStyle]="avatarStyle"></cometchat-groups>
</div>
3. StatusIndicator Style
To apply customized styles to the Status Indicator component in the Groups Component, You can use the following code snippet. For further insights on Status Indicator Styles refer
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
statusIndicatorStyle: any = ({
height: '20px',
width: '20px',
backgroundColor: 'red'
});
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups
[statusIndicatorStyle]="statusIndicatorStyle"
></cometchat-groups>
</div>
4. ListItem Style
To apply customized styles to the List Item
component in the Groups
Component, you can use the following code snippet. For further insights on List Item
Styles refer
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, ListItemStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
listItemStyle: ListItemStyle = new ListItemStyle({
background: "transparent",
padding: "5px",
border: "1px solid #e9b8f5",
titleColor: "#8830f2",
borderRadius: "20px",
width: "100% !important"
});
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [listItemStyle]="listItemStyle"></cometchat-groups>
</div>
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { TitleAlignment } from '@cometchat/uikit-resources';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
titleAlignment = TitleAlignment.center;
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups
[title]="'Your Custom Title'"
[titleAlignment]="titleAlignment"
[hideSearch]="true"
></cometchat-groups>
</div>
Default:
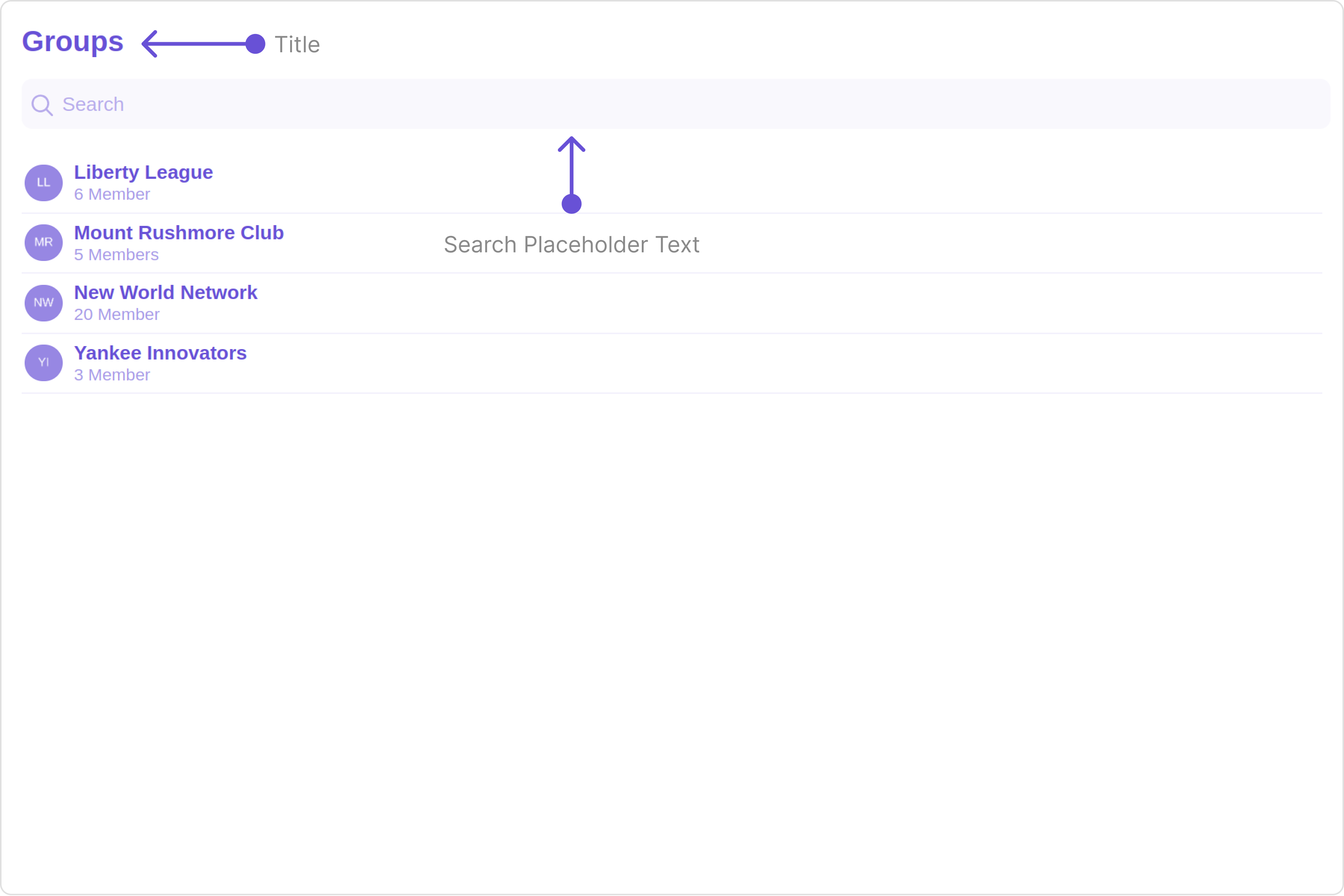
Custom:
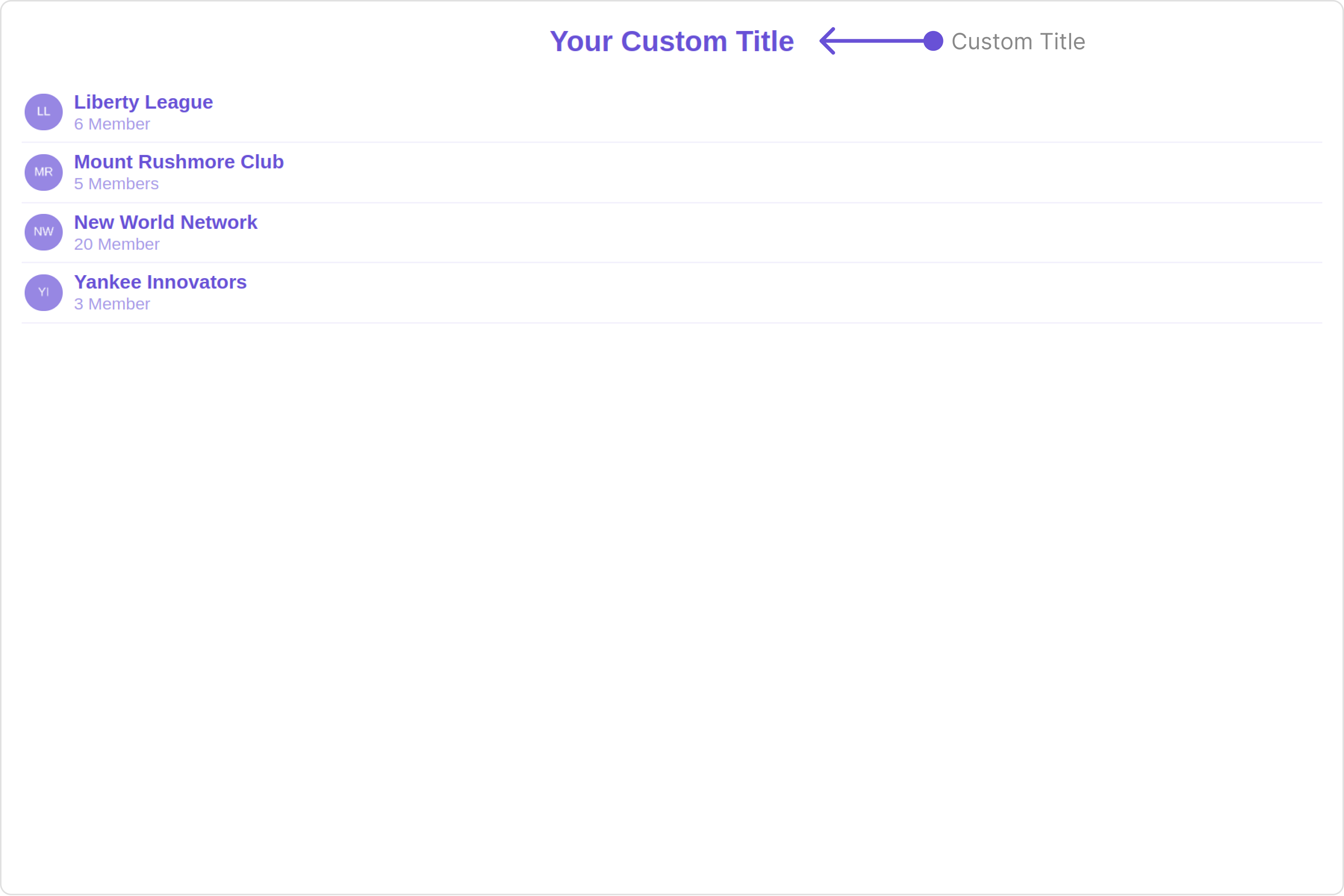
Below is a list of customizations along with corresponding code snippets:
Advance
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
ListItemView
With this property, you can assign a custom ListItem to the Groups Component.
