Real-time chat functionality has become a cornerstone of enhancing user engagement and fostering seamless communication in modern web applications. The versatility of real-time chat extends beyond mere messaging to transform digital experiences across diverse domains.
In this article, you'll learn how to create a real-time messaging application using the CometChat UI Kit for React. The application will support one-to-one messaging, channels, and audio and video calls. It will also have advanced features like support for presence indicators, typing status, read receipts, and more.
Prerequisites
To complete this tutorial, you'll need the following:
Node.js installed on your local machine
A code editor and a web browser
You'll need to create a CometChat account and set up a new app within your account. This will provide you with an app ID, API key, and region, which you'll need later in the development process.
Developing the react chat app
To build the chat application, you'll do the following:
1. Create a CometChat app
2. Set up the project
3. Implement a basic login system
4. Implement one-to-one chatting and channels
5. Add various features to the application to make the chatting experience richer
Creating a CometChat application
To create a CometChat application, you'll need to register for a free account if you don't have one already. In the account creation process, you will also create a new application.
Add real-time chat with minimal effort using CometChat
After the app has been successfully created, head over to the Credentials section and take note of the app ID, region, and auth key
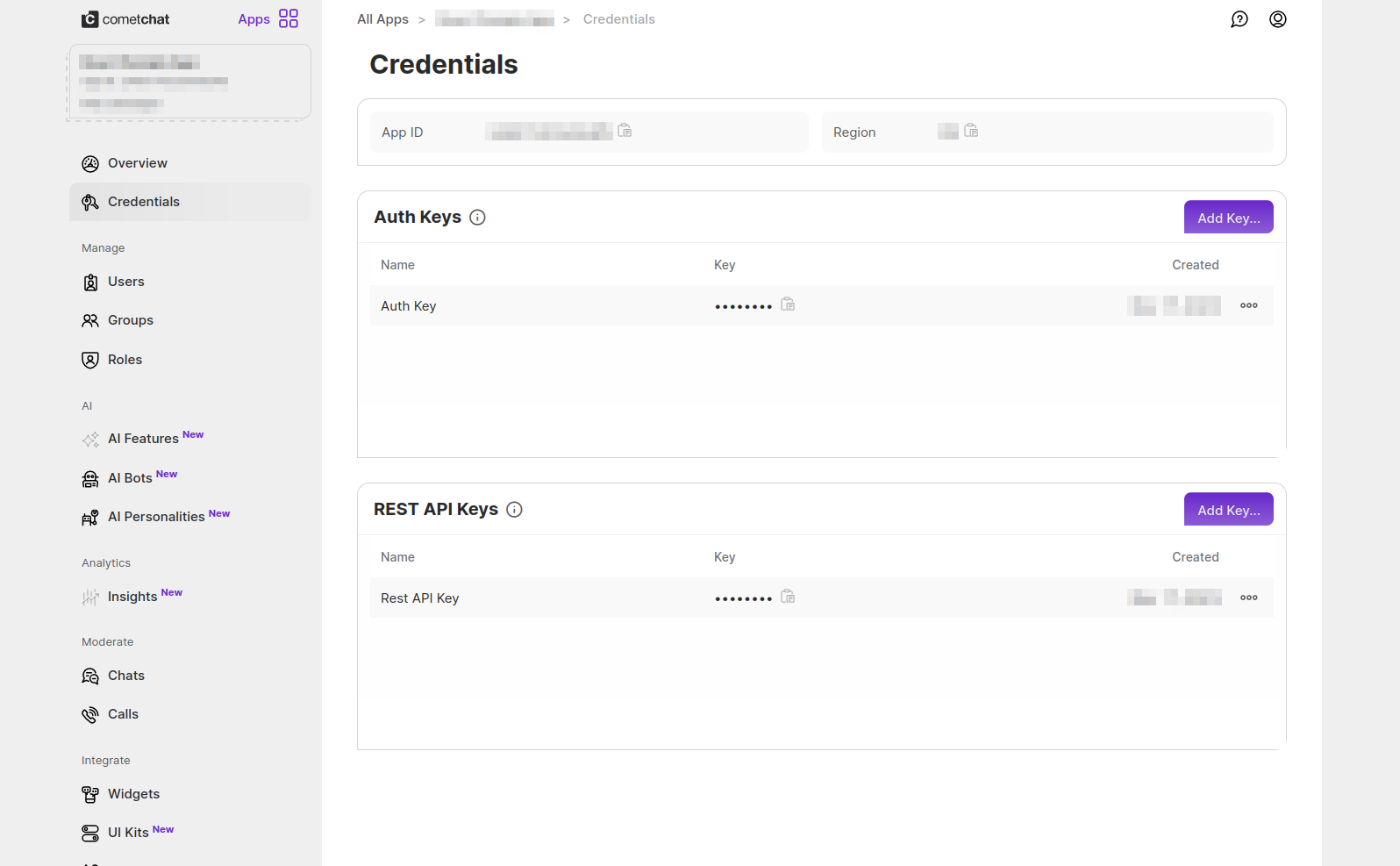
Setting up the project
Now that you have the credentials to connect to the CometChat application, you can create the React application. You'll use Vite as the build tool. To do this, execute the following command in your terminal
After the React application has been created, execute the following commands to cd
into the project folder and install the necessary dependencies:
If your npm version is between 3 and 6, you'll also need to install the following peer dependencies:
These dependencies add the CometChat React UI Kit to the project.
