Integration
Enable Push notifications
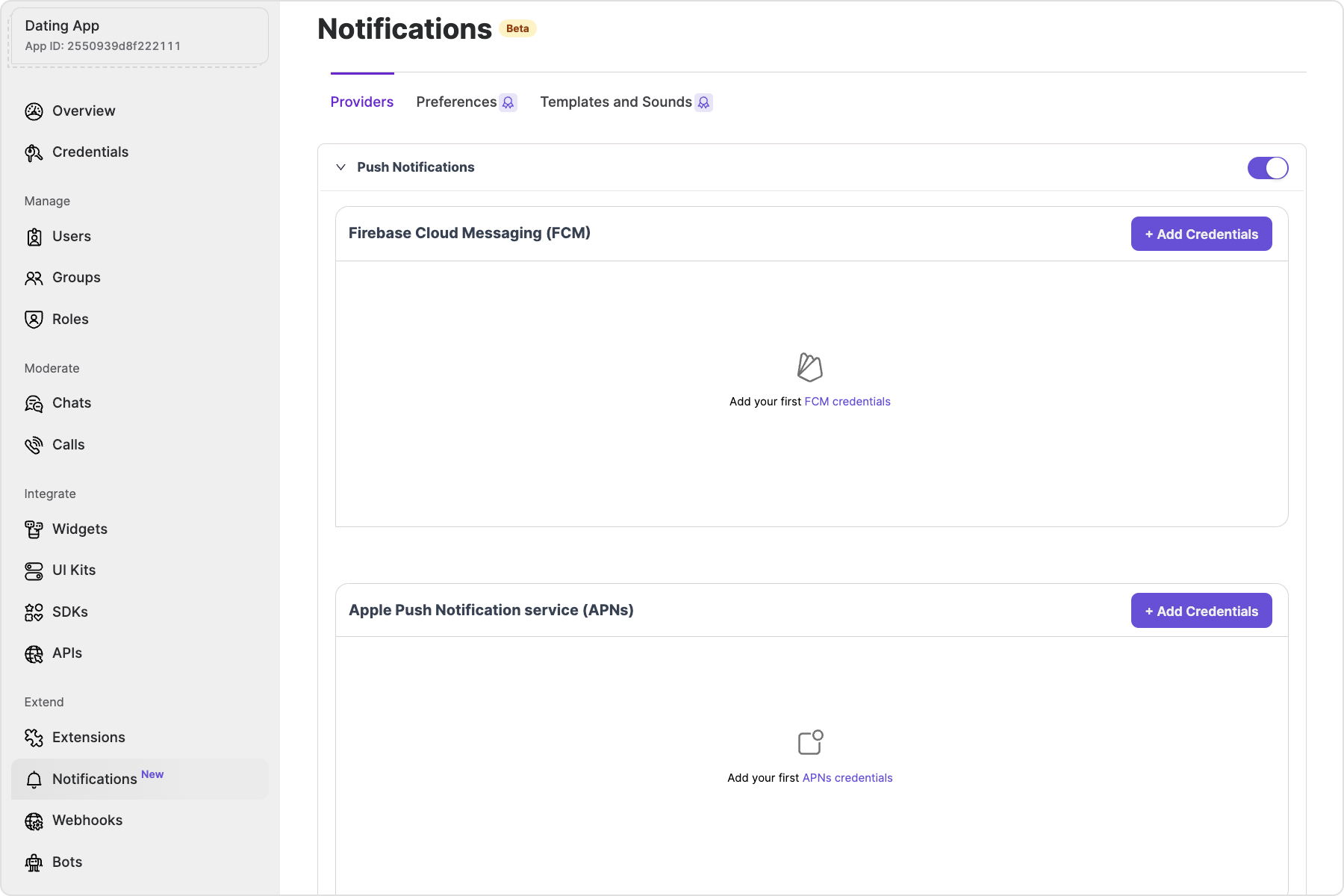
- Sign in to the CometChat dashboard.
- In the right navigation pane, choose "Notifications" from the "Extend" category.
- Enable the Push notifications feature.
- Continue to configure Push notifications by clicking on "Configure".
Add Providers
Firebase Cloud Messaging (FCM) and Apple Push Notification Service (APNS) are the two primary supported providers for sending push notifications.
Add FCM credentials
Pre-requisite
Generate a service account key for your Firebase application by navigating to the "Service accounts" section within the "Project settings" of the Firebase Cloud Messaging Dashboard. Generate new private key and download the JSON file. This will be required in the next steps.
