Conversations with Messages
Overview
The CometChatConversationsWithMessages
is a Composite Widget encompassing widgets such as Conversations, Messages, and Contacts. Each of these widgets contributes to the functionality and structure of the overall CometChatConversationsWithMessages
widget.
- Android
- iOS
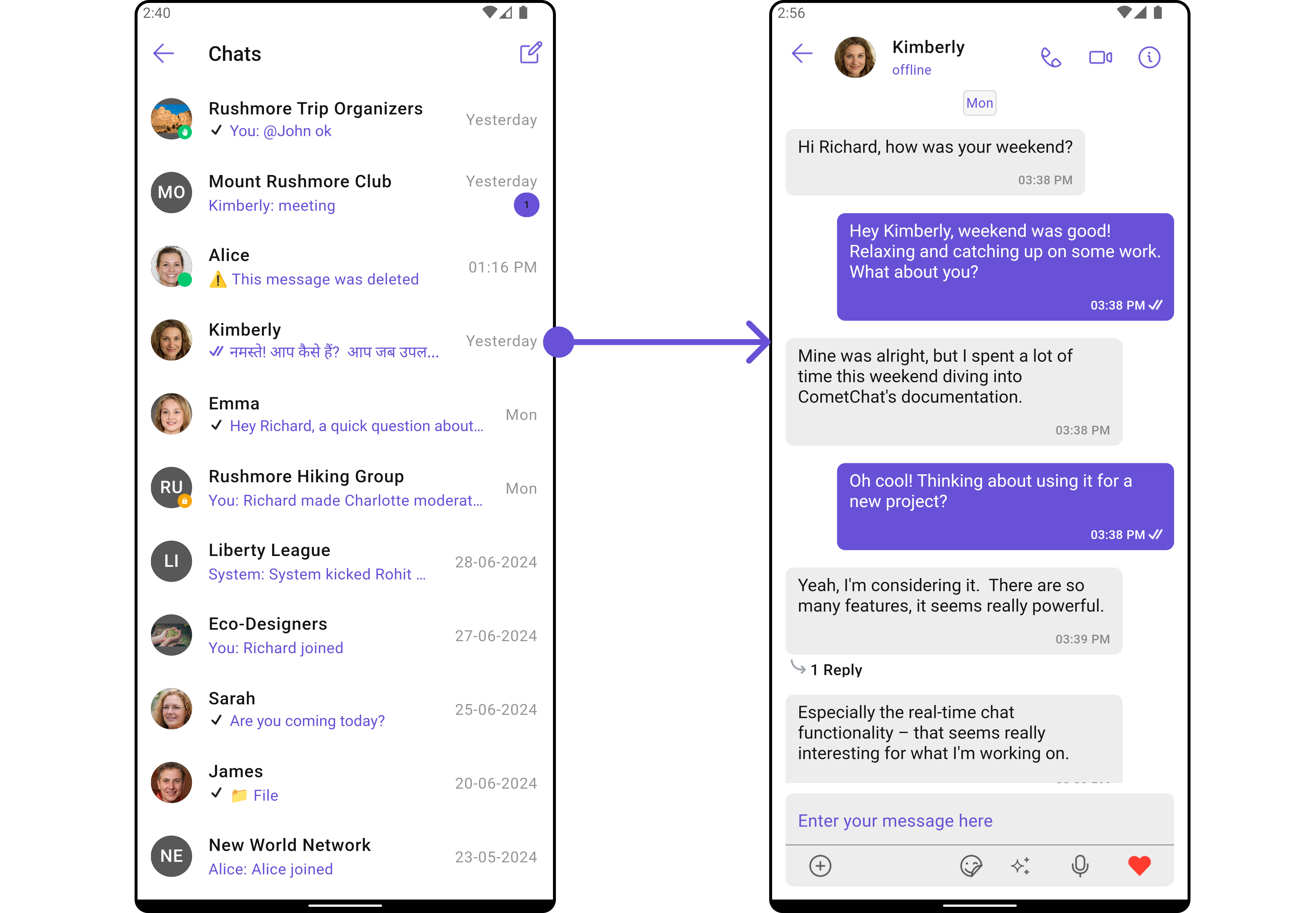
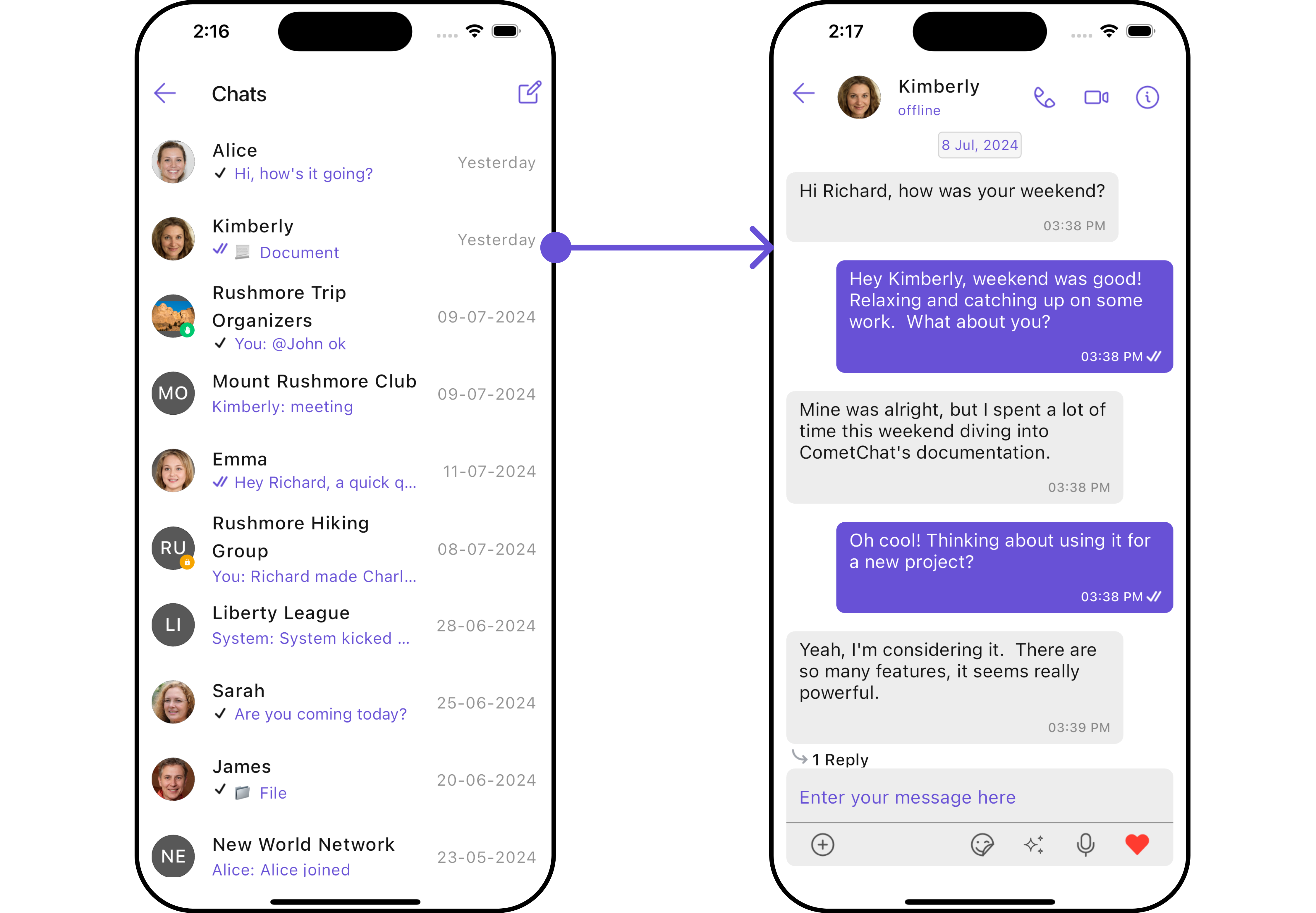
Widgets | Description |
---|---|
Conversations | The Conversations widget is designed to display a list of either User or Group . This essentially represents your recent conversation history. |
Messages | The Messages widget is designed to manage the messaging interaction for either individual User or Group conversations. |
Contacts | The CometChatContacts widget is specifically designed to facilitate the display and management of both User and Groups . |
Usage
Integration
Since CometChatConversationsWithMessages
is a widget, it can be launched either by a button click or through any event's trigger. It inherits all the customizable properties and methods of CometChatConversations.
You can launch CometChatConversationsWithMessages
directly using Navigator.push
, or you can define it as a widget within the build
method of your State
class.
1. Using Navigator to Launch CometChatConversationsWithMessages
- Dart
Navigator.push(context, MaterialPageRoute(builder: (context) => const CometChatConversationsWithMessages()));
2. Embedding CometChatConversationsWithMessages
as a Widget in the build Method
- Dart
import 'package:cometchat_chat_uikit/cometchat_chat_uikit.dart';
import 'package:flutter/material.dart';
class ConversationsWithMessages extends StatefulWidget {
const ConversationsWithMessages({super.key});
State<ConversationsWithMessages> createState() => _ConversationsWithMessagesState();
}
class _ConversationsWithMessagesState extends State<ConversationsWithMessages> {
Widget build(BuildContext context) {
return const Scaffold(
body: SafeArea(
child: CometChatConversationsWithMessages()
)
);
}
}
List of properties exposed by CometChatConversationsWithMessages
Property | Data Type | Description |
---|---|---|
user | User? | The user object representing the current user. |
group | Group? | The group object representing the current group. |
theme | Theme? | The theme object for customizing the appearance of the widget. |
conversationsConfiguration | ConversationsConfiguration? | Configuration settings for the conversations list. |
messageConfiguration | MessageConfiguration? | Configuration settings for individual messages. |
startConversationConfiguration | StartConversationConfiguration? | Configuration settings for starting new conversations. |
Actions
Actions dictate how a widget functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the widget to fit your specific needs.
While the CometChatConversationsWithMessages
widget does not have its actions, its sub-widgets - Conversation, Messages, and Contacts - each have their own set of actions.
The actions of these widgets can be overridden through the use of the Configurations object of each widget. Here is an example code snippet.
