ShortCut Formatter
Introduction
The ShortCutFormatter class extends the CometChatTextFormatter class to provide a mechanism for handling shortcuts within messages. This guide will walk you through the process of using ShortCutFormatter to implement shortcut extensions in your CometChat application.
Setup
- Create the ShortCutFormatter Class: Define the
ShortCutFormatter
class by extending theCometChatTextFormatter
class.
- Java
- Kotlin
public class ShortCutFormatter extends CometChatTextFormatter {
// Class implementation
private HashMap<String, String> messageShortcuts;
private List<SuggestionItem> shortcuts;
}
class ShortCutFormatterKotlin : CometChatTextFormatter('!') {
// Class implementation
private val messageShortcuts: HashMap<String, String> = HashMap()
private val shortcuts: MutableList<SuggestionItem> = ArrayList()
}
- Constructor: Initialize the
messageShortcuts
map andshortcuts
list in the constructor.
- Java
- Kotlin
public ShortCutFormatter() {
super('!');
messageShortcuts = new HashMap<>();
prepareShortCuts();
shortcuts = new ArrayList<>();
}
init {
prepareShortCuts()
}
- Prepare Shortcuts: Implement the
prepareShortCuts()
method to fetch shortcuts from the server using CometChat extension.
- Java
- Kotlin
private void prepareShortCuts() {
// Implementation to fetch shortcuts from server
CometChat.callExtension("message-shortcuts", "GET", "/v1/fetch", null, new CometChat.CallbackListener<JSONObject>() {
@Override
public void onSuccess(JSONObject responseObject) {
Iterator<String> keysItr;
try {
JSONObject shortcutObject = responseObject.getJSONObject("data").getJSONObject("shortcuts");
keysItr = shortcutObject.keys();
while (keysItr.hasNext()) {
String key = keysItr.next();
String value = shortcutObject.getString(key);
messageShortcuts.put(key, value);
}
} catch (JSONException e) {
e.printStackTrace();
}
}
@Override
public void onError(CometChatException e) {
}
});
}
private fun prepareShortCuts() {
CometChat.callExtension(
"message-shortcuts",
"GET",
"/v1/fetch",
null,
object : CometChat.CallbackListener<JSONObject>() {
override fun onSuccess(responseObject: JSONObject) {
try {
val shortcutObject =
responseObject.getJSONObject("data").getJSONObject("shortcuts")
val keysItr: Iterator<String> = shortcutObject.keys()
while (keysItr.hasNext()) {
val key = keysItr.next()
val value = shortcutObject.getString(key)
messageShortcuts[key] = value
}
} catch (e: JSONException) {
e.printStackTrace()
}
}
override fun onError(e: CometChatException) {}
})
}
- Override Search Method: Override the
search()
method to search for shortcuts based on the entered query.
- Java
- Kotlin
@Override
public void search(@NonNull Context context, String queryString) {
// Implementation to search for shortcuts
String query = getTrackingCharacter() + queryString;
shortcuts.clear();
if (messageShortcuts.containsKey(query)) {
SuggestionItem suggestionItem = new SuggestionItem("", query + " => " + messageShortcuts.get(query), null, null, messageShortcuts.get(query), null, null);
suggestionItem.setHideLeadingIcon(true);
shortcuts.add(suggestionItem);
}
setSuggestionItemList(shortcuts);
}
override fun search(context: Context, queryString: String?) {
val query = trackingCharacter.toString() + queryString
shortcuts.clear()
if (messageShortcuts.containsKey(query)) {
val suggestionItem = SuggestionItem(
"",
"$query => ${messageShortcuts[query]}",
null,
null,
messageShortcuts[query],
null,
null
)
suggestionItem.isHideLeadingIcon = true
shortcuts.add(suggestionItem)
}
suggestionItemList.value = shortcuts
}
- Handle Scroll to Bottom: Override the
onScrollToBottom()
method if needed.
- Java
- Kotlin
@Override
public void onScrollToBottom() {
// Implementation if needed
}
override fun onScrollToBottom() {
TODO("Not yet implemented")
}
Usage
- Initialization: Initialize an instance of
ShortCutFormatter
in your application.
- Java
- Kotlin
ShortCutFormatter shortCutFormatter = new ShortCutFormatter();
val shortCutFormatter = ShortCutFormatter()
- Integration: Integrating the
ShortCutFormatter
into your CometChat application involves incorporating it within your project to handle message shortcuts. If you're utilizing the CometChatConversationsWithMessages component, you can seamlessly integrate the ShortCutFormatter to manage shortcut functionalities within your application.
