Getting Started with CometChat React UI Kit for Next.js
The CometChat UI Kit for React streamlines the integration of in-app chat functionality by providing a comprehensive set of prebuilt UI components. It offers seamless theming options, including light and dark modes, customizable fonts, colors, and extensive styling capabilities.
With built-in support for one-to-one and group conversations, developers can efficiently enable chat features within their applications. Follow this guide to quickly integrate chat functionality using the CometChat React UI Kit.
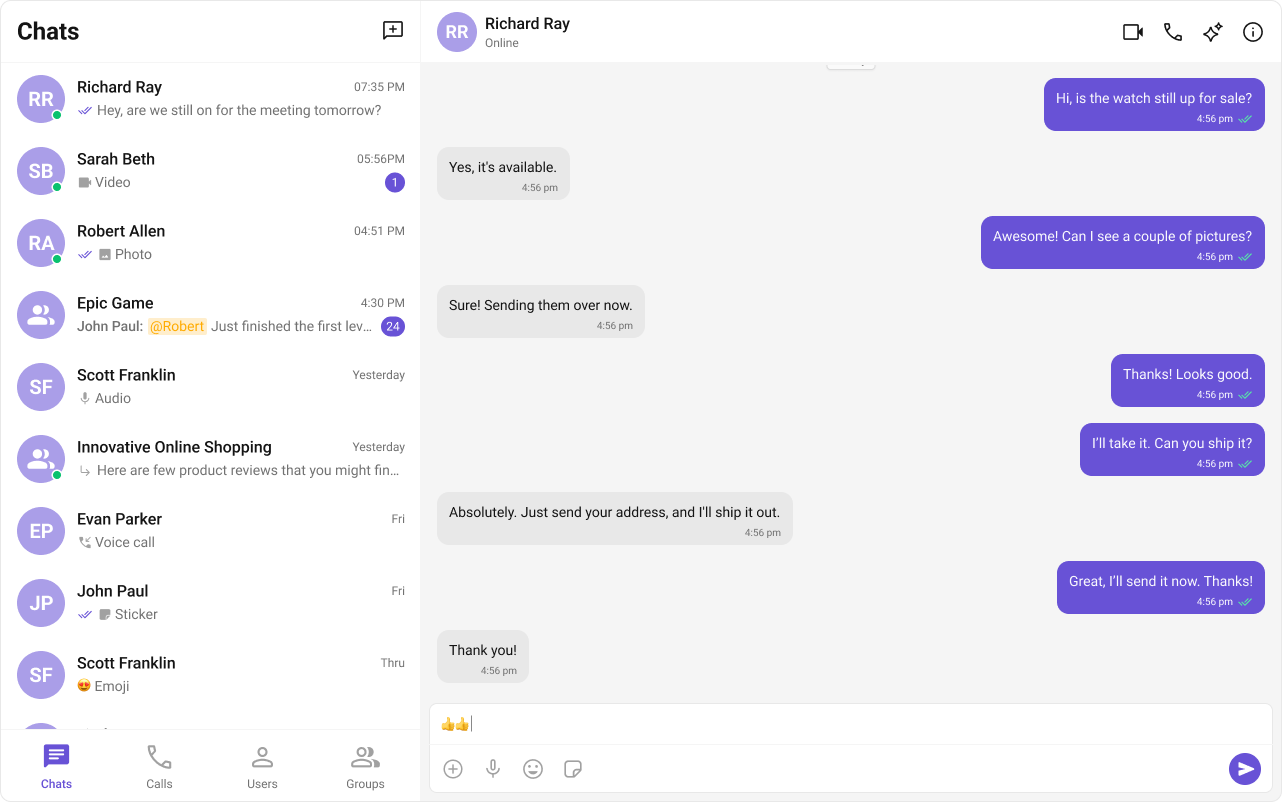
Prerequisites
Before installing the CometChat UI Kit for React, you must first create a CometChat application via the CometChat Dashboard. The dashboard provides all the essential chat service components, including:
- User Management
- Group Chat & Messaging
- Voice & Video Calling
- Real-time Notifications
To initialize the UI Kit, you will need the following credentials from your CometChat application:
- App ID
- Auth Key
- Region
Ensure you have these details ready before proceeding with the installation and configuration.
Register & Set Up CometChat
Follow these steps to register on CometChat and set up your development environment.