Next, create a .env
file in the project root folder to store the CometChat connection credentials. Add the following to the file:
Replace the placeholder values with the corresponding CometChat credentials that you got from the previous section.
The next step is to initialize the CometChat UI Kit. You do this by calling the init()
method to initialize the settings required for CometChat. It's recommended to call this method on the app's startup—in this case, the src/main.jsx
file.
Replace the placeholder values with the corresponding CometChat credentials that you got from the previous section.
The next step is to initialize the CometChat UI Kit. You do this by calling the init()
method to initialize the settings required for CometChat. It's recommended to call this method on the app's startup—in this case, the src/main.jsx
file.
Open the src/main.jsx
file and replace the existing code with the following:
The code above uses UIKitSettingsBuilder
to construct the settings for the UI Kit, incorporating essential information such as the CometChat application ID, region, and authentication key from environment variables. It then attempts to initialize the CometChat UI Kit with these settings, logging a success message if the initialization is completed and an error message if any issues arise.
Implementing a Basic Authentication System
You'll now implement a basic authentication system that allows you to log in to the app as different users. CometChat provides five users for testing: SUPERHERO1
, SUPERHERO2
, SUPERHERO3
, SUPERHERO4
, and SUPERHERO5
. CometChat allows you to log in using either the auth key or an auth token. This guide uses the auth key.
Open the src/App.jsx
file and replace the existing code with the following:
This code defines a getUser()
function that leverages the CometChatUIKit.getLoggedinUser()
method to check if a user is logged in. This method updates the user
variable and is called once when the page loads. The code also leverages conditional rendering based on the user
variable. If the user is logged in, it renders the ChatUI
page, and if not, it renders the Login
page.
Create a file at src/pages/Login.jsx
and add the code below:
This code defines a page that allows a user to log in as one of the test users offered by CometChat.
Next, create a file at src/pages/ChatUI.jsx
and add the code below:
This code shows the user that they are logged in. You'll implement the chat functionality later on this page.
To test the authentication system, run the server using the command npm run dev
and navigate to http://localhost:5173/
in your browser:
Implementing one-to-one chatting and channels
CometChat provides various prebuilt UI components that you can use to implement the chat functionality for your app. There are many different ways you can create a chat application using these prebuilt components.
For this guide, you'll use the `CometChatConversationsWithMessages`component. This component displays the recent conversations between users in a one-to-one chat or a group chat.
It also allows you to send text, images, videos, and other forms of media as messages to communicate with other users in real time. You can also make audio and voice calls using this component. Additionally, you can access a list of users and groups registered on CometChat and initiate conversations with them.
