iOS - FCM
Learn how to send out Push Notifications to your iOS using Firebase Cloud Messaging or FCM.
You can refer to our setup using the Apple Push Notifications service (APNs).
Firebase Project Setup
Visit Firebase Console and login/signup using your Gmail ID.
Step 1: Create a new Firebase Project
On your Firebase Console, create a new project.
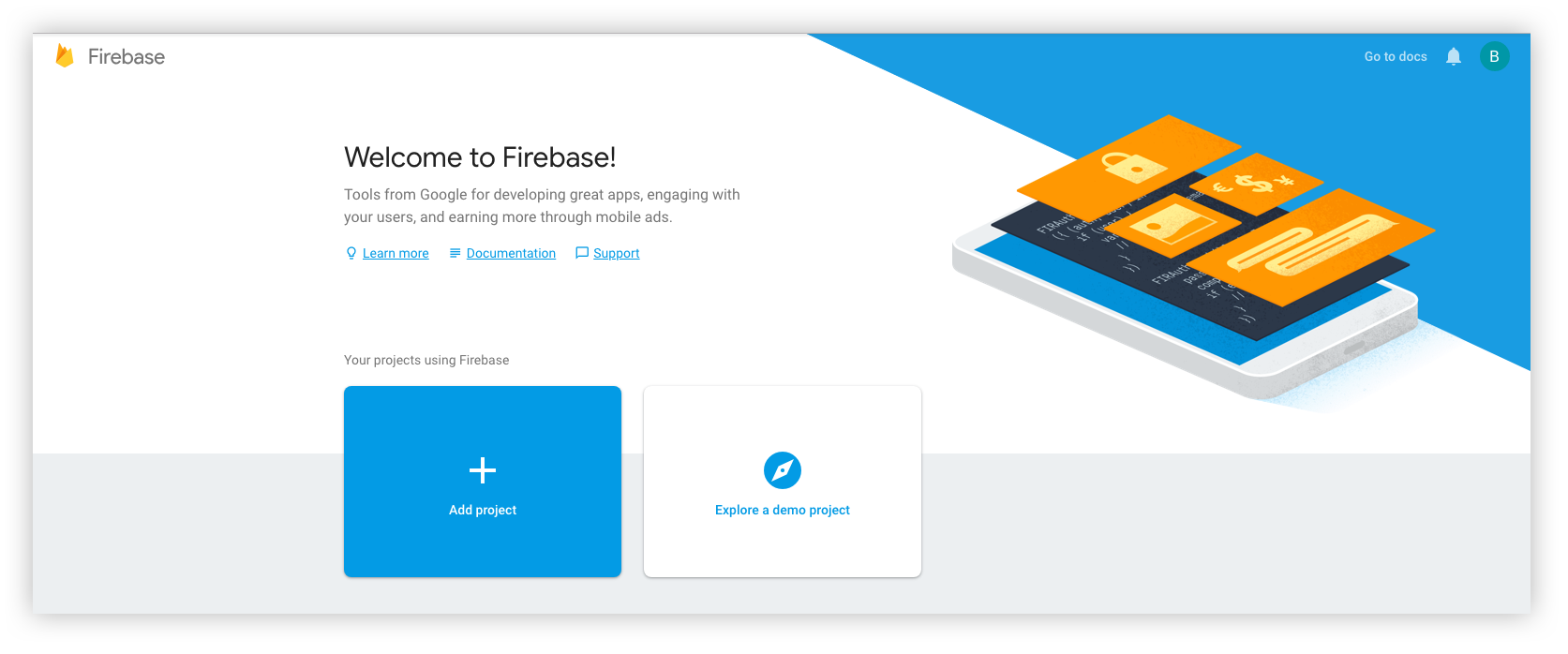
This is a simple 3 step process where:
- You give a name to your project
- Add Google Analytics to your project (Optional)
- Configure Google Analytics account (Optional)
Click on Create and you are ready to go.
Step 2: Add Firebase to your iOS App
- Click on the iOS icon as shown on the screen below.
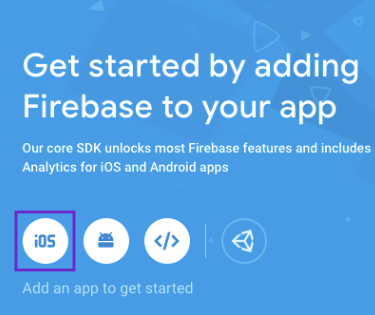
- Register your Android app by providing the following details: a. iOS bundle name b. App nickname (optional) c. App Store ID (optional)
- Download the GoogleService-Info.plist file and place it in the mentioned location of your project. Move your config file into the root of your Xcode project. If prompted, select to add the config file to all targets as follows.
