Groups
Overview
CometChatGroups
functions as a standalone Component, showcasing an accessible list of all available groups. It provides an integral search functionality, allowing you to locate any specific groups swiftly and easily. For each group listed, the group name is displayed by default, in conjunction with the group icon when available. Additionally, it provides a useful feature by displaying the number of members in each group as a subtitle, offering valuable context about group size and activity level.
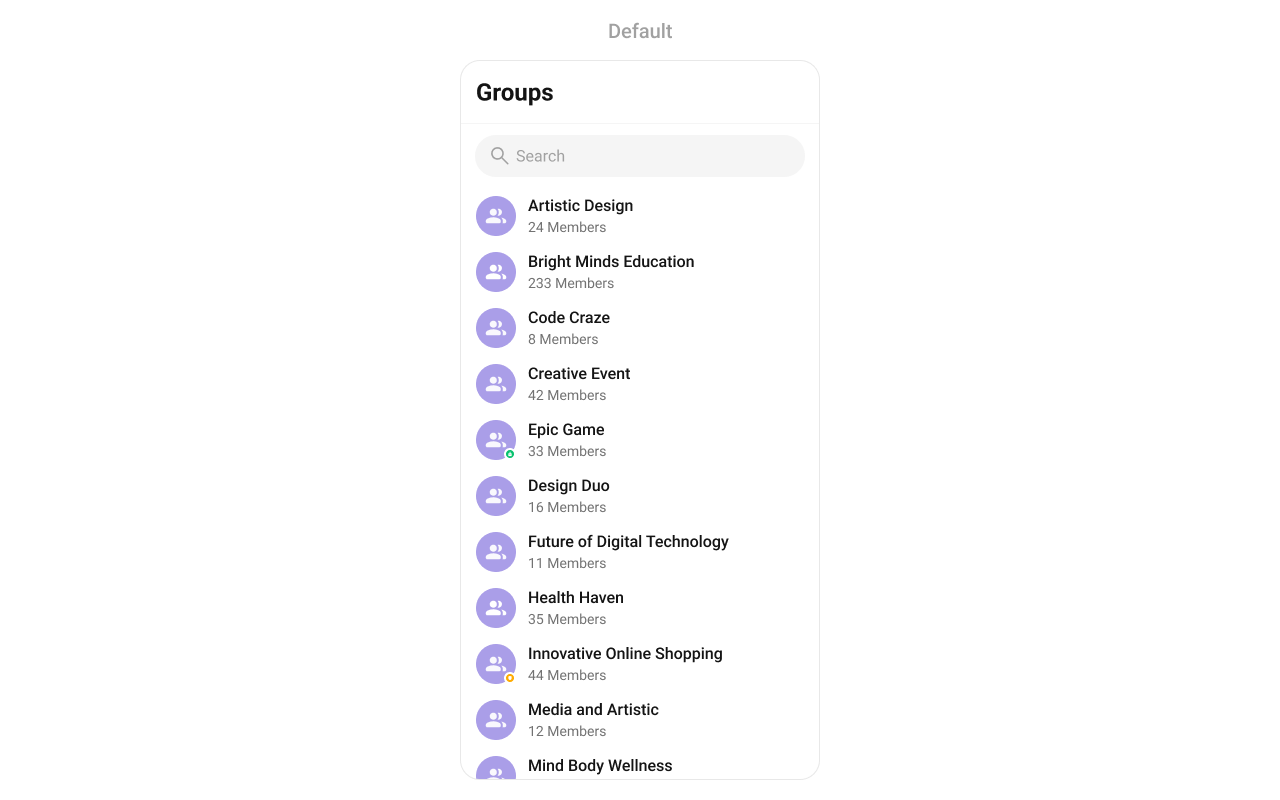
Usage
Integration
The following code snippet illustrates how you can can launch CometChatGroups
.
