Integration
SMS Notifications integration is possible using Twilio as a provider or a custom provider. With Custom SMS provider, you can integrate using SMS providers other than Twilio.
Twilio
We have partnered with Twilio for sending SMS Notifications so need to set up an account on Twilio before you start using the extension.
Create a new App on Twilio
- Once you log in to Twilio, create a new app.
- Make a note of Account SID and Auth Token for later use.
- Click on "Get a Trial number" to get the Sender number. (Use the paid number if you already have one)
- Make a note of the sender's phone number for later use.
Store contact details
Store the phone number of your users by using our Update Contact details API.
Enable SMS Notifications
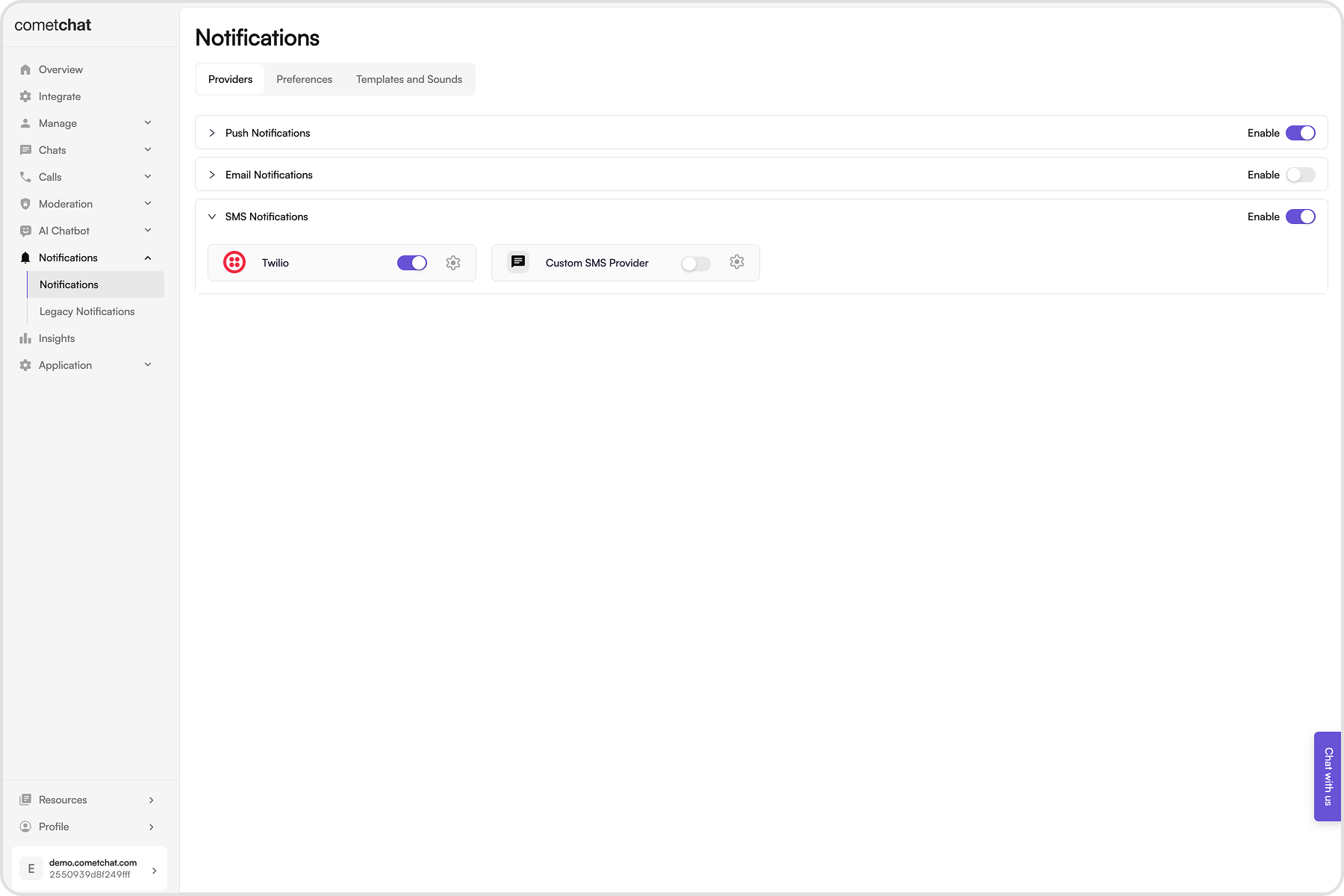
- Login to CometChat dashboard and select your app.