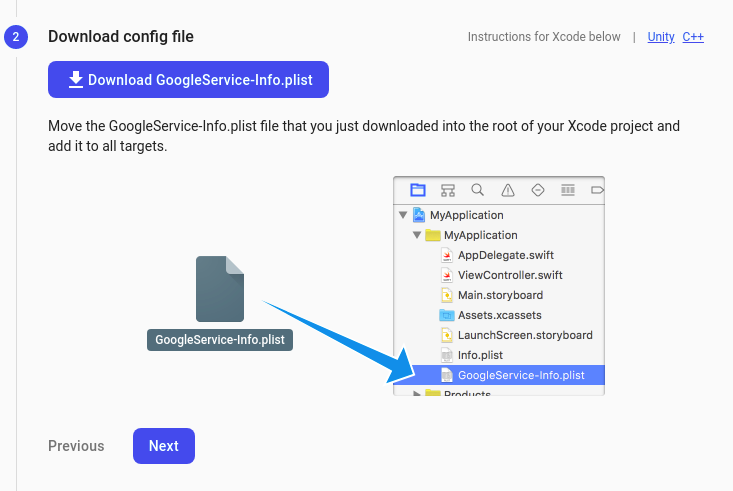
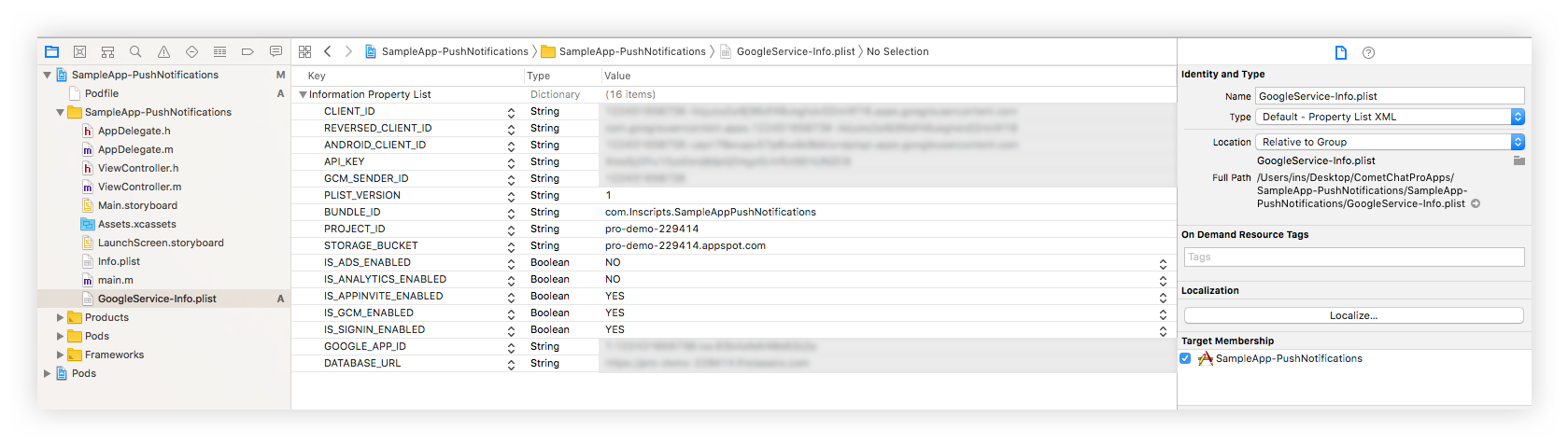
- We will Add Firebase SDK and Initialisation code later. So, click on 'Next', 'Next', and 'Continue to the Console'.
Step 3: Download the service account file
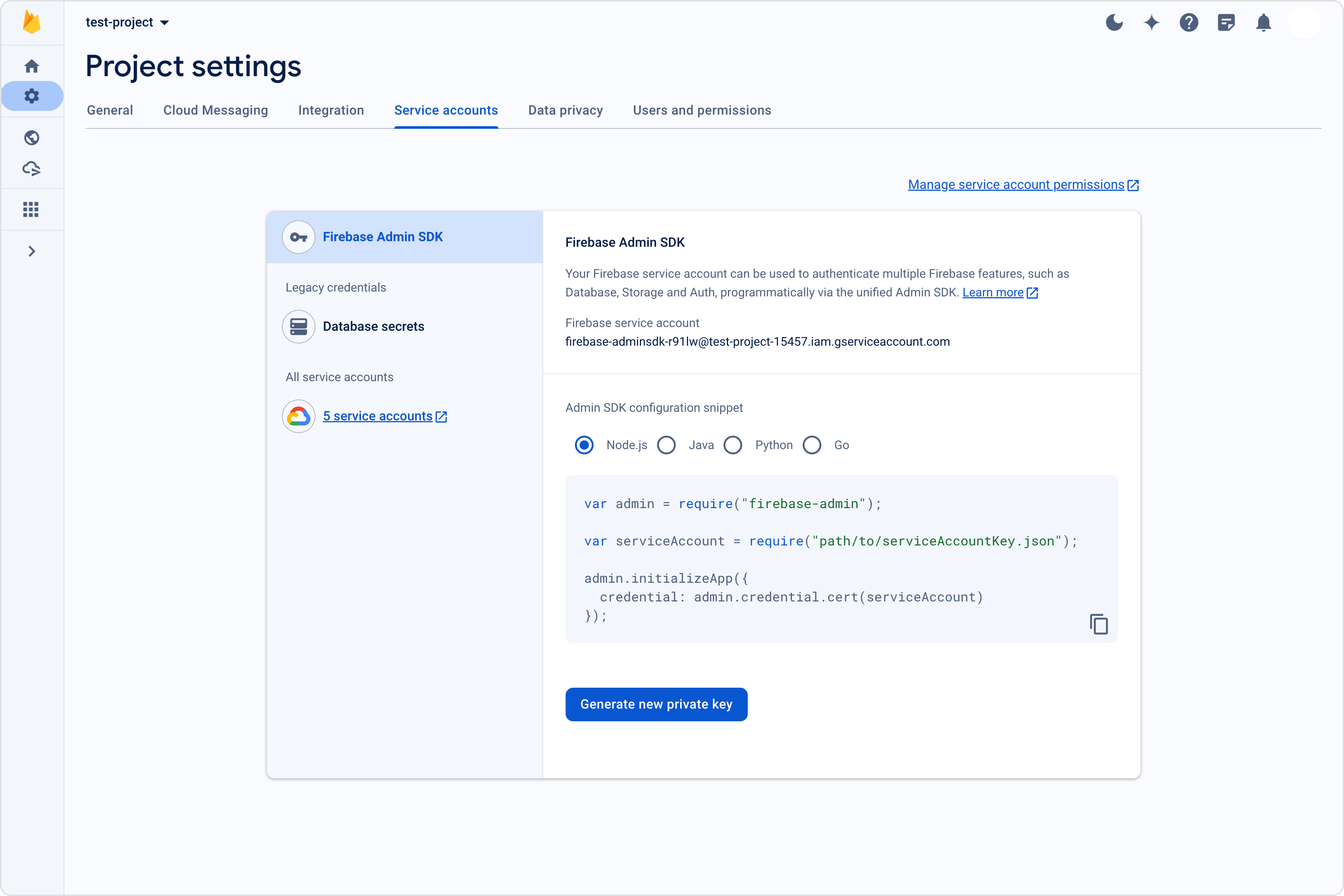
Extension settings
Step 1: Enable the extension
- Login to CometChat and select your app.