- Dart
CometChatConversationsWithMessages(
conversationsConfiguration: ConversationsConfiguration(
onItemTap: (conversation) {
// TODO("Not yet implemented")
}
),
)
- Android
- iOS
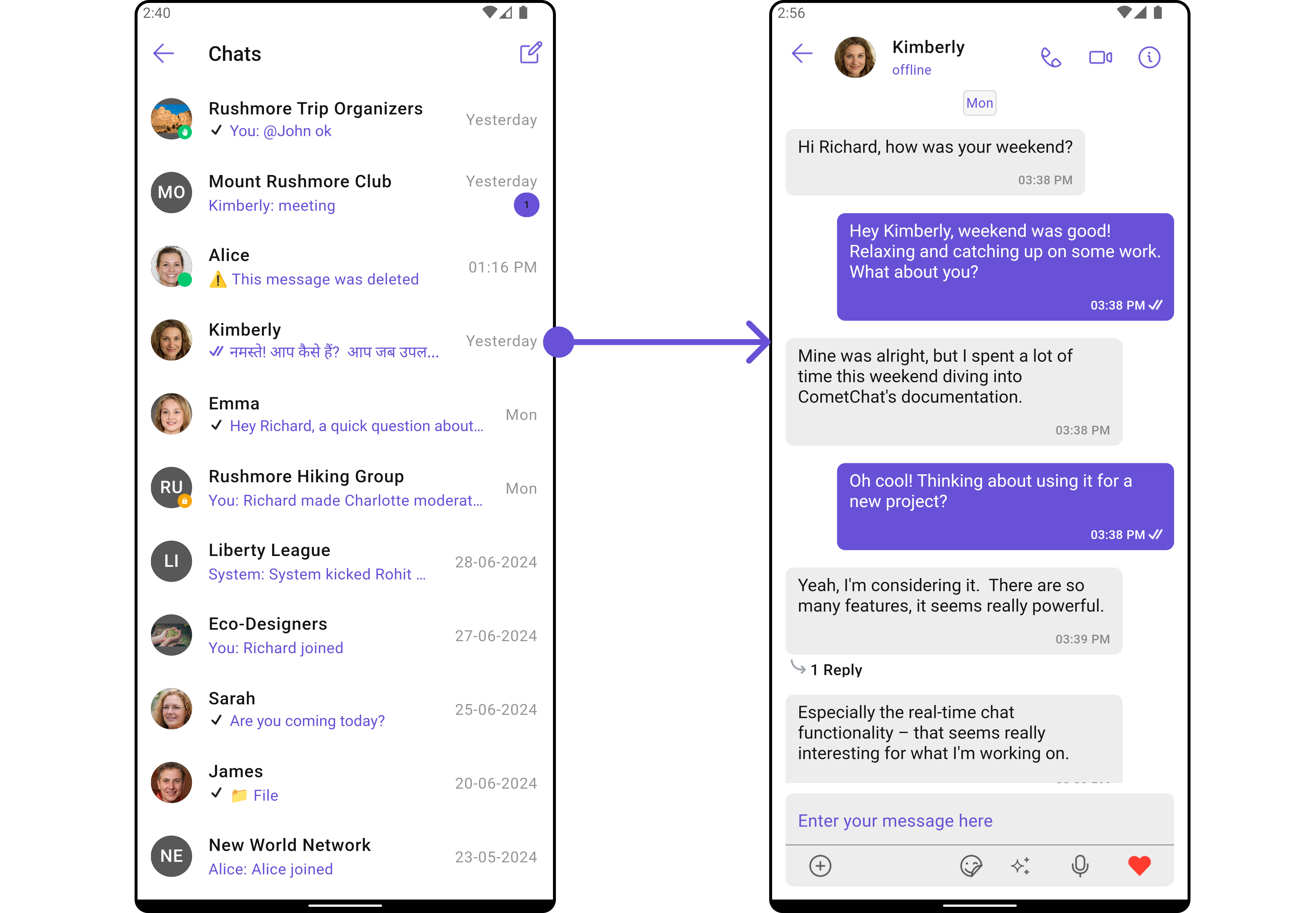
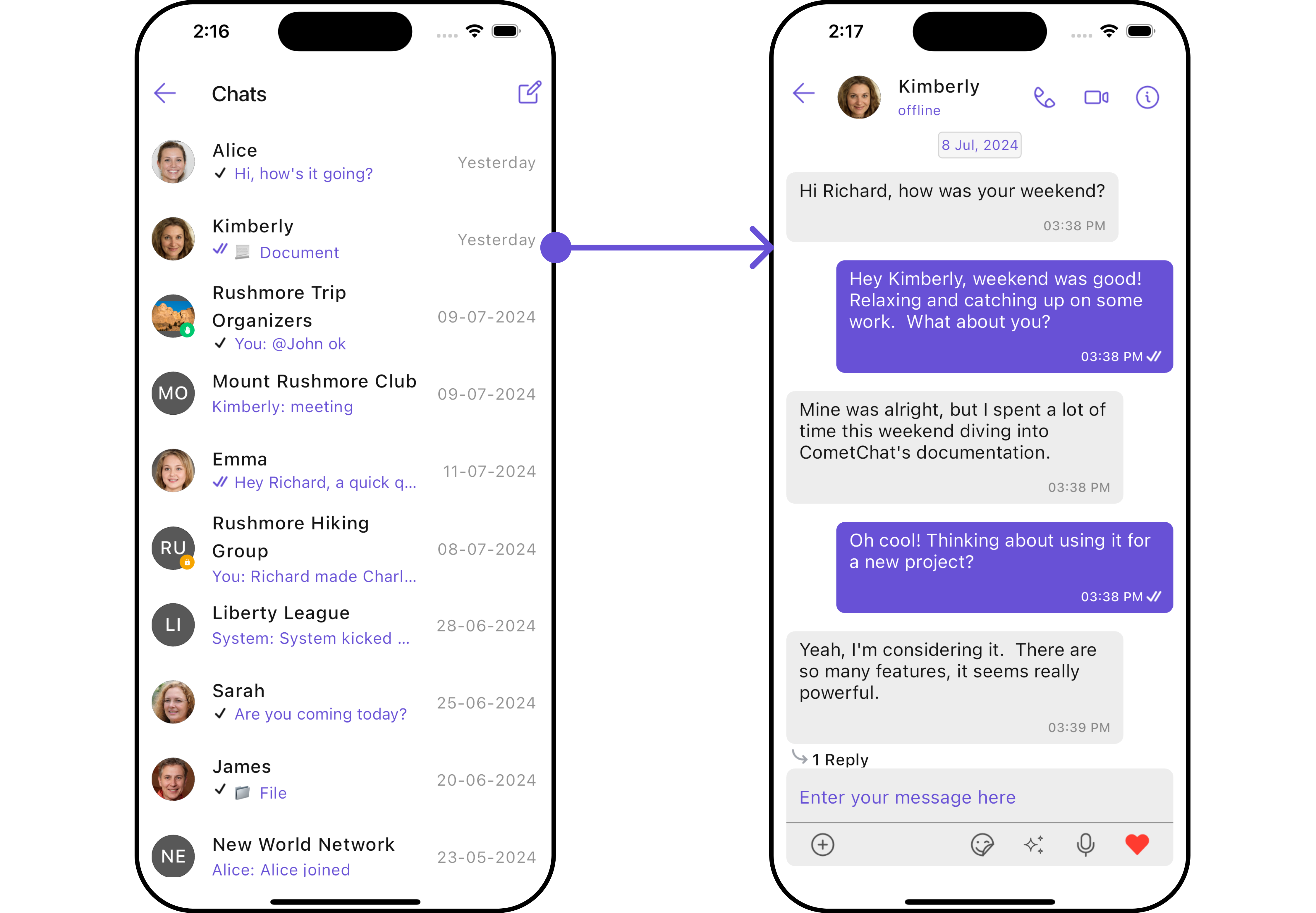
Filters
Filters allow you to customize the data displayed in a list within a Widget. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of ChatSDK.
