Overview
Our React Chat UI Kit lets developers easily add text, voice & video to your website. It a fully polished UI and the complete business logic.
Don't forget to check out the Key Concepts for your React Chat UI Kit before proceeding.
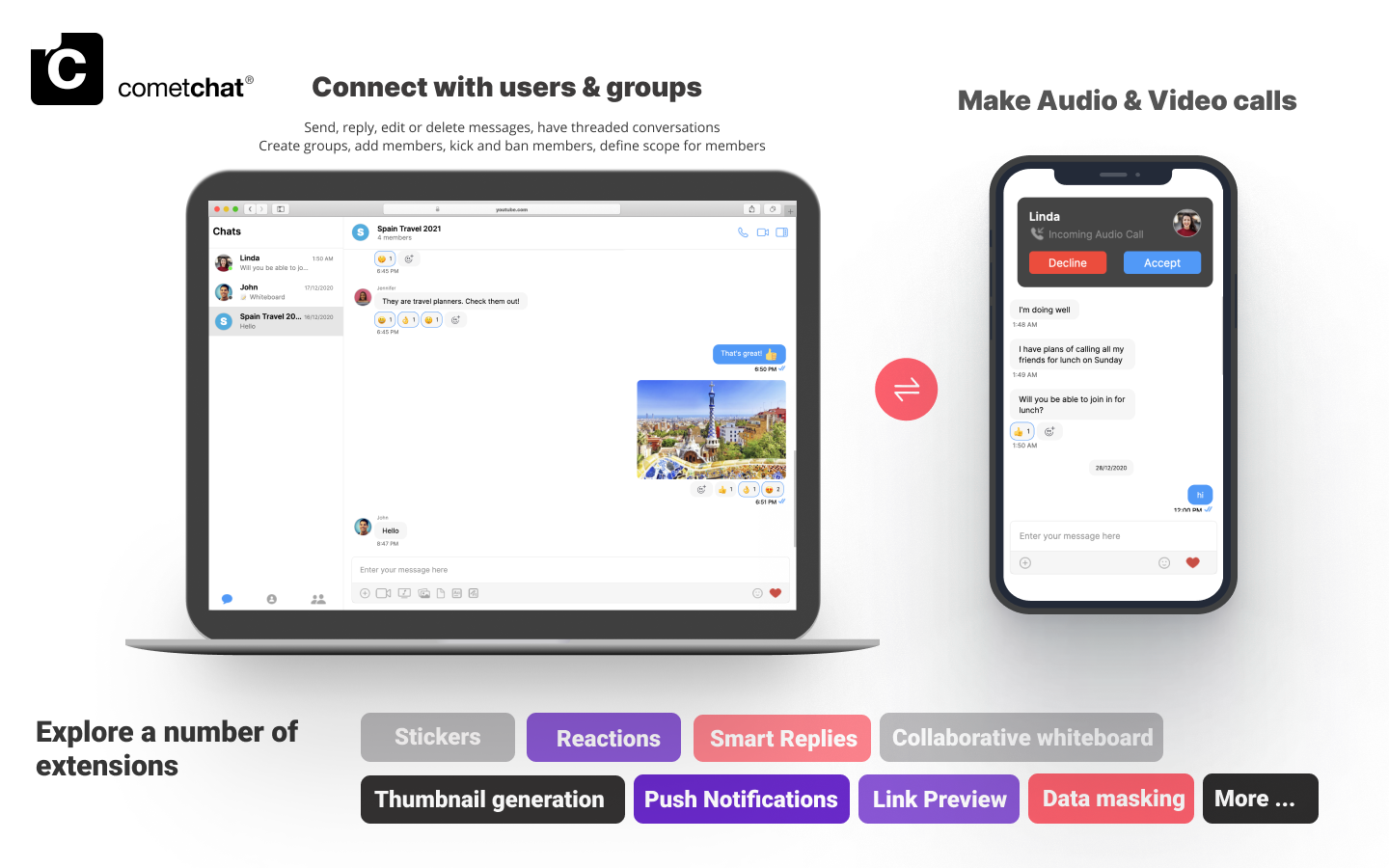
- Seamless scaling to over 1M+ concurrent users
- Faster connection & response times
- Higher rate limits
- Supports up to 100K users in a group
- Unlimited groups
- Support for Transient Messages
- Real-time user & group members count
- And more!
CometChat's React UI Kit’s customizable UI components simplify the process of integrating text chat and voice/video calling features to your website or mobile application in a few minutes.
I want to checkout React UI Kit
Follow the steps mentioned in the README.md
file.
Kindly, click on below button to download our React UI Kit.
React UI KitView on GithubI want to explore the sample app
Kindly, click on below button to download our React Sample App.
React Sample AppView on GithubPrerequisites
Before you begin, ensure you have met the following requirements:
- CLI
npm install react@17.0.2
- CLI
npm install react-dom@17.0.2
- CLI
npm install react-scripts@4.0.3
Installing the React Chat UI Kit
Please follow the steps provided in the Key Concepts to create V3 apps before you proceed.
Setup
-
Register on CometChat
- To install React UI Kit, you need to first register on the CometChat Dashboard. Click here to Sign Up.
-
Get your application keys
- Create a new app.
- Head over to the QuickStart or API & Auth Keys section and note the App ID, Auth Key, and Region.