- Navigate to Notifications > Notifications in the left-hand menu.
- Enable SMS notifications feature.
Save Twilio credentials
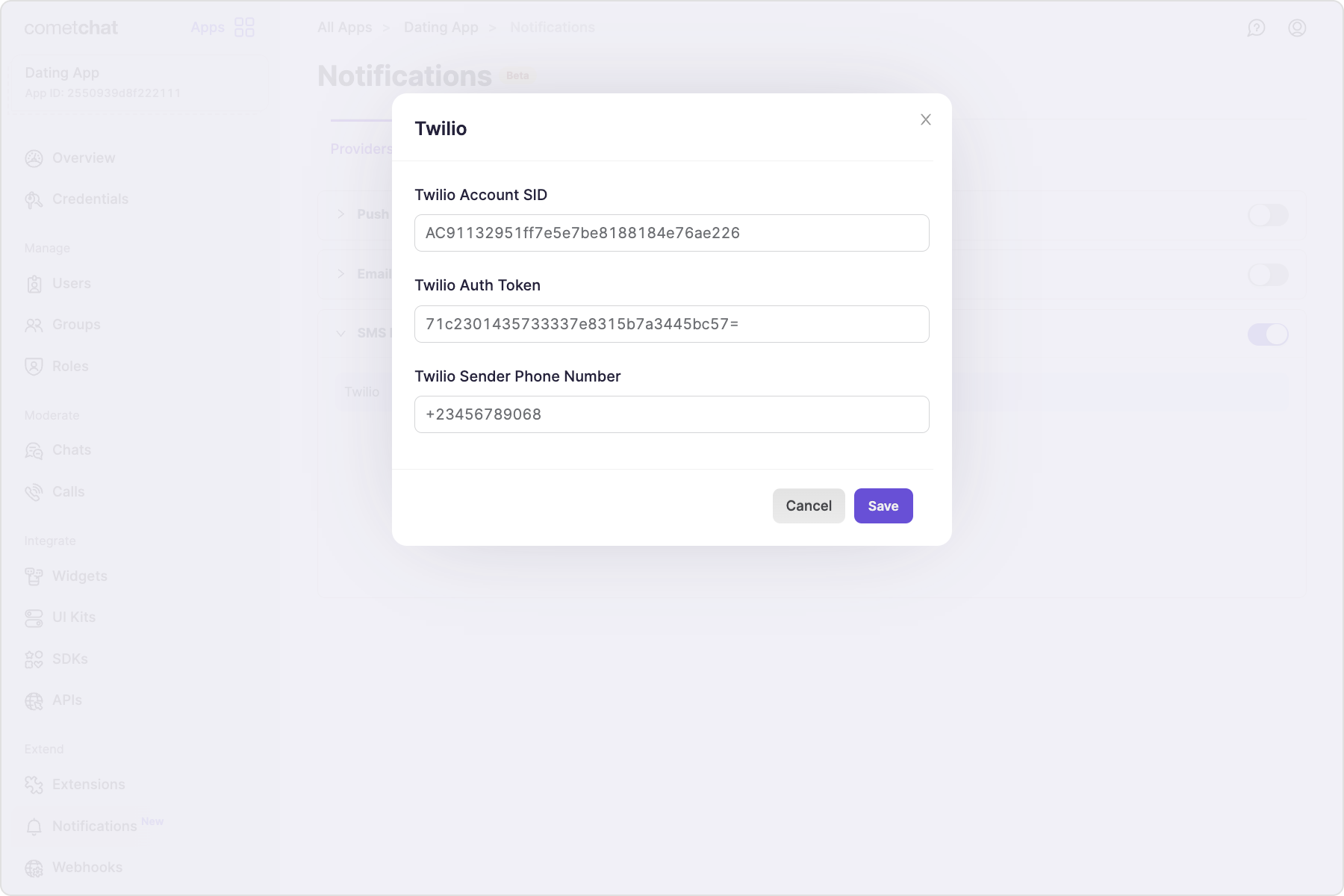
Save the following details:
- Twilio Account SID
- Twilio Auth token
- Twilio sender phone number
Save user's timezone
A user's timezone is required to allow them to set a schedule for receiving notifications. In case the timezone is not registered, the default timezone for
- For US region: EST
- For EU region: GMT
- For IN region: Asia/Kolkata
The timezone can be registered for a user from the SDK using the updateTimezone()
method of CometChatNotifications
class.
