Colors
Overview
Colors in CometChatTheme allow you to maintain a consistent visual identity across your application by providing predefined colors for various UI elements, such as text, buttons, backgrounds, alerts, and more. These color definitions seamlessly adapt to both light and dark themes, ensuring an optimal user experience across different modes.
The color resources are divided into the following categories:
- Primary Colors: Define the main theme of the application.
- Neutral Colors: Used for backgrounds, borders, and secondary UI elements.
- Alert Colors: Highlight states like success, warning, error, or information.
- Text Colors: Used for typography.
- Icon Colors: Define icon appearances.
- Button Colors: Customize button backgrounds, icons, and text.
CometChat provides separate color definitions for light mode and dark mode, enabling automatic theme adaptation.
Usage
Default Colors
CometChat provides predefined color sets for both light and dark modes, ensuring optimal visual contrast and accessibility across your application.
These colors can be accessed through the theme value
, which is obtained using the useTheme()
hook.
Example: Colors
- Light Mode Colors
- Dark Mode Colors
import { ColorValue } from "react-native";
const primaryColor = "#6852D6";
const extendedPrimaryColors = {
extendedPrimary50: "<generated based on primaryColor>",
extendedPrimary100: "<generated based on primaryColor>",
//generated based on
};
const neutralColors = {
neutral50: "#FFFFFF",
neutral100: "#FAFAFA",
//other colors
};
const alertColors = {
info: "#0B7BEA",
warning: "#FFAB00",
//other colors
};
const staticColors = {
staticBlack: "#141414",
staticWhite: "#FFFFFF",
};
export const defaultColorLight = {
primary: primaryColor,
...extendedPrimaryColors,
...neutralColors,
...alertColors,
...staticColors,
background1: neutralColors.neutral50,
background2: neutralColors.neutral100,
background3: neutralColors.neutral200,
background4: neutralColors.neutral300,
//other derived colors
};
type EachColorValue<T extends typeof defaultColorLight> = {
[P in keyof T]: ColorValue;
};
//color type
export type Color = EachColorValue<typeof defaultColorLight>;
import { Color } from "./light"; //type imported from light
const primaryColor = "#6852D6";
const extendedPrimaryColors = {
extendedPrimary50: "<generated based on primaryColor>",
extendedPrimary100: "<generated based on primaryColor>",
//other derived colors
};
const neutralColors = {
neutral50: "#141414",
neutral100: "#1A1A1A",
//other derived colors
};
const alertColors = {
info: "#0D66BF",
warning: "#D08D04",
success: "#0B9F5D",
error: "#C73C3E",
};
const staticColors = {
staticBlack: "#141414",
staticWhite: "#FFFFFF",
};
export const defaultColorDark: Color = {
primary: primaryColor,
...extendedPrimaryColors,
...neutralColors,
...alertColors,
...staticColors,
background1: neutralColors.neutral50,
background2: neutralColors.neutral100,
background3: neutralColors.neutral200,
background4: neutralColors.neutral300,
//other derived colors
};
Extended colors 50-100
are generated based on the following blend percentages.
export enum Brightness {
LIGHT = 0,
DARK = 1,
}
getBlendColorsPercentage(brightness: Brightness): {
[key: number]: number;
} {
return brightness === Brightness.LIGHT
? {
50: 0.96,
100: 0.88,
200: 0.77,
300: 0.66,
400: 0.55,
500: 0.44,
600: 0.33,
700: 0.22,
800: 0.11,
900: 0.11,
}
: {
50: 0.8,
100: 0.72,
200: 0.64,
300: 0.56,
400: 0.48,
500: 0.4,
600: 0.32,
700: 0.24,
800: 0.16,
900: 0.08,
};
}
//baseColor is the primary color
blendColors(baseColor: string, blendColor: string, percentage: number): string {
const { r: rBase, g: gBase, b: bBase } = this.hexToRgb(baseColor);
const { r: rBlend, g: gBlend, b: bBlend } = this.hexToRgb(blendColor);
const r = Math.round(rBase * (1 - percentage) + rBlend * percentage);
const g = Math.round(gBase * (1 - percentage) + gBlend * percentage);
const b = Math.round(bBase * (1 - percentage) + bBlend * percentage);
return this.rgbToHex(r, g, b);
}
For more details on how other colors are generated Click Here.
Extended primary colors, as well as background, text, button, and other UI colors, are automatically derived from the provided primary and neutral colors. However, any colors explicitly overridden in the theme via CometChatThemeProvider will take precedence over the generated values.
