Call Buttons
Overview
The Call Button
is a Component provides users with the ability to make calls, access call-related functionalities, and control call settings. Clicking this button typically triggers the call to be placed to the desired recipient.
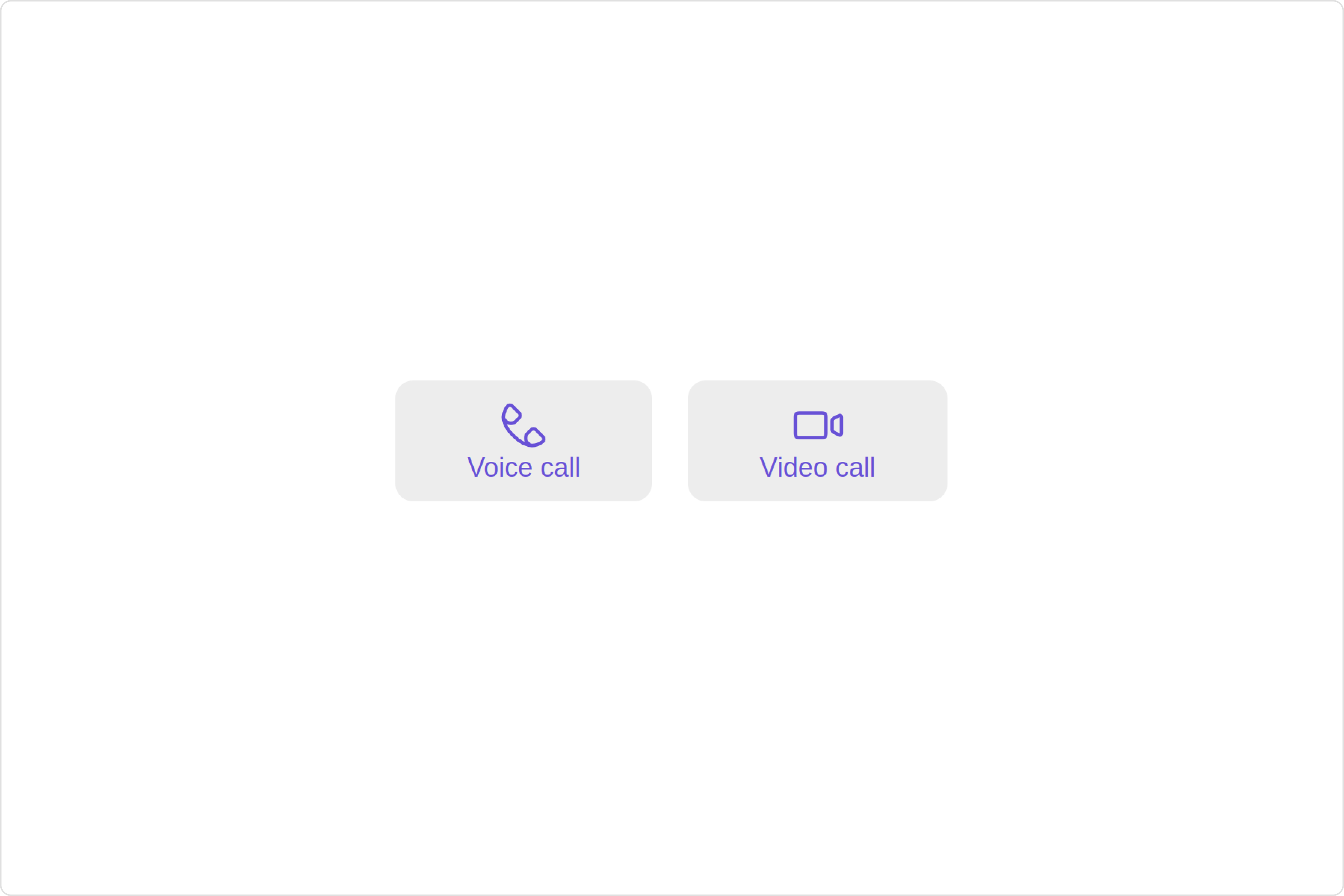
Usage
Integration
- CallButtonDemo.tsx
- App.tsx
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
import React from "react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("richard-uid").then((user) => {
setChatUser(user);
});
}, []);
return <CometChatCallButtons user={chatUser} />;
};
export default CallButtonDemo;
import { CallButtonDemo } from "./CallButtonDemo";
export default function App() {
return (
<div className="App">
<div>
<CallButtonDemo />
</div>
</div>
);
}
Actions
Actions dictate how a component functions. They are divided into two types: Predefined and User-defined. You can override either type, allowing you to tailor the behavior of the component to fit your specific needs.
