Building a Conversation List + Message View
The Conversation List + Message View layout offers a seamless two-panel chat interface, commonly used in modern messaging applications like WhatsApp Web, Slack, and Microsoft Teams.
This design enables users to switch between conversations effortlessly while keeping the chat window open, ensuring a smooth, real-time messaging experience.
User Interface Overview
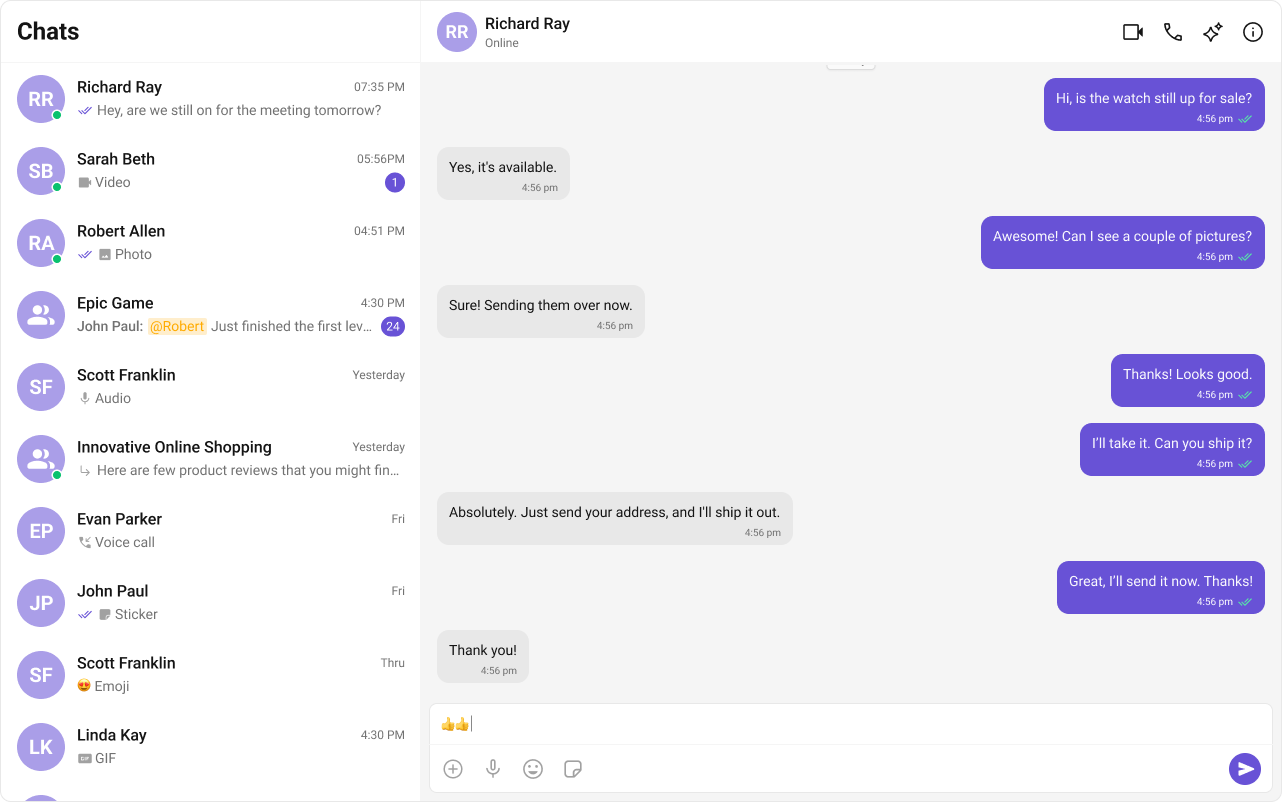
This layout is structured into three key sections:
- Sidebar (Conversation List) – Displays active conversations, including users and groups.
- Message View – Shows chat messages for the selected conversation in real-time.
- Message Composer – Provides an input field for typing and sending messages, along with support for media, emojis, and reactions.
Step-by-Step Guide
Step 1: Create Sidebar
Let's create the Sidebar
component which will render different conversations.
