This tutorial is outdated. We're currently working on an updated version. In the meantime, for instructions on building a chat app using CometChat, please visit our documentation.
Mobile chat applications are transforming and revolutionizing not only the way we communicate, but also the way we do business. Once the exclusive realm of big tech organizations, mobile chat apps are now becoming more commonplace because of the rise of microservices like Chat APIs.
In this android chat and messaging app tutorial, we will be developing an android application with in-app chat using the CometChat Chat UI Kit. The most exciting part is that our chat kits can be easily setup to add chat to any android application in less than 30 minutes.
Now, let’s start working on everything you need to build your own android chat app!
Outlining the App

Before every project, the scope has to be defined. Hence, we have outlined the scope so that we know exactly what should be there in a real-time android chat app and what shouldn’t. Here are a few things that you should consider:
The app should have the following screens -
Login & Signup screens
A screen to display a list of all the chats
Chat message screen to do the actual chatting & display list of all the messages exchanged between in a chat.
A screen for the user to select a contact and to start a new chat.
Now that we have agreed on the scope, let’s jump straight into it.
Building the App
I. Setting Up the CometChat SDKs
Before starting with CometChat, it is important to understand how CometChat works! The Key Concepts page of CometChat documentation can help you with that.
Before diving into the code, we’ll need to set up CometChat in the application. CometChat Quick Start document does a wonderful job explaining the steps in detail.
We’ll outline the steps from the above document:
Here's how we will initialize the CometChat SDK via the Application class:
II. Developing the Login & Registration Flow

In an actual production app, I’d use my custom back-end application or Firebase for user management. Here, we’ll use CometChat’s User Management as a shortcut.
The registration page will ask for the user’s name and contact details. CometChat needs a unique identifier of every user. Here, we’ll consider mobile number as the user’s unique identifier - uid.
Now that we have our user’s name and uid, we’ll use CometChat’s createUser SDK method to create the user in our CometChat’s database. Then, in the final step, we’ll call CometChat’s login SDK method to log the user into our app.
You can also view your registered users in the CometChat Dashboard.
Let’s see the entire process in the code below.
We’ll also create a Login screen for already-registered users. Even they would be entering their mobile numbers to log in. We would use CometChat’s login function to accomplish this.
Here’s the code:
Note: I have skipped all the validation part here. The idea is to first complete the solution and then we add all the error handling and other bells and whistles. But in a production app, do make sure that you add validations on the fields to avoid crashes and unexpected behaviors.
After successful login, users should be routed to the screen which displays a list of all the user’s chats. We’ll call this screen → My Conversations.
You’ll notice that (in the code) on both the screens, in the Login onSuccess method, I am routing the screen to ConversationsActivity. That’s our “My Conversations” screen. We’ll be setting this screen up next.
III. Building Chat Screens
1. Setting Up the Conversation Screen

