Slow mode
Slow down messages to make them legible!
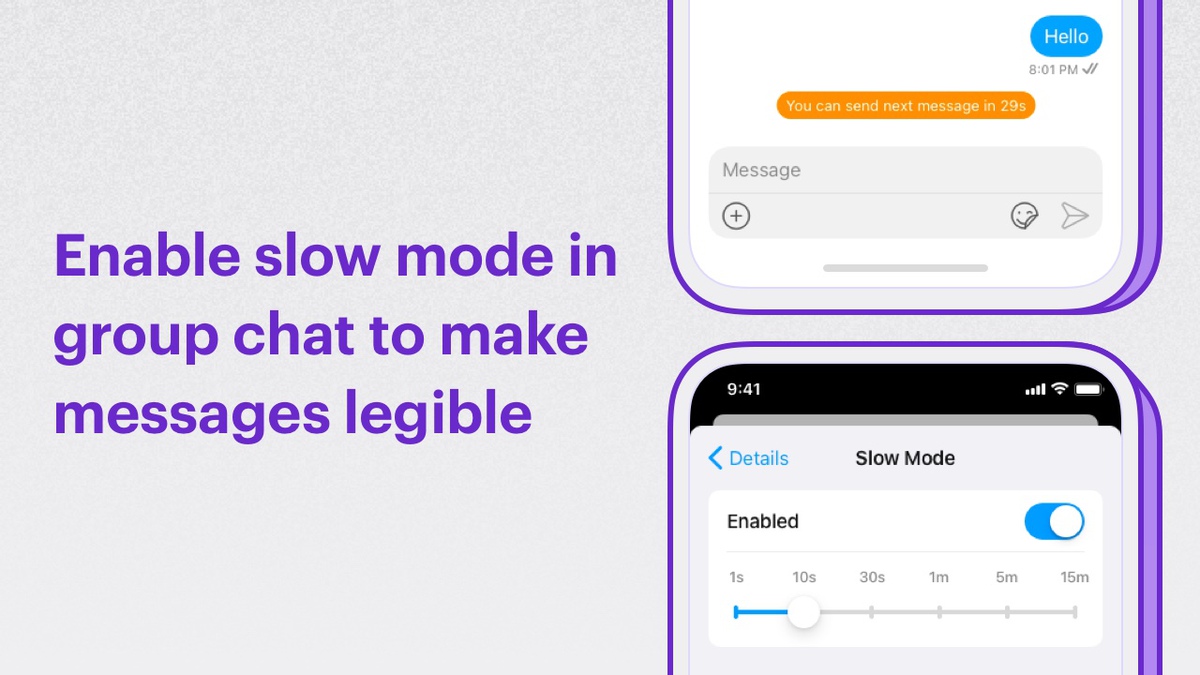
Extension settings
- Login to CometChat and select your app.
- Go to the Extensions section and enable the Slow mode extension.
How does Slow mode work?
Slow mode extension works great in groups with a large number of participants. Especially, during a live event with a potential for a flood of messages being sent every second. When the extension is enabled and enforced in a group, it allows the participants to send messages only after a certain intervals. This helps to keep chats readable for everyone during large events.
The extension has the following 4 parts:
- Enabling the slow mode for a group
- Disabling the slow mode for a group
- Enforcing slow mode for participants
- Fetching the slow mode details
Enabling slow mode
Slow mode can be enabled only by the group admins or moderators. Participants cannot enable the slow mode.
Once slow mode is enabled in a group, the information is shared with its members in real-time as a custom message. With this, it can be enforced immediately.
You need to implement the onCustomMessageReceived
listener in order to receive the Slow mode related messages. The message sent has the category of custom
and type extension_slow-mode
.
Following are the inputs required to enable slow mode in a particular group:
Parameter | Type | Description |
---|---|---|
guid | string | The group's ID in which the slow mode needs to be enabled. |
slowDownTimeInMS | int | The time in milliseconds for which the participants have to wait before being able to send the consecutive message. |
You can make use of the callExtension
method exposed by CometChat SDKs to enable slow mode as an admin/moderator.
- Javascript
- Java
- Swift
CometChat.callExtension('slow-mode', 'POST', 'v1/configure', {
"guid": "cometchat-guid-1",
"slowDownTimeInMS": 660000,
}).then(response => {
// Success true
})
.catch(error => {
// Error occured
});
import org.json.simple.JSONObject;
JSONObject body=new JSONObject();
body.put("guid", "cometchat-guid-1");
body.put("slowDownTimeInMS", 660000);
CometChat.callExtension("slow-mode", "POST", "/v1/configure", body,
new CometChat.CallbackListener < JSONObject > () {
@Override
public void onSuccess(JSONObject jsonObject) {
//On Success
}
@Override
public void onError(CometChatException e) {
//On Failure
}
});
CometChat.callExtension(slug: "slow-mode", type: .post, endPoint: "v1/configure", body: ["guid": "cometchat-guid-1" ,"slowDownTimeInMS": 660000] as [String : Any], onSuccess: { (response) in
// Success
}) { (error) in
// Error occured
}
Disabling slow mode
Slow mode can be disabled only by the group admins or moderators. Participants cannot disable the slow mode.
