Reaction List
Overview
The Reaction List
component provides a comprehensive display of reactions associated with a message, showcasing which reactions were made by individual users. Additionaly, users can interact with the list to remove their own reactions.
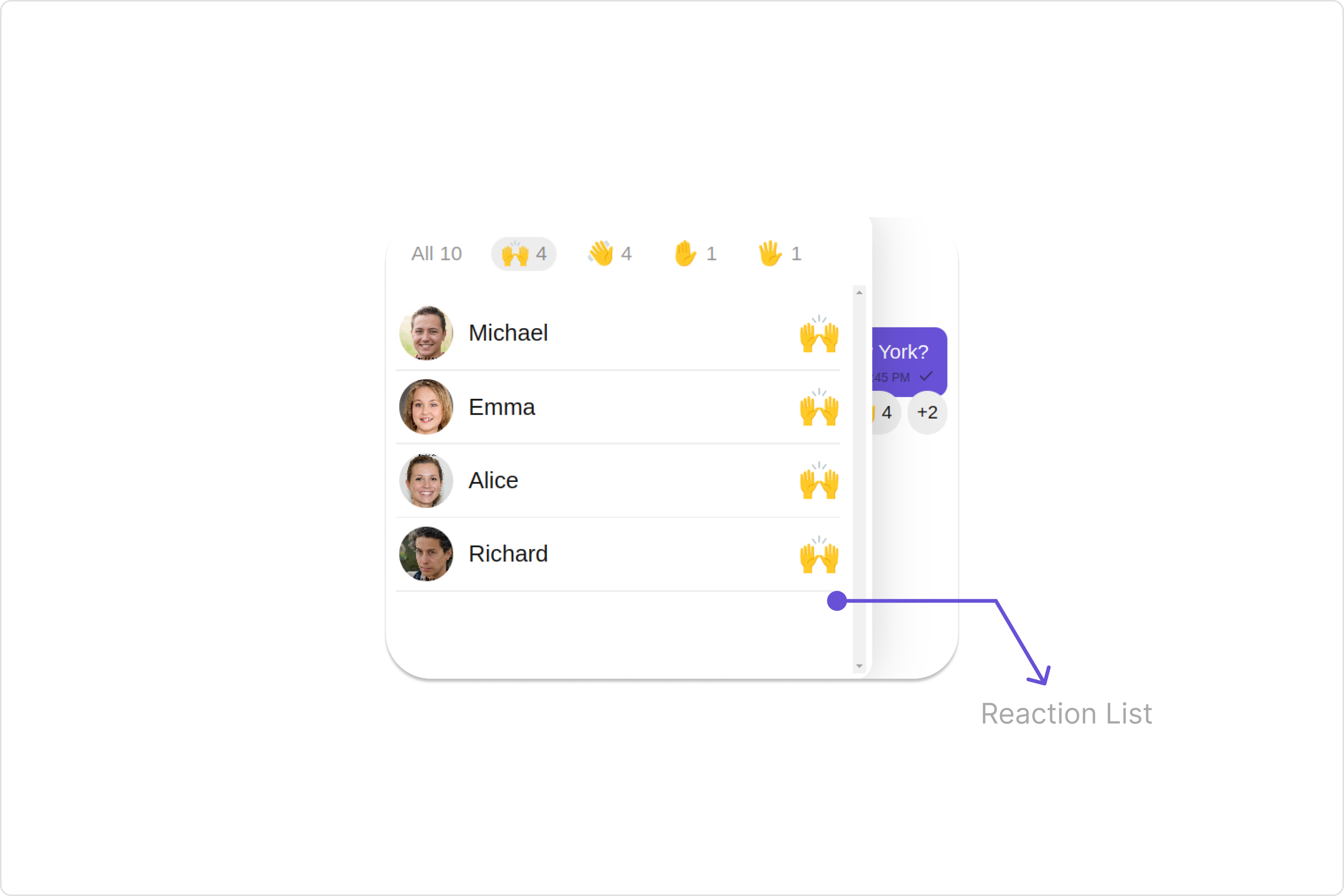
Usage
Integration
The following code snippet illustrates how you can directly incorporate the Reactions list component into your app.
