Overview
The CometChat Vue UI Kit is developed to keep developers in mind and aims to reduce development efforts significantly.
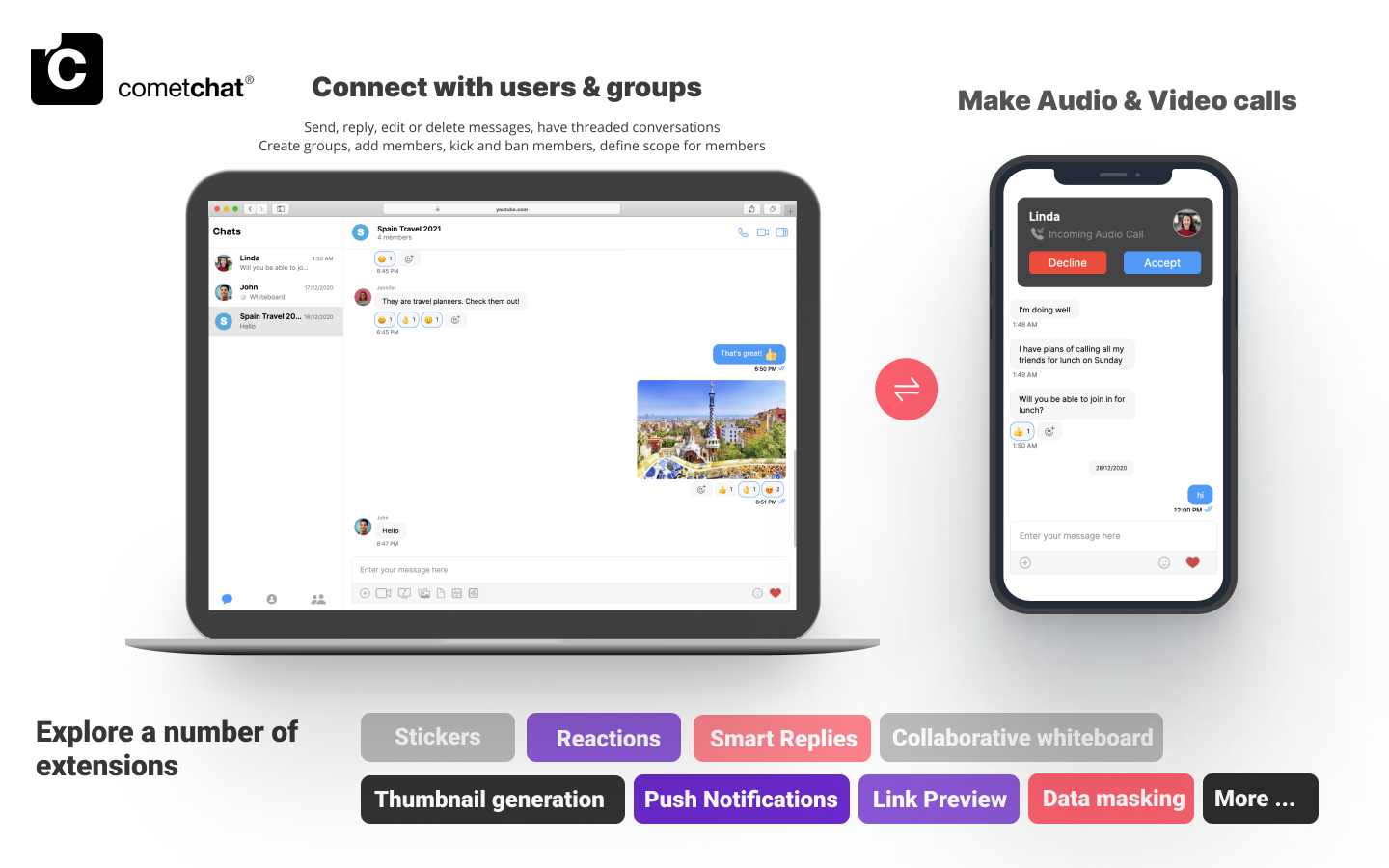
The UI Kitβs customizable UI components simplify the process of integrating text chat and voice/video calling features to your website or mobile application in a few minutes.
I want to checkout Vue UI Kit
Follow the steps mentioned in the README.md
file.
Kindly, click on below button to download our Vue UI Kit.
Vue UI KitView on GithubI want to explore the sample app
Kindly, click on below button to download our Vue Sample App.
Vue Sample AppView on GithubPrerequisitesβ
Before you begin, ensure you have met the following requirements:
For installing Vue 2
- CLI
npm install vue@2.6.14
For installing Vue 3
- CLI
npm install vue@3.2.11
Installing Vue UI Kitβ
Setupβ
-
Register on CometChat π§
- To install Vue UI Kit, you need to first register on the CometChat Dashboard. Click here to Sign Up.
-
Get your application keys π a. Create a new app. b. Head over to the QuickStart or API & Auth Keys section and note the App ID, Auth Key, and Region.
