This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
We use a lot of social and chat applications every day, including Facebook, Instagram, Twitter, and WhatsApp. One of the most widely used features is live chat. Using the CometChat React UI Kit, React, and Node.js, you will learn how to build an Instagram clone with minimal effort.
What You Will Build
This tutorial will help you to create the Instagram clone in React.js and Node.js and will cover the following topics:
1. Authentication
A way for users to log in and register
Login the logged-in user to CometChat
Add API call when a user registers so that the user is created in CometChat
User superhero dummy users for this tutorial
2. User Profiles
A tab to display user details.
Display all posts made by the user.
3. Follow Requests
Users can follow other users by simply clicking a ‘Follow’ button on any users profile
4. Create New Post
Functionality to upload new image/video as reel, story, or post
5. Feed
A tab where users can see content uploaded by other users
Functionality to Like posts
Ability to share posts in chat
6. Reels
Ability for users to upload videos
Display these reels in the Feeds tab
7. Notifications
Notifications for new messages, likes and follow requests
A tab where users can see all the latest notifications.
8. Chat
Ability to text all other users
Voice & Video calling
Reactions, Media sharing, and read receipts
What You Will Need
In order to follow this tutorial, you need to have a basic understanding of React.js, Node.js and MySQL database. You will also need:
Node.js installed on your machine
CometChat Pro account
MySQL installed on your machine
Installing the App Dependencies
Create a new project named i_nstagram-clone_ by running the following statement:
Copy the dependencies from here, and then run the below statement:
npm i
Create a CometChat App
You will need to create a new App:
Add real-time chat with minimal effort using CometChat
Login to your CometChat dashboard
Click on ‘Add New App’
Provide a name for your app and select the region you want to use.

Remove Existing Users and Groups
CometChat will automatically create some Users and Groups to use with your application. You can safely remove these as you will be creating new users that will be shared between your chat application and CometChat.
Configure the CometChat SDK
Once your App has been created you can find your APP ID and Auth Key on the ‘Quick Start’ page. These will be used by the CometChat SDK to access your application on the CometChat platform

Create a file called .env in the root folder of your project and add you APP ID, Auth Key and Region like in the example below:
Project Structure - Client Side
The image below reveals the project structure. Make sure you see the folder arrangement before proceeding.

Project Structure - Client Side
Now, let's make the rest of the project components as seen in the image above.
Project Structure - Server Side
To create a new project, you just need to create a folder which is called “instagram-clone” and then run “npm init”. You can choose any name for your project. After following the instruction, you will a brand new Node.js project. The image below reveals the project structure of our Instagram clone application. Make sure you see the folder arrangement before proceeding.

