Message List
Overview
MessageList
is a Composite Component that displays a list of messages and effectively manages real-time operations. It includes various types of messages such as Text Messages, Media Messages, Stickers, and more.
MessageList
is primarily a list of the base component MessageBubble. The MessageBubble Component is utilized to create different types of chat bubbles depending on the message type.
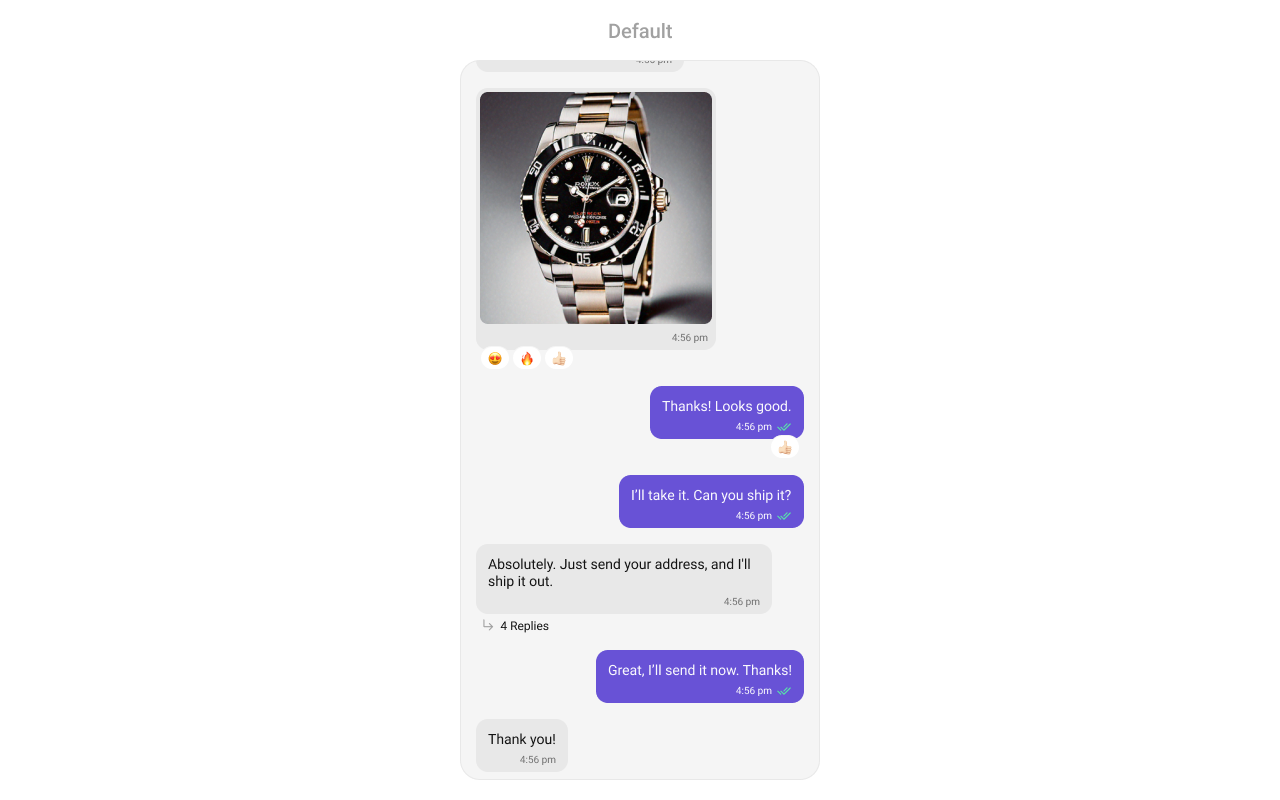
Usage
Integration
The following code snippet illustrates how you can directly incorporate the CometChatMessageList
component into your app.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
//code
return <CometChatMessageList group={group}></CometChatMessageList>;
Simply adding the CometChatMessageList
component to the layout will result in an error. To fetch messages, you need to supplement it with user
or group
prop.
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onThreadRepliesPress
onThreadRepliesPress
is triggered when you press on the threaded message bubble.