- app.module.ts
- app.component.ts
- app.component.html
import { CUSTOM_ELEMENTS_SCHEMA, NgModule } from "@angular/core";
import { BrowserModule } from "@angular/platform-browser";
import { AppComponent } from "./app.component";
@NgModule({
imports: [BrowserModule],
declarations: [AppComponent],
providers: [],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA],
})
export class AppModule {}
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { ReactionListStyle } from '@cometchat/uikit-shared';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public messageObject!: CometChat.TextMessage;
ngOnInit(): void {
CometChat.getMessageDetails("messageId").then((message) => {
this.messageObject = message;
}).catch((error) => {
console.error('Error fetching message details:', error);
});
}
reactionListStyle = new ReactionListStyle({
background:'#9a41fa',
border:'2px solid #6e2eb3',
borderRadius:'20px',
activeEmojiBackground:'#d4b2f7',
subtitleTextColor:'#f4ebff',
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-reaction-list
*ngIf="messageObject"
[messageObject]="messageObject"
[reactionListStyle]="reactionListStyle"
></cometchat-reaction-list>
</div>
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. reactionItemClicked
The reactionItemClicked
event gets activated when a user clicks on a reaction item within the CometChat Reaction List component. This event provides a way to capture and respond to user interactions with specific reactions.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public messageObject!: CometChat.TextMessage;
ngOnInit(): void {
CometChat.getMessageDetails("messageId").then((message) => {
this.messageObject = message;
}).catch((error) => {
console.error('Error fetching message details:', error);
});
}
public handleReactionOnItemClicked = () => {
console.log("your custom on reaction item click actions");