Step 1: Register on CometChat
To use CometChat UI Kit, you first need to register on the CometChat Dashboard.
Step 2: Get Your Application Keys
After registering, create a new app and retrieve your authentication details:
- Navigate to Application, then select the Credentials section.
- Note down the following keys:
- App ID
- Auth Key
- Region
Each CometChat application can be integrated with a single client app. Users within the same application can communicate across multiple platforms, including web and mobile.
Step 3: Set Up Your Development Environment
Ensure your system meets the following prerequisites before proceeding with integration.
System Requirements:
- Node.js installed on your machine.
- A code editor like Visual Studio Code or Cursor.
- npm or Yarn package manager installed.
Built With
The CometChat UI Kit for React relies on the following technologies:
Technology | Description | Installation Command |
---|---|---|
Node.js | JavaScript runtime environment | - |
npm | Node Package Manager | - |
React | JavaScript library for UI development | npm install react@18.x |
React DOM | React package for rendering UI | npm install react-dom@18.x |
Getting Started
Step 1: Create a Next.js Project
- Open your code editor (e.g., VS Code, Cursor).
- Initialize a new Next.js project using one of the following methods:
- npx
- vite
Using Create Next.js App (Recommended)
npx create-next-app@latest my-app --typescript
cd my-app
Using Vite (Faster alternative)
npm create vite@latest my-app --template next-ts
cd my-app
- Open the project directory in your code editor.
- Begin developing your Next.js application in the "app" directory..
- Install additional dependencies as needed.
Step 2: Install Dependencies
The CometChat UI Kit for React is an extension of the CometChat JavaScript SDK.
Installing it will automatically include the core Chat SDK, enabling seamless integration.
npm install @cometchat/chat-uikit-react
Step 3: Initialize CometChat UI Kit
Before using any features of the CometChat UI Kit or CometChat SDK, you must initialize the required settings.
- TypeScript
- JavaScript
import { CometChatUIKit, UIKitSettingsBuilder } from "@cometchat/chat-uikit-react";
/**
* CometChat Constants - Replace with your actual credentials
*/
const COMETCHAT_CONSTANTS = {
APP_ID: "APP_ID", // Replace with your actual App ID from CometChat
REGION: "REGION", // Replace with your App's Region
AUTH_KEY: "AUTH_KEY", // Replace with your Auth Key (leave blank if using Auth Token)
};
/**
* Configure the CometChat UI Kit using the UIKitSettingsBuilder.
* This setup determines how the chat UI behaves.
*/
const UIKitSettings = new UIKitSettingsBuilder()
.setAppId(COMETCHAT_CONSTANTS.APP_ID) // Assign the App ID
.setRegion(COMETCHAT_CONSTANTS.REGION) // Assign the App's Region
.setAuthKey(COMETCHAT_CONSTANTS.AUTH_KEY) // Assign the Authentication Key (if applicable)
.subscribePresenceForAllUsers() // Enable real-time presence updates for all users
.build(); // Build the final configuration
/**
* Initialize the CometChat UI Kit with the configured settings.
* Once initialized successfully, you can proceed with user authentication and chat features.
*/
CometChatUIKit.init(UIKitSettings)!
.then(() => {
console.log("CometChat UI Kit initialized successfully.");
// You can now call login function to authenticate users
})
.catch((error) => {
console.error("CometChat UI Kit initialization failed:", error); // Log errors if initialization fails
});
import { CometChatUIKit, UIKitSettingsBuilder } from "@cometchat/chat-uikit-react";
/**
* CometChat Constants - Replace with your actual credentials
*/
const COMETCHAT_CONSTANTS = {
APP_ID: "APP_ID", // Replace with your actual App ID from CometChat
REGION: "REGION", // Replace with your App's Region
AUTH_KEY: "AUTH_KEY", // Replace with your Auth Key (leave blank if using Auth Token)
};
/**
* Configure the CometChat UI Kit using the UIKitSettingsBuilder.
* This setup determines how the chat UI behaves.
*/
const UIKitSettings = new UIKitSettingsBuilder()
.setAppId(COMETCHAT_CONSTANTS.APP_ID) // Assign the App ID
.setRegion(COMETCHAT_CONSTANTS.REGION) // Assign the App's Region
.setAuthKey(COMETCHAT_CONSTANTS.AUTH_KEY) // Assign the Authentication Key (if applicable)
.subscribePresenceForAllUsers() // Enable real-time presence updates for all users
.build(); // Build the final configuration
/**
* Initialize the CometChat UI Kit with the configured settings.