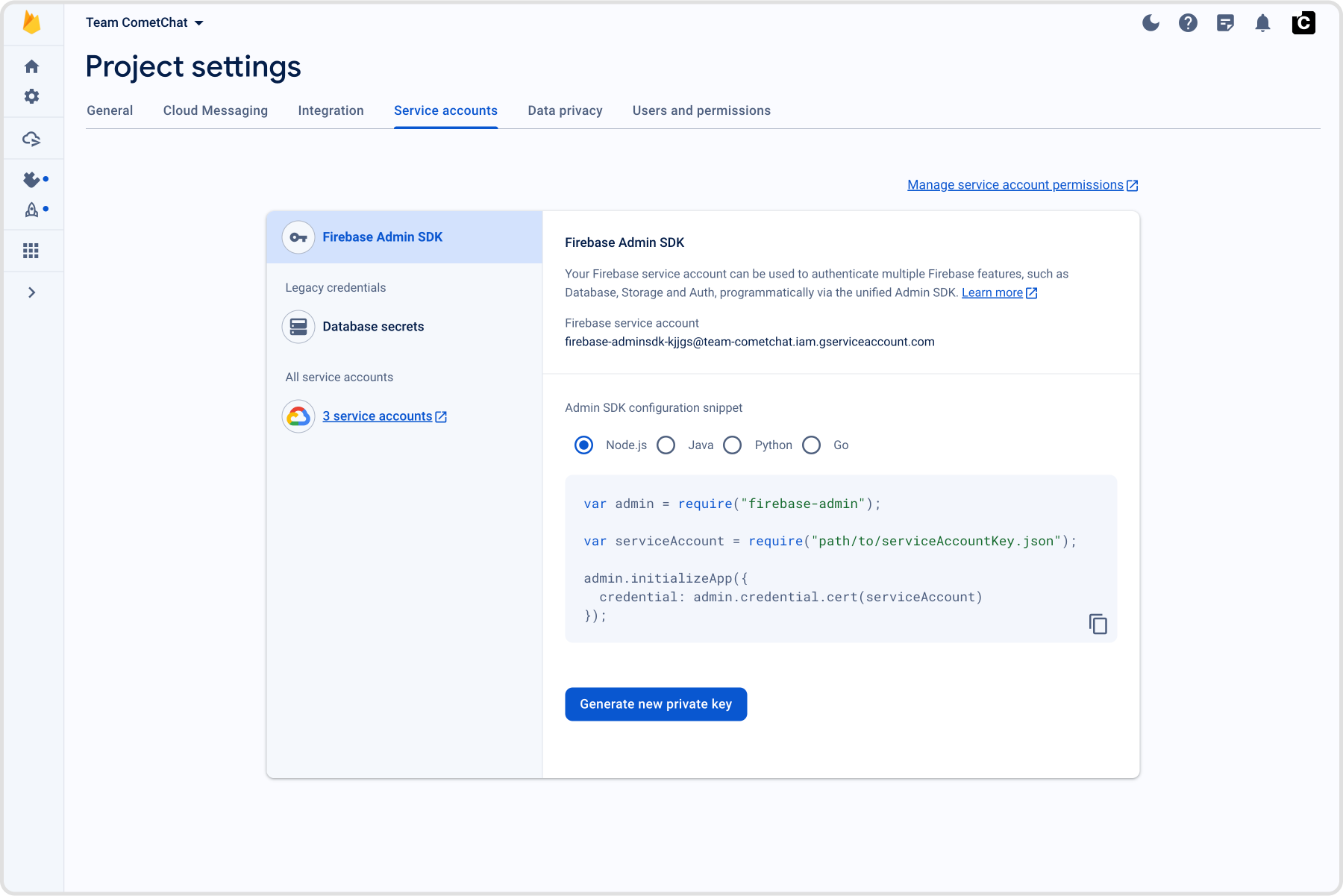
Add credentials
- Select the "+ Add Credentials" button.
- In the dialogue that appears, provide a unique, memorable identifier for your provider.
- Upload the service account JSON file you previously acquired.
- Specify whether the push payload should include the "notification" key. Additional information on this configuration is available in FCM's documentation - About FCM messages.
Similarly, you can add multiple FCM Credentials in case you have multiple FCM projects for your apps.