The onThreadRepliesPress
action doesn't have a predefined behavior. You can override this action using the following code snippet.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const threadRepliesCallback = (
msg: CometChat.BaseMessage,
messageBubbleView: () => JSX.Element | null
) => {
//handle thread
};
return (
<CometChatMessageList
group={group}
onThreadRepliesPress={threadRepliesCallback}
></CometChatMessageList>
);
2. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the MessageList component.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const errorHandler = (e: CometChat.CometChatException) => {
//handle error
};
return (
<CometChatMessageList
group={group}
onError={errorHandler}
></CometChatMessageList>
);
onReactionLongPress
Function triggered when a user long presses on a reaction pill, allowing developers to override the default behavior.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const onReactionLongPress = (
reaction: CometChat.ReactionCount,
messageObject: CometChat.BaseMessage
) => {};
return (
<CometChatMessageList
group={group}
onReactionLongPress={onReactionLongPress}
></CometChatMessageList>
);
onAddReactionPress
Function triggered when a user clicks on the 'Add More Reactions' button, allowing developers to handle this action.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const onAddReactionPress = () => {};
return (
<CometChatMessageList
group={group}
onAddReactionPress={onAddReactionPress}
></CometChatMessageList>
);
onReactionPress
Function triggered when a reaction is clicked, enabling developers to customize reaction interactions.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const onReactionPress = (
reaction: CometChat.ReactionCount,
messageObject: CometChat.BaseMessage
) => {};
return (
<CometChatMessageList
group={group}
onReactionPress={onReactionPress}
></CometChatMessageList>
);
onReactionListItemPress
Function triggered when a reaction list item is clicked, allowing developers to override its behavior in CometChatReactionsList.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const onReactionListItemPress = (
reaction: CometChat.Reaction,
messageObject: CometChat.BaseMessage
) => {};
return (
<CometChatMessageList
group={group}
onReactionListItemPress={onReactionListItemPress}
></CometChatMessageList>
);
Filters
You can adjust the MessagesRequestBuilder
in the MessageList Component to customize your message list. Numerous options are available to alter the builder to meet your specific needs. For additional details on MessagesRequestBuilder