Folder Structure
Create a CometChatSelector
folder inside your src/app
directory and add the following files:
src/app/
│── CometChatSelector/
│ ├── CometChatSelector.tsx
│ ├── CometChatSelector.css
Download the Icon
These icons are available in the CometChat UI Kit assets folder. You can find them at:
🔗 GitHub Assets Folder
- TypeScript
- CSS
import { useEffect, useState } from "react";
import { Conversation, Group, User } from "@cometchat/chat-sdk-javascript";
import { CometChatConversations, CometChatUIKitLoginListener } from "@cometchat/chat-uikit-react";
import { CometChat } from '@cometchat/chat-sdk-javascript';
import "./CometChatSelector.css";
// Define props interface for component
interface SelectorProps {
onSelectorItemClicked?: (input: User | Group | Conversation, type: string) => void;
}
// CometChatSelector component definition
export const CometChatSelector = (props: SelectorProps) => {
const {
onSelectorItemClicked = () => { }, // Default function if no prop is provided
} = props;
// State to store the currently logged-in user
const [loggedInUser, setLoggedInUser] = useState<CometChat.User | null>();
// State to track the currently selected item (conversation, user, group, or call)
const [activeItem, setActiveItem] = useState<
CometChat.Conversation | CometChat.User | CometChat.Group | CometChat.Call | undefined
>();
useEffect(() => {
// Retrieve the logged-in user from CometChat's login listener
let loggedInUser = CometChatUIKitLoginListener.getLoggedInUser();
setLoggedInUser(loggedInUser);
}, [CometChatUIKitLoginListener?.getLoggedInUser()]); // Dependency array to trigger effect when user changes
return (
<>
{/* Render CometChatConversations only if a user is logged in */}
{loggedInUser && (
<>
<CometChatConversations
activeConversation={activeItem instanceof CometChat.Conversation ? activeItem : undefined}
onItemClick={(e) => {
setActiveItem(e); // Update the selected item state
onSelectorItemClicked(e, "updateSelectedItem"); // Trigger callback with selected item
}}
/>
</>
)}
</>
);
};
/* Style for the icon in the header menu of the conversation list */
.selector-wrapper .cometchat-conversations .cometchat-list__header-menu .cometchat-button__icon {
background: var(--cometchat-icon-color-primary);
}
/* Change background color of icon on hover */
.cometchat-conversations .cometchat-list__header-menu .cometchat-button__icon:hover {
background: var(--cometchat-icon-color-highlight);
}
/* Remove the right border from the search bar */
.cometchat-list__header-search-bar {
border-right: none;
}
/* Align submenu items to the left */
.cometchat .cometchat-menu-list__sub-menu-list-item {
text-align: left;
}
/* Set specific width and positioning for submenu list */
.cometchat .cometchat-conversations .cometchat-menu-list__sub-menu-list {
width: 212px;
top: 40px !important;
left: 172px !important;
}
/* Style the logged-in user section with a bottom border */
#logged-in-user {
border-bottom: 2px solid var(--cometchat-border-color-default, #E8E8E8);
}
/* Prevent cursor interaction on logged-in user menu items */
#logged-in-user .cometchat-menu-list__sub-menu-item-title,
#logged-in-user .cometchat-menu-list__sub-menu-list-item {
cursor: default;
}
/* Style for logout icon with error color */
.cometchat-menu-list__sub-menu-list-item-icon-log-out {
background-color: var(--cometchat-error-color, #F44649);
}
/* Style for logout text with error color */
.cometchat-menu-list__sub-menu-item-title-log-out {
color: var(--cometchat-error-color, #F44649);
}
/* Allow pointer interaction on submenu items inside chat menu */
.chat-menu .cometchat .cometchat-menu-list__sub-menu-item-title {
cursor: pointer;
}
/* Remove shadow from submenu inside chat menu */
.chat-menu .cometchat .cometchat-menu-list__sub-menu {
box-shadow: none;
}
/* Style for submenu icons inside chat menu */
.chat-menu .cometchat .cometchat-menu-list__sub-menu-icon {
background-color: var(--cometchat-icon-color-primary, #141414);
width: 24px;
height: 24px;
}
Step 2: Render Experience
Now we will create the CometChatNoSSR.tsx
& CometChatNoSSR.css
files.
Here, we will initialize the CometChat UI Kit, log in a user, and build the messaging experience using CometChatMessageHeader
, CometChatMessageList
, and CometChatMessageComposer
components.