Example
Default:
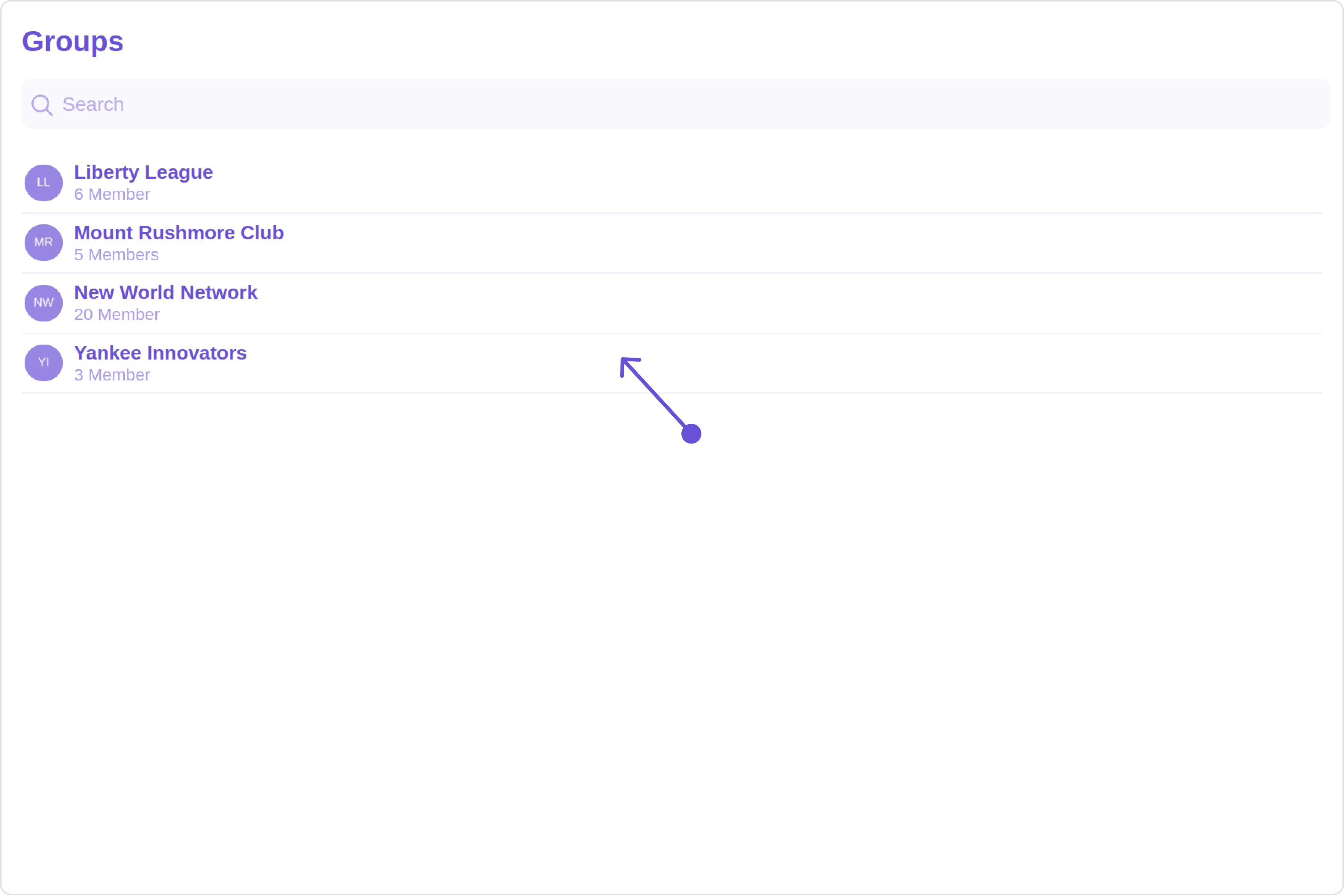
Custom:
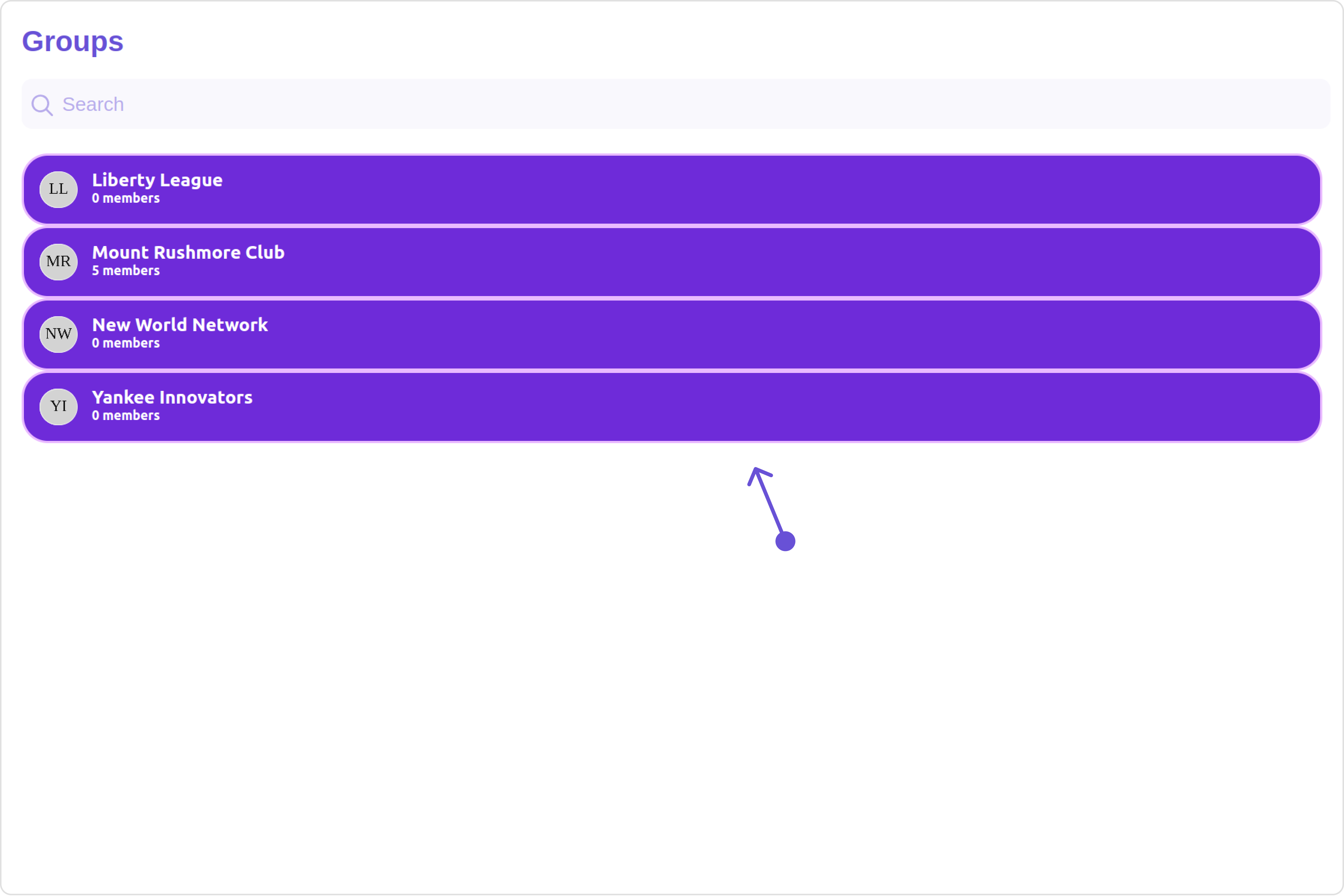
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups
[listItemView]="listItemViewTemplateGroup"
></cometchat-groups>
</div>
<ng-template #listItemViewTemplateGroup let-group>
<div
[ngStyle]="{
display: 'flex',
alignItems: 'left',
padding: '10px',
border: '2px solid #e9baff',
borderRadius: '20px',
background: '#ffffff'
}"
>
<cometchat-avatar
[image]="group.getIcon()"
[name]="group.getName()"
></cometchat-avatar>