- Go to the Extensions section and Enable the Push Notifications extension.
- Open the settings for this extension and save the following settings.
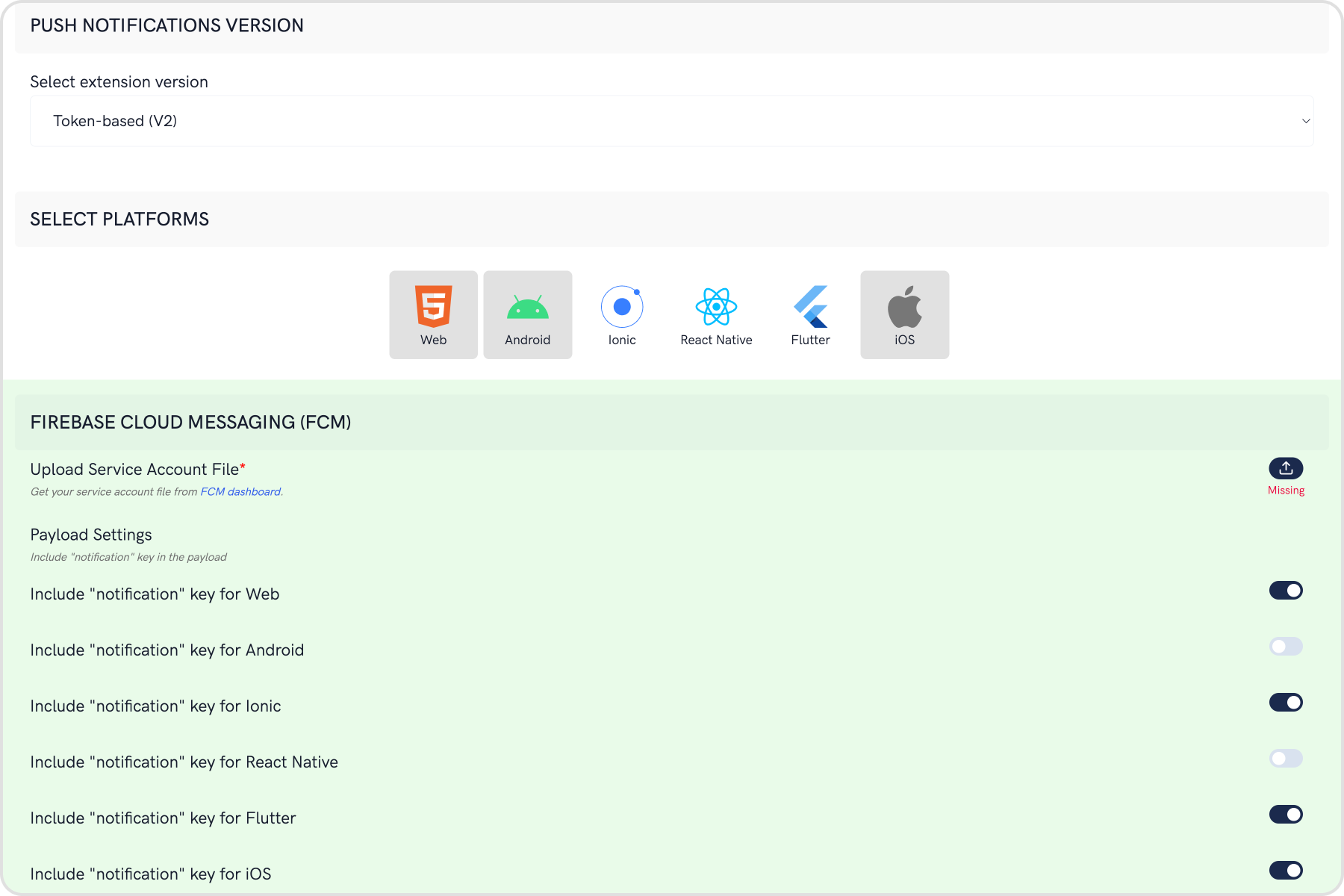
Step 2: Save your settings
On the Settings page you need to enter the following:
- Set extension version
- If you are setting it for the first time, Select
V2
to start using the enhanced version of the Push Notification extension. The enhanced version uses Token-based approach for sending Push Notifications and is simple to implement. - If you already have an app using
V1
and want to migrate your app to useV2
, then SelectV1 & V2
option. This ensures that the users viewing the older version of your app also receive Push Notifications. - Eventually, when all your users are on the latest version of your app, you can change this option to
V2
, thus turning offV1
(Topic-based) Push Notifications completely.
- Select the platforms that you want to support
- Select from Web, Android, Ionic, React Native, Flutter & iOS.
- Notification payload settings
- You can control if the notification key should be in the Payload or not. Learn more about the FCM Messages here.