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-reaction-list
*ngIf="messageObject"
[messageObject]="messageObject"
[reactionItemClicked]="handleReactionOnItemClicked"
></cometchat-reaction-list>
</div>
Filters
Filters allow you to customize the data displayed in a list within a Component
. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders
of Chat SDK.
You can adjust the ReactionsRequestBuilder
in the Reaction list Component to customize your Reaction list. Numerous options are available to alter the builder to meet your specific needs. For additional details on ReactionsRequestBuilder
, please visit ReactionsRequestBuilder.
In the example below, we demonstrate the application of a filter to the reactions list
. This filter allows you to specify a limit of the users who have reacted to the perticular message.
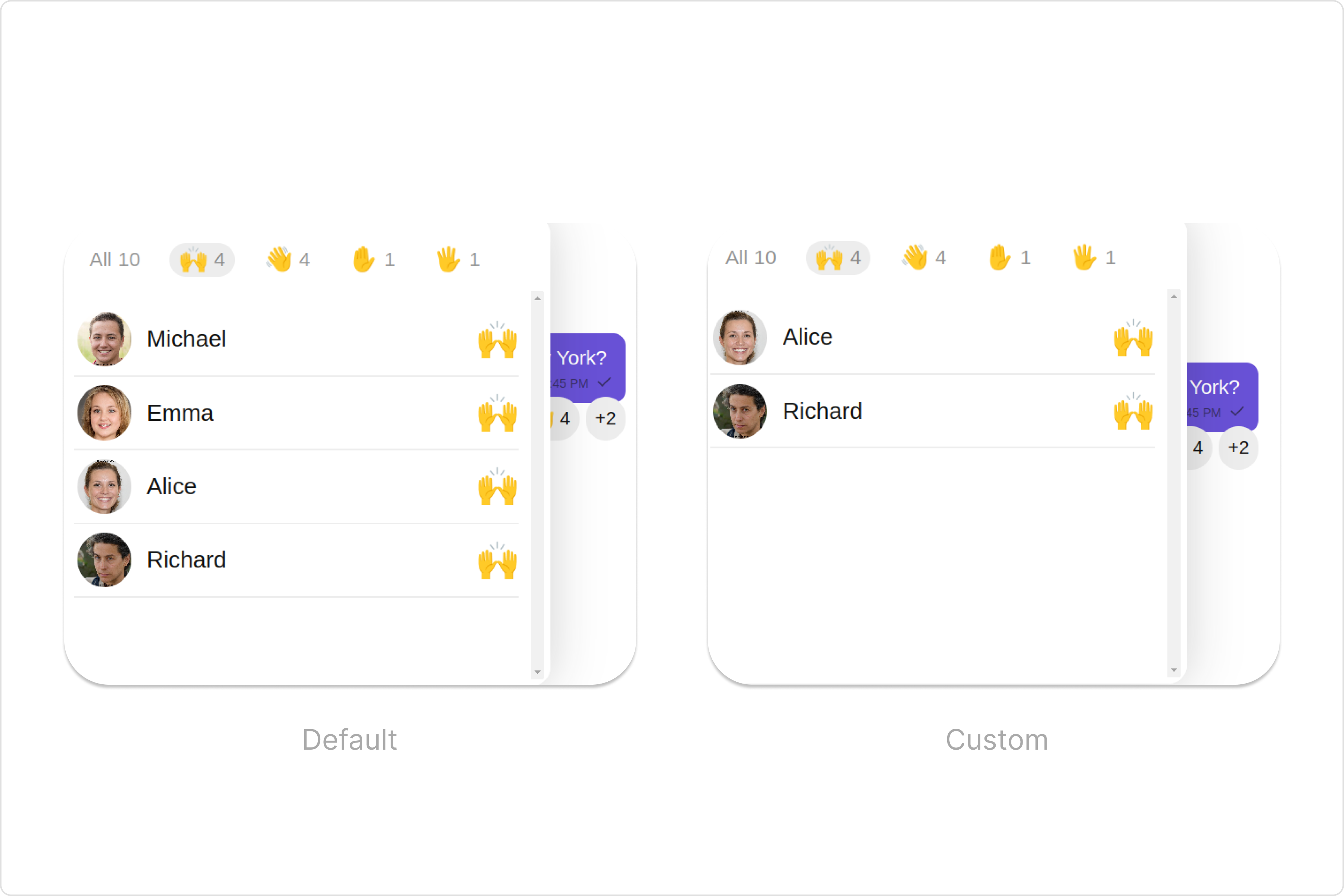
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public messageObject!: CometChat.TextMessage;
ngOnInit(): void {
CometChat.getMessageDetails("messageId").then((message) => {
this.messageObject = message;
}).catch((error) => {
console.error('Error fetching message details:', error);
});
}
reactionsRequestBuilder = new CometChat.ReactionsRequestBuilder().setLimit(2);
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-reaction-list
*ngIf="messageObject"
[messageObject]="messageObject"
[reactionsRequestBuilder]="reactionsRequestBuilder"
></cometchat-reaction-list>
</div>
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The Reactions list
component does not produce any events.
Customization
To fit your app's design requirements, you can customize the appearance of the Reaction List component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. reactionListStyle
To customize the appearance, you can assign a reactionListStyle
object to the Reactions List
component.