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
return <CometChatGroups />;
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and Group-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
onItemPress
Function invoked when a Group item is clicked, typically used to open a Group profile or chat screen.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
const onPressHandler = (user: CometChat.Group) => {
//code
};
return <CometChatGroups onItemPress={onPressHandler} />;
onItemLongPress
Function executed when a Group item is long-pressed, allowing additional actions like delete or block.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
const onLongPressHandler = (user: CometChat.Group) => {
//code
};
return <CometChatGroups onItemLongPress={onLongPressHandler} />;
onBack
onBack
is triggered when you press the back button in the app bar. It has a predefined behavior; when clicked, it navigates to the previous activity. However, you can override this action using the following code snippet.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
const onBackHandler = () => {
//code
};
return <CometChatGroups hideBackButton={false} onBack={onBackHandler} />;
onSelection
Called when a item from the fetched list is selected, useful for multi-selection features.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
const onSelectionHandler = (selection: Array<CometChat.Group>) => {
//code
};
return (
<CometChatGroups selectionMode={"single"} onSelection={onSelectionHandler} />
);
onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the groups component.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
const onErrorHandler = (error: CometChat.CometChatException) => {
console.log("Error");
};
return <CometChatGroups onError={onErrorHandler} />;
Filters
Filters allow you to customize the data displayed in a list within a Component. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders of Chat SDK.
1. GroupsRequestBuilder
The GroupsRequestBuilder enables you to filter and customize the group list based on available parameters in GroupsRequestBuilder. This feature allows you to create more specific and targeted queries when fetching groups. The following are the parameters available in GroupsRequestBuilder
Methods | Type | Description |
---|---|---|
setLimit | number | sets the number groups that can be fetched in a single request, suitable for pagination |
setSearchKeyword | string | used for fetching groups matching the passed string |
joinedOnly | boolean | to fetch only joined groups |
friendsOnly | boolean | used for fetching only those users in which logged in user is a member |
setTags | Array<string> | used for fetching groups containing the passed tags |
withTags | boolean | used to fetch tags data along with the list of groups |
Example
In the example below, we are applying a filter to the Group List based on only joined groups and setting the limit to ten.
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const groupsRequestBuilder = new CometChat.GroupsRequestBuilder()
.setLimit(10)
.joinedOnly(true);
return (
<CometChatGroups
selectionMode="none"
groupsRequestBuilder={groupsRequestBuilder}
/>
);
}
2. SearchRequestBuilder
The SearchRequestBuilder uses GroupsRequestBuilder enables you to filter and customize the search list based on available parameters in GroupsRequestBuilder.
Example
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-react-native";
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
function App(): React.JSX.Element {
const searchRequestBuilder = new CometChat.GroupsRequestBuilder()
.setLimit(10)
.setSearchKeyword("custom-keyword");
return (
<CometChatGroups