, please visit MessagesRequestBuilder.
In the example below, we are applying a filter to the messages based on a search substring and for a specific user. This means that only messages that contain the search term and are associated with the specified user will be displayed
- App.tsx
import { CometChat } from '@cometchat/chat-sdk-react-native';
import { CometChatMessageList } from '@cometchat/chat-uikit-react-native';
function App(): React.JSX.Element {
const [chatUser, setChatUser] = React.useState<CometChat.User| undefined>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
})
}, []);
const messageRequestBuilder = new CometChat.MessagesRequestBuilder().setLimit(5);
return (
<>
{ chatUser && <CometChatMessageList
user={chatUser}
messageRequestBuilder={messageRequestBuilder}
/>
}
</>
);
}
The following parameters in messageRequestBuilder will always be altered inside the message list
- UID
- GUID
- types
- categories
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The list of events emitted by the Message List component is as follows.
Event | Description |
---|---|
openChat | this event alerts the listeners if the logged-in user has opened a user or a group chat |
ccMessageEdited | Triggers whenever a loggedIn user edits any message from the list of messages. It will have two states such as: inProgress & sent |
ccMessageDeleted | Triggers whenever a loggedIn user deletes any message from the list of messages |
ccActiveChatChanged | This event is triggered when the user navigates to a particular chat window. |
ccMessageRead | Triggers whenever a loggedIn user reads any message. |
ccMessageDelivered | Triggers whenever messages are marked as delivered for the loggedIn user |
Adding CometChatMessageEvents
Listener's
- JavaScript
import { CometChatUIEventHandler } from "@cometchat/chat-uikit-react-native";
CometChatUIEventHandler.addUIListener("UI_LISTENER_ID", {
openChat: ({ user, group }) => {
//code
},
});
CometChatUIEventHandler.addMessageListener("MESSAGE_LISTENER_ID", {
ccMessageEdited: ({ message }) => {
//code
},
});
CometChatUIEventHandler.addMessageListener("MESSAGE_LISTENER_ID", {
ccMessageDeleted: ({ message }) => {
//code
},
});
CometChatUIEventHandler.addMessageListener("MESSAGE_LISTENER_ID", {
ccMessageDelivered: ({ message }) => {
//code
},
});
CometChatUIEventHandler.addMessageListener("MESSAGE_LISTENER_ID", {
ccMessageRead: ({ message }) => {
//code
},
});
CometChatUIEventHandler.addMessageListener("MESSAGE_LISTENER_ID", {
addMessageListener: ({ message, user, group, theme, parentMessageId }) => {