Example
In this example, we are employing the reactionListStyle
.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import { ReactionListStyle } from '@cometchat/uikit-shared';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public messageObject!: CometChat.TextMessage;
ngOnInit(): void {
CometChat.getMessageDetails("messageId").then((message) => {
this.messageObject = message;
}).catch((error) => {
console.error('Error fetching message details:', error);
});
}
reactionListStyle = new ReactionListStyle({
background:'#9a41fa',
border:'2px solid #6e2eb3',
borderRadius:'20px',
activeEmojiBackground:'#d4b2f7',
subtitleTextColor:'#f4ebff',
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-reaction-list
*ngIf="messageObject"
[messageObject]="messageObject"
[reactionListStyle]="reactionListStyle"
></cometchat-reaction-list>
</div>
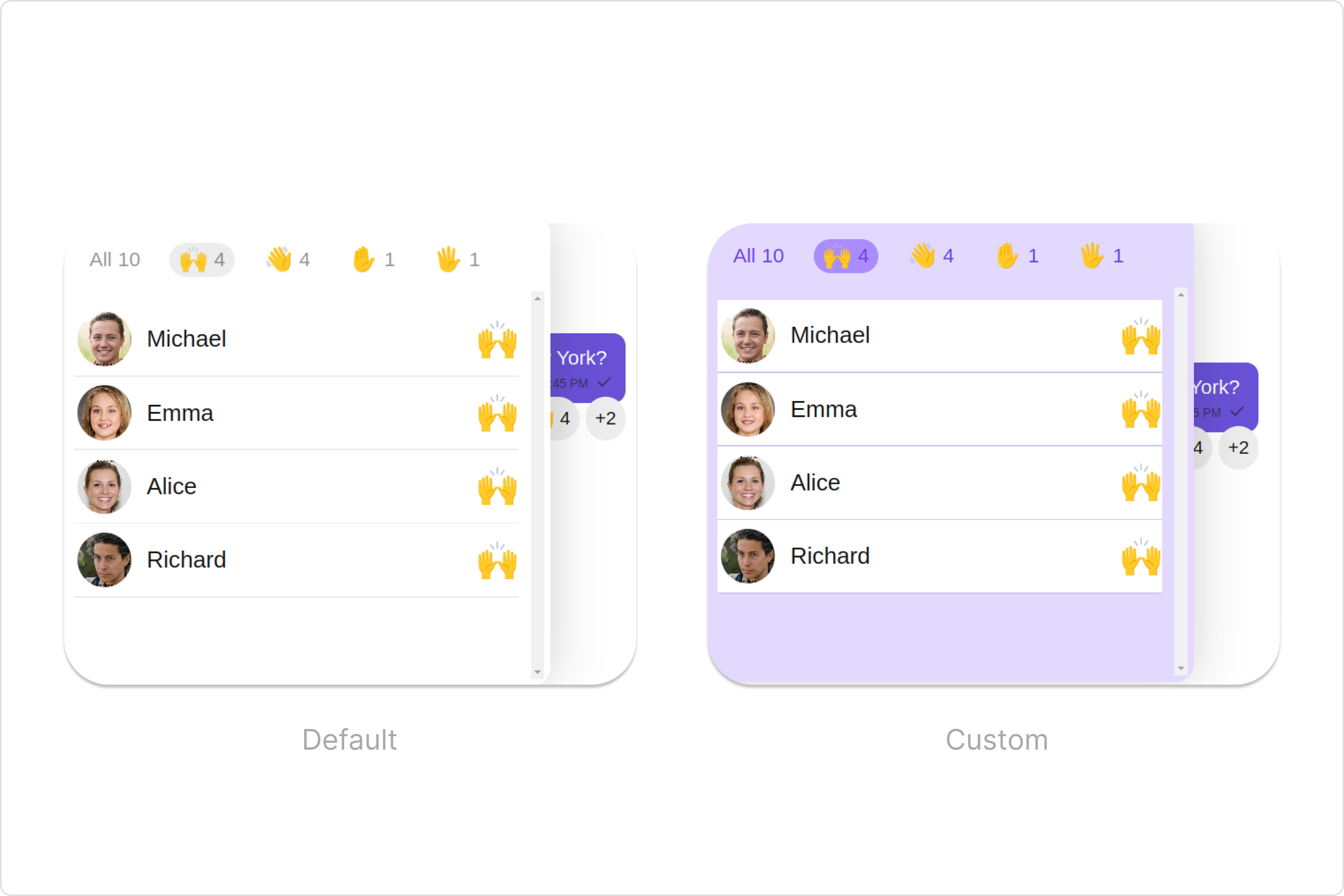
List of properties exposed by ReactionsListStyle
Property | Description | Code |
---|---|---|
border | Used to set border | border?: string, |
borderRadius | Used to set border radius | borderRadius?: string; |
background | Used to set background colour | background?: string; |
height | Used to set height | height?: string; |
width | Used to set width | width?: string; |
sliderEmojiFont | used to set the font of slider emoji | sliderEmojiFont?: string; |
sliderEmojiCountFont | used to set the font of the slider emoji count | sliderEmojiCountFont?: string; |
sliderEmojiCountColor | used to set the color of the slider emoji count | sliderEmojiCountColor?: string; |
activeEmojiBackground | used to set the active emoji background | activeEmojiBackground?: string; |
loadingIconTint | used to set the loading icon color | loadingIconTint?: string; |
errorIconTint | used to set the error icon color | errorIconTint?: string; |
tailViewFont | used to set the tail view font | tailViewFont?: string; |
subtitleTextFont | used to set the subtitle text font | subtitleTextFont?: string; |
subtitleTextColor | used to set the subtitle text color | subtitleTextColor?: string; |
dividerTint | used to set the divider color | dividerTint?: string; |
2. Avatar Style
If you want to apply customized styles to the Avatar
component within the Reaction List
Component, you can use the following code snippet. For more information you can refer Avatar Styles.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, AvatarStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public messageObject!: CometChat.TextMessage;
ngOnInit(): void {
CometChat.getMessageDetails("messageId").then((message) => {
this.messageObject = message;
}).catch((error) => {
console.error('Error fetching message details:', error);
});
}
avatarStyle = new AvatarStyle({
backgroundColor: "#cdc2ff",
border: "2px solid #6745ff",