<div [ngStyle]="{ display: 'flex', paddingLeft: '10px' }">
<div
[ngStyle]="{
fontWeight: 'bold',
color: '#937aff',
fontSize: '14px',
marginTop: '5px'
}"
>
{{ group.getName() }}
<div
[ngStyle]="{
color: '#cfc4ff',
fontSize: '10px',
textAlign: 'left'
}"
>
{{ group.getMembersCount() }} members
</div>
</div>
</div>
</div>
</ng-template>
SubtitleView
You can customize the subtitle view for each group item to meet your requirements
Default:
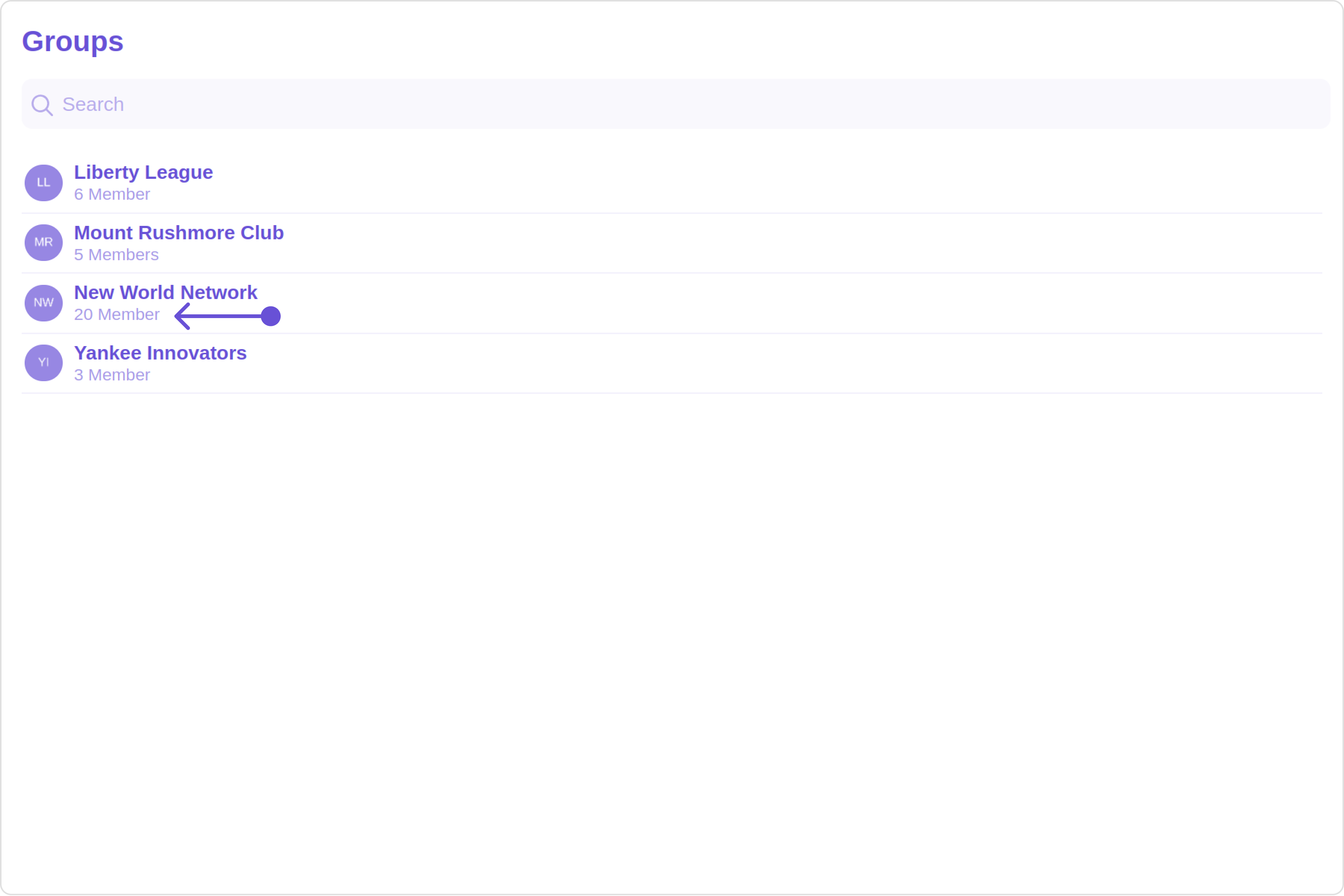
Custom:
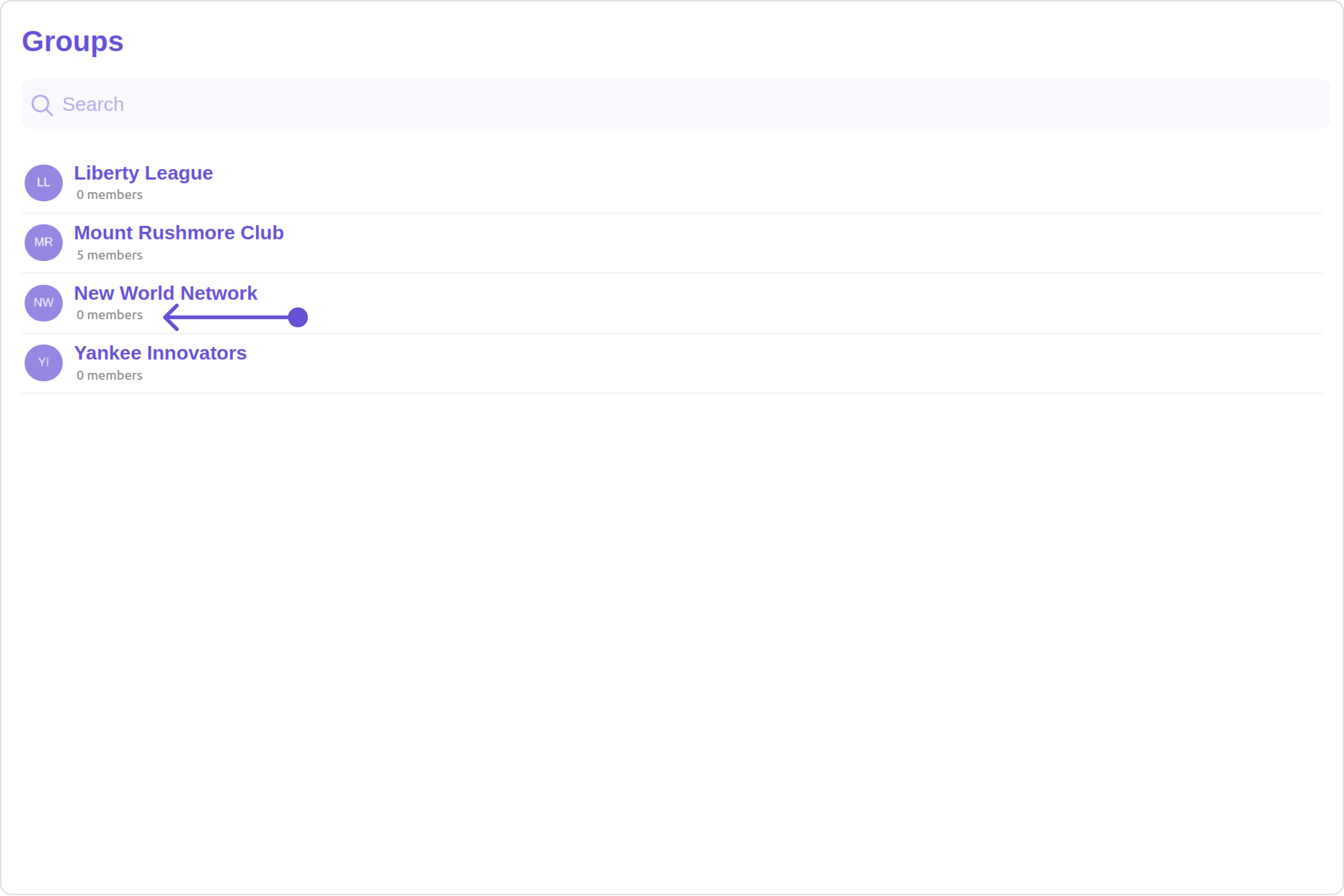