1. onVoiceCallClick
onVoiceCallClick
is triggered when you click the voice call button of the Call Buttons
component. You can override this action using the following code snippet.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
import React from "react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
const handleOnVoiceCallClick = () => {
console.log("Your Custom on voice call click actions");
};
return (
<CometChatCallButtons
user={chatUser}
onVoiceCallClick={handleOnVoiceCallClick}
/>
);
};
export default CallButtonDemo;
import React, { useState, useEffect } from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = useState(null);
useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
const handleOnVoiceCallClick = () => {
console.log("Your Custom on voice call click actions");
};
return (
<CometChatCallButtons
user={chatUser}
onVoiceCallClick={handleOnVoiceCallClick}
/>
);
};
export default CallButtonDemo;
2. onVideoCallClick
onVideoCallClick
is triggered when you click the video call button of the Call Buttons
component. You can override this action using the following code snippet.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
import React from "react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
const handleOnVideoCallClick = () => {
console.log("Your Custom on video call click actions");
};
return (
<CometChatCallButtons
user={chatUser}
onVideoCallClick={handleOnVideoCallClick}
/>
);
};
export default CallButtonDemo;
import React, { useState, useEffect } from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = useState(null);
useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
const handleOnVideoCallClick = () => {
console.log("Your Custom on video call click actions");
};
return (
<CometChatCallButtons
user={chatUser}
onVideoCallClick={handleOnVideoCallClick}
/>
);
};
export default CallButtonDemo;
3. onError
This action doesn't change the behavior of the component but rather listens for any errors that occur in the Call Button component.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
import React from "react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
const handleOnError = () => {
console.log("Your Custom on error actions");
};
return <CometChatCallButtons user={chatUser} onError={handleOnError} />;
};
export default CallButtonDemo;
import React, { useState, useEffect } from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = useState(null);
useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
const handleOnError = () => {
console.log("Your Custom on error actions");
};
return <CometChatCallButtons user={chatUser} onError={handleOnError} />;
};
export default CallButtonDemo;
Filters
Filters allow you to customize the data displayed in a list within a Component
. You can filter the list based on your specific criteria, allowing for a more customized. Filters can be applied using RequestBuilders
of Chat SDK.
The Call Buttons
component does not have any exposed filters.
Events
Events are emitted by a Component
. By using event you can extend existing functionality. Being global events, they can be applied in Multiple Locations and are capable of being Added or Removed.
The list of events emitted by the Call Buttons
component is as follows.
Event | Description |
---|---|
ccCallRejected | This event is triggered when the initiated call is rejected by the receiver. |
ccCallEnded | This event is triggered when the initiated call successfully ends. |
ccOutgoingCall | This event is triggered when the user initiates a voice/video call. |
ccMessageSent | This event is triggered when the sent message is in transit and also when it is received by the receiver. |
- Add Listener
const ccCallRejected = CometChatCallEvents.ccCallRejected.subscribe(
(call: CometChat.Call) => {
//Your Code
}
);
const ccCallEnded = CometChatCallEvents.ccCallEnded.subscribe(
(call: CometChat.Call) => {
//Your Code
}
);
const ccOutgoingCall = CometChatCallEvents.ccOutgoingCall.subscribe(
(call: CometChat.Call) => {
//Your Code
}
);
const ccMessageSent = CometChatMessageEvents.ccMessageSent.subscribe(() => {
//Your Code
});
- Remove Listener
ccCallRejected?.unsubscribe();
ccCallEnded?.unsubscribe();
ccOutgoingCall?.unsubscribe();
ccMessageSent?.unsubscribe();
Customization
To fit your app's design requirements, you can customize the appearance of the Call Buttons component. We provide exposed methods that allow you to modify the experience and behavior according to your specific needs.
Style
Using Style you can customize the look and feel of the component in your app, These parameters typically control elements such as the color, size, shape, and fonts used within the component.
1. CallButtons Style
To customize the appearance, you can assign a CallButtonsStyle
object to the Call Buttons
component.