Project Structure - Server Side
Each subfolder and file will be explained in detail in the following section:
public: contains all html, css, javascript files for the UI.
routes: contains all files that will be used to handle the API requests.
screenshots: this folder contains images that are used for README.md file.
.env: contains environment variables that will be used in the application.
.gitignore: this file contains files that will be ignored when committing the code. In this case, we do not want to commit the “config.js” file because it contains the secret keys of the Javascript chat application.
package.json: contains all dependencies of the application.
README.md: describes the application and provides steps by steps to run the application.
instagram.sql: contains scripts that you need to use to create the database and its tables.
Installing the Instagram Clone Dependencies
Step 1: You need to have Node.js installed on your machine
Step 2: Copy dependencies and devDependencies from the package.json file.
Step 3: Run “npm i” to install the dependencies for the application.
Setting Up Database
As mentioned above, we will use the MySQL database. For this reason, this section describes how to create the database and its table. Before proceeding, you need to make sure that you have installed the MySQL database on your computer already. To create the database and its table, you need to get the SQL here.
The above sql specifies that we need to create the below tables:
1. user_account: stores the user’s information.
2. post: stores the post’s information.
3. post_reaction: stores the information about the users who like the individual post.
4. user_notification: stores the notification for the individual user.
5. user_follower: stores the followers for each user.
Each table will be explained in the follow part:
1. user_account:
id - id of the user.
user_email - email of the user.
user_password - password of the user.
user_full_name - full name of the user.
user_avatar - avatar of the user.
user_number_of_posts - the number of posts for the user.
user_number_of_followers - the number of followers for the user.
user_number_of_following - the number of followers that the user is following.
According to the requirements, the user can upload their posts, the post could be an image or a video. The post table will be used to store that information.
2. post:
id - the post’s id. it will be auto-increment if a new post is inserted to the database.
post_content - the post’s content, it could be the image link or the video link because the end-users can upload the image and video.
post_category - it is an integer value, it determines the post is an image or a video. If the value is 1, it means that the post is an image, and if the value is 2, the post is a video.
post_created_date - the post’s created date.
post_created_by - contains the id of the user who created the post.
The end-users can like the posts. To achieve that, we need to create the post_reaction table to store the post’s reactions.
3. post_reaction:
id - the id of the post’s reaction. It will be auto-increment.
post_id - the post’s id.
user_id - the id of the user who likes the post.
The end-users can view the list of notifications. For example, if someone likes their posts or follows their profiles. The notifications list will be updated, the user_notification table is used to store that information.
4. user_notification:
id - the id of the notification. it will be auto-increment.
notification_image - the notification’s image.
notification_message - the notifications’ content.
user_id - the id of the user that the notifications belong to.
In the Instagram clone, we also implement a way that allow the end-users to follow each other. The user_follow table is used to store the follower of an individual user.
5. user_follower
id - the primary key of the table. it will be auto-increment.
follower_id - the id of the follower.
user_id - the id of the user.
Aside from that, we are using Node.js with MySQL database. Hence, we need to connect to the database in our Node.js application, The best practice is to create a .env file and store environment variables. To create the .env file, please follow the below steps:
Create a file which is call “.env” inside the root folder of your project.
Replace the below information with your database connection information.
Create a Node.js Server
Inside the root folder of your project, you need to create a file which is called “index.js”. The full source code of the index.js file can be found here. It is the main file that will be ran when running the following statements
*Note: In this project, we will use the “nodemon” library because the application will be reloaded automatically whenever there is any change in your code.
The above code snippet specifies that we are including some libraries to help them create a server. In this case, we need to use the express framework and the multer library for uploading files because we are building a dating site in Node.js. Therefore, the application should allow the user to upload their avatar. Aside from that, the mysql library for connecting with the MySQL database and so on. The database connection information that will be read from the .env file and this file should be included in the .gitignore file.
On the other hand, we are requiring all API routes of the application at line 45. However, we have not define it, yet. Please do not worry about it, we will discuss about it in the following section.
Creating the Routes Folder
The routes folder will contain API routes in the application. Inside this folder, we need to create another “index.js” file. We will export a function that will take responsibility for combining all API routes, that function accepts an object as a parameter. The object will contain the express app object, the database connector object, the upload object - created by the “multer” library. Those objects will be used in different API routes as you can see in the below code snippet. The full source code of the “index.js” file can be found here.
Note: We will define six API routes in the application. the “authRoutes” is used for authenticated purpose, the “userRoutes” is used for user management. The “followerRoutes” is used to manage the followers, the “notificationRoutes” is used to manage the notifications, the “postRoutes” is used to manage the posts. The last but not least, the “reactionRoutes” is used to manage the reactions in the application. All of them will be discussed in the following section.
Creating APIs with Node.js
In this section, we will develop the APIs that will be needed to build our Instagram clone. The below endpoints describes the information about the APIs for the application.
/login: check user’s credentials and ensure that the user can login to the application, or not.
/users/create: create a new user in the application.
/users/:id: get the user’s information by id. It means that if we would like to load the user’s information, we just need to call this API and attach the id of the selected user to the API endpoint.
/users/followers: after an user follows or unfollows someone, we need to have an API to update the number of followers of the selected user.
/users/posts: the end-users can create post in the Instagram clone. For this reason, we need to update the number of posts after the end-users has created a post.
/posts: as mentioned above, the end-users can create post. Therefore, the application needs to have an API to insert a new post to the database.
/posts: on the home page, the end-users can see the posts of each other. Hence, we create an API to get all posts from the application and order them by their created date. It means that the newest post will be on the top.
/posts/:id: after the user has selected a post, the application displays the detail information of the selected post so we create an API to retrieve the post’s information by its id.
/posts/reactions: the users can like/unlike any post. Therefore, we have an API to update the number of reactions of the selected post.
/posts/categories: We can view the user’s profile and on that page, we can click on the toggle button to view the list of images or view the list of videos. In this tutorial, we can them as post’s category. Therefore, we need to have an API to load the list of posts by its category.
/followers/get: If the current authenticated user views the profile of someone, we want to check wether the authenticated user has followed the selected profile, or not. To do that, the applications needs to have an API that accept the follower’s id, the user’s id, and then find the record in the database. If the data existed in the database, it means that the authenticated user has followed the selected profile.
/followers/create: if the authenticated user has clicked on the “Follow” button, we should add a new record in the user_follower table because the authenticated user would like to follow the selected profile.
/followers/delete: after the authenticated user has followed someone, the user can click on the “Follow” button again to reverse the action - unfollow the selected profile. Therefore, we create an API to delete the inserted record in the user_follower table.
/reactions/get: If the current authenticated user views a post, we want to check wether the authenticated user has liked the post, or not. To do that, the applications needs to have an API that accept the post’s id, the user’s id, and then find the record in the database. If the data existed in the database, it means that the authenticated user has liked the selected post
/reactions/create: if the authenticated user has liked a post, we should add a new record in the post_reaction table.