selectionMode="none"
searchRequestBuilder={searchRequestBuilder}
/>
);
}
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The list of events emitted by the Groups component is as follows.
Events | Description |
---|---|
ccGroupCreated | This will get triggered when the logged in user creates a group |
ccGroupDeleted | This will get triggered when the logged in user deletes a group |
ccGroupLeft | This will get triggered when the logged in user leaves a group |
ccGroupMemberScopeChanged | This will get triggered when the logged in user changes the scope of another group member |
ccGroupMemberBanned | This will get triggered when the logged in user bans a group member from the group |
ccGroupMemberKicked | This will get triggered when the logged in user kicks another group member from the group |
ccGroupMemberUnbanned | This will get triggered when the logged in user unbans a user banned from the group |
ccGroupMemberJoined | This will get triggered when the logged in user joins a group |
ccGroupMemberAdded | This will get triggered when the logged in user add new members to the group |
ccOwnershipChanged | This will get triggered when the logged in user transfer the ownership of their group to some other member |
1. Add CometChatGroupEvents
Listener's
- Adding Listeners
import { CometChatUIEventHandler } from "@cometchat/chat-uikit-react-native";
CometChatUIEventHandler.addGroupListener("GROUP_LISTENER_ID", {
ccGroupLeft: ({ leftGroup }) => {
//code
},
});
CometChatUIEventHandler.addGroupListener("GROUP_LISTENER_ID", {
ccGroupDeleted: ({ group }) => {
//code
},
});
2. Removing CometChatGroupEvents
Listener's
- Removing Listeners
import { CometChatUIEventHandler } from "@cometchat/chat-uikit-react-native";
CometChatUIEventHandler.removeGroupListener("GROUP_LISTENER_ID");
Customization
To fit your app's design requirements, you can customize the appearance of the groups component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
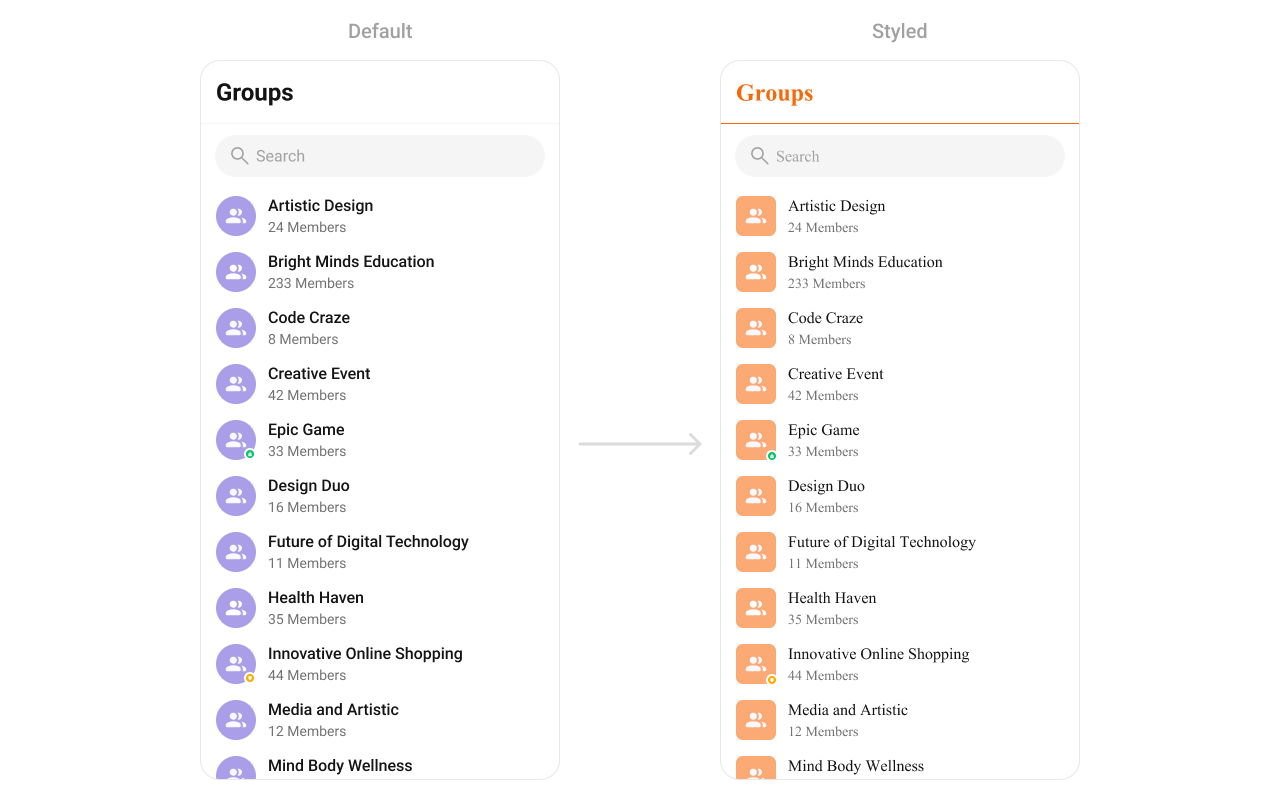
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
return (
<CometChatGroups
style={{
titleStyle: {
color: "#FBAA75",
},
titleSeparatorStyle: {
borderBottomColor: "#FBAA75",
},
itemStyle: {
avatarStyle: {
containerStyle: {
borderRadius: 8,
backgroundColor: "#FBAA75",
},
imageStyle: {
borderRadius: 8,
},
},
},
}}
></CometChatGroups>
);
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
hideHeader | Used to toggle visibility for the toolbar/header | hideHeader?: boolean |
hideBackButton | Used to toggle visibility for back button | hideBackButton?: boolean |
hideSearch | Used to toggle visibility for search box | hideSearch?: boolean |
hideError | Used to hide error on fetching users | hideError?: boolean |
selectionMode | This method determines the selection mode for groups, enabling users to select either a single user or multiple groups at once. | selectionMode={SelectionMode.multiple} |
hideSubmitButton | Used to toggle the visibility of the submit button when selectionMode is enabled. | hideBackButton={true} |
searchPlaceholderText | Used to set search placeholder text | searchPlaceholderText?: string; |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
LoadingView
Configures a custom loading view displayed while groups are being fetched.
Use Cases:
- Show a spinner with "Loading groups..." text.
- Display a skeleton loader for a smooth UI experience.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
const getLoadingView = (): JSX.Element => {
//your custom loading view
//return jsx;
};
return <CometChatGroups LoadingView={getLoadingView}></CometChatGroups>;
EmptyView
Defines a view that appears when no groups are available.