While the CometChatConversationsWithMessages
widget does not have filters, its widgets do, For more detail on individual filters of its widget refer to Conversations Filters and Messages Filters.
By utilizing the Configurations object of its widgets, you can apply filters.
In the following example, we're filtering Conversation to only show User
- Dart
CometChatConversationsWithMessages(
conversationsConfiguration: ConversationsConfiguration(
conversationsRequestBuilder: ConversationsRequestBuilder()
..limit = 5
..conversationType = "user"
),
)
Events
Events are emitted by a Widget
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The CometChatConversationsWithMessages
does not produce any events but its subwidget does. For a full list of these events, you can refer to Conversations events and Messages events.
In the following example, we're incorporating observers for the ConversationDeleted
event of Conversations
widget.
This ccConversationDeleted
will be emitted when the user deletes a conversation
- Dart
import 'package:cometchat_chat_uikit/cometchat_chat_uikit.dart';
class _YourScreenState extends State<YourScreen> with CometChatConversationEventListener {
void initState() {
super.initState();
CometChatConversationEvents.addConversationListListener("listenerId", this); // Add the listener
}
void dispose(){
super.dispose();
CometChatConversationEvents.removeConversationListListener("listenerId"); // Remove the listener
}
void ccConversationDeleted(Conversation conversation) {
// TODO("Not yet implemented")
}
}
Customization
To fit your app's design requirements, you have the ability to customize the appearance of the CometChatConversationsWithMessages
widget. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the widget in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the widget. CometChatConversationsWithMessages widget doesn't have its own style parameters. But you can customize its widget styles. For more details on individual widget styles, you can refer Conversation Styles, Messages Styles, and Contacts Styles
Styles can be applied to SubWidgets using their respective configurations.
- Dart
CometChatConversationsWithMessages(
conversationsConfiguration: ConversationsConfiguration(
avatarStyle: AvatarStyle(
border: Border.all(width: 2),
borderRadius: 20,
background: Colors.red
),
statusIndicatorStyle: const StatusIndicatorStyle(
borderRadius: 10,
width: 2
),
dateStyle: const DateStyle (
contentPadding: EdgeInsets.all(2),
background: Colors.grey
)
),
)
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the widget. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
Set User
You can utilize the user
function with a User object as input to CometChatConversationsWithMessages
. This will automatically guide you to the Messages widget for the designated User
.
- Dart
CometChatConversationsWithMessages(
user: User(
name: "",
uid: "",
avatar: ""
),
)
Set Group
You can utilize the set(group: Group?)
function with a Group object as input to CometChatConversationsWithMessages
. This will automatically guide you to the Messages widget for the designated Group
.
- Dart
CometChatConversationsWithMessages(
group: Group(
guid: "",
name: "",
type: ""
),
)
Widgets
Nearly all functionality customizations are available for the composite widget. Using Configuration, you can modify the properties of its widgets to suit your needs.
You can find the list of all Functionality customization of individual widgets in Conversations , Messages, and Contacts
- Dart
CometChatConversationsWithMessages(
conversationsConfiguration: ConversationsConfiguration(
hideReceipt: true,
title: "Your Title",
hideSeparator: true,
hideStartConversation: true,
backButton: Icon(Icons.add_alert_outlined, color: Color(0xFF6851D6),)
),
)
Advanced
For advanced-level customization, you can set custom views to the widget. This lets you tailor each aspect of the widget to fit your exact needs and application aesthetics. You can create and define your own widget and then incorporate those into the widget.
By utilizing the Configuration object of each widget, you can apply advanced-level customizations to the ConversationsWithMessages.
- Dart
CometChatConversationsWithMessages(
conversationsConfiguration: ConversationsConfiguration(),
)
To find all the details on individual Widget advance customization you can refer, Conversations Advance,Messages Advance and Contacts Advance
ConversationsWithMessages uses advanced-level customization of both Conversation & Messages widgets to achieve its default behavior.