Once the user logs in to the app, we would be showing the user’s latest conversations. This screen contains a list of all his one-to-one and group conversations.
For this, we will have to first create an activity - ConversationsActivity.
In our layout file - activity_conversations.xml, add the following snippet to use the CometChatConversationList. Adding this will give you a fully working list of conversations.
CometChatConversationList is a part of CometChat’s Android UI Kit. We won’t have to create RecyclerView, Adapter or any custom development of any sorts to display our conversation list. That’s right! CometChat’s UI Kit handles it all for us.
2. Setting Up User Selection Screen for New Chat
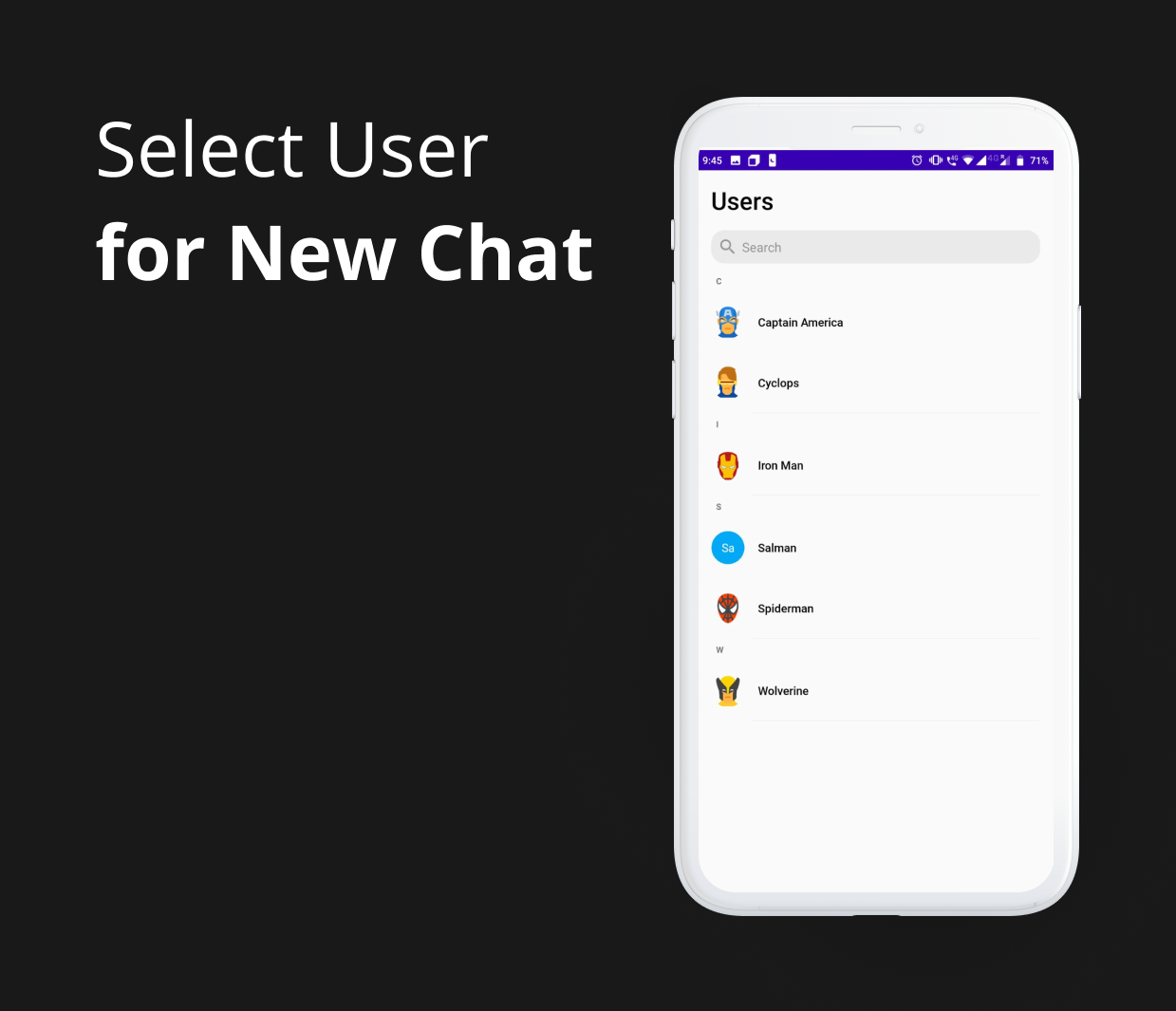
We need a button on the conversations screen for the user to click and initiate a new chat.
Let’s add it:
And the routing function in the code would be like this:
This User selection screen needs to show a list of all the registered users here. Let’s go ahead and implement the activity to display a list of all the users registered in our app.
In our XML layout file - activity_select_contact.xml, add the following snippet to use the CometChatUserList. Adding this will give you a fully working list of users.
CometChatUserList is also a part of CometChat’s Android Chat UI Kit. Like CometChatConversationList, we won’t have to create RecyclerView, Adapter or any custom development of any sorts to display our user list. We won’t even have to call the API ourselves!
We have both our Conversations List Screen and New User Selection Screen ready. Now, the final missing piece of the puzzle that’s pending is the actual Chat screen! We need to route the user to this Chat screen when the user clicks on any of the conversation or user.
Let’s get our code rolling and finish it!
3. Implementing the Chat Screen

Once the user clicks on a Conversation or Contact item, we would route the user to CometChat’s Chat Message screen. We would use CometChatMessageListActivity from CometChat’s Android UI Kit library itself. It displays a list of all the messages, images, and the history of the entire chat with that person. It is capable of sending and receiving different types of messages such as text, image, and documents.
No UI development or API calls is needed, which means we can get on with our chat feature.
Like we said earlier, we need to route the user to this Chat screen from both our Conversations List Screen and User Selection Screen. CometChat has a solution for this too — it has ready-made click listener events for both these lists.
Let’s first add it in our Conversations List Screen:
And now, add the same in the User Selection Screen:
That’s it! We have our Chat application ready in less than 30 minutes!
Try it out yourself!
Here’s the repository to check out the complete code and try out the app yourself!
https://github.com/cometchat-pro-tutorials/QuickChat-Android
Steps to run the demo app —
Head to CometChat Pro and create an account
From the dashboard, create a new app and name it whatever you like. For me, it’s "QuickChat"
Once created, click on Explore
Go to the API & Auth Keys tab in the side menu
Copy the App ID, Auth Key & API Key.
Download the repository from the above Github URL.
Replace the App_ID and Auth_Key from the AppConfig file.
Boom! Run the app!
Conclusion
In this tutorial, we built a chat application that leverages CometChat UI Kit for Android and allows users to register and use the application to chat with others. You saw how easy it is to install and set up CometChat and quickly create new screens and implement complex functionalities. Moreover, you learned how easy it is to customize the behavior and build any kind of chat or messaging experience.
I hope this tutorial was helpful and also insightful and I can wait to see how you utilize the great possibilities made by CometChat alongside Android.
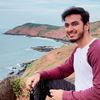
Nabil Kazi
CometChat