This functionality is available in the following SDK versions:
- Android SDK version 4.0.9 and above
- iOS SDK version 4.0.51 and above
- Web SDK version 4.0.8 and above
- React Native SDK version 4.0.10 and above
- Ionic Cordova SDK version 4.0.8 and above
- Flutter SDK version 4.0.15 and above
Receive notifications
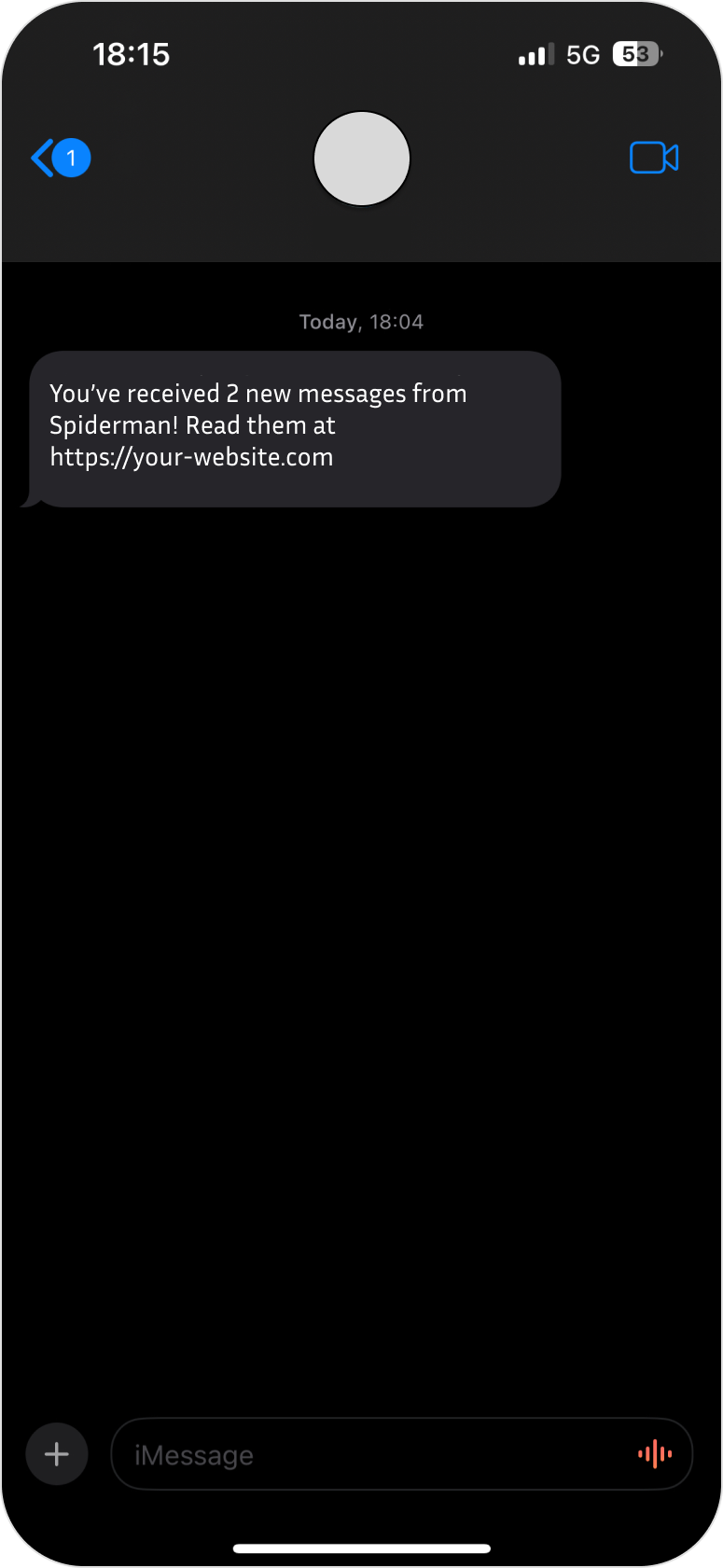
Send a message to any user and keep the conversation unread for the designated amount of time to receive an SMS notification.
Custom SMS provider
Custom provider allows you to make use of providers apart from Twilio for triggering SMS notifications. This is implemented using webhook URL which gets all the required details that can be used to trigger SMS notifications.
Pre-requisite
- Your webhook endpoint must be accessible over
HTTPS
. This is essential to ensure the security and integrity of data transmission. - This URL should be publicly accessible from the internet.