//code
},
});
Removing Listeners
- JavaScript
import { CometChatUIEventHandler } from "@cometchat/chat-uikit-react-native";
CometChatUIEventHandler.removeUIListener("UI_LISTENER_ID");
CometChatUIEventHandler.removeMessageListener("MESSAGE_LISTENER_ID");
Customization
To fit your app's design requirements, you can customize the appearance of the conversation component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
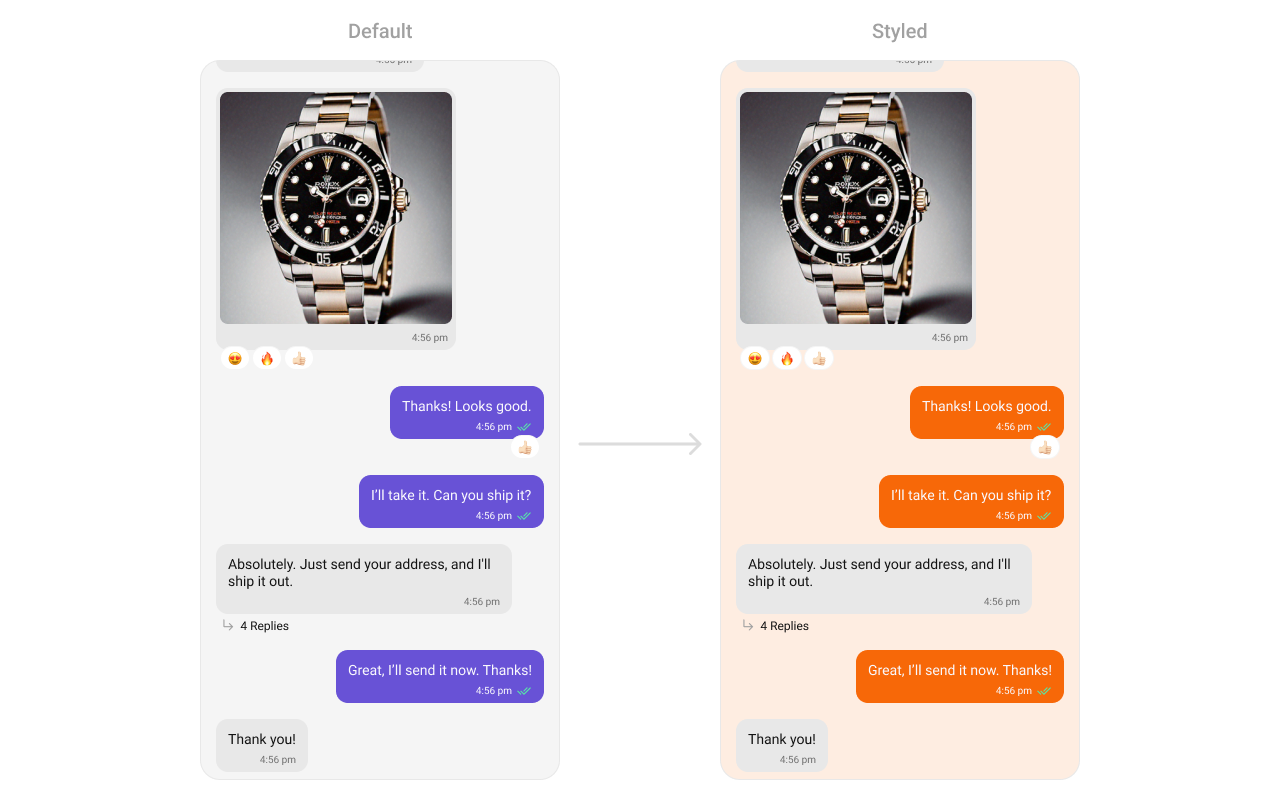
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
return (
<CometChatMessageList
group={group}
style={{
containerStyle: {
backgroundColor: "#FEEDE1",
},
outgoingMessageBubbleStyles: {
containerStyle: {
backgroundColor: "#F76808",
},
},
}}
></CometChatMessageList>
);
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
Below is a list of customizations along with corresponding code snippets
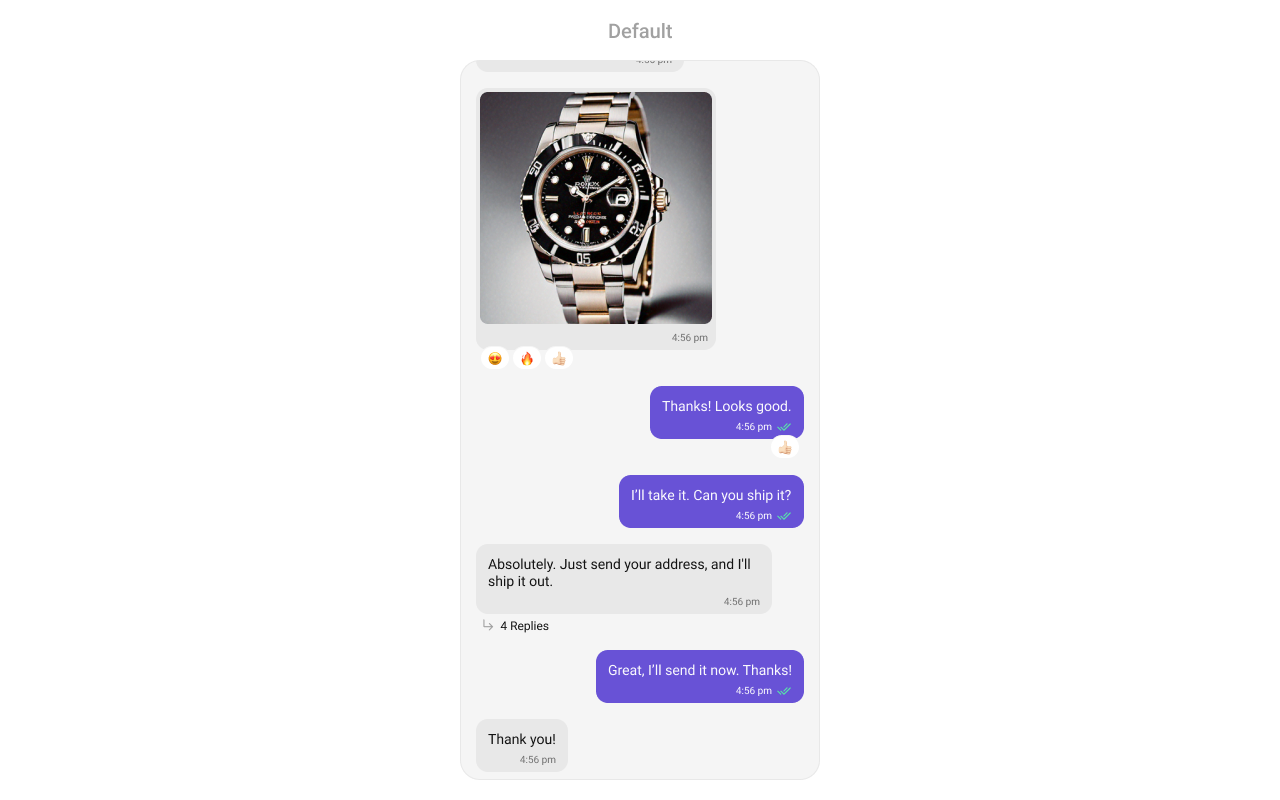
Property | Description | Code |
---|---|---|
user | Used to pass user object of which header specific details will be shown | user={chatUser} |
group | Used to pass group object of which header specific details will be shown | group={chatGroup} |
alignment | used to set the alignmet of messages in CometChatMessageList. It can be either leftAligned or standard. Type: MessageListAlignmentType | alignment={"standard"} |
disableSoundForMessages | used to enable/disable sound for incoming/outgoing messages , default false | disableSoundForMessages={true} |
customSoundForMessages | used to set custom sound for outgoing message | customSoundForMessages="your custom sound for messages" |
showAvatar | used to toggle visibility for avatar | showAvatar={true} |
scrollToBottomOnNewMessage | should scroll to bottom on new message? , by default false | scrollToBottomOnNewMessages={true} |
hideReceipt | Used to control the visibility of read receipts without affecting the functionality of marking messages as read and delivered. | hideReceipt={true} |
hideError | used to toggle visibility of error state in MessageList | hideError={true} |
quickReactionList | The list of quick reactions to be set.This list will replace the predefined set of reactions | quickReactionList=["👻","😈","🙀","🤡","❤️"]; |
Advance
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
Templates
CometChatMessageTemplate is a pre-defined structure for creating message views that can be used as a starting point or blueprint for creating message views often known as message bubbles. For more information, you can refer to CometChatMessageTemplate.