Use Cases:
- Show a message like "No groups found, create one now!".
- Display an illustration with a "Create New Group" button.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
const getEmptyView = (): JSX.Element => {
//your custom loading view
//return jsx;
};
return <CometChatGroups EmptyView={getEmptyView}></CometChatGroups>;
ErrorView
Configures the UI when an error occurs while fetching groups.
Use Cases:
- Display a retry button with "Failed to load groups, try again.".
- Show a friendly error illustration.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
const getErrorView = (): JSX.Element => {
//your custom loading view
//return jsx;
};
return <CometChatGroups ErrorView={getErrorView}></CometChatGroups>;
LeadingView
Sets a custom leading view that appears at the start of each group item.
Use Cases:
- Display the group profile picture.
- Add an icon indicating Public or Private groups.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
const getLeadingView = (item: CometChat.Group): JSX.Element => {
//your custom loading view
//return jsx;
};
return <CometChatGroups LeadingView={getLeadingView}></CometChatGroups>;
TitleView
Customizes the title view of each group item, which typically displays the group name.
Use Cases:
- Style group names with custom fonts and colors.
- Show a verified badge for official groups.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
const getTitleView = (item: CometChat.Group): JSX.Element => {
//your custom loading view
//return jsx;
};
return <CometChatGroups TitleView={getTitleView}></CometChatGroups>;
TrailingView
Allows custom elements to be added at the end of each group item, such as buttons or indicators.
Use Cases:
- Show unread message counts.
- Add a quick Join or Leave button.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
const getTrailingView = (item: CometChat.Group): JSX.Element => {
//your custom loading view
//return jsx;
};
return <CometChatGroups TrailingView={getTrailingView}></CometChatGroups>;
ItemView
Assigns a fully custom ListItem layout to the Groups component, replacing the default design.
Use Cases:
- Add a description below the group name.
- Customize layout to include additional metadata.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
const getItemView = (item: CometChat.Group): JSX.Element => {
//your custom loading view
//return jsx;
};
return <CometChatGroups ItemView={getItemView}></CometChatGroups>;
Example
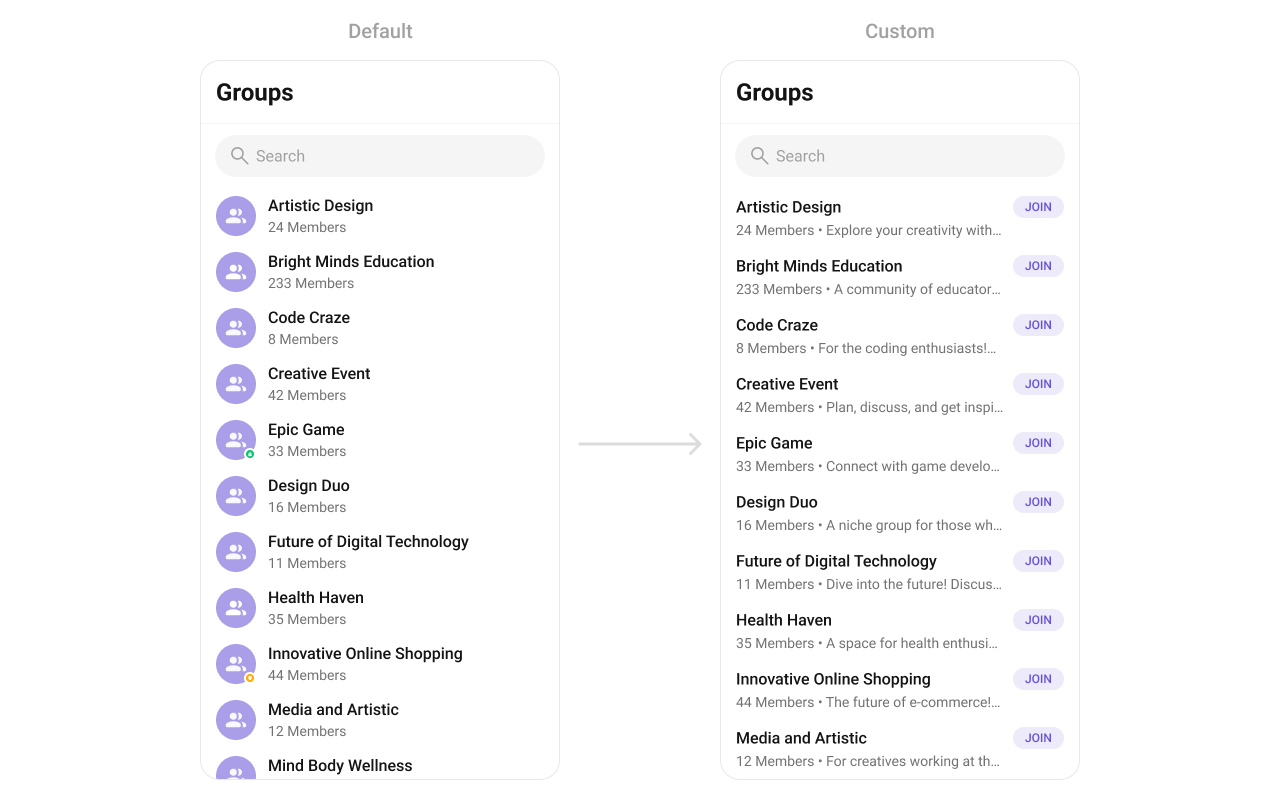
SubTitleView
Customizes the subtitle view for each group item, which typically displays extra information.