To integrate this component into your application, add the following import
statements at the top of the src/pages/ChatUI.jsx
file:
Next, add some styles for the component before the return
statement:
Lastly, in the same file, replace the You are logged in.
message with the following:
Navigate to http://localhost:5173/
in your browser, and you should have a styled chat interface:



To test one-to-one messaging, select the new message button from the sidebar, and you should be able to see a list of the users:


Creating Groups
CometChat's Group with Messages component allows you to create groups directly from the UI Kit. However, this would work best in a UI where you are displaying one-to-one conversations and groups on different screens. To maintain the current UI, you will explore a more advanced option for creating groups using the CometChat [JavaScript SDK](https://www.cometchat.com/docs/v4/javascript-chat-sdk/overview).
You will create a simple modal to display a form to create the group. To create the modal, you'll use the react-model pm package, which you can install using the command below:
Next create 'src/components/GroupCreationComponent.jsx and add the code below:
This code renders a button for creating a new group. When the button is clicked, it opens a modal that allows the user to input a group name. The modal contains the input field for the group name, along with "Create Group" and "Cancel" buttons.
The handleCreateGroup
function leverages the CometChat JavaScript SDK to create a group . This function generates a new group object using CometChat.Group
with a unique identifier based on the current timestamp and a random number, the name of the group provided by the user, and the type of group. The type of group can either be CometChat.GROUP_TYPE.PUBLIC
, CometChat.GROUP_TYPE.PASSWORD
, or CometChat.GROUP_TYPE.PRIVATE
. After generating the group object, the CometChat.createGroup
function is called to create the group, and the group object is passed as an argument. If the group is successfully created, the code closes the modal and calls the setCurrentGroup
function with the newly created group. This function is passed as a prop to this component; you'll implement it later.
Now that you have implemented the functionality to create a group, you need to create a button that will show the modal. To do this, first open the src/pages/ChatUI.jsx
file and add the following code to track the state of the current group, which will help to open a group after it's created:
Next, in the same file, add the following code before the return
statement:
Make sure you add the following import
statements to the same file:
Lastly, pass the configuration to the CometChatConversationsWithMessages
component:
To test this, navigate to http://localhost:5173/
, and you'll notice that the "New Group" button appears in the sidebar:

Click the button to reveal the modal, input the group name, and click Create Group:

Once the group is created, the new group conversation will be opened, and you can add members and start sending messages:
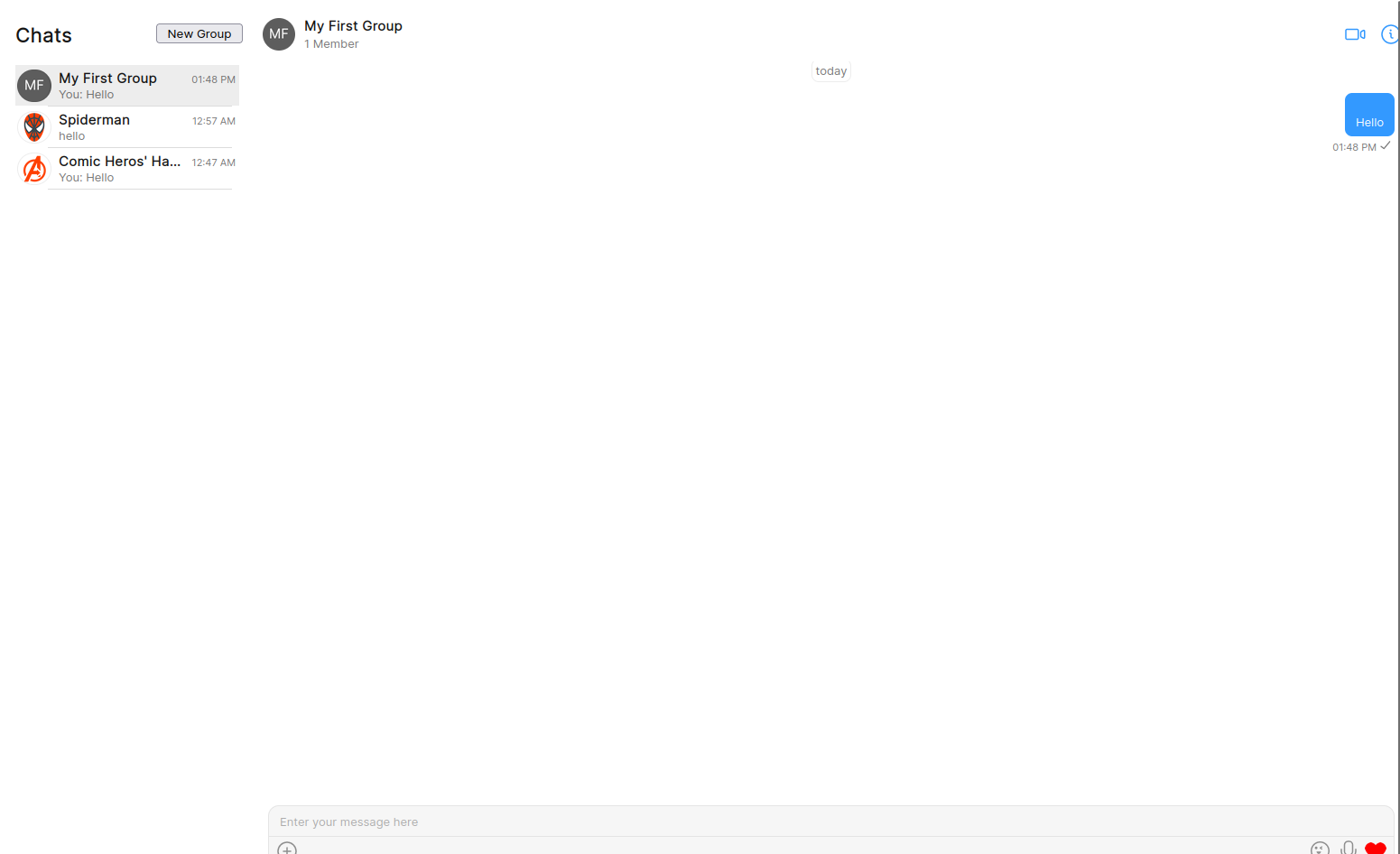