You can set message Templates to MessageList by using the following code snippet
- App.tsx
import { CometChat } from '@cometchat/chat-sdk-react-native';
import { CometChatMessageList, BorderStyleInterface, AvatarStyleInterface } from '@cometchat/chat-uikit-react-native';
function App(): React.JSX.Element {
const [chatUser, setChatUser] = React.useState<CometChat.User| undefined>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
})
}, []);
const getTemplates = () => {
let templates : CometChatMessageTemplate[] = ChatConfigurator.getDataSource().getAllMessageTemplates(theme);
templates.map((data) => {
if(data.type == "text") {
data.ContentView = (messageObject: CometChat.BaseMessage, alignment: MessageBubbleAlignmentType) => {
const textMessage : CometChat.TextMessage = (messageObject as CometChat.TextMessage);
return <Text style={{backgroundColor: "#fff5fd", padding: 10}}>{textMessage.getText()}</Text>
}
}
});
return templates;
};
return (
<>
{ chatUser && <CometChatMessageList
user={chatUser}
templates={getTemplates()}
/>
}
</>
);
}
DateSeperatorPattern
Specifies a custom format for displaying sticky date separators in the chat.
Use Cases:
- Customize date formats to match regional preferences.
- Use relative formats like "Yesterday" instead of full dates.
- Highlight weekend conversations with different styles.
- App.tsx
import React from "react";
import { CometChat } from '@cometchat/chat-sdk-react-native';
import { CometChatMessageList, BorderStyleInterface, AvatarStyleInterface } from '@cometchat/chat-uikit-react-native';
function App(): React.JSX.Element {
const [chatUser, setChatUser] = React.useState<CometChat.User| undefined>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
})
}, []);
const customDateSeparatorPattern = (date: number) => {
const timeStampInSeconds = new Date(date * 1000);
const day = String(timeStampInSeconds.getUTCDate()).padStart(2, '0');
const month = String(timeStampInSeconds.getUTCMonth() + 1).padStart(2, '0'); // Months are 0-indexed
const year = timeStampInSeconds.getUTCFullYear();
const hours = String(timeStampInSeconds.getUTCHours()).padStart(2, '0');
const minutes = String(timeStampInSeconds.getUTCMinutes()).padStart(2, '0');
return `${day}/${month}/${year}, ${hours}:${minutes}`;
}
return (
<>
{ chatUser && <CometChatMessageList
user={chatUser}
dateSeperatorPattern = {customDateSeparatorPattern}
/>
}
</>
);
}
DatePattern
Defines the format in which time appears for each message bubble.
Use Cases:
- Use 12-hour or 24-hour formats based on user preference.
- Display relative time ("Just now", "5 min ago").
- Add AM/PM indicators for clarity.
- App.tsx
import React from "react";
import { CometChat } from '@cometchat/chat-sdk-react-native';
import { CometChatMessageList, BorderStyleInterface, AvatarStyleInterface } from '@cometchat/chat-uikit-react-native';
function App(): React.JSX.Element {
const [chatUser, setChatUser] = React.useState<CometChat.User| undefined>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
})
}, []);
const generateDateString = (message: CometChat.BaseMessage) : any => {
const days = [
'Sunday',
'Monday',
'Tuesday',
'Wednesday',
'Thursday',
'Friday',
'Saturday',
];
const timeStamp = message['sentAt'];
if(timeStamp) {
const timeStampInSeconds = new Date(timeStamp * 1000);
const day = days[timeStampInSeconds.getUTCDay()].substring(0, 3);
const hours = timeStampInSeconds.getUTCHours();
const minutes = String(timeStampInSeconds.getUTCMinutes()).padStart(2, '0');
return `${day}, ${hours}:${minutes}`;
}
return `---, --:--`;
};
return (
<>
{ chatUser && <CometChatMessageList
user={chatUser}
datePattern={generateDateString}
/>
}
</>
);
}
LoadingView
Customizes the loading indicator when messages are being fetched.
Use Cases:
- Show a spinner or skeleton loader for smooth UX.
- Display a "Fetching messages..." text.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const getLoadingView = (): JSX.Element => {
//return jsx;
};
return (
<CometChatMessageList
group={group}
LoadingView={getLoadingView}
></CometChatMessageList>
);
EmptyView
Defines a custom view to be displayed when no messages are available.
Use Cases:
- Show a friendly message like "No messages yet. Start the conversation!".
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const getEmptyView = (): JSX.Element => {
//return jsx;
};
return (
<CometChatMessageList
group={group}
EmptyView={getEmptyView}
></CometChatMessageList>
);
ErrorView
Custom error state view displayed when fetching messages fails.
Use Cases:
- Show a retry button when an error occurs.