Use Cases:
- Show last message preview.
- Display the number of members.
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
const getSubtitleView = (item: CometChat.Group): JSX.Element => {
//your custom loading view
//return jsx;
};
return <CometChatGroups SubtitleView={getSubtitleView}></CometChatGroups>;
Example
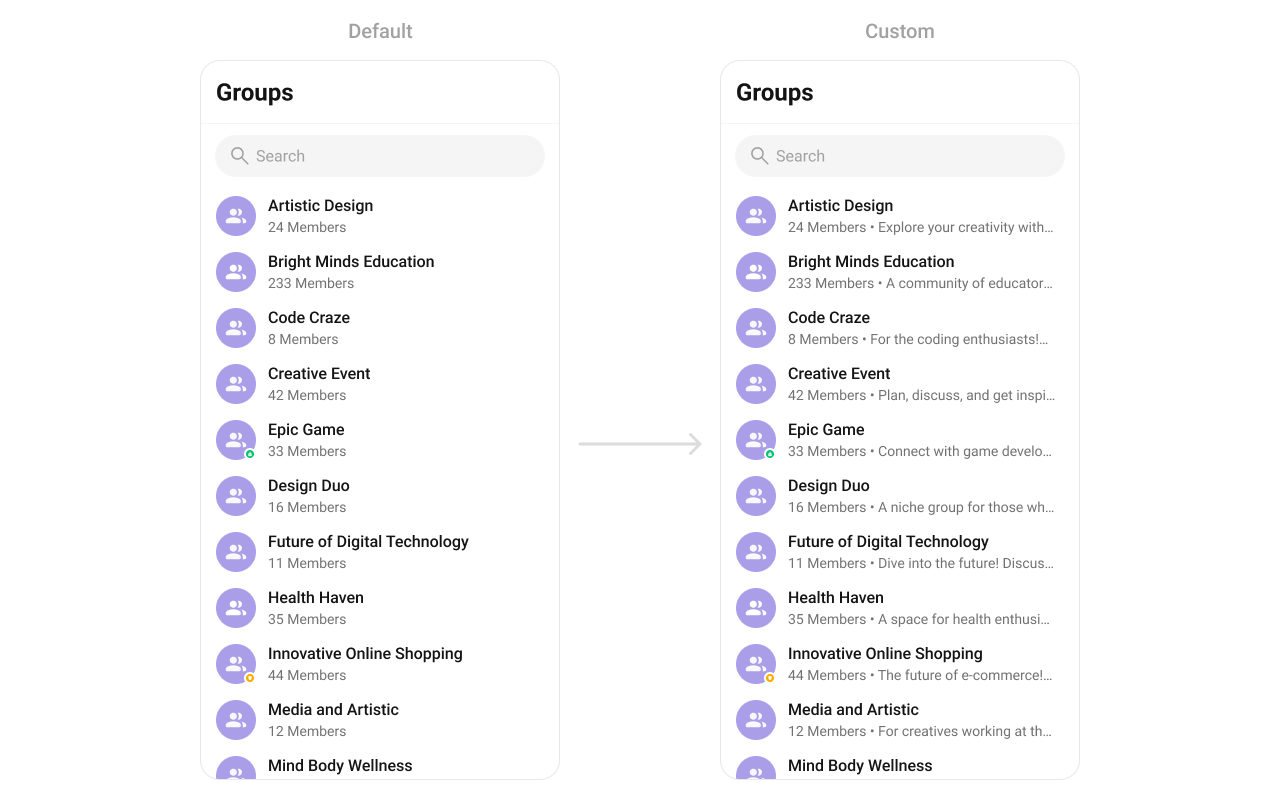
AppBarOptions
Customizes the overflow menu (three-dot ⋮ icon) with additional options.