- Notification Triggers
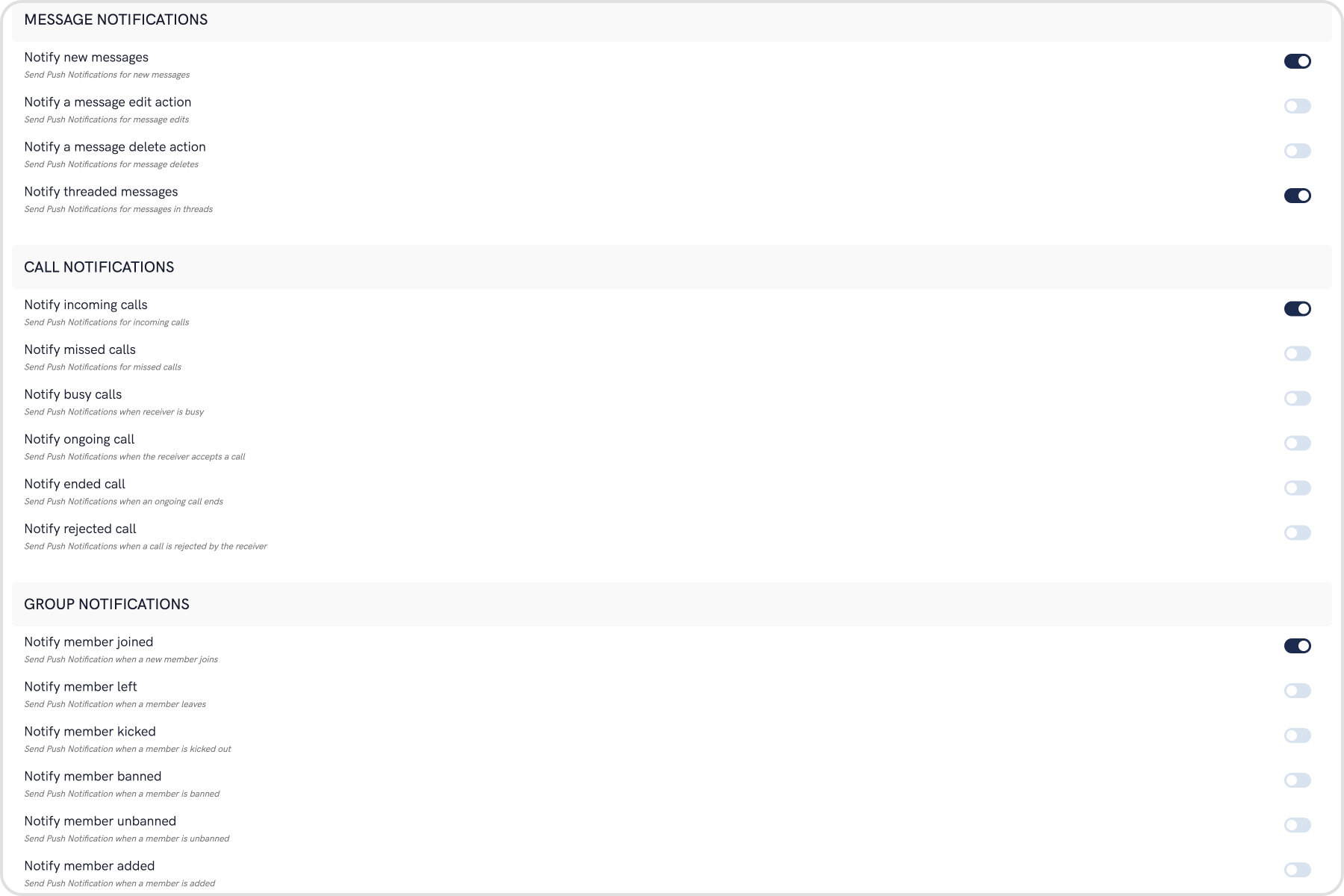
- Select the triggers for sending Push Notifications. These triggers can be classified into 3 main categories:
- Message Notifications
- Call Notifications
- Group Notifications
- These are pretty self-explanatory and you can toggle them as per your requirement.
Get APNS Credentials
The following steps in this section are written on the assumption that you already have an app ID assigned to your client app.
Step 1: Create a Certificate Signing Request
To obtain a signing certificate required to sign apps for installation on iOS devices, you should first create a certificate signing request (CSR) file through Keychain Access on your Mac.
- Open the Keychain Access from the utility folder, go to Keychain Access > Certificate Assistant > Request a Certificate From a Certificate Authority, and then click.
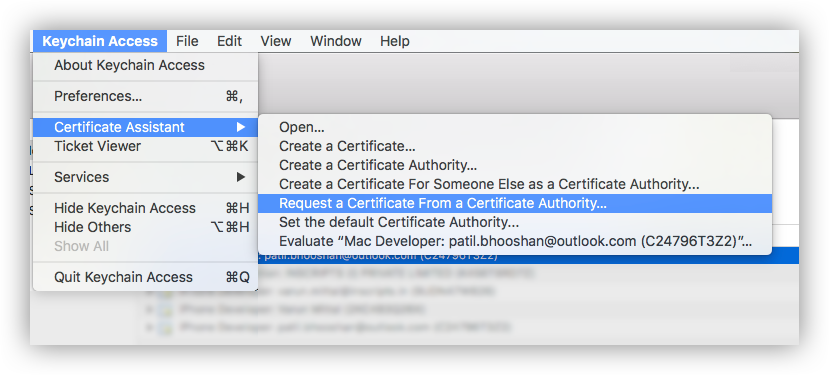
- The Certificate Information dialog box appears. Enter the email address that you use in your Apple Developer account, and enter a common name for your private key. Don't enter CA email address, choose Saved to disk, and then click the Continue button.
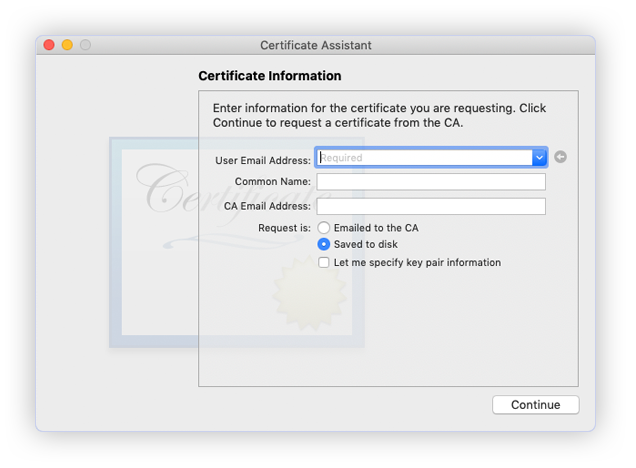
- Specify the name of your CSR to save and choose the location to save the file on your local disk. Then your CSR file is created, which contains a public/private key pair.
Step 2: Create an SSL certificate
- Sign in to your account at the Apple Developer Member Center.
- Go to Certificates, Identifiers & Profiles. In the Identifiers > App IDs and select the Push Notifications service under Application Services
- Click the Edit button.