- ConversationsWithMessages utilizes the SetMenu function of the
Conversations
subwidget to navigate the user from Conversations to Contacts
- Android
- iOS
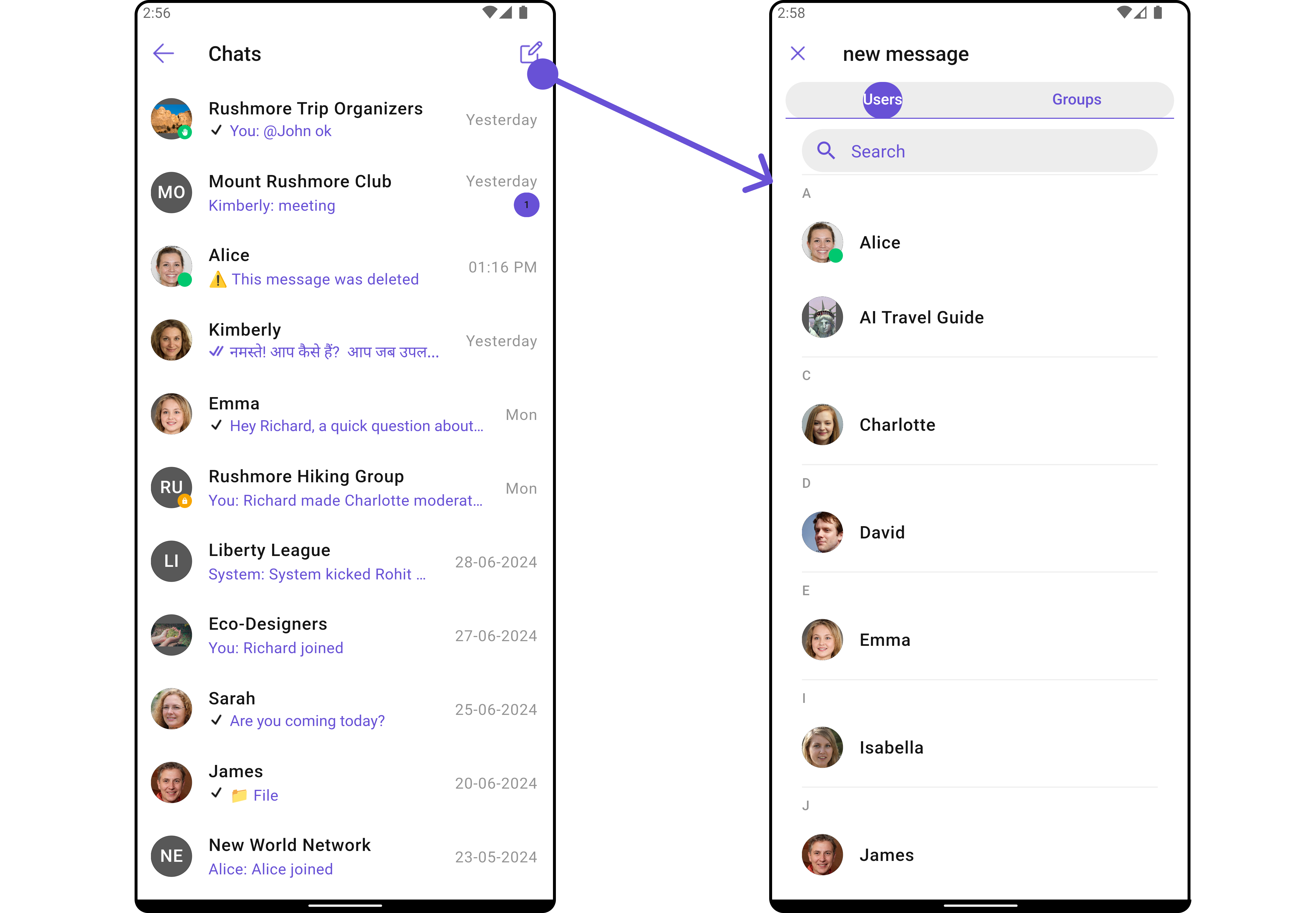
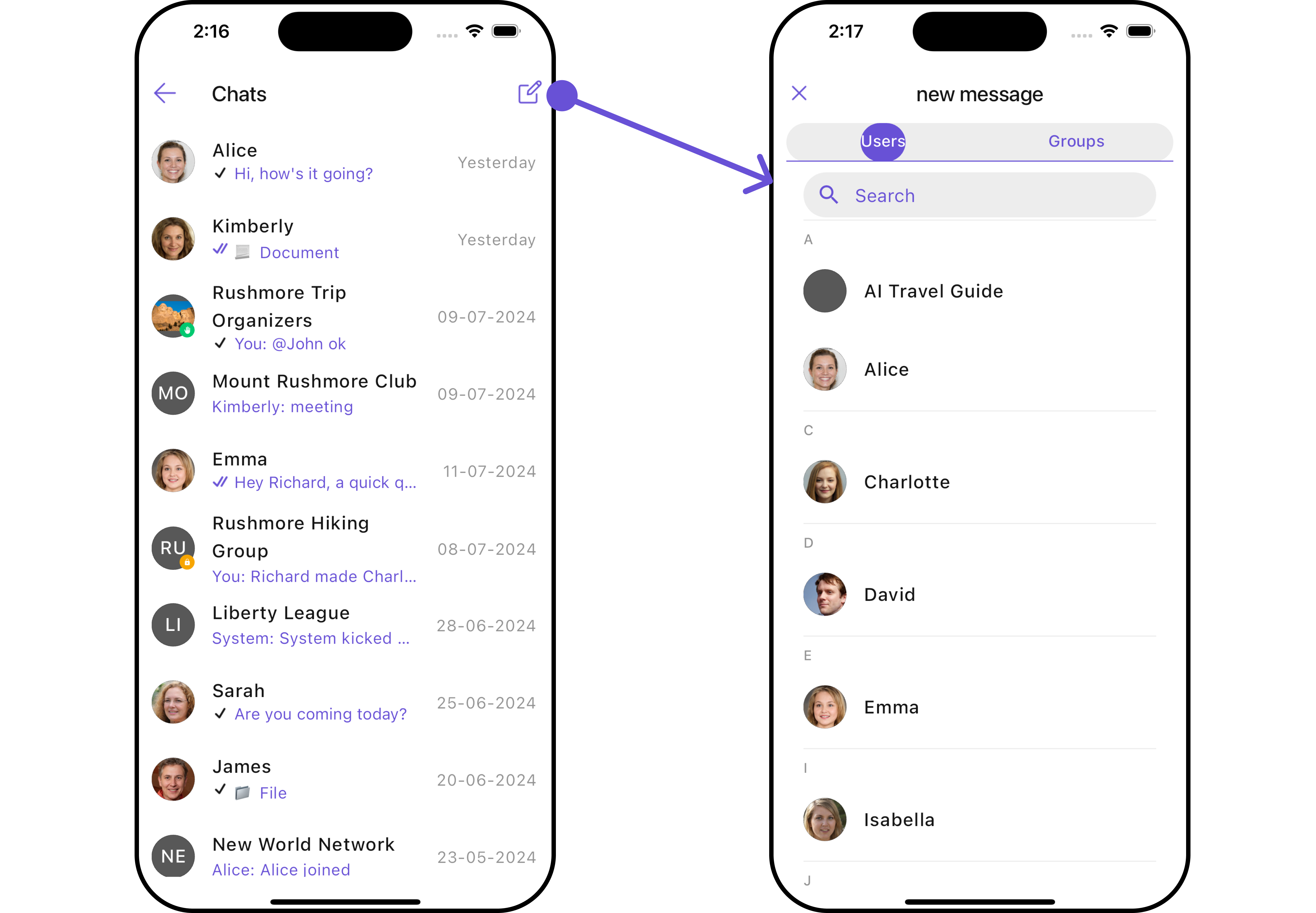
- ConversationsWithMessages utilizes the SetMenu function of the
Messages
subwidget to navigate the user from Messages to Details.