* Once initialized successfully, you can proceed with user authentication and chat features.
*/
CometChatUIKit.init(UIKitSettings)
.then(() => {
console.log("CometChat UI Kit initialized successfully.");
// You can now call login function to authenticate users
})
.catch((error) => {
console.error("CometChat UI Kit initialization failed:", error); // Log errors if initialization fails
});
Ensure you replace the placeholders with your actual CometChat credentials.
Step 4: User Login
To authenticate a user, you need a UID
. You can either:
-
Create new users on the CometChat Dashboard, CometChat SDK Method or via the API.
-
Use pre-generated test users:
cometchat-uid-1
cometchat-uid-2
cometchat-uid-3
cometchat-uid-4
cometchat-uid-5
The Login method returns a User object containing all relevant details of the logged-in user.
Security Best Practices
- The Auth Key method is recommended for proof-of-concept (POC) development and early-stage testing.
- For production environments, it is strongly advised to use an Auth Token instead of an Auth Key to enhance security and prevent unauthorized access.
- TypeScript
- JavaScript
import { CometChatUIKit } from "@cometchat/chat-uikit-react";
const UID = "UID"; // Replace with your actual UID
CometChatUIKit.getLoggedinUser().then((user: CometChat.User | null) => {
if (!user) {
// If no user is logged in, proceed with login
CometChatUIKit.login(UID)
.then((user: CometChat.User) => {
console.log("Login Successful:", { user });
// Mount your app
})
.catch(console.log);
} else {
// If user is already logged in, mount your app
}
});
import { CometChatUIKit } from "@cometchat/chat-uikit-react";
const UID = "UID"; // Replace with your actual UID
CometChatUIKit.getLoggedinUser().then((user) => {
if (!user) {
// If no user is logged in, proceed with login
CometChatUIKit.login(UID)
.then((user) => {
console.log("Login Successful:", { user });
// Mount your app
})
.catch(console.log);
} else {
// If user is already logged in, mount your app
}
});
Step 5: Choose a Chat Experience
Integrate a conversation view that suits your application's UX requirements. Below are the available options:
1️⃣ Conversation List + Message View
Best for: Applications that need a two-panel layout, such as web-based chat interfaces (e.g., WhatsApp Web, Slack).
Features:
- Two-panel layout – Displays the conversation list on the left and the active chat window on the right.
- One-to-one & group conversations – Seamless switching between private and group chats.
- Multiple conversations – Effortlessly switch between different chat windows.
- Easy navigation – Intuitive UI for finding and accessing chats quickly.
- Tap-to-view on mobile – In mobile layouts, tapping a conversation opens the Message View, optimizing space.
- Real-time updates – Auto-refreshes messages and conversation lists.
- Message sync – Ensures messages stay updated across all sessions and devices.