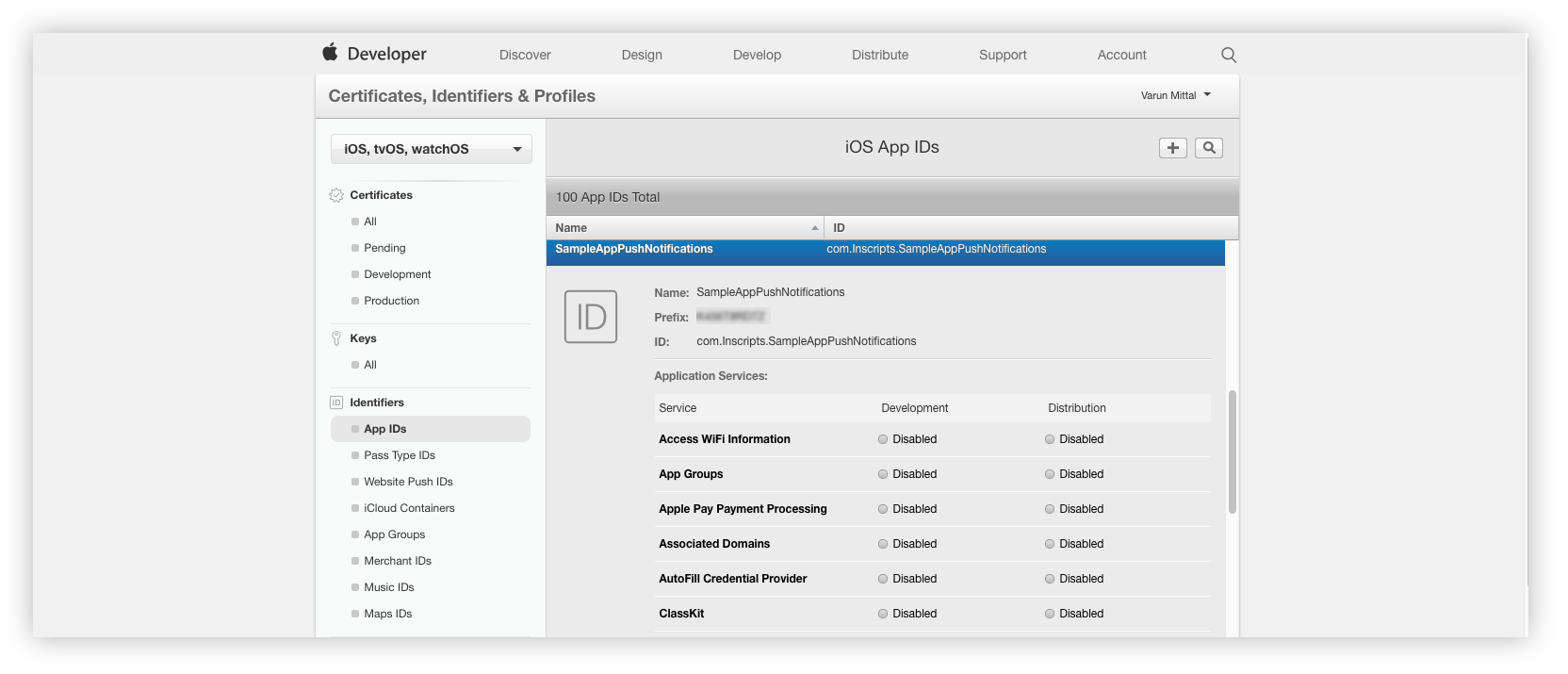
- Under the Push Notifications service, choose which SSL certificate to create either Development or Production.
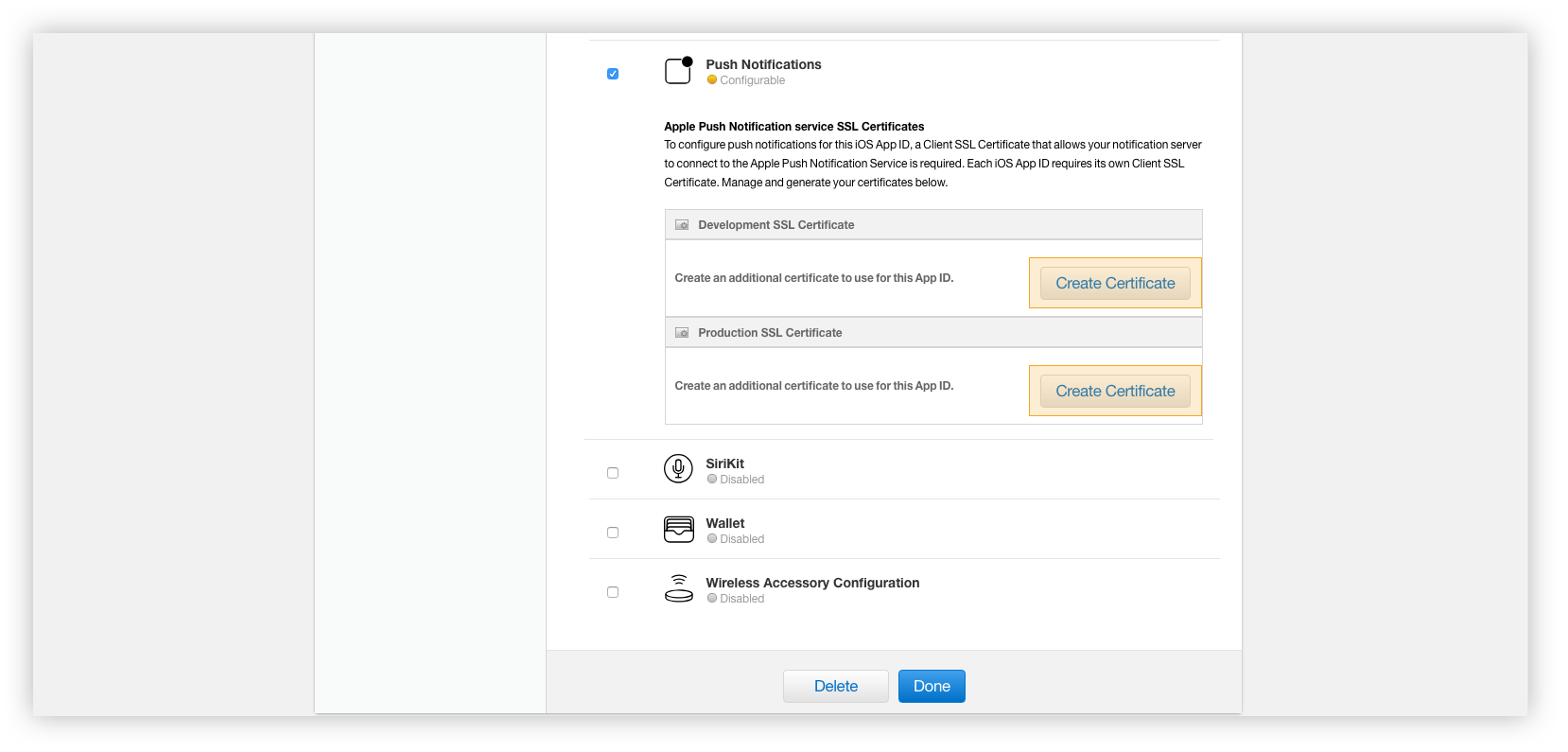
- In the Generate your certificate pane that appears after the selection, under Upload CSR file., upload the CSR file you created through the Choose File... button. To complete the process, choose Continue. When the certificate is ready, choose Download to save it to your Mac.