- Android
- iOS
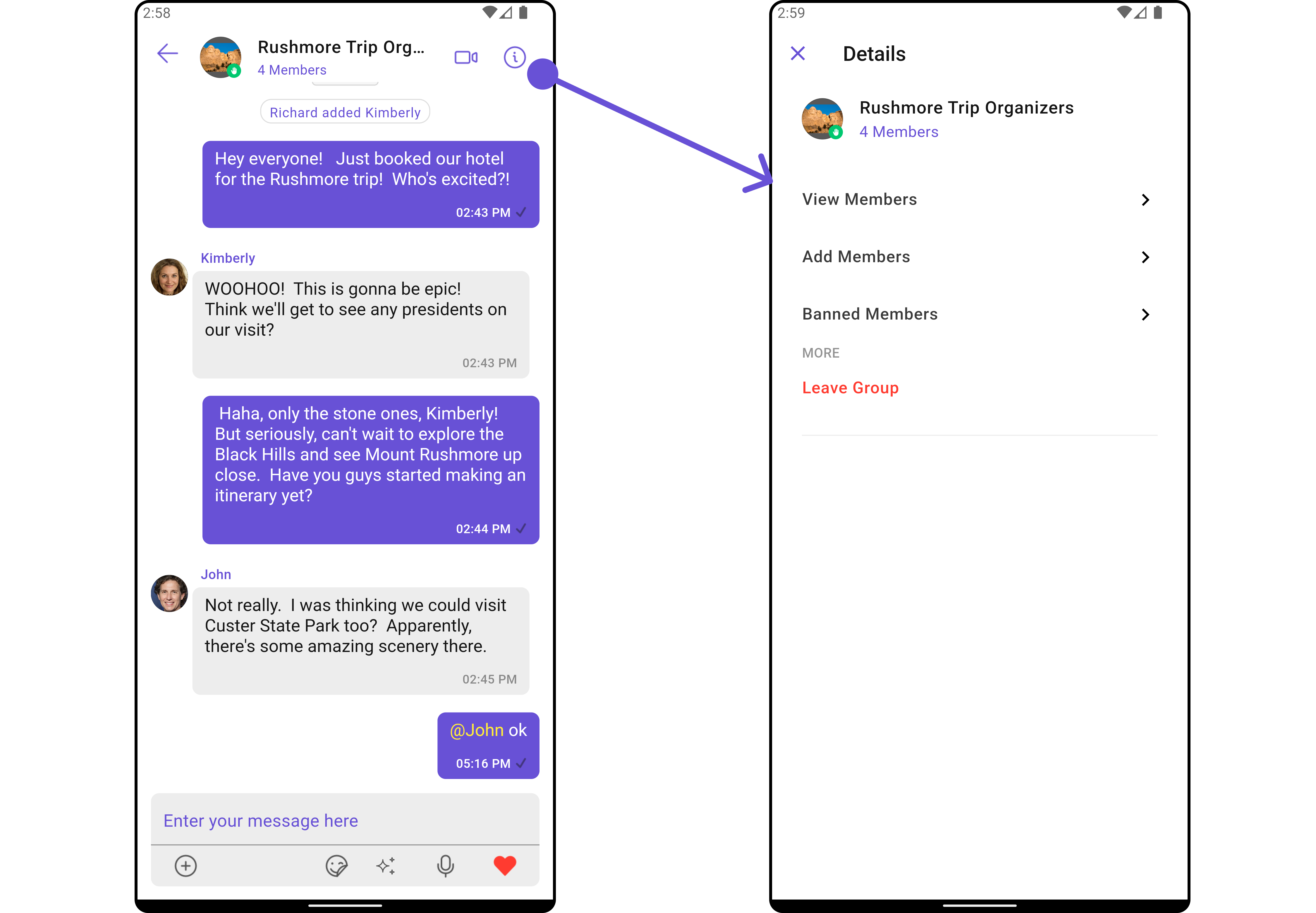
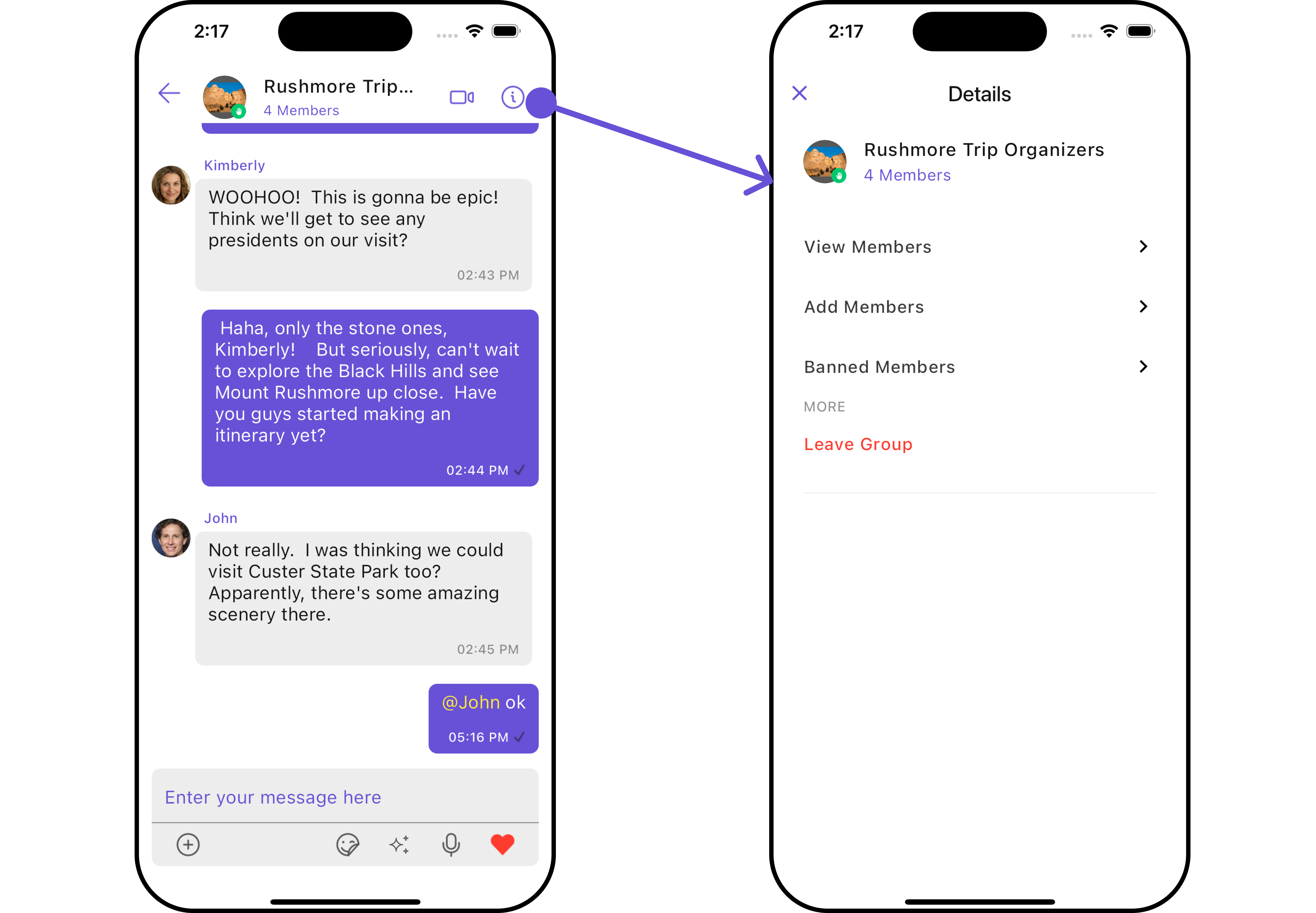
Configurations
Configurations offer the ability to customize the properties of each widget within a Composite Widget.
CometChatConversationsWithMessages has Conversations
, Messages
, and Contacts
widget. Hence, each of these widgets will have its individual `Configuration``.
Configurations