Customizing Colors
You can override the default colors to align them with your application's branding.
Colors must be supplied as hex values.
Example: Changing the primary and textPrimary colors.
- Typescript
return (
<SafeAreaView style={{ flex: 1 }}>
<CometChatThemeProvider
theme={{
light: {
color: {
// The extended primary colors that are not explicitly defined will be auto-generated
primary: "#b2b200",
// Since textPrimary is explicitly defined, it won't be auto-generated
textPrimary: "#7f7f00",
},
},
}}
>
{/* Component */}
</CometChatThemeProvider>
</SafeAreaView>
);
To know more about theme structure, visit the theme interface.
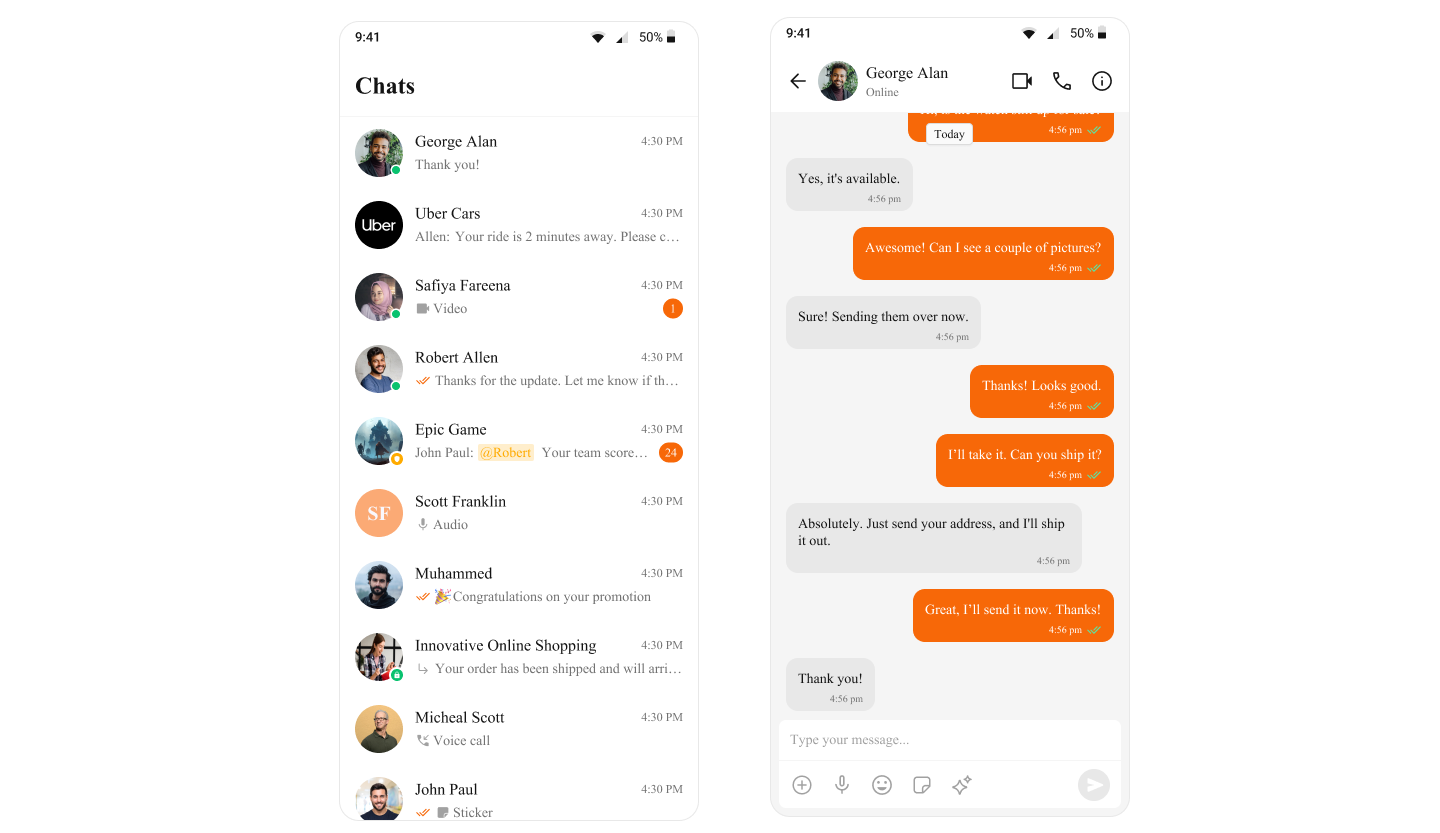