Once slow mode is disabled in a group, the information is shared with its members in real-time as a custom message. With this, it can be turned off for that group immediately.
Following are the inputs required to enable slow mode in a particular group:
Parameter | Type | Description |
---|---|---|
guid | string | The group's ID in which the slow mode needs to be disabled. |
You have to implement the onCustomMessageReceived
listener in order to receive the Slow mode related messages. The message sent has the category of custom
and type extension_slow-mode
.
You can make use of the callExtension
method exposed by CometChat SDKs to disable slow mode as an admin/moderator.
- Javascript
- Java
- Swift
CometChat.callExtension('slow-mode', 'DELETE', 'v1/configure', {
"guid": "cometchat-guid-1"
}).then(response => {
// Success true
})
.catch(error => {
// Error occured
});
import org.json.simple.JSONObject;
JSONObject body=new JSONObject();
body.put("guid", "cometchat-guid-1");
CometChat.callExtension("slow-mode", "DELETE", "/v1/configure", body,
new CometChat.CallbackListener < JSONObject > () {
@Override
public void onSuccess(JSONObject jsonObject) {
//On Success
}
@Override
public void onError(CometChatException e) {
//On Failure
}
});
CometChat.callExtension(slug: "slow-mode", type: .delete, endPoint: "v1/configure", body: nil, onSuccess: { (response) in
// Success
}) { (error) in
// Error occured
}
Enforcing slow mode
This is handled by the extension for groups that have slow mode enabled as mentioned below:
1. For participants of a group
If the moderator or admin of a group has enabled slow mode with the time of 1 min, then the participants will have to wait for 1 minute after sending a message. Once the participant has waited for 1 min, he/she then becomes eligible to send out the next message.
If there's an attempt by the participants to send a message before the interval has expired for them, the message gets blocked by the extension.
2. For admins and moderators
The extension does not restrict moderators and admins of a group. Slow mode is enforced only for the participants.
Groups that are created from the dashboard do not have an admin or moderator. Hence, care needs to be taken to add a member to such groups and change their scope to either moderator or admin.
3. Change in member scope
If a participant is made an admin or moderator of a group, the slow mode is no longer applicable for him/her.
Similarly, if an admin or moderator of a group is demoted to a participant, the slow mode will be applicable immediately to him/her as mentioned above.
Fetching slow mode details
As mentioned above, the details about the enabling or disabling the slow mode are shared in real-time with the members as a custom message. But, it might happen that a few members are offline or are added later on to the group. Hence, it is important to make the same details available to them for a consistent experience.
You can make use of the callExtension
method exposed by the CometChat SDKs to fetch the details about slow mode as a member of a group.
- Javascript
- Java
- Swift
const guid = "cometchat-guid-1";
CometChat.callExtension('slow-mode', 'GET', `v1/fetch-configuration?guid=${guid}`, null).then(response => {
// Configuration for the mentioned group
})
.catch(error => {
// Error occured
});
CometChat.callExtension("slow-mode", "GET", "/v1/fetch-configuration?guid=cometchat-guid-1", null,
new CometChat.CallbackListener < JSONObject > () {
@Override
public void onSuccess(JSONObject jsonObject) {
//On Success
}
@Override
public void onError(CometChatException e) {
//On Failure
}
});
CometChat.callExtension(slug: "slow-mode", type: .get, endPoint: "v1/fetch-configuration?guid=cometchat-guid-1", body: nil, onSuccess: { (response) in
// Success
}) { (error) in
// Error occured
}
The response has the following format:
Parameters | Type | Description |
---|---|---|
isSlowed | boolean | Whether the slow mode is enabled in the mentioned group.If false, the following fields are not present in the response. |
slowDownTimeInMS | int | The time interval for which a participant has to wait for sending messages. |
lastMessageSentAtTimestamp | timestamp | The timestamp at which the last message was sent by the logged in user in the mentioned group.If the scope of the logged in user is Admin or Moderator in the mentioned group, this field is not included in the response. |
Implementation
1. For admins and moderators
When a group chat is opened, and the scope of the logged-in user is either an admin or a moderator of that group, he/she should be able to toggle slow mode for that group.
When a group has multiple admins/moderators, and one of the admins enables (or disables) the slow mode for a group, the UI for other admins/moderators should update and show the control to disable (or enable) the slow mode. This can be achieved in real-time due to the custom message that is sent.
Whenever, an admin or a moderator in a group switches conversations to a different group, use the fetch-configuration call to check for the above mentioned details. It may happen, that the logged in user is admin/moderator for one group but a participant in another.
2. For participants of a group
When a group chat is opened, and the scope of the logged-in user is participant, he/she should be able to send messages only after the the configured intervals.
You can enforce the blocking behaviour by disabling the message composer or the send button on the UI. It will get enabled only once the participant has waited for the defined amount of slow-down time.
While the participant is waiting, a timer can be displayed to indicate the amount of time left after which they can send the next message.