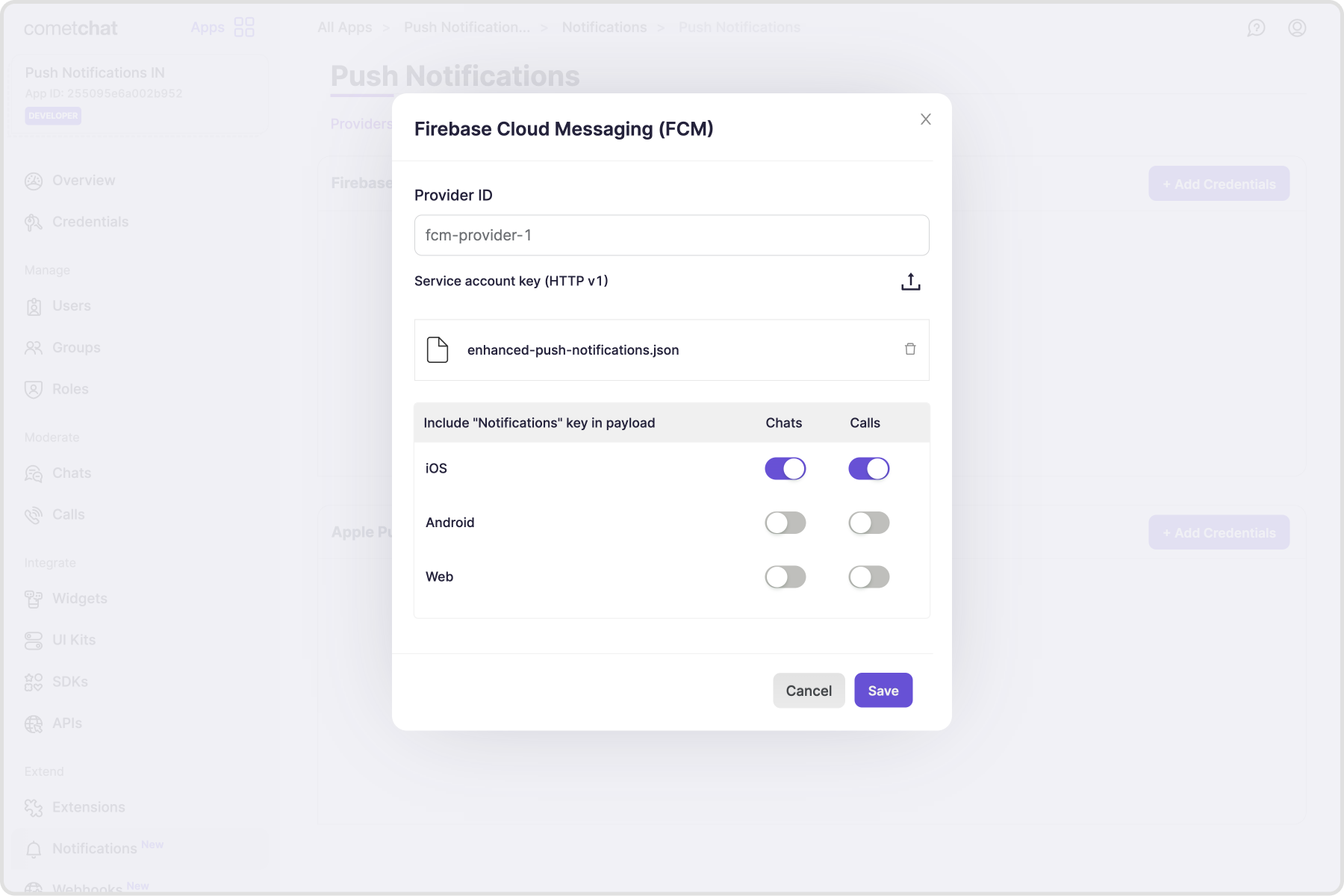
Add APNS credentials
Pre-requisite
- To generate a .p8 key file, go to Apple developer account, then select "Certificates, IDs & Profiles".
- Select Keys and click on the "+" button to add a new key.
- In the new key page, type in your key name and check the Apple Push Notification service (APNs) box, then click "Continue" and click "Register".
- Then proceed to download the key file by clicking Download.
- Make note of the Key ID, Team ID and your Bundle ID. These are required in the next steps.
Additional information on this configuration is available in Apple's documentation - Create a private key to access a service
Add credentials
- Select the "+ Add Credentials" button.
- Enable the toggle if your app is in the Production. For apps under development, the toggle has to be disabled.
- In the dialogue that appears, provide a unique, memorable identifier for your provider.
- Store the Key ID, Team ID, Bundle ID for your app.