- Ensure that your endpoint supports the
HTTP POST
method. Event payloads will be delivered viaHTTP POST
requests inJSON
format. - Configure your endpoint to respond immediately to the CometChat server with a 200 OK response. The response should be sent within 2 seconds of receiving the request.
- For security, it is recommended to set up Basic Authentication that is usually used for server-to-server calls. This requires you to configure a username and password. Whenever your webhook URL is triggered, the HTTP Header will contain:
Authorization: Basic <Base64-encoded-credentials>
Add credentials
- Click on the "+ Add Credentials" button.
- Enable the provider.
- Enter the publically accessible Webhook URL.
- It is recommended to enable Basic Authentication.
- Enter the username and password.
- Enabling the "Trigger only if phone number is stored with CometChat" setting requires users' phone numbers to be stored with CometChat using the Update Contact details API. When enabled, the webhook is triggered only for those users. If this setting is disabled, the webhook triggers regardless of whether users' phone numbers are stored with CometChat.
- Save the credentials.
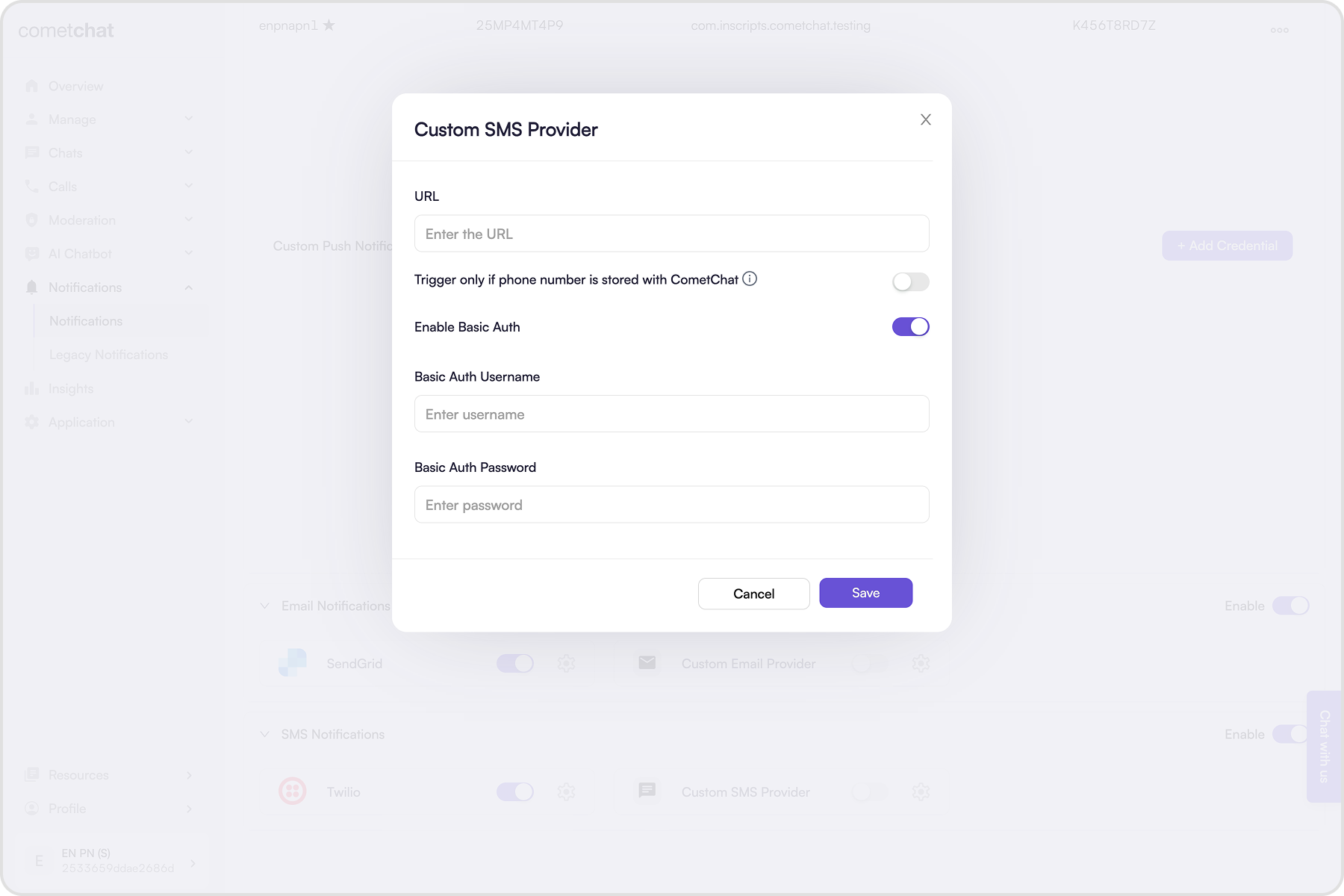
How does it work?
