Message Shortcuts
The Message Shortcuts extension enables your users to send each other predefined messages.
For example, !hb can be automatically expanded to Happy birthday!
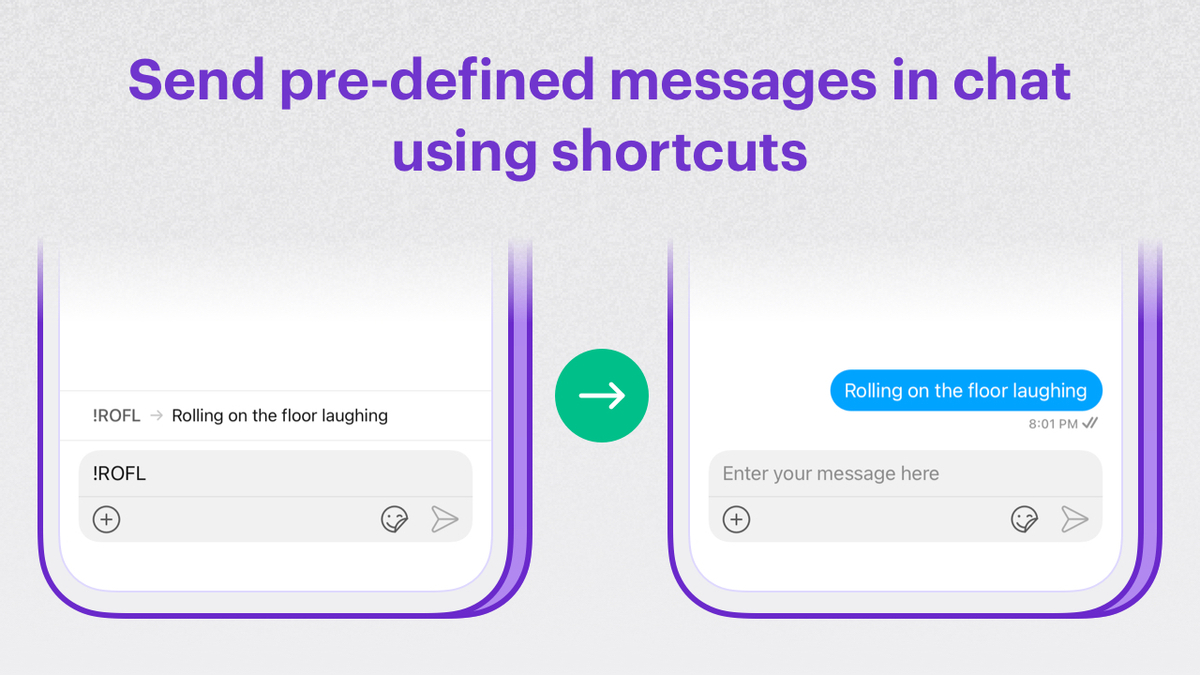
Extension settings
- Login to CometChat and select your app.
- Go to the Extensions section and enable the Message shortcuts extension.
- Open the settings for this extension.
- You should a list of Global Message shortcuts.
- Edit the existing shortcuts or add new ones.
- Save your settings.
How does it work?
The shortcuts saved in the Extension's settings are Global message shortcuts that can be accessed by all the users of your app. The shortcuts saved by users are accessible only to them along with the Global message shortcuts.
With the Message Shortcuts extension, you can:
- Fetch all shortcuts on your user's device.
- Allow users to edit, define or delete shortcuts.
- Send predefined message by typing shortcuts.
Implementation
1. Fetch all shortcuts
Once the user has successfully logged in, you can request the shortcuts from the extension. Additionally, you can provide a button to refresh the shortcuts.
Make use of the callExtension
method exposed by the CometChat SDK to fetch the shortcuts.
- Javascript
- Java
- Swift
CometChat.callExtension('message-shortcuts', 'GET', 'v1/fetch', null)
.then(shortcuts => {
// Save these shortcuts locally.
})
.catch(error => {
// Some error occured
});
CometChat.callExtension("message-shortcuts", "GET", "/v1/fetch", null,
new CometChat.CallbackListener<JSONObject>() {
@Override
public void onSuccess(JSONObject responseObject) {
// Shortcuts received here.
}
@Override
public void onError(CometChatException e) {
// Some error occured.
}
});
CometChat.callExtension(slug: "message-shortcuts", type: .get, endPoint: "v1/fetch", body: nil, onSuccess: { (response) in
print("Stickers",response)
}) { (error) in
print("Error",error?.errorCode, error?.errorDescription)
}
The response will have the following JSON structure:
- JSON
{
"shortcuts": {
"!hbd": "Happy Birthday! 🥳",
"!cu": "See you later.",
"!ty": "Hey! Thanks a lot! 😊",
"!wc": "You're welcome!"
}
}
2. Modify shortcuts
Shortcuts can be added, edited or deleted by your users. You need to have a section in your front-end application that allows the users to do so. The extension accepts a final list of shortcuts after all the modifications have been done by the user.
Make use of the callExtension
method exposed by the CometChat SDK to submit the final customized list.
- Javascript
- Java
- Swift
const finalList = {
shortcuts: {
"!hbd":"Happy birthday! Have fun!"
}
};
CometChat.callExtension('message-shortcuts', 'POST', 'v1/update', finalList)
.then(response => {
// Updated successfully.
})
.catch(error => {
// Some error occured
});
import org.json.simple.JSONObject;
JSONObject finalList = new JSONObject();
JSONObject shortcuts = new JSONObject();
shortcuts.put("!hbd", "Happy birthday! Have fun!");
shortcuts.put("!ttyl", "Talk to you later");
finalList.put("shortcuts", shortcuts);
CometChat.callExtension("message-shortcuts", "POST", "/v1/update", finalList,
new CometChat.CallbackListener < JSONObject > () {
@Override
public void onSuccess(JSONObject jsonObject) {
//On Success
}
@Override
public void onError(CometChatException e) {
//On Failure
}
});
let finalList = ["shortcuts": ["!hbd": "Happy Birthday!" ,
"!cu" : "See you later.",
"!ty", "Hey! Thanks a lot! :blush:",
"!wc", "You're welcome!"]]
CometChat.callExtension(slug: "message-shortcuts", type: .post, endPoint: "v1/update", body: finalList, onSuccess: { (response) in
// Updated successfully.
}) { (error) in
// Error occured
}
3. Use shortcuts
The UI implementation can be as follows:
- When
!
is typed in the message composer, a list pops up above the message composer with all the available shortcuts and their predefined values. - On typing the next letter after the
!
typed earlier, the list gets filtered with the shortcuts that start with that letter. - Clicking on any shortcut from the list, expands the corresponding predefined message in the message composer.