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [subtitleView]="subtitleTemplate"></cometchat-groups>
</div>
<ng-template #subtitleTemplateUser let-group>
<div
[ngStyle]="{
display: 'flex',
alignItems: 'left',
padding: '10px',
fontSize: '10px'
}"
>
<div [ngStyle]="{ color: 'gray' }">Your Custom Subtitle View</div>
</div>
</ng-template>
LoadingStateView
You can set a custom loader view using loadingStateView
to match the loading view of your app.
Default:
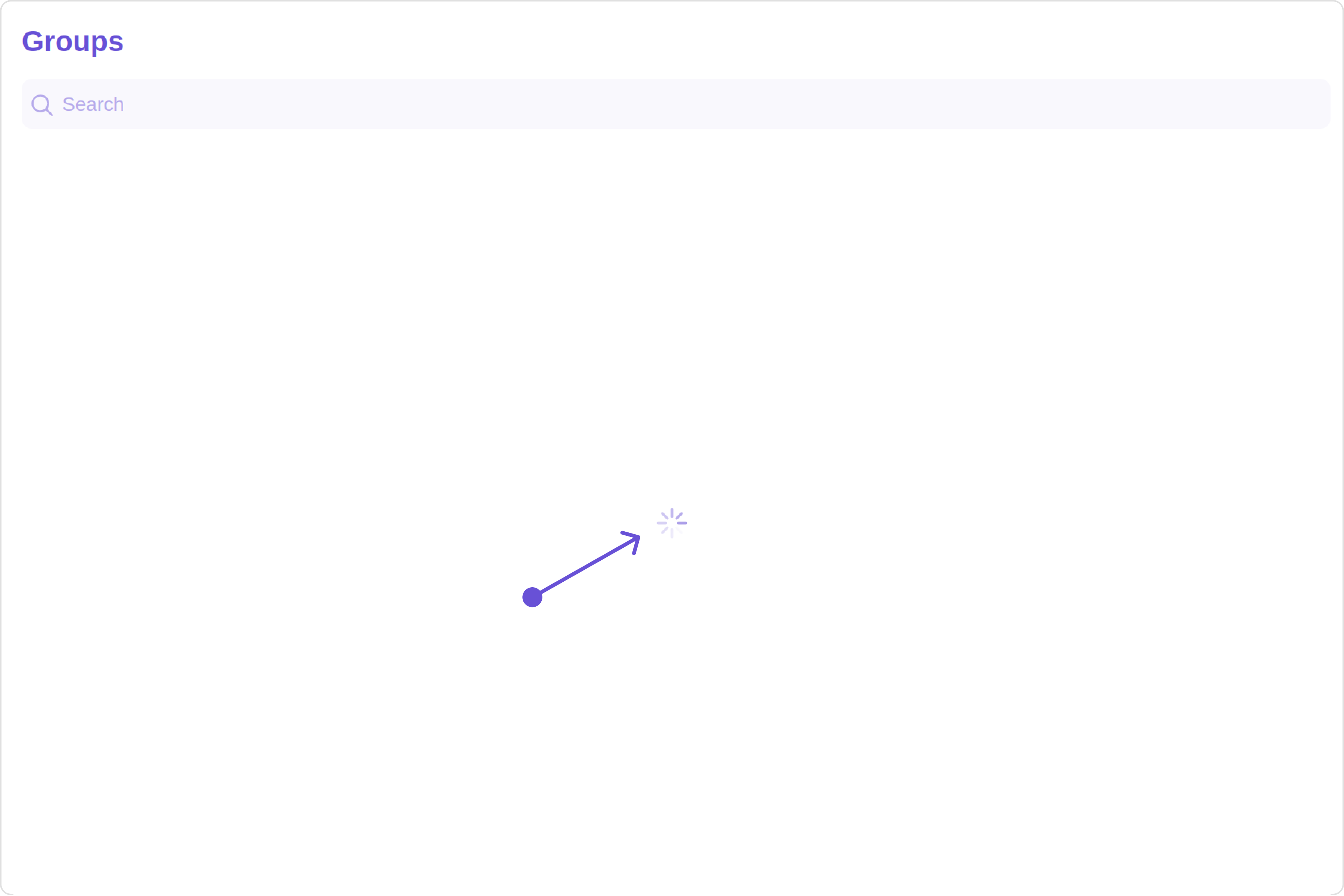
Custom:
