Outgoing Call
Overview
The CometChatOutgoingCall
Widget is a visual representation of a user-initiated call, whether it's a voice or video call. It serves as an interface for managing outgoing calls, providing users with essential options to control the call experience. This Widget typically includes information about the call recipient, call controls for canceling the call, and feedback on the call status, such as indicating when the call is in progress.
- Android
- iOS
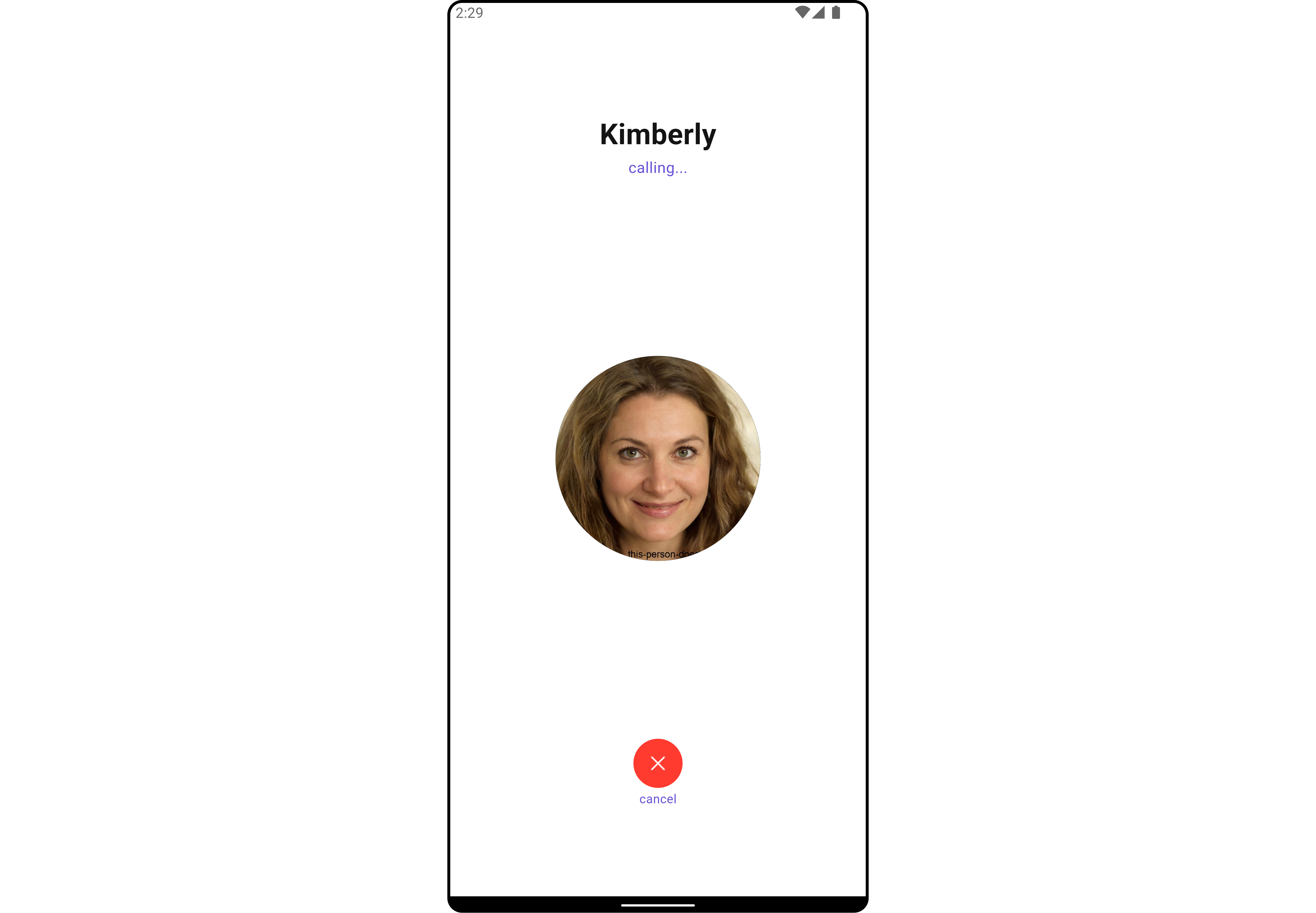
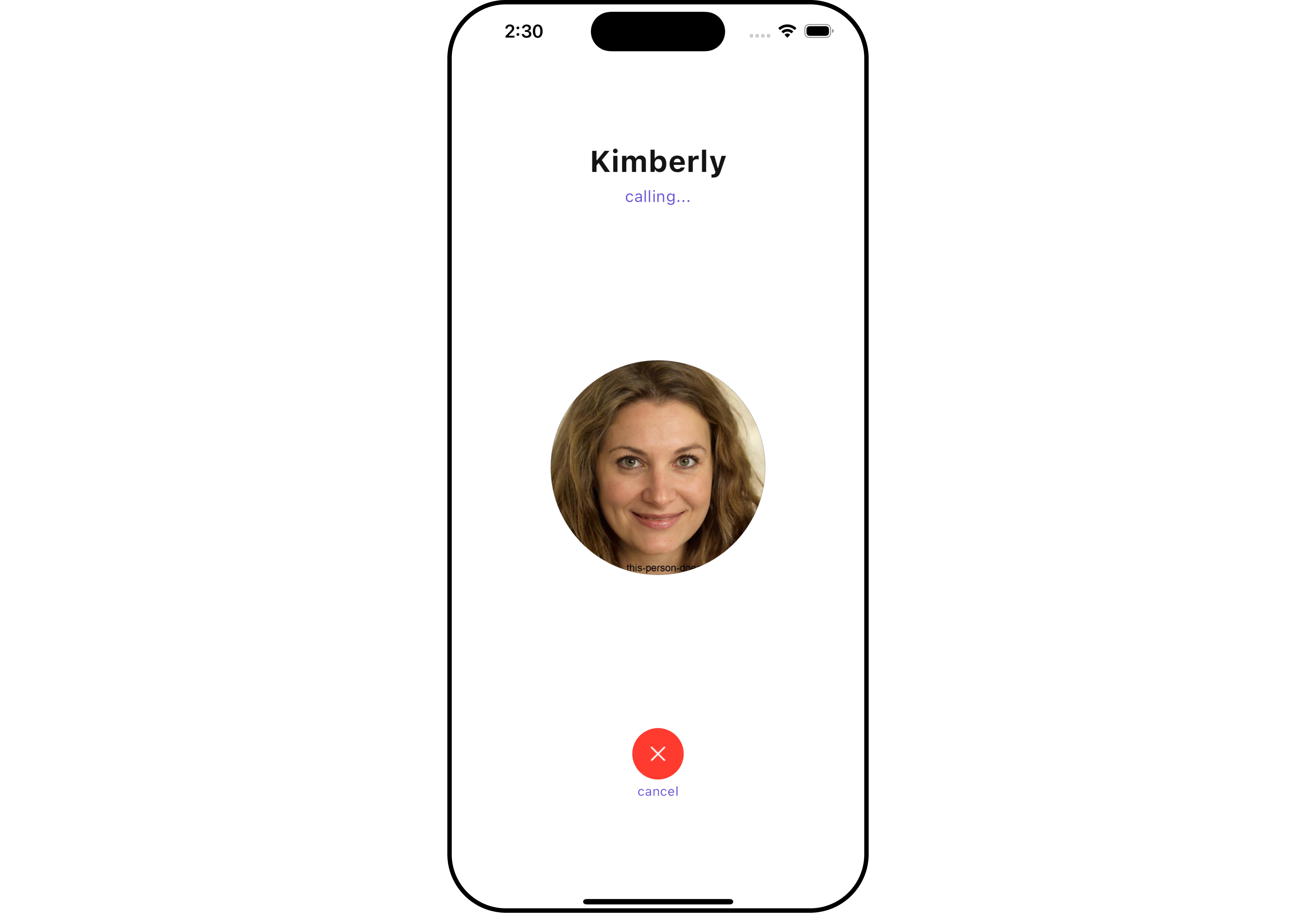
You can launch CometChatOutgoingCall
directly using Navigator.push
, or you can define it as a widget within the build
method of your State
class.
1. Using Navigator to Launch CometChatOutgoingCall
- Dart
Navigator.push(context, MaterialPageRoute(builder: (context) => CometChatOutgoingCall(user: user, call: callObject))); // User object and Call object is required to launch the incoming call widget.
2. Embedding CometChatOutgoingCall
as a Widget in the build Method
- Dart
import 'package:cometchat_calls_uikit/cometchat_calls_uikit.dart';
import 'package:flutter/material.dart';
class OutgoingCallExample extends StatefulWidget {
const OutgoingCallExample({super.key});
State<OutgoingCallExample> createState() => _OutgoingCallExampleState();
}
class _OutgoingCallExampleState extends State<OutgoingCallExample> {
Widget build(BuildContext context) {
return Scaffold(
body: SafeArea(
child: CometChatOutgoingCall(
user: user, // User Object
call: callObject
), // User object and Call object is required to launch the incoming call widget.
),
);
}
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onDecline
The onDecline
action is typically triggered when the call is ended, carrying out default actions. However, with the following code snippet, you can effortlessly customize or override this default behavior to meet your specific needs.
- Dart
CometChatOutgoingCall(
user: user, // User Object
call: callObject, // Call Object
onDecline: (BuildContext context, Call call) {
// TODO("Not yet implemented")
},
)
2. onError
You can customize this behavior by using the provided code snippet to override the onError
and improve error handling.
- Dart
CometChatOutgoingCall(
user: user, // User Object
call: callObject, // Call Object
onError: (e) {
// TODO("Not yet implemented")
},
)