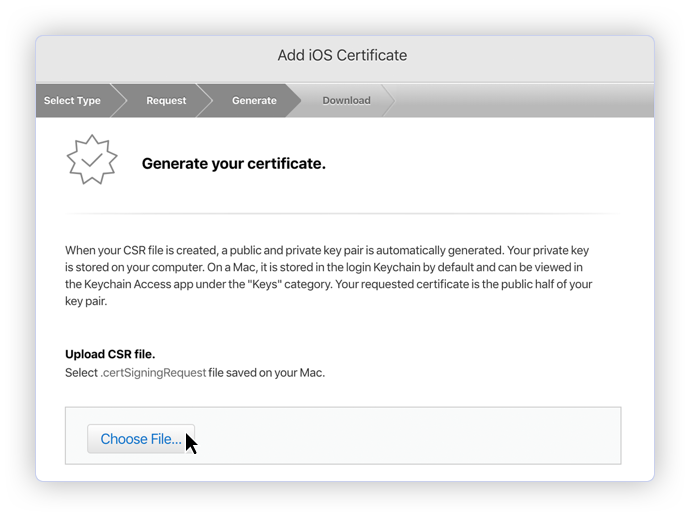
- In order to install the downloaded certificate to the KeyChain Access on your Mac, double-click it. You can find the certificate in the KeyChain Access > login > Certificates.
Step 3: Export and update .p12 file to Firebase
- Type a name for the .p12 file and save it to your Mac.
- Browse to the location where you saved your key, select it, and click Open. Add the key ID for the key (available in Certificates, Identifiers & Profiles in the Apple Developer Member Center) and export it.
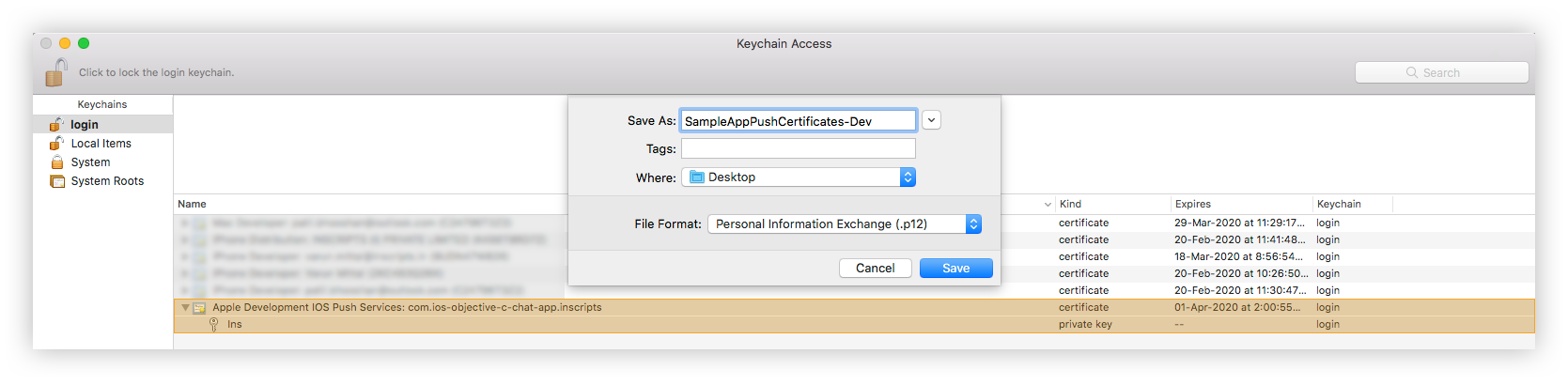
Step 4: Upload your APNs Certificates
- Go to Firebase console and open your project.
- Inside your iOS project in the Firebase console, select settings and then select the
Cloud Messaging
tab. - Scroll down to iOS app configuration, click the Upload button for APNS certificate.
- Browse to the location where you saved your APNs Certificates, select it, and click Open.