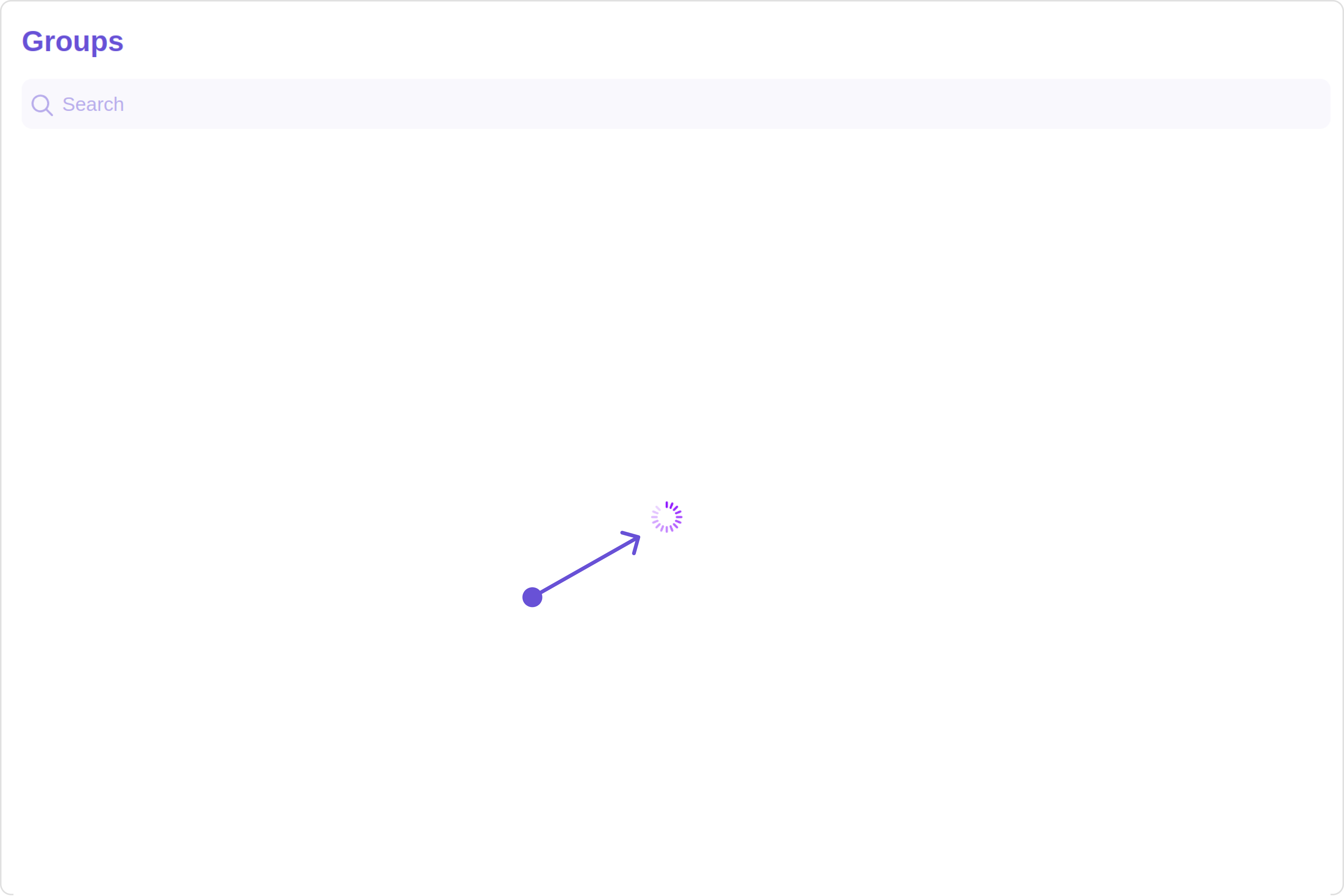
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, LoaderStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
getLoaderStyle: LoaderStyle = new LoaderStyle({
iconTint: "red",
background: "transparent",
height: "20px",
width: "20px",
border: "none",
borderRadius: "0",
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [loadingStateView]="loadingStateView"></cometchat-groups>
</div>
<ng-template #loadingStateView>
<cometchat-loader
iconURL="icon"
[loaderStyle]="getLoaderStyle"
></cometchat-loader>