-
Add the CometChat dependency π¦
- CLI
npm install @cometchat-pro/chat@2.4.1-beta1 --save
Configure CometChat inside your appβ
- Import CometChat SDK
- Javascript
import { CometChat } from "@cometchat-pro/chat";
- Initialize CometChat π
The init() method initializes the settings required for CometChat. We suggest calling the init() method on app startup, preferably in the created() method of the Application class.
- Javascript
const appID = "APP_ID";
const region = "REGION";
const appSetting = new CometChat.AppSettingsBuilder()
.subscribePresenceForAllUsers()
.setRegion(region)
.build();
CometChat.init(appID, appSetting).then(
() => {
console.log("Initialization completed successfully");
// You can now call login function.
},
error => {
console.log("Initialization failed with error:", error);
// Check the reason for error and take appropriate action.
}
);
Replace APP_ID and REGION with your CometChat App ID and Region in the above code
- Create user
This method takes a User
object and the Auth Key
as input parameters and returns the created User
object if the request is successful.
- Javascript
let authKey = "AUTH_KEY";
var uid = "user1";
var name = "Kevin";
var user = new CometChat.User(uid);
user.setName(name);
CometChat.createUser(user, authKey).then(
user => {
console.log("user created", user);
},error => {
console.log("error", error);
}
)
- Login your user π€
This method takes UID and Auth Key as input parameters and returns the User object containing all the information of the logged-in user.
- Javascript
const authKey = "AUTH_KEY";
const uid = "cometchat-uid-1";
CometChat.login(uid, authKey).then(
user => {
console.log("Login Successful:", { user });
},
error => {
console.log("Login failed with exception:", { error });
}
);
Replace AUTH_KEY with your CometChat Auth Key in the above code.
We have set up 5 users for testing having UIDs: cometchat-uid-1, cometchat-uid-2, cometchat-uid-3, cometchat-uid-4, and cometchat-uid-5.
We have used uid cometchat-uid-1 as an example here. You can create a User from CometChat Dashboard as well.
Add UI Kit to your projectβ
- Clone this repository.
- CLI
git clone https://github.com/cometchat-pro/cometchat-pro-vue-ui-kit.git -b v2
- Copy the folder to your source folder.
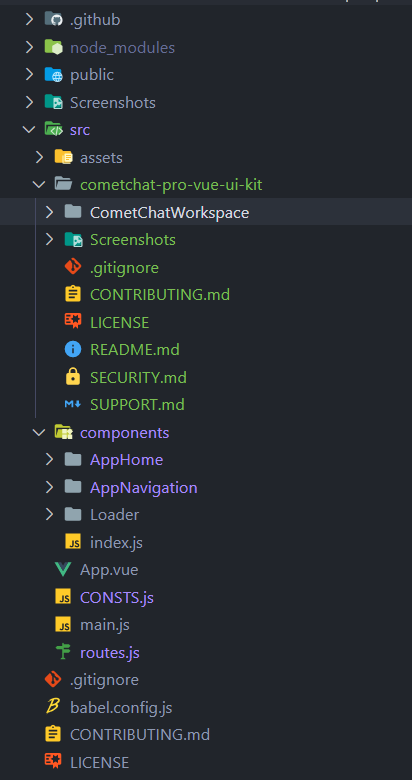
- Copy all the dependencies from package.json of
cometchat-pro-vue-ui-kit
into your project's package.json and install them. - We are using emoji-mart-vue-fast Please install respective library depending on your Vue version
-
- For Vue2 :
npm install emoji-mart-vue-fast@7.0.7
- For Vue3 :
npm install emoji-mart-vue-fast@8.0.3
- For Vue2 :
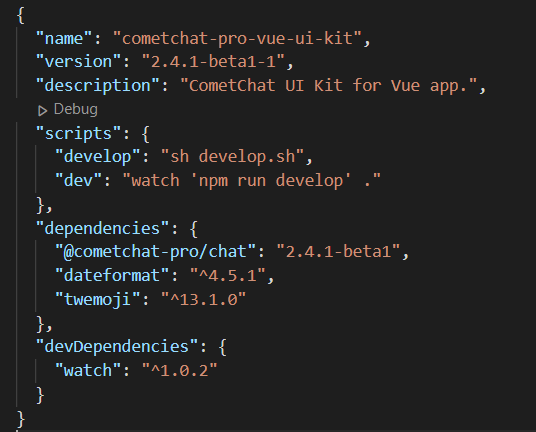
Launch CometChatβ
Using the CometChatUI
component from the UI Kit, you can launch a fully functional chat application.
In this component, all the UI Components are interlinked and work together to launch a fully functional chat on your website/application.
Usageβ
- Vue
<template>
<div id="app">
<CometChatUI />
</div>
</template>
<script>
import { CometChatUI } from "./cometchat-pro-vue-ui-kit/CometChatWorkspace/src";
export default {
name: "App",
components: {
CometChatUI,
}
};
</script>
Checkout our sample appβ
Visit our Vue sample app repo to run the Vue sample app.