/reactions/delete: after the authenticated user has liked a post, the user can click on the “heart” icon again to reverse the action - remove the like. Therefore, we create an API to delete the inserted record in the post_reaction table.
/notifications/create: after the users have liked any post or followed any profile. The notifications will be created, and sent to the owner of the selected posts/profiles.
/notifications/:id: the authenticated user can view the list of notifications by click on the “heart” icon on the header as you can see in the demo video. Hence, the application needs to have an API to load the notification by the user’s id.
The Login API
The login API will receive the user’s credentials and validate that information. If the user’s information is valid, the user can log in to the application and vice versa. To create the login API, you need to create the “auth.js” file inside the “routes” folder. The full source code of the login API can be found here.
its method is POST and the request payload should contain the user’s email and user’s password. If the user’s information is valid, the authenticated information will be returned as the response. Following that, a warning message will be returned if the user’s credentials are not valid. Your can refer to the code snippet below for more information.
The User APIs
This section will describe the APIs for user management. Firstly, we need to create the “users.js” file inside the “routes” folder. It will contains some API endpoints such as creating a new user account, updating number of followers/posts, retrieving the user’s information by id. The full fouce code can be found here.
The Create User API
In this part, we will develop an API to create a new account in the application. The API will have the below information. Its method is POST and we will send the form data format from the client side and the request should contain the user’s id, user’s email, user’s password, user’s avatar, user’s full name.
The above information is required. If you miss something in the request payload, a warning message will be returned back from the response. Moreover, the API will check the user’s email has been used in the application, or not. If it existed in the system, a warning message will be returned to inform the user about that. You can refer to the below code snippet for more information.
The Update Number of Followers API
According to the requirement, the end-users can follow each other by clicking on the “Follow” button. Therefore, the application needs to have an API to update the number of followers.
The API requires an JSON object with two properties - the user’s id and the number of followers that will be used to update the selected record in the database. You can refer to the below code snippet for more information.
The Update Number of Posts API
The end-user create a post as an image or a video in the Instagram clone. After a post has been created, we need to update the number of posts for that user.
The API accepts an JSON object with two properties - the id of the user and the number of posts that will be used to update the record in the database. You can refer to the below code snippet.
The Get User By Id API
We can view the profile of any user. Hence, the client side will can the get user by id API to load the user’s information. its method is GET, and we need to attach the user’s id to the API endpoint so the back-end will know which user should be loaded from the database.
The Post APIs
This section will describe the APIs for post management. Firstly, we need to create the “posts.js” file inside the “routes” folder. It will contains some API endpoints such as creating a post, get all posts, updating the number of reactions, getting a post by its id, retrieving the list of posts by the selected category. The full fouce code can be found here.
The Create Post API
As mentioned above, the end-users can create post. Therefore, the application needs to have an API to insert a new post to the database. In this case, we use the multer library to upload the file, and the file could be an image or a video.
The Get Posts API
On the home page, the end-users can see the posts of each other. Hence, we create an API to get all posts from the application and order them by their created date. It means that the newest post will be on the top.
The Get Post By Id API
After the user has selected a post, the application displays the detail information of the selected post so we create an API to retrieve the post’s information by its id.
The Update Number of Reactions API
The users can like/unlike any post. Therefore, we have an API to update the number of reactions of the selected post.
The Get Posts By Category API
We can view the user’s profile and on that page, we can click on the toggle button to view the list of images or view the list of videos. In this tutorial, we can them as post’s category. Therefore, we need to have an API to load the list of posts by its category.
The Follower APIs
This section will describe the APIs for follower management. Firstly, we need to create the “followers.js” file inside the “routes” folder. It will contains some API endpoints such as checking existing follower relationship, create a new follower, delete a follower. The full fouce code can be found here.
The Check Existing Following API
If the current authenticated user views the profile of someone, we want to check wether the authenticated user has followed the selected profile, or not. To do that, the applications needs to have an API that accept the follower’s id, the user’s id, and then find the record in the database. If the data existed in the database, it means that the authenticated user has followed the selected profile.
The Create Follower API
If the authenticated user has clicked on the “Follow” button, we should add a new record in the user_follower table because the authenticated user would like to follow the selected profile.
The Delete Follower API
After the authenticated user has followed someone, the user can click on the “Follow” button again to reverse the action - unfollow the selected profile. Therefore, we create an API to delete the inserted record in the user_follower table.
The Reaction APIs
This section will describe the APIs for reaction management. Firstly, we need to create the “reactions.js” file inside the “routes” folder. It will contains some API endpoints such as checking existing reaction, create a new reaction, delete a reaction. The full fouce code can be found here.
The Check Existing Reaction API
If the current authenticated user views a post, we want to check wether the authenticated user has liked the post, or not. To do that, the applications needs to have an API that accept the post’s id, the user’s id, and then find the record in the database. If the data existed in the database, it means that the authenticated user has liked the selected post.
The Create Reaction API
If the authenticated user has liked a post, we should add a new record in the post_reaction table.
The Delete Reaction API
After the authenticated user has liked a post, the user can click on the “heart” icon again to reverse the action - remove the like. Therefore, we create an API to delete the inserted record in the post_reaction table.
The Notification APIs
This section will describe the APIs for notification management. Firstly, we need to create the “notifications.js” file inside the “routes” folder. It will contains some API endpoints such as create a notification, and get the list of notification by the user’s id. The full fouce code can be found here.
The Create Notification API
After the users have liked any post or followed any profile. The notifications will be created, and sent to the owner of the selected posts/profiles.
The Get Notification By User Id API
The authenticated user can view the list of notifications by click on the “heart” icon on the header as you can see in the demo video. Hence, the application needs to have an API to load the notification by the user’s id.
Configuring Styling for the Application
We have created the APIs that will be needed for the Instagram clone. It is time to write code for the client side and interact with the back-end services. Inside your project structure, open the index.css files and paste the codes here. index.css file will contain all CSS of the application.
Creating React Context
In this application, we need to pass some data between the components. We can save several options to achieve that such as using state management libraries, RxJS, and so on. However, we can get in-built support from React by using the React Context API. the React Context API helps us pass data through the component tree without passing down at every level. Please create the “context.js” file inside the “src” folder. The full source code of the “context.js” file can be found here.
Initializing CometChat for the Application.
The below code initializes CometChat in your app before it spins up. The App.js file uses your CometChat API Credentials. We will get CometChat API Credentials from the .env file. Please do not share your secret keys on GitHub.
Actually, App.js does not contain only the above code. It also contains other business logic of the application.
The App.js File
The App.js file is responsible for rendering different components by the given routes. For example, it will render the login page if the user has not logged in, yet or it renders the home page if the user has signed in to the system. On the other hand, it will be used to initialize CometChat. The full source code of the App.js file can be found here.
The Loading Component