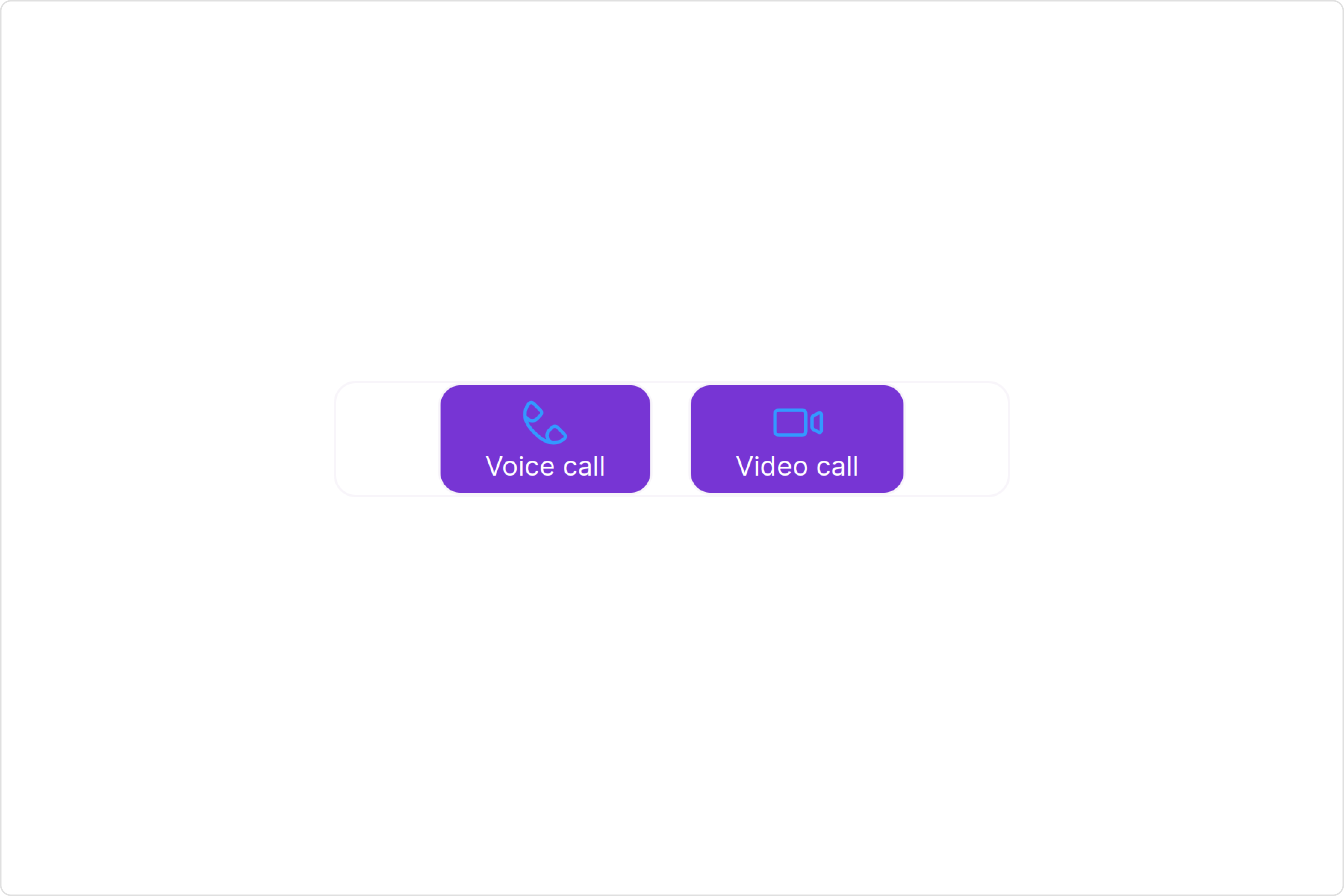
Example
In this example, we are employing the callButtonsStyle
.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatCallButtons,
CallButtonsStyle,
} from "@cometchat/chat-uikit-react";
import React from "react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
const callButtonsStyle = new CallButtonsStyle({
background: "#e5c9f5",
height: "50px",
width: "400px",
border: "1px solid #f8f5fa",
buttonBackground: "#7735d4",
buttonBorderRadius: "10px",
videoCallIconTextColor: "#ffffff",
voiceCallIconTextColor: "#ffffff",
buttonPadding: "20px",
});
return (
<CometChatCallButtons user={chatUser} callButtonsStyle={callButtonsStyle} />
);
};
export default CallButtonDemo;
import React, { useState, useEffect } from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import {
CometChatCallButtons,
CallButtonsStyle,
} from "@cometchat/chat-uikit-react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = useState(null);
useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
const callButtonsStyle = new CallButtonsStyle({
background: "#e5c9f5",
height: "50px",
width: "400px",
border: "1px solid #f8f5fa",
buttonBackground: "#7735d4",
buttonBorderRadius: "10px",
videoCallIconTextColor: "#ffffff",
voiceCallIconTextColor: "#ffffff",
buttonPadding: "20px",
});
return (
<CometChatCallButtons user={chatUser} callButtonsStyle={callButtonsStyle} />
);
};
export default CallButtonDemo;
The following properties are exposed by CallButtonsStyle
:
Property | Description | Code |
---|---|---|
border | Used to set border | border?: string, |
borderRadius | Used to set border radius | borderRadius?: string; |
background | Used to set background colour | background?: string; |