src/app/
│── CometChatNoSSR/
│ ├── CometChatNoSSR.tsx
│ ├── CometChatNoSSR.css
- TypeScript
- CSS
import React, { useEffect, useState } from "react";
import {
CometChatMessageComposer,
CometChatMessageHeader,
CometChatMessageList,
CometChatUIKit,
UIKitSettingsBuilder
} from "@cometchat/chat-uikit-react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatSelector } from "../CometChatSelector/CometChatSelector";
import "./CometChatNoSSR.css";
// Constants for CometChat configuration
const COMETCHAT_CONSTANTS = {
APP_ID: "",
REGION: "",
AUTH_KEY: "",
};
// Functional Component
const CometChatNoSSR: React.FC = () => {
// State to store the logged-in user
const [user, setUser] = useState<CometChat.User | undefined>(undefined);
// State to store selected user or group
const [selectedUser, setSelectedUser] = useState<CometChat.User | undefined>(undefined);
const [selectedGroup, setSelectedGroup] = useState<CometChat.Group | undefined>(undefined);
useEffect(() => {
// Initialize UIKit settings
const UIKitSettings = new UIKitSettingsBuilder()
.setAppId(COMETCHAT_CONSTANTS.APP_ID)
.setRegion(COMETCHAT_CONSTANTS.REGION)
.setAuthKey(COMETCHAT_CONSTANTS.AUTH_KEY)
.subscribePresenceForAllUsers()
.build();
// Initialize CometChat UIKit
CometChatUIKit.init(UIKitSettings)
?.then(() => {
console.log("Initialization completed successfully");
// Check if user is already logged in
CometChatUIKit.getLoggedinUser().then((loggedInUser) => {
if (!loggedInUser) {
// Perform login if no user is logged in
CometChatUIKit.login("cometchat-uid-1")
.then((user) => {
console.log("Login Successful", { user });
setUser(user);
})
.catch((error) => console.error("Login failed", error));
} else {
console.log("Already logged-in", { loggedInUser });
setUser(loggedInUser);
}
});
})
.catch((error) => console.error("Initialization failed", error));
}, []);
return user ? (
<div className="conversations-with-messages">
{/* Sidebar with conversation list */}
<div className="conversations-wrapper">
<CometChatSelector
onSelectorItemClicked={(activeItem) => {
let item = activeItem;
// Extract the conversation participant
if (activeItem instanceof CometChat.Conversation) {
item = activeItem.getConversationWith();
}
// Update states based on the type of selected item
if (item instanceof CometChat.User) {
setSelectedUser(item as CometChat.User);
setSelectedGroup(undefined);
} else if (item instanceof CometChat.Group) {
setSelectedUser(undefined);
setSelectedGroup(item as CometChat.Group);
} else {
setSelectedUser(undefined);
setSelectedGroup(undefined);
}
}}
/>
</div>
{/* Message view section */}
{selectedUser || selectedGroup ? (
<div className="messages-wrapper">
<CometChatMessageHeader user={selectedUser} group={selectedGroup} />
<CometChatMessageList user={selectedUser} group={selectedGroup} />
<CometChatMessageComposer user={selectedUser} group={selectedGroup} />
</div>
) : (
<div className="empty-conversation">Select Conversation to start</div>
)}
</div>
) : undefined;
};
export default CometChatNoSSR;
/* Layout for the main conversations and messages container */
.conversations-with-messages {
display: flex;
height: 100%;
width: 100%;
}
/* Sidebar wrapper for conversations */
.conversations-wrapper {
height: 100%;
width: 480px; /* Fixed width for conversation list */
overflow: hidden;
display: flex;
flex-direction: column;
height: inherit;
}
/* Hide overflow for the conversation list */
.conversations-wrapper > .cometchat {
overflow: hidden;
}
/* Message section layout */
.messages-wrapper {
width: calc(100% - 480px); /* Take remaining space */
height: 100%;
display: flex;
flex-direction: column;
}
/* Display styling for when no conversation is selected */
.empty-conversation {
height: 100%;
width: 100%;
display: flex;
justify-content: center;
align-items: center;
background: white;
color: var(--cometchat-text-color-secondary, #727272);
font: var(--cometchat-font-body-regular, 400 14px Roboto);
}
/* Ensure message composer does not have rounded corners */
.cometchat .cometchat-message-composer {
border-radius: 0px;
}
Step 3: Disabling SSR for CometChatNoSSR.tsx
in Next.js
In this update, we will disable Server-Side Rendering (SSR) for CometChatNoSSR.tsx
while keeping the rest of the application’s SSR functionality intact. This ensures that the CometChat UI Kit components load only on the client-side, preventing SSR-related issues.
Disabling SSR in index.tsx
Modify your index.tsx
file to dynamically import the CometChatNoSSR.tsx
component with { ssr: false }
.
import { Inter } from "next/font/google";
import dynamic from "next/dynamic";
const inter = Inter({ subsets: ["latin"] });
// Dynamically import CometChat component with SSR disabled
const CometChatComponent = dynamic(() => import("./CometChatNoSSR"), {
ssr: false,
});
export default function Home() {
return <CometChatComponent />;
}
Why disable SSR?
- The CometChat UI Kit depends on browser APIs (window, document, WebSockets).
- Next.js pre-renders components on the server, which can cause errors with browser-specific features.
- By setting
{ ssr: false }
, we ensure that CometChatNoSSR.tsx only loads on the client.
Step 4: Import CometChat UI Kit CSS
Next, add the following import statement to global.css to ensure CometChat UI Kit styles are included.
@import url("../../node_modules/@cometchat/chat-uikit-react/dist/styles/css-variables.css");
Step 5: Run the project
npm start
Next Steps
Enhance the User Experience
- Advanced Customizations – Personalize the chat UI to align with your brand.