The Loading Component
The loading component will be shown when the system performs some side effects such as interacting with the back-end services or calling CometChat APIs and so on. This component will be used to increase user experience. If we do not have this component, the end-users cannot know when the data is loaded successfully. Please create the “common” folder inside the “src” folder. The “common” folder is used to store the common components that will be used in different places in your application. After that, you need to create the “Loading.js” file inside the “common” folder. The full source code of the loading component can be found here.
The Login Component

The Login Component
This component is responsible for authenticating our users. It accepts the user credentials and either signs him up or in, depending on if he is new to our application. See the code below and observe how our app interacts with the login API and the CometChat SDK. Please create the “login” folder inside the “src” folder, and create the “Login.js” file inside the “login” folder. The full source code can be found here.
We store the authenticated Information to the local storage for further usage such as getting that information in different places in the application, prevent the end-users come back to the login page after signing in to the application.
To login to the CometChat, we call the cometChat.login function, you can refer to the below code snippet for more information.
The above code indicates that we are using withModal as a higher-order component. This higher-order component will be used to reuse the code of showing and hiding the custom modal. In this case, we want to show the sign-up modal to let end-users register new accounts. We will discuss the sign-up component in the following section.
The withModal - Higher-Order Component
As mentioned above, we would like to show the SignUp component as a modal. Actually, we have multiple modals in the application, and we should avoid duplicating common functionalities such as showing/hiding the modals, and so on. To reuse the common logic, we can have several options. In this project, we will create a higher-order component called “withModal”. That higher-oder component helps us to avoid duplicating code and we can customize the UI for the modals. Please follow the below code snippet for more information. Please create the “Modal.js” file inside the common folder. The full source code can be found here.
The Sign Up Component