- XML
<com.cometchat.chatuikit.conversationswithmessages.CometChatConversationsWithMessages
android:id="@+id/conversationWithMessages"
android:layout_width="match_parent"
android:layout_height="match_parent" />
- Java
- Kotlin
// Integrate ShortCutFormatter into your CometChat application
CometChatConversationsWithMessages cometChatConversationsWithMessages = findViewById(R.id.conversationWithMessages);
List<CometChatTextFormatter> cometChatTextFormatters = CometChatUIKit.getDataSource().getTextFormatters(this);
cometChatTextFormatters.add(new ShortCutFormatter());
cometChatConversationsWithMessages.setMessagesConfiguration(new MessagesConfiguration().setMessageComposerConfiguration(new MessageComposerConfiguration().setTextFormatters(cometChatTextFormatters)));
val cometChatConversationsWithMessages: CometChatConversationsWithMessages =
findViewById<CometChatConversationsWithMessages>(R.id.conversationWithMessages)
val cometChatTextFormatters = CometChatUIKit.getDataSource().getTextFormatters(this)
cometChatTextFormatters.add(ShortCutFormatter())
cometChatConversationsWithMessages.messagesConfiguration =
MessagesConfiguration().setMessageComposerConfiguration(
MessageComposerConfiguration().setTextFormatters(cometChatTextFormatters)
)
Example
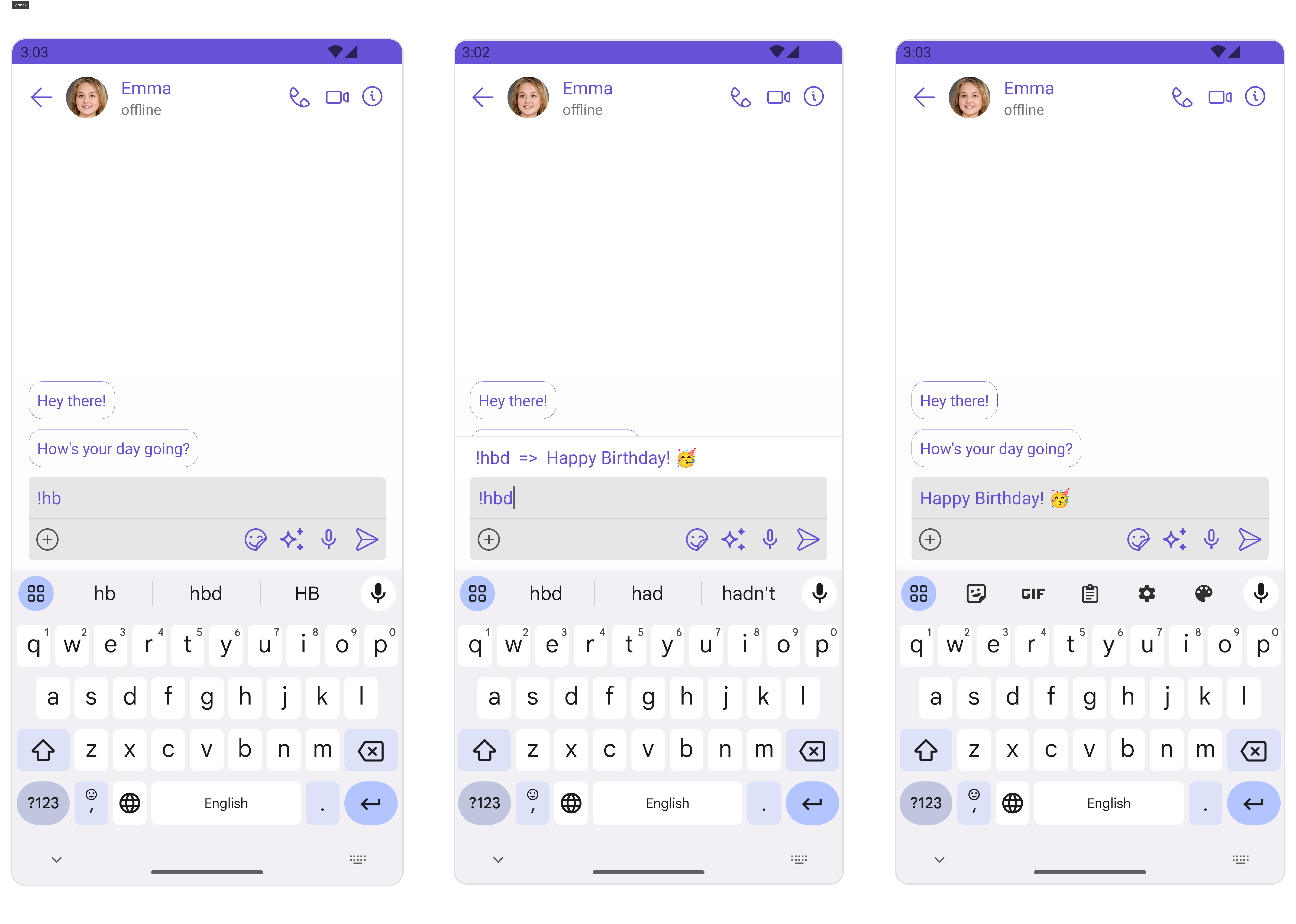