height | Used to set height | height?: string; |
width | Used to set width | width?: string; |
voiceCallIconTint | Used to set voice call icon tint | voiceCallIconTint?: string, |
videoCallIconTint | Used to set video call icon tint | videoCallIconTint?: string; |
voiceCallIconTextFont | Used to set voice call icon text font | voiceCallIconTextFont?: string; |
videoCallIconTextFont | Used to set video call icon text font | videoCallIconTextFont?: string; |
voiceCallIconTextColor | Used to set voice call icon text color | voiceCallIconTextColor?: string; |
videoCallIconTextColor | Used to set video call icon text color | videoCallIconTextColor?: string; |
buttonBackground | Used to set button background color | buttonBackground?: string; |
buttonBorder | Used to set button border | buttonBorder?: string; |
buttonBorderRadius | Used to set button border radius | buttonBorderRadius?: string; |
buttonPadding | Used to set button padding | buttonPadding?: string; |
Functionality
These are a set of small functional customizations that allow you to fine-tune the overall experience of the component. With these, you can change text, set custom icons, and toggle the visibility of UI elements.
Here is a code snippet demonstrating how you can customize the functionality of the Call Buttons
component.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
import React from "react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
return (
<CometChatCallButtons
user={chatUser}
videoCallIconHoverText="Your Custom Video Call Icon Hover Text"
videoCallIconText="Your Custom Video Call Icon Text"
/>
);
};
export default CallButtonDemo;