The Sign Up Component
The sign-up component will help end-users to register new accounts. This component will do two things. The first thing is to register new accounts by calling the create user API. Aside from that, it also registers new accounts on CometChat by using the CometChat SDK. Please create the “register” folder inside the “src” folder, and create the “SignUp.js” file inside it. The full source code can be found here.
To create a new CometChat account, we call cometChat.createUser function. You can refer to the below code snippet below for more information.
Add The CometChat UI to Our Application
Before we can use the CometChat Pro React UI kit, we need to add it in our project so that we can reference it. In this case, we are using React UI Kit v3.0. To do that, follow the next steps:
Step 1: Clone the CometChat React UI Kit Repository like so:
Step 2: Copy the folder of the CometChat Pro React UI Kit you just cloned into the "src" folder of your project:

Copy the cloned folder in the src folder

React UI Kit Dependencies
Step 3: Copy all the dependencies from the package.json file of the CometChat Pro React UI Kit folder and paste them in the dependencies section of the package.json file of your project.
Step 4: Save the file and install the dependencies like so: npm install
As soon as the installation is completed, you now have access to all the React UI Components. The React UI kit contains different chat UI components for different purposes as you can see in the documentation here. It includes:
1. CometChatUI
2. CometChatUserListWithMessages
3. CometChatGroupListWithMessages
4. CometChatConversationListWithMessages
5. CometChatMessages
6. CometChatUserList
7. CometChatGroupList
8. CometChatConversationList

CometChatMessages Component

CometChatMessages Component - Voice and Video Calling
The Header Component
The Header component is used to render the Header of the Instagram clone. Following that, it contains some menu items. The end-users can select those items and the application will perform the corresponding actions. Please create the “Header.js” file inside the “common” component because the Header component will be reused in different places in the application. The full source code of the Header component can be found here.
The Create Post Component

The Create Post Component
After the end-user has selected the plus icon on the header. The Create component will be displayed as a modal. That’s why we see at the export statement of the Header component, we use withModal higher-order component with the Create component. As you can see in the above image, we can click on the “Upload” button to create a new post. In this case, we can upload an image or a video, the application will interact with the back-end service by calling the create post API. Please create the “create” folder inside the “src” folder, and then create the “Create.js” file inside it. The full source code of the Create component can be found here.
The Home Component
The Home component acts as a container component. It means that it will interact with the back-end service (calling the get posts API), get the response and pass the data to the child components via props. Other component inside it will perform as presentational components - render the data on UI. The Home component will contain 2 components inside it - the Header component that we have mentioned above and the Posts component. the Posts component is used to render the list of posts on the UI, we discuss it in the following section. Please create the “home” folder inside the “src” folder, and create the “Home.js” file inside it. The full source code of the Home component can be found here.
The Posts Component
As mentioned above, the Posts component is used to render the list of posts on the UI. After the end-users has accessed the home page, the Home component will call the get posts API, get the response, and then pass the response the the Posts component via posts prop. The Posts component takes that prop and render the result on the UI as you can see in the above image. Please create the “post” folder inside the “src” folder, and create the “Posts” component inside it. The full source code can be found here.
Each item in the list will be rendered by using the Post component that would be discussed in the following section.
The Post Component
Each item in the list will be rendered by using the Post component that would be discussed. After the Posts component get the list of posts from the Home component, it will use the map function to render the data on the UI, each item from the list will be passed to the Post component. Therefore, the Post component takes the responsibility for rendering a single post. Please create the “Post.js” file inside the “posts” folder. The full source code can be found here.
The Detail Component