- Display a friendly message like "Couldn't load messages. Check your connection.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const getErrorView = (): JSX.Element => {
//return jsx;
};
return (
<CometChatMessageList
group={group}
ErrorView={getErrorView}
></CometChatMessageList>
);
TextFormatters
Assigns the list of text formatters. If the provided list is not null, it sets the list. Otherwise, it assigns the default text formatters retrieved from the data source. To configure the existing Mentions look and feel check out MentionsFormatter Guide
- App.tsx
- CustomMentionsFormatter.ts
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const getTextFomatters = () => {
const textFormatters: CometChatTextFormatter[] = [];
const customMentionsFormatter = new CustomMentionsFormatter();
textFormatters.push(customMentionsFormatter);
return textFormatters;
};
return (
<CometChatMessageList
group={group}
textFormatters={getTextFomatters()}
></CometChatMessageList>
);
import {
CometChatMentionsFormatter,
CometChatTheme,
CometChatUIKit,
} from "@cometchat/chat-uikit-react-native";
/**
* Represents the CometChatMentionsFormatter class.
* This class extends the CometChatTextFormatter class and provides methods for handling mentions in text.
* @extends CometChatTextFormatter
*/
export class CustomMentionsFormatter extends CometChatMentionsFormatter {
/**
* Sets the mentions style.
*
* @param {CometChatTheme["mentionsStyle"]} mentionsStyle - The mentions style to be set.
*/
setMentionsStyle(mentionsStyle: CometChatTheme["mentionsStyle"]) {
if (mentionsStyle) {
this.mentionsStyle = mentionsStyle;
return;
}
if (!this.messageObject) {
return;
}
const isMessageSentByLoggedInUser =
this.messageObject.getSender().getUid() ===
CometChatUIKit.loggedInUser!.getUid();
if (isMessageSentByLoggedInUser) {
this.mentionsStyle = {
selfTextStyle: {
color: "#30A46C",
},
textStyle: {
color: "#FFFFFF",
},
};
} else {
this.mentionsStyle = {
selfTextStyle: {
color: "#30A46C",
},
textStyle: {
color: "#D6409F",
},
};
}
}
}
Example
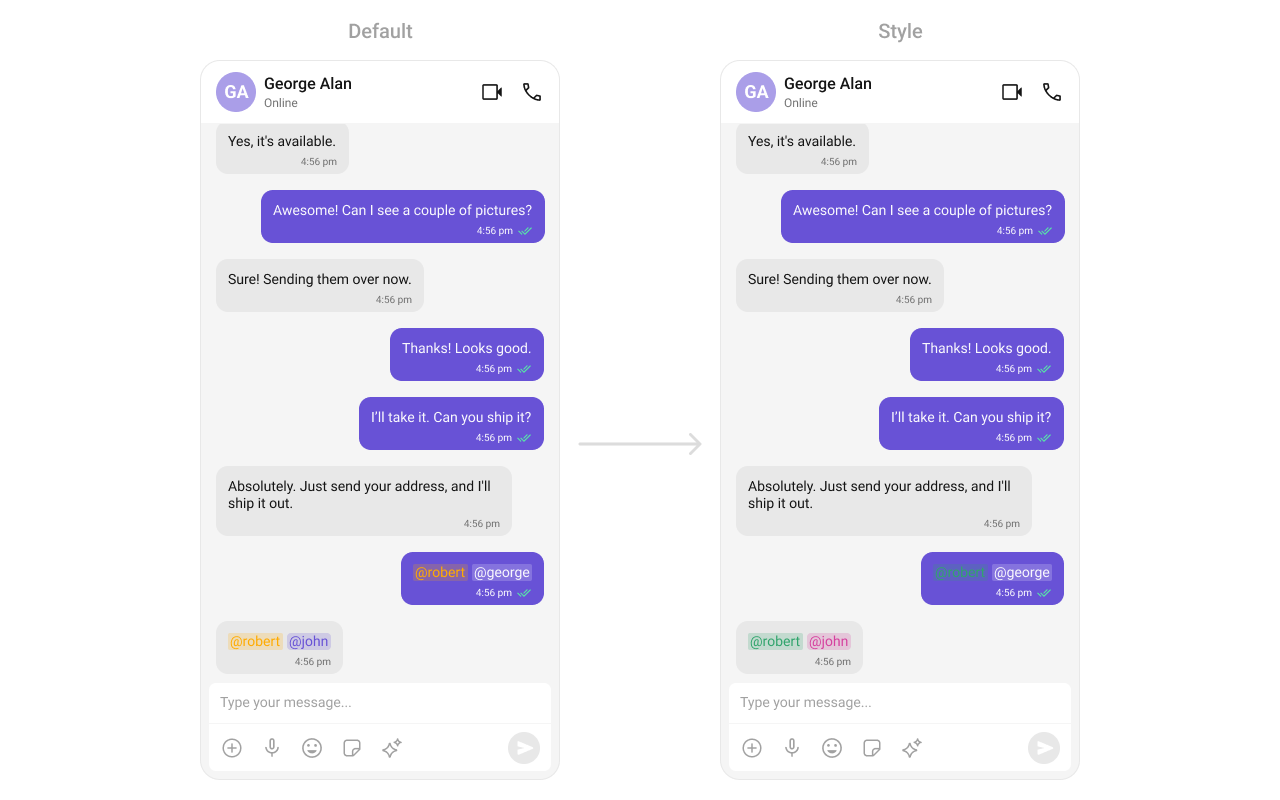
HeaderView
This method allows you to set a custom header view for the message list. By providing a View object, you can customize the appearance and content of the header displayed at the top of the message list.