Note: The groups feature you're implementing here is very basic as it's intended solely for demonstration. You can always improve the look of the New Group button and add screens in the flow to add users to the group right after creating it.
Adding Features to the Application
The prebuilt components allow you to configure several features for your application, which should help enrich the user experience. You do this by configuring the properties of the CometChatConversations
component. The component you used in this application is CometChatConversationsWithMessages
, which is a parent component of CometChatConversations
. CometChat makes it convenient and easy to customize a child component via a parent component.
For this application, you'll configure presence indicators, typing status, read receipts, and notifications. To do this, open the src/pages/ChatUI.jsx
file and add the following key-value pairs inside the conversationConfig
object:
In the code above:
- disableUsersPresence
determines whether a user will be able to see if a particular user is currently online or offline.
- disableTyping
determines whether typing events are enabled and if the typing indicators will be displayed.
- disableReceipt
determines whether the visual indication that the message has been read or viewed by the recipient is shown.
- disableSoundForMessages
determines whether a sound is produced for all incoming messages.
Please note that this configuration only applies to the conversations list, and you will still be able to see read receipts inside the chat screen. You can configure other aspects of the chat conversations, as explained in the official documentation.
Adding More Advanced Features
CometChat also offers more advanced features that you can add to your chat application. These include moderation, canned conversation starters, and video and audio calling. These features are specific to certain use cases. In the following sections, you'll learn what they are, why they are important, and how you can use CometChat to implement them.
Moderation
Moderation refers to the implementation of mechanisms and tools to monitor, control, and manage user-generated content within the platform. It plays a crucial role in maintaining a safe and respectful environment for users by preventing and addressing inappropriate or harmful content, such as offensive language, harassment, or any form of misconduct. CometChat provides several extensions that provide chat moderation features to help filter out content that violates the set community standards.
To implement moderation in your chat app, access your application from the CometChat dashboard and select Extensions from the sidebar. Scroll down to the Moderation section, and you will see several extensions that you can use to implement moderation.

As a simple example, you can implement sentiment analysis to make sure that your users cannot send messages with negative sentiment. On the Sentiment Analysis card, click the Enable button. After the extension has been enabled, select the cog icon to access the extension's settings.