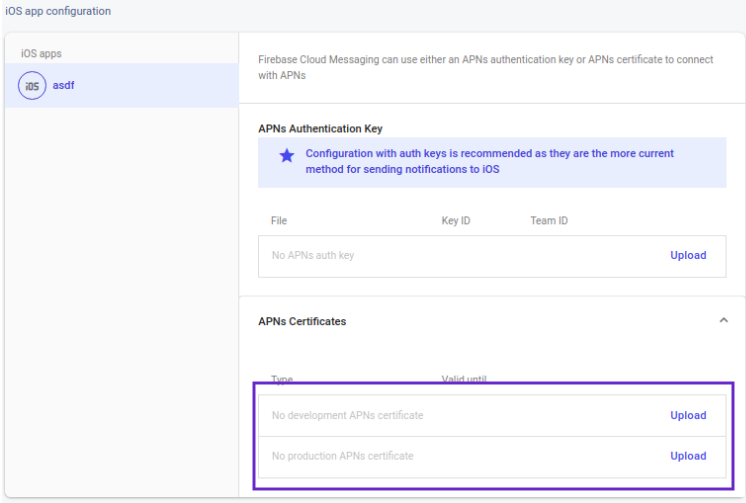
iOS App Setup
Step 1: Initial Firebase Cloud Messaging client setup
- Add the Firebase SDK, Add the firebase pods that you want to install. You can include a Pod in your Podfile like this:
- Swift
pod 'Firebase_Core'
pod 'Firebase_Messaging'
- Import the Firebase module in your
ApplicationDelegate:
- Swift
- Objective C
import Firebase
@import Firebase;
- Configure a FirebaseApp shared instance, typically in your application's
application:didFinishLaunchingWithOptions: method:
- Swift
- Objective C
FirebaseApp.configure()
[FIRApp configure];
Step 2: Register the FCM Token
- Get the FCM Token for remote notifications, typically in your application's
application:didFinishLaunchingWithOptions: method:
- Swift
- Objective C
Messaging.messaging().delegate = self
if #available(iOS 10.0, *) {
UNUserNotificationCenter.current().delegate = self
let authOptions: UNAuthorizationOptions = [.alert, .badge, .sound]
UNUserNotificationCenter.current().requestAuthorization(
options: authOptions,
completionHandler: {
_,
_ in
})
} else {
let settings: UIUserNotificationSettings =
UIUserNotificationSettings(types: [.alert, .badge, .sound], categories: nil)
application.registerUserNotificationSettings(settings)
}
application.registerForRemoteNotifications()
[FIRMessaging messaging].delegate = self;
if ([UNUserNotificationCenter class] != nil) {
[UNUserNotificationCenter currentNotificationCenter].delegate = self;
UNAuthorizationOptions authOptions = UNAuthorizationOptionAlert | UNAuthorizationOptionSound | UNAuthorizationOptionBadge;
[[UNUserNotificationCenter currentNotificationCenter] requestAuthorizationWithOptions: authOptions completionHandler: ^ (BOOL granted, NSError * _Nullable error) {
// ...
}
];
} else {
UIUserNotificationType allNotificationTypes = (UIUserNotificationTypeSound | UIUserNotificationTypeAlert | UIUserNotificationTypeBadge);
UIUserNotificationSettings * settings = [UIUserNotificationSettings settingsForTypes: allNotificationTypes categories: nil];
[application registerUserNotificationSettings: settings];
}
[application registerForRemoteNotifications];
- Swift
- Objective C
func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) {
print("Unable to register for remote notifications: \\(error.localizedDescription)")
}
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
print("APNs token retrieved: \\(deviceToken)")
Messaging.messaging().apnsToken = deviceToken
}
-(void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error
{
NSLog(@"Unable to register for remote notifications: %@", error);
}
-(void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken
{
NSLog(@"APNs device token retrieved: %@", deviceToken);
[FIRMessaging messaging].APNSToken = deviceToken;
}
- Register the FCM token with our Push Notifications extension on success of CometChat.login
- Swift
let authKey = "XXXX XXXX XXXXX"
CometChat.login(UID: UID, authKey: authKey, onSuccess: { (user) in
DispatchQueue.main.async {
if let token = UserDefaults.standard.value(forKey: "fcmToken") as? String {
CometChat.registerTokenForPushNotification(token: token, onSuccess: { (success) in
print("onSuccess to registerTokenForPushNotification: \\(success)")
}) { (error) in
print("error to registerTokenForPushNotification")
}
}
- This also needs to be done when you refresh your FCM Token
- Swift
extension AppDelegate : MessagingDelegate {
// [START refresh_token]
func messaging(_ messaging: Messaging, didReceiveRegistrationToken fcmToken: String) {
print("Firebase registration token: \\(fcmToken)")
UserDefaults.standard.set(fcmToken, forKey: "fcmToken")
CometChat.registerTokenForPushNotification(token: fcmToken, onSuccess: { (sucess) in
print("token registered \\(sucess)")
}) { (error) in
print("token registered error \\(String(describing: error?.errorDescription))")
}
let dataDict:[String: String] = ["token": fcmToken]
NotificationCenter.default.post(name: Notification.Name("FCMToken"), object: nil, userInfo: dataDict)
}
}
Step 3: Start receiving Push Notifications
- Receive remote notification, typically in your application's
App Delegate:
- Swift
- Objective C
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable: Any]) {
// Print full message.
print(userInfo)
}
func application(_ application: UIApplication, didReceiveRemoteNotification userInfo: [AnyHashable: Any], fetchCompletionHandler completionHandler: @escaping(UIBackgroundFetchResult) -> Void) {
// Print full message.
print(userInfo)
completionHandler(UIBackgroundFetchResult.newData)
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo {
// Print full message.
NSLog(@"%@", userInfo);
}
- (void)application:(UIApplication *)application didReceiveRemoteNotification:(NSDictionary *)userInfo
fetchCompletionHandler:(void (^)(UIBackgroundFetchResult))completionHandler {
// Print full message.