- Upload the .p8 file
- Enable "Include content-available" if you want to receive background notifications. However, this is not recommended as the background notifications are throttled. Additional information is available in Apple's documentation - Pushing background updates to your App
- Enable "Include mutable-content" if you want to modify the notification before it is displayed to the user. Additional information is available in Apple's documentation - Modifying content on newly delivered notifications
Similarly, you can add multiple APNS Credentials in case you have multiple apps with different Bundle IDs.
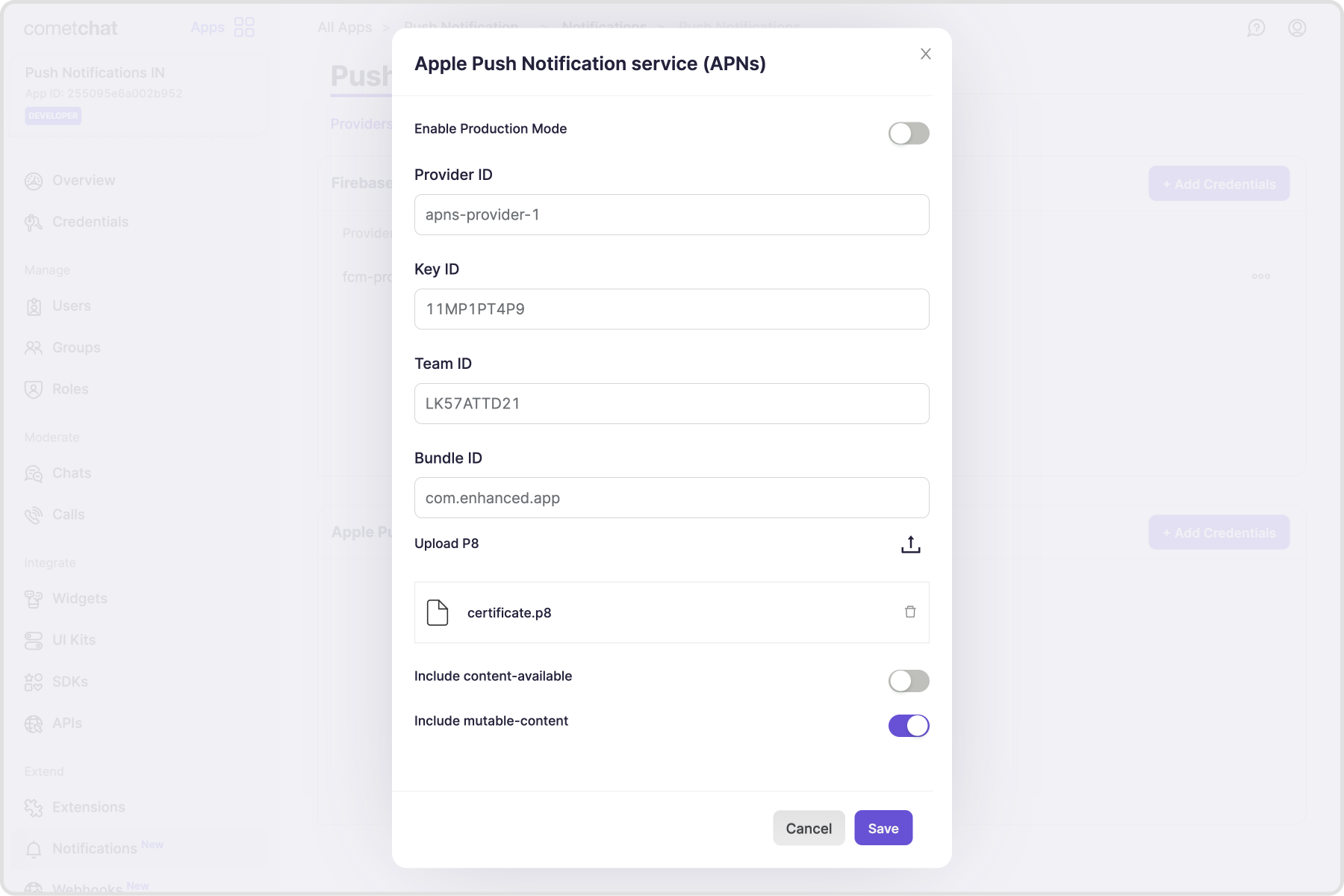
Tokens management
Pre-requisite
To successfully integrate push notifications in your app for any particular platform, you need to make sure you are using the appropriate version of the CometChat SDK that supports push notifications.