Use Cases:
- Add a custom branding/logo to the chat.
- Display chat status ("John is typing...").
- Show last seen status.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const getMessageListHeaderView = ({
user,
group,
id,
}: {
user?: CometChat.User;
group?: CometChat.Group;
id?: {
uid?: string;
guid?: string;
parentMessageId?: string;
};
}) => {
//return jsx;
};
return (
<CometChatMessageList
group={group}
HeaderView={getMessageListHeaderView}
></CometChatMessageList>
);
Example
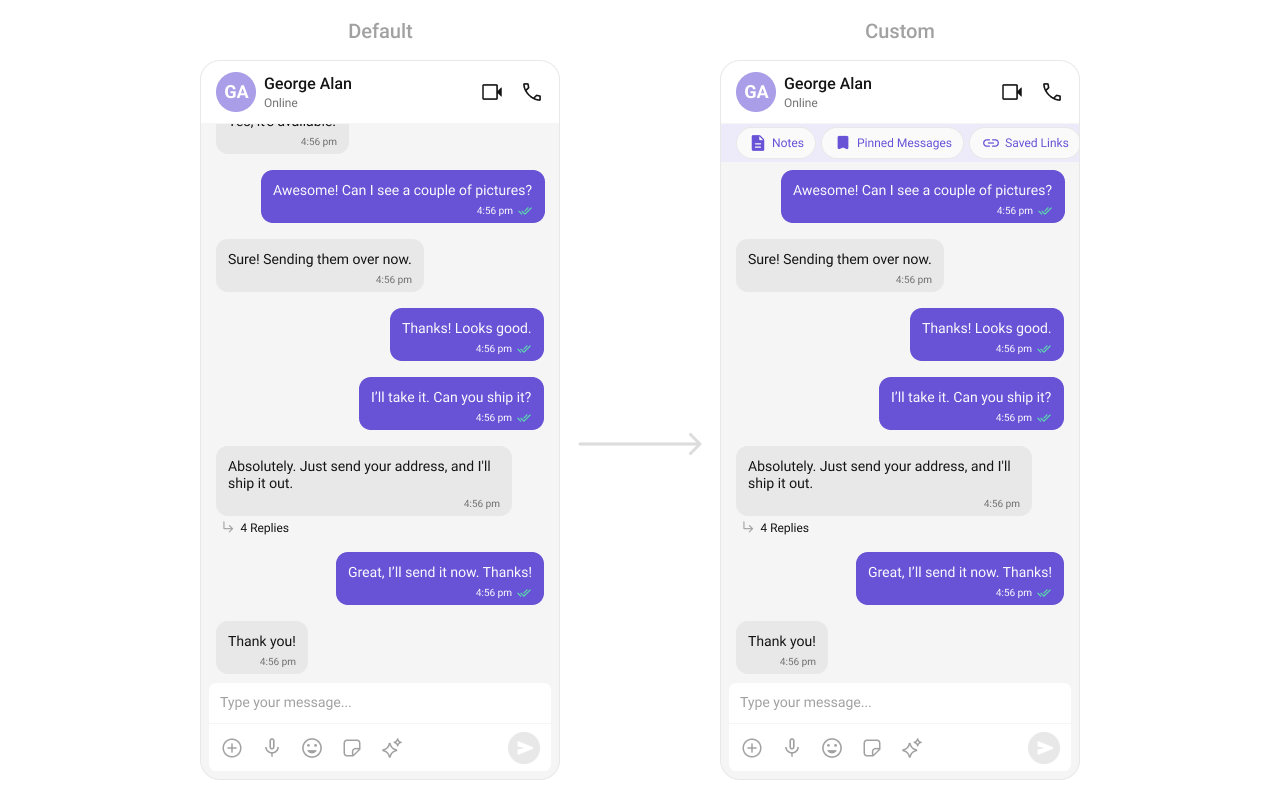
FooterView
This method allows you to set a custom footer view for the message list. By providing a View object, you can customize the appearance and content of the footer displayed at the bottom of the message list.