</ng-template>
EmptyStateView
You can set a custom EmptyStateView
using emptyStateView
to match the empty view of your app.
Default:
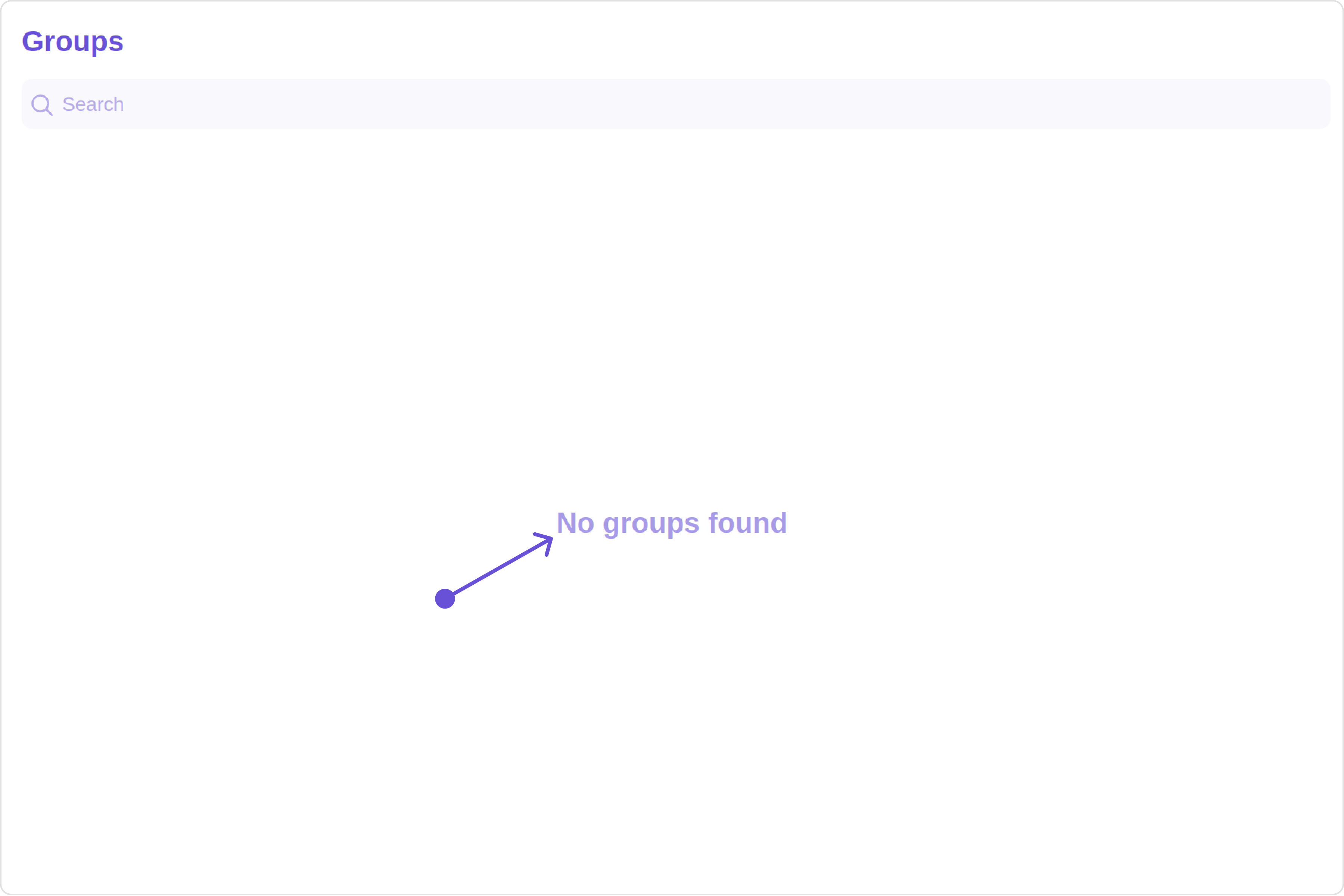
Custom:
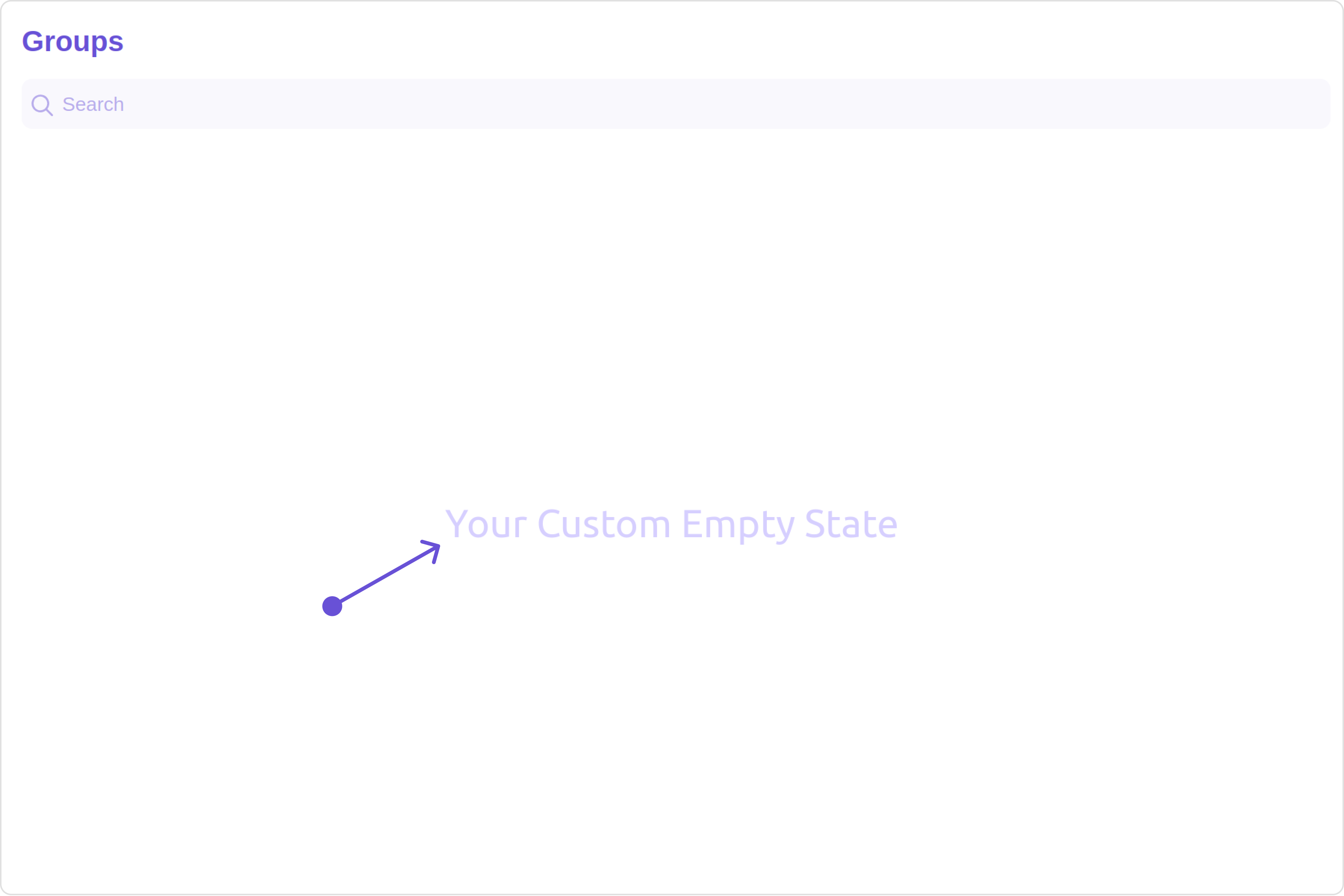
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [emptyStateView]="emptyStateView"></cometchat-groups>
</div>
<ng-template #emptyStateView>
<div>Your Custom Empty State</div>
</ng-template>
ErrorStateView
You can set a custom ErrorStateView
using errorStateView
to match the error view of your app.