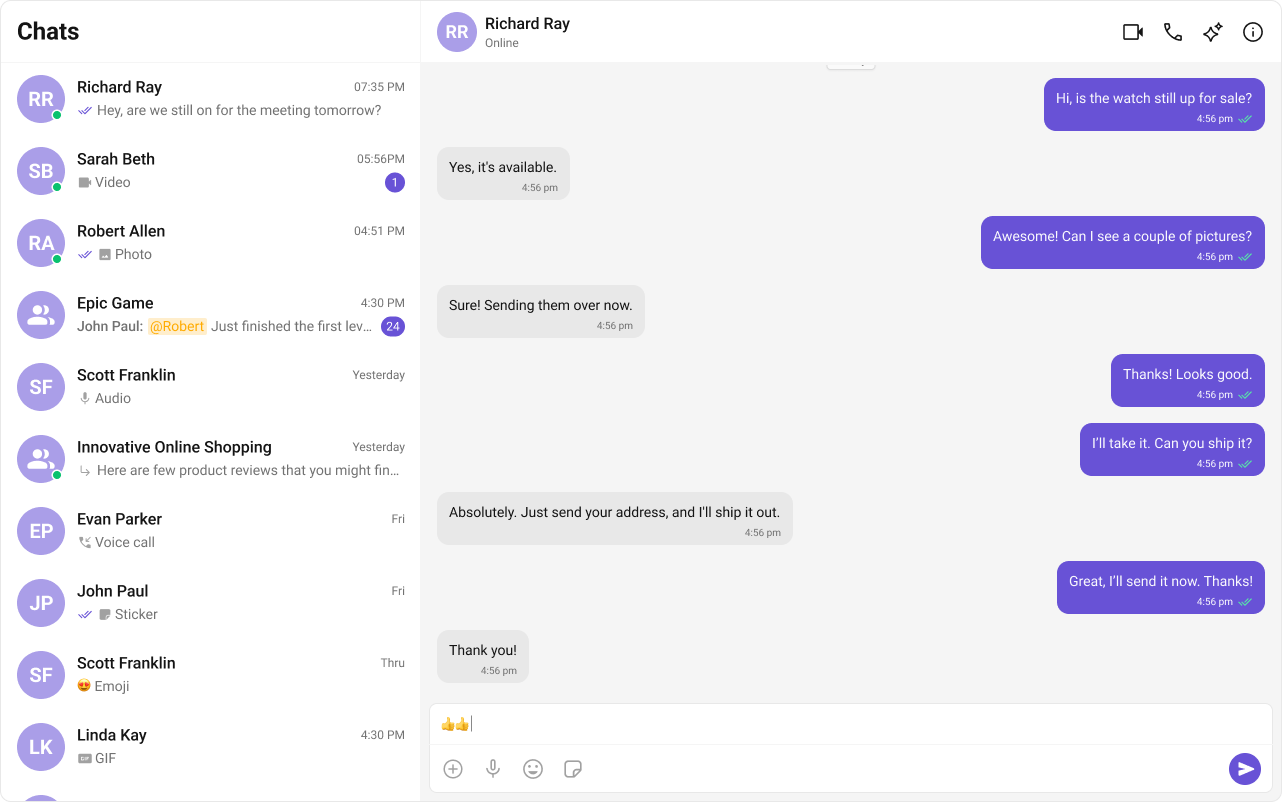
Recommended for:
- Desktop-first applications
- Apps requiring a rich user experience with seamless navigation
- Platforms supporting both individual and group messaging
- Mobile-friendly apps needing a tap-to-open message view
Integrate Conversation List + Message
2️⃣ One-to-One/Group Chat
Best for: Apps that require a focused, direct messaging experience without a sidebar.
Features:
- Dedicated chat window – Ideal for one-on-one or group messaging.
- No conversation list – Users directly enter the chat without navigating through a list.
- Supports both One-to-One and Group Chats – Easily configurable with minor code modifications.
- Optimized for mobile – Full-screen chat experience without distractions.
- Seamless real-time communication – Auto-updates messages for a smooth experience.
- Ideal for support chat or community-based messaging.

Recommended for:
- Support chat applications – Direct user-agent communication.
- Apps focusing on direct messaging – No distractions from other conversations.
- Community or group chat applications – A structured way to interact in groups.
- Mobile-first applications – Designed for compact and dedicated messaging experiences.
Integrate One-to-One/Group Chat
3️⃣ Tab-Based Chat Experience
Best for: Apps that need a structured, multi-feature navigation system for seamless interaction between chats, calls, users, and settings.
Features:
- Tab Navigation – Easily switch between Chat, Call Logs, Users, and Settings.
- Dedicated Chat Window – Full-screen messaging experience for focused communication.
- No Sidebar – Unlike multi-panel UI, this design prioritizes individual interactions.
- Unified Experience – Users can seamlessly manage conversations, call history, and settings from a single interface.
- Scalable for future features – Easily extend to include more functionalities such as notifications or contact management.
- Optimized for both desktop and mobile – Ensures a smooth experience across different screen sizes.