import React, { useState, useEffect } from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons } from "@cometchat/chat-uikit-react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = useState(null);
useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
return (
<CometChatCallButtons
user={chatUser}
videoCallIconHoverText="Your Custom Video Call Icon Hover Text"
videoCallIconText="Your Custom Video Call Icon Text"
/>
);
};
export default CallButtonDemo;
Default:
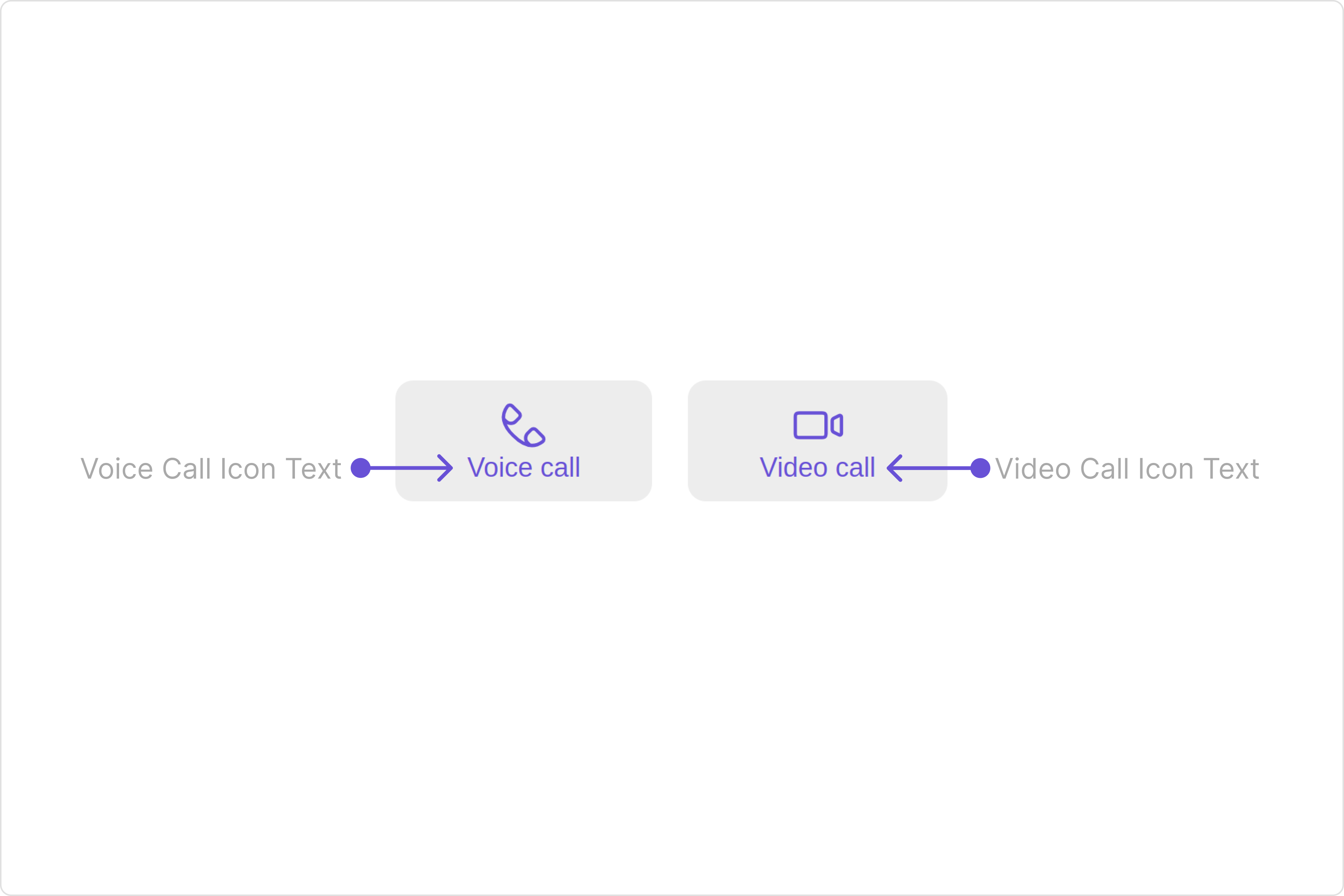
Custom:
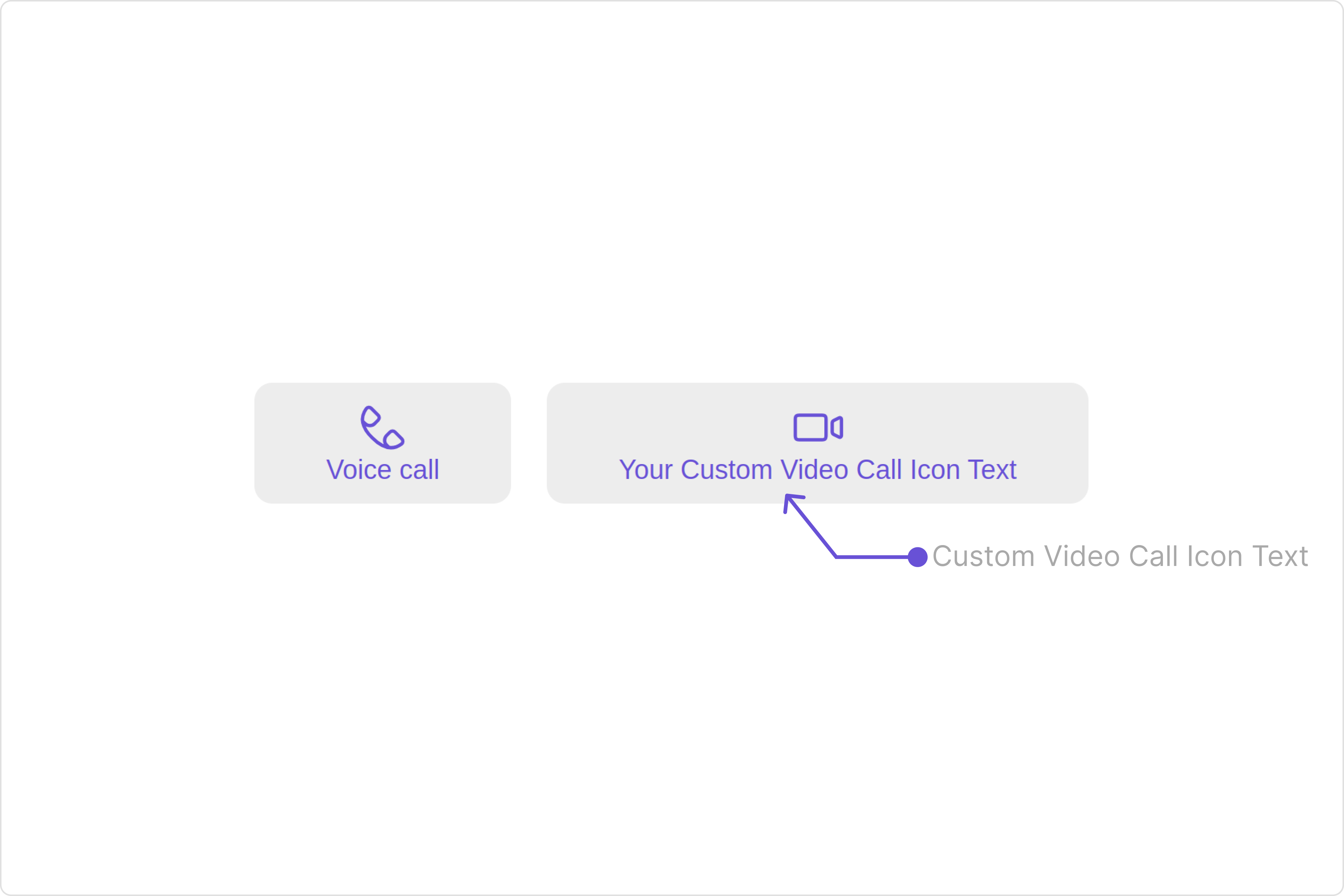
Below is a list of customizations along with corresponding code snippets
Property | Description | Code |
---|---|---|
videoCallIconHoverText | Used to set the custom text or tooltip displayed on the video call button on mouse over. | videoCallIconHoverText='Your Custom Video Call Icon Hover Text' |
voiceCallIconHoverText | Used to set the custom text or tooltip displayed on the voice call button on mouse over. | voiceCallIconHoverText='Your Custom Voice Call Icon Hover text' |
videoCallIconText | Used to set custom video call icon text | videoCallIconText='Your Custom Video Call Icon Text' |
voiceCallIconText | Used to set custom voice call icon text | voiceCallIconText='Your Custom Voice Call Icon Text' |
videoCallIconURL | Used to set custom Icon for Video Call Button | videoCallIconURL='Custom Video Call Icon URL' |
voiceCallIconURL | Used to set custom Icon for Voice Call Button | videoCallIconURL='Custom Voice Call Icon URL' |
group | Used to set the group object for Call Buttons | group={chatGroup} |
user | Sets the user object for Call Buttons | user={chatUser} |
Advanced