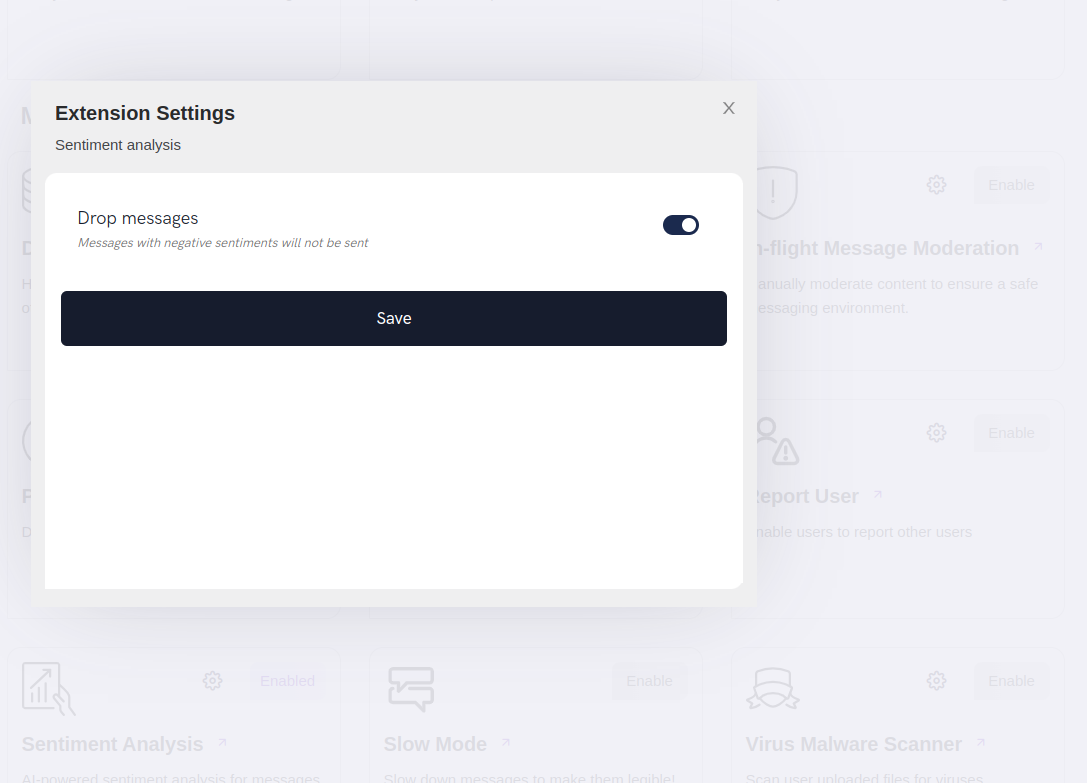
On the Extension Settings page, select Drop messages and click the Save button. This ensures that messages with negative sentiment will not be sent.
Go back to your application and try to send a message with the content "I hate you!". This message will be blocked by the sentiment analysis extension. You can verify that by checking the console logs in the image below. In a real-world application, you should implement alerts to notify the user that the message has been blocked.
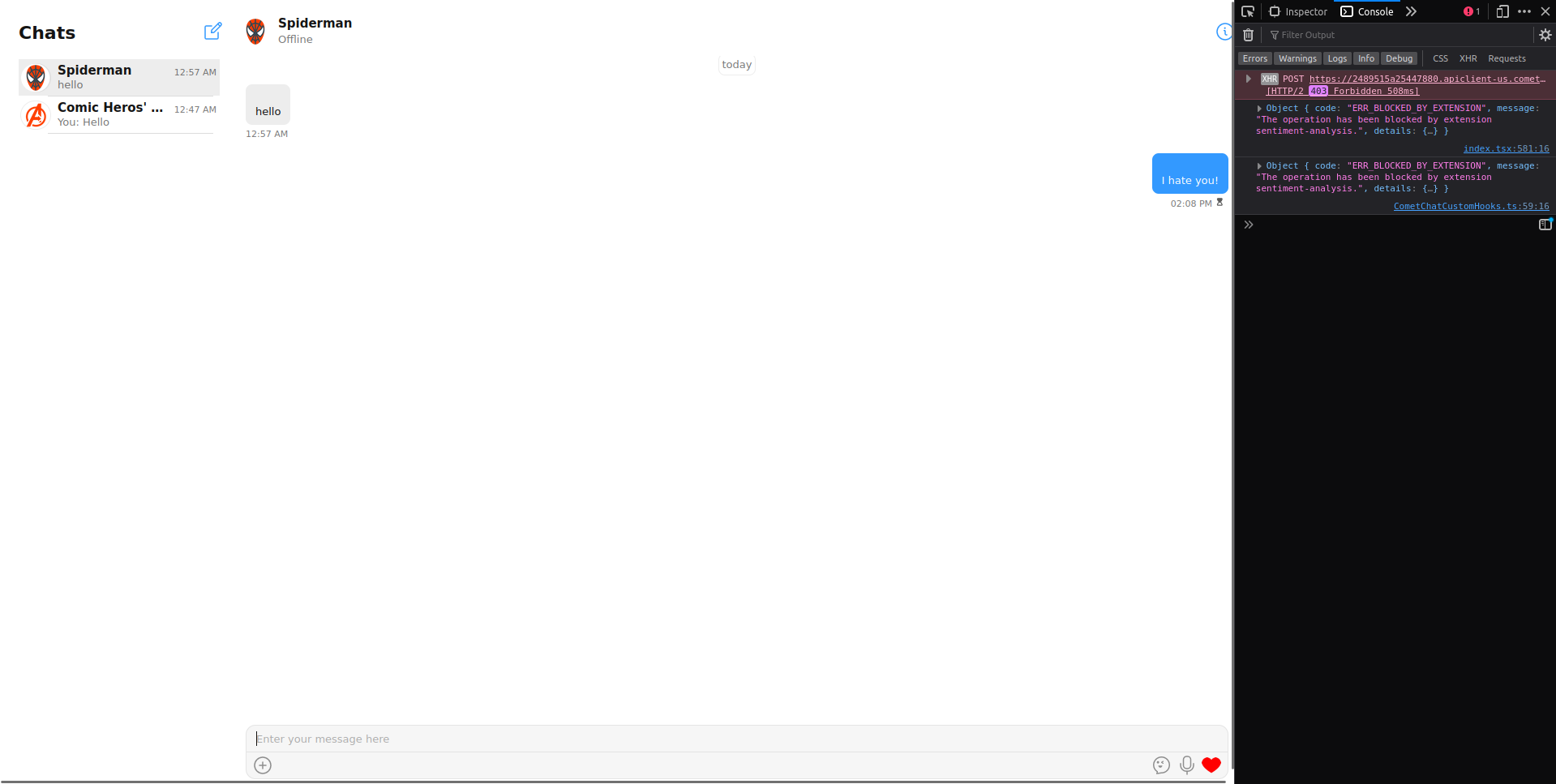
Video and Audio Calling
An audio and video calling feature enables users to engage in real-time voice and video conversations, transcending the limitations of text-based communication. CometChat provides a seamless solution to integrate this feature into your application. All you need to do is install the "Calls" SDK using the following command:
The calls SDK you just installed will enable Call Buttons component in the message header menu. With these buttons, users can easily initiate voice and video calls with other participants.
To test this out, stop your Vite server using Ctrl + C
or Cmd + C
and restart it with the command npm run dev
. Open any chat, and you will see the call buttons on the top right corner.

Canned Conversation Starters
Canned conversation starters are prewritten, ready-to-use prompts or messages that users can deploy to initiate discussions with others. These prompts serve as icebreakers, easing the initiation of conversations and providing users with engaging and contextually relevant suggestions.
CometChat leverages AI models from OpenAI to support canned conversation starters. CometChat AI is able to provide conversation starters by analyzing your tone and writing style by going through the recent messages that you sent via the platform.
To implement canned conversation starters in your application, you need to contact the CometChat sales team to enable AI features for your application.
Conclusion
In this guide, you learned how to create a real-time chat application using CometChat , a powerful toolkit that facilitates the incorporation of real-time chat features into React applications with ease. By supporting features such as audio and video calling, canned conversation starters, and robust moderation capabilities, CometChat empowers developers to create dynamic and secure chat applications tailored to the evolving needs of today's users.
Unlock the full spectrum of possibilities by taking the first step towards seamless and feature-rich communication and signing up for a free CometChat account.
You can access the full project code on GitHub.
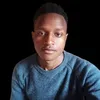
Kevin Kimani