Default:
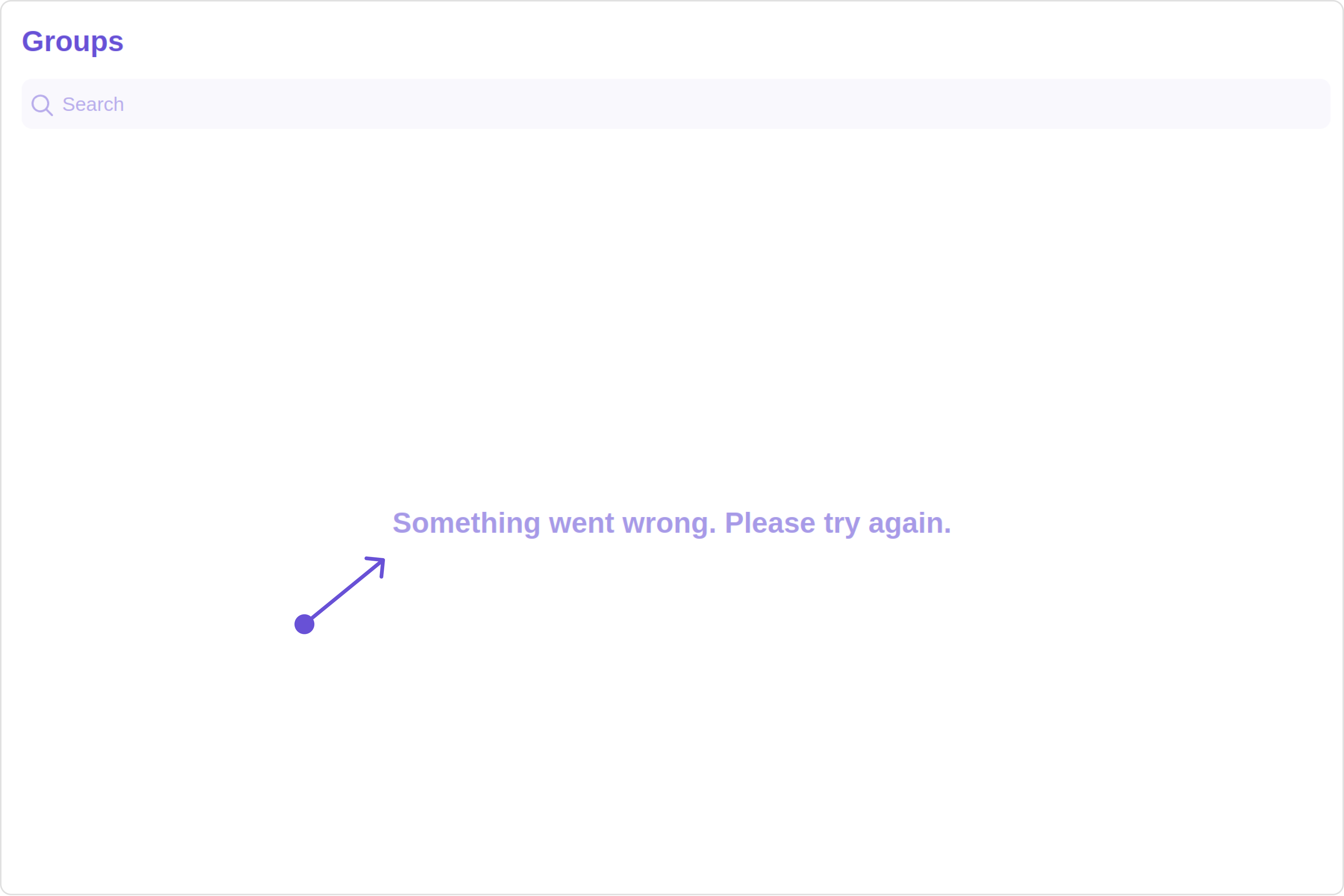
Custom:
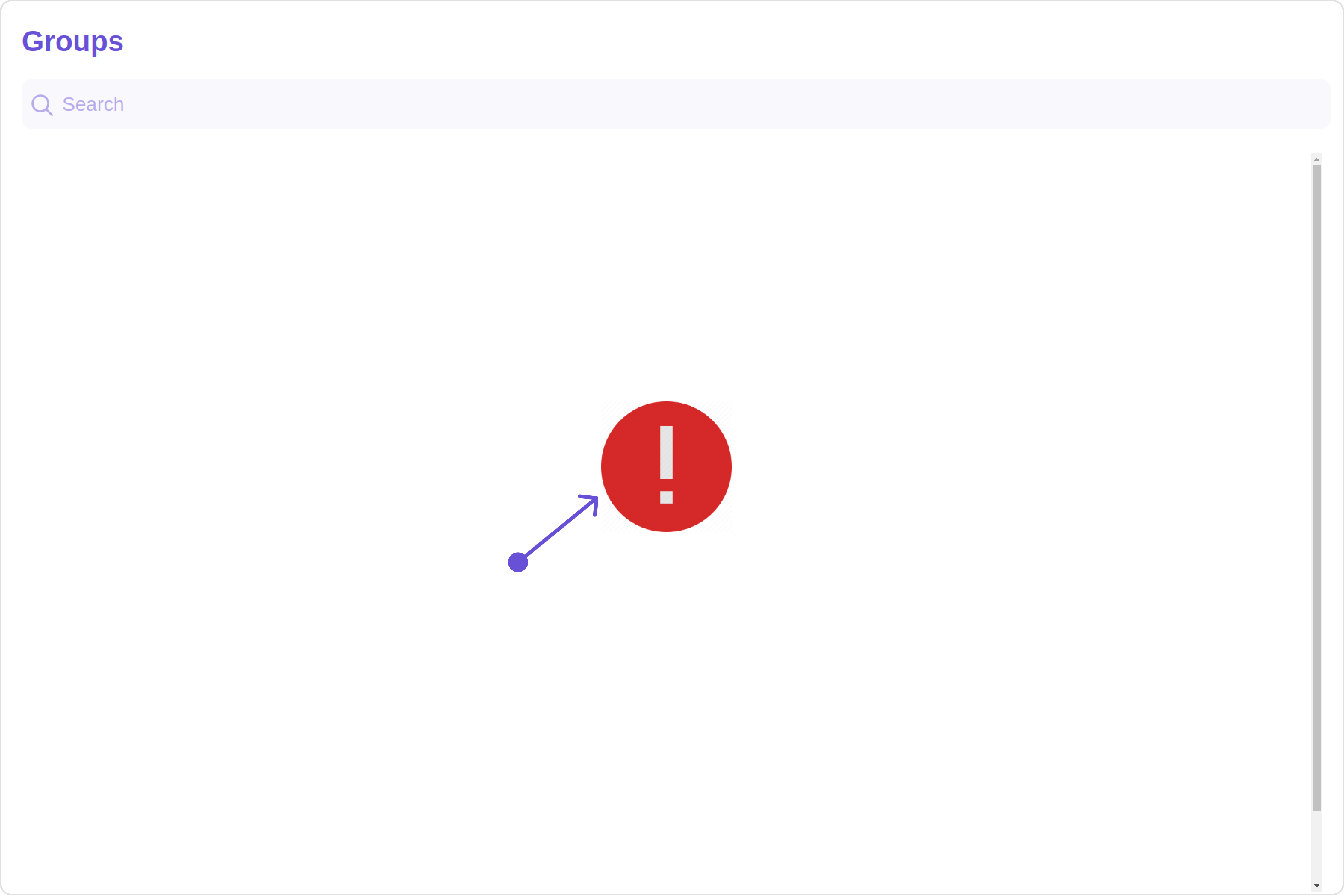
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [errorStateView]="errorStateView"></cometchat-groups>
</div>
<ng-template #errorStateView>
<div style="height: 100vh; width: 100vw">
<img
src="icon"
alt="error icon"
style="height:100px; width: 100px; justify-content: center; margin-top: 250px; margin-right: 700px;"
/>
</div>
</ng-template>
Menus
You can set the Custom Menu view to add more options to the Groups component.