- Android SDK version 4.0.9 and above
- iOS SDK version 4.0.51 and above
- Web SDK version 4.0.8 and above
- React Native SDK version 4.0.10 and above
- Ionic Cordova SDK version 4.0.8 and above
- Flutter SDK version 4.0.15 and above
Register push token after login
Push tokens, obtained from the APIs or SDKs provided by the platforms or frameworks in use, must be registered on behalf of the logged in user with the CometChat backend. For this purpose, CometChat SDKs v4+ offer the following functions:
Push token registration should be completed in two scenarios:
- Following the success of
CometChat.login()
& receiving user's permission to receiver Push notifications. - When a refresh token becomes available.
To register a token, use the CometChatNotifications.registerPushToken()
method from the SDK. This method accepts the following parameters.
Parameter | Type | Description |
---|---|---|
pushToken | String | The
|
platform | String | The
|
providerId | String | The
|
- JavaScript
- Android
- iOS
- Flutter
// This is applicable for web, React native, Ionic cordova
// CometChat.init() success.
// CometChat.login() success.
// User has granted permission to display push notifications.
// For web
CometChatNotifications.registerPushToken(
pushToken,
CometChatNotifications.PushPlatforms.FCM_WEB,
'fcm-provider-2'
)
.then((payload) => {
console.log('Token registration successful');
})
.catch((err) => {
console.log('Token registration failed:', err);
});
// For React Native Android
CometChatNotifications.registerPushToken(
pushToken,
CometChatNotifications.PushPlatforms.FCM_REACT_NATIVE_ANDROID,
'fcm-provider-2'
)
.then((payload) => {
console.log('Token registration successful');
})
.catch((err) => {
console.log('Token registration failed:', err);
});
// For React Native iOS
CometChatNotifications.registerPushToken(
pushToken,
CometChatNotifications.PushPlatforms.FCM_REACT_NATIVE_IOS,
'fcm-provider-2'
)
.then((payload) => {
console.log('Token registration successful');
})
.catch((err) => {
console.log('Token registration failed:', err);
});
// For Ionic cordova Android
CometChatNotifications.registerPushToken(
pushToken,
CometChatNotifications.PushPlatforms.FCM_IONIC_CORDOVA_ANDROID,
'fcm-provider-2'
)
.then((payload) => {
console.log('Token registration successful');
})
.catch((err) => {
console.log('Token registration failed:', err);
});
// For Ionic cordova iOS
CometChatNotifications.registerPushToken(
pushToken,
CometChatNotifications.PushPlatforms.FCM_IONIC_CORDOVA_IOS,
'fcm-provider-2'
)
.then((payload) => {
console.log('Token registration successful');
})
.catch((err) => {
console.log('Token registration failed:', err);
});
// Similary, use this method to register refresh token.
// CometChat.init() success.
// CometChat.login() success.
// User has granted permission to display push notifications.
CometChatNotifications.registerPushToken(pushToken, PushPlatforms.FCM_ANDROID, "fcm-provider-2", new CometChat.CallbackListener<String>() {
@Override
public void onSuccess(String s) {
Log.e(TAG, "onSuccess: CometChat Notification Registered : "+s );
listener.onSuccess(s);
}
@Override
public void onError(CometChatException e) {
Log.e(TAG, "onError: Notification Registration Failed : "+e.getMessage());
listener.onError(e);
}
});
// Similary, use this method to register refresh token.
// CometChat.init() success.
// CometChat.login() success.
// User has granted permission to display push notifications.
CometChatNotifications.registerPushToken(pushToken: pushToken, platform: CometChatNotifications.PushPlatforms.FCM_IOS, providerId: "apns-provider-2", onSuccess: { (success) in
print("registerPushToken: \(success)")
}) { (error) in
print("registerPushToken: \(error.errorCode) \(error.errorDescription)")
}
// Similary, use this method to register refresh token.
// CometChat.init() success.
// CometChat.login() success.
// User has granted permission to display push notifications.