Use Cases:
- Add options like "Invite Members", "Report Group".
- Enable admin-specific options like "Manage Group Settings".
- App.tsx
import { CometChatGroups } from "@cometchat/chat-uikit-react-native";
//code
return (
<CometChatGroups
AppBarOptions={() => {
return (
<TouchableOpacity
onPress={() => {
//do something
}}
>
<Text>Custom App Bar Options</Text>
</TouchableOpacity>
);
}}
></CometChatGroups>
);
Example
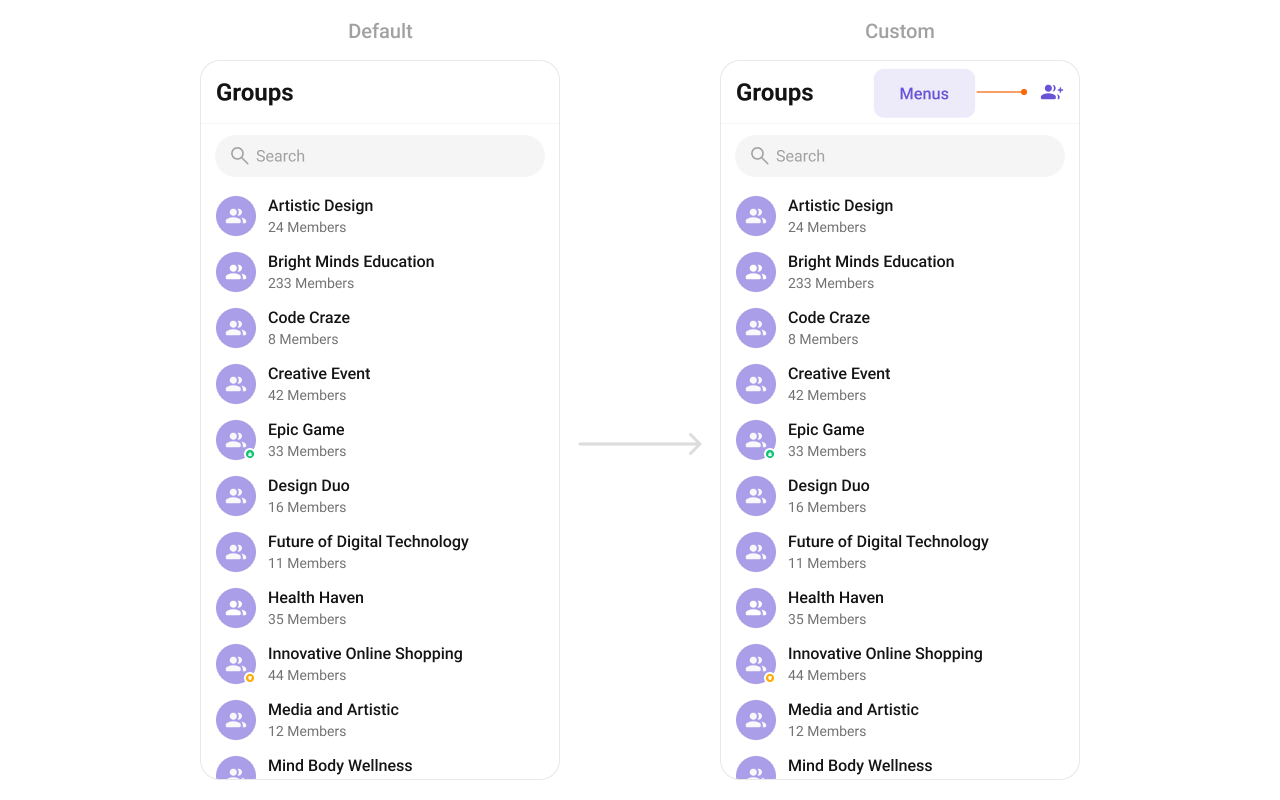