borderRadius: "10px",
outerViewBorderColor: "#ca45ff",
outerViewBorderRadius: "5px",
nameTextColor: "#4554ff"
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-reaction-list
*ngIf="messageObject"
[messageObject]="messageObject"
[avatarStyle]="avatarStyle"
></cometchat-reaction-list>
</div>
3. ListItem Style
If you want to apply customized styles to the List Item
component within the Reaction List
Component, you can use the following code snippet. For more information, you can refer ListItem Styles.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit, ListItemStyle } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public messageObject!: CometChat.TextMessage;
ngOnInit(): void {
CometChat.getMessageDetails("messageId").then((message) => {
this.messageObject = message;
}).catch((error) => {
console.error('Error fetching message details:', error);
});
}
listItemStyle: ListItemStyle = new ListItemStyle({
background: "transparent",
padding: "5px",
border: "1px solid #e9b8f5",
titleColor: "#8830f2",
borderRadius: "20px",
width: "100% !important"
});
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-reaction-list
*ngIf="messageObject"
[messageObject]="messageObject"
[listitemstyle]="listItemStyle"
></cometchat-reaction-list>
</div>
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
- app.component.ts
- app.component.html
import { CometChat } from '@cometchat/chat-sdk-javascript';
import { Component, OnInit } from '@angular/core';
import { CometChatThemeService, CometChatUIKit } from '@cometchat/chat-uikit-angular';
import "@cometchat/uikit-elements";
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent {
constructor(private themeService:CometChatThemeService) {
themeService.theme.palette.setMode("light")
themeService.theme.palette.setPrimary({ light: "#6851D6", dark: "#6851D6" })
}
public messageObject!: CometChat.TextMessage;
ngOnInit(): void {
CometChat.getMessageDetails("messageId").then((message) => {
this.messageObject = message;
}).catch((error) => {
console.error('Error fetching message details:', error);
});
}
onLogin(UID?: any) {
CometChatUIKit.login({ uid: UID }).then(
(user) => {
setTimeout(() => {
window.location.reload();
}, 1000);
},
(error) => {
console.log("Login failed with exception:", { error });
}
);
}
}
<div class="fullwidth">
<cometchat-reaction-list
*ngIf="messageObject"
[messageObject]="messageObject"
[loadingIconURL]="your custom icon"
[errorIconURL]="your custom icon"
></cometchat-reaction-list>
</div>
Below is a customizations list along with corresponding code snippets
Property | Description | Code |
---|---|---|
loadingIconURL | used to set the custom loading icon | loadingIconURL="'your custom loading icon url'" |
errorIconURL | used to set the error icon | errorIconURL="'your custom error icon url'" |