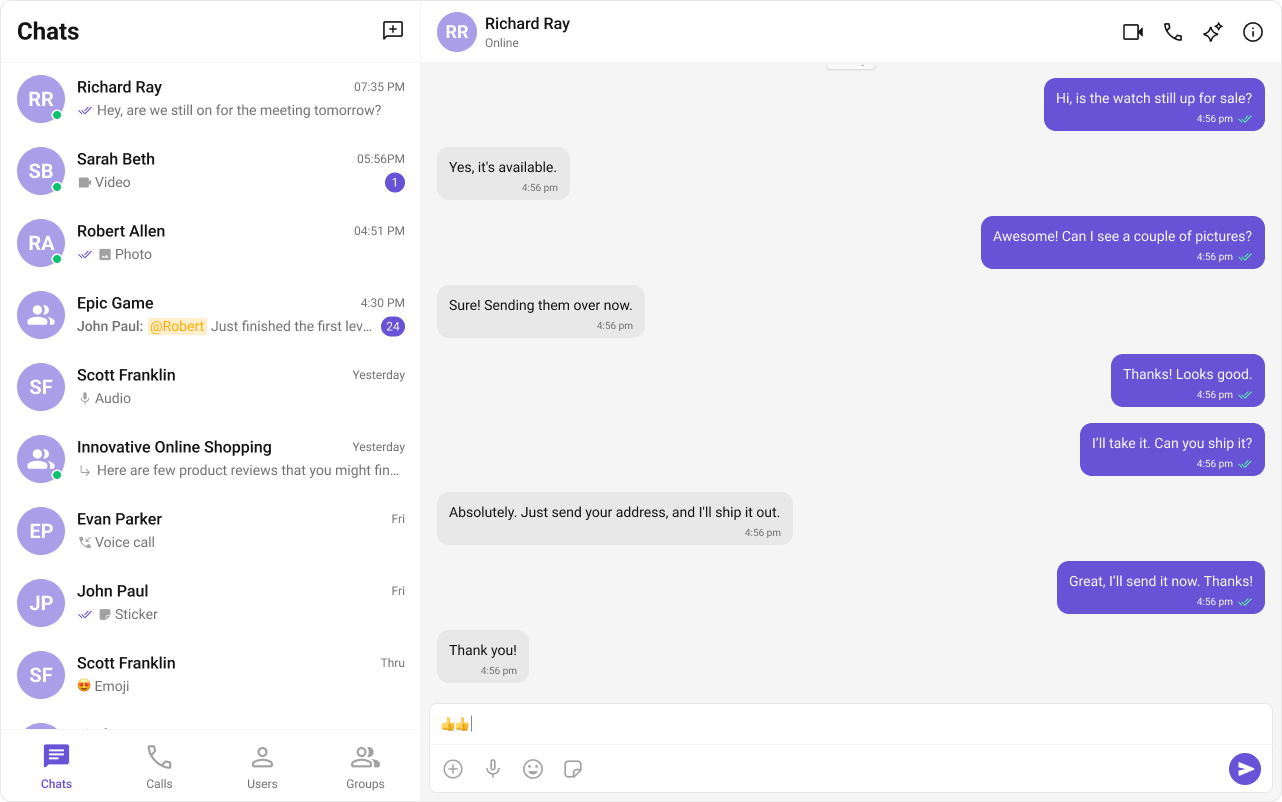
Recommended for:
- Apps requiring structured navigation – Clearly separate chat, calls, and settings.
- Multi-feature chat apps – Supporting different functionalities in an organized way.
- Mobile-first applications – Ideal for apps needing tab-based UI for easy access to features.
- Support & enterprise chat solutions – Perfect for help desks, business chat platforms, and customer support apps.
Build Your Own Chat Experience
Best for: Developers who need complete control over their chat interface, allowing customization of components, themes, and features to align with their app’s design and functionality. Whether you're enhancing an existing chat experience or building from scratch, this approach provides the flexibility to tailor every aspect to your needs.
Recommended for:
- Apps that require a fully customized chat experience.
- Developers who want to extend functionalities and modify UI components.
- Businesses integrating chat seamlessly into existing platforms.
Key Areas to Explore:
- React Sample App – Fully functional sample applications to accelerate your development.
- Core Features – Learn about messaging, real-time updates, and other essential capabilities.
- Components – Utilize prebuilt UI elements or customize them to fit your design.
- Themes – Adjust colors, fonts, and styles to match your branding.
- Build Your Own UI – Prefer a custom UI over our UI Kits? Explore our SDKs to create a tailored chat experience.
Next Steps
Now that you’ve selected your chat experience, proceed to the integration guide:
- Integrate Conversation List + Message
- Integrate One-to-One Chat
- Integrate Tab-Based Chat
- Advanced Customizations