// For Android (FCM)
CometChatNotifications.registerPushToken(
PushPlatforms.FCM_FLUTTER_ANDROID,
providerId: "fcm-provider-1",
fcmToken: token,
onSuccess: (response) {
debugPrint("registerPushToken:success ${response.toString()}");
},
onError: (e) {
debugPrint("registerPushToken:error ${e.toString()}");
},
);
// For iOS (FCM)
CometChatNotifications.registerPushToken(
PushPlatforms.FCM_FLUTTER_IOS,
providerId: "fcm-provider-1",
fcmToken: token,
onSuccess: (response) {
debugPrint("registerPushToken:success ${response.toString()}");
},
onError: (e) {
debugPrint("registerPushToken:error ${e.toString()}");
},
);
// For ios (APNS Device token)
CometChatNotifications.registerPushToken(
PushPlatforms.APNS_FLUTTER_DEVICE,
providerId: "apns-provider-1",
deviceToken: token,
onSuccess: (response) {
debugPrint("registerPushToken:success ${response.toString()}");
},
onError: (e) {
debugPrint("registerPushToken:error ${e.toString()}");
},
);
// For ios (APNS VoIP token)
CometChatNotifications.registerPushToken(
PushPlatforms.APNS_FLUTTER_VOIP,
providerId: "apns-provider-1",
voipToken: token,
onSuccess: (response) {
debugPrint("registerPushToken:success ${response.toString()}");
},
onError: (e) {
debugPrint("registerPushToken:error ${e.toString()}");
},
);
// Similary, use this method to register refresh token.
Unregister push token before logout
Typically, push token unregistration should occur prior to user logout, using the CometChat.logout()
method.
For token unregistration, use the CometChatNotifications.unregisterPushToken()
method provided by the SDKs.
- JavaScript
- Android
- iOS
- Flutter
// This is applicable for web, React native, Ionic cordova
await CometChatNotifications.unregisterPushToken();
// Followed by CometChat.logout();
CometChatNotifications.unregisterPushToken(new CometChat.CallbackListener<String>() {
@Override
public void onSuccess(String s) {
// Success callback
}
@Override
public void onError(CometChatException e) {
// Error callback
}
});
// Followed by CometChat.logout();
CometChatNotifications.unregisterPushToken { success in
print("unregisterPushToken: \(success)")
} onError: { error in
print("unregisterPushToken: \(error.errorCode) \(error.errorDescription)")
}
// Followed by CometChat.logout();
CometChatNotifications.unregisterPushToken(onSuccess: (response) {
debugPrint("unregisterPushToken:success ${response.toString()}");
}, onError: (e) {
debugPrint("unregisterPushToken:error ${e.toString()}");
});
Handle incoming Push notifications
Push notifications should be managed primarily when the app is in the background or in a terminated state.
For web and mobile applications, the Firebase Cloud Messaging (FCM) SDK offers handler functions to receive Push Notifications.
For iOS applications, the FCM SDK can be used as described previously, or alternatively, PushKit can be implemented for enhanced integration and management of chat and call (VoIP) notifications.
The push payload delivered to the user's device includes the following information, which can be customized to suit the desired notification style:
- Details available in the payload
{
// Notification details
"title": "Andrew Joseph", // The title of the notification to be displayed
"body": "Hello!", // The body of the notification to be displayed
// Sender's details
"sender": "cometchat-uid-1", // UID of the user who sent the said message.
"senderName": "Andrew Joseph", // Name of the user who sent the said message.
"senderAvatar": "https://assets.cometchat.io/sampleapp/v2/users/cometchat-uid-1.webp", // Avatar URL of the user.
// Receiver's details
"receiver": "cometchat-uid-2", // UID or GUID of the receiver.
"receiverName": "George Alan", // Name of the user or group.
"receiverAvatar": "https://assets.cometchat.io/sampleapp/v2/users/cometchat-uid-2.webp", // Avatar URL of the receiver.
"receiverType": "user", // Values can be "user" or "group"
// Message details
"tag": "123", // The ID of the said message that can be used as the ID of the notification to be displayed.
"conversationId": "cometchat-uid-1_user_cometchat-uid-2", // The ID of the conversation that the said message belongs to.
"type": "chat", // Values can be "call" or "chat". If this is "call", the below details will be available.
// Call details
"callAction": "initiated", // Values can be "initiated" or "cancelled" or "unanswered" or "ongoing" or "rejected" or "ended" or "busy"
"sessionId": "v1.123.aik2", // The unique sessionId of the said call that can be used as unique identifier in CallKit or ConnectionService.
"callType": "audio", // Values can be "audio" or "video"
}
Sample apps
Check out our sample apps for understanding the implementation.
React - Enhanced Push notifications sample app
Push notifications sample app for the web
View on GithubReact Native - Enhanced Push notifications sample app
Push notifications sample app for React Native
View on GithubAndroid - Enhanced Push notifications sample app
Push notifications sample app for Android
View on GithubFlutter - Enhanced Push notifications sample app
Push notifications sample app for Flutter
View on Github