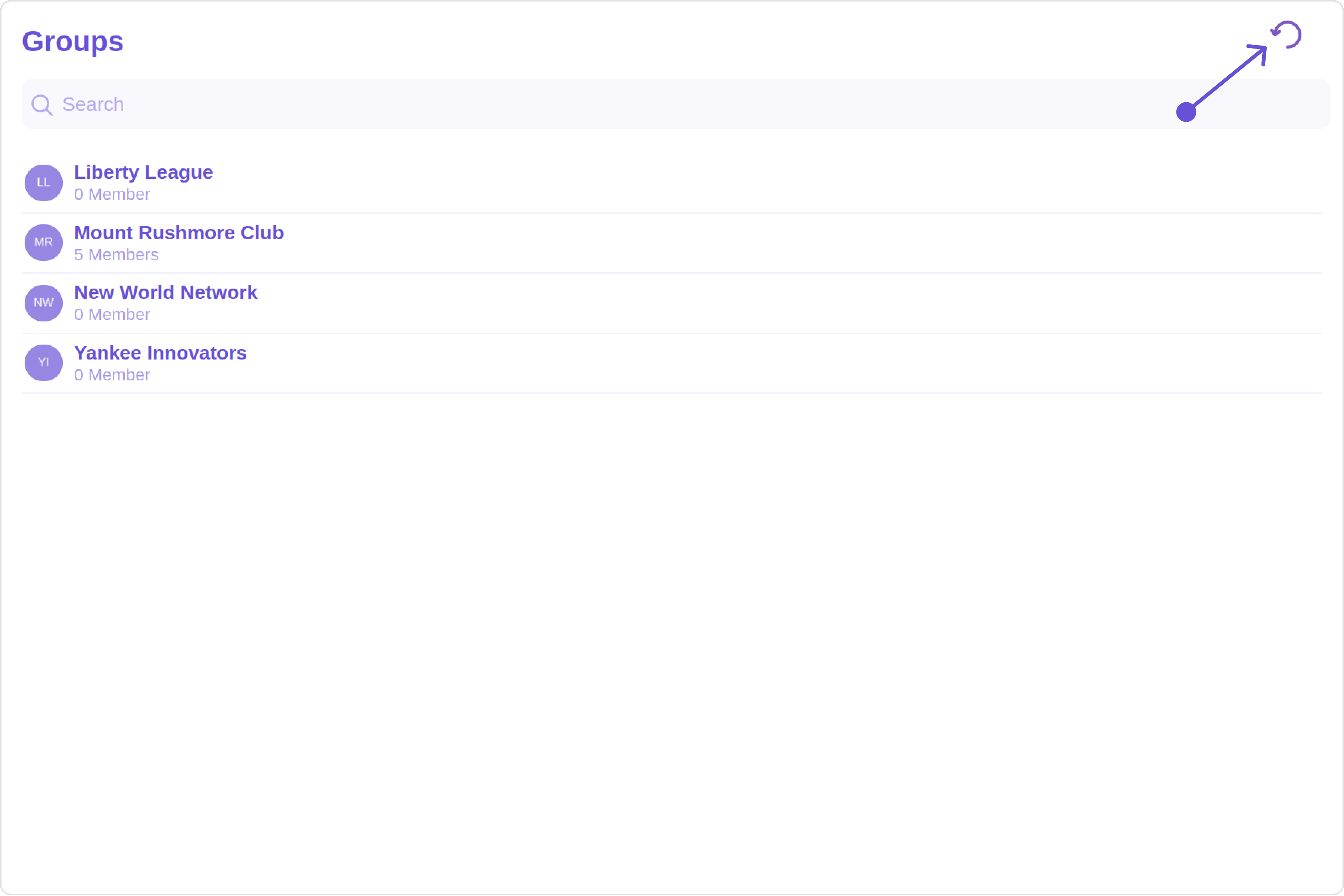
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
handleReload(): void {
window.location.reload();
}
getButtonStyle() {
return {
height: '20px',
width: '20px',
border: 'none',
borderRadius: '0',
background: 'transparent'
};
}
getButtonIconStyle() {
return {
color: '#7E57C2'
};
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [menu]="menuTemplate"></cometchat-groups>
</div>
<ng-template #menuTemplate>
<div style="margin-right: 20px;">
<button [ngStyle]="getButtonStyle()" (click)="handleReload()">
<img src="img" [ngStyle]="getButtonIconStyle()" alt="Reload Icon" />
</button>
</div>
</ng-template>
Options
You can set the Custom options to the Groups component.
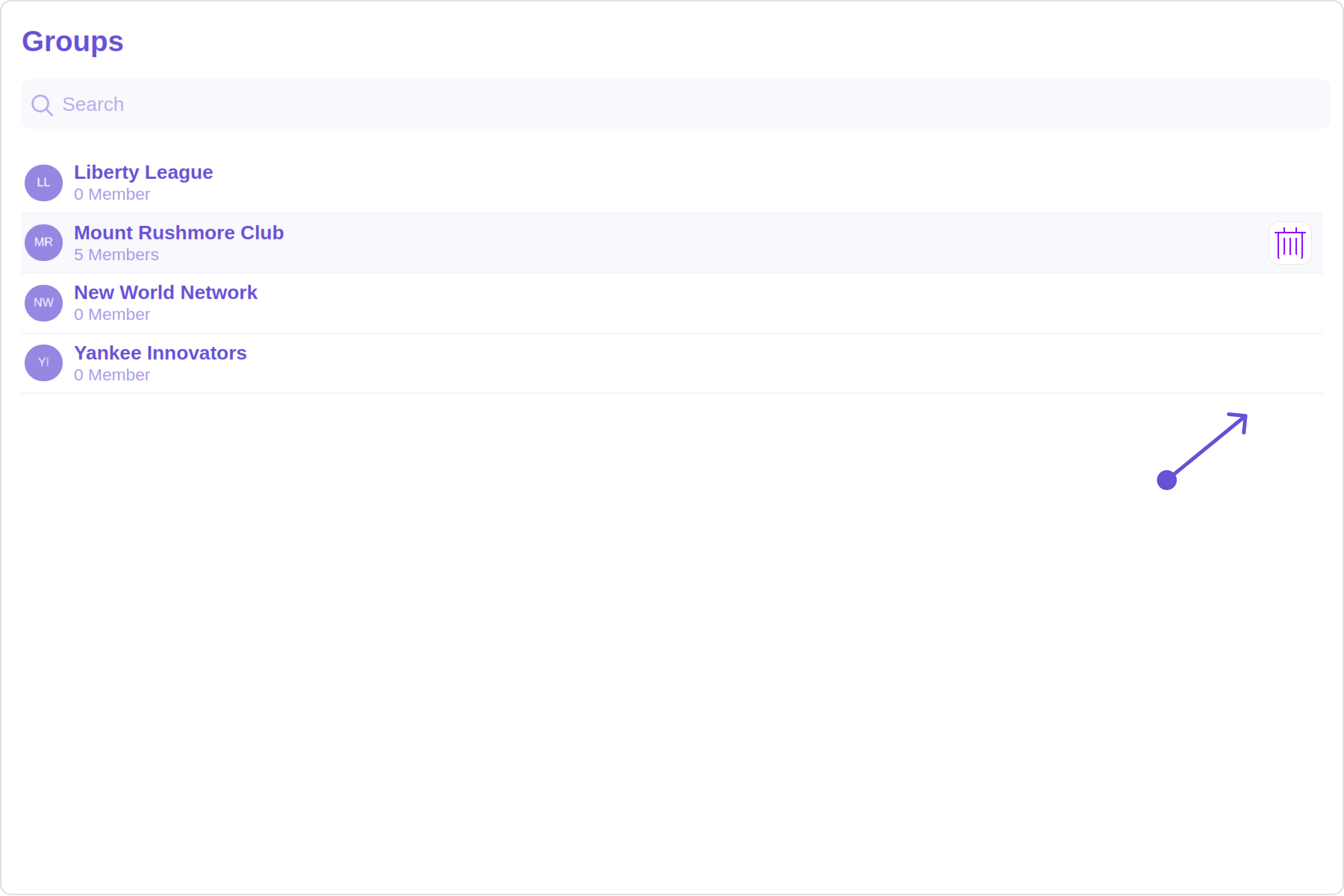
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { CometChatOption } from '@cometchat/uikit-resources';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
getOptions = (user: any) => {
const customOptions = [
new CometChatOption({
id: "1",
title: "Title",
iconURL: "icon",
backgroundColor: "red",
onClick: () => {
console.log("Custom option clicked for user:", user);
},
}),
];
return customOptions;
};
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-groups [options]="getOptions"></cometchat-groups>
</div>