The Custom provider is triggered once for an event in one-on-one conversation. In case of notifying users in a group, the custom provider is triggered once for each user present in that group.
- One-on-one conversation
- Group conversation
{
"trigger": "sms-notification-payload-generated",
"data": {
"to": {
"uid": "cometchat-uid-1",
"phno": "+919299334134", // Optional
"name": "Andrew Joseph"
},
"messages": [
{
"sender": {
"uid": "cometchat-uid-4",
"avatar": "https://assets.cometchat.io/sampleapp/v2/users/cometchat-uid-4.webp",
"name": "Susan Marie"
},
"message": "Are we meeting on this weekend?"
},
{
"sender": {
"uid": "cometchat-uid-4",
"avatar": "https://assets.cometchat.io/sampleapp/v2/users/cometchat-uid-4.webp",
"name": "Susan Marie"
},
"message": "📷 Has shared an image"
}
],
"senderDetails": {
"uid": "cometchat-uid-4",
"name": "Susan Marie",
"avatar": "https://assets.cometchat.io/sampleapp/v2/users/cometchat-uid-4.webp"
},
"smsContent": "You've received new messages from Susan Marie! Read them at https://your-website.com/chat."
},
"appId": "app123",
"region": "us/eu/in",
"webhook": "custom"
}
{
"trigger": "sms-notification-payload-generated",
"data": {
"to": {
"uid": "cometchat-uid-1",
"phno": "+919299334134", // Optional
"name": "Andrew Joseph"
},
"messages": [
{
"sender": {
"uid": "cometchat-uid-5",
"avatar": "https://assets.cometchat.io/sampleapp/v2/users/cometchat-uid-5.webp",
"name": "John Paul"
},
"message": "Hello all! What's up?"
},
{
"sender": {
"uid": "cometchat-uid-4",
"avatar": "https://assets.cometchat.io/sampleapp/v2/users/cometchat-uid-4.webp",
"name": "Susan Marie"
},
"message": "This is the place I was thinking about"
}
],
"groupDetails": {
"guid": "cometchat-guid-1",
"name": "Hiking Group",
"icon": "https://assets.cometchat.io/sampleapp/v2/groups/cometchat-guid-1.webp"
},
"smsContent": "You've received new messages in Hiking Group! Read them at https://your-website.com."
},
"appId": "app123",
"region": "us/eu/in",
"webhook": "custom"
}
Sample server-side code
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(express.json());
// Optional: Basic authentication middleware
const basicAuth = (req, res, next) => {
const authHeader = req.headers['authorization'];
if (!authHeader || !authHeader.startsWith('Basic ')) {
return res.status(401).json({ message: 'Unauthorized' });
}
next();
};
const triggerSMSNotification = async (to, data) => {
let { name, uid, phno } = to;
let { groupDetails, senderDetails, smsContent } = data;
if (groupDetails) {
console.log('Received webhook for group SMS notification');
}
if (senderDetails) {
console.log('Received webhook for one-on-one SMS notification');
}
if (phno == null) {
// Your implementation to fetch Phone number
phno = await fetchPhoneNumberFor(uid);
}
// Your implementation for sending the SMS notification
await sendSMS(phno, smsContent);
};
app.post('/webhook', basicAuth, (req, res) => {
const { trigger, data, appId, region, webhook } = req.body;
if (
trigger !== 'sms-notification-payload-generated' ||
webhook !== 'custom'
) {
return res.status(400).json({ message: 'Invalid trigger or webhook type' });
}
console.log('Received Webhook:', JSON.stringify(req.body, null, 2));
triggerSMSNotification(to, data)
.then((result) => {
console.log(
'Successfully triggered SMS notification for',
appId,
to.uid,
result
);
})
.catch((error) => {
console.error(
'Something went wrong while triggering SMS notification for',
appId,
to.uid,
error.message
);
});
res.status(200).json({ message: 'Webhook received successfully' });
});
app.listen(PORT, () => {
console.log(`Server is running on port ${PORT}`);
});
Next steps
Have a look at the available preferences and templates for SMS notifications.