Use Cases:
- Add quick reply buttons.
- Display typing indicators ("John is typing...").
- Show a disclaimer or privacy notice.
- App.tsx
import { CometChatMessageList } from "@cometchat/chat-uikit-react-native";
import { CometChat } from "@cometchat/chat-sdk-react-native";
//code
const getMessageListFooterView = ({
user,
group,
id,
}: {
user?: CometChat.User;
group?: CometChat.Group;
id?: {
uid?: string;
guid?: string;
parentMessageId?: string;
};
}) => {
//return jsx;
};
return (
<CometChatMessageList
group={group}
FooterView={getMessageListFooterView}
></CometChatMessageList>
);
Example
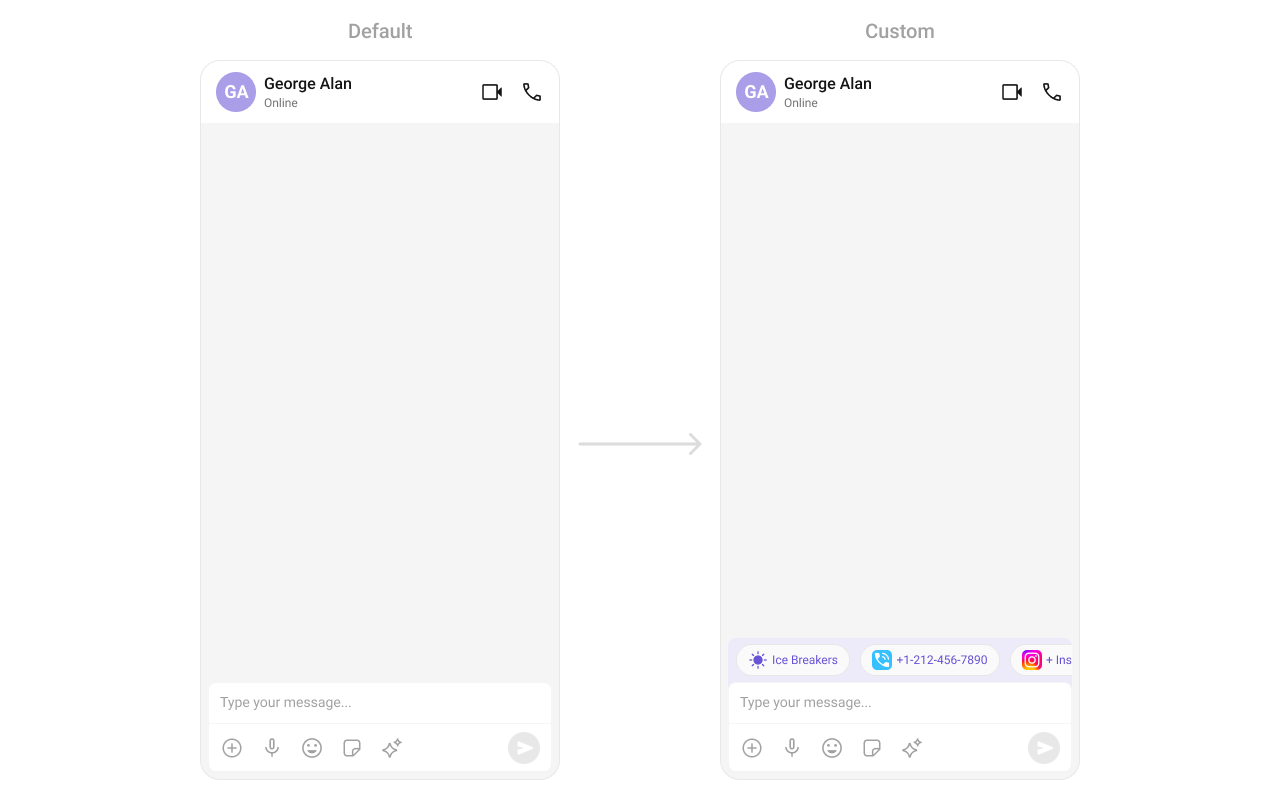