-
Add the CometChat dependency
- CLI
npm install @cometchat-pro/chat@3.0.11 --save
Configure CometChat inside your app
- Import CometChat SDK
- Javascript
import { CometChat } from "@cometchat-pro/chat";
- Initialize CometChat
The init() method initializes the settings required for CometChat. We suggest calling the init() method on app startup, preferably in the index.js file.
- Javascript
const appID = "APP_ID";
const region = "REGION";
const appSetting = new CometChat.AppSettingsBuilder().subscribePresenceForAllUsers().setRegion(region).build();
CometChat.init(appID, appSetting).then(
() => {
console.log("Initialization completed successfully");
// You can now call login function.
},
error => {
console.log("Initialization failed with error:", error);
// Check the reason for error and take appropriate action.
}
);
Replace APP_ID and REGION with your CometChat App ID and Region in the above code.
- Create user
This method takes a User
object and the Auth Key
as input parameters and returns the created User
object if the request is successful.
- Javascript
let authKey = "AUTH_KEY";
var uid = "user1";
var name = "Kevin";
var user = new CometChat.User(uid);
user.setName(name);
CometChat.createUser(user, authKey).then(
user => {
console.log("user created", user);
},error => {
console.log("error", error);
}
)
Replace AUTH_KEY with your CometChat Auth Key in the above code.
- Login your user
This method takes UID
and Auth Key
as input parameters and returns the User object containing all the information of the logged-in user.
- Javascript
const authKey = "AUTH_KEY";
const uid = "cometchat-uid-1";
CometChat.login(uid, authKey).then(
user => {
console.log("Login Successful:", { user });
},
error => {
console.log("Login failed with exception:", { error });
}
);
Replace AUTH_KEY with your CometChat Auth Key in the above code.
We have set up 5 users for testing having UIDs: cometchat-uid-1, cometchat-uid-2, cometchat-uid-3, cometchat-uid-4, and cometchat-uid-5.
We have used uid cometchat-uid-1 as an example here. You can create a User from CometChat Dashboard as well.
Add the React UI Kit to your project
- Clone this repository
- CLI
git clone https://github.com/cometchat-pro/cometchat-pro-react-ui-kit.git
- Copy the cloned repository to your source folder
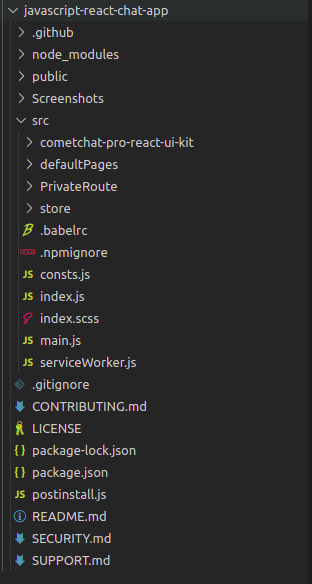
- Copy all the dependencies from package.json into your project's package.json and install them
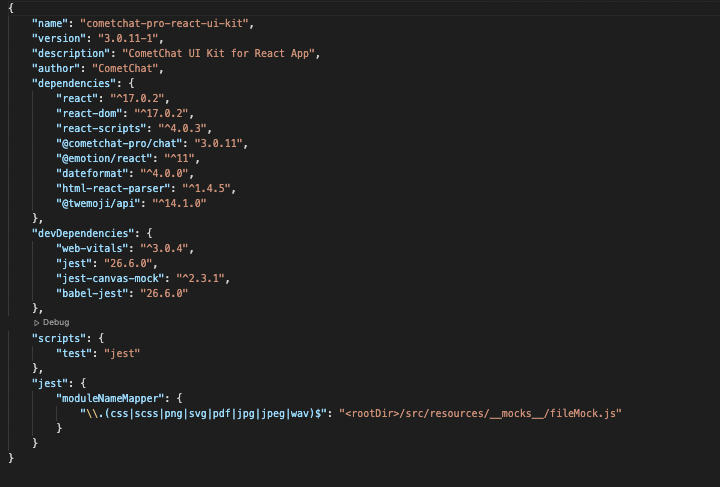
Launch CometChat
Using the CometChatUI component, you can launch a fully functional chat application. In this component, all the UI Screens and UI Components are interlinked and work together to launch a fully functional chat on your website/application.
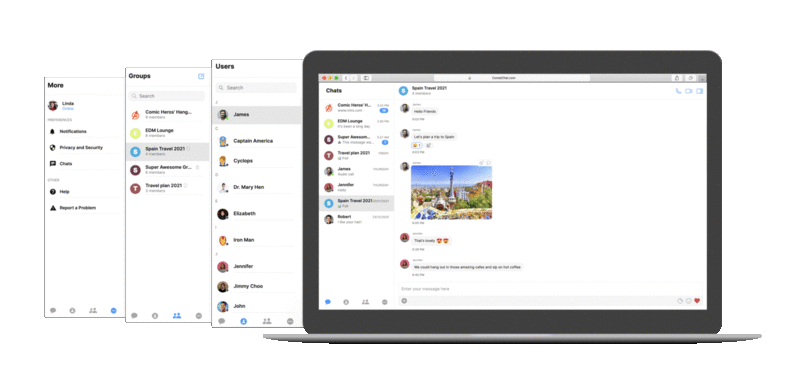
Usage
- React
import { CometChatUI } from "./CometChatWorkspace/src";
class App extends React.Component {
render() {
return (
<div style={{width: '800px', height:'800px' }}>
<CometChatUI />
</div>
);
}
}
Check out our React chat sample app
Visit our React sample app repository to run the sample app yourself.