NSLog(@"%@", userInfo);
completionHandler(UIBackgroundFetchResultNewData);
}
- Receive Notification for
CustomMessage
:
To receive and display notifications for CustomMessage
, the developer needs to set metadata
while sending the CustomMessage
value as follows:
- Swift
- Objective C
var receiverID = "cometchat-uid-1";
var message = [
"someRandomKey": "someRandomData"
];
var customMessage = CustomMessage(receiverUid: receiverID, receiverType: ReceiverTypeUser, customData: message);
// to display custom notification banner add this , "pushNotification" key is not to modify, although you can modify banner text as shown beow //
var customNotificationDisplayText = [
"pushNotification": "notification_banner_text_here";
];
// set it as metadata of `Custom message`
customMessage.metaData = customNotificationDisplayText;
CometChat.sendCustomMessage(withMessage: customMessage, onSuccess: { sentMessage in
print("sentMessage \\(sentMessage.stringValue)");
}, onError: { error in
if let error = error?.errorDescription() {
print("error sending custom message \\(error)");
}
});
NSString * receiverID = @ "cometchat-uid-1";
NSDictionary * message = [NSDictionary dictionaryWithObjectsAndKeys: @ "someRandomData", @ "someRandomKey", nil];
CustomMessage * customMessage = [
[CustomMessage alloc] initWithReceiverUid: receiverID receiverType: ReceiverTypeUser customData: message
];
// to display custom notification banner add this //
NSDictionary * customNotificationDisplayText = [NSDictionary dictionaryWithObjectsAndKeys: @ "notification_banner_text_here", @ "pushNotification", nil];
[customMessage setMetaData: customNotificationDisplayText];
[CometChat sendCustomMessageWithMessage: customMessage onSuccess: ^ (CustomMessage * _Nonnull sentMessage) {
NSLog(@ "sentMessage %@", [sentMessage stringValue]);
}
onError: ^ (CometChatException * _Nullable error) {
NSLog(@ "error sending custom message %@", [error errorDescription]);
}
];
Push Notification Payload sample for text and media messages-
- Text message
- Media message
{
"alert": "Nancy Grace: Text Message",
"sound": "default",
"title": "CometChat",
"message": {
"receiver": "cometchat-uid-4",
"data": {
"entities": {
"receiver": {
"entityType": "user",
"entity": {
"uid": "cometchat-uid-4",
"role": "default",
"name": "Susan Marie",
"avatar": "http://data.cometchat.com/assets/images/avatars/cometchat-uid-4.webp",
"status": "offline"
}
},
"sender": {
"entityType": "user",
"entity": {
"uid": "cometchat-uid-3",
"role": "default",
"name": "Nancy Grace",
"avatar": "https://data.cometchat.com/assets/images/avatars/cometchat-uid-3.webp",
"status": "offline"
}
}
},
"text": "Text Message"
},
"sender": "cometchat-uid-3",
"receiverType": "user",
"id": "142",
"sentAt": 1555668711,
"category": "message",
"type": "text"
}
}
{
"alert": "Nancy Grace: has sent an image",
"sound": "default",
"title": "CometChat",
"message": {
"receiver": "cometchat-uid-4",
"data": {
"attachments": [
{
"extension": "png",
"size": 14327,
"name": "extension_leftpanel.png",
"mimeType": "image/png",
"url": "https://s3-eu-west-1.amazonaws.com/data.cometchat.com/1255466c41bd7f/media/1555671238_956450103_extension_leftpanel.png"
}
],
"entities": {
"receiver": {
"entityType": "user",
"entity": {
"uid": "cometchat-uid-4",
"role": "default",
"name": "Susan Marie",
"avatar": "https://data.cometchat.com/assets/images/avatars/cometchat-uid-4.webp",
"status": "offline"
}
},
"sender": {
"entityType": "user",
"entity": {
"uid": "cometchat-uid-3",
"role": "default",
"name": "Nancy Grace",
"avatar": "https://data.cometchat.com/assets/images/avatars/cometchat-uid-3.webp",
"status": "offline"
}
}
},
"url": "https://s3-eu-west-1.amazonaws.com/data.cometchat.com/1255466c41bd7f/media/1555671238_956450103_extension_leftpanel.png"
},
"sender": "cometchat-uid-3",
"receiverType": "user",
"id": "145",
"sentAt": 1555671238,
"category": "message",
"type": "image"
}
}
Advanced
Convert Push Notification payload to Message object
CometChat pro SDK provides a method CometChat.CometChatHelper.processMessage()
which will take the JSON received in The push notification as input, and return the corresponding TextMessage
, MediaMessage
,CustomMessage
or Call
object in return. Once the message object is received, you can use the entity as per your requirements.
This code needs to be added to the willPresent notification
method of the UNUserNotificationCenterDelegate
delegate.
- Swift
func userNotificationCenter(_ center: UNUserNotificationCenter,
willPresent notification: UNNotification,
withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
if let userInfo = notification.request.content.userInfo as? [String : Any], let messageObject = userInfo["message"], let str = messageObject as? String, let dict = str.stringTodictionary() {
if let baseMessage = CometChat.processMessage(dict).0 {
switch baseMessage.messageCategory {
case .message:
if let message = baseMessage as? BaseMessage {
switch message.messageType {
case .text:
print("text Messagge is: \\((message as?TextMessage)?.stringValue())")
case .image:
print("image Messagge is: \\((message as? MediaMessage)?.stringValue())")
case .video:
print("video Messagge is: \\((message as? MediaMessage)?.stringValue())")
case .audio:
print("audio Messagge is: \\((message as? MediaMessage)?.stringValue())")
case .file:
print("file Messagge is: \\((message as? MediaMessage)?.stringValue())")
case .custom:
print("custom Messagge is: \\((message as? MediaMessage)?.stringValue())")
case .groupMember: break
@unknown default:break}
}
case .action: break
case .call:
if let call = baseMessage as? Call {
print("call is: \\(call.stringValue())")
}
case .custom:
if let customMessage = baseMessage as? CustomMessage {
print("customMessage is: \\(customMessage.stringValue())")
}
@unknown default: break
}
}
}
completionHandler([.alert, .badge, .sound])
}
extension String {
func stringTodictionary() -> [String:Any]? {
var dictonary:[String:Any]?
if let data = self.data(using: .utf8) {
do {
dictonary = try JSONSerialization.jsonObject(with: data, options: []) as? [String : Any]
if let myDictionary = dictonary
{
return myDictionary;
}
} catch let error as NSError {
print(error)
}
}
return dictonary;
}
}