The Detail Component
The end-users can select any item on the list of posts, the Detail component will be displayed as a modal, and show the detail information of the selected post including the author’s name, author’s avatar, number of likes, share button, follow button, and post’s content, the uses can like or remove their likes by clicking on the “heart” icon or copy the link of post to share in the chat by clicking on the “share” icon. Aside from that, to follow/unfollow any user, the end-users can click on the “follow” button. To achieve the above features, the Detail component will interact with the load post API, check existing reaction/follower API, create follower/reaction API, and delete follower/reaction API. Please create the “Detail.js” file inside the “post” folder. The full source code can be found here.
The Share Component

The Share Component
According to the requirements, the end-users can share the post in the chat. In this case, after the end-users have clicked on the “share” button on the Detail component, the application will copy the link, and the users can share that link in their chatbox. The Share component gets the selected post id on the URL, and then call the load post by id API to show the data on the UI. Please create the “Share.js” in the “post” folder. The full source code can be found here.
The Profile Component

The Profile Component
After clicking on the author’s name on the Detail component or selecting the user’s avatar on the Header component, we can view the corresponding profile. The Profile component act as the container component, it interacts with the APIs to get the data and pass that data to its children via props. In this case, the application needs to call the load user by id API and the load posts by category API. To render the list of posts on the UI, we reuses the Posts and Post component as mentioned above. Please create the “profile” folder inside the “src” folder and create the “Profile” component inside it. The full source code can be found here.
The User Profile Component

The User Profile Component
The UserProfile component is used to render the user’s information including the user’s avatar, user’s name, number of posts, and number of followers. After the Profile component has called the load user by id API, the response will be passed to the UserProfile component via prop. Please create the “UserProfile.js” file inside the “profile” folder. The full source code can be found here.
The Actions Component

The Actions Component
If we view the profile of someone, we can view their images and their videos. The Actions component is used to render two options for us. If the “posts” option is selected, the list of images of the selected user will be rendered. Following that, if the “videos” option is selected, the list of videos will be shown. Please create the “Actions.js” file inside the “profile” folder. The full source code can be found here.
The Notifications Component

The Notifications Component
To view the list of notifications, the users click on the “heart” icon on the Header component. The Notifications component is used to render the list of notifications. It call the get notifications api by user id. Please create the “notification” folder and create the “Notifications.js” file inside it. The full source code can be found here.
The Notification Component

The Notification Component
Each notification will be rendered by using the Notification component. The Notifications component interact with the API, get the response and render the result on the UI by using the map function. Each item in the list will be passed to the Notification component via prop. Please create the “Notification.js” file inside the “notification” folder. The full source code can be found here.
The Chat Component

The Chat Component
According to the requirements, the end-users can do the following actions:
Ability to text all other users
Voice & Video calling
Reactions, Media sharing, and read receipts
To achieve the above features, we will the CometChat React UI Kit. We have integrated the UI Kit in the above section. Please create the “chat” folder inside the “src” folder, and create the “Chat.js” file inside it. In the Chat component, we use the <CometChatUI /> component to render the chat UI. The <CometChatUI /> component provides the full features that you will need to a professional chat application. The full source code can be found here.
The Logout Feature

The Logout Feature
The end-users can click on the last icon on the Header component, a confirmation dialog will be displayed to make sure that the users would like to log out from the application, if the users click on the “Ok” button, the application will clear the authenticated information from the localStorage, log out the user from the CometChat by calling cometChat.logout() function, reset the corresponding state. You can refer to the below code snippet for more information.
Wrapping Up
In conclusion, we have done an amazing job in developing an Instagram clone by leveraging React.js, Node.js, CometChat SDK, and React UI Kit. You’ve been introduced to the chemistry behind Instagram and how the CometChat SDK makes the Instagram clone buildable.
You have seen how to build most of the chat functionalities such as real-time messaging using CometChat. I hope you enjoyed this tutorial and that you were able to successfully add mentions into your React chat app. Jump into the comments and let me know your experience.
About the Author
Hiep Le is a software engineer. He takes a huge interest in building software products and is a full-time software engineer. Most of his work is focused on one thing - to help people learn.
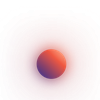
Hiep Le
CometChat