expose properties that are available in its individual widgets.
Conversations
You can customize the properties of the Conversations widget by making use of the ConversationsConfiguration. You can accomplish this by employing the .conversationsConfiguration
method as demonstrated below:
- Dart
CometChatConversationsWithMessages(
conversationsConfiguration: ConversationsConfiguration(),
)
Example
- Dart
CometChatConversationsWithMessages(
conversationsConfiguration: ConversationsConfiguration(
avatarStyle: AvatarStyle(
border: Border.all(width: 2),
borderRadius: 20,
background: Colors.red
),
statusIndicatorStyle: const StatusIndicatorStyle(
borderRadius: 10,
width: 2
),
dateStyle: const DateStyle (
contentPadding: EdgeInsets.all(2),
background: Colors.grey
)
),
)
- Android
- iOS
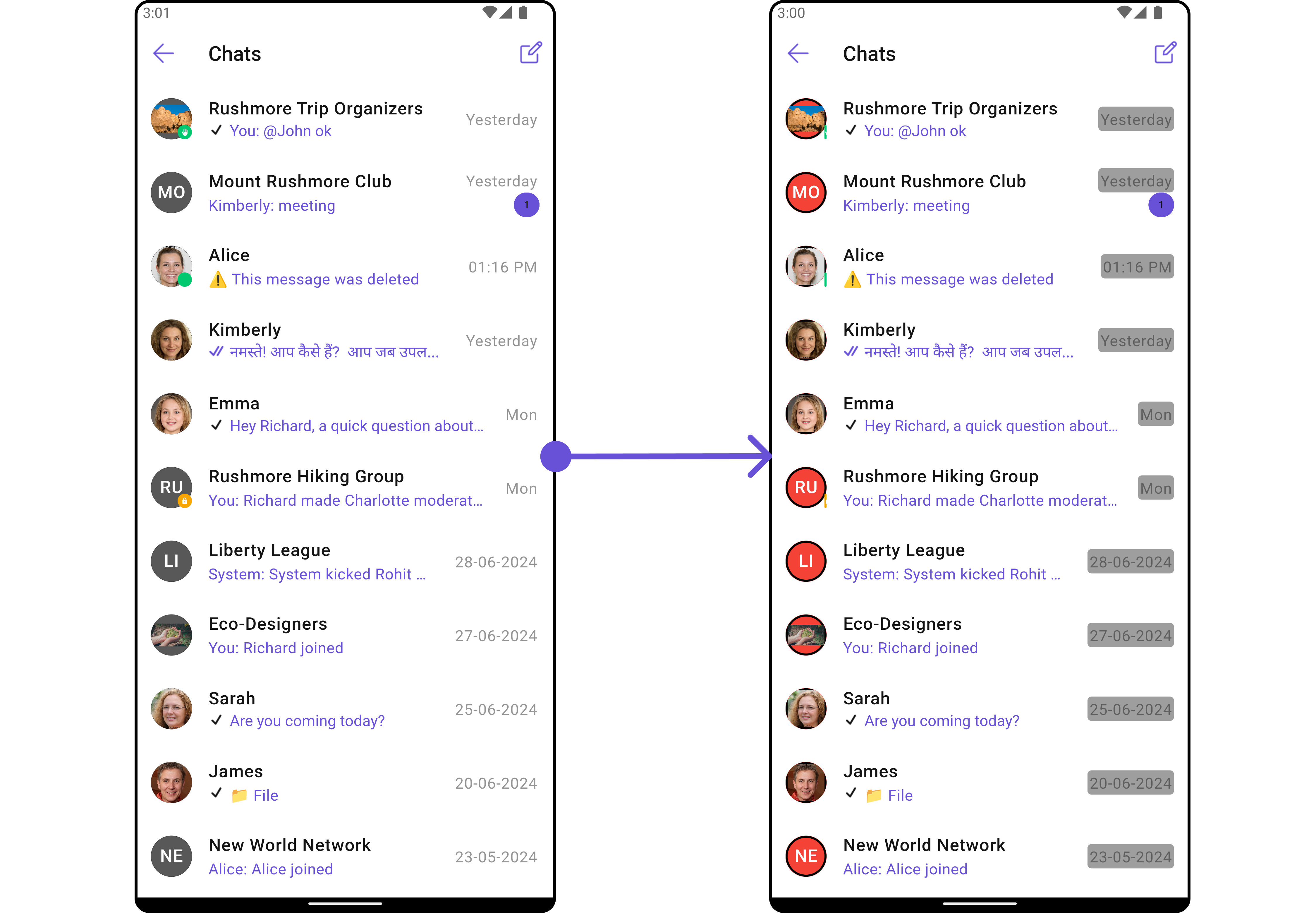
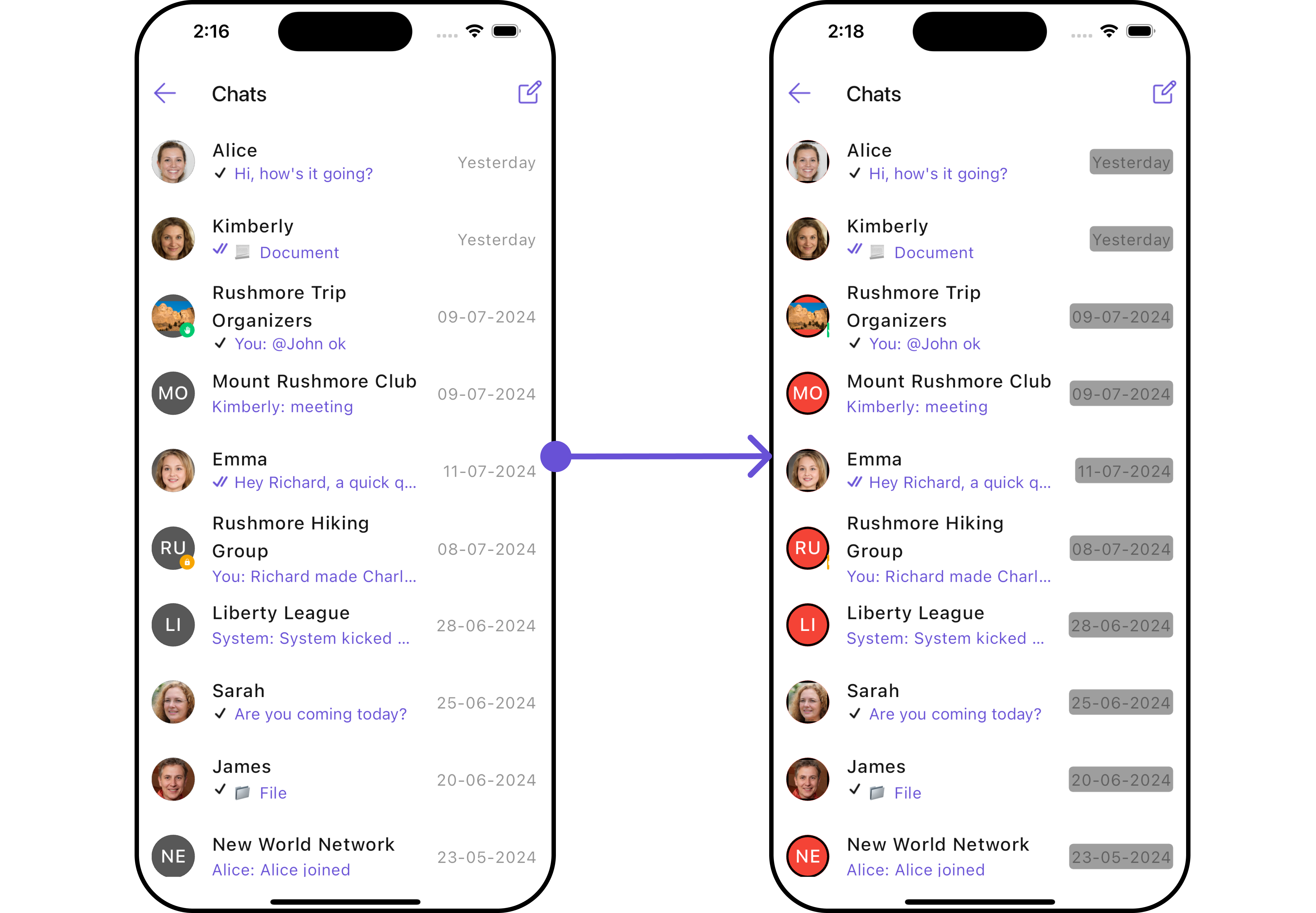