For advanced-level customization, you can set custom views to the component. This lets you tailor each aspect of the component to fit your exact needs and application aesthetics. You can create and define your views, layouts, and UI elements and then incorporate those into the component.
the Call Buttons
component does not offer any advanced functionalities beyond this level of customization.
Configuration
Configurations offer the ability to customize the properties of each individual component within a Composite Component.
The Call Buttons Component is a Composite Component and it has a specific set of configuration for each of its components.
OngoingCall
If you want to customize the properties of the OngoingCall Component inside Call Buttons Component, you need use the CallScreenConfiguration
object.
The OngoingCallConfiguration
provides access to all the Action, Filters, Styles, Functionality, and Advanced properties of the OngoingCall component.
Please note that the properties marked with the symbol are not accessible within the Configuration Object.
Example
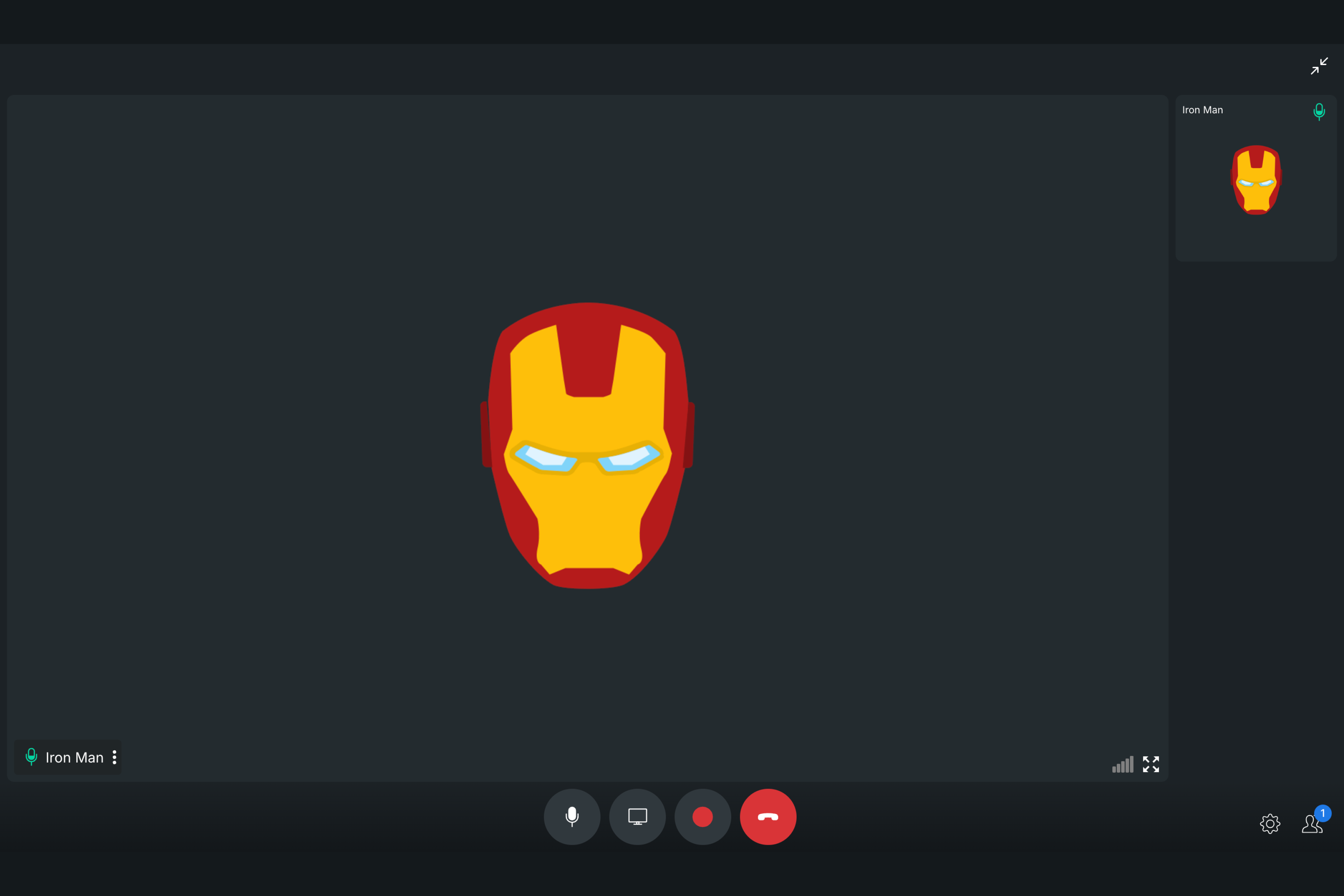
In this example, we will show how you can display the call recording button in the OngoingCall component using ongoingCallConfiguration
.
- TypeScript
- JavaScript
import React, { useState, useEffect } from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons, CallScreenConfiguration } from "@cometchat/chat-uikit-react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = useState(null);
useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
function customSettingBuilder(audioOnlyCall, user, group) {
const builder = new CometChatUIKitCalls.CallSettingsBuilder();
if (user) {
builder
.enableDefaultLayout(true)
.setIsAudioOnlyCall(true)
.showRecordingButton(true);
} else if (group) {
builder
.enableDefaultLayout(true)
.setIsAudioOnlyCall(false)
.showRecordingButton(true);
}
return builder;
}
return (
<CometChatCallButtons
user={chatUser}
ongoingCallConfiguration={new CallScreenConfiguration({
callSettingsBuilder: customSettingBuilder,
})}
/>
);
};
export default CallButtonDemo;
import React, { useState, useEffect } from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons, CallScreenConfiguration } from "@cometchat/chat-uikit-react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = useState(null);
useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
function customSettingBuilder(audioOnlyCall: boolean, user?: CometChat.User, group?: CometChat.Group) {
const builder = new CometChatUIKitCalls.CallSettingsBuilder();
if (user) {
builder
.enableDefaultLayout(true)
.setIsAudioOnlyCall(true)
.startRecordingOnCallStart(false)
.showRecordingButton(true);
} else if (group) {
builder
.enableDefaultLayout(true)
.setIsAudioOnlyCall(false)
.startRecordingOnCallStart(false)
.showRecordingButton(true);
}
return builder;
}
return (
<CometChatCallButtons
user={chatUser}
ongoingCallConfiguration={new CallScreenConfiguration({
callSettingsBuilder: customSettingBuilder,
})}
/>
);
};
export default CallButtonDemo;
OutGoingCall
If you want to customize the properties of the OutGoingCall Component inside Call Buttons Component, you need use the OutGoingCallConfiguration
object.
The OutGoingCallConfiguration
provides access to all the Action, Filters, Styles, Functionality, and Advanced properties of the OutGoingCall component.
Please note that the properties marked with the symbol are not accessible within the Configuration Object.
Example
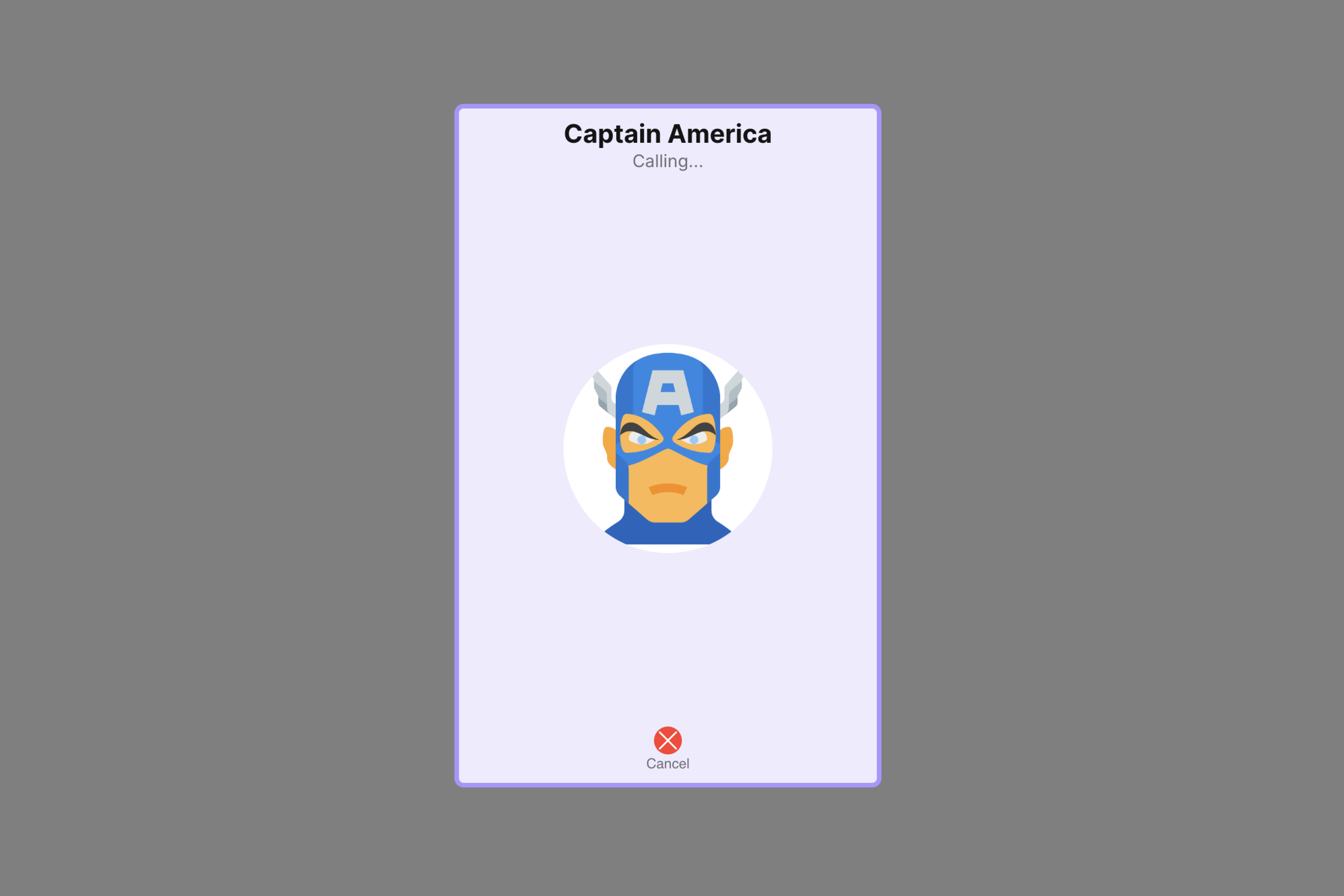
In this example, we will be changing the background color of OutGoingCall component using OutGoingCallConfiguration
.
- TypeScript
- JavaScript
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons, OutGoingCallConfiguration } from "@cometchat/chat-uikit-react";
import React from "react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = React.useState<CometChat.User>();
React.useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
return (
<CometChatCallButtons
user={chatUser}
outgoingCallConfiguration={new OutgoingCallConfiguration({
outgoingCallStyle: {
background: '#efebff',
border: '4px solid #ab94ff',
},
})}
/>
);
};
export default CallButtonDemo;
import React, { useState, useEffect } from "react";
import { CometChat } from "@cometchat/chat-sdk-javascript";
import { CometChatCallButtons, OutgoingCallConfiguration } from "@cometchat/chat-uikit-react";
const CallButtonDemo = () => {
const [chatUser, setChatUser] = useState(null);
useEffect(() => {
CometChat.getUser("uid").then((user) => {
setChatUser(user);
});
}, []);
return (
<CometChatCallButtons
user={chatUser}
outgoingCallConfiguration={new OutgoingCallConfiguration({
outgoingCallStyle: {
background: '#efebff',
border: '4px solid #ab94ff',
},
})